Shadow Root getElementsByClassName
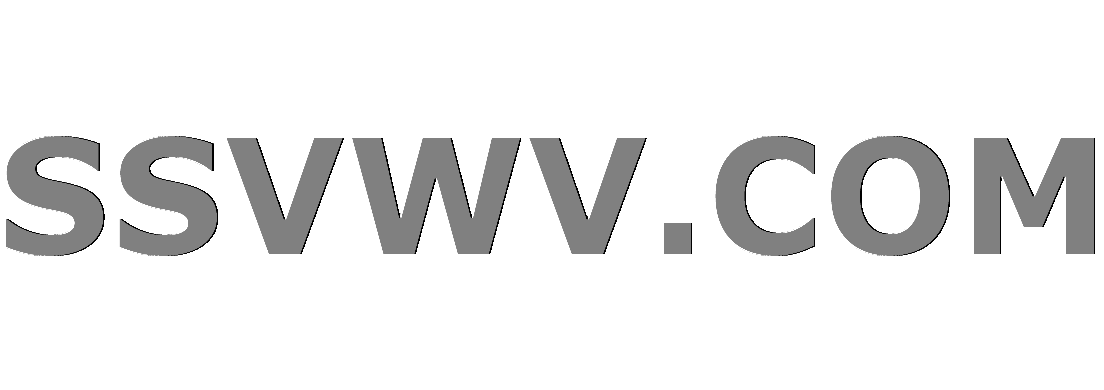
Multi tool use
I am using LitElement to create custom Web Components. I am fairly new at it and decided to try making image slideshow. I used W3Schools slideshow as reference
while modifying it to work as LitElement.
The problem is, that when I am trying to use document.getElementByClassName I am not getting anything. I am familiar with this issue since I am working with Shadow DOM so I changed it to this.shadowRoot.getElementsByClassName. Unfortunately, I get told that what I am trying to use is not a function. How Do I get elements by class name when I am working with LitElement and shadow dom? In case you want to see how my component looks like, here is the code:
import { LitElement, html} from '@polymer/lit-element';
class ImageGalleryElement extends LitElement {
static get properties() { return {
slideIndex: { type: Number },
}};
constructor(){
super();
this.slideIndex=1;
this.showSlides(this.slideIndex);
}
// Next/previous controls
plusSlides(n) {
this.showSlides(this.slideIndex += n);
}
// Thumbnail image controls
currentSlide(n) {
this.showSlides(this.slideIndex = n);
}
showSlides(n) {
var i;
console.dir(this.shadowRoot);
var slides = this.shadowRoot.getElementsByClassName("mySlides");
console.dir(slides);
var dots = this.shadowRoot.getElementsByClassName("dot");
if (n > slides.length) {this.slideIndex = 1}
if (n < 1) {this.slideIndex = slides.length}
for (i = 0; i < slides.length; i++) {
slides[i].style.display = "none";
}
for (i = 0; i < dots.length; i++) {
dots[i].className = dots[i].className.replace(" active", "");
}
slides[this.slideIndex-1].style.display = "block";
dots[this.slideIndex-1].className += " active";
}
render(){
return html`
<style>
* {box-sizing:border-box}
/* Slideshow container */
.slideshow-container {
max-width: 1000px;
position: relative;
margin: auto;
}
/* Hide the images by default */
.mySlides {
display: none;
}
/* Next & previous buttons */
.prev, .next {
cursor: pointer;
position: absolute;
top: 50%;
width: auto;
margin-top: -22px;
padding: 16px;
color: white;
font-weight: bold;
font-size: 18px;
transition: 0.6s ease;
border-radius: 0 3px 3px 0;
user-select: none;
}
/* Position the "next button" to the right */
.next {
right: 0;
border-radius: 3px 0 0 3px;
}
/* On hover, add a black background color with a little bit see-through */
.prev:hover, .next:hover {
background-color: rgba(0,0,0,0.8);
}
/* Caption text */
.text {
color: #f2f2f2;
font-size: 15px;
padding: 8px 12px;
position: absolute;
bottom: 8px;
width: 100%;
text-align: center;
}
/* Number text (1/3 etc) */
.numbertext {
color: #f2f2f2;
font-size: 12px;
padding: 8px 12px;
position: absolute;
top: 0;
}
/* The dots/bullets/indicators */
.dot {
cursor: pointer;
height: 15px;
width: 15px;
margin: 0 2px;
background-color: #bbb;
border-radius: 50%;
display: inline-block;
transition: background-color 0.6s ease;
}
.active, .dot:hover {
background-color: #717171;
}
/* Fading animation */
.fade {
-webkit-animation-name: fade;
-webkit-animation-duration: 1.5s;
animation-name: fade;
animation-duration: 1.5s;
}
@-webkit-keyframes fade {
from {opacity: .4}
to {opacity: 1}
}
@keyframes fade {
from {opacity: .4}
to {opacity: 1}
}
</style>
<!-- Slideshow container -->
<div class="slideshow-container">
<!-- Full-width images with number and caption text -->
<div class="mySlides fade">
<div class="numbertext">1 / 3</div>
<img src="../../img/img-snow-wide" style="width:100%">
<div class="text">Caption Text</div>
</div>
<div class="mySlides fade">
<div class="numbertext">2 / 3</div>
<img src="../../img/img-nature-wide" style="width:100%">
<div class="text">Caption Two</div>
</div>
<div class="mySlides fade">
<div class="numbertext">3 / 3</div>
<img src="../../img/img-mountains-wide" style="width:100%">
