Undefined is not an object (evaluating 'this.props.navigation.navigate') React native
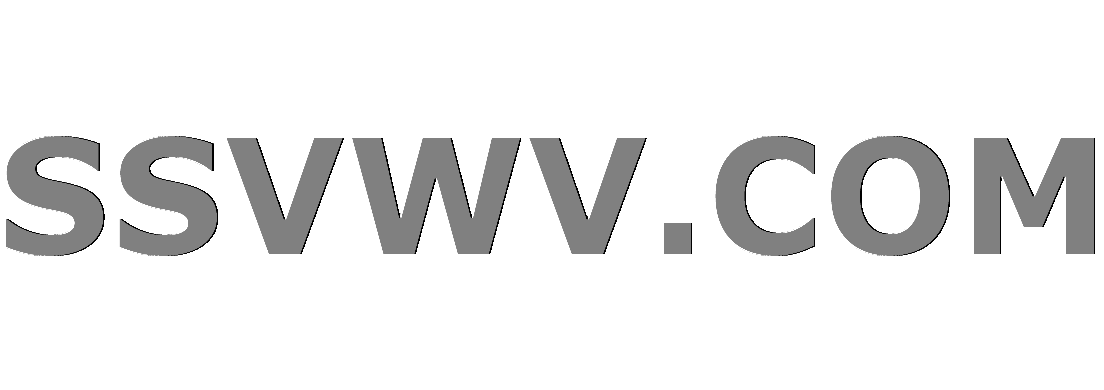
Multi tool use
what I'm trying to do is to custom android back buttom, so when I'm in a screen, when I press the back button, the screen must navigate through the MyProducts
, but I'm receiving this error
import React, { Component } from 'react';
import { View, StyleSheet, Text, Keyboard, Animated } from 'react-native';
import { Textarea, Form, Item, Input, Label, Button } from 'native-base';
import jwt_decode from 'jwt-decode';
class CreateProduct extends Component {
constructor(props) {
super(props);
this.keyboardHeight = new Animated.Value(0);
this.imageHeight = new Animated.Value(199);
this.state = {
isButtonsHidden: false,
title: null,
description: '',
isDialogVisible: false,
messageError: '',
};
}
registerProduct = () => {
const { state } = this.props.navigation;
const token = state.params ? state.params.token : undefined;
const user = jwt_decode(token);
fetch(createProductPath, {
method: 'POST',
body: formData,
headers: {
'Accept': 'application/json',
'Content-Type': 'multipart/form-data',
},
})
.then((response) => {
return response.json();
})
.then((responseJson) => {
if (responseJson.error != null) {
this.setState({ messageError: responseJson.error })
this.setState({ isDialogVisible: true })
}
else {
this.props.navigation.navigate('MyProducts', { token: token });
}
})
}
componentDidMount() {
BackHandler.addEventListener('hardwareBackPress', this.handleBackButton);
}
componentWillUnmount() {
BackHandler.removeEventListener('hardwareBackPress', this.handleBackButton);
}
handleBackButton() {
this.props.navigation.navigate('MyProducts', { token: token });
return true;
}
render() {
const { state } = this.props.navigation;
var token = state.params ? state.params.token : undefined;
return (
<Animated.View
style={[styles.container, { paddingBottom: this.keyboardHeight }]}
>
<Form style={styles.description}>
<Item floatingLabel>
<Label>Title</Label>
<Input
onChangeText={(title) => { this.setState({ title }) }}
/>
</Item>
<Textarea
onChangeText={(description) => { this.setState({ description }) }}
/>
</Form>
<ToogleView hide={this.state.isButtonsHidden}>
<View style={styles.buttonContainer}>
<Button
onPress={() => { this.props.navigation.navigate('MyProducts', { token: token }); }}
danger
>
<Text style={{ color: 'white' }}> CANCEL </Text>
</Button>
<Button
onPress={this.registerProduct}
success
>
<Text style={{ color: 'white' }}> SAVE </Text>
</Button>
</View>
</ToogleView>
</Animated.View>
);
}
}
export default CreateProduct;
const styles = StyleSheet.create({
container: {
backgroundColor: 'white',
flex: 1
},
description: {
flex: 1,
height: '35%',
width: '95%',
},
buttonContainer: {
flexDirection: 'row',
justifyContent: 'space-around',
paddingBottom: 10,
},
});
For reasons unknown to me, my props is undefined when i saw it with a console.log
, so I can't pass throught the
handleBackButton() {
this.props.navigation.navigate('MyProducts', { token: token });
}
Is there a good way to do this? I appreciate.

