iOS - Share video on Facebook with share dialog
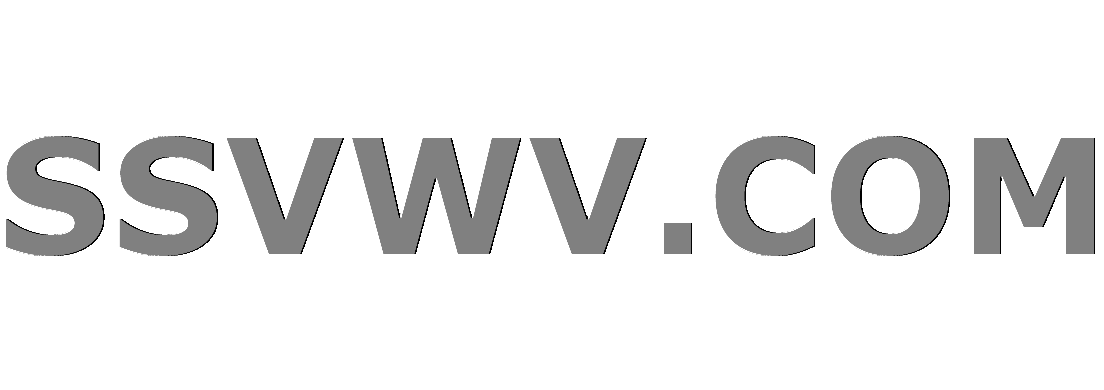
Multi tool use
My app had an option to share an online video to Facebook using graph API and was working until August 2018. I just discovered that publish_action got deprecated and that we cannot use graph API in order to share videos on Facebook.
So I tried another solution: to download the video locally, copy it to assets and then use the old FBSDKShareDialog to share it(setting the url on iOS bellow 11 and setting the asset on iOS >= 11), but the post on Facebook appeared without the video, only the text.
Then I tried it using the new ShareDialog class and because it works only with the video URL, after I copied it to assets I could not get its asset URL, but its file URL so I got the following error in the logs:
"Error while sharing video content Error Domain=com.facebook.sdk.share Code=2 "(null)" UserInfo={com.facebook.sdk:FBSDKErrorArgumentValueKey=file:///var/mobile/Media/DCIM/105APPLE/IMG_5953.MP4, com.facebook.sdk:FBSDKErrorDeveloperMessageKey=Must refer to an asset file., com.facebook.sdk:FBSDKErrorArgumentNameKey=videoURL}".
Here is my code. Please tell me what should I change in order to share a video on Facebook. If I share the URL then it shows only the URL in the post and I cannot show the video bellow.
Thanks in advance!
private func downloadVideoForUploadOnFacebook(urlString: String, videoId: Int, isMyVideo: Bool, successHandler: @escaping () -> Void, failureHandler: @escaping (String) -> Void) {
guard let url = URL(string: urlString) else {delegate.stopActivityIndicator(); return}
let request = Alamofire.request(URLRequest(url: url))
request.responseData {[weak self] (response) in
if let data = response.result.value {
let documentsURL = FileManager.default.urls(for: .documentDirectory, in: .userDomainMask).first!
let videoURL = documentsURL.appendingPathComponent("video.mp4")
do {
try data.write(to: videoURL)
self?.showShareDialog(localVideoUrl: videoURL, completion: {
print("success")
successHandler()
}, failure: { (error) in
print(error)
failureHandler(error)
})
} catch {
print("Something went wrong!")
}
}
}.downloadProgress { (progress) in
print(progress)
}
}
private func showShareDialog(localVideoUrl: URL, completion: @escaping () -> Void, failure: @escaping (String) -> Void) {
guard let navigationController = UIApplication.shared.keyWindow?.rootViewController as? UINavigationController, let vc = navigationController.viewControllers.last else {return}
if PHPhotoLibrary.authorizationStatus() == .authorized {
showFacebookShareDialog(localVideoUrl: localVideoUrl, vc: vc, completion: completion, failure: failure)
} else {
PHPhotoLibrary.requestAuthorization({[weak self] (status) in
switch status {
case .authorized:
self?.showFacebookShareDialog(localVideoUrl: localVideoUrl, vc: vc, completion: completion, failure: failure)
default:
print(status)
failure("Authorization error")
}
})
}
}
private func showFacebookShareDialog(localVideoUrl: URL, vc: UIViewController, completion: @escaping () -> Void, failure: @escaping (String) -> Void) {
var placeHolder : PHObjectPlaceholder?
