JQuery : How to add list of objects in Ajax
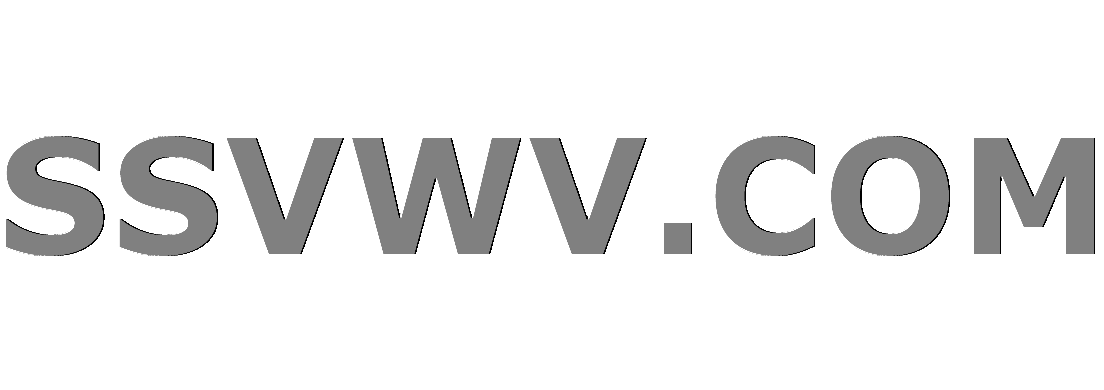
Multi tool use
up vote
0
down vote
favorite
It's one of my first time I using JQuery/AJAX
and I would like to get your help in order to give a list of objects in my dropdown field.
I have this function :
function get_sub_method_options(keep_cur) {
var sel_option = $('select#id_method-group').find("option:selected");
var sel_val = sel_option.val();
console.log(sel_val);
if (sel_val === '') {
console.log('none sorting group');
$("select#id_method-sub_method").empty();
$("select#id_method-sub_method").append('<option value=""> test </option>'); //here add list of all submethods
return;
}
data = {
'test_method': $('select#id_method-test_method').find("option:selected").val(),
'group': sel_val
};
$.ajax({
method: "GET",
url: '{% url 'ajax_method_submethod' %}',
data: data
}).done(function (result) {
reset_select('id_method-sub_method');
for (var i = 0; i < result['results'].length; i++) {
if (keep_cur > 0 & keep_cur == result['results'][i].id)
$("select#id_method-sub_method").append('<option value="' + result['results'][i].id + '" selected>' + result['results'][i].text + '</option>');
else
$("select#id_method-sub_method").append('<option value="' + result['results'][i].id + '">' + result['results'][i].text + '</option>');
}
;
});
}
And I have a Python/Django function :
def ajax_method_submethod(request):
test_method_id = request.GET.get("test_method", "")
group_id = request.GET.get("group", "")
sub_methods_all = SubMethod.objects.all()
sub_methods = Method.objects.filter(test_method_id=test_method_id).filter(
group_id=group_id
)
results2 =
for item in sub_methods_all:
results2.append({'id': item.id, 'text': item.name})
print(results2)
results =
for item in sub_methods:
results.append({'id': item.sub_method.id, 'text': item.sub_method.name})
return HttpResponse(json.dumps({'err': 'nil', 'results': results, 'results2': results2}), content_type='application/json')
I would like to import into my dropdown field values from results2
(python file) when I have sel_val
is empty :
if (sel_val === '') {
...
}
So how I could rewrite this part :
if (sel_val === '') {
console.log('none sorting group');
$("select#id_method-sub_method").empty();
$("select#id_method-sub_method").append('<option value=""> test </option>'); //here add list of all submethods
return;
}
Like this :
if (sel_val === '') {
$("select#id_method-sub_method").empty();
for (var i = 0; i < result['results2'].length; i++) {
$("select#id_method-sub_method").append('<option value="' + result['results2'][i].id + '">' + result['results2'][i].text + '</option>'); //here add list of all submethods
}
return;
}
with for loop
and append all elements from results2
to my dropdown field ? The issue is actually with result['results2']
Hopfully it's readable and understandable.
Thank you !
EDIT :
As @Darren Crabb said, it's a possible out of scope.
