How to insert data into a database using jQuery / Ajax in ASP.NET MVC?
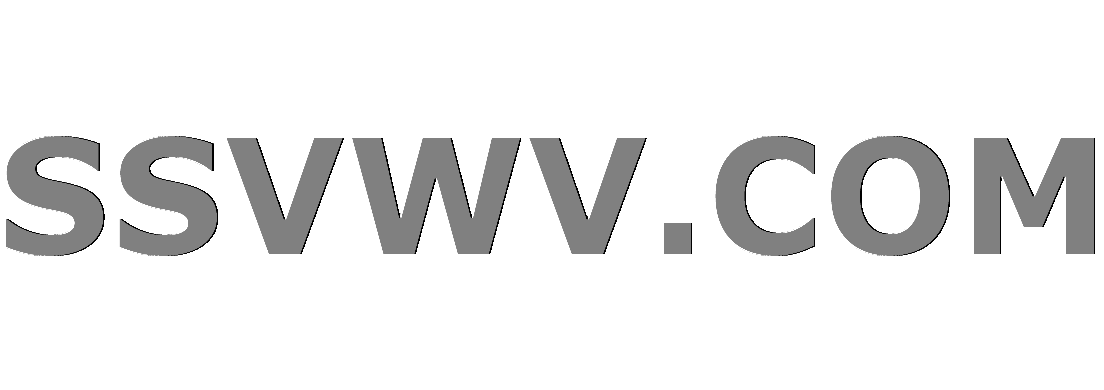
Multi tool use
I have been stuck for hours trying to find the bugs when trying to insert data into database using ajax. When I click submit button, it just reloads but does not save. I am confused about this problem. I have searched many blogs but did not find accurate solution to this problem.
Model class
public class Semester
{
[Key]
public int SemesterId { get; set; }
[Display(Name = "Semester Code")]
[Required(ErrorMessage = "please enter semester code")]
public string SemesterCode { get; set; }
[Display(Name = "Semester Name")]
[Required(ErrorMessage = "Please enter semeter name")]
public string SemesterName { get; set; }
}
Controller
[HttpGet]
public ActionResult SaveSemesterGet()
{
return View();
}
public JsonResult AsCreateSemester(Semester semester){
db.Semesters.Add(semester);
db.SaveChanges();
return Json(semester, JsonRequestBehavior.AllowGet);
}
Index view:
@model CampusManagementApp.Models.Semester
<h2>SaveSemesterGet</h2>
<form method="POST">
@Html.AntiForgeryToken()
<div >
<h4>Semester</h4>
@Html.ValidationSummary(true)
<div class="form-group">
@Html.LabelFor(model => model.SemesterCode, new { @class = "control-
label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.SemesterCode)
@Html.ValidationMessageFor(model => model.SemesterCode)
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.SemesterName, new { @class = "control-
label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.SemesterName)
@Html.ValidationMessageFor(model => model.SemesterName)
</div>
</div>
<div class="form-group">
<div class="col-md-offset-2 col-md-10">
<input type="submit" id="btn" value="SAVE"/>
</div>
</div>
</div>
<div>
@* @Html.ActionLink("Back to List", "Index")*@
</div>
@section Scripts {
@Scripts.Render("~/bundles/jqueryval")
}
<script src="~/Scripts/jquery-1.10.2.min.js"></script>
<script src="~/Scripts/jquery.validate.min.js"></script>
<script src="~/Scripts/jquery.validate.unobtrusive.min.js"></script>
<script>
$(document).ready(function() {
$("#btn").click(function(a) {
a.preventDefault();
var code = $("#SemesterCode").val();
var name = $("#SemesterName").val();
var jsonData = {
SemesterCode: code,
SemesterName: name
};
$.ajax({
type: "POST",
url: '@Url.Action("AsCreateSemester", "Semester")',
data: JSON.stringify(jsonData),
dataType:"json",
success:function(data) {
alert(data.SemesterName+"successfully inserted");
},
failure: function () {
alert("not successfull");
}
});
</script>
What can be done to get rid of this problem?
c# jquery ajax asp.net-mvc
|
show 2 more comments
I have been stuck for hours trying to find the bugs when trying to insert data into database using ajax. When I click submit button, it just reloads but does not save. I am confused about this problem. I have searched many blogs but did not find accurate solution to this problem.
