No database provider has been configured for this DbContext - ASP.Net Core 2.1
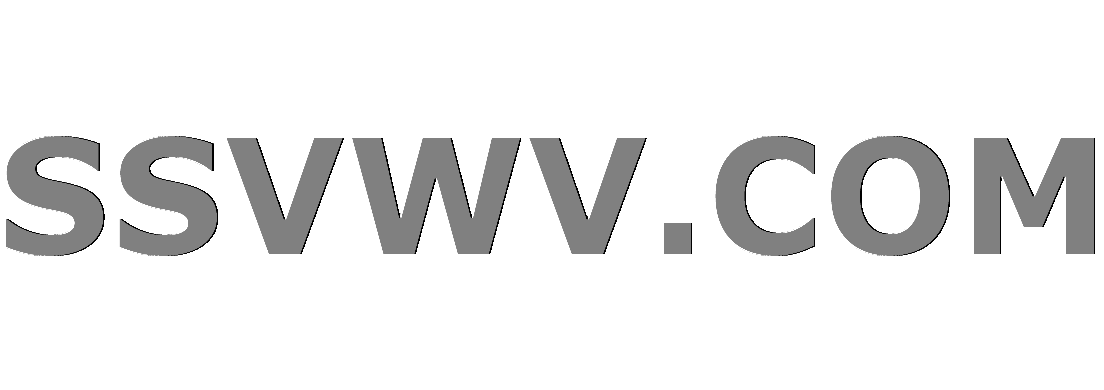
Multi tool use
I am getting the below error :
InvalidOperationException: No database provider has been configured for this DbContext. A provider can be configured by overriding the DbContext.OnConfiguring method or by using AddDbContext on the application service provider. If AddDbContext is used, then also ensure that your DbContext type accepts a DbContextOptions object in its constructor and passes it to the base constructor for DbContext.
Startup.cs :
public void ConfigureServices(IServiceCollection services)
{
services.AddSingleton<IHttpContextAccessor, HttpContextAccessor>();
services.AddDistributedMemoryCache();
services.AddSession(options=>{options.IdleTimeout = TimeSpan.FromMinutes(1);});services.AddAuthentication(CookieAuthenticationDefaults.AuthenticationScheme).AddCookie(options =>
{
options.Cookie.HttpOnly = true;
options.ExpireTimeSpan = TimeSpan.FromMinutes(20);
options.Cookie.Name = "SampleCore";
options.LoginPath = "/log-in";
//options.AccessDeniedPath = "/access-denied";
options.LogoutPath = "/log-off";
options.ReturnUrlParameter = "postBack";
});
services.AddMvc(options =>
{
options.Filters.Add(new AutoValidateAntiforgeryTokenAttribute());
}).SetCompatibilityVersion(CompatibilityVersion.Version_2_1);
string connection = Configuration.GetConnectionString("MyConnection");
services.AddDbContext<EmployeeContext>(options => options.UseSqlServer(connection), ServiceLifetime.Scoped);
}
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
app.UseStaticFiles();
app.UseSession();
app.UseCookiePolicy();
app.UseAuthentication();
app.UseCustomErrorPages();
app.UseMvc();
}
UseCustomErrorPages Extension :
public async Task Invoke(HttpContext context)
{
await _next(context); // Getting error at this line
if (context.Response.StatusCode == 404 && !context.Response.HasStarted)
{
string originalPath = context.Request.Path.Value;
context.Items["originalPath"] = originalPath;
context.Request.Path = "/page-not-found";
await _next(context);
}
else if (context.Response.StatusCode == 500)
{
string originalPath = context.Request.Path.Value;
context.Items["originalPath"] = originalPath;
context.Request.Path = "/error";
await _next(context);
}
else if(context.Response.StatusCode == 401)
{
string originalPath = context.Request.Path.Value;
context.Items["originalPath"] = originalPath;
context.Request.Path = "/access-denied";
await _next(context);
}
}
DbContext :
public partial class EmployeeContext : DbContext
{
public EmployeeContext(DbContextOptions<EmployeeContext> options)
: base(options)
{
}
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder)
{
if (!optionsBuilder.IsConfigured)
{
var connectionString = "connectionString";
optionsBuilder.UseSqlServer(connectionString);
base.OnConfiguring(optionsBuilder);
}
}
public virtual DbSet<TblEmployee> TblEmployee { get; set; }
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
//Code
}
}
csproj file :
<ItemGroup>
<PackageReference Include="Microsoft.AspNetCore.App" />
<PackageReference Include="Microsoft.AspNetCore.Razor.Design" Version="2.1.2" PrivateAssets="All" />
<PackageReference Include="Microsoft.EntityFrameworkCore" Version="2.1.4" />
<PackageReference Include="Microsoft.EntityFrameworkCore.SqlServer" Version="2.1.4" />
<PackageReference Include="Microsoft.EntityFrameworkCore.SqlServer.Design" Version="1.1.6" />
<PackageReference Include="Microsoft.EntityFrameworkCore.Tools" Version="2.1.4">
<PrivateAssets>all</PrivateAssets>
<IncludeAssets>runtime; build; native; contentfiles; analyzers</IncludeAssets>
</PackageReference>
<PackageReference Include="Microsoft.VisualStudio.Web.CodeGeneration.Design" Version="2.1.6" />
Controller :
private EmployeeContext _context;
public HomeController(EmployeeContext context)
{
_context = context;
}
public List<TblEmployee> GetEmployees
{
List<TblEmployee> getEmployees = _context.TblEmployee.ToList();
return getEmployees ;
}
appsettings.json :
{
"Logging": {
"LogLevel": {
"Default": "Warning"
}
},
"ConnectionStrings": {
"MyConnection": "connection string"
},
"AllowedHosts": "*"
}
I don't understand where I did wrong. Kindly help
c# entity-framework asp.net-core dependency-injection
|
show 2 more comments
I am getting the below error :
