Dagger 2: Inject 2 different scopes into one object
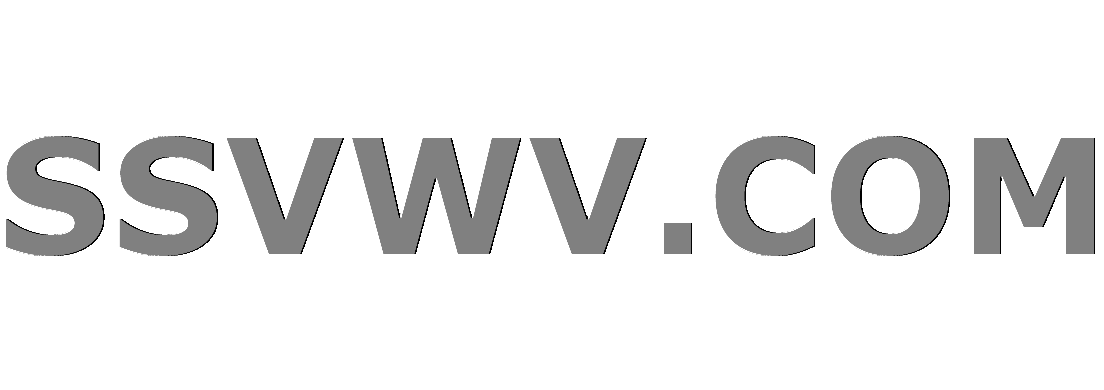
Multi tool use
Before someone starts marking this question as duplicated, let me say that I already checked this post, but it didn't solve my issue.
As the title says, I need to inject two objects from different scopes in other object.
Let's say we have the next situation:
We have a screen where we let a user sign up and then, in the same screen, we do some operation base on a user session (ex: do some sync).
For my project I was planning to use subcomponents. I have the next code (simplified) so far based on this post:
@Singleton
@Component(modules = {AppModule.class})
public interface AppComponent {
static AppComponent init(MyApplication application) {
return DaggerAppComponent.builder()
.appModule(new AppModule(application))
.build();
}
UserSessionComponent plusUserSessionComponent(UserSessionModule userSessionModule);
void inject(MyApplication application);
}
@Module
public class AppModule {
private MyApplication myApplication;
public AppModule(@NonNull MyApplication myApplication) {
this.myApplication = myApplication;
}
@Provides
@Singleton
public AppSignUp provideAppSignUp() {
return new AppSignUp();
}
}
@UserSessionScope
@Subcomponent(modules = {UserSessionModule.class})
public interface UserSessionComponent {
static UserSessionComponent init(AppComponent appComponent,
UserSession userSession) {
return appComponent.plusUserSessionComponent(userSession);
}
void inject(MyApplication myApplication);
}
@Module
public class UserSessionModule {
private UserSession userSession;
public UserSessionModule(@NonNull UserSession userSession) {
this.userSession = userSession;
}
@Provides
@UserSessionScope
public UserSessionSyncHelper provideUserSession(UserSession userSession) {
return new UserSessionSyncHelper(userSession);
}
@Provides
@UserSessionScope
public UserSessionSync provideUserSession(UserSessionSyncHelper userSessionSyncHelper) {
return new UserSessionSync(userSessionSyncHelper);
}
}
Now, in order to make it simple now, let's say I have a component (an activity, viewmodel, presenter or whatever) which will have this two dependencies:
public MyActivity extends AppCompatActivity {
@Inject
AppSignUp appSignUp;
@Inject
UserSessionSync userSessionSync;
public void onCreate(Bundle savedInstanceState) {
//to inject appSignUp
MyApplication.getAppComponent().inject(this);
}
public onUserCreated(UserSession userSession) {
//to inject userSessionSync
MyApplication.getUserSessionComponent().inject(this);
}
}
My problem here is that Dagger will complain in the first inject because AppModile does not have userSessionSync. So the question is, how can I solve this issue? Does Dagger have any feature for this scenario?
I could only think in the next solution so far:
Make UserSessionSync independent from Dagger framework and inside
public class UserSessionSync {
@Inject
UserSessionSyncHelper userSessionSyncHelper;
public UserSessionSync(){
MyApplication.getUserSessionComponent().inject(this)
}
}
In my opinion, this is not a good way to deal with this problem.

add a comment |
Before someone starts marking this question as duplicated, let me say that I already checked this post, but it didn't solve my issue.