So I rewrote my JS like this :
if (!sel_val) {
reset_select('id_method-sub_method');
$("select#id_method-sub_method").empty();
var all = "{{ results2 }}";
for (var i = 0; i < all.length; i++) {
$("select#id_method-sub_method").append('<option value="' + all[i].id + '">' + all[i].text + '</option>'); //here add list of all submethods
}
return;
}
And I defined in my python file : context['results2'] = SubMethod.objects.all()
I get this :
javascript jquery ajax
|
show 2 more comments
up vote
0
down vote
favorite
It's one of my first time I using JQuery/AJAX
and I would like to get your help in order to give a list of objects in my dropdown field.
I have this function :
function get_sub_method_options(keep_cur) {
var sel_option = $('select#id_method-group').find("option:selected");
var sel_val = sel_option.val();
console.log(sel_val);
if (sel_val === '') {
console.log('none sorting group');
$("select#id_method-sub_method").empty();
$("select#id_method-sub_method").append('<option value=""> test </option>'); //here add list of all submethods
return;
}
data = {
'test_method': $('select#id_method-test_method').find("option:selected").val(),
'group': sel_val
};
$.ajax({
method: "GET",
url: '{% url 'ajax_method_submethod' %}',
data: data
}).done(function (result) {
reset_select('id_method-sub_method');
for (var i = 0; i < result['results'].length; i++) {
if (keep_cur > 0 & keep_cur == result['results'][i].id)
$("select#id_method-sub_method").append('<option value="' + result['results'][i].id + '" selected>' + result['results'][i].text + '</option>');
else
$("select#id_method-sub_method").append('<option value="' + result['results'][i].id + '">' + result['results'][i].text + '</option>');
}
;
});
}
And I have a Python/Django function :
def ajax_method_submethod(request):
test_method_id = request.GET.get("test_method", "")
group_id = request.GET.get("group", "")
sub_methods_all = SubMethod.objects.all()
sub_methods = Method.objects.filter(test_method_id=test_method_id).filter(
group_id=group_id
)
results2 =
for item in sub_methods_all:
results2.append({'id': item.id, 'text': item.name})
print(results2)
results =
for item in sub_methods:
results.append({'id': item.sub_method.id, 'text': item.sub_method.name})
return HttpResponse(json.dumps({'err': 'nil', 'results': results, 'results2': results2}), content_type='application/json')
I would like to import into my dropdown field values from results2
(python file) when I have sel_val
is empty :
if (sel_val === '') {
...
}
So how I could rewrite this part :
if (sel_val === '') {
console.log('none sorting group');
$("select#id_method-sub_method").empty();
$("select#id_method-sub_method").append('<option value=""> test </option>'); //here add list of all submethods
return;
}
Like this :
if (sel_val === '') {
$("select#id_method-sub_method").empty();
for (var i = 0; i < result['results2'].length; i++) {
$("select#id_method-sub_method").append('<option value="' + result['results2'][i].id + '">' + result['results2'][i].text + '</option>'); //here add list of all submethods
}
return;
}
with for loop
and append all elements from results2
to my dropdown field ? The issue is actually with result['results2']
Hopfully it's readable and understandable.
Thank you !
EDIT :
As @Darren Crabb said, it's a possible out of scope.
So I rewrote my JS like this :
if (!sel_val) {
reset_select('id_method-sub_method');
$("select#id_method-sub_method").empty();
var all = "{{ results2 }}";
for (var i = 0; i < all.length; i++) {
$("select#id_method-sub_method").append('<option value="' + all[i].id + '">' + all[i].text + '</option>'); //here add list of all submethods
}
return;
}
And I defined in my python file : context['results2'] = SubMethod.objects.all()
I get this :
javascript jquery ajax
What is wrong with the "Like this" approach shown? SHow us whatresult['results2']
looks like
– charlietfl
Nov 19 at 15:27
1
"The issue is actually with result['results2']"...and what exactly is the issue? What goes wrong?
– ADyson
Nov 19 at 15:27
I get an issue in my console :result is not defined
. I don't know where I have to set this piece of code. Furthermore, I don't know if it's correct to make things like this because it's my first JQuery/Ajax code. In my python file,results2 = [{'id': 449, 'text': '50% cell culture infective dose (CCID50)'}, {'id': 176, 'text': 'Acetic acid in synthetic peptides'}, ...
– Ducky
Nov 19 at 15:30
Might be worth checking the network activity in your console, especially on the file being accessed by the ajax call. This should show you the headers, response etc. for each file listed (if you click on them) where you can check to make sure the correct results are being passed back to your client-side code. This happens in Firefox console at least - not sure about other browsers. That often helps a lot when working with ajax.