<div class="text">Caption Three</div>
</div>
<!-- Next and previous buttons -->
<a class="prev" @click="${this.plusSlides(-1)}" >❮</a>
<a class="next" @click="${this.plusSlides(1)}">❯</a>
</div>
<br>
<!-- The dots/circles -->
<div style="text-align:center">
<span class="dot" @click="${this.currentSlide(1)}"></span>
<span class="dot" @click="${this.currentSlide(2)}"></span>
<span class="dot" @click="${this.currentSlide(3)}"></span>
</div>
`;
}
}
// Register the element with the browser
customElements.define('image-gallery-element', ImageGalleryElement);
shadow-dom getelementsbyclassname lit-element
add a comment |
I am using LitElement to create custom Web Components. I am fairly new at it and decided to try making image slideshow. I used W3Schools slideshow as reference
while modifying it to work as LitElement.
The problem is, that when I am trying to use document.getElementByClassName I am not getting anything. I am familiar with this issue since I am working with Shadow DOM so I changed it to this.shadowRoot.getElementsByClassName. Unfortunately, I get told that what I am trying to use is not a function. How Do I get elements by class name when I am working with LitElement and shadow dom? In case you want to see how my component looks like, here is the code:
import { LitElement, html} from '@polymer/lit-element';
class ImageGalleryElement extends LitElement {
static get properties() { return {
slideIndex: { type: Number },
}};
constructor(){
super();
this.slideIndex=1;
this.showSlides(this.slideIndex);
}
// Next/previous controls
plusSlides(n) {
this.showSlides(this.slideIndex += n);
}
// Thumbnail image controls
currentSlide(n) {
this.showSlides(this.slideIndex = n);
}
showSlides(n) {
var i;
console.dir(this.shadowRoot);
var slides = this.shadowRoot.getElementsByClassName("mySlides");
console.dir(slides);
var dots = this.shadowRoot.getElementsByClassName("dot");
if (n > slides.length) {this.slideIndex = 1}
if (n < 1) {this.slideIndex = slides.length}
for (i = 0; i < slides.length; i++) {
slides[i].style.display = "none";
}
for (i = 0; i < dots.length; i++) {
dots[i].className = dots[i].className.replace(" active", "");
}
slides[this.slideIndex-1].style.display = "block";
dots[this.slideIndex-1].className += " active";
}
render(){
return html`
<style>
* {box-sizing:border-box}
/* Slideshow container */
.slideshow-container {
max-width: 1000px;
position: relative;
margin: auto;
}
/* Hide the images by default */
.mySlides {
display: none;
}
/* Next & previous buttons */
.prev, .next {
cursor: pointer;
position: absolute;
top: 50%;
width: auto;
margin-top: -22px;
padding: 16px;
color: white;
font-weight: bold;
font-size: 18px;
transition: 0.6s ease;
border-radius: 0 3px 3px 0;
user-select: none;
}
/* Position the "next button" to the right */
.next {
right: 0;
border-radius: 3px 0 0 3px;
}
/* On hover, add a black background color with a little bit see-through */
.prev:hover, .next:hover {
background-color: rgba(0,0,0,0.8);
}
/* Caption text */
.text {
color: #f2f2f2;
font-size: 15px;
padding: 8px 12px;
position: absolute;
bottom: 8px;
width: 100%;
text-align: center;
}
/* Number text (1/3 etc) */
.numbertext {
color: #f2f2f2;
font-size: 12px;
padding: 8px 12px;
position: absolute;
top: 0;
}
/* The dots/bullets/indicators */
.dot {
cursor: pointer;
height: 15px;
width: 15px;
margin: 0 2px;
background-color: #bbb;
border-radius: 50%;
display: inline-block;
transition: background-color 0.6s ease;
}
.active, .dot:hover {
background-color: #717171;
}
/* Fading animation */
.fade {
-webkit-animation-name: fade;
-webkit-animation-duration: 1.5s;
animation-name: fade;
animation-duration: 1.5s;
}
@-webkit-keyframes fade {
from {opacity: .4}
to {opacity: 1}
}
@keyframes fade {
from {opacity: .4}
to {opacity: 1}
}
</style>
