Find median value of 3 user inputted numbers in C# Without output: Numbers entered: x, y, z Minimum Value: a...
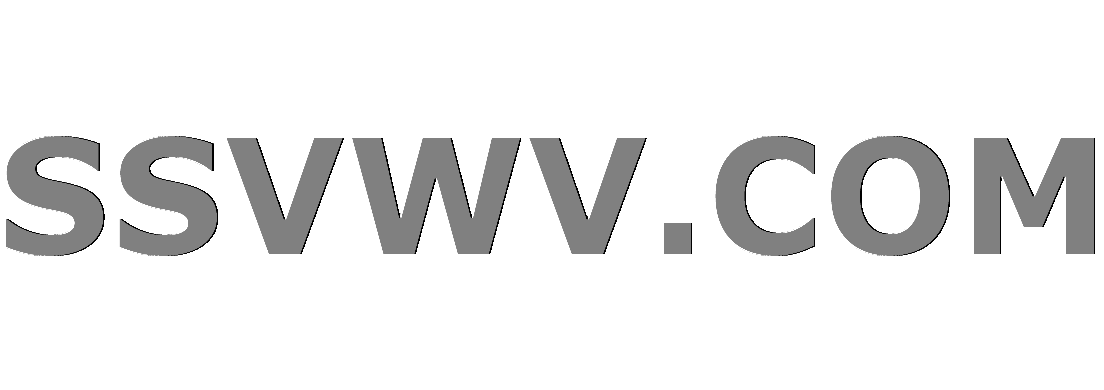
Multi tool use
I am trying to find the Median of the three user entered numbers. Please see below code, any help is appreciated and a newbie explanation of why. From what I understand they should be sorted into a list and then the middle number found. I am trying to output the below in a robust way in case I want to ask the user for 4 numbers.
I am trying to output: Numbers entered: x, y, z
Minimum Value: a
Median Value: b
using System;
public class Assignment
{
public static void Main()
{
int num1;
int num2;
int num3;
Console.WriteLine("Enter 3 numbers");
num1 = Int32.Parse(Console.ReadLine());
num2 = Int32.Parse(Console.ReadLine());
num3 = Int32.Parse(Console.ReadLine());
Console.WriteLine("Numbers entered: {0} {1} {2}", num1, num2, num3);
if(num1<num2)
if(num2<num3)
{
Console.WriteLine("Minimum Value:"+num1);
}
else
{
Console.WriteLine("Minimum Value:"+num3);
}
else
if(num2<num3)
{
Console.WriteLine( "Minimum Value:"+num2);
}
else
{
Console.WriteLine("Minimum Value:"+num3 );
}
}
}
c#
add a comment |
I am trying to find the Median of the three user entered numbers. Please see below code, any help is appreciated and a newbie explanation of why. From what I understand they should be sorted into a list and then the middle number found. I am trying to output the below in a robust way in case I want to ask the user for 4 numbers.
I am trying to output: Numbers entered: x, y, z
Minimum Value: a
Median Value: b
using System;
public class Assignment
{
public static void Main()
{
int num1;
int num2;
int num3;
Console.WriteLine("Enter 3 numbers");
num1 = Int32.Parse(Console.ReadLine());
num2 = Int32.Parse(Console.ReadLine());
num3 = Int32.Parse(Console.ReadLine());
Console.WriteLine("Numbers entered: {0} {1} {2}", num1, num2, num3);
if(num1<num2)
if(num2<num3)
{
Console.WriteLine("Minimum Value:"+num1);
}
else
{
Console.WriteLine("Minimum Value:"+num3);
}
else
if(num2<num3)
{
Console.WriteLine( "Minimum Value:"+num2);
}
else
{
Console.WriteLine("Minimum Value:"+num3 );
}
}
}
c#
add a comment |
I am trying to find the Median of the three user entered numbers. Please see below code, any help is appreciated and a newbie explanation of why. From what I understand they should be sorted into a list and then the middle number found. I am trying to output the below in a robust way in case I want to ask the user for 4 numbers.