Model class
public class Semester
{
[Key]
public int SemesterId { get; set; }
[Display(Name = "Semester Code")]
[Required(ErrorMessage = "please enter semester code")]
public string SemesterCode { get; set; }
[Display(Name = "Semester Name")]
[Required(ErrorMessage = "Please enter semeter name")]
public string SemesterName { get; set; }
}
Controller
[HttpGet]
public ActionResult SaveSemesterGet()
{
return View();
}
public JsonResult AsCreateSemester(Semester semester){
db.Semesters.Add(semester);
db.SaveChanges();
return Json(semester, JsonRequestBehavior.AllowGet);
}
Index view:
@model CampusManagementApp.Models.Semester
<h2>SaveSemesterGet</h2>
<form method="POST">
@Html.AntiForgeryToken()
<div >
<h4>Semester</h4>
@Html.ValidationSummary(true)
<div class="form-group">
@Html.LabelFor(model => model.SemesterCode, new { @class = "control-
label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.SemesterCode)
@Html.ValidationMessageFor(model => model.SemesterCode)
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.SemesterName, new { @class = "control-
label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.SemesterName)
@Html.ValidationMessageFor(model => model.SemesterName)
</div>
</div>
<div class="form-group">
<div class="col-md-offset-2 col-md-10">
<input type="submit" id="btn" value="SAVE"/>
</div>
</div>
</div>
<div>
@* @Html.ActionLink("Back to List", "Index")*@
</div>
@section Scripts {
@Scripts.Render("~/bundles/jqueryval")
}
<script src="~/Scripts/jquery-1.10.2.min.js"></script>
<script src="~/Scripts/jquery.validate.min.js"></script>
<script src="~/Scripts/jquery.validate.unobtrusive.min.js"></script>
<script>
$(document).ready(function() {
$("#btn").click(function(a) {
a.preventDefault();
var code = $("#SemesterCode").val();
var name = $("#SemesterName").val();
var jsonData = {
SemesterCode: code,
SemesterName: name
};
$.ajax({
type: "POST",
url: '@Url.Action("AsCreateSemester", "Semester")',
data: JSON.stringify(jsonData),
dataType:"json",
success:function(data) {
alert(data.SemesterName+"successfully inserted");
},
failure: function () {
alert("not successfull");
}
});
</script>
What can be done to get rid of this problem?
c# jquery ajax asp.net-mvc
Add the attributeHttpPost
to the action you are using to post data.
– Llazar
Nov 25 '18 at 20:12
You are executing the ajax call onload of window which happens before a user has a chance to interact with the page. Move that javascript to a function that you can call when the button is clicked.
– Crowcoder
Nov 25 '18 at 21:41
not clear about your commnet ,what can be done @crowcoder
– Talukder
Nov 25 '18 at 21:51
Disregard, I missed the part where you wired the click event. Did you try dougF's answer?
– Crowcoder
Nov 25 '18 at 22:03
with regards,it dougF's answer does not work
– Talukder
Nov 25 '18 at 22:47
|
show 2 more comments
I have been stuck for hours trying to find the bugs when trying to insert data into database using ajax. When I click submit button, it just reloads but does not save. I am confused about this problem. I have searched many blogs but did not find accurate solution to this problem.
Model class
public class Semester
{
[Key]
public int SemesterId { get; set; }
[Display(Name = "Semester Code")]
[Required(ErrorMessage = "please enter semester code")]
public string SemesterCode { get; set; }
[Display(Name = "Semester Name")]
[Required(ErrorMessage = "Please enter semeter name")]
public string SemesterName { get; set; }
}
Controller
[HttpGet]
public ActionResult SaveSemesterGet()
{
return View();
}
public JsonResult AsCreateSemester(Semester semester){
db.Semesters.Add(semester);
db.SaveChanges();
return Json(semester, JsonRequestBehavior.AllowGet);
}
Index view:
@model CampusManagementApp.Models.Semester
<h2>SaveSemesterGet</h2>
<form method="POST">
@Html.AntiForgeryToken()
<div >
<h4>Semester</h4>
@Html.ValidationSummary(true)
<div class="form-group">
@Html.LabelFor(model => model.SemesterCode, new { @class = "control-
label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.SemesterCode)
@Html.ValidationMessageFor(model => model.SemesterCode)
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.SemesterName, new { @class = "control-
label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.SemesterName)
@Html.ValidationMessageFor(model => model.SemesterName)
</div>
</div>
<div class="form-group">
<div class="col-md-offset-2 col-md-10">
<input type="submit" id="btn" value="SAVE"/>
</div>
</div>
</div>
<div>
@* @Html.ActionLink("Back to List", "Index")*@
</div>
@section Scripts {
@Scripts.Render("~/bundles/jqueryval")
}
<script src="~/Scripts/jquery-1.10.2.min.js"></script>
<script src="~/Scripts/jquery.validate.min.js"></script>
<script src="~/Scripts/jquery.validate.unobtrusive.min.js"></script>
<script>
$(document).ready(function() {
$("#btn").click(function(a) {
a.preventDefault();
var code = $("#SemesterCode").val();
var name = $("#SemesterName").val();
var jsonData = {
SemesterCode: code,
SemesterName: name
};
$.ajax({
type: "POST",
url: '@Url.Action("AsCreateSemester", "Semester")',
data: JSON.stringify(jsonData),
dataType:"json",
success:function(data) {
alert(data.SemesterName+"successfully inserted");
},
failure: function () {
alert("not successfull");
}
});
</script>
What can be done to get rid of this problem?