InvalidOperationException: No database provider has been configured for this DbContext. A provider can be configured by overriding the DbContext.OnConfiguring method or by using AddDbContext on the application service provider. If AddDbContext is used, then also ensure that your DbContext type accepts a DbContextOptions object in its constructor and passes it to the base constructor for DbContext.
Startup.cs :
public void ConfigureServices(IServiceCollection services)
{
services.AddSingleton<IHttpContextAccessor, HttpContextAccessor>();
services.AddDistributedMemoryCache();
services.AddSession(options=>{options.IdleTimeout = TimeSpan.FromMinutes(1);});services.AddAuthentication(CookieAuthenticationDefaults.AuthenticationScheme).AddCookie(options =>
{
options.Cookie.HttpOnly = true;
options.ExpireTimeSpan = TimeSpan.FromMinutes(20);
options.Cookie.Name = "SampleCore";
options.LoginPath = "/log-in";
//options.AccessDeniedPath = "/access-denied";
options.LogoutPath = "/log-off";
options.ReturnUrlParameter = "postBack";
});
services.AddMvc(options =>
{
options.Filters.Add(new AutoValidateAntiforgeryTokenAttribute());
}).SetCompatibilityVersion(CompatibilityVersion.Version_2_1);
string connection = Configuration.GetConnectionString("MyConnection");
services.AddDbContext<EmployeeContext>(options => options.UseSqlServer(connection), ServiceLifetime.Scoped);
}
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
app.UseStaticFiles();
app.UseSession();
app.UseCookiePolicy();
app.UseAuthentication();
app.UseCustomErrorPages();
app.UseMvc();
}
UseCustomErrorPages Extension :
public async Task Invoke(HttpContext context)
{
await _next(context); // Getting error at this line
if (context.Response.StatusCode == 404 && !context.Response.HasStarted)
{
string originalPath = context.Request.Path.Value;
context.Items["originalPath"] = originalPath;
context.Request.Path = "/page-not-found";
await _next(context);
}
else if (context.Response.StatusCode == 500)
{
string originalPath = context.Request.Path.Value;
context.Items["originalPath"] = originalPath;
context.Request.Path = "/error";
await _next(context);
}
else if(context.Response.StatusCode == 401)
{
string originalPath = context.Request.Path.Value;
context.Items["originalPath"] = originalPath;
context.Request.Path = "/access-denied";
await _next(context);
}
}
DbContext :
public partial class EmployeeContext : DbContext
{
public EmployeeContext(DbContextOptions<EmployeeContext> options)
: base(options)
{
}
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder)
{
if (!optionsBuilder.IsConfigured)
{
var connectionString = "connectionString";
optionsBuilder.UseSqlServer(connectionString);
base.OnConfiguring(optionsBuilder);
}
}
public virtual DbSet<TblEmployee> TblEmployee { get; set; }
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
//Code
}
}
csproj file :
<ItemGroup>
<PackageReference Include="Microsoft.AspNetCore.App" />
<PackageReference Include="Microsoft.AspNetCore.Razor.Design" Version="2.1.2" PrivateAssets="All" />
<PackageReference Include="Microsoft.EntityFrameworkCore" Version="2.1.4" />
<PackageReference Include="Microsoft.EntityFrameworkCore.SqlServer" Version="2.1.4" />
<PackageReference Include="Microsoft.EntityFrameworkCore.SqlServer.Design" Version="1.1.6" />
<PackageReference Include="Microsoft.EntityFrameworkCore.Tools" Version="2.1.4">
<PrivateAssets>all</PrivateAssets>
<IncludeAssets>runtime; build; native; contentfiles; analyzers</IncludeAssets>
</PackageReference>
<PackageReference Include="Microsoft.VisualStudio.Web.CodeGeneration.Design" Version="2.1.6" />
Controller :
private EmployeeContext _context;
public HomeController(EmployeeContext context)
{
_context = context;
}
public List<TblEmployee> GetEmployees
{
List<TblEmployee> getEmployees = _context.TblEmployee.ToList();
return getEmployees ;
}
appsettings.json :
{
"Logging": {
"LogLevel": {
"Default": "Warning"
}
},
"ConnectionStrings": {
"MyConnection": "connection string"
},
"AllowedHosts": "*"
}
I don't understand where I did wrong. Kindly help
c# entity-framework asp.net-core dependency-injection
SUGGESTION: Make sure you have a valid connection string in yourappsettings.json
. The usual name is "DefaultConnection"; your .json needs a connection string with the name ""MyConnection".
