Concrete type is not assignable to generic type
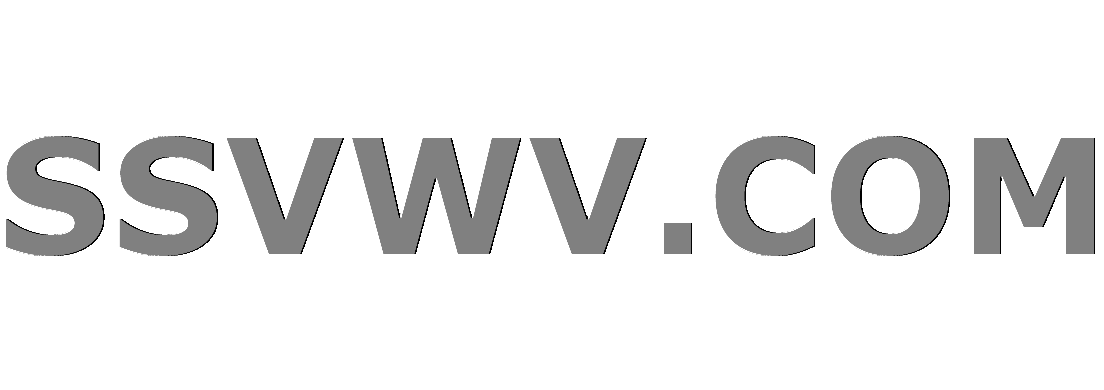
Multi tool use
up vote
1
down vote
favorite
type Id = <A>(a: A) => A
const id: Id = (a: number) => a;
If I use generics in this way the compiler will return an error saying
Type '(a: number) => number' is not assignable to type 'Id'.
Types of parameters 'a' and 'a' are incompatible.
Type 'A' is not assignable to type 'number'.
I know it can be solved with
type Id<A> = (a: A) => A
But what if I can't declare A at every point. Is there a way to just have it flow through, or be inferred?
typescript
add a comment |
up vote
1
down vote
favorite
type Id = <A>(a: A) => A
const id: Id = (a: number) => a;
If I use generics in this way the compiler will return an error saying
Type '(a: number) => number' is not assignable to type 'Id'.
Types of parameters 'a' and 'a' are incompatible.
Type 'A' is not assignable to type 'number'.
I know it can be solved with
type Id<A> = (a: A) => A
But what if I can't declare A at every point. Is there a way to just have it flow through, or be inferred?
typescript
1
If you remove the type annotation from the id const it should be assignable to types of Id<number>
– Michael
Nov 18 at 21:19
@Michael that's correct. But as soon as I want to do something witha
the compiler starts complaining:const id: Id = (a) => (a + 1);
Operator '+' cannot be applied to types 'A' and '1'.
– bbz
Nov 19 at 8:12
@Michael is telling you (@bbz) that you should just writeconst id = (a: number) => (a + 1);
. You don't need a type annotation onid
at all.
– jcalz
Nov 19 at 18:41
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
type Id = <A>(a: A) => A
const id: Id = (a: number) => a;
If I use generics in this way the compiler will return an error saying
Type '(a: number) => number' is not assignable to type 'Id'.
Types of parameters 'a' and 'a' are incompatible.
Type 'A' is not assignable to type 'number'.
I know it can be solved with
type Id<A> = (a: A) => A
But what if I can't declare A at every point. Is there a way to just have it flow through, or be inferred?
typescript
type Id = <A>(a: A) => A
const id: Id = (a: number) => a;
If I use generics in this way the compiler will return an error saying
Type '(a: number) => number' is not assignable to type 'Id'.
Types of parameters 'a' and 'a' are incompatible.
Type 'A' is not assignable to type 'number'.
I know it can be solved with
type Id<A> = (a: A) => A
But what if I can't declare A at every point. Is there a way to just have it flow through, or be inferred?
typescript
typescript
asked Nov 18 at 19:12
bbz
638
638
1
If you remove the type annotation from the id const it should be assignable to types of Id<number>
– Michael
Nov 18 at 21:19
@Michael that's correct. But as soon as I want to do something witha
the compiler starts complaining:const id: Id = (a) => (a + 1);
Operator '+' cannot be applied to types 'A' and '1'.
– bbz
Nov 19 at 8:12
@Michael is telling you (@bbz) that you should just writeconst id = (a: number) => (a + 1);
. You don't need a type annotation onid
at all.
– jcalz
Nov 19 at 18:41
add a comment |
1
If you remove the type annotation from the id const it should be assignable to types of Id<number>
– Michael
Nov 18 at 21:19
@Michael that's correct. But as soon as I want to do something witha
the compiler starts complaining:const id: Id = (a) => (a + 1);
Operator '+' cannot be applied to types 'A' and '1'.
– bbz
Nov 19 at 8:12
@Michael is telling you (@bbz) that you should just writeconst id = (a: number) => (a + 1);
. You don't need a type annotation onid
at all.
– jcalz
Nov 19 at 18:41
1
1
If you remove the type annotation from the id const it should be assignable to types of Id<number>
– Michael
Nov 18 at 21:19
If you remove the type annotation from the id const it should be assignable to types of Id<number>
– Michael
Nov 18 at 21:19
@Michael that's correct. But as soon as I want to do something with
a
the compiler starts complaining: const id: Id = (a) => (a + 1);
Operator '+' cannot be applied to types 'A' and '1'.
– bbz
Nov 19 at 8:12
@Michael that's correct. But as soon as I want to do something with
a
the compiler starts complaining: const id: Id = (a) => (a + 1);
Operator '+' cannot be applied to types 'A' and '1'.