c# jquery ajax asp.net-mvc
I have been stuck for hours trying to find the bugs when trying to insert data into database using ajax. When I click submit button, it just reloads but does not save. I am confused about this problem. I have searched many blogs but did not find accurate solution to this problem.
Model class
public class Semester
{
[Key]
public int SemesterId { get; set; }
[Display(Name = "Semester Code")]
[Required(ErrorMessage = "please enter semester code")]
public string SemesterCode { get; set; }
[Display(Name = "Semester Name")]
[Required(ErrorMessage = "Please enter semeter name")]
public string SemesterName { get; set; }
}
Controller
[HttpGet]
public ActionResult SaveSemesterGet()
{
return View();
}
public JsonResult AsCreateSemester(Semester semester){
db.Semesters.Add(semester);
db.SaveChanges();
return Json(semester, JsonRequestBehavior.AllowGet);
}
Index view:
@model CampusManagementApp.Models.Semester
<h2>SaveSemesterGet</h2>
<form method="POST">
@Html.AntiForgeryToken()
<div >
<h4>Semester</h4>
@Html.ValidationSummary(true)
<div class="form-group">
@Html.LabelFor(model => model.SemesterCode, new { @class = "control-
label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.SemesterCode)
@Html.ValidationMessageFor(model => model.SemesterCode)
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.SemesterName, new { @class = "control-
label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.SemesterName)
@Html.ValidationMessageFor(model => model.SemesterName)
</div>
</div>
<div class="form-group">
<div class="col-md-offset-2 col-md-10">
<input type="submit" id="btn" value="SAVE"/>
</div>
</div>
</div>
<div>
@* @Html.ActionLink("Back to List", "Index")*@
</div>
@section Scripts {
@Scripts.Render("~/bundles/jqueryval")
}
<script src="~/Scripts/jquery-1.10.2.min.js"></script>
<script src="~/Scripts/jquery.validate.min.js"></script>
<script src="~/Scripts/jquery.validate.unobtrusive.min.js"></script>
<script>
$(document).ready(function() {
$("#btn").click(function(a) {
a.preventDefault();
var code = $("#SemesterCode").val();
var name = $("#SemesterName").val();
var jsonData = {
SemesterCode: code,
SemesterName: name
};
$.ajax({
type: "POST",
url: '@Url.Action("AsCreateSemester", "Semester")',
data: JSON.stringify(jsonData),
dataType:"json",
success:function(data) {
alert(data.SemesterName+"successfully inserted");
},
failure: function () {
alert("not successfull");
}
});
</script>
What can be done to get rid of this problem?
c# jquery ajax asp.net-mvc
c# jquery ajax asp.net-mvc
edited Nov 27 '18 at 8:50
Talukder
asked Nov 25 '18 at 20:07
TalukderTalukder
267
267
Add the attributeHttpPost
to the action you are using to post data.
– Llazar
Nov 25 '18 at 20:12
You are executing the ajax call onload of window which happens before a user has a chance to interact with the page. Move that javascript to a function that you can call when the button is clicked.
– Crowcoder
Nov 25 '18 at 21:41
not clear about your commnet ,what can be done @crowcoder
– Talukder
Nov 25 '18 at 21:51
Disregard, I missed the part where you wired the click event. Did you try dougF's answer?
– Crowcoder
Nov 25 '18 at 22:03
with regards,it dougF's answer does not work
– Talukder
Nov 25 '18 at 22:47
|
show 2 more comments
Add the attributeHttpPost
to the action you are using to post data.
– Llazar
Nov 25 '18 at 20:12
You are executing the ajax call onload of window which happens before a user has a chance to interact with the page. Move that javascript to a function that you can call when the button is clicked.
– Crowcoder
Nov 25 '18 at 21:41
not clear about your commnet ,what can be done @crowcoder
– Talukder
Nov 25 '18 at 21:51
Disregard, I missed the part where you wired the click event. Did you try dougF's answer?