add a comment |
what I'm trying to do is to custom android back buttom, so when I'm in a screen, when I press the back button, the screen must navigate through the MyProducts
, but I'm receiving this error
import React, { Component } from 'react';
import { View, StyleSheet, Text, Keyboard, Animated } from 'react-native';
import { Textarea, Form, Item, Input, Label, Button } from 'native-base';
import jwt_decode from 'jwt-decode';
class CreateProduct extends Component {
constructor(props) {
super(props);
this.keyboardHeight = new Animated.Value(0);
this.imageHeight = new Animated.Value(199);
this.state = {
isButtonsHidden: false,
title: null,
description: '',
isDialogVisible: false,
messageError: '',
};
}
registerProduct = () => {
const { state } = this.props.navigation;
const token = state.params ? state.params.token : undefined;
const user = jwt_decode(token);
fetch(createProductPath, {
method: 'POST',
body: formData,
headers: {
'Accept': 'application/json',
'Content-Type': 'multipart/form-data',
},
})
.then((response) => {
return response.json();
})
.then((responseJson) => {
if (responseJson.error != null) {
this.setState({ messageError: responseJson.error })
this.setState({ isDialogVisible: true })
}
else {
this.props.navigation.navigate('MyProducts', { token: token });
}
})
}
componentDidMount() {
BackHandler.addEventListener('hardwareBackPress', this.handleBackButton);
}
componentWillUnmount() {
BackHandler.removeEventListener('hardwareBackPress', this.handleBackButton);
}
handleBackButton() {
this.props.navigation.navigate('MyProducts', { token: token });
return true;
}
render() {
const { state } = this.props.navigation;
var token = state.params ? state.params.token : undefined;
return (
<Animated.View
style={[styles.container, { paddingBottom: this.keyboardHeight }]}
>
<Form style={styles.description}>
<Item floatingLabel>
<Label>Title</Label>
<Input
onChangeText={(title) => { this.setState({ title }) }}
/>
</Item>
<Textarea
onChangeText={(description) => { this.setState({ description }) }}
/>
</Form>
<ToogleView hide={this.state.isButtonsHidden}>
<View style={styles.buttonContainer}>
<Button
onPress={() => { this.props.navigation.navigate('MyProducts', { token: token }); }}
danger
>
<Text style={{ color: 'white' }}> CANCEL </Text>
</Button>
<Button
onPress={this.registerProduct}
success
>
<Text style={{ color: 'white' }}> SAVE </Text>
</Button>
</View>
</ToogleView>
</Animated.View>
);
}
}
export default CreateProduct;
const styles = StyleSheet.create({
container: {
backgroundColor: 'white',
flex: 1
},
description: {
flex: 1,
height: '35%',
width: '95%',
},
buttonContainer: {
flexDirection: 'row',
justifyContent: 'space-around',
paddingBottom: 10,
},
});
For reasons unknown to me, my props is undefined when i saw it with a console.log
, so I can't pass throught the
handleBackButton() {
this.props.navigation.navigate('MyProducts', { token: token });
}
Is there a good way to do this? I appreciate.

add a comment |
what I'm trying to do is to custom android back buttom, so when I'm in a screen, when I press the back button, the screen must navigate through the MyProducts
, but I'm receiving this error
import React, { Component } from 'react';
import { View, StyleSheet, Text, Keyboard, Animated } from 'react-native';
import { Textarea, Form, Item, Input, Label, Button } from 'native-base';
import jwt_decode from 'jwt-decode';
class CreateProduct extends Component {
constructor(props) {
super(props);
this.keyboardHeight = new Animated.Value(0);
this.imageHeight = new Animated.Value(199);
this.state = {
isButtonsHidden: false,
title: null,
description: '',
isDialogVisible: false,
messageError: '',
};
}
registerProduct = () => {
const { state } = this.props.navigation;
const token = state.params ? state.params.token : undefined;
const user = jwt_decode(token);
fetch(createProductPath, {
method: 'POST',
body: formData,
headers: {
'Accept': 'application/json',
'Content-Type': 'multipart/form-data',
},
})
.then((response) => {
return response.json();
})
.then((responseJson) => {
if (responseJson.error != null) {
this.setState({ messageError: responseJson.error })
this.setState({ isDialogVisible: true })
}
else {
this.props.navigation.navigate('MyProducts', { token: token });
}
})
}
componentDidMount() {
BackHandler.addEventListener('hardwareBackPress', this.handleBackButton);
}
componentWillUnmount() {
BackHandler.removeEventListener('hardwareBackPress', this.handleBackButton);