– Darren Crabb
Nov 19 at 16:13
1
It looks like you're trying to access 'result' out of scope. As far as I can tell result is only defined in the return callback of the ajax function where it will contain the response of the call, it's not defined anywhere in the top part of the code.
– Darren Crabb
Nov 19 at 16:25
|
show 2 more comments
up vote
0
down vote
favorite
up vote
0
down vote
favorite
It's one of my first time I using JQuery/AJAX
and I would like to get your help in order to give a list of objects in my dropdown field.
I have this function :
function get_sub_method_options(keep_cur) {
var sel_option = $('select#id_method-group').find("option:selected");
var sel_val = sel_option.val();
console.log(sel_val);
if (sel_val === '') {
console.log('none sorting group');
$("select#id_method-sub_method").empty();
$("select#id_method-sub_method").append('<option value=""> test </option>'); //here add list of all submethods
return;
}
data = {
'test_method': $('select#id_method-test_method').find("option:selected").val(),
'group': sel_val
};
$.ajax({
method: "GET",
url: '{% url 'ajax_method_submethod' %}',
data: data
}).done(function (result) {
reset_select('id_method-sub_method');
for (var i = 0; i < result['results'].length; i++) {
if (keep_cur > 0 & keep_cur == result['results'][i].id)
$("select#id_method-sub_method").append('<option value="' + result['results'][i].id + '" selected>' + result['results'][i].text + '</option>');
else
$("select#id_method-sub_method").append('<option value="' + result['results'][i].id + '">' + result['results'][i].text + '</option>');
}
;
});
}
And I have a Python/Django function :
def ajax_method_submethod(request):
test_method_id = request.GET.get("test_method", "")
group_id = request.GET.get("group", "")
sub_methods_all = SubMethod.objects.all()
sub_methods = Method.objects.filter(test_method_id=test_method_id).filter(
group_id=group_id
)
results2 =
for item in sub_methods_all:
results2.append({'id': item.id, 'text': item.name})
print(results2)
results =
for item in sub_methods:
results.append({'id': item.sub_method.id, 'text': item.sub_method.name})
return HttpResponse(json.dumps({'err': 'nil', 'results': results, 'results2': results2}), content_type='application/json')
I would like to import into my dropdown field values from results2
(python file) when I have sel_val
is empty :
if (sel_val === '') {
...
}
So how I could rewrite this part :
if (sel_val === '') {
console.log('none sorting group');
$("select#id_method-sub_method").empty();
$("select#id_method-sub_method").append('<option value=""> test </option>'); //here add list of all submethods
return;
}
Like this :
if (sel_val === '') {
$("select#id_method-sub_method").empty();
for (var i = 0; i < result['results2'].length; i++) {
$("select#id_method-sub_method").append('<option value="' + result['results2'][i].id + '">' + result['results2'][i].text + '</option>'); //here add list of all submethods
}
return;
}
with for loop
and append all elements from results2
to my dropdown field ? The issue is actually with result['results2']
Hopfully it's readable and understandable.
Thank you !
EDIT :
As @Darren Crabb said, it's a possible out of scope.
So I rewrote my JS like this :
if (!sel_val) {
reset_select('id_method-sub_method');
$("select#id_method-sub_method").empty();
var all = "{{ results2 }}";
for (var i = 0; i < all.length; i++) {
$("select#id_method-sub_method").append('<option value="' + all[i].id + '">' + all[i].text + '</option>'); //here add list of all submethods
}
return;
}
And I defined in my python file : context['results2'] = SubMethod.objects.all()
I get this :
javascript jquery ajax
It's one of my first time I using JQuery/AJAX
and I would like to get your help in order to give a list of objects in my dropdown field.