– Crowcoder
Nov 25 '18 at 22:03
with regards,it dougF's answer does not work
– Talukder
Nov 25 '18 at 22:47
Add the attribute
HttpPost
to the action you are using to post data.– Llazar
Nov 25 '18 at 20:12
Add the attribute
HttpPost
to the action you are using to post data.– Llazar
Nov 25 '18 at 20:12
You are executing the ajax call onload of window which happens before a user has a chance to interact with the page. Move that javascript to a function that you can call when the button is clicked.
– Crowcoder
Nov 25 '18 at 21:41
You are executing the ajax call onload of window which happens before a user has a chance to interact with the page. Move that javascript to a function that you can call when the button is clicked.
– Crowcoder
Nov 25 '18 at 21:41
not clear about your commnet ,what can be done @crowcoder
– Talukder
Nov 25 '18 at 21:51
not clear about your commnet ,what can be done @crowcoder
– Talukder
Nov 25 '18 at 21:51
Disregard, I missed the part where you wired the click event. Did you try dougF's answer?
– Crowcoder
Nov 25 '18 at 22:03
Disregard, I missed the part where you wired the click event. Did you try dougF's answer?
– Crowcoder
Nov 25 '18 at 22:03
with regards,it dougF's answer does not work
– Talukder
Nov 25 '18 at 22:47
with regards,it dougF's answer does not work
– Talukder
Nov 25 '18 at 22:47
|
show 2 more comments
3 Answers
3
active
oldest
votes
Why are you using a submit button? Since the JS function you made is handling the post, you don't need to use a submit button, which is causing the entire page to reload. Just use a normal button.
<input type="button" id="btn" value="SAVE"/>
chanded the input type but it doees not respond and save the data.
– Talukder
Nov 25 '18 at 22:46
When you debug, is the JS being fired at all?
– Doug F
Nov 25 '18 at 22:48
@Talukder if the JS isn't being fired when you debug, you might also have to change how you wired up the button. Instead of $("#btn").click(function() { maybe use $("#btn").on("click", function() { just in case for some reason the jquery isn't finding the button on page load.
– Doug F
Nov 25 '18 at 22:55
add a comment |
You have several issues in the view:
1) You're subscribed to click
event of a submit button without preventing default action which submitting the form. This triggered POST action which redirect away into the controller action and reload the page without leaving any time for AJAX function to execute. You should use preventDefault()
to cancel the default action of the button.
2) The controller which receives AJAX callback does not have [HttpPost]
attribute while type: "POST"
option is used. Consider adding [HttpPost]
attribute on top of controller name.
3) Your AJAX property names list does not match with property names defined inside model class. Consider using a viewmodel which accepts two properties and use it as action parameter.
Therefore, the AJAX callback should have setup as in example below:
Viewmodel
public class SemesterVM
{
public string SemesterCode { get; set; }
public string SemesterName { get; set; }
}
View
<input type="button" id="btn" value="SAVE" />
JS (click event)
$('#btn').click(function (e) {
e.preventDefault(); // prevent default submit
var code = $("#SemesterCode").val();
var name = $("#SemesterName").val();
// all property names must be same as the viewmodel has
var jsonData = {
SemesterCode: code,
SemesterName: name
};
$.ajax({
type: "POST",
url: '@Url.Action("SaveSemester", "Student")',
contentType: "application/json;charset=utf-8",
data: JSON.stringify(jsonData),
dataType: "json",
success: function() {
// do something
}
});
});
Controller Action
[HttpPost]
public JsonResult SaveSemester(SemesterVM semester)
{
// add semester data
return Json(semester);
}
Being updated code as above it saves null value in the database.
– Talukder
Nov 27 '18 at 8:38
UsingModelState.IsValid
doesn't make sense in AJAX callback. Also you're not includingHttpPost
attribute - this makes your AJAX call never reach the controller action which usesGET
.
– Tetsuya Yamamoto
Nov 27 '18 at 8:44
since I have used request behavior.allow get ,it is necessary to add [httppost],i have seen many articles not use [httppost ] at the Json request, and modelstate is eliminated. Tetsuya Yamamoto
– Talukder
Nov 27 '18 at 8:49
By default JSON data usesPOST
, if you want to allowGET
operation then you need to addJsonRequestBehavior.AllowGet
. Related: Why is JsonRequestBehavior needed?.
– Tetsuya Yamamoto
Nov 27 '18 at 8:53
i have run program as You say but it also save null values,is it occurs problem in ajax method? - Tetsuya Yamamoto
– Talukder
Nov 27 '18 at 9:12
|
show 2 more comments
After changing the ajax() method like below it works.