}
handleBackButton() {
this.props.navigation.navigate('MyProducts', { token: token });
return true;
}
render() {
const { state } = this.props.navigation;
var token = state.params ? state.params.token : undefined;
return (
<Animated.View
style={[styles.container, { paddingBottom: this.keyboardHeight }]}
>
<Form style={styles.description}>
<Item floatingLabel>
<Label>Title</Label>
<Input
onChangeText={(title) => { this.setState({ title }) }}
/>
</Item>
<Textarea
onChangeText={(description) => { this.setState({ description }) }}
/>
</Form>
<ToogleView hide={this.state.isButtonsHidden}>
<View style={styles.buttonContainer}>
<Button
onPress={() => { this.props.navigation.navigate('MyProducts', { token: token }); }}
danger
>
<Text style={{ color: 'white' }}> CANCEL </Text>
</Button>
<Button
onPress={this.registerProduct}
success
>
<Text style={{ color: 'white' }}> SAVE </Text>
</Button>
</View>
</ToogleView>
</Animated.View>
);
}
}
export default CreateProduct;
const styles = StyleSheet.create({
container: {
backgroundColor: 'white',
flex: 1
},
description: {
flex: 1,
height: '35%',
width: '95%',
},
buttonContainer: {
flexDirection: 'row',
justifyContent: 'space-around',
paddingBottom: 10,
},
});
For reasons unknown to me, my props is undefined when i saw it with a console.log
, so I can't pass throught the
handleBackButton() {
this.props.navigation.navigate('MyProducts', { token: token });
}
Is there a good way to do this? I appreciate.

what I'm trying to do is to custom android back buttom, so when I'm in a screen, when I press the back button, the screen must navigate through the MyProducts
, but I'm receiving this error
import React, { Component } from 'react';
import { View, StyleSheet, Text, Keyboard, Animated } from 'react-native';
import { Textarea, Form, Item, Input, Label, Button } from 'native-base';
import jwt_decode from 'jwt-decode';
class CreateProduct extends Component {
constructor(props) {
super(props);
this.keyboardHeight = new Animated.Value(0);
this.imageHeight = new Animated.Value(199);
this.state = {
isButtonsHidden: false,
title: null,
description: '',
isDialogVisible: false,
messageError: '',
};
}
registerProduct = () => {
const { state } = this.props.navigation;
const token = state.params ? state.params.token : undefined;
const user = jwt_decode(token);
fetch(createProductPath, {
method: 'POST',
body: formData,
headers: {
'Accept': 'application/json',
'Content-Type': 'multipart/form-data',
},
})
.then((response) => {
return response.json();
})
.then((responseJson) => {
if (responseJson.error != null) {
this.setState({ messageError: responseJson.error })
this.setState({ isDialogVisible: true })
}
else {
this.props.navigation.navigate('MyProducts', { token: token });
}
})
}
componentDidMount() {
BackHandler.addEventListener('hardwareBackPress', this.handleBackButton);
}
componentWillUnmount() {
BackHandler.removeEventListener('hardwareBackPress', this.handleBackButton);
}
handleBackButton() {
this.props.navigation.navigate('MyProducts', { token: token });
return true;
}
render() {
const { state } = this.props.navigation;
var token = state.params ? state.params.token : undefined;
return (
<Animated.View
style={[styles.container, { paddingBottom: this.keyboardHeight }]}
>
<Form style={styles.description}>
<Item floatingLabel>
<Label>Title</Label>
<Input
onChangeText={(title) => { this.setState({ title }) }}
/>
</Item>
<Textarea
onChangeText={(description) => { this.setState({ description }) }}
/>
</Form>
<ToogleView hide={this.state.isButtonsHidden}>
<View style={styles.buttonContainer}>
<Button
onPress={() => { this.props.navigation.navigate('MyProducts', { token: token }); }}
danger
>
<Text style={{ color: 'white' }}> CANCEL </Text>
</Button>
<Button
onPress={this.registerProduct}
success
>
<Text style={{ color: 'white' }}> SAVE </Text>
</Button>
</View>
</ToogleView>
</Animated.View>
);
}
}
export default CreateProduct;
const styles = StyleSheet.create({
container: {
backgroundColor: 'white',
flex: 1
},
description: {
flex: 1,
height: '35%',
width: '95%',
},
buttonContainer: {
flexDirection: 'row',
justifyContent: 'space-around',
paddingBottom: 10,
},
});
For reasons unknown to me, my props is undefined when i saw it with a console.log
, so I can't pass throught the
handleBackButton() {
this.props.navigation.navigate('MyProducts', { token: token });
}
Is there a good way to do this? I appreciate.