do {
try PHPhotoLibrary.shared().performChangesAndWait {
let creationRequest = PHAssetChangeRequest.creationRequestForAssetFromVideo(atFileURL: localVideoUrl )
placeHolder = creationRequest?.placeholderForCreatedAsset
}
}
catch {
print(error)
failure(error.localizedDescription)
}
let result = PHAsset.fetchAssets(withLocalIdentifiers: [placeHolder!.localIdentifier], options: nil)
if let phAsset = result.firstObject {
getURL(ofPhotoWith: phAsset, completionHandler: {[weak self] (url) in
guard let url = url else {return}
self?.showNewDialog(localVideoUrl: url, vc: vc, completion: completion, failure: failure)
// if #available(iOS 11.0, *) {
// self?.showOldDialog(asset: phAsset, vc: vc)
// } else {
// self?.showOldDialog(localVideoUrl: url, vc: vc)
// }
})
} else {
failure("Error at assets")
}
}
func showOldDialog(localVideoUrl: URL, vc: UIViewController) {
let video = FBSDKShareVideo()
video.videoURL = localVideoUrl
let content = FBSDKShareVideoContent()
content.video = video
let shareDialog: FBSDKShareDialog = FBSDKShareDialog()
shareDialog.shareContent = content
shareDialog.fromViewController = vc
DispatchQueue.main.async {
shareDialog.show()
}
}
func showOldDialog(asset: PHAsset, vc: UIViewController) {
let video = FBSDKShareVideo()
video.videoAsset = asset
let content = FBSDKShareVideoContent()
content.video = video
let shareDialog: FBSDKShareDialog = FBSDKShareDialog()
shareDialog.shareContent = content
shareDialog.fromViewController = vc
DispatchQueue.main.async {
shareDialog.show()
}
}
func showNewDialog(localVideoUrl: URL, vc: UIViewController, completion: @escaping () -> Void, failure: @escaping (String) -> Void) {
let video = FacebookShare.Video(url: localVideoUrl)
let content = VideoShareContent(video: video)
let shareDialog = ShareDialog(content: content)
shareDialog.mode = .native
shareDialog.failsOnInvalidData = true
shareDialog.presentingViewController = vc
shareDialog.completion = { result in
switch result {
case .success:
print("Share succeeded")
completion()
case .failed:
print(result)
failure("Error while sharing on facebook")
case .cancelled:
print("Share cancelled")
}
}
DispatchQueue.main.async {
do {
try shareDialog.show()
} catch {
print(error)
failure("Error while sharing video content (error)")
}
}
}
facebook facebook-ios-sdk
add a comment |
My app had an option to share an online video to Facebook using graph API and was working until August 2018. I just discovered that publish_action got deprecated and that we cannot use graph API in order to share videos on Facebook.
So I tried another solution: to download the video locally, copy it to assets and then use the old FBSDKShareDialog to share it(setting the url on iOS bellow 11 and setting the asset on iOS >= 11), but the post on Facebook appeared without the video, only the text.
Then I tried it using the new ShareDialog class and because it works only with the video URL, after I copied it to assets I could not get its asset URL, but its file URL so I got the following error in the logs:
"Error while sharing video content Error Domain=com.facebook.sdk.share Code=2 "(null)" UserInfo={com.facebook.sdk:FBSDKErrorArgumentValueKey=file:///var/mobile/Media/DCIM/105APPLE/IMG_5953.MP4, com.facebook.sdk:FBSDKErrorDeveloperMessageKey=Must refer to an asset file., com.facebook.sdk:FBSDKErrorArgumentNameKey=videoURL}".
Here is my code. Please tell me what should I change in order to share a video on Facebook. If I share the URL then it shows only the URL in the post and I cannot show the video bellow.