$.ajax({
type: "POST",
url: '@Url.Action("AsCreateSemester", "Semester")',
data: {
SemesterCode: code,
SemesterName: name
},
success:function(data) {
alert(data.SemesterName+"successfully inserted");
},
failure: function () {
alert("not successfull");
}
});
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53471427%2fhow-to-insert-data-into-a-database-using-jquery-ajax-in-asp-net-mvc%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
Why are you using a submit button? Since the JS function you made is handling the post, you don't need to use a submit button, which is causing the entire page to reload. Just use a normal button.
<input type="button" id="btn" value="SAVE"/>
chanded the input type but it doees not respond and save the data.
– Talukder
Nov 25 '18 at 22:46
When you debug, is the JS being fired at all?
– Doug F
Nov 25 '18 at 22:48
@Talukder if the JS isn't being fired when you debug, you might also have to change how you wired up the button. Instead of $("#btn").click(function() { maybe use $("#btn").on("click", function() { just in case for some reason the jquery isn't finding the button on page load.
– Doug F
Nov 25 '18 at 22:55
add a comment |
Why are you using a submit button? Since the JS function you made is handling the post, you don't need to use a submit button, which is causing the entire page to reload. Just use a normal button.
<input type="button" id="btn" value="SAVE"/>
chanded the input type but it doees not respond and save the data.
– Talukder
Nov 25 '18 at 22:46
When you debug, is the JS being fired at all?
– Doug F
Nov 25 '18 at 22:48
@Talukder if the JS isn't being fired when you debug, you might also have to change how you wired up the button. Instead of $("#btn").click(function() { maybe use $("#btn").on("click", function() { just in case for some reason the jquery isn't finding the button on page load.
– Doug F
Nov 25 '18 at 22:55
add a comment |
Why are you using a submit button? Since the JS function you made is handling the post, you don't need to use a submit button, which is causing the entire page to reload. Just use a normal button.
<input type="button" id="btn" value="SAVE"/>
Why are you using a submit button? Since the JS function you made is handling the post, you don't need to use a submit button, which is causing the entire page to reload. Just use a normal button.
<input type="button" id="btn" value="SAVE"/>
answered Nov 25 '18 at 20:15


Doug FDoug F
7391717
7391717
chanded the input type but it doees not respond and save the data.
– Talukder
Nov 25 '18 at 22:46
When you debug, is the JS being fired at all?
– Doug F
Nov 25 '18 at 22:48
@Talukder if the JS isn't being fired when you debug, you might also have to change how you wired up the button. Instead of $("#btn").click(function() { maybe use $("#btn").on("click", function() { just in case for some reason the jquery isn't finding the button on page load.
– Doug F
Nov 25 '18 at 22:55
add a comment |
chanded the input type but it doees not respond and save the data.
– Talukder
Nov 25 '18 at 22:46
When you debug, is the JS being fired at all?
– Doug F
Nov 25 '18 at 22:48
@Talukder if the JS isn't being fired when you debug, you might also have to change how you wired up the button. Instead of $("#btn").click(function() { maybe use $("#btn").on("click", function() { just in case for some reason the jquery isn't finding the button on page load.
– Doug F
Nov 25 '18 at 22:55
chanded the input type but it doees not respond and save the data.
– Talukder
Nov 25 '18 at 22:46
chanded the input type but it doees not respond and save the data.
– Talukder
Nov 25 '18 at 22:46
When you debug, is the JS being fired at all?
– Doug F
Nov 25 '18 at 22:48
When you debug, is the JS being fired at all?
– Doug F
Nov 25 '18 at 22:48
@Talukder if the JS isn't being fired when you debug, you might also have to change how you wired up the button. Instead of $("#btn").click(function() { maybe use $("#btn").on("click", function() { just in case for some reason the jquery isn't finding the button on page load.
– Doug F
Nov 25 '18 at 22:55
@Talukder if the JS isn't being fired when you debug, you might also have to change how you wired up the button. Instead of $("#btn").click(function() { maybe use $("#btn").on("click", function() { just in case for some reason the jquery isn't finding the button on page load.
– Doug F
Nov 25 '18 at 22:55
add a comment |
You have several issues in the view:
1) You're subscribed to click
event of a submit button without preventing default action which submitting the form. This triggered POST action which redirect away into the controller action and reload the page without leaving any time for AJAX function to execute. You should use preventDefault()
to cancel the default action of the button.
2) The controller which receives AJAX callback does not have [HttpPost]
attribute while type: "POST"
option is used. Consider adding [HttpPost]
attribute on top of controller name.
