Redux Reducer function has empty state
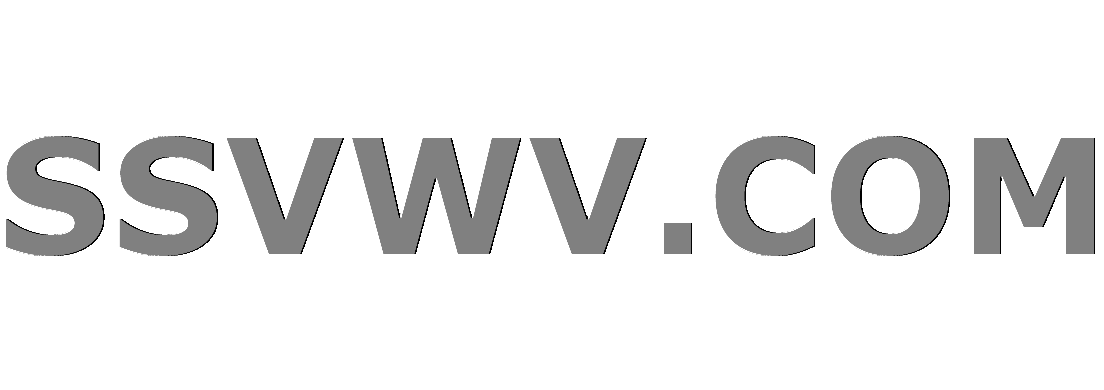
Multi tool use
This is a bit weird for me, because my state array is empty in function of the same reducer while not in other.
So, my reducer looks like this -
export function booksHasErrored(state = false, action) {
//console.log(action.type);
switch (action.type) {
case 'BOOKS_HAS_ERRORED':
return action.hasErrored;
default:
return state;
}
}
export function booksIsLoading(state = false, action) {
switch (action.type) {
case 'BOOKS_IS_LOADING':
return action.isLoading;
default:
return state;
}
}
export function books(state = , action) {
switch (action.type) {
case 'BOOKS_FETCH_DATA_SUCCESS':
return action.books;
default:
return state;
}
}
export function updateBook(state = , action) {
console.log('updateBook');
console.log(state);
console.log(action);
switch (action.type) {
case 'BOOK_UPDATE':
return state;
default:
return state;
}
}
In this reducer, function books has got state with a list of all my books. But, the updateBook function always shows empty state array. In my web application, first books function is called to get a list of all my books from API call and then update function is called and user navigates to update the book. I expected my updateBook function to receive the state object with all its items.
This is how I am combining my reducers -
import {combineReducers} from 'redux';
import {routerReducer} from 'react-router-redux';
import { books, booksHasErrored, booksIsLoading,updateBook } from './books';
import { search, searchHasErrored, searchIsLoading } from './search';
import { authors, authorsHasErrored, authorsIsLoading } from './authors';
//import authors from './authors';
//console.log(books);
const rootReducer = combineReducers({ books,booksHasErrored,booksIsLoading,updateBook,authors,search, searchHasErrored, searchIsLoading, authors, authorsHasErrored, authorsIsLoading,routing:routerReducer});
export default rootReducer;
Please let me know if you need more information and I will add it here.
react-redux redux-thunk
add a comment |
This is a bit weird for me, because my state array is empty in function of the same reducer while not in other.
So, my reducer looks like this -
export function booksHasErrored(state = false, action) {
//console.log(action.type);
switch (action.type) {
case 'BOOKS_HAS_ERRORED':
return action.hasErrored;
default:
return state;
}
}
export function booksIsLoading(state = false, action) {
switch (action.type) {
case 'BOOKS_IS_LOADING':
return action.isLoading;
default:
return state;
}
}
export function books(state = , action) {
switch (action.type) {
case 'BOOKS_FETCH_DATA_SUCCESS':
return action.books;
default:
return state;
}
}
export function updateBook(state = , action) {
console.log('updateBook');
console.log(state);
console.log(action);
switch (action.type) {
case 'BOOK_UPDATE':
return state;
default:
return state;
}
}
In this reducer, function books has got state with a list of all my books. But, the updateBook function always shows empty state array. In my web application, first books function is called to get a list of all my books from API call and then update function is called and user navigates to update the book. I expected my updateBook function to receive the state object with all its items.
