Invoking AWS Lambda endpoint
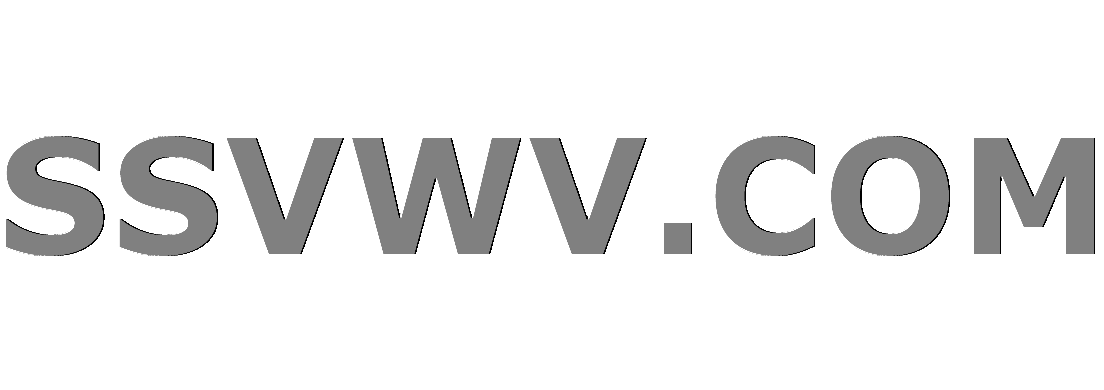
Multi tool use
I implemented AWS lambda method to learn what I can do with it.
What I have now:
- AWS lambda itself
- API Gateway
- Amazon CloudWatch Logs
In API Gateway configs I see next options:
- Endpoint: https://xyz.execute-api.us-east-2.amazonaws.com/dummy/testStore
- API key: QW123E45RTY6
- Authorization: NONE
Behind this lambda I have Java code, implementing com.amazonaws.services.lambda.runtime.RequestStreamHandler and a REST controller behind it, something like that:
@Path("/tester")
public class TestResource {
private static final Logger LOG = LoggerFactory.getLogger(MethodHandles.lookup().lookupClass());
private TestRepository testRepository;
public void setTestRepository(TestRepository testRepository) {
this.testRepository = testRepository;
}
@POST
@Path("/{identifier}")
@Produces(MediaType.TEXT_PLAIN)
@Consumes(MediaType.WILDCARD)
public Response store(@PathParam("identifier") String identifier, @QueryParam("hashcode") String hashcode) {
try {
this.testRepository.store(identifier, hashcode);
} catch (RuntimeException ex) {
LOG.error("Failed to store pair {}, {}", identifier, hashcode, ex);
throw new InternalServerErrorException(ex);
}
return Response.noContent().build();
}
}
Now I tried invoking this service through Postman:
Option 1 - Invoke what I saw in API Gateway:
- URL: https://xyz.execute-api.us-east-2.amazonaws.com/dummy/testStore
- Method: POST
- Headers:
- Key: x-api-key
- Value: QW123E45RTY6
Result: 404 Not Found, see the appropriate logs in AWS CloudWatch.
Option 2 - Invoke my method:
- URL: https://xyz.execute-api.us-east-2.amazonaws.com/dummy/testStore/tester/qwerty123
- Method: POST
- Headers:
- Key: x-api-key
- Value: QW123E45RTY6
Result: 403 Forbidden, no logs in AWS CloudWatch.
{
"message": "Missing Authentication Token"
}
I fully understand why Option 1 did not work, but what went wrong with Option 2? Could you please help me understanding what I missed?
rest amazon-web-services aws-lambda
add a comment |
I implemented AWS lambda method to learn what I can do with it.