3) Your AJAX property names list does not match with property names defined inside model class. Consider using a viewmodel which accepts two properties and use it as action parameter.
Therefore, the AJAX callback should have setup as in example below:
Viewmodel
public class SemesterVM
{
public string SemesterCode { get; set; }
public string SemesterName { get; set; }
}
View
<input type="button" id="btn" value="SAVE" />
JS (click event)
$('#btn').click(function (e) {
e.preventDefault(); // prevent default submit
var code = $("#SemesterCode").val();
var name = $("#SemesterName").val();
// all property names must be same as the viewmodel has
var jsonData = {
SemesterCode: code,
SemesterName: name
};
$.ajax({
type: "POST",
url: '@Url.Action("SaveSemester", "Student")',
contentType: "application/json;charset=utf-8",
data: JSON.stringify(jsonData),
dataType: "json",
success: function() {
// do something
}
});
});
Controller Action
[HttpPost]
public JsonResult SaveSemester(SemesterVM semester)
{
// add semester data
return Json(semester);
}
Being updated code as above it saves null value in the database.
– Talukder
Nov 27 '18 at 8:38
UsingModelState.IsValid
doesn't make sense in AJAX callback. Also you're not includingHttpPost
attribute - this makes your AJAX call never reach the controller action which usesGET
.
– Tetsuya Yamamoto
Nov 27 '18 at 8:44
since I have used request behavior.allow get ,it is necessary to add [httppost],i have seen many articles not use [httppost ] at the Json request, and modelstate is eliminated. Tetsuya Yamamoto
– Talukder
Nov 27 '18 at 8:49
By default JSON data usesPOST
, if you want to allowGET
operation then you need to addJsonRequestBehavior.AllowGet
. Related: Why is JsonRequestBehavior needed?.
– Tetsuya Yamamoto
Nov 27 '18 at 8:53
i have run program as You say but it also save null values,is it occurs problem in ajax method? - Tetsuya Yamamoto
– Talukder
Nov 27 '18 at 9:12
|
show 2 more comments
You have several issues in the view:
1) You're subscribed to click
event of a submit button without preventing default action which submitting the form. This triggered POST action which redirect away into the controller action and reload the page without leaving any time for AJAX function to execute. You should use preventDefault()
to cancel the default action of the button.
2) The controller which receives AJAX callback does not have [HttpPost]
attribute while type: "POST"
option is used. Consider adding [HttpPost]
attribute on top of controller name.
3) Your AJAX property names list does not match with property names defined inside model class. Consider using a viewmodel which accepts two properties and use it as action parameter.
Therefore, the AJAX callback should have setup as in example below:
Viewmodel
public class SemesterVM
{
public string SemesterCode { get; set; }
public string SemesterName { get; set; }
}
View
<input type="button" id="btn" value="SAVE" />
JS (click event)
$('#btn').click(function (e) {
e.preventDefault(); // prevent default submit
var code = $("#SemesterCode").val();
var name = $("#SemesterName").val();
// all property names must be same as the viewmodel has
var jsonData = {
SemesterCode: code,
SemesterName: name
};
$.ajax({
type: "POST",
url: '@Url.Action("SaveSemester", "Student")',
contentType: "application/json;charset=utf-8",
data: JSON.stringify(jsonData),
dataType: "json",
success: function() {
// do something
}
});
});
Controller Action
[HttpPost]
public JsonResult SaveSemester(SemesterVM semester)
{
// add semester data
return Json(semester);
}
Being updated code as above it saves null value in the database.
– Talukder
Nov 27 '18 at 8:38
UsingModelState.IsValid
doesn't make sense in AJAX callback. Also you're not includingHttpPost
attribute - this makes your AJAX call never reach the controller action which usesGET
.
– Tetsuya Yamamoto
Nov 27 '18 at 8:44
since I have used request behavior.allow get ,it is necessary to add [httppost],i have seen many articles not use [httppost ] at the Json request, and modelstate is eliminated. Tetsuya Yamamoto
– Talukder
Nov 27 '18 at 8:49
By default JSON data usesPOST
, if you want to allowGET
operation then you need to addJsonRequestBehavior.AllowGet
. Related: Why is JsonRequestBehavior needed?.
– Tetsuya Yamamoto
Nov 27 '18 at 8:53
i have run program as You say but it also save null values,is it occurs problem in ajax method? - Tetsuya Yamamoto
– Talukder
Nov 27 '18 at 9:12
|
show 2 more comments
You have several issues in the view:
1) You're subscribed to click
event of a submit button without preventing default action which submitting the form. This triggered POST action which redirect away into the controller action and reload the page without leaving any time for AJAX function to execute. You should use preventDefault()
to cancel the default action of the button.
