printing hello world in assembly using msdn api
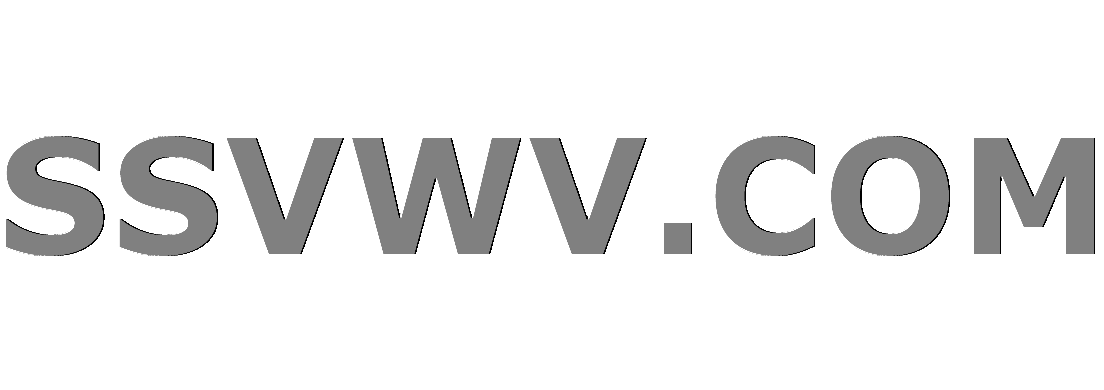
Multi tool use
I'm starting to learn assembly language just today and I'm using masm. I tried to print hello world using msdn api
here's my code:
1 .386
2 .model flat, stdcall
3 option casemap :none
4
5 include masm32includekernel32.inc
6 include masm32includemasm32.inc
7 includelib masm32libkernel32.lib
8 includelib masm32libmasm32.lib
9
10 .data
11 stroutput db "Hello World",0
12
13 .code
14 main:
15 push stroutput
16 call printf ; print hello world
17 add esp, 4
18 end main
but I got an error:
hello.asm(15) : error A2070: invalid instruction operands
hello.asm(16) : error A2006: undefined symbol : _printf
EDIT I tried to modified my code based on the suggestions:
.686
.model flat, stdcall
EXTERN printf : proc
include masm32includekernel32.inc
include masm32includeuser32.inc
includelib masm32libkernel32.lib
includelib masm32libuser32.lib
.data
msgCaption db 'Try', 0
.code
Main:
push offset msgCaption
call printf
push eax
End Main
but still got an error:
hello.obj : error LNK2001: unresolved external symbol _printf
assembly x86 masm msdn
|
show 5 more comments
I'm starting to learn assembly language just today and I'm using masm. I tried to print hello world using msdn api
here's my code:
1 .386
2 .model flat, stdcall
3 option casemap :none
4
5 include masm32includekernel32.inc
6 include masm32includemasm32.inc
7 includelib masm32libkernel32.lib
8 includelib masm32libmasm32.lib
9
10 .data
11 stroutput db "Hello World",0
12
13 .code
14 main:
15 push stroutput
16 call printf ; print hello world
17 add esp, 4
18 end main
but I got an error:
hello.asm(15) : error A2070: invalid instruction operands
hello.asm(16) : error A2006: undefined symbol : _printf
EDIT I tried to modified my code based on the suggestions:
.686
.model flat, stdcall
EXTERN printf : proc
include masm32includekernel32.inc
include masm32includeuser32.inc
includelib masm32libkernel32.lib
includelib masm32libuser32.lib
.data
msgCaption db 'Try', 0
.code
Main:
push offset msgCaption
call printf
push eax
End Main
but still got an error:
hello.obj : error LNK2001: unresolved external symbol _printf
assembly x86 masm msdn
Where do you think the definition of _printf will be? (Obviously) you don't define it, and it's not in kernel32 or masm32. Try looking at this.