What I have now:
- AWS lambda itself
- API Gateway
- Amazon CloudWatch Logs
In API Gateway configs I see next options:
- Endpoint: https://xyz.execute-api.us-east-2.amazonaws.com/dummy/testStore
- API key: QW123E45RTY6
- Authorization: NONE
Behind this lambda I have Java code, implementing com.amazonaws.services.lambda.runtime.RequestStreamHandler and a REST controller behind it, something like that:
@Path("/tester")
public class TestResource {
private static final Logger LOG = LoggerFactory.getLogger(MethodHandles.lookup().lookupClass());
private TestRepository testRepository;
public void setTestRepository(TestRepository testRepository) {
this.testRepository = testRepository;
}
@POST
@Path("/{identifier}")
@Produces(MediaType.TEXT_PLAIN)
@Consumes(MediaType.WILDCARD)
public Response store(@PathParam("identifier") String identifier, @QueryParam("hashcode") String hashcode) {
try {
this.testRepository.store(identifier, hashcode);
} catch (RuntimeException ex) {
LOG.error("Failed to store pair {}, {}", identifier, hashcode, ex);
throw new InternalServerErrorException(ex);
}
return Response.noContent().build();
}
}
Now I tried invoking this service through Postman:
Option 1 - Invoke what I saw in API Gateway:
- URL: https://xyz.execute-api.us-east-2.amazonaws.com/dummy/testStore
- Method: POST
- Headers:
- Key: x-api-key
- Value: QW123E45RTY6
Result: 404 Not Found, see the appropriate logs in AWS CloudWatch.
Option 2 - Invoke my method:
- URL: https://xyz.execute-api.us-east-2.amazonaws.com/dummy/testStore/tester/qwerty123
- Method: POST
- Headers:
- Key: x-api-key
- Value: QW123E45RTY6
Result: 403 Forbidden, no logs in AWS CloudWatch.
{
"message": "Missing Authentication Token"
}
I fully understand why Option 1 did not work, but what went wrong with Option 2? Could you please help me understanding what I missed?
rest amazon-web-services aws-lambda
add a comment |
I implemented AWS lambda method to learn what I can do with it.
What I have now:
- AWS lambda itself
- API Gateway
- Amazon CloudWatch Logs
In API Gateway configs I see next options:
- Endpoint: https://xyz.execute-api.us-east-2.amazonaws.com/dummy/testStore
- API key: QW123E45RTY6
- Authorization: NONE
Behind this lambda I have Java code, implementing com.amazonaws.services.lambda.runtime.RequestStreamHandler and a REST controller behind it, something like that:
@Path("/tester")
public class TestResource {
private static final Logger LOG = LoggerFactory.getLogger(MethodHandles.lookup().lookupClass());
private TestRepository testRepository;
public void setTestRepository(TestRepository testRepository) {
this.testRepository = testRepository;
}
@POST
@Path("/{identifier}")
@Produces(MediaType.TEXT_PLAIN)
@Consumes(MediaType.WILDCARD)
public Response store(@PathParam("identifier") String identifier, @QueryParam("hashcode") String hashcode) {
try {
this.testRepository.store(identifier, hashcode);
} catch (RuntimeException ex) {
LOG.error("Failed to store pair {}, {}", identifier, hashcode, ex);
throw new InternalServerErrorException(ex);
}
return Response.noContent().build();
}
}
Now I tried invoking this service through Postman:
Option 1 - Invoke what I saw in API Gateway:
- URL: https://xyz.execute-api.us-east-2.amazonaws.com/dummy/testStore
- Method: POST
- Headers:
- Key: x-api-key
- Value: QW123E45RTY6
Result: 404 Not Found, see the appropriate logs in AWS CloudWatch.
Option 2 - Invoke my method:
- URL: https://xyz.execute-api.us-east-2.amazonaws.com/dummy/testStore/tester/qwerty123
- Method: POST
- Headers:
- Key: x-api-key
- Value: QW123E45RTY6
Result: 403 Forbidden, no logs in AWS CloudWatch.
{
"message": "Missing Authentication Token"
}
I fully understand why Option 1 did not work, but what went wrong with Option 2? Could you please help me understanding what I missed?
rest amazon-web-services aws-lambda
I implemented AWS lambda method to learn what I can do with it.