As the title says, I need to inject two objects from different scopes in other object.
Let's say we have the next situation:
We have a screen where we let a user sign up and then, in the same screen, we do some operation base on a user session (ex: do some sync).
For my project I was planning to use subcomponents. I have the next code (simplified) so far based on this post:
@Singleton
@Component(modules = {AppModule.class})
public interface AppComponent {
static AppComponent init(MyApplication application) {
return DaggerAppComponent.builder()
.appModule(new AppModule(application))
.build();
}
UserSessionComponent plusUserSessionComponent(UserSessionModule userSessionModule);
void inject(MyApplication application);
}
@Module
public class AppModule {
private MyApplication myApplication;
public AppModule(@NonNull MyApplication myApplication) {
this.myApplication = myApplication;
}
@Provides
@Singleton
public AppSignUp provideAppSignUp() {
return new AppSignUp();
}
}
@UserSessionScope
@Subcomponent(modules = {UserSessionModule.class})
public interface UserSessionComponent {
static UserSessionComponent init(AppComponent appComponent,
UserSession userSession) {
return appComponent.plusUserSessionComponent(userSession);
}
void inject(MyApplication myApplication);
}
@Module
public class UserSessionModule {
private UserSession userSession;
public UserSessionModule(@NonNull UserSession userSession) {
this.userSession = userSession;
}
@Provides
@UserSessionScope
public UserSessionSyncHelper provideUserSession(UserSession userSession) {
return new UserSessionSyncHelper(userSession);
}
@Provides
@UserSessionScope
public UserSessionSync provideUserSession(UserSessionSyncHelper userSessionSyncHelper) {
return new UserSessionSync(userSessionSyncHelper);
}
}
Now, in order to make it simple now, let's say I have a component (an activity, viewmodel, presenter or whatever) which will have this two dependencies:
public MyActivity extends AppCompatActivity {
@Inject
AppSignUp appSignUp;
@Inject
UserSessionSync userSessionSync;
public void onCreate(Bundle savedInstanceState) {
//to inject appSignUp
MyApplication.getAppComponent().inject(this);
}
public onUserCreated(UserSession userSession) {
//to inject userSessionSync
MyApplication.getUserSessionComponent().inject(this);
}
}
My problem here is that Dagger will complain in the first inject because AppModile does not have userSessionSync. So the question is, how can I solve this issue? Does Dagger have any feature for this scenario?
I could only think in the next solution so far:
Make UserSessionSync independent from Dagger framework and inside
public class UserSessionSync {
@Inject
UserSessionSyncHelper userSessionSyncHelper;
public UserSessionSync(){
MyApplication.getUserSessionComponent().inject(this)
}
}
In my opinion, this is not a good way to deal with this problem.

add a comment |
Before someone starts marking this question as duplicated, let me say that I already checked this post, but it didn't solve my issue.
As the title says, I need to inject two objects from different scopes in other object.
Let's say we have the next situation:
We have a screen where we let a user sign up and then, in the same screen, we do some operation base on a user session (ex: do some sync).
For my project I was planning to use subcomponents. I have the next code (simplified) so far based on this post:
@Singleton
@Component(modules = {AppModule.class})
public interface AppComponent {
static AppComponent init(MyApplication application) {
return DaggerAppComponent.builder()
.appModule(new AppModule(application))
.build();
}
UserSessionComponent plusUserSessionComponent(UserSessionModule userSessionModule);
void inject(MyApplication application);
}
@Module
public class AppModule {
private MyApplication myApplication;
public AppModule(@NonNull MyApplication myApplication) {
this.myApplication = myApplication;
}
@Provides
@Singleton
public AppSignUp provideAppSignUp() {
return new AppSignUp();
}
}
@UserSessionScope
@Subcomponent(modules = {UserSessionModule.class})
public interface UserSessionComponent {
static UserSessionComponent init(AppComponent appComponent,
UserSession userSession) {
return appComponent.plusUserSessionComponent(userSession);
}
void inject(MyApplication myApplication);
}
@Module
public class UserSessionModule {
private UserSession userSession;
public UserSessionModule(@NonNull UserSession userSession) {
this.userSession = userSession;
}
@Provides
@UserSessionScope
public UserSessionSyncHelper provideUserSession(UserSession userSession) {
return new UserSessionSyncHelper(userSession);
}
@Provides
@UserSessionScope
public UserSessionSync provideUserSession(UserSessionSyncHelper userSessionSyncHelper) {
return new UserSessionSync(userSessionSyncHelper);
}
}
Now, in order to make it simple now, let's say I have a component (an activity, viewmodel, presenter or whatever) which will have this two dependencies:
public MyActivity extends AppCompatActivity {
@Inject
AppSignUp appSignUp;
@Inject
UserSessionSync userSessionSync;
public void onCreate(Bundle savedInstanceState) {
//to inject appSignUp
MyApplication.getAppComponent().inject(this);
}
public onUserCreated(UserSession userSession) {
//to inject userSessionSync
MyApplication.getUserSessionComponent().inject(this);
}
}
My problem here is that Dagger will complain in the first inject because AppModile does not have userSessionSync. So the question is, how can I solve this issue? Does Dagger have any feature for this scenario?