<!-- Slideshow container -->
<div class="slideshow-container">
<!-- Full-width images with number and caption text -->
<div class="mySlides fade">
<div class="numbertext">1 / 3</div>
<img src="../../img/img-snow-wide" style="width:100%">
<div class="text">Caption Text</div>
</div>
<div class="mySlides fade">
<div class="numbertext">2 / 3</div>
<img src="../../img/img-nature-wide" style="width:100%">
<div class="text">Caption Two</div>
</div>
<div class="mySlides fade">
<div class="numbertext">3 / 3</div>
<img src="../../img/img-mountains-wide" style="width:100%">
<div class="text">Caption Three</div>
</div>
<!-- Next and previous buttons -->
<a class="prev" @click="${this.plusSlides(-1)}" >❮</a>
<a class="next" @click="${this.plusSlides(1)}">❯</a>
</div>
<br>
<!-- The dots/circles -->
<div style="text-align:center">
<span class="dot" @click="${this.currentSlide(1)}"></span>
<span class="dot" @click="${this.currentSlide(2)}"></span>
<span class="dot" @click="${this.currentSlide(3)}"></span>
</div>
`;
}
}
// Register the element with the browser
customElements.define('image-gallery-element', ImageGalleryElement);
shadow-dom getelementsbyclassname lit-element
add a comment |
I am using LitElement to create custom Web Components. I am fairly new at it and decided to try making image slideshow. I used W3Schools slideshow as reference
while modifying it to work as LitElement.
The problem is, that when I am trying to use document.getElementByClassName I am not getting anything. I am familiar with this issue since I am working with Shadow DOM so I changed it to this.shadowRoot.getElementsByClassName. Unfortunately, I get told that what I am trying to use is not a function. How Do I get elements by class name when I am working with LitElement and shadow dom? In case you want to see how my component looks like, here is the code:
import { LitElement, html} from '@polymer/lit-element';
class ImageGalleryElement extends LitElement {
static get properties() { return {
slideIndex: { type: Number },
}};
constructor(){
super();
this.slideIndex=1;
this.showSlides(this.slideIndex);
}
// Next/previous controls
plusSlides(n) {
this.showSlides(this.slideIndex += n);
}
// Thumbnail image controls
currentSlide(n) {
this.showSlides(this.slideIndex = n);
}
showSlides(n) {
var i;
console.dir(this.shadowRoot);
var slides = this.shadowRoot.getElementsByClassName("mySlides");
console.dir(slides);
var dots = this.shadowRoot.getElementsByClassName("dot");
if (n > slides.length) {this.slideIndex = 1}
if (n < 1) {this.slideIndex = slides.length}
for (i = 0; i < slides.length; i++) {
slides[i].style.display = "none";
}
for (i = 0; i < dots.length; i++) {
dots[i].className = dots[i].className.replace(" active", "");
}
slides[this.slideIndex-1].style.display = "block";
dots[this.slideIndex-1].className += " active";
}
render(){
return html`
<style>
* {box-sizing:border-box}
/* Slideshow container */
.slideshow-container {
max-width: 1000px;
position: relative;
margin: auto;
}
/* Hide the images by default */
.mySlides {
display: none;
}
/* Next & previous buttons */
.prev, .next {
cursor: pointer;
position: absolute;
top: 50%;
width: auto;
margin-top: -22px;
padding: 16px;
color: white;
font-weight: bold;
font-size: 18px;
transition: 0.6s ease;
border-radius: 0 3px 3px 0;
user-select: none;
}
/* Position the "next button" to the right */
.next {
right: 0;
border-radius: 3px 0 0 3px;
}
/* On hover, add a black background color with a little bit see-through */
.prev:hover, .next:hover {
background-color: rgba(0,0,0,0.8);
}
/* Caption text */
.text {
color: #f2f2f2;
font-size: 15px;
padding: 8px 12px;
position: absolute;
bottom: 8px;
width: 100%;
text-align: center;
}
/* Number text (1/3 etc) */
.numbertext {
color: #f2f2f2;
font-size: 12px;
padding: 8px 12px;
position: absolute;
top: 0;
}