– paulsm4
Nov 22 '18 at 8:11
Thanks for your reply @paulsm4. I am able to read the connection string from appsettings.json file.
– Portgas Dragon
Nov 22 '18 at 8:17
TWO SUGGESTIONS: 1) Change your debug level to "Trace", 2) Try to recreate the problem in a small, self contained example
– paulsm4
Nov 22 '18 at 18:49
Fail to reproduce your issue with your code, is there any demo project to reproduce your issue? Have you run command to create and update the database?
– Tao Zhou
Nov 23 '18 at 8:17
Hi @TaoZhou, Thanks for your reply. I have an already existing db. So I ran a scaffold command to get the entities in my project.
– Portgas Dragon
Nov 26 '18 at 6:11
|
show 2 more comments
I am getting the below error :
InvalidOperationException: No database provider has been configured for this DbContext. A provider can be configured by overriding the DbContext.OnConfiguring method or by using AddDbContext on the application service provider. If AddDbContext is used, then also ensure that your DbContext type accepts a DbContextOptions object in its constructor and passes it to the base constructor for DbContext.
Startup.cs :
public void ConfigureServices(IServiceCollection services)
{
services.AddSingleton<IHttpContextAccessor, HttpContextAccessor>();
services.AddDistributedMemoryCache();
services.AddSession(options=>{options.IdleTimeout = TimeSpan.FromMinutes(1);});services.AddAuthentication(CookieAuthenticationDefaults.AuthenticationScheme).AddCookie(options =>
{
options.Cookie.HttpOnly = true;
options.ExpireTimeSpan = TimeSpan.FromMinutes(20);
options.Cookie.Name = "SampleCore";
options.LoginPath = "/log-in";
//options.AccessDeniedPath = "/access-denied";
options.LogoutPath = "/log-off";
options.ReturnUrlParameter = "postBack";
});
services.AddMvc(options =>
{
options.Filters.Add(new AutoValidateAntiforgeryTokenAttribute());
}).SetCompatibilityVersion(CompatibilityVersion.Version_2_1);
string connection = Configuration.GetConnectionString("MyConnection");
services.AddDbContext<EmployeeContext>(options => options.UseSqlServer(connection), ServiceLifetime.Scoped);
}
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
app.UseStaticFiles();
app.UseSession();
app.UseCookiePolicy();
app.UseAuthentication();
app.UseCustomErrorPages();
app.UseMvc();
}
UseCustomErrorPages Extension :
public async Task Invoke(HttpContext context)
{
await _next(context); // Getting error at this line
if (context.Response.StatusCode == 404 && !context.Response.HasStarted)
{
string originalPath = context.Request.Path.Value;
context.Items["originalPath"] = originalPath;
context.Request.Path = "/page-not-found";
await _next(context);
}
else if (context.Response.StatusCode == 500)
{
string originalPath = context.Request.Path.Value;
context.Items["originalPath"] = originalPath;
context.Request.Path = "/error";
await _next(context);
}
else if(context.Response.StatusCode == 401)
{
string originalPath = context.Request.Path.Value;
context.Items["originalPath"] = originalPath;
context.Request.Path = "/access-denied";
await _next(context);
}
}
DbContext :
public partial class EmployeeContext : DbContext
{
public EmployeeContext(DbContextOptions<EmployeeContext> options)
: base(options)
{
}
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder)
{
if (!optionsBuilder.IsConfigured)
{
var connectionString = "connectionString";
optionsBuilder.UseSqlServer(connectionString);
base.OnConfiguring(optionsBuilder);
}
}
public virtual DbSet<TblEmployee> TblEmployee { get; set; }
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
//Code
}
}
csproj file :
<ItemGroup>
<PackageReference Include="Microsoft.AspNetCore.App" />
<PackageReference Include="Microsoft.AspNetCore.Razor.Design" Version="2.1.2" PrivateAssets="All" />
<PackageReference Include="Microsoft.EntityFrameworkCore" Version="2.1.4" />
