DJANGO MULTIPLE IMAGES UPLOAD FOR A POST
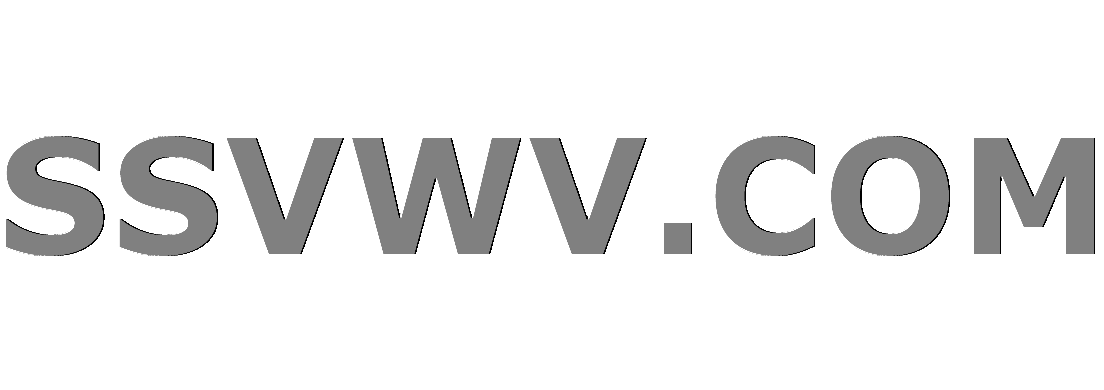
Multi tool use
Please help me. I am new to Django, cannot undertsand the following. I have subclass of CreateView for creating a post. I'm creating a rental site project where people can post their apart and attach files (images) to it. One should have possibility to attach as many images as he wants to ONE form. I have found in Internet a decision that I need to use 2 models - 1 model for post + 1 separate model for images. Is it so?
Post form is created and handled in my views.py by sublass of CreateView. Now how to connect new separate model for images with my CreateView ? And save all images to the current Post Form?
Thanks in advance
models.py
class Post(models.Model):
post_author = models.ForeignKey(settings.AUTH_USER_MODEL, on_delete=models.CASCADE)
post_title = models.CharField(_('Title'),max_length=200, null=True, blank=False)
post_type = models.CharField(_('Type'),max_length=18, choices=post_type_list, default='appart')
post_content = models.TextField(_('Your post description'),blank=True)
post_created_date = models.DateTimeField(default=timezone.now, help_text=_('Post creation date and time.'),)
post_published_date = models.DateTimeField(null=True, blank=True, help_text=_('Post publication date and time.'),)
def get_absolute_url(self):
return reverse("posts:post_details", kwargs={'pk':self.pk,'post_type':self.post_type})
class Post_Gallery(models.Model):
post = models.ForeignKey(Post, on_delete=models.CASCADE)
current_hour = datetime.datetime.now()
images = models.ImageField(_('Upload some pictures'),upload_to='uploads/%Y/%m/%d/{}'.format(current_hour.hour), max_length=100, null=True, blank=True)
def __unicode__(self):
return self.images.url
forms.py
class NewPostForm(forms.ModelForm):
class Meta:
model = Post
fields = [
'post_title','post_type','post_content',
]
class GalleryForm(forms.ModelForm):
class Meta:
model = Post_Gallery
fields = ['images',]
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.fields['images'].widget.attrs.update({'multiple': True, 'accept': 'image/jpg,image/jpeg,image/png,image/gif',} )
actual views.py
class NewPostView(CreateView):
form_class = NewPostForm
template_name = 'posts/submit-post.html'
model = Post
@method_decorator(login_required)
def dispatch(self, *args, **kwargs):
return super().dispatch(*args, **kwargs)
def form_valid(self, form):
form.instance.post_author = self.request.user
return super().form_valid(form)
def get(self, request):
form_class = self.get_form_class()
form = self.get_form(form_class)
return render(self.request, 'posts/submit-post.html', {'form': form})
python django upload image-upload multiple-file-upload
add a comment |
Please help me. I am new to Django, cannot undertsand the following. I have subclass of CreateView for creating a post. I'm creating a rental site project where people can post their apart and attach files (images) to it. One should have possibility to attach as many images as he wants to ONE form. I have found in Internet a decision that I need to use 2 models - 1 model for post + 1 separate model for images. Is it so?