I am trying to output: Numbers entered: x, y, z
Minimum Value: a
Median Value: b
using System;
public class Assignment
{
public static void Main()
{
int num1;
int num2;
int num3;
Console.WriteLine("Enter 3 numbers");
num1 = Int32.Parse(Console.ReadLine());
num2 = Int32.Parse(Console.ReadLine());
num3 = Int32.Parse(Console.ReadLine());
Console.WriteLine("Numbers entered: {0} {1} {2}", num1, num2, num3);
if(num1<num2)
if(num2<num3)
{
Console.WriteLine("Minimum Value:"+num1);
}
else
{
Console.WriteLine("Minimum Value:"+num3);
}
else
if(num2<num3)
{
Console.WriteLine( "Minimum Value:"+num2);
}
else
{
Console.WriteLine("Minimum Value:"+num3 );
}
}
}
c#
I am trying to find the Median of the three user entered numbers. Please see below code, any help is appreciated and a newbie explanation of why. From what I understand they should be sorted into a list and then the middle number found. I am trying to output the below in a robust way in case I want to ask the user for 4 numbers.
I am trying to output: Numbers entered: x, y, z
Minimum Value: a
Median Value: b
using System;
public class Assignment
{
public static void Main()
{
int num1;
int num2;
int num3;
Console.WriteLine("Enter 3 numbers");
num1 = Int32.Parse(Console.ReadLine());
num2 = Int32.Parse(Console.ReadLine());
num3 = Int32.Parse(Console.ReadLine());
Console.WriteLine("Numbers entered: {0} {1} {2}", num1, num2, num3);
if(num1<num2)
if(num2<num3)
{
Console.WriteLine("Minimum Value:"+num1);
}
else
{
Console.WriteLine("Minimum Value:"+num3);
}
else
if(num2<num3)
{
Console.WriteLine( "Minimum Value:"+num2);
}
else
{
Console.WriteLine("Minimum Value:"+num3 );
}
}
}
c#
c#
edited Oct 8 '17 at 10:31
Daniel Loudon
676116
676116
asked Oct 7 '17 at 22:00
Luke HealdLuke Heald
186
186
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
You're code won't compile how it's written right now, but I can see what you're trying to do. You could change it up so it would compile, but it's not the a particularly efficient way of solving it.
Right now, I think you're attempting to write code to check each scenario of the potential order of numbers. You can do it for 3 numbers relatively easy, but what if you want to extend your code to check a list of 10 or 100 numbers?
I think you should add user input to a list, sort the list, then just return the middle value of the list. It's less code and more reliable.
public class FindMedian
{
public List<int> numbersList = new List<int>(); // list to store user input
// constructor
public FindMedian(int n)
{
Console.WriteLine("Please Enter " + n + " numbers. nn");
for (int i = 1; i < n + 1; i++)
{
Console.WriteLine("Number " + i + " : ");
numbersList.Add(GetUserInput()); // call's GetUserInput
}
PrintData();
}
// returns int from Console. Will continue to run until a valid int is entered
private int GetUserInput()
{
int temp = 0;
bool numberValid = false;
while (!numberValid)
{
try
{
temp = int.Parse(Console.ReadLine());
numberValid = true;
}
catch
{
Console.WriteLine("Invalid entry. Please try again.n");
numberValid = false;
}
}
return temp;
}
// prints data after user enteres the correct number of ints
private void PrintData()
{
// If list contains no data
if (numbersList.Count < 3)
{
Console.WriteLine("No User Data Entered");
return;
}
numbersList.Sort(); // Sorts list from smallest to largest
int minimum = numbersList[0];
int median;
// if list count is even and median is average of two middle numbers
if (numbersList.Count % 2 == 0)
{
median = (numbersList[(numbersList.Count / 2) - 2] + numbersList[(numbersList.Count / 2) - 1] / 2);
}
// if list count is odd and median is just middle number
else
{
median = numbersList[(numbersList.Count / 2)];
}
Console.WriteLine("Minimum Number : " + minimum);
Console.WriteLine("Median Number : " + median);
}
}
Hello, Thank you for the detailed response that is easy to follow. As I am learning the hard way a bracket here and there makes all the difference. Please could you edit your answer so that I can copy and paste it into the code editor and run the program, that way I can backwards engineer. I've tried modifying my version with yours and of course it didn't work.