I have this function :
function get_sub_method_options(keep_cur) {
var sel_option = $('select#id_method-group').find("option:selected");
var sel_val = sel_option.val();
console.log(sel_val);
if (sel_val === '') {
console.log('none sorting group');
$("select#id_method-sub_method").empty();
$("select#id_method-sub_method").append('<option value=""> test </option>'); //here add list of all submethods
return;
}
data = {
'test_method': $('select#id_method-test_method').find("option:selected").val(),
'group': sel_val
};
$.ajax({
method: "GET",
url: '{% url 'ajax_method_submethod' %}',
data: data
}).done(function (result) {
reset_select('id_method-sub_method');
for (var i = 0; i < result['results'].length; i++) {
if (keep_cur > 0 & keep_cur == result['results'][i].id)
$("select#id_method-sub_method").append('<option value="' + result['results'][i].id + '" selected>' + result['results'][i].text + '</option>');
else
$("select#id_method-sub_method").append('<option value="' + result['results'][i].id + '">' + result['results'][i].text + '</option>');
}
;
});
}
And I have a Python/Django function :
def ajax_method_submethod(request):
test_method_id = request.GET.get("test_method", "")
group_id = request.GET.get("group", "")
sub_methods_all = SubMethod.objects.all()
sub_methods = Method.objects.filter(test_method_id=test_method_id).filter(
group_id=group_id
)
results2 =
for item in sub_methods_all:
results2.append({'id': item.id, 'text': item.name})
print(results2)
results =
for item in sub_methods:
results.append({'id': item.sub_method.id, 'text': item.sub_method.name})
return HttpResponse(json.dumps({'err': 'nil', 'results': results, 'results2': results2}), content_type='application/json')
I would like to import into my dropdown field values from results2
(python file) when I have sel_val
is empty :
if (sel_val === '') {
...
}
So how I could rewrite this part :
if (sel_val === '') {
console.log('none sorting group');
$("select#id_method-sub_method").empty();
$("select#id_method-sub_method").append('<option value=""> test </option>'); //here add list of all submethods
return;
}
Like this :
if (sel_val === '') {
$("select#id_method-sub_method").empty();
for (var i = 0; i < result['results2'].length; i++) {
$("select#id_method-sub_method").append('<option value="' + result['results2'][i].id + '">' + result['results2'][i].text + '</option>'); //here add list of all submethods
}
return;
}
with for loop
and append all elements from results2
to my dropdown field ? The issue is actually with result['results2']
Hopfully it's readable and understandable.
Thank you !
EDIT :
As @Darren Crabb said, it's a possible out of scope.
So I rewrote my JS like this :
if (!sel_val) {
reset_select('id_method-sub_method');
$("select#id_method-sub_method").empty();
var all = "{{ results2 }}";
for (var i = 0; i < all.length; i++) {
$("select#id_method-sub_method").append('<option value="' + all[i].id + '">' + all[i].text + '</option>'); //here add list of all submethods
}
return;
}
And I defined in my python file : context['results2'] = SubMethod.objects.all()
I get this :
javascript jquery ajax
javascript jquery ajax
edited Nov 19 at 16:45
asked Nov 19 at 15:22


Ducky
2,52011552
2,52011552
What is wrong with the "Like this" approach shown? SHow us whatresult['results2']
looks like
– charlietfl
Nov 19 at 15:27
1
"The issue is actually with result['results2']"...and what exactly is the issue? What goes wrong?
– ADyson
Nov 19 at 15:27
I get an issue in my console :result is not defined
. I don't know where I have to set this piece of code. Furthermore, I don't know if it's correct to make things like this because it's my first JQuery/Ajax code. In my python file,results2 = [{'id': 449, 'text': '50% cell culture infective dose (CCID50)'}, {'id': 176, 'text': 'Acetic acid in synthetic peptides'}, ...
– Ducky
Nov 19 at 15:30
Might be worth checking the network activity in your console, especially on the file being accessed by the ajax call. This should show you the headers, response etc. for each file listed (if you click on them) where you can check to make sure the correct results are being passed back to your client-side code. This happens in Firefox console at least - not sure about other browsers. That often helps a lot when working with ajax.
– Darren Crabb
Nov 19 at 16:13
1
It looks like you're trying to access 'result' out of scope. As far as I can tell result is only defined in the return callback of the ajax function where it will contain the response of the call, it's not defined anywhere in the top part of the code.
– Darren Crabb
Nov 19 at 16:25
|
show 2 more comments
What is wrong with the "Like this" approach shown? SHow us whatresult['results2']
looks like
– charlietfl
Nov 19 at 15:27
1
"The issue is actually with result['results2']"...and what exactly is the issue? What goes wrong?
– ADyson
Nov 19 at 15:27
I get an issue in my console :result is not defined
. I don't know where I have to set this piece of code. Furthermore, I don't know if it's correct to make things like this because it's my first JQuery/Ajax code. In my python file,results2 = [{'id': 449, 'text': '50% cell culture infective dose (CCID50)'}, {'id': 176, 'text': 'Acetic acid in synthetic peptides'}, ...