Post form is created and handled in my views.py by sublass of CreateView. Now how to connect new separate model for images with my CreateView ? And save all images to the current Post Form?
Thanks in advance
models.py
class Post(models.Model):
post_author = models.ForeignKey(settings.AUTH_USER_MODEL, on_delete=models.CASCADE)
post_title = models.CharField(_('Title'),max_length=200, null=True, blank=False)
post_type = models.CharField(_('Type'),max_length=18, choices=post_type_list, default='appart')
post_content = models.TextField(_('Your post description'),blank=True)
post_created_date = models.DateTimeField(default=timezone.now, help_text=_('Post creation date and time.'),)
post_published_date = models.DateTimeField(null=True, blank=True, help_text=_('Post publication date and time.'),)
def get_absolute_url(self):
return reverse("posts:post_details", kwargs={'pk':self.pk,'post_type':self.post_type})
class Post_Gallery(models.Model):
post = models.ForeignKey(Post, on_delete=models.CASCADE)
current_hour = datetime.datetime.now()
images = models.ImageField(_('Upload some pictures'),upload_to='uploads/%Y/%m/%d/{}'.format(current_hour.hour), max_length=100, null=True, blank=True)
def __unicode__(self):
return self.images.url
forms.py
class NewPostForm(forms.ModelForm):
class Meta:
model = Post
fields = [
'post_title','post_type','post_content',
]
class GalleryForm(forms.ModelForm):
class Meta:
model = Post_Gallery
fields = ['images',]
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.fields['images'].widget.attrs.update({'multiple': True, 'accept': 'image/jpg,image/jpeg,image/png,image/gif',} )
actual views.py
class NewPostView(CreateView):
form_class = NewPostForm
template_name = 'posts/submit-post.html'
model = Post
@method_decorator(login_required)
def dispatch(self, *args, **kwargs):
return super().dispatch(*args, **kwargs)
def form_valid(self, form):
form.instance.post_author = self.request.user
return super().form_valid(form)
def get(self, request):
form_class = self.get_form_class()
form = self.get_form(form_class)
return render(self.request, 'posts/submit-post.html', {'form': form})
python django upload image-upload multiple-file-upload
add a comment |
Please help me. I am new to Django, cannot undertsand the following. I have subclass of CreateView for creating a post. I'm creating a rental site project where people can post their apart and attach files (images) to it. One should have possibility to attach as many images as he wants to ONE form. I have found in Internet a decision that I need to use 2 models - 1 model for post + 1 separate model for images. Is it so?
Post form is created and handled in my views.py by sublass of CreateView. Now how to connect new separate model for images with my CreateView ? And save all images to the current Post Form?