I could only think in the next solution so far:
Make UserSessionSync independent from Dagger framework and inside
public class UserSessionSync {
@Inject
UserSessionSyncHelper userSessionSyncHelper;
public UserSessionSync(){
MyApplication.getUserSessionComponent().inject(this)
}
}
In my opinion, this is not a good way to deal with this problem.

Before someone starts marking this question as duplicated, let me say that I already checked this post, but it didn't solve my issue.
As the title says, I need to inject two objects from different scopes in other object.
Let's say we have the next situation:
We have a screen where we let a user sign up and then, in the same screen, we do some operation base on a user session (ex: do some sync).
For my project I was planning to use subcomponents. I have the next code (simplified) so far based on this post:
@Singleton
@Component(modules = {AppModule.class})
public interface AppComponent {
static AppComponent init(MyApplication application) {
return DaggerAppComponent.builder()
.appModule(new AppModule(application))
.build();
}
UserSessionComponent plusUserSessionComponent(UserSessionModule userSessionModule);
void inject(MyApplication application);
}
@Module
public class AppModule {
private MyApplication myApplication;
public AppModule(@NonNull MyApplication myApplication) {
this.myApplication = myApplication;
}
@Provides
@Singleton
public AppSignUp provideAppSignUp() {
return new AppSignUp();
}
}
@UserSessionScope
@Subcomponent(modules = {UserSessionModule.class})
public interface UserSessionComponent {
static UserSessionComponent init(AppComponent appComponent,
UserSession userSession) {
return appComponent.plusUserSessionComponent(userSession);
}
void inject(MyApplication myApplication);
}
@Module
public class UserSessionModule {
private UserSession userSession;
public UserSessionModule(@NonNull UserSession userSession) {
this.userSession = userSession;
}
@Provides
@UserSessionScope
public UserSessionSyncHelper provideUserSession(UserSession userSession) {
return new UserSessionSyncHelper(userSession);
}
@Provides
@UserSessionScope
public UserSessionSync provideUserSession(UserSessionSyncHelper userSessionSyncHelper) {
return new UserSessionSync(userSessionSyncHelper);
}
}
Now, in order to make it simple now, let's say I have a component (an activity, viewmodel, presenter or whatever) which will have this two dependencies:
public MyActivity extends AppCompatActivity {
@Inject
AppSignUp appSignUp;
@Inject
UserSessionSync userSessionSync;
public void onCreate(Bundle savedInstanceState) {
//to inject appSignUp
MyApplication.getAppComponent().inject(this);
}
public onUserCreated(UserSession userSession) {
//to inject userSessionSync
MyApplication.getUserSessionComponent().inject(this);
}
}
My problem here is that Dagger will complain in the first inject because AppModile does not have userSessionSync. So the question is, how can I solve this issue? Does Dagger have any feature for this scenario?
I could only think in the next solution so far:
Make UserSessionSync independent from Dagger framework and inside
public class UserSessionSync {
@Inject
UserSessionSyncHelper userSessionSyncHelper;
public UserSessionSync(){
MyApplication.getUserSessionComponent().inject(this)
}
}
In my opinion, this is not a good way to deal with this problem.


asked Nov 20 at 17:53


Leandro Ocampo
692515
692515
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Tried using Dagger-Android, works fine. Also module is not really needed for AppSignUp. @Inject constructor() with @Singleton will do it. Under the hood, it uses subcomponents.