– Luke Heald
Oct 7 '17 at 23:36
add a comment |
Smells like homework
int num1 = Convert.ToInt32(Console.ReadLine());
int num2 = Convert.ToInt32(Console.ReadLine());
int num3 = Convert.ToInt32(Console.ReadLine());
List<int> list1 = new List<int>();
list1.Add(num1);
list1.Add(num2);
list1.Add(num3);
Console.WriteLine($"Min:{list.Min()}");
list1.Sort();
int c = list1.Count()%2==0?list1.Count()+1:list1.Count();
int Med = list1[c/2];
//Console.WriteLine($"Max:{list1.Max()");
I'm on phone so formatting my code is a hard ..
You can skip the creation of three ints and use a
list1.Add(Int.Parse(Console.ReadLine()));
if you wish or for 'n' numbers
bool b = true;
int num=0;
List<int>() l = new List<int>();
Do{
Console.Write("Enter number: ");
b =
int.TryParse(Console.ReadLine(), out num);
l.Add(num);
}While(b);
//insert min, max and median of (List) l here
Hey man, Thanks for the answer. It's definitely homework but only our second workshop, we actually haven't had any lessons on it yet. It's a 'find you own solutions' Then be taught how to do it. After a couple hours googling I ended up here. I'll give the above a try and see if I can figure it out, it's important to me that I understand it more than getting the right answer.
– Luke Heald
Oct 7 '17 at 22:29
Hey man I do SSD A level I know the feel I updated answer
– Daniel Loudon
Oct 7 '17 at 22:30
I'm on phone, updating my answer is a bit nifty and I rushed it - keep checking updates
– Daniel Loudon
Oct 7 '17 at 22:34
Thanks again, appreciate the help. Will keep checking back.
– Luke Heald
Oct 7 '17 at 22:39
Edited.. for n numbers
– Daniel Loudon
Oct 7 '17 at 22:45
|
show 4 more comments
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f46625540%2ffind-median-value-of-3-user-inputted-numbers-in-c-sharp-without-output-numbers%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You're code won't compile how it's written right now, but I can see what you're trying to do. You could change it up so it would compile, but it's not the a particularly efficient way of solving it.
Right now, I think you're attempting to write code to check each scenario of the potential order of numbers. You can do it for 3 numbers relatively easy, but what if you want to extend your code to check a list of 10 or 100 numbers?
I think you should add user input to a list, sort the list, then just return the middle value of the list. It's less code and more reliable.
public class FindMedian
{
public List<int> numbersList = new List<int>(); // list to store user input
// constructor
public FindMedian(int n)
{
Console.WriteLine("Please Enter " + n + " numbers. nn");
for (int i = 1; i < n + 1; i++)
{
Console.WriteLine("Number " + i + " : ");
numbersList.Add(GetUserInput()); // call's GetUserInput
}
PrintData();
}
// returns int from Console. Will continue to run until a valid int is entered
private int GetUserInput()
{
int temp = 0;
bool numberValid = false;
while (!numberValid)
{
try
{
temp = int.Parse(Console.ReadLine());
numberValid = true;
}
catch
{
Console.WriteLine("Invalid entry. Please try again.n");
numberValid = false;
}
}
return temp;
}
// prints data after user enteres the correct number of ints
private void PrintData()
{
// If list contains no data
if (numbersList.Count < 3)
{
Console.WriteLine("No User Data Entered");
return;
}
numbersList.Sort(); // Sorts list from smallest to largest
int minimum = numbersList[0];
int median;
// if list count is even and median is average of two middle numbers
if (numbersList.Count % 2 == 0)
{
median = (numbersList[(numbersList.Count / 2) - 2] + numbersList[(numbersList.Count / 2) - 1] / 2);
}
// if list count is odd and median is just middle number
else
{
median = numbersList[(numbersList.Count / 2)];
}
Console.WriteLine("Minimum Number : " + minimum);
Console.WriteLine("Median Number : " + median);
}
}
Hello, Thank you for the detailed response that is easy to follow. As I am learning the hard way a bracket here and there makes all the difference. Please could you edit your answer so that I can copy and paste it into the code editor and run the program, that way I can backwards engineer. I've tried modifying my version with yours and of course it didn't work.
– Luke Heald
Oct 7 '17 at 23:36
add a comment |
You're code won't compile how it's written right now, but I can see what you're trying to do. You could change it up so it would compile, but it's not the a particularly efficient way of solving it.
