Converting a JSON object with multiple lists as fields
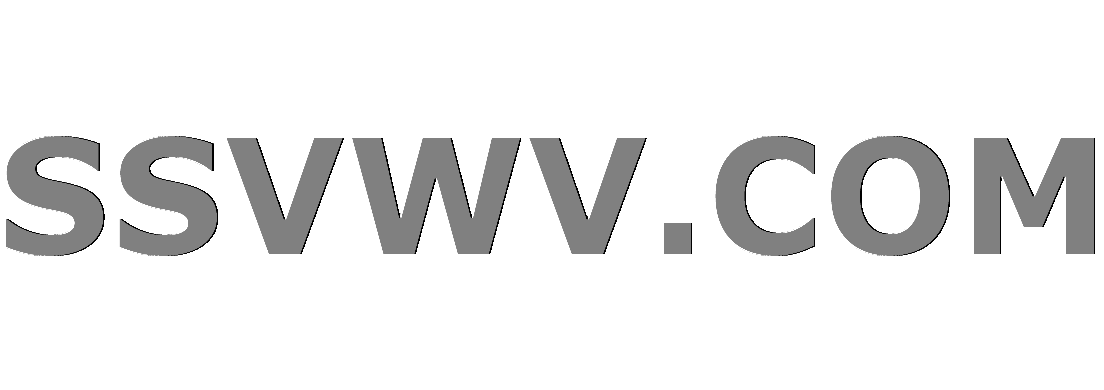
Multi tool use
I'm using jackson's ObjectMapper to convert JSON files into Java objects.
How do I convert a JSON object that has an array as one of its fields? Example below:
{
"list":[
{
"value":"example"
},
{
"value":"example2"
}
]
}
ObjectMapper
converts this into a LinkedHashMap
with lines
as the key and the value is an ArrayList
with LinkedHashMaps
, which have value
and example
and so on.
Is there a way to read this json as an Object with a field list
that is a List/Array containing objects which would fit (in this case, a simple object with String value
as a field)?
java json jackson
add a comment |
I'm using jackson's ObjectMapper to convert JSON files into Java objects.
How do I convert a JSON object that has an array as one of its fields? Example below:
{
"list":[
{
"value":"example"
},
{
"value":"example2"
}
]
}
ObjectMapper
converts this into a LinkedHashMap
with lines
as the key and the value is an ArrayList
with LinkedHashMaps
, which have value
and example
and so on.
Is there a way to read this json as an Object with a field list
that is a List/Array containing objects which would fit (in this case, a simple object with String value
as a field)?
java json jackson
add a comment |
I'm using jackson's ObjectMapper to convert JSON files into Java objects.
How do I convert a JSON object that has an array as one of its fields? Example below:
{
"list":[
{
"value":"example"
},
{
"value":"example2"
}
]
}
ObjectMapper
converts this into a LinkedHashMap
with lines
as the key and the value is an ArrayList
with LinkedHashMaps
, which have value
and example
and so on.
Is there a way to read this json as an Object with a field list
that is a List/Array containing objects which would fit (in this case, a simple object with String value
as a field)?
java json jackson
I'm using jackson's ObjectMapper to convert JSON files into Java objects.
How do I convert a JSON object that has an array as one of its fields? Example below:
{
"list":[
{
"value":"example"
},
{
"value":"example2"
}
]
}
ObjectMapper
converts this into a LinkedHashMap
with lines
as the key and the value is an ArrayList
with LinkedHashMaps
, which have value
and example
and so on.
Is there a way to read this json as an Object with a field list
that is a List/Array containing objects which would fit (in this case, a simple object with String value
as a field)?
java json jackson
java json jackson
asked Nov 20 at 18:22
kulijana
237
237
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
Found a way to do this. This solution is based around Gson
instead of ObjectMapper
.
Basically Gson manages to convert arrays into the fields as I wanted it to happen here, instead of generating a lot of LinkedHashMaps
.
add a comment |
You can achieve this with Jackson in a straight-forward way.
For this you need to model the JSON structure by some Java classes.
First, you need a class for modeling the whole JSON content
(let us call it Root
) with a list
property.
public class Root {
private List<Item> list;
// public getter and setter (omitted here for brevity)
}
Next, you need a class for modeling the list items
(let us call it Item
) with a value
property.
public class Item {
private String value;
// public getter and setter (omitted here for brevity)
}
Then you are able to read JSON content into a Java Root
object by using
one of ObjectMapper
's readValue(..., Class<T>)
methods.