– David Wohlferd
Nov 26 '18 at 7:54
When you want to use the printf function you had to declare it extern: extern printf
– Mike
Nov 26 '18 at 8:42
push stroutput
is incorrect since that is the same aspush [stroutput]
. It should have beenpush offset stroutput
– Michael Petch
Nov 26 '18 at 8:54
you'll needpush OFFSET stroutput
to push the address instead of the data, since this is MASM syntax not NASM. Also, you might needcall _printf
, or maybe not because apparently masm is prepending the leading underscore for you? Anyway,printf
is a C library function, not an WinAPI system call. You won't find it inkernel32.dll/lib
– Peter Cordes
Nov 26 '18 at 8:55
Why the numbers at the start of each line?
– usr2564301
Nov 26 '18 at 9:04
|
show 5 more comments
I'm starting to learn assembly language just today and I'm using masm. I tried to print hello world using msdn api
here's my code:
1 .386
2 .model flat, stdcall
3 option casemap :none
4
5 include masm32includekernel32.inc
6 include masm32includemasm32.inc
7 includelib masm32libkernel32.lib
8 includelib masm32libmasm32.lib
9
10 .data
11 stroutput db "Hello World",0
12
13 .code
14 main:
15 push stroutput
16 call printf ; print hello world
17 add esp, 4
18 end main
but I got an error:
hello.asm(15) : error A2070: invalid instruction operands
hello.asm(16) : error A2006: undefined symbol : _printf
EDIT I tried to modified my code based on the suggestions:
.686
.model flat, stdcall
EXTERN printf : proc
include masm32includekernel32.inc
include masm32includeuser32.inc
includelib masm32libkernel32.lib
includelib masm32libuser32.lib
.data
msgCaption db 'Try', 0
.code
Main:
push offset msgCaption
call printf
push eax
End Main
but still got an error:
hello.obj : error LNK2001: unresolved external symbol _printf
assembly x86 masm msdn
I'm starting to learn assembly language just today and I'm using masm. I tried to print hello world using msdn api
here's my code:
1 .386
2 .model flat, stdcall
3 option casemap :none
4
5 include masm32includekernel32.inc
6 include masm32includemasm32.inc
7 includelib masm32libkernel32.lib
8 includelib masm32libmasm32.lib
9
10 .data
11 stroutput db "Hello World",0
12
13 .code
14 main:
15 push stroutput
16 call printf ; print hello world
17 add esp, 4
18 end main
but I got an error:
hello.asm(15) : error A2070: invalid instruction operands
hello.asm(16) : error A2006: undefined symbol : _printf
EDIT I tried to modified my code based on the suggestions:
.686
.model flat, stdcall
EXTERN printf : proc
include masm32includekernel32.inc
include masm32includeuser32.inc
includelib masm32libkernel32.lib
includelib masm32libuser32.lib
.data
msgCaption db 'Try', 0
.code
Main:
push offset msgCaption
call printf
push eax
End Main
but still got an error:
hello.obj : error LNK2001: unresolved external symbol _printf
assembly x86 masm msdn
assembly x86 masm msdn
edited Nov 26 '18 at 9:35
frrelmj
asked Nov 26 '18 at 7:45
frrelmjfrrelmj
44
44
Where do you think the definition of _printf will be? (Obviously) you don't define it, and it's not in kernel32 or masm32. Try looking at this.
– David Wohlferd
Nov 26 '18 at 7:54
When you want to use the printf function you had to declare it extern: extern printf
– Mike
Nov 26 '18 at 8:42
push stroutput
is incorrect since that is the same aspush [stroutput]
. It should have beenpush offset stroutput
– Michael Petch
Nov 26 '18 at 8:54
you'll needpush OFFSET stroutput
to push the address instead of the data, since this is MASM syntax not NASM. Also, you might needcall _printf
, or maybe not because apparently masm is prepending the leading underscore for you? Anyway,printf
is a C library function, not an WinAPI system call. You won't find it inkernel32.dll/lib
– Peter Cordes
Nov 26 '18 at 8:55
Why the numbers at the start of each line?