@Module
abstract class AppModule {
@Module
companion object {
@JvmStatic
@Provides
@Singleton
fun providesAppSignUp() = AppSignUp()
}
}
@Singleton
@Component( modules = [AndroidSupportInjectionModule::class, ActivityBindingModule::class, AppModule::class] )
interface AppComponent : AndroidInjector<MainApplication>
@Module
interface ActivityBindingModule {
@UserScope
@ContributesAndroidInjector( modules = [MainActivityModule::class] )
fun mainActivity() : MainActivity
}
@Module
abstract class MainActivityModule {
@Module
companion object {
@JvmStatic
@Provides
@UserScope
fun providesUserSession() = UserSession()
}
}
class UserSession
class AppSignUp
@Scope
annotation class UserScope
class MainApplication : DaggerApplication() {
@Inject
lateinit var appSignUp : AppSignUp
override fun applicationInjector(): AndroidInjector<out DaggerApplication> {
return DaggerAppComponent.builder().build()
}
}
class MainActivity : DaggerAppCompatActivity() {
@Inject
lateinit var appSignUp: AppSignUp
@Inject
lateinit var appSignUp1: AppSignUp
@Inject
lateinit var userSession: UserSession
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
}
}
Thanks for your answer but your code shows how to inject objects in the activity and that is not my problem. Actually, I am using that but I didn't add it to this example. In your code, though the userSession is defined with userScope, it is being injected since the very beginning in the activity and it works as a singleton, on the other hand, in my example, the userSession has a custom scope and it should be injected when the app has a new user session (with the code I wrote that part is solved, though I do not know how a class can be injected with different objects having different scopes).
– Leandro Ocampo
Nov 20 at 22:52
Take a look at github.com/frogermcs/GithubClient. GithubClientApplication has the user management and has the explicit logic to reset upon user log out (kind of).
– Vairavan
Nov 21 at 0:28
@LeandroOcampo In that case, UserComponent is a subcomponent of AppComponent and has visibility into the parent bindings. Invoking inject on UserComponent takes care of injecting bindings provided by AppComponent.
– Vairavan
Nov 21 at 0:53
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53398783%2fdagger-2-inject-2-different-scopes-into-one-object%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Tried using Dagger-Android, works fine. Also module is not really needed for AppSignUp. @Inject constructor() with @Singleton will do it. Under the hood, it uses subcomponents.
@Module
abstract class AppModule {
@Module
companion object {
@JvmStatic
@Provides
@Singleton
fun providesAppSignUp() = AppSignUp()
}
}
@Singleton
@Component( modules = [AndroidSupportInjectionModule::class, ActivityBindingModule::class, AppModule::class] )
interface AppComponent : AndroidInjector<MainApplication>
@Module
interface ActivityBindingModule {
@UserScope
@ContributesAndroidInjector( modules = [MainActivityModule::class] )
fun mainActivity() : MainActivity
}
@Module
abstract class MainActivityModule {
@Module
companion object {
@JvmStatic
@Provides
@UserScope
fun providesUserSession() = UserSession()
}
}
class UserSession
class AppSignUp
@Scope
annotation class UserScope
class MainApplication : DaggerApplication() {
@Inject
lateinit var appSignUp : AppSignUp
override fun applicationInjector(): AndroidInjector<out DaggerApplication> {
return DaggerAppComponent.builder().build()
}
}
class MainActivity : DaggerAppCompatActivity() {
@Inject
lateinit var appSignUp: AppSignUp
@Inject
lateinit var appSignUp1: AppSignUp
@Inject
lateinit var userSession: UserSession
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
}
}
Thanks for your answer but your code shows how to inject objects in the activity and that is not my problem. Actually, I am using that but I didn't add it to this example. In your code, though the userSession is defined with userScope, it is being injected since the very beginning in the activity and it works as a singleton, on the other hand, in my example, the userSession has a custom scope and it should be injected when the app has a new user session (with the code I wrote that part is solved, though I do not know how a class can be injected with different objects having different scopes).
– Leandro Ocampo
Nov 20 at 22:52
Take a look at github.com/frogermcs/GithubClient. GithubClientApplication has the user management and has the explicit logic to reset upon user log out (kind of).
– Vairavan
Nov 21 at 0:28
@LeandroOcampo In that case, UserComponent is a subcomponent of AppComponent and has visibility into the parent bindings. Invoking inject on UserComponent takes care of injecting bindings provided by AppComponent.
– Vairavan
Nov 21 at 0:53
add a comment |
Tried using Dagger-Android, works fine. Also module is not really needed for AppSignUp. @Inject constructor() with @Singleton will do it. Under the hood, it uses subcomponents.