Thanks in advance!
private func downloadVideoForUploadOnFacebook(urlString: String, videoId: Int, isMyVideo: Bool, successHandler: @escaping () -> Void, failureHandler: @escaping (String) -> Void) {
guard let url = URL(string: urlString) else {delegate.stopActivityIndicator(); return}
let request = Alamofire.request(URLRequest(url: url))
request.responseData {[weak self] (response) in
if let data = response.result.value {
let documentsURL = FileManager.default.urls(for: .documentDirectory, in: .userDomainMask).first!
let videoURL = documentsURL.appendingPathComponent("video.mp4")
do {
try data.write(to: videoURL)
self?.showShareDialog(localVideoUrl: videoURL, completion: {
print("success")
successHandler()
}, failure: { (error) in
print(error)
failureHandler(error)
})
} catch {
print("Something went wrong!")
}
}
}.downloadProgress { (progress) in
print(progress)
}
}
private func showShareDialog(localVideoUrl: URL, completion: @escaping () -> Void, failure: @escaping (String) -> Void) {
guard let navigationController = UIApplication.shared.keyWindow?.rootViewController as? UINavigationController, let vc = navigationController.viewControllers.last else {return}
if PHPhotoLibrary.authorizationStatus() == .authorized {
showFacebookShareDialog(localVideoUrl: localVideoUrl, vc: vc, completion: completion, failure: failure)
} else {
PHPhotoLibrary.requestAuthorization({[weak self] (status) in
switch status {
case .authorized:
self?.showFacebookShareDialog(localVideoUrl: localVideoUrl, vc: vc, completion: completion, failure: failure)
default:
print(status)
failure("Authorization error")
}
})
}
}
private func showFacebookShareDialog(localVideoUrl: URL, vc: UIViewController, completion: @escaping () -> Void, failure: @escaping (String) -> Void) {
var placeHolder : PHObjectPlaceholder?
do {
try PHPhotoLibrary.shared().performChangesAndWait {
let creationRequest = PHAssetChangeRequest.creationRequestForAssetFromVideo(atFileURL: localVideoUrl )
placeHolder = creationRequest?.placeholderForCreatedAsset
}
}
catch {
print(error)
failure(error.localizedDescription)
}
let result = PHAsset.fetchAssets(withLocalIdentifiers: [placeHolder!.localIdentifier], options: nil)
if let phAsset = result.firstObject {
getURL(ofPhotoWith: phAsset, completionHandler: {[weak self] (url) in
guard let url = url else {return}
self?.showNewDialog(localVideoUrl: url, vc: vc, completion: completion, failure: failure)
// if #available(iOS 11.0, *) {
// self?.showOldDialog(asset: phAsset, vc: vc)
// } else {
// self?.showOldDialog(localVideoUrl: url, vc: vc)
// }
})
} else {
failure("Error at assets")
}
}
func showOldDialog(localVideoUrl: URL, vc: UIViewController) {
let video = FBSDKShareVideo()
video.videoURL = localVideoUrl
let content = FBSDKShareVideoContent()
content.video = video
let shareDialog: FBSDKShareDialog = FBSDKShareDialog()
shareDialog.shareContent = content
shareDialog.fromViewController = vc
DispatchQueue.main.async {
shareDialog.show()
}
}
func showOldDialog(asset: PHAsset, vc: UIViewController) {
let video = FBSDKShareVideo()
video.videoAsset = asset
let content = FBSDKShareVideoContent()
content.video = video
let shareDialog: FBSDKShareDialog = FBSDKShareDialog()
shareDialog.shareContent = content
shareDialog.fromViewController = vc
DispatchQueue.main.async {
shareDialog.show()
}
}
func showNewDialog(localVideoUrl: URL, vc: UIViewController, completion: @escaping () -> Void, failure: @escaping (String) -> Void) {
let video = FacebookShare.Video(url: localVideoUrl)
let content = VideoShareContent(video: video)
let shareDialog = ShareDialog(content: content)
shareDialog.mode = .native
shareDialog.failsOnInvalidData = true
shareDialog.presentingViewController = vc
shareDialog.completion = { result in
switch result {
case .success:
print("Share succeeded")
completion()
case .failed:
print(result)
failure("Error while sharing on facebook")
case .cancelled:
print("Share cancelled")
}
}
DispatchQueue.main.async {
do {
try shareDialog.show()
} catch {
print(error)
failure("Error while sharing video content (error)")
}
}
}
facebook facebook-ios-sdk
I am experiencing the exact same problem... Still investigating... :(
– Gaetan
Nov 28 '18 at 22:56
add a comment |
My app had an option to share an online video to Facebook using graph API and was working until August 2018. I just discovered that publish_action got deprecated and that we cannot use graph API in order to share videos on Facebook.