Thanks in advance
models.py
class Post(models.Model):
post_author = models.ForeignKey(settings.AUTH_USER_MODEL, on_delete=models.CASCADE)
post_title = models.CharField(_('Title'),max_length=200, null=True, blank=False)
post_type = models.CharField(_('Type'),max_length=18, choices=post_type_list, default='appart')
post_content = models.TextField(_('Your post description'),blank=True)
post_created_date = models.DateTimeField(default=timezone.now, help_text=_('Post creation date and time.'),)
post_published_date = models.DateTimeField(null=True, blank=True, help_text=_('Post publication date and time.'),)
def get_absolute_url(self):
return reverse("posts:post_details", kwargs={'pk':self.pk,'post_type':self.post_type})
class Post_Gallery(models.Model):
post = models.ForeignKey(Post, on_delete=models.CASCADE)
current_hour = datetime.datetime.now()
images = models.ImageField(_('Upload some pictures'),upload_to='uploads/%Y/%m/%d/{}'.format(current_hour.hour), max_length=100, null=True, blank=True)
def __unicode__(self):
return self.images.url
forms.py
class NewPostForm(forms.ModelForm):
class Meta:
model = Post
fields = [
'post_title','post_type','post_content',
]
class GalleryForm(forms.ModelForm):
class Meta:
model = Post_Gallery
fields = ['images',]
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.fields['images'].widget.attrs.update({'multiple': True, 'accept': 'image/jpg,image/jpeg,image/png,image/gif',} )
actual views.py
class NewPostView(CreateView):
form_class = NewPostForm
template_name = 'posts/submit-post.html'
model = Post
@method_decorator(login_required)
def dispatch(self, *args, **kwargs):
return super().dispatch(*args, **kwargs)
def form_valid(self, form):
form.instance.post_author = self.request.user
return super().form_valid(form)
def get(self, request):
form_class = self.get_form_class()
form = self.get_form(form_class)
return render(self.request, 'posts/submit-post.html', {'form': form})
python django upload image-upload multiple-file-upload
Please help me. I am new to Django, cannot undertsand the following. I have subclass of CreateView for creating a post. I'm creating a rental site project where people can post their apart and attach files (images) to it. One should have possibility to attach as many images as he wants to ONE form. I have found in Internet a decision that I need to use 2 models - 1 model for post + 1 separate model for images. Is it so?
Post form is created and handled in my views.py by sublass of CreateView. Now how to connect new separate model for images with my CreateView ? And save all images to the current Post Form?
Thanks in advance
models.py
class Post(models.Model):
post_author = models.ForeignKey(settings.AUTH_USER_MODEL, on_delete=models.CASCADE)
post_title = models.CharField(_('Title'),max_length=200, null=True, blank=False)
post_type = models.CharField(_('Type'),max_length=18, choices=post_type_list, default='appart')
post_content = models.TextField(_('Your post description'),blank=True)
post_created_date = models.DateTimeField(default=timezone.now, help_text=_('Post creation date and time.'),)
post_published_date = models.DateTimeField(null=True, blank=True, help_text=_('Post publication date and time.'),)
def get_absolute_url(self):
return reverse("posts:post_details", kwargs={'pk':self.pk,'post_type':self.post_type})
class Post_Gallery(models.Model):
post = models.ForeignKey(Post, on_delete=models.CASCADE)
current_hour = datetime.datetime.now()
images = models.ImageField(_('Upload some pictures'),upload_to='uploads/%Y/%m/%d/{}'.format(current_hour.hour), max_length=100, null=True, blank=True)
def __unicode__(self):
return self.images.url
forms.py
class NewPostForm(forms.ModelForm):
class Meta:
model = Post
fields = [
'post_title','post_type','post_content',
]
class GalleryForm(forms.ModelForm):
class Meta:
model = Post_Gallery
fields = ['images',]
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.fields['images'].widget.attrs.update({'multiple': True, 'accept': 'image/jpg,image/jpeg,image/png,image/gif',} )
actual views.py
class NewPostView(CreateView):
form_class = NewPostForm
template_name = 'posts/submit-post.html'
model = Post
@method_decorator(login_required)
def dispatch(self, *args, **kwargs):
return super().dispatch(*args, **kwargs)
def form_valid(self, form):
form.instance.post_author = self.request.user
return super().form_valid(form)
def get(self, request):
form_class = self.get_form_class()
form = self.get_form(form_class)
return render(self.request, 'posts/submit-post.html', {'form': form})
python django upload image-upload multiple-file-upload
python django upload image-upload multiple-file-upload
edited Nov 22 '18 at 8:38


MD. Khairul Basar
2,797102138
2,797102138
asked Nov 22 '18 at 8:09
atanasatanas
12
12
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53426402%2fdjango-multiple-images-upload-for-a-post%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53426402%2fdjango-multiple-images-upload-for-a-post%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
aZJeOPzBKYzaT1kcPf2kqtHy4eEmzeOAd WMQe7kIJKfaN