Right now, I think you're attempting to write code to check each scenario of the potential order of numbers. You can do it for 3 numbers relatively easy, but what if you want to extend your code to check a list of 10 or 100 numbers?
I think you should add user input to a list, sort the list, then just return the middle value of the list. It's less code and more reliable.
public class FindMedian
{
public List<int> numbersList = new List<int>(); // list to store user input
// constructor
public FindMedian(int n)
{
Console.WriteLine("Please Enter " + n + " numbers. nn");
for (int i = 1; i < n + 1; i++)
{
Console.WriteLine("Number " + i + " : ");
numbersList.Add(GetUserInput()); // call's GetUserInput
}
PrintData();
}
// returns int from Console. Will continue to run until a valid int is entered
private int GetUserInput()
{
int temp = 0;
bool numberValid = false;
while (!numberValid)
{
try
{
temp = int.Parse(Console.ReadLine());
numberValid = true;
}
catch
{
Console.WriteLine("Invalid entry. Please try again.n");
numberValid = false;
}
}
return temp;
}
// prints data after user enteres the correct number of ints
private void PrintData()
{
// If list contains no data
if (numbersList.Count < 3)
{
Console.WriteLine("No User Data Entered");
return;
}
numbersList.Sort(); // Sorts list from smallest to largest
int minimum = numbersList[0];
int median;
// if list count is even and median is average of two middle numbers
if (numbersList.Count % 2 == 0)
{
median = (numbersList[(numbersList.Count / 2) - 2] + numbersList[(numbersList.Count / 2) - 1] / 2);
}
// if list count is odd and median is just middle number
else
{
median = numbersList[(numbersList.Count / 2)];
}
Console.WriteLine("Minimum Number : " + minimum);
Console.WriteLine("Median Number : " + median);
}
}
Hello, Thank you for the detailed response that is easy to follow. As I am learning the hard way a bracket here and there makes all the difference. Please could you edit your answer so that I can copy and paste it into the code editor and run the program, that way I can backwards engineer. I've tried modifying my version with yours and of course it didn't work.
– Luke Heald
Oct 7 '17 at 23:36
add a comment |
You're code won't compile how it's written right now, but I can see what you're trying to do. You could change it up so it would compile, but it's not the a particularly efficient way of solving it.
Right now, I think you're attempting to write code to check each scenario of the potential order of numbers. You can do it for 3 numbers relatively easy, but what if you want to extend your code to check a list of 10 or 100 numbers?
I think you should add user input to a list, sort the list, then just return the middle value of the list. It's less code and more reliable.
public class FindMedian
{
public List<int> numbersList = new List<int>(); // list to store user input
// constructor
public FindMedian(int n)
{
Console.WriteLine("Please Enter " + n + " numbers. nn");
for (int i = 1; i < n + 1; i++)
{
Console.WriteLine("Number " + i + " : ");
numbersList.Add(GetUserInput()); // call's GetUserInput
}
PrintData();
}
// returns int from Console. Will continue to run until a valid int is entered
private int GetUserInput()
{
int temp = 0;
bool numberValid = false;
while (!numberValid)
{
try
{
temp = int.Parse(Console.ReadLine());
numberValid = true;
}
catch
{
Console.WriteLine("Invalid entry. Please try again.n");
numberValid = false;
}
}
return temp;
}
// prints data after user enteres the correct number of ints
private void PrintData()
{
// If list contains no data
if (numbersList.Count < 3)
{
Console.WriteLine("No User Data Entered");
return;
}
numbersList.Sort(); // Sorts list from smallest to largest
int minimum = numbersList[0];
int median;
// if list count is even and median is average of two middle numbers
if (numbersList.Count % 2 == 0)
{
median = (numbersList[(numbersList.Count / 2) - 2] + numbersList[(numbersList.Count / 2) - 1] / 2);
}
// if list count is odd and median is just middle number
else
{
median = numbersList[(numbersList.Count / 2)];
}
Console.WriteLine("Minimum Number : " + minimum);
Console.WriteLine("Median Number : " + median);
}
}
You're code won't compile how it's written right now, but I can see what you're trying to do. You could change it up so it would compile, but it's not the a particularly efficient way of solving it.