/* The dots/bullets/indicators */
.dot {
cursor: pointer;
height: 15px;
width: 15px;
margin: 0 2px;
background-color: #bbb;
border-radius: 50%;
display: inline-block;
transition: background-color 0.6s ease;
}
.active, .dot:hover {
background-color: #717171;
}
/* Fading animation */
.fade {
-webkit-animation-name: fade;
-webkit-animation-duration: 1.5s;
animation-name: fade;
animation-duration: 1.5s;
}
@-webkit-keyframes fade {
from {opacity: .4}
to {opacity: 1}
}
@keyframes fade {
from {opacity: .4}
to {opacity: 1}
}
</style>
<!-- Slideshow container -->
<div class="slideshow-container">
<!-- Full-width images with number and caption text -->
<div class="mySlides fade">
<div class="numbertext">1 / 3</div>
<img src="../../img/img-snow-wide" style="width:100%">
<div class="text">Caption Text</div>
</div>
<div class="mySlides fade">
<div class="numbertext">2 / 3</div>
<img src="../../img/img-nature-wide" style="width:100%">
<div class="text">Caption Two</div>
</div>
<div class="mySlides fade">
<div class="numbertext">3 / 3</div>
<img src="../../img/img-mountains-wide" style="width:100%">
<div class="text">Caption Three</div>
</div>
<!-- Next and previous buttons -->
<a class="prev" @click="${this.plusSlides(-1)}" >❮</a>
<a class="next" @click="${this.plusSlides(1)}">❯</a>
</div>
<br>
<!-- The dots/circles -->
<div style="text-align:center">
<span class="dot" @click="${this.currentSlide(1)}"></span>
<span class="dot" @click="${this.currentSlide(2)}"></span>
<span class="dot" @click="${this.currentSlide(3)}"></span>
</div>
`;
}
}
// Register the element with the browser
customElements.define('image-gallery-element', ImageGalleryElement);
shadow-dom getelementsbyclassname lit-element
I am using LitElement to create custom Web Components. I am fairly new at it and decided to try making image slideshow. I used W3Schools slideshow as reference
while modifying it to work as LitElement.
The problem is, that when I am trying to use document.getElementByClassName I am not getting anything. I am familiar with this issue since I am working with Shadow DOM so I changed it to this.shadowRoot.getElementsByClassName. Unfortunately, I get told that what I am trying to use is not a function. How Do I get elements by class name when I am working with LitElement and shadow dom? In case you want to see how my component looks like, here is the code:
import { LitElement, html} from '@polymer/lit-element';
class ImageGalleryElement extends LitElement {
static get properties() { return {
slideIndex: { type: Number },
}};
constructor(){
super();
this.slideIndex=1;
this.showSlides(this.slideIndex);
}
// Next/previous controls
plusSlides(n) {
this.showSlides(this.slideIndex += n);
}
// Thumbnail image controls
currentSlide(n) {
this.showSlides(this.slideIndex = n);
}
showSlides(n) {
var i;
console.dir(this.shadowRoot);
var slides = this.shadowRoot.getElementsByClassName("mySlides");
console.dir(slides);
var dots = this.shadowRoot.getElementsByClassName("dot");
if (n > slides.length) {this.slideIndex = 1}
if (n < 1) {this.slideIndex = slides.length}
for (i = 0; i < slides.length; i++) {
slides[i].style.display = "none";
}
for (i = 0; i < dots.length; i++) {
dots[i].className = dots[i].className.replace(" active", "");
}
slides[this.slideIndex-1].style.display = "block";
dots[this.slideIndex-1].className += " active";
}
render(){
return html`
<style>
* {box-sizing:border-box}
/* Slideshow container */
.slideshow-container {
max-width: 1000px;
position: relative;
margin: auto;
}
/* Hide the images by default */
.mySlides {
display: none;
}
/* Next & previous buttons */
.prev, .next {
cursor: pointer;
position: absolute;
top: 50%;
width: auto;
margin-top: -22px;
padding: 16px;
color: white;
font-weight: bold;
font-size: 18px;
transition: 0.6s ease;
border-radius: 0 3px 3px 0;