This is how I am combining my reducers -
import {combineReducers} from 'redux';
import {routerReducer} from 'react-router-redux';
import { books, booksHasErrored, booksIsLoading,updateBook } from './books';
import { search, searchHasErrored, searchIsLoading } from './search';
import { authors, authorsHasErrored, authorsIsLoading } from './authors';
//import authors from './authors';
//console.log(books);
const rootReducer = combineReducers({ books,booksHasErrored,booksIsLoading,updateBook,authors,search, searchHasErrored, searchIsLoading, authors, authorsHasErrored, authorsIsLoading,routing:routerReducer});
export default rootReducer;
Please let me know if you need more information and I will add it here.
react-redux redux-thunk
Do you have to declare the parameter state as empty in the function? Maybe this is impacting, try to set state instead of state =
– Eliâ Melfior
Nov 23 '18 at 10:22
1
state = is just an es6 way of setting a default empty array.
– nasaa
Nov 23 '18 at 11:43
add a comment |
This is a bit weird for me, because my state array is empty in function of the same reducer while not in other.
So, my reducer looks like this -
export function booksHasErrored(state = false, action) {
//console.log(action.type);
switch (action.type) {
case 'BOOKS_HAS_ERRORED':
return action.hasErrored;
default:
return state;
}
}
export function booksIsLoading(state = false, action) {
switch (action.type) {
case 'BOOKS_IS_LOADING':
return action.isLoading;
default:
return state;
}
}
export function books(state = , action) {
switch (action.type) {
case 'BOOKS_FETCH_DATA_SUCCESS':
return action.books;
default:
return state;
}
}
export function updateBook(state = , action) {
console.log('updateBook');
console.log(state);
console.log(action);
switch (action.type) {
case 'BOOK_UPDATE':
return state;
default:
return state;
}
}
In this reducer, function books has got state with a list of all my books. But, the updateBook function always shows empty state array. In my web application, first books function is called to get a list of all my books from API call and then update function is called and user navigates to update the book. I expected my updateBook function to receive the state object with all its items.
This is how I am combining my reducers -
import {combineReducers} from 'redux';
import {routerReducer} from 'react-router-redux';
import { books, booksHasErrored, booksIsLoading,updateBook } from './books';
import { search, searchHasErrored, searchIsLoading } from './search';
import { authors, authorsHasErrored, authorsIsLoading } from './authors';
//import authors from './authors';
//console.log(books);
const rootReducer = combineReducers({ books,booksHasErrored,booksIsLoading,updateBook,authors,search, searchHasErrored, searchIsLoading, authors, authorsHasErrored, authorsIsLoading,routing:routerReducer});
export default rootReducer;
Please let me know if you need more information and I will add it here.
react-redux redux-thunk
This is a bit weird for me, because my state array is empty in function of the same reducer while not in other.
So, my reducer looks like this -
export function booksHasErrored(state = false, action) {
//console.log(action.type);
switch (action.type) {
case 'BOOKS_HAS_ERRORED':
return action.hasErrored;
default:
return state;
}
}
export function booksIsLoading(state = false, action) {
switch (action.type) {
case 'BOOKS_IS_LOADING':
return action.isLoading;
default:
return state;
}
}
export function books(state = , action) {
switch (action.type) {
case 'BOOKS_FETCH_DATA_SUCCESS':
return action.books;
default:
return state;
}
}
export function updateBook(state = , action) {
console.log('updateBook');
console.log(state);
console.log(action);
switch (action.type) {
case 'BOOK_UPDATE':
return state;
default:
return state;
}
}
In this reducer, function books has got state with a list of all my books. But, the updateBook function always shows empty state array. In my web application, first books function is called to get a list of all my books from API call and then update function is called and user navigates to update the book. I expected my updateBook function to receive the state object with all its items.
This is how I am combining my reducers -
import {combineReducers} from 'redux';
import {routerReducer} from 'react-router-redux';
import { books, booksHasErrored, booksIsLoading,updateBook } from './books';
import { search, searchHasErrored, searchIsLoading } from './search';
import { authors, authorsHasErrored, authorsIsLoading } from './authors';
//import authors from './authors';
//console.log(books);
const rootReducer = combineReducers({ books,booksHasErrored,booksIsLoading,updateBook,authors,search, searchHasErrored, searchIsLoading, authors, authorsHasErrored, authorsIsLoading,routing:routerReducer});
export default rootReducer;
Please let me know if you need more information and I will add it here.
react-redux redux-thunk
react-redux redux-thunk
asked Nov 21 '18 at 15:04


nasaanasaa
1,32873462
1,32873462
Do you have to declare the parameter state as empty in the function? Maybe this is impacting, try to set state instead of state =
– Eliâ Melfior
Nov 23 '18 at 10:22
1
state = is just an es6 way of setting a default empty array.