So I tried another solution: to download the video locally, copy it to assets and then use the old FBSDKShareDialog to share it(setting the url on iOS bellow 11 and setting the asset on iOS >= 11), but the post on Facebook appeared without the video, only the text.
Then I tried it using the new ShareDialog class and because it works only with the video URL, after I copied it to assets I could not get its asset URL, but its file URL so I got the following error in the logs:
"Error while sharing video content Error Domain=com.facebook.sdk.share Code=2 "(null)" UserInfo={com.facebook.sdk:FBSDKErrorArgumentValueKey=file:///var/mobile/Media/DCIM/105APPLE/IMG_5953.MP4, com.facebook.sdk:FBSDKErrorDeveloperMessageKey=Must refer to an asset file., com.facebook.sdk:FBSDKErrorArgumentNameKey=videoURL}".
Here is my code. Please tell me what should I change in order to share a video on Facebook. If I share the URL then it shows only the URL in the post and I cannot show the video bellow.
Thanks in advance!
private func downloadVideoForUploadOnFacebook(urlString: String, videoId: Int, isMyVideo: Bool, successHandler: @escaping () -> Void, failureHandler: @escaping (String) -> Void) {
guard let url = URL(string: urlString) else {delegate.stopActivityIndicator(); return}
let request = Alamofire.request(URLRequest(url: url))
request.responseData {[weak self] (response) in
if let data = response.result.value {
let documentsURL = FileManager.default.urls(for: .documentDirectory, in: .userDomainMask).first!
let videoURL = documentsURL.appendingPathComponent("video.mp4")
do {
try data.write(to: videoURL)
self?.showShareDialog(localVideoUrl: videoURL, completion: {
print("success")
successHandler()
}, failure: { (error) in
print(error)
failureHandler(error)
})
} catch {
print("Something went wrong!")
}
}
}.downloadProgress { (progress) in
print(progress)
}
}
private func showShareDialog(localVideoUrl: URL, completion: @escaping () -> Void, failure: @escaping (String) -> Void) {
guard let navigationController = UIApplication.shared.keyWindow?.rootViewController as? UINavigationController, let vc = navigationController.viewControllers.last else {return}
if PHPhotoLibrary.authorizationStatus() == .authorized {
showFacebookShareDialog(localVideoUrl: localVideoUrl, vc: vc, completion: completion, failure: failure)
} else {
PHPhotoLibrary.requestAuthorization({[weak self] (status) in
switch status {
case .authorized:
self?.showFacebookShareDialog(localVideoUrl: localVideoUrl, vc: vc, completion: completion, failure: failure)
default:
print(status)
failure("Authorization error")
}
})
}
}
private func showFacebookShareDialog(localVideoUrl: URL, vc: UIViewController, completion: @escaping () -> Void, failure: @escaping (String) -> Void) {
var placeHolder : PHObjectPlaceholder?