For example reading from a File
:
ObjectMapper objectMapper = new ObjectMapper();
Root root = objectMapper.readValue(new File("example.json"), Root.class);
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53399210%2fconverting-a-json-object-with-multiple-lists-as-fields%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Found a way to do this. This solution is based around Gson
instead of ObjectMapper
.
Basically Gson manages to convert arrays into the fields as I wanted it to happen here, instead of generating a lot of LinkedHashMaps
.
add a comment |
Found a way to do this. This solution is based around Gson
instead of ObjectMapper
.
Basically Gson manages to convert arrays into the fields as I wanted it to happen here, instead of generating a lot of LinkedHashMaps
.
add a comment |
Found a way to do this. This solution is based around Gson
instead of ObjectMapper
.
Basically Gson manages to convert arrays into the fields as I wanted it to happen here, instead of generating a lot of LinkedHashMaps
.
Found a way to do this. This solution is based around Gson
instead of ObjectMapper
.
Basically Gson manages to convert arrays into the fields as I wanted it to happen here, instead of generating a lot of LinkedHashMaps
.
answered Nov 20 at 19:02
kulijana
237
237
add a comment |
add a comment |
You can achieve this with Jackson in a straight-forward way.
For this you need to model the JSON structure by some Java classes.
First, you need a class for modeling the whole JSON content
(let us call it Root
) with a list
property.
public class Root {
private List<Item> list;
// public getter and setter (omitted here for brevity)
}
Next, you need a class for modeling the list items
(let us call it Item
) with a value
property.
public class Item {
private String value;
// public getter and setter (omitted here for brevity)
}
Then you are able to read JSON content into a Java Root
object by using
one of ObjectMapper
's readValue(..., Class<T>)
methods.
For example reading from a File
:
ObjectMapper objectMapper = new ObjectMapper();
Root root = objectMapper.readValue(new File("example.json"), Root.class);
add a comment |
You can achieve this with Jackson in a straight-forward way.
For this you need to model the JSON structure by some Java classes.
First, you need a class for modeling the whole JSON content
(let us call it Root
) with a list
property.
public class Root {
private List<Item> list;
// public getter and setter (omitted here for brevity)
}
Next, you need a class for modeling the list items
(let us call it Item
) with a value
property.
public class Item {
private String value;
// public getter and setter (omitted here for brevity)
}
Then you are able to read JSON content into a Java Root
object by using
one of ObjectMapper
's readValue(..., Class<T>)
methods.
For example reading from a File
:
ObjectMapper objectMapper = new ObjectMapper();
Root root = objectMapper.readValue(new File("example.json"), Root.class);
add a comment |
You can achieve this with Jackson in a straight-forward way.
For this you need to model the JSON structure by some Java classes.
First, you need a class for modeling the whole JSON content
(let us call it Root
) with a list
property.
public class Root {
private List<Item> list;
// public getter and setter (omitted here for brevity)
}
Next, you need a class for modeling the list items
(let us call it Item
) with a value
property.
public class Item {
private String value;
// public getter and setter (omitted here for brevity)
}
Then you are able to read JSON content into a Java Root
object by using
one of ObjectMapper
's readValue(..., Class<T>)
methods.
For example reading from a File
:
ObjectMapper objectMapper = new ObjectMapper();
Root root = objectMapper.readValue(new File("example.json"), Root.class);
You can achieve this with Jackson in a straight-forward way.
For this you need to model the JSON structure by some Java classes.
First, you need a class for modeling the whole JSON content
(let us call it Root
) with a list
property.
public class Root {
private List<Item> list;
// public getter and setter (omitted here for brevity)
}
Next, you need a class for modeling the list items
(let us call it Item
) with a value
property.
public class Item {
private String value;
// public getter and setter (omitted here for brevity)
}
Then you are able to read JSON content into a Java Root
object by using
one of ObjectMapper
's readValue(..., Class<T>)
methods.
For example reading from a File
:
ObjectMapper objectMapper = new ObjectMapper();
Root root = objectMapper.readValue(new File("example.json"), Root.class);
edited Nov 20 at 19:16
answered Nov 20 at 19:10


Thomas Fritsch
4,943121933
4,943121933
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53399210%2fconverting-a-json-object-with-multiple-lists-as-fields%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
lc7oPMAKiXTxoDQIXUX1prNyGRHeUfK9pM36TA oJ1TpfD6YqV6BvvY1IkECWTnmFoQd7SWI3Jf