– usr2564301
Nov 26 '18 at 9:04
|
show 5 more comments
Where do you think the definition of _printf will be? (Obviously) you don't define it, and it's not in kernel32 or masm32. Try looking at this.
– David Wohlferd
Nov 26 '18 at 7:54
When you want to use the printf function you had to declare it extern: extern printf
– Mike
Nov 26 '18 at 8:42
push stroutput
is incorrect since that is the same aspush [stroutput]
. It should have beenpush offset stroutput
– Michael Petch
Nov 26 '18 at 8:54
you'll needpush OFFSET stroutput
to push the address instead of the data, since this is MASM syntax not NASM. Also, you might needcall _printf
, or maybe not because apparently masm is prepending the leading underscore for you? Anyway,printf
is a C library function, not an WinAPI system call. You won't find it inkernel32.dll/lib
– Peter Cordes
Nov 26 '18 at 8:55
Why the numbers at the start of each line?
– usr2564301
Nov 26 '18 at 9:04
Where do you think the definition of _printf will be? (Obviously) you don't define it, and it's not in kernel32 or masm32. Try looking at this.
– David Wohlferd
Nov 26 '18 at 7:54
Where do you think the definition of _printf will be? (Obviously) you don't define it, and it's not in kernel32 or masm32. Try looking at this.
– David Wohlferd
Nov 26 '18 at 7:54
When you want to use the printf function you had to declare it extern: extern printf
– Mike
Nov 26 '18 at 8:42
When you want to use the printf function you had to declare it extern: extern printf
– Mike
Nov 26 '18 at 8:42
push stroutput
is incorrect since that is the same as push [stroutput]
. It should have been push offset stroutput
– Michael Petch
Nov 26 '18 at 8:54
push stroutput
is incorrect since that is the same as push [stroutput]
. It should have been push offset stroutput
– Michael Petch
Nov 26 '18 at 8:54
you'll need
push OFFSET stroutput
to push the address instead of the data, since this is MASM syntax not NASM. Also, you might need call _printf
, or maybe not because apparently masm is prepending the leading underscore for you? Anyway, printf
is a C library function, not an WinAPI system call. You won't find it in kernel32.dll/lib
– Peter Cordes
Nov 26 '18 at 8:55
you'll need
push OFFSET stroutput
to push the address instead of the data, since this is MASM syntax not NASM. Also, you might need call _printf
, or maybe not because apparently masm is prepending the leading underscore for you? Anyway, printf
is a C library function, not an WinAPI system call. You won't find it in kernel32.dll/lib
– Peter Cordes
Nov 26 '18 at 8:55
Why the numbers at the start of each line?
– usr2564301
Nov 26 '18 at 9:04
Why the numbers at the start of each line?
– usr2564301
Nov 26 '18 at 9:04
|
show 5 more comments
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53476632%2fprinting-hello-world-in-assembly-using-msdn-api%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53476632%2fprinting-hello-world-in-assembly-using-msdn-api%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
JNkDH5k17KEpM 1lNz4QmE7,c bzfRO4WS5hG25,yhwA03jrizWKFpEK5tqD5mW Hh9MV,pTAgzB40idaEhd5GXA
Where do you think the definition of _printf will be? (Obviously) you don't define it, and it's not in kernel32 or masm32. Try looking at this.
– David Wohlferd
Nov 26 '18 at 7:54
When you want to use the printf function you had to declare it extern: extern printf
– Mike
Nov 26 '18 at 8:42
push stroutput
is incorrect since that is the same aspush [stroutput]
. It should have beenpush offset stroutput
– Michael Petch
Nov 26 '18 at 8:54
you'll need
push OFFSET stroutput
to push the address instead of the data, since this is MASM syntax not NASM. Also, you might needcall _printf
, or maybe not because apparently masm is prepending the leading underscore for you? Anyway,printf
is a C library function, not an WinAPI system call. You won't find it inkernel32.dll/lib
– Peter Cordes
Nov 26 '18 at 8:55
Why the numbers at the start of each line?
– usr2564301
Nov 26 '18 at 9:04