do {
try PHPhotoLibrary.shared().performChangesAndWait {
let creationRequest = PHAssetChangeRequest.creationRequestForAssetFromVideo(atFileURL: localVideoUrl )
placeHolder = creationRequest?.placeholderForCreatedAsset
}
}
catch {
print(error)
failure(error.localizedDescription)
}
let result = PHAsset.fetchAssets(withLocalIdentifiers: [placeHolder!.localIdentifier], options: nil)
if let phAsset = result.firstObject {
getURL(ofPhotoWith: phAsset, completionHandler: {[weak self] (url) in
guard let url = url else {return}
self?.showNewDialog(localVideoUrl: url, vc: vc, completion: completion, failure: failure)
// if #available(iOS 11.0, *) {
// self?.showOldDialog(asset: phAsset, vc: vc)
// } else {
// self?.showOldDialog(localVideoUrl: url, vc: vc)
// }
})
} else {
failure("Error at assets")
}
}
func showOldDialog(localVideoUrl: URL, vc: UIViewController) {
let video = FBSDKShareVideo()
video.videoURL = localVideoUrl
let content = FBSDKShareVideoContent()
content.video = video
let shareDialog: FBSDKShareDialog = FBSDKShareDialog()
shareDialog.shareContent = content
shareDialog.fromViewController = vc
DispatchQueue.main.async {
shareDialog.show()
}
}
func showOldDialog(asset: PHAsset, vc: UIViewController) {
let video = FBSDKShareVideo()
video.videoAsset = asset
let content = FBSDKShareVideoContent()
content.video = video
let shareDialog: FBSDKShareDialog = FBSDKShareDialog()
shareDialog.shareContent = content
shareDialog.fromViewController = vc
DispatchQueue.main.async {
shareDialog.show()
}
}
func showNewDialog(localVideoUrl: URL, vc: UIViewController, completion: @escaping () -> Void, failure: @escaping (String) -> Void) {
let video = FacebookShare.Video(url: localVideoUrl)
let content = VideoShareContent(video: video)
let shareDialog = ShareDialog(content: content)
shareDialog.mode = .native
shareDialog.failsOnInvalidData = true
shareDialog.presentingViewController = vc
shareDialog.completion = { result in
switch result {
case .success:
print("Share succeeded")
completion()
case .failed:
print(result)
failure("Error while sharing on facebook")
case .cancelled:
print("Share cancelled")
}
}
DispatchQueue.main.async {
do {
try shareDialog.show()
} catch {
print(error)
failure("Error while sharing video content (error)")
}
}
}
facebook facebook-ios-sdk
My app had an option to share an online video to Facebook using graph API and was working until August 2018. I just discovered that publish_action got deprecated and that we cannot use graph API in order to share videos on Facebook.
So I tried another solution: to download the video locally, copy it to assets and then use the old FBSDKShareDialog to share it(setting the url on iOS bellow 11 and setting the asset on iOS >= 11), but the post on Facebook appeared without the video, only the text.
Then I tried it using the new ShareDialog class and because it works only with the video URL, after I copied it to assets I could not get its asset URL, but its file URL so I got the following error in the logs:
"Error while sharing video content Error Domain=com.facebook.sdk.share Code=2 "(null)" UserInfo={com.facebook.sdk:FBSDKErrorArgumentValueKey=file:///var/mobile/Media/DCIM/105APPLE/IMG_5953.MP4, com.facebook.sdk:FBSDKErrorDeveloperMessageKey=Must refer to an asset file., com.facebook.sdk:FBSDKErrorArgumentNameKey=videoURL}".
Here is my code. Please tell me what should I change in order to share a video on Facebook. If I share the URL then it shows only the URL in the post and I cannot show the video bellow.
Thanks in advance!
private func downloadVideoForUploadOnFacebook(urlString: String, videoId: Int, isMyVideo: Bool, successHandler: @escaping () -> Void, failureHandler: @escaping (String) -> Void) {
guard let url = URL(string: urlString) else {delegate.stopActivityIndicator(); return}
let request = Alamofire.request(URLRequest(url: url))
request.responseData {[weak self] (response) in
if let data = response.result.value {
let documentsURL = FileManager.default.urls(for: .documentDirectory, in: .userDomainMask).first!
let videoURL = documentsURL.appendingPathComponent("video.mp4")
do {
try data.write(to: videoURL)
self?.showShareDialog(localVideoUrl: videoURL, completion: {
print("success")
successHandler()
}, failure: { (error) in
print(error)
failureHandler(error)
})
} catch {
print("Something went wrong!")