user-select: none;
}
/* Position the "next button" to the right */
.next {
right: 0;
border-radius: 3px 0 0 3px;
}
/* On hover, add a black background color with a little bit see-through */
.prev:hover, .next:hover {
background-color: rgba(0,0,0,0.8);
}
/* Caption text */
.text {
color: #f2f2f2;
font-size: 15px;
padding: 8px 12px;
position: absolute;
bottom: 8px;
width: 100%;
text-align: center;
}
/* Number text (1/3 etc) */
.numbertext {
color: #f2f2f2;
font-size: 12px;
padding: 8px 12px;
position: absolute;
top: 0;
}
/* The dots/bullets/indicators */
.dot {
cursor: pointer;
height: 15px;
width: 15px;
margin: 0 2px;
background-color: #bbb;
border-radius: 50%;
display: inline-block;
transition: background-color 0.6s ease;
}
.active, .dot:hover {
background-color: #717171;
}
/* Fading animation */
.fade {
-webkit-animation-name: fade;
-webkit-animation-duration: 1.5s;
animation-name: fade;
animation-duration: 1.5s;
}
@-webkit-keyframes fade {
from {opacity: .4}
to {opacity: 1}
}
@keyframes fade {
from {opacity: .4}
to {opacity: 1}
}
</style>
<!-- Slideshow container -->
<div class="slideshow-container">
<!-- Full-width images with number and caption text -->
<div class="mySlides fade">
<div class="numbertext">1 / 3</div>
<img src="../../img/img-snow-wide" style="width:100%">
<div class="text">Caption Text</div>
</div>
<div class="mySlides fade">
<div class="numbertext">2 / 3</div>
<img src="../../img/img-nature-wide" style="width:100%">
<div class="text">Caption Two</div>
</div>
<div class="mySlides fade">
<div class="numbertext">3 / 3</div>
<img src="../../img/img-mountains-wide" style="width:100%">
<div class="text">Caption Three</div>
</div>
<!-- Next and previous buttons -->
<a class="prev" @click="${this.plusSlides(-1)}" >❮</a>
<a class="next" @click="${this.plusSlides(1)}">❯</a>
</div>
<br>
<!-- The dots/circles -->
<div style="text-align:center">
<span class="dot" @click="${this.currentSlide(1)}"></span>
<span class="dot" @click="${this.currentSlide(2)}"></span>
<span class="dot" @click="${this.currentSlide(3)}"></span>
</div>
`;
}
}
// Register the element with the browser
customElements.define('image-gallery-element', ImageGalleryElement);
shadow-dom getelementsbyclassname lit-element
shadow-dom getelementsbyclassname lit-element
edited Nov 24 '18 at 19:40
Filip Skukan
asked Nov 24 '18 at 17:46


Filip SkukanFilip Skukan
8917
8917
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
The getElementsByClassName()
method works only on a HTML Document or element.
The shadowRoot
is a Document Fragment, not a Document nor a HTML element.
Instead you should use querySelectorAll()
.
I changed it to var slides = this.shadowRoot.querySelectorAll(".mySlides"); but when I log it I have an empty list. That causes me to get an error: Cannot read property 'style' of undefined when I try to access the style property of the slides.
– Filip Skukan
Nov 25 '18 at 13:18
2
Oh, i see, I am immediately invoking the click event by writing: @click="${this.plusSlides(-1)}" in the render method. I changed it to @click="${(e) => { this.plusSlides(-1); }}" and changed getElementByClassName() with querySelectorAll() and now it works great!
– Filip Skukan
Nov 25 '18 at 13:32
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53460850%2fshadow-root-getelementsbyclassname%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
The getElementsByClassName()
method works only on a HTML Document or element.
The shadowRoot
is a Document Fragment, not a Document nor a HTML element.
Instead you should use querySelectorAll()
.
I changed it to var slides = this.shadowRoot.querySelectorAll(".mySlides"); but when I log it I have an empty list. That causes me to get an error: Cannot read property 'style' of undefined when I try to access the style property of the slides.