2) The controller which receives AJAX callback does not have [HttpPost]
attribute while type: "POST"
option is used. Consider adding [HttpPost]
attribute on top of controller name.
3) Your AJAX property names list does not match with property names defined inside model class. Consider using a viewmodel which accepts two properties and use it as action parameter.
Therefore, the AJAX callback should have setup as in example below:
Viewmodel
public class SemesterVM
{
public string SemesterCode { get; set; }
public string SemesterName { get; set; }
}
View
<input type="button" id="btn" value="SAVE" />
JS (click event)
$('#btn').click(function (e) {
e.preventDefault(); // prevent default submit
var code = $("#SemesterCode").val();
var name = $("#SemesterName").val();
// all property names must be same as the viewmodel has
var jsonData = {
SemesterCode: code,
SemesterName: name
};
$.ajax({
type: "POST",
url: '@Url.Action("SaveSemester", "Student")',
contentType: "application/json;charset=utf-8",
data: JSON.stringify(jsonData),
dataType: "json",
success: function() {
// do something
}
});
});
Controller Action
[HttpPost]
public JsonResult SaveSemester(SemesterVM semester)
{
// add semester data
return Json(semester);
}
You have several issues in the view:
1) You're subscribed to click
event of a submit button without preventing default action which submitting the form. This triggered POST action which redirect away into the controller action and reload the page without leaving any time for AJAX function to execute. You should use preventDefault()
to cancel the default action of the button.
2) The controller which receives AJAX callback does not have [HttpPost]
attribute while type: "POST"
option is used. Consider adding [HttpPost]
attribute on top of controller name.
3) Your AJAX property names list does not match with property names defined inside model class. Consider using a viewmodel which accepts two properties and use it as action parameter.
Therefore, the AJAX callback should have setup as in example below:
Viewmodel
public class SemesterVM
{
public string SemesterCode { get; set; }
public string SemesterName { get; set; }
}
View
<input type="button" id="btn" value="SAVE" />
JS (click event)
$('#btn').click(function (e) {
e.preventDefault(); // prevent default submit
var code = $("#SemesterCode").val();
var name = $("#SemesterName").val();
// all property names must be same as the viewmodel has
var jsonData = {
SemesterCode: code,
SemesterName: name
};
$.ajax({
type: "POST",
url: '@Url.Action("SaveSemester", "Student")',
contentType: "application/json;charset=utf-8",
data: JSON.stringify(jsonData),
dataType: "json",
success: function() {
// do something
}
});
});
Controller Action
[HttpPost]
public JsonResult SaveSemester(SemesterVM semester)
{
// add semester data
return Json(semester);
}
answered Nov 26 '18 at 1:34
Tetsuya YamamotoTetsuya Yamamoto
17k42342
17k42342
Being updated code as above it saves null value in the database.
– Talukder
Nov 27 '18 at 8:38
UsingModelState.IsValid
doesn't make sense in AJAX callback. Also you're not includingHttpPost
attribute - this makes your AJAX call never reach the controller action which usesGET
.
– Tetsuya Yamamoto
Nov 27 '18 at 8:44
since I have used request behavior.allow get ,it is necessary to add [httppost],i have seen many articles not use [httppost ] at the Json request, and modelstate is eliminated. Tetsuya Yamamoto
– Talukder
Nov 27 '18 at 8:49
By default JSON data usesPOST
, if you want to allowGET
operation then you need to addJsonRequestBehavior.AllowGet
. Related: Why is JsonRequestBehavior needed?.
– Tetsuya Yamamoto
Nov 27 '18 at 8:53
i have run program as You say but it also save null values,is it occurs problem in ajax method? - Tetsuya Yamamoto
– Talukder
Nov 27 '18 at 9:12
|
show 2 more comments
Being updated code as above it saves null value in the database.
– Talukder
Nov 27 '18 at 8:38
UsingModelState.IsValid
doesn't make sense in AJAX callback. Also you're not includingHttpPost
attribute - this makes your AJAX call never reach the controller action which usesGET
.
– Tetsuya Yamamoto
Nov 27 '18 at 8:44
since I have used request behavior.allow get ,it is necessary to add [httppost],i have seen many articles not use [httppost ] at the Json request, and modelstate is eliminated. Tetsuya Yamamoto
– Talukder
Nov 27 '18 at 8:49
By default JSON data usesPOST
, if you want to allowGET
operation then you need to addJsonRequestBehavior.AllowGet
. Related: Why is JsonRequestBehavior needed?.