– bbz
Nov 19 at 8:12
@Michael is telling you (@bbz) that you should just write
const id = (a: number) => (a + 1);
. You don't need a type annotation on id
at all.– jcalz
Nov 19 at 18:41
@Michael is telling you (@bbz) that you should just write
const id = (a: number) => (a + 1);
. You don't need a type annotation on id
at all.– jcalz
Nov 19 at 18:41
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
I think what you're experiencing is the difference between these two type definitions.
type Func1<A> = (a: A) => A;
type Func2 = <A>(a: A) => A;
Any function of type Func1<A>
must specify its type at the time it is defined.
const func1: Func1<number> = (a: number): number => a;
func1(10); // works
func1("x"); // fails - we already decided it is of type `number`
Anything of type Func2
must not specify its type until it is called.
const func2: Func2 = <X>(a: X): X => a;
func2(10); // works
func2("x"); // works
Here is a playground link that illustrates.
The problem you were experiencing happened because you tried to specify the type of the function when you defined it instead of when you called it.
Thanks Shaun. Like I mentioned I'm aware of the difference. But your answer makes it a lot more clear!
– bbz
Nov 19 at 8:20
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
I think what you're experiencing is the difference between these two type definitions.
type Func1<A> = (a: A) => A;
type Func2 = <A>(a: A) => A;
Any function of type Func1<A>
must specify its type at the time it is defined.
const func1: Func1<number> = (a: number): number => a;
func1(10); // works
func1("x"); // fails - we already decided it is of type `number`
Anything of type Func2
must not specify its type until it is called.
const func2: Func2 = <X>(a: X): X => a;
func2(10); // works
func2("x"); // works
Here is a playground link that illustrates.
The problem you were experiencing happened because you tried to specify the type of the function when you defined it instead of when you called it.
Thanks Shaun. Like I mentioned I'm aware of the difference. But your answer makes it a lot more clear!
– bbz
Nov 19 at 8:20
add a comment |
up vote
1
down vote
I think what you're experiencing is the difference between these two type definitions.
type Func1<A> = (a: A) => A;
type Func2 = <A>(a: A) => A;
Any function of type Func1<A>
must specify its type at the time it is defined.
const func1: Func1<number> = (a: number): number => a;
func1(10); // works
func1("x"); // fails - we already decided it is of type `number`
Anything of type Func2
must not specify its type until it is called.
const func2: Func2 = <X>(a: X): X => a;
func2(10); // works
func2("x"); // works
Here is a playground link that illustrates.
The problem you were experiencing happened because you tried to specify the type of the function when you defined it instead of when you called it.
Thanks Shaun. Like I mentioned I'm aware of the difference. But your answer makes it a lot more clear!
– bbz
Nov 19 at 8:20
add a comment |
up vote
1
down vote
up vote
1
down vote
I think what you're experiencing is the difference between these two type definitions.
type Func1<A> = (a: A) => A;
type Func2 = <A>(a: A) => A;
Any function of type Func1<A>
must specify its type at the time it is defined.
const func1: Func1<number> = (a: number): number => a;
func1(10); // works
func1("x"); // fails - we already decided it is of type `number`
Anything of type Func2
must not specify its type until it is called.
const func2: Func2 = <X>(a: X): X => a;
func2(10); // works
func2("x"); // works
Here is a playground link that illustrates.
The problem you were experiencing happened because you tried to specify the type of the function when you defined it instead of when you called it.
I think what you're experiencing is the difference between these two type definitions.
type Func1<A> = (a: A) => A;
type Func2 = <A>(a: A) => A;
Any function of type Func1<A>
must specify its type at the time it is defined.
const func1: Func1<number> = (a: number): number => a;
func1(10); // works
func1("x"); // fails - we already decided it is of type `number`
Anything of type Func2
must not specify its type until it is called.
const func2: Func2 = <X>(a: X): X => a;
func2(10); // works
func2("x"); // works
Here is a playground link that illustrates.
The problem you were experiencing happened because you tried to specify the type of the function when you defined it instead of when you called it.
edited Nov 19 at 1:08
answered Nov 19 at 0:50


Shaun Luttin
55.9k32215276
55.9k32215276
Thanks Shaun. Like I mentioned I'm aware of the difference. But your answer makes it a lot more clear!
– bbz
Nov 19 at 8:20
add a comment |
Thanks Shaun. Like I mentioned I'm aware of the difference. But your answer makes it a lot more clear!
– bbz
Nov 19 at 8:20
Thanks Shaun. Like I mentioned I'm aware of the difference. But your answer makes it a lot more clear!
– bbz
Nov 19 at 8:20
Thanks Shaun. Like I mentioned I'm aware of the difference. But your answer makes it a lot more clear!
– bbz
Nov 19 at 8:20
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53364524%2fconcrete-type-is-not-assignable-to-generic-type%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Ha7A OBV7c,JlfsuGijXj57IJj,N,Q,b,EI 92OcPCo8j,6k37wxy6kGFkX P
1
If you remove the type annotation from the id const it should be assignable to types of Id<number>
– Michael
Nov 18 at 21:19
@Michael that's correct. But as soon as I want to do something with
a
the compiler starts complaining:const id: Id = (a) => (a + 1);
Operator '+' cannot be applied to types 'A' and '1'.
– bbz
Nov 19 at 8:12
@Michael is telling you (@bbz) that you should just write
const id = (a: number) => (a + 1);
. You don't need a type annotation onid
at all.– jcalz
Nov 19 at 18:41