@Module
abstract class AppModule {
@Module
companion object {
@JvmStatic
@Provides
@Singleton
fun providesAppSignUp() = AppSignUp()
}
}
@Singleton
@Component( modules = [AndroidSupportInjectionModule::class, ActivityBindingModule::class, AppModule::class] )
interface AppComponent : AndroidInjector<MainApplication>
@Module
interface ActivityBindingModule {
@UserScope
@ContributesAndroidInjector( modules = [MainActivityModule::class] )
fun mainActivity() : MainActivity
}
@Module
abstract class MainActivityModule {
@Module
companion object {
@JvmStatic
@Provides
@UserScope
fun providesUserSession() = UserSession()
}
}
class UserSession
class AppSignUp
@Scope
annotation class UserScope
class MainApplication : DaggerApplication() {
@Inject
lateinit var appSignUp : AppSignUp
override fun applicationInjector(): AndroidInjector<out DaggerApplication> {
return DaggerAppComponent.builder().build()
}
}
class MainActivity : DaggerAppCompatActivity() {
@Inject
lateinit var appSignUp: AppSignUp
@Inject
lateinit var appSignUp1: AppSignUp
@Inject
lateinit var userSession: UserSession
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
}
}
Thanks for your answer but your code shows how to inject objects in the activity and that is not my problem. Actually, I am using that but I didn't add it to this example. In your code, though the userSession is defined with userScope, it is being injected since the very beginning in the activity and it works as a singleton, on the other hand, in my example, the userSession has a custom scope and it should be injected when the app has a new user session (with the code I wrote that part is solved, though I do not know how a class can be injected with different objects having different scopes).
– Leandro Ocampo
Nov 20 at 22:52
Take a look at github.com/frogermcs/GithubClient. GithubClientApplication has the user management and has the explicit logic to reset upon user log out (kind of).
– Vairavan
Nov 21 at 0:28
@LeandroOcampo In that case, UserComponent is a subcomponent of AppComponent and has visibility into the parent bindings. Invoking inject on UserComponent takes care of injecting bindings provided by AppComponent.
– Vairavan
Nov 21 at 0:53
add a comment |
Tried using Dagger-Android, works fine. Also module is not really needed for AppSignUp. @Inject constructor() with @Singleton will do it. Under the hood, it uses subcomponents.
@Module
abstract class AppModule {
@Module
companion object {
@JvmStatic
@Provides
@Singleton
fun providesAppSignUp() = AppSignUp()
}
}
@Singleton
@Component( modules = [AndroidSupportInjectionModule::class, ActivityBindingModule::class, AppModule::class] )
interface AppComponent : AndroidInjector<MainApplication>
@Module
interface ActivityBindingModule {
@UserScope
@ContributesAndroidInjector( modules = [MainActivityModule::class] )
fun mainActivity() : MainActivity
}
@Module
abstract class MainActivityModule {
@Module
companion object {
@JvmStatic
@Provides
@UserScope
fun providesUserSession() = UserSession()
}
}
class UserSession
class AppSignUp
@Scope
annotation class UserScope
class MainApplication : DaggerApplication() {
@Inject
lateinit var appSignUp : AppSignUp
override fun applicationInjector(): AndroidInjector<out DaggerApplication> {
return DaggerAppComponent.builder().build()
}
}
class MainActivity : DaggerAppCompatActivity() {
@Inject
lateinit var appSignUp: AppSignUp
@Inject
lateinit var appSignUp1: AppSignUp
@Inject
lateinit var userSession: UserSession
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
}
}
Tried using Dagger-Android, works fine. Also module is not really needed for AppSignUp. @Inject constructor() with @Singleton will do it. Under the hood, it uses subcomponents.