}
}
}.downloadProgress { (progress) in
print(progress)
}
}
private func showShareDialog(localVideoUrl: URL, completion: @escaping () -> Void, failure: @escaping (String) -> Void) {
guard let navigationController = UIApplication.shared.keyWindow?.rootViewController as? UINavigationController, let vc = navigationController.viewControllers.last else {return}
if PHPhotoLibrary.authorizationStatus() == .authorized {
showFacebookShareDialog(localVideoUrl: localVideoUrl, vc: vc, completion: completion, failure: failure)
} else {
PHPhotoLibrary.requestAuthorization({[weak self] (status) in
switch status {
case .authorized:
self?.showFacebookShareDialog(localVideoUrl: localVideoUrl, vc: vc, completion: completion, failure: failure)
default:
print(status)
failure("Authorization error")
}
})
}
}
private func showFacebookShareDialog(localVideoUrl: URL, vc: UIViewController, completion: @escaping () -> Void, failure: @escaping (String) -> Void) {
var placeHolder : PHObjectPlaceholder?
do {
try PHPhotoLibrary.shared().performChangesAndWait {
let creationRequest = PHAssetChangeRequest.creationRequestForAssetFromVideo(atFileURL: localVideoUrl )
placeHolder = creationRequest?.placeholderForCreatedAsset
}
}
catch {
print(error)
failure(error.localizedDescription)
}
let result = PHAsset.fetchAssets(withLocalIdentifiers: [placeHolder!.localIdentifier], options: nil)
if let phAsset = result.firstObject {
getURL(ofPhotoWith: phAsset, completionHandler: {[weak self] (url) in
guard let url = url else {return}
self?.showNewDialog(localVideoUrl: url, vc: vc, completion: completion, failure: failure)
// if #available(iOS 11.0, *) {
// self?.showOldDialog(asset: phAsset, vc: vc)
// } else {
// self?.showOldDialog(localVideoUrl: url, vc: vc)
// }
})
} else {
failure("Error at assets")
}
}
func showOldDialog(localVideoUrl: URL, vc: UIViewController) {
let video = FBSDKShareVideo()
video.videoURL = localVideoUrl
let content = FBSDKShareVideoContent()
content.video = video
let shareDialog: FBSDKShareDialog = FBSDKShareDialog()
shareDialog.shareContent = content
shareDialog.fromViewController = vc
DispatchQueue.main.async {
shareDialog.show()
}
}
func showOldDialog(asset: PHAsset, vc: UIViewController) {
let video = FBSDKShareVideo()
video.videoAsset = asset
let content = FBSDKShareVideoContent()
content.video = video
let shareDialog: FBSDKShareDialog = FBSDKShareDialog()
shareDialog.shareContent = content
shareDialog.fromViewController = vc
DispatchQueue.main.async {
shareDialog.show()
}
}
func showNewDialog(localVideoUrl: URL, vc: UIViewController, completion: @escaping () -> Void, failure: @escaping (String) -> Void) {
let video = FacebookShare.Video(url: localVideoUrl)
let content = VideoShareContent(video: video)
let shareDialog = ShareDialog(content: content)
shareDialog.mode = .native
shareDialog.failsOnInvalidData = true
shareDialog.presentingViewController = vc
shareDialog.completion = { result in
switch result {
case .success:
print("Share succeeded")
completion()
case .failed:
print(result)
failure("Error while sharing on facebook")
case .cancelled:
print("Share cancelled")
}
}
DispatchQueue.main.async {
do {
try shareDialog.show()
} catch {
print(error)
failure("Error while sharing video content (error)")
}
}
}
facebook facebook-ios-sdk
facebook facebook-ios-sdk
asked Nov 22 '18 at 10:17
Claudia FiteroClaudia Fitero
624
624
I am experiencing the exact same problem... Still investigating... :(
– Gaetan
Nov 28 '18 at 22:56
add a comment |
I am experiencing the exact same problem... Still investigating... :(
– Gaetan
Nov 28 '18 at 22:56
I am experiencing the exact same problem... Still investigating... :(
– Gaetan
Nov 28 '18 at 22:56
I am experiencing the exact same problem... Still investigating... :(
– Gaetan
Nov 28 '18 at 22:56
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53428654%2fios-share-video-on-facebook-with-share-dialog%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53428654%2fios-share-video-on-facebook-with-share-dialog%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
zu nI0J,N,AH 1ocJ8TUUOaVOExCwUaG4c,lieL Ld6ykrVMuP 63AygU0wK9FnMuvO6G5 g
I am experiencing the exact same problem... Still investigating... :(
– Gaetan
Nov 28 '18 at 22:56