– Ducky
Nov 19 at 15:30
Might be worth checking the network activity in your console, especially on the file being accessed by the ajax call. This should show you the headers, response etc. for each file listed (if you click on them) where you can check to make sure the correct results are being passed back to your client-side code. This happens in Firefox console at least - not sure about other browsers. That often helps a lot when working with ajax.
– Darren Crabb
Nov 19 at 16:13
1
It looks like you're trying to access 'result' out of scope. As far as I can tell result is only defined in the return callback of the ajax function where it will contain the response of the call, it's not defined anywhere in the top part of the code.
– Darren Crabb
Nov 19 at 16:25
What is wrong with the "Like this" approach shown? SHow us what
result['results2']
looks like– charlietfl
Nov 19 at 15:27
What is wrong with the "Like this" approach shown? SHow us what
result['results2']
looks like– charlietfl
Nov 19 at 15:27
1
1
"The issue is actually with result['results2']"...and what exactly is the issue? What goes wrong?
– ADyson
Nov 19 at 15:27
"The issue is actually with result['results2']"...and what exactly is the issue? What goes wrong?
– ADyson
Nov 19 at 15:27
I get an issue in my console :
result is not defined
. I don't know where I have to set this piece of code. Furthermore, I don't know if it's correct to make things like this because it's my first JQuery/Ajax code. In my python file, results2 = [{'id': 449, 'text': '50% cell culture infective dose (CCID50)'}, {'id': 176, 'text': 'Acetic acid in synthetic peptides'}, ...
– Ducky
Nov 19 at 15:30
I get an issue in my console :
result is not defined
. I don't know where I have to set this piece of code. Furthermore, I don't know if it's correct to make things like this because it's my first JQuery/Ajax code. In my python file, results2 = [{'id': 449, 'text': '50% cell culture infective dose (CCID50)'}, {'id': 176, 'text': 'Acetic acid in synthetic peptides'}, ...
– Ducky
Nov 19 at 15:30
Might be worth checking the network activity in your console, especially on the file being accessed by the ajax call. This should show you the headers, response etc. for each file listed (if you click on them) where you can check to make sure the correct results are being passed back to your client-side code. This happens in Firefox console at least - not sure about other browsers. That often helps a lot when working with ajax.
– Darren Crabb
Nov 19 at 16:13
Might be worth checking the network activity in your console, especially on the file being accessed by the ajax call. This should show you the headers, response etc. for each file listed (if you click on them) where you can check to make sure the correct results are being passed back to your client-side code. This happens in Firefox console at least - not sure about other browsers. That often helps a lot when working with ajax.
– Darren Crabb
Nov 19 at 16:13
1
1
It looks like you're trying to access 'result' out of scope. As far as I can tell result is only defined in the return callback of the ajax function where it will contain the response of the call, it's not defined anywhere in the top part of the code.
– Darren Crabb
Nov 19 at 16:25
It looks like you're trying to access 'result' out of scope. As far as I can tell result is only defined in the return callback of the ajax function where it will contain the response of the call, it's not defined anywhere in the top part of the code.
– Darren Crabb
Nov 19 at 16:25
|
show 2 more comments
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53377729%2fjquery-how-to-add-list-of-objects-in-ajax%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
iTKG5 YaXjFelnXUeVy7U5MNNra26hE6j,rK a
What is wrong with the "Like this" approach shown? SHow us what
result['results2']
looks like– charlietfl
Nov 19 at 15:27
1
"The issue is actually with result['results2']"...and what exactly is the issue? What goes wrong?
– ADyson
Nov 19 at 15:27
I get an issue in my console :
result is not defined
. I don't know where I have to set this piece of code. Furthermore, I don't know if it's correct to make things like this because it's my first JQuery/Ajax code. In my python file,results2 = [{'id': 449, 'text': '50% cell culture infective dose (CCID50)'}, {'id': 176, 'text': 'Acetic acid in synthetic peptides'}, ...
– Ducky
Nov 19 at 15:30
Might be worth checking the network activity in your console, especially on the file being accessed by the ajax call. This should show you the headers, response etc. for each file listed (if you click on them) where you can check to make sure the correct results are being passed back to your client-side code. This happens in Firefox console at least - not sure about other browsers. That often helps a lot when working with ajax.
– Darren Crabb
Nov 19 at 16:13
1
It looks like you're trying to access 'result' out of scope. As far as I can tell result is only defined in the return callback of the ajax function where it will contain the response of the call, it's not defined anywhere in the top part of the code.
– Darren Crabb
Nov 19 at 16:25