How to insert data for one to many relationship between two tables in WPF c# using Entity Framework?
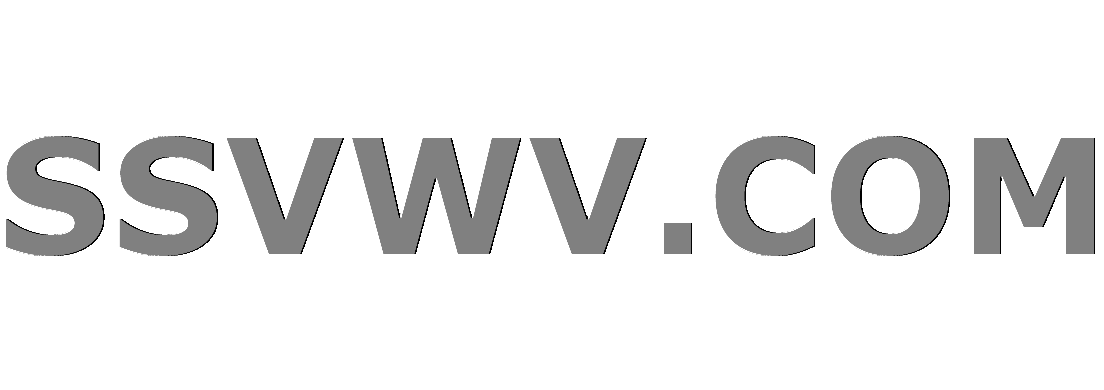
Multi tool use
try
{
foreach (IEnumerable<string> row in itemgridshow.Items)
{
var valueslist = row.ToList();
Order_Item oi = new Order_Item();
oi.Item_Description = valueslist[0].ToString();
oi.Item_Copy = int.Parse(valueslist[1].ToString());
oi.Item_Price = int.Parse(valueslist[2].ToString());
oi.Item_Amount = int.Parse(valueslist[3].ToString());
oi.Item_Image = valueslist[4].ToString();
neworder.Order_Item.Add(oi);
db.Order_Item.Add(oi);
}
db.Order_Master.Add(neworder);
db.SaveChanges();
}
Order_Master
is the primary table and Order_Item
is the secondary table. I want to insert Order_Master
record & many Order_item
details in a single save button operation.
db
is the context name.
For order_item
data is pass through datagrid (itemgrid.items) in string array.
All looks good and above code doesn't cause any errors, but data is not inserted into the database. Please help me to fix this issue.
c# .net wpf entity-framework desktop-application
add a comment |
try
{
foreach (IEnumerable<string> row in itemgridshow.Items)
{
var valueslist = row.ToList();
Order_Item oi = new Order_Item();
oi.Item_Description = valueslist[0].ToString();
oi.Item_Copy = int.Parse(valueslist[1].ToString());
oi.Item_Price = int.Parse(valueslist[2].ToString());
oi.Item_Amount = int.Parse(valueslist[3].ToString());
oi.Item_Image = valueslist[4].ToString();
neworder.Order_Item.Add(oi);
db.Order_Item.Add(oi);
}
db.Order_Master.Add(neworder);
db.SaveChanges();
}
Order_Master
is the primary table and Order_Item
is the secondary table. I want to insert Order_Master
record & many Order_item
details in a single save button operation.
db
is the context name.
For order_item
data is pass through datagrid (itemgrid.items) in string array.
All looks good and above code doesn't cause any errors, but data is not inserted into the database. Please help me to fix this issue.
c# .net wpf entity-framework desktop-application
Where isneworder
declared, it at least can't be seen in this code excerpt. Also I'm not certain how smart EF is if you try to add anorder_item
toneworder
before the latter exists in the database.
– Wubbler
Nov 24 '18 at 14:38
neworder is declared outside try block. sorry i forgot to mention.
– chirag
Nov 24 '18 at 14:42
We need to see how neworder is being declared. Also, how is neworder.Order_Item being instantiated? You're calling Add on it inside of your loop, but we never see it initialized. Also, how are your entities configured. Your code is syntactically correct and has a few extra calls you don't need, so if it's not saving, it's likely something wrong with the entities.
– Jason Armstrong
Nov 24 '18 at 15:00
so, do you get any Exceptions in the catch part of your code?