edited Nov 24 '18 at 16:51
yoongi_s
asked Nov 23 '18 at 4:37
yoongi_syoongi_s
74
74
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
Make handleBackButton function as arrow function
handleBackButton = () => {
this.props.navigation.navigate('MyProducts', { token: token });
return true
}
add a comment |
Can you try changing your calls to handleBackButton to bind to this?
componentDidMount() {
BackHandler.addEventListener('hardwareBackPress', this.handleBackButton.bind(this));
}
componentWillUnmount() {
BackHandler.removeEventListener('hardwareBackPress', this.handleBackButton.bind(this));
}
handleBackButton() {
this.props.navigation.navigate('MyProducts', { token: token });
return true;
}
Yes, I've tried, but the error still appears. @Abinesh answer solved it. Thanks anyway :)
– yoongi_s
Nov 25 '18 at 19:17
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53440715%2fundefined-is-not-an-object-evaluating-this-props-navigation-navigate-react-n%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Make handleBackButton function as arrow function
handleBackButton = () => {
this.props.navigation.navigate('MyProducts', { token: token });
return true
}
add a comment |
Make handleBackButton function as arrow function
handleBackButton = () => {
this.props.navigation.navigate('MyProducts', { token: token });
return true
}
add a comment |
Make handleBackButton function as arrow function
handleBackButton = () => {
this.props.navigation.navigate('MyProducts', { token: token });
return true
}
Make handleBackButton function as arrow function
handleBackButton = () => {
this.props.navigation.navigate('MyProducts', { token: token });
return true
}
answered Nov 23 '18 at 4:42


Abinesh JoyelAbinesh Joyel
1,0621511
1,0621511
add a comment |
add a comment |
Can you try changing your calls to handleBackButton to bind to this?
componentDidMount() {
BackHandler.addEventListener('hardwareBackPress', this.handleBackButton.bind(this));
}
componentWillUnmount() {
BackHandler.removeEventListener('hardwareBackPress', this.handleBackButton.bind(this));
}
handleBackButton() {
this.props.navigation.navigate('MyProducts', { token: token });
return true;
}
Yes, I've tried, but the error still appears. @Abinesh answer solved it. Thanks anyway :)
– yoongi_s
Nov 25 '18 at 19:17
add a comment |
Can you try changing your calls to handleBackButton to bind to this?
componentDidMount() {
BackHandler.addEventListener('hardwareBackPress', this.handleBackButton.bind(this));
}
componentWillUnmount() {
BackHandler.removeEventListener('hardwareBackPress', this.handleBackButton.bind(this));
}
handleBackButton() {
this.props.navigation.navigate('MyProducts', { token: token });
return true;
}
Yes, I've tried, but the error still appears. @Abinesh answer solved it. Thanks anyway :)
– yoongi_s
Nov 25 '18 at 19:17
add a comment |
Can you try changing your calls to handleBackButton to bind to this?
componentDidMount() {
BackHandler.addEventListener('hardwareBackPress', this.handleBackButton.bind(this));
}
componentWillUnmount() {
BackHandler.removeEventListener('hardwareBackPress', this.handleBackButton.bind(this));
}
handleBackButton() {
this.props.navigation.navigate('MyProducts', { token: token });
return true;
}
Can you try changing your calls to handleBackButton to bind to this?
componentDidMount() {
BackHandler.addEventListener('hardwareBackPress', this.handleBackButton.bind(this));
}
componentWillUnmount() {
BackHandler.removeEventListener('hardwareBackPress', this.handleBackButton.bind(this));
}
handleBackButton() {
this.props.navigation.navigate('MyProducts', { token: token });
return true;
}
answered Nov 24 '18 at 17:56


Kevin van ZylKevin van Zyl
107210
107210
Yes, I've tried, but the error still appears. @Abinesh answer solved it. Thanks anyway :)
– yoongi_s
Nov 25 '18 at 19:17
add a comment |
Yes, I've tried, but the error still appears. @Abinesh answer solved it. Thanks anyway :)
– yoongi_s
Nov 25 '18 at 19:17
Yes, I've tried, but the error still appears. @Abinesh answer solved it. Thanks anyway :)
– yoongi_s
Nov 25 '18 at 19:17
Yes, I've tried, but the error still appears. @Abinesh answer solved it. Thanks anyway :)
– yoongi_s
Nov 25 '18 at 19:17
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53440715%2fundefined-is-not-an-object-evaluating-this-props-navigation-navigate-react-n%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
K,9 L3vzsXWTvZ5rfl29ZDDaeI0V