Right now, I think you're attempting to write code to check each scenario of the potential order of numbers. You can do it for 3 numbers relatively easy, but what if you want to extend your code to check a list of 10 or 100 numbers?
I think you should add user input to a list, sort the list, then just return the middle value of the list. It's less code and more reliable.
public class FindMedian
{
public List<int> numbersList = new List<int>(); // list to store user input
// constructor
public FindMedian(int n)
{
Console.WriteLine("Please Enter " + n + " numbers. nn");
for (int i = 1; i < n + 1; i++)
{
Console.WriteLine("Number " + i + " : ");
numbersList.Add(GetUserInput()); // call's GetUserInput
}
PrintData();
}
// returns int from Console. Will continue to run until a valid int is entered
private int GetUserInput()
{
int temp = 0;
bool numberValid = false;
while (!numberValid)
{
try
{
temp = int.Parse(Console.ReadLine());
numberValid = true;
}
catch
{
Console.WriteLine("Invalid entry. Please try again.n");
numberValid = false;
}
}
return temp;
}
// prints data after user enteres the correct number of ints
private void PrintData()
{
// If list contains no data
if (numbersList.Count < 3)
{
Console.WriteLine("No User Data Entered");
return;
}
numbersList.Sort(); // Sorts list from smallest to largest
int minimum = numbersList[0];
int median;
// if list count is even and median is average of two middle numbers
if (numbersList.Count % 2 == 0)
{
median = (numbersList[(numbersList.Count / 2) - 2] + numbersList[(numbersList.Count / 2) - 1] / 2);
}
// if list count is odd and median is just middle number
else
{
median = numbersList[(numbersList.Count / 2)];
}
Console.WriteLine("Minimum Number : " + minimum);
Console.WriteLine("Median Number : " + median);
}
}
answered Oct 7 '17 at 23:08
edm2282edm2282
1294
1294
Hello, Thank you for the detailed response that is easy to follow. As I am learning the hard way a bracket here and there makes all the difference. Please could you edit your answer so that I can copy and paste it into the code editor and run the program, that way I can backwards engineer. I've tried modifying my version with yours and of course it didn't work.
– Luke Heald
Oct 7 '17 at 23:36
add a comment |
Hello, Thank you for the detailed response that is easy to follow. As I am learning the hard way a bracket here and there makes all the difference. Please could you edit your answer so that I can copy and paste it into the code editor and run the program, that way I can backwards engineer. I've tried modifying my version with yours and of course it didn't work.
– Luke Heald
Oct 7 '17 at 23:36
Hello, Thank you for the detailed response that is easy to follow. As I am learning the hard way a bracket here and there makes all the difference. Please could you edit your answer so that I can copy and paste it into the code editor and run the program, that way I can backwards engineer. I've tried modifying my version with yours and of course it didn't work.
– Luke Heald
Oct 7 '17 at 23:36
Hello, Thank you for the detailed response that is easy to follow. As I am learning the hard way a bracket here and there makes all the difference. Please could you edit your answer so that I can copy and paste it into the code editor and run the program, that way I can backwards engineer. I've tried modifying my version with yours and of course it didn't work.
– Luke Heald
Oct 7 '17 at 23:36
add a comment |
Smells like homework
int num1 = Convert.ToInt32(Console.ReadLine());
int num2 = Convert.ToInt32(Console.ReadLine());
int num3 = Convert.ToInt32(Console.ReadLine());
List<int> list1 = new List<int>();
list1.Add(num1);
list1.Add(num2);
list1.Add(num3);
Console.WriteLine($"Min:{list.Min()}");
list1.Sort();
int c = list1.Count()%2==0?list1.Count()+1:list1.Count();
int Med = list1[c/2];
//Console.WriteLine($"Max:{list1.Max()");
I'm on phone so formatting my code is a hard ..
You can skip the creation of three ints and use a
list1.Add(Int.Parse(Console.ReadLine()));
if you wish or for 'n' numbers
bool b = true;
int num=0;
List<int>() l = new List<int>();
Do{
Console.Write("Enter number: ");
b =
int.TryParse(Console.ReadLine(), out num);
l.Add(num);
}While(b);
//insert min, max and median of (List) l here
Hey man, Thanks for the answer. It's definitely homework but only our second workshop, we actually haven't had any lessons on it yet. It's a 'find you own solutions' Then be taught how to do it. After a couple hours googling I ended up here. I'll give the above a try and see if I can figure it out, it's important to me that I understand it more than getting the right answer.