– Marty
Nov 24 '18 at 15:08
add a comment |
try
{
foreach (IEnumerable<string> row in itemgridshow.Items)
{
var valueslist = row.ToList();
Order_Item oi = new Order_Item();
oi.Item_Description = valueslist[0].ToString();
oi.Item_Copy = int.Parse(valueslist[1].ToString());
oi.Item_Price = int.Parse(valueslist[2].ToString());
oi.Item_Amount = int.Parse(valueslist[3].ToString());
oi.Item_Image = valueslist[4].ToString();
neworder.Order_Item.Add(oi);
db.Order_Item.Add(oi);
}
db.Order_Master.Add(neworder);
db.SaveChanges();
}
Order_Master
is the primary table and Order_Item
is the secondary table. I want to insert Order_Master
record & many Order_item
details in a single save button operation.
db
is the context name.
For order_item
data is pass through datagrid (itemgrid.items) in string array.
All looks good and above code doesn't cause any errors, but data is not inserted into the database. Please help me to fix this issue.
c# .net wpf entity-framework desktop-application
try
{
foreach (IEnumerable<string> row in itemgridshow.Items)
{
var valueslist = row.ToList();
Order_Item oi = new Order_Item();
oi.Item_Description = valueslist[0].ToString();
oi.Item_Copy = int.Parse(valueslist[1].ToString());
oi.Item_Price = int.Parse(valueslist[2].ToString());
oi.Item_Amount = int.Parse(valueslist[3].ToString());
oi.Item_Image = valueslist[4].ToString();
neworder.Order_Item.Add(oi);
db.Order_Item.Add(oi);
}
db.Order_Master.Add(neworder);
db.SaveChanges();
}
Order_Master
is the primary table and Order_Item
is the secondary table. I want to insert Order_Master
record & many Order_item
details in a single save button operation.
db
is the context name.
For order_item
data is pass through datagrid (itemgrid.items) in string array.
All looks good and above code doesn't cause any errors, but data is not inserted into the database. Please help me to fix this issue.
c# .net wpf entity-framework desktop-application
c# .net wpf entity-framework desktop-application
edited Nov 24 '18 at 16:43
marc_s
579k12911181265
579k12911181265
asked Nov 24 '18 at 14:19


chiragchirag
616
616
Where isneworder
declared, it at least can't be seen in this code excerpt. Also I'm not certain how smart EF is if you try to add anorder_item
toneworder
before the latter exists in the database.
– Wubbler
Nov 24 '18 at 14:38
neworder is declared outside try block. sorry i forgot to mention.
– chirag
Nov 24 '18 at 14:42
We need to see how neworder is being declared. Also, how is neworder.Order_Item being instantiated? You're calling Add on it inside of your loop, but we never see it initialized. Also, how are your entities configured. Your code is syntactically correct and has a few extra calls you don't need, so if it's not saving, it's likely something wrong with the entities.
– Jason Armstrong
Nov 24 '18 at 15:00
so, do you get any Exceptions in the catch part of your code?
– Marty
Nov 24 '18 at 15:08
add a comment |
Where isneworder
declared, it at least can't be seen in this code excerpt. Also I'm not certain how smart EF is if you try to add anorder_item
toneworder
before the latter exists in the database.
– Wubbler
Nov 24 '18 at 14:38
neworder is declared outside try block. sorry i forgot to mention.
– chirag
Nov 24 '18 at 14:42
We need to see how neworder is being declared. Also, how is neworder.Order_Item being instantiated? You're calling Add on it inside of your loop, but we never see it initialized. Also, how are your entities configured. Your code is syntactically correct and has a few extra calls you don't need, so if it's not saving, it's likely something wrong with the entities.
– Jason Armstrong
Nov 24 '18 at 15:00
so, do you get any Exceptions in the catch part of your code?
– Marty
Nov 24 '18 at 15:08
Where is
neworder
declared, it at least can't be seen in this code excerpt. Also I'm not certain how smart EF is if you try to add an order_item
to neworder
before the latter exists in the database.– Wubbler
Nov 24 '18 at 14:38
Where is
neworder
declared, it at least can't be seen in this code excerpt. Also I'm not certain how smart EF is if you try to add an order_item
to neworder
before the latter exists in the database.– Wubbler
Nov 24 '18 at 14:38
neworder is declared outside try block. sorry i forgot to mention.
– chirag
Nov 24 '18 at 14:42
neworder is declared outside try block. sorry i forgot to mention.