– Tetsuya Yamamoto
Nov 27 '18 at 8:53
i have run program as You say but it also save null values,is it occurs problem in ajax method? - Tetsuya Yamamoto
– Talukder
Nov 27 '18 at 9:12
Being updated code as above it saves null value in the database.
– Talukder
Nov 27 '18 at 8:38
Being updated code as above it saves null value in the database.
– Talukder
Nov 27 '18 at 8:38
Using
ModelState.IsValid
doesn't make sense in AJAX callback. Also you're not including HttpPost
attribute - this makes your AJAX call never reach the controller action which uses GET
.– Tetsuya Yamamoto
Nov 27 '18 at 8:44
Using
ModelState.IsValid
doesn't make sense in AJAX callback. Also you're not including HttpPost
attribute - this makes your AJAX call never reach the controller action which uses GET
.– Tetsuya Yamamoto
Nov 27 '18 at 8:44
since I have used request behavior.allow get ,it is necessary to add [httppost],i have seen many articles not use [httppost ] at the Json request, and modelstate is eliminated. Tetsuya Yamamoto
– Talukder
Nov 27 '18 at 8:49
since I have used request behavior.allow get ,it is necessary to add [httppost],i have seen many articles not use [httppost ] at the Json request, and modelstate is eliminated. Tetsuya Yamamoto
– Talukder
Nov 27 '18 at 8:49
By default JSON data uses
POST
, if you want to allow GET
operation then you need to add JsonRequestBehavior.AllowGet
. Related: Why is JsonRequestBehavior needed?.– Tetsuya Yamamoto
Nov 27 '18 at 8:53
By default JSON data uses
POST
, if you want to allow GET
operation then you need to add JsonRequestBehavior.AllowGet
. Related: Why is JsonRequestBehavior needed?.– Tetsuya Yamamoto
Nov 27 '18 at 8:53
i have run program as You say but it also save null values,is it occurs problem in ajax method? - Tetsuya Yamamoto
– Talukder
Nov 27 '18 at 9:12
i have run program as You say but it also save null values,is it occurs problem in ajax method? - Tetsuya Yamamoto
– Talukder
Nov 27 '18 at 9:12
|
show 2 more comments
After changing the ajax() method like below it works.
$.ajax({
type: "POST",
url: '@Url.Action("AsCreateSemester", "Semester")',
data: {
SemesterCode: code,
SemesterName: name
},
success:function(data) {
alert(data.SemesterName+"successfully inserted");
},
failure: function () {
alert("not successfull");
}
});
add a comment |
After changing the ajax() method like below it works.
$.ajax({
type: "POST",
url: '@Url.Action("AsCreateSemester", "Semester")',
data: {
SemesterCode: code,
SemesterName: name
},
success:function(data) {
alert(data.SemesterName+"successfully inserted");
},
failure: function () {
alert("not successfull");
}
});
add a comment |
After changing the ajax() method like below it works.
$.ajax({
type: "POST",
url: '@Url.Action("AsCreateSemester", "Semester")',
data: {
SemesterCode: code,
SemesterName: name
},
success:function(data) {
alert(data.SemesterName+"successfully inserted");
},
failure: function () {
alert("not successfull");
}
});
After changing the ajax() method like below it works.
$.ajax({
type: "POST",
url: '@Url.Action("AsCreateSemester", "Semester")',
data: {
SemesterCode: code,
SemesterName: name
},
success:function(data) {
alert(data.SemesterName+"successfully inserted");
},
failure: function () {
alert("not successfull");
}
});
answered Nov 27 '18 at 9:32
TalukderTalukder
267
267
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53471427%2fhow-to-insert-data-into-a-database-using-jquery-ajax-in-asp-net-mvc%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
pZ5YjqPmnPn5 BmjA,Y0cD376I7QJ9V gX GIxftz4G,hhghQmoz9tVSXAPGRz4iV20VUZTOsdsk
Add the attribute
HttpPost
to the action you are using to post data.– Llazar
Nov 25 '18 at 20:12
You are executing the ajax call onload of window which happens before a user has a chance to interact with the page. Move that javascript to a function that you can call when the button is clicked.
– Crowcoder
Nov 25 '18 at 21:41
not clear about your commnet ,what can be done @crowcoder
– Talukder
Nov 25 '18 at 21:51
Disregard, I missed the part where you wired the click event. Did you try dougF's answer?
– Crowcoder
Nov 25 '18 at 22:03
with regards,it dougF's answer does not work
– Talukder
Nov 25 '18 at 22:47