– nasaa
Nov 23 '18 at 11:43
add a comment |
Do you have to declare the parameter state as empty in the function? Maybe this is impacting, try to set state instead of state =
– Eliâ Melfior
Nov 23 '18 at 10:22
1
state = is just an es6 way of setting a default empty array.
– nasaa
Nov 23 '18 at 11:43
Do you have to declare the parameter state as empty in the function? Maybe this is impacting, try to set state instead of state =
– Eliâ Melfior
Nov 23 '18 at 10:22
Do you have to declare the parameter state as empty in the function? Maybe this is impacting, try to set state instead of state =
– Eliâ Melfior
Nov 23 '18 at 10:22
1
1
state = is just an es6 way of setting a default empty array.
– nasaa
Nov 23 '18 at 11:43
state = is just an es6 way of setting a default empty array.
– nasaa
Nov 23 '18 at 11:43
add a comment |
1 Answer
1
active
oldest
votes
I might have discovered your problem, you are not returning the full state, you are returning the action
export function books(state = , action) {
switch (action.type) {
case 'BOOKS_FETCH_DATA_SUCCESS':
return action.books;
default:
return state;
}
}
You need to create a new state and mutate it, and then return it, example:
export function books(state = , action) {
switch (action.type) {
case 'BOOKS_FETCH_DATA_SUCCESS':
return state.set('books', action.books);
default:
return state;
}
}
Can you change it and see if it works?
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53414910%2fredux-reducer-function-has-empty-state%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I might have discovered your problem, you are not returning the full state, you are returning the action
export function books(state = , action) {
switch (action.type) {
case 'BOOKS_FETCH_DATA_SUCCESS':
return action.books;
default:
return state;
}
}
You need to create a new state and mutate it, and then return it, example:
export function books(state = , action) {
switch (action.type) {
case 'BOOKS_FETCH_DATA_SUCCESS':
return state.set('books', action.books);
default:
return state;
}
}
Can you change it and see if it works?
add a comment |
I might have discovered your problem, you are not returning the full state, you are returning the action
export function books(state = , action) {
switch (action.type) {
case 'BOOKS_FETCH_DATA_SUCCESS':
return action.books;
default:
return state;
}
}
You need to create a new state and mutate it, and then return it, example:
export function books(state = , action) {
switch (action.type) {
case 'BOOKS_FETCH_DATA_SUCCESS':
return state.set('books', action.books);
default:
return state;
}
}
Can you change it and see if it works?
add a comment |
I might have discovered your problem, you are not returning the full state, you are returning the action
export function books(state = , action) {
switch (action.type) {
case 'BOOKS_FETCH_DATA_SUCCESS':
return action.books;
default:
return state;
}
}
You need to create a new state and mutate it, and then return it, example:
export function books(state = , action) {
switch (action.type) {
case 'BOOKS_FETCH_DATA_SUCCESS':
return state.set('books', action.books);
default:
return state;
}
}
Can you change it and see if it works?
I might have discovered your problem, you are not returning the full state, you are returning the action
export function books(state = , action) {
switch (action.type) {
case 'BOOKS_FETCH_DATA_SUCCESS':
return action.books;
default:
return state;
}
}
You need to create a new state and mutate it, and then return it, example:
export function books(state = , action) {
switch (action.type) {
case 'BOOKS_FETCH_DATA_SUCCESS':
return state.set('books', action.books);
default:
return state;
}
}
Can you change it and see if it works?
answered Nov 23 '18 at 11:47


Eliâ MelfiorEliâ Melfior
9011
9011
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53414910%2fredux-reducer-function-has-empty-state%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
jSaB tqyx0mX3sD,fmzu5c67
Do you have to declare the parameter state as empty in the function? Maybe this is impacting, try to set state instead of state =
– Eliâ Melfior
Nov 23 '18 at 10:22
1
state = is just an es6 way of setting a default empty array.
– nasaa
Nov 23 '18 at 11:43