– chirag
Nov 24 '18 at 14:42
We need to see how neworder is being declared. Also, how is neworder.Order_Item being instantiated? You're calling Add on it inside of your loop, but we never see it initialized. Also, how are your entities configured. Your code is syntactically correct and has a few extra calls you don't need, so if it's not saving, it's likely something wrong with the entities.
– Jason Armstrong
Nov 24 '18 at 15:00
We need to see how neworder is being declared. Also, how is neworder.Order_Item being instantiated? You're calling Add on it inside of your loop, but we never see it initialized. Also, how are your entities configured. Your code is syntactically correct and has a few extra calls you don't need, so if it's not saving, it's likely something wrong with the entities.
– Jason Armstrong
Nov 24 '18 at 15:00
so, do you get any Exceptions in the catch part of your code?
– Marty
Nov 24 '18 at 15:08
so, do you get any Exceptions in the catch part of your code?
– Marty
Nov 24 '18 at 15:08
add a comment |
1 Answer
1
active
oldest
votes
I don't see any logic issues with the code snippet above.
Try restructuring the code, encapsulating the context usage might uncover why the save isn't happening.
public void SaveOrder(IEnumerable<string> rows)
{
// Create a new order and add items
var neworder = new Order_Master();
foreach (var row in rows)
{
var valueslist = row.ToList();
var oi = new Order_Item();
oi.Item_Description = valueslist[0].ToString();
// Set more properties...
// Add the item to the order
neworder.Order_Item.Add(oi);
}
// Instance a context, add the order and save
try
{
using (var db = new OrderContext())
{
db.Order_Master.Add(neworder);
db.SaveChanges();
}
}
catch (Exception e)
{
throw new Exception($"Failed to save to database: {e.Message}");
}
}
Entity Model should look something like this:
public class OrderContext : DbContext
{
public DbSet<Order_Master> Order_Master { get; set; }
public DbSet<Order_Item> Order_Items { get; set; }
// More entities...
}
public class Order_Item
{
public int Id { get; set; }
public string Item_Description { get; set; }
// More properties...
}
public class Order_Master
{
public Order_Master()
{
Order_Item = new List<Order_Item>();
}
public ICollection<Order_Item> Order_Item { get; set; }
public int Id { get; set; }
public string Order_Description { get; set; }
// More properties...
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53459082%2fhow-to-insert-data-for-one-to-many-relationship-between-two-tables-in-wpf-c-shar%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I don't see any logic issues with the code snippet above.
Try restructuring the code, encapsulating the context usage might uncover why the save isn't happening.
public void SaveOrder(IEnumerable<string> rows)
{
// Create a new order and add items
var neworder = new Order_Master();
foreach (var row in rows)
{
var valueslist = row.ToList();
var oi = new Order_Item();
oi.Item_Description = valueslist[0].ToString();
// Set more properties...
// Add the item to the order
neworder.Order_Item.Add(oi);
}
// Instance a context, add the order and save
try
{
using (var db = new OrderContext())
{
db.Order_Master.Add(neworder);
db.SaveChanges();
}
}
catch (Exception e)
{
throw new Exception($"Failed to save to database: {e.Message}");
}
}
Entity Model should look something like this:
public class OrderContext : DbContext
{
public DbSet<Order_Master> Order_Master { get; set; }
public DbSet<Order_Item> Order_Items { get; set; }
// More entities...
}
public class Order_Item
{
public int Id { get; set; }
public string Item_Description { get; set; }
// More properties...
}
public class Order_Master
{
public Order_Master()
{
Order_Item = new List<Order_Item>();
}
public ICollection<Order_Item> Order_Item { get; set; }
public int Id { get; set; }
public string Order_Description { get; set; }
// More properties...
}
add a comment |
I don't see any logic issues with the code snippet above.
Try restructuring the code, encapsulating the context usage might uncover why the save isn't happening.
public void SaveOrder(IEnumerable<string> rows)
{
// Create a new order and add items
var neworder = new Order_Master();
foreach (var row in rows)
{
var valueslist = row.ToList();
var oi = new Order_Item();
oi.Item_Description = valueslist[0].ToString();
// Set more properties...