– Filip Skukan
Nov 25 '18 at 13:18
2
Oh, i see, I am immediately invoking the click event by writing: @click="${this.plusSlides(-1)}" in the render method. I changed it to @click="${(e) => { this.plusSlides(-1); }}" and changed getElementByClassName() with querySelectorAll() and now it works great!
– Filip Skukan
Nov 25 '18 at 13:32
add a comment |
The getElementsByClassName()
method works only on a HTML Document or element.
The shadowRoot
is a Document Fragment, not a Document nor a HTML element.
Instead you should use querySelectorAll()
.
I changed it to var slides = this.shadowRoot.querySelectorAll(".mySlides"); but when I log it I have an empty list. That causes me to get an error: Cannot read property 'style' of undefined when I try to access the style property of the slides.
– Filip Skukan
Nov 25 '18 at 13:18
2
Oh, i see, I am immediately invoking the click event by writing: @click="${this.plusSlides(-1)}" in the render method. I changed it to @click="${(e) => { this.plusSlides(-1); }}" and changed getElementByClassName() with querySelectorAll() and now it works great!
– Filip Skukan
Nov 25 '18 at 13:32
add a comment |
The getElementsByClassName()
method works only on a HTML Document or element.
The shadowRoot
is a Document Fragment, not a Document nor a HTML element.
Instead you should use querySelectorAll()
.
The getElementsByClassName()
method works only on a HTML Document or element.
The shadowRoot
is a Document Fragment, not a Document nor a HTML element.
Instead you should use querySelectorAll()
.
edited Nov 24 '18 at 23:32
answered Nov 24 '18 at 23:19


SupersharpSupersharp
14.1k23071
14.1k23071
I changed it to var slides = this.shadowRoot.querySelectorAll(".mySlides"); but when I log it I have an empty list. That causes me to get an error: Cannot read property 'style' of undefined when I try to access the style property of the slides.
– Filip Skukan
Nov 25 '18 at 13:18
2
Oh, i see, I am immediately invoking the click event by writing: @click="${this.plusSlides(-1)}" in the render method. I changed it to @click="${(e) => { this.plusSlides(-1); }}" and changed getElementByClassName() with querySelectorAll() and now it works great!
– Filip Skukan
Nov 25 '18 at 13:32
add a comment |
I changed it to var slides = this.shadowRoot.querySelectorAll(".mySlides"); but when I log it I have an empty list. That causes me to get an error: Cannot read property 'style' of undefined when I try to access the style property of the slides.
– Filip Skukan
Nov 25 '18 at 13:18
2
Oh, i see, I am immediately invoking the click event by writing: @click="${this.plusSlides(-1)}" in the render method. I changed it to @click="${(e) => { this.plusSlides(-1); }}" and changed getElementByClassName() with querySelectorAll() and now it works great!
– Filip Skukan
Nov 25 '18 at 13:32
I changed it to var slides = this.shadowRoot.querySelectorAll(".mySlides"); but when I log it I have an empty list. That causes me to get an error: Cannot read property 'style' of undefined when I try to access the style property of the slides.
– Filip Skukan
Nov 25 '18 at 13:18
I changed it to var slides = this.shadowRoot.querySelectorAll(".mySlides"); but when I log it I have an empty list. That causes me to get an error: Cannot read property 'style' of undefined when I try to access the style property of the slides.
– Filip Skukan
Nov 25 '18 at 13:18
2
2
Oh, i see, I am immediately invoking the click event by writing: @click="${this.plusSlides(-1)}" in the render method. I changed it to @click="${(e) => { this.plusSlides(-1); }}" and changed getElementByClassName() with querySelectorAll() and now it works great!
– Filip Skukan
Nov 25 '18 at 13:32
Oh, i see, I am immediately invoking the click event by writing: @click="${this.plusSlides(-1)}" in the render method. I changed it to @click="${(e) => { this.plusSlides(-1); }}" and changed getElementByClassName() with querySelectorAll() and now it works great!
– Filip Skukan
Nov 25 '18 at 13:32
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53460850%2fshadow-root-getelementsbyclassname%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
pDh,hRf0 J4S8ORWu6a,T n3Rzj,3,4D,5r,64Jk x,iiaSQC,Z7hJMiT,AKKy9w z44zyx0hX,G4EPhK l HYTIAv