@Module
abstract class AppModule {
@Module
companion object {
@JvmStatic
@Provides
@Singleton
fun providesAppSignUp() = AppSignUp()
}
}
@Singleton
@Component( modules = [AndroidSupportInjectionModule::class, ActivityBindingModule::class, AppModule::class] )
interface AppComponent : AndroidInjector<MainApplication>
@Module
interface ActivityBindingModule {
@UserScope
@ContributesAndroidInjector( modules = [MainActivityModule::class] )
fun mainActivity() : MainActivity
}
@Module
abstract class MainActivityModule {
@Module
companion object {
@JvmStatic
@Provides
@UserScope
fun providesUserSession() = UserSession()
}
}
class UserSession
class AppSignUp
@Scope
annotation class UserScope
class MainApplication : DaggerApplication() {
@Inject
lateinit var appSignUp : AppSignUp
override fun applicationInjector(): AndroidInjector<out DaggerApplication> {
return DaggerAppComponent.builder().build()
}
}
class MainActivity : DaggerAppCompatActivity() {
@Inject
lateinit var appSignUp: AppSignUp
@Inject
lateinit var appSignUp1: AppSignUp
@Inject
lateinit var userSession: UserSession
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
}
}
answered Nov 20 at 20:50


Vairavan
10317
10317
Thanks for your answer but your code shows how to inject objects in the activity and that is not my problem. Actually, I am using that but I didn't add it to this example. In your code, though the userSession is defined with userScope, it is being injected since the very beginning in the activity and it works as a singleton, on the other hand, in my example, the userSession has a custom scope and it should be injected when the app has a new user session (with the code I wrote that part is solved, though I do not know how a class can be injected with different objects having different scopes).
– Leandro Ocampo
Nov 20 at 22:52
Take a look at github.com/frogermcs/GithubClient. GithubClientApplication has the user management and has the explicit logic to reset upon user log out (kind of).
– Vairavan
Nov 21 at 0:28
@LeandroOcampo In that case, UserComponent is a subcomponent of AppComponent and has visibility into the parent bindings. Invoking inject on UserComponent takes care of injecting bindings provided by AppComponent.
– Vairavan
Nov 21 at 0:53
add a comment |
Thanks for your answer but your code shows how to inject objects in the activity and that is not my problem. Actually, I am using that but I didn't add it to this example. In your code, though the userSession is defined with userScope, it is being injected since the very beginning in the activity and it works as a singleton, on the other hand, in my example, the userSession has a custom scope and it should be injected when the app has a new user session (with the code I wrote that part is solved, though I do not know how a class can be injected with different objects having different scopes).
– Leandro Ocampo
Nov 20 at 22:52
Take a look at github.com/frogermcs/GithubClient. GithubClientApplication has the user management and has the explicit logic to reset upon user log out (kind of).
– Vairavan
Nov 21 at 0:28
@LeandroOcampo In that case, UserComponent is a subcomponent of AppComponent and has visibility into the parent bindings. Invoking inject on UserComponent takes care of injecting bindings provided by AppComponent.
– Vairavan
Nov 21 at 0:53
Thanks for your answer but your code shows how to inject objects in the activity and that is not my problem. Actually, I am using that but I didn't add it to this example. In your code, though the userSession is defined with userScope, it is being injected since the very beginning in the activity and it works as a singleton, on the other hand, in my example, the userSession has a custom scope and it should be injected when the app has a new user session (with the code I wrote that part is solved, though I do not know how a class can be injected with different objects having different scopes).
– Leandro Ocampo
Nov 20 at 22:52
Thanks for your answer but your code shows how to inject objects in the activity and that is not my problem. Actually, I am using that but I didn't add it to this example. In your code, though the userSession is defined with userScope, it is being injected since the very beginning in the activity and it works as a singleton, on the other hand, in my example, the userSession has a custom scope and it should be injected when the app has a new user session (with the code I wrote that part is solved, though I do not know how a class can be injected with different objects having different scopes).
– Leandro Ocampo
Nov 20 at 22:52
Take a look at github.com/frogermcs/GithubClient. GithubClientApplication has the user management and has the explicit logic to reset upon user log out (kind of).
– Vairavan
Nov 21 at 0:28
Take a look at github.com/frogermcs/GithubClient. GithubClientApplication has the user management and has the explicit logic to reset upon user log out (kind of).
– Vairavan
Nov 21 at 0:28
@LeandroOcampo In that case, UserComponent is a subcomponent of AppComponent and has visibility into the parent bindings. Invoking inject on UserComponent takes care of injecting bindings provided by AppComponent.
– Vairavan
Nov 21 at 0:53
@LeandroOcampo In that case, UserComponent is a subcomponent of AppComponent and has visibility into the parent bindings. Invoking inject on UserComponent takes care of injecting bindings provided by AppComponent.
– Vairavan
Nov 21 at 0:53
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53398783%2fdagger-2-inject-2-different-scopes-into-one-object%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
abuqC8uZ8P,GL x7aNMG