// Add the item to the order
neworder.Order_Item.Add(oi);
}
// Instance a context, add the order and save
try
{
using (var db = new OrderContext())
{
db.Order_Master.Add(neworder);
db.SaveChanges();
}
}
catch (Exception e)
{
throw new Exception($"Failed to save to database: {e.Message}");
}
}
Entity Model should look something like this:
public class OrderContext : DbContext
{
public DbSet<Order_Master> Order_Master { get; set; }
public DbSet<Order_Item> Order_Items { get; set; }
// More entities...
}
public class Order_Item
{
public int Id { get; set; }
public string Item_Description { get; set; }
// More properties...
}
public class Order_Master
{
public Order_Master()
{
Order_Item = new List<Order_Item>();
}
public ICollection<Order_Item> Order_Item { get; set; }
public int Id { get; set; }
public string Order_Description { get; set; }
// More properties...
}
add a comment |
I don't see any logic issues with the code snippet above.
Try restructuring the code, encapsulating the context usage might uncover why the save isn't happening.
public void SaveOrder(IEnumerable<string> rows)
{
// Create a new order and add items
var neworder = new Order_Master();
foreach (var row in rows)
{
var valueslist = row.ToList();
var oi = new Order_Item();
oi.Item_Description = valueslist[0].ToString();
// Set more properties...
// Add the item to the order
neworder.Order_Item.Add(oi);
}
// Instance a context, add the order and save
try
{
using (var db = new OrderContext())
{
db.Order_Master.Add(neworder);
db.SaveChanges();
}
}
catch (Exception e)
{
throw new Exception($"Failed to save to database: {e.Message}");
}
}
Entity Model should look something like this:
public class OrderContext : DbContext
{
public DbSet<Order_Master> Order_Master { get; set; }
public DbSet<Order_Item> Order_Items { get; set; }
// More entities...
}
public class Order_Item
{
public int Id { get; set; }
public string Item_Description { get; set; }
// More properties...
}
public class Order_Master
{
public Order_Master()
{
Order_Item = new List<Order_Item>();
}
public ICollection<Order_Item> Order_Item { get; set; }
public int Id { get; set; }
public string Order_Description { get; set; }
// More properties...
}
I don't see any logic issues with the code snippet above.
Try restructuring the code, encapsulating the context usage might uncover why the save isn't happening.
public void SaveOrder(IEnumerable<string> rows)
{
// Create a new order and add items
var neworder = new Order_Master();
foreach (var row in rows)
{
var valueslist = row.ToList();
var oi = new Order_Item();
oi.Item_Description = valueslist[0].ToString();
// Set more properties...
// Add the item to the order
neworder.Order_Item.Add(oi);
}
// Instance a context, add the order and save
try
{
using (var db = new OrderContext())
{
db.Order_Master.Add(neworder);
db.SaveChanges();
}
}
catch (Exception e)
{
throw new Exception($"Failed to save to database: {e.Message}");
}
}
Entity Model should look something like this:
public class OrderContext : DbContext
{
public DbSet<Order_Master> Order_Master { get; set; }
public DbSet<Order_Item> Order_Items { get; set; }
// More entities...
}
public class Order_Item
{
public int Id { get; set; }
public string Item_Description { get; set; }
// More properties...
}
public class Order_Master
{
public Order_Master()
{
Order_Item = new List<Order_Item>();
}
public ICollection<Order_Item> Order_Item { get; set; }
public int Id { get; set; }
public string Order_Description { get; set; }
// More properties...
}
answered Nov 24 '18 at 16:30
robaudasrobaudas
903411
903411
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53459082%2fhow-to-insert-data-for-one-to-many-relationship-between-two-tables-in-wpf-c-shar%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
gjx9aR
Where is
neworder
declared, it at least can't be seen in this code excerpt. Also I'm not certain how smart EF is if you try to add anorder_item
toneworder
before the latter exists in the database.– Wubbler
Nov 24 '18 at 14:38
neworder is declared outside try block. sorry i forgot to mention.
– chirag
Nov 24 '18 at 14:42
We need to see how neworder is being declared. Also, how is neworder.Order_Item being instantiated? You're calling Add on it inside of your loop, but we never see it initialized. Also, how are your entities configured. Your code is syntactically correct and has a few extra calls you don't need, so if it's not saving, it's likely something wrong with the entities.
– Jason Armstrong
Nov 24 '18 at 15:00
so, do you get any Exceptions in the catch part of your code?
– Marty
Nov 24 '18 at 15:08