What I have now:
- AWS lambda itself
- API Gateway
- Amazon CloudWatch Logs
In API Gateway configs I see next options:
- Endpoint: https://xyz.execute-api.us-east-2.amazonaws.com/dummy/testStore
- API key: QW123E45RTY6
- Authorization: NONE
Behind this lambda I have Java code, implementing com.amazonaws.services.lambda.runtime.RequestStreamHandler and a REST controller behind it, something like that:
@Path("/tester")
public class TestResource {
private static final Logger LOG = LoggerFactory.getLogger(MethodHandles.lookup().lookupClass());
private TestRepository testRepository;
public void setTestRepository(TestRepository testRepository) {
this.testRepository = testRepository;
}
@POST
@Path("/{identifier}")
@Produces(MediaType.TEXT_PLAIN)
@Consumes(MediaType.WILDCARD)
public Response store(@PathParam("identifier") String identifier, @QueryParam("hashcode") String hashcode) {
try {
this.testRepository.store(identifier, hashcode);
} catch (RuntimeException ex) {
LOG.error("Failed to store pair {}, {}", identifier, hashcode, ex);
throw new InternalServerErrorException(ex);
}
return Response.noContent().build();
}
}
Now I tried invoking this service through Postman:
Option 1 - Invoke what I saw in API Gateway:
- URL: https://xyz.execute-api.us-east-2.amazonaws.com/dummy/testStore
- Method: POST
- Headers:
- Key: x-api-key
- Value: QW123E45RTY6
Result: 404 Not Found, see the appropriate logs in AWS CloudWatch.
Option 2 - Invoke my method:
- URL: https://xyz.execute-api.us-east-2.amazonaws.com/dummy/testStore/tester/qwerty123
- Method: POST
- Headers:
- Key: x-api-key
- Value: QW123E45RTY6
Result: 403 Forbidden, no logs in AWS CloudWatch.
{
"message": "Missing Authentication Token"
}
I fully understand why Option 1 did not work, but what went wrong with Option 2? Could you please help me understanding what I missed?
rest amazon-web-services aws-lambda
rest amazon-web-services aws-lambda
asked Nov 25 '18 at 19:30


stepiostepio
111110
111110
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
This message occurs (most of the times) when you try to call URL that doesn't exist.
Please make sure you are calling http://api-gateway-name/stage-name/resource-name
Also, make sure you have deployed your API.
Thank you for your hint, you was right that URL was wrong, but I did not understand why it was wrong before I dived into the AWS client's code. I'm going to paste my finding as a separate answer here.
– stepio
Dec 2 '18 at 13:35
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53471088%2finvoking-aws-lambda-endpoint%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
This message occurs (most of the times) when you try to call URL that doesn't exist.
Please make sure you are calling http://api-gateway-name/stage-name/resource-name
Also, make sure you have deployed your API.
Thank you for your hint, you was right that URL was wrong, but I did not understand why it was wrong before I dived into the AWS client's code. I'm going to paste my finding as a separate answer here.
– stepio
Dec 2 '18 at 13:35
add a comment |
This message occurs (most of the times) when you try to call URL that doesn't exist.
Please make sure you are calling http://api-gateway-name/stage-name/resource-name
Also, make sure you have deployed your API.
Thank you for your hint, you was right that URL was wrong, but I did not understand why it was wrong before I dived into the AWS client's code. I'm going to paste my finding as a separate answer here.
– stepio
Dec 2 '18 at 13:35
add a comment |
This message occurs (most of the times) when you try to call URL that doesn't exist.
Please make sure you are calling http://api-gateway-name/stage-name/resource-name
Also, make sure you have deployed your API.
This message occurs (most of the times) when you try to call URL that doesn't exist.
Please make sure you are calling http://api-gateway-name/stage-name/resource-name
Also, make sure you have deployed your API.
edited Nov 25 '18 at 21:20
answered Nov 25 '18 at 20:50
AlexKAlexK
889513
889513
Thank you for your hint, you was right that URL was wrong, but I did not understand why it was wrong before I dived into the AWS client's code. I'm going to paste my finding as a separate answer here.
– stepio
Dec 2 '18 at 13:35
add a comment |
Thank you for your hint, you was right that URL was wrong, but I did not understand why it was wrong before I dived into the AWS client's code. I'm going to paste my finding as a separate answer here.
– stepio
Dec 2 '18 at 13:35
Thank you for your hint, you was right that URL was wrong, but I did not understand why it was wrong before I dived into the AWS client's code. I'm going to paste my finding as a separate answer here.
– stepio
Dec 2 '18 at 13:35
Thank you for your hint, you was right that URL was wrong, but I did not understand why it was wrong before I dived into the AWS client's code. I'm going to paste my finding as a separate answer here.
– stepio
Dec 2 '18 at 13:35
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53471088%2finvoking-aws-lambda-endpoint%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
SZ7I0cvm EHZ H fJIhBTnvf6XNYxvw4Ape