– Luke Heald
Oct 7 '17 at 22:29
Hey man I do SSD A level I know the feel I updated answer
– Daniel Loudon
Oct 7 '17 at 22:30
I'm on phone, updating my answer is a bit nifty and I rushed it - keep checking updates
– Daniel Loudon
Oct 7 '17 at 22:34
Thanks again, appreciate the help. Will keep checking back.
– Luke Heald
Oct 7 '17 at 22:39
Edited.. for n numbers
– Daniel Loudon
Oct 7 '17 at 22:45
|
show 4 more comments
Smells like homework
int num1 = Convert.ToInt32(Console.ReadLine());
int num2 = Convert.ToInt32(Console.ReadLine());
int num3 = Convert.ToInt32(Console.ReadLine());
List<int> list1 = new List<int>();
list1.Add(num1);
list1.Add(num2);
list1.Add(num3);
Console.WriteLine($"Min:{list.Min()}");
list1.Sort();
int c = list1.Count()%2==0?list1.Count()+1:list1.Count();
int Med = list1[c/2];
//Console.WriteLine($"Max:{list1.Max()");
I'm on phone so formatting my code is a hard ..
You can skip the creation of three ints and use a
list1.Add(Int.Parse(Console.ReadLine()));
if you wish or for 'n' numbers
bool b = true;
int num=0;
List<int>() l = new List<int>();
Do{
Console.Write("Enter number: ");
b =
int.TryParse(Console.ReadLine(), out num);
l.Add(num);
}While(b);
//insert min, max and median of (List) l here
Hey man, Thanks for the answer. It's definitely homework but only our second workshop, we actually haven't had any lessons on it yet. It's a 'find you own solutions' Then be taught how to do it. After a couple hours googling I ended up here. I'll give the above a try and see if I can figure it out, it's important to me that I understand it more than getting the right answer.
– Luke Heald
Oct 7 '17 at 22:29
Hey man I do SSD A level I know the feel I updated answer
– Daniel Loudon
Oct 7 '17 at 22:30
I'm on phone, updating my answer is a bit nifty and I rushed it - keep checking updates
– Daniel Loudon
Oct 7 '17 at 22:34
Thanks again, appreciate the help. Will keep checking back.
– Luke Heald
Oct 7 '17 at 22:39
Edited.. for n numbers
– Daniel Loudon
Oct 7 '17 at 22:45
|
show 4 more comments
Smells like homework
int num1 = Convert.ToInt32(Console.ReadLine());
int num2 = Convert.ToInt32(Console.ReadLine());
int num3 = Convert.ToInt32(Console.ReadLine());
List<int> list1 = new List<int>();
list1.Add(num1);
list1.Add(num2);
list1.Add(num3);
Console.WriteLine($"Min:{list.Min()}");
list1.Sort();
int c = list1.Count()%2==0?list1.Count()+1:list1.Count();
int Med = list1[c/2];
//Console.WriteLine($"Max:{list1.Max()");
I'm on phone so formatting my code is a hard ..
You can skip the creation of three ints and use a
list1.Add(Int.Parse(Console.ReadLine()));
if you wish or for 'n' numbers
bool b = true;
int num=0;
List<int>() l = new List<int>();
Do{
Console.Write("Enter number: ");
b =
int.TryParse(Console.ReadLine(), out num);
l.Add(num);
}While(b);
//insert min, max and median of (List) l here
Smells like homework
int num1 = Convert.ToInt32(Console.ReadLine());
int num2 = Convert.ToInt32(Console.ReadLine());
int num3 = Convert.ToInt32(Console.ReadLine());
List<int> list1 = new List<int>();
list1.Add(num1);
list1.Add(num2);
list1.Add(num3);
Console.WriteLine($"Min:{list.Min()}");
list1.Sort();
int c = list1.Count()%2==0?list1.Count()+1:list1.Count();
int Med = list1[c/2];
//Console.WriteLine($"Max:{list1.Max()");
I'm on phone so formatting my code is a hard ..
You can skip the creation of three ints and use a
list1.Add(Int.Parse(Console.ReadLine()));
if you wish or for 'n' numbers
bool b = true;
int num=0;
List<int>() l = new List<int>();
Do{
Console.Write("Enter number: ");
b =
int.TryParse(Console.ReadLine(), out num);
l.Add(num);
}While(b);
//insert min, max and median of (List) l here
edited Oct 7 '17 at 22:58
answered Oct 7 '17 at 22:27
Daniel LoudonDaniel Loudon
676116
676116
Hey man, Thanks for the answer. It's definitely homework but only our second workshop, we actually haven't had any lessons on it yet. It's a 'find you own solutions' Then be taught how to do it. After a couple hours googling I ended up here. I'll give the above a try and see if I can figure it out, it's important to me that I understand it more than getting the right answer.
– Luke Heald
Oct 7 '17 at 22:29
Hey man I do SSD A level I know the feel I updated answer
– Daniel Loudon
Oct 7 '17 at 22:30
I'm on phone, updating my answer is a bit nifty and I rushed it - keep checking updates
– Daniel Loudon
Oct 7 '17 at 22:34
Thanks again, appreciate the help. Will keep checking back.
– Luke Heald
Oct 7 '17 at 22:39
Edited.. for n numbers
– Daniel Loudon
Oct 7 '17 at 22:45
|
show 4 more comments
Hey man, Thanks for the answer. It's definitely homework but only our second workshop, we actually haven't had any lessons on it yet. It's a 'find you own solutions' Then be taught how to do it. After a couple hours googling I ended up here. I'll give the above a try and see if I can figure it out, it's important to me that I understand it more than getting the right answer.
– Luke Heald
Oct 7 '17 at 22:29
Hey man I do SSD A level I know the feel I updated answer
– Daniel Loudon
Oct 7 '17 at 22:30
I'm on phone, updating my answer is a bit nifty and I rushed it - keep checking updates
– Daniel Loudon
Oct 7 '17 at 22:34
Thanks again, appreciate the help. Will keep checking back.
– Luke Heald
Oct 7 '17 at 22:39
Edited.. for n numbers
– Daniel Loudon
Oct 7 '17 at 22:45
Hey man, Thanks for the answer. It's definitely homework but only our second workshop, we actually haven't had any lessons on it yet. It's a 'find you own solutions' Then be taught how to do it. After a couple hours googling I ended up here. I'll give the above a try and see if I can figure it out, it's important to me that I understand it more than getting the right answer.
– Luke Heald
Oct 7 '17 at 22:29
Hey man, Thanks for the answer. It's definitely homework but only our second workshop, we actually haven't had any lessons on it yet. It's a 'find you own solutions' Then be taught how to do it. After a couple hours googling I ended up here. I'll give the above a try and see if I can figure it out, it's important to me that I understand it more than getting the right answer.
– Luke Heald
Oct 7 '17 at 22:29
Hey man I do SSD A level I know the feel I updated answer
– Daniel Loudon
Oct 7 '17 at 22:30
Hey man I do SSD A level I know the feel I updated answer
– Daniel Loudon
Oct 7 '17 at 22:30
I'm on phone, updating my answer is a bit nifty and I rushed it - keep checking updates
– Daniel Loudon
Oct 7 '17 at 22:34
I'm on phone, updating my answer is a bit nifty and I rushed it - keep checking updates
– Daniel Loudon
Oct 7 '17 at 22:34
Thanks again, appreciate the help. Will keep checking back.
– Luke Heald
Oct 7 '17 at 22:39
Thanks again, appreciate the help. Will keep checking back.
– Luke Heald
Oct 7 '17 at 22:39
Edited.. for n numbers
– Daniel Loudon
Oct 7 '17 at 22:45
Edited.. for n numbers
– Daniel Loudon
Oct 7 '17 at 22:45
|
show 4 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f46625540%2ffind-median-value-of-3-user-inputted-numbers-in-c-sharp-without-output-numbers%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
9EBXtQD2oYTvK,b1XBJ0 VSjMd,XQd7jH,8QLy0CgDyx3MWjsg,xJPUN np0yFA0YjoghOMsP,F7E B,G3