<PackageReference Include="Microsoft.EntityFrameworkCore.SqlServer" Version="2.1.4" />
<PackageReference Include="Microsoft.EntityFrameworkCore.SqlServer.Design" Version="1.1.6" />
<PackageReference Include="Microsoft.EntityFrameworkCore.Tools" Version="2.1.4">
<PrivateAssets>all</PrivateAssets>
<IncludeAssets>runtime; build; native; contentfiles; analyzers</IncludeAssets>
</PackageReference>
<PackageReference Include="Microsoft.VisualStudio.Web.CodeGeneration.Design" Version="2.1.6" />
Controller :
private EmployeeContext _context;
public HomeController(EmployeeContext context)
{
_context = context;
}
public List<TblEmployee> GetEmployees
{
List<TblEmployee> getEmployees = _context.TblEmployee.ToList();
return getEmployees ;
}
appsettings.json :
{
"Logging": {
"LogLevel": {
"Default": "Warning"
}
},
"ConnectionStrings": {
"MyConnection": "connection string"
},
"AllowedHosts": "*"
}
I don't understand where I did wrong. Kindly help
c# entity-framework asp.net-core dependency-injection
I am getting the below error :
InvalidOperationException: No database provider has been configured for this DbContext. A provider can be configured by overriding the DbContext.OnConfiguring method or by using AddDbContext on the application service provider. If AddDbContext is used, then also ensure that your DbContext type accepts a DbContextOptions object in its constructor and passes it to the base constructor for DbContext.
Startup.cs :
public void ConfigureServices(IServiceCollection services)
{
services.AddSingleton<IHttpContextAccessor, HttpContextAccessor>();
services.AddDistributedMemoryCache();
services.AddSession(options=>{options.IdleTimeout = TimeSpan.FromMinutes(1);});services.AddAuthentication(CookieAuthenticationDefaults.AuthenticationScheme).AddCookie(options =>
{
options.Cookie.HttpOnly = true;
options.ExpireTimeSpan = TimeSpan.FromMinutes(20);
options.Cookie.Name = "SampleCore";
options.LoginPath = "/log-in";
//options.AccessDeniedPath = "/access-denied";
options.LogoutPath = "/log-off";
options.ReturnUrlParameter = "postBack";
});
services.AddMvc(options =>
{
options.Filters.Add(new AutoValidateAntiforgeryTokenAttribute());
}).SetCompatibilityVersion(CompatibilityVersion.Version_2_1);
string connection = Configuration.GetConnectionString("MyConnection");
services.AddDbContext<EmployeeContext>(options => options.UseSqlServer(connection), ServiceLifetime.Scoped);
}
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
app.UseStaticFiles();
app.UseSession();
app.UseCookiePolicy();
app.UseAuthentication();
app.UseCustomErrorPages();
app.UseMvc();
}
UseCustomErrorPages Extension :
public async Task Invoke(HttpContext context)
{
await _next(context); // Getting error at this line
if (context.Response.StatusCode == 404 && !context.Response.HasStarted)
{
string originalPath = context.Request.Path.Value;
context.Items["originalPath"] = originalPath;
context.Request.Path = "/page-not-found";
await _next(context);
}
else if (context.Response.StatusCode == 500)
{
string originalPath = context.Request.Path.Value;
context.Items["originalPath"] = originalPath;
context.Request.Path = "/error";
await _next(context);
}
else if(context.Response.StatusCode == 401)
{
string originalPath = context.Request.Path.Value;
context.Items["originalPath"] = originalPath;
context.Request.Path = "/access-denied";
await _next(context);
}
}
DbContext :
public partial class EmployeeContext : DbContext
{
public EmployeeContext(DbContextOptions<EmployeeContext> options)
: base(options)
{
}
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder)
{
if (!optionsBuilder.IsConfigured)
{
var connectionString = "connectionString";
optionsBuilder.UseSqlServer(connectionString);
base.OnConfiguring(optionsBuilder);
}
}
public virtual DbSet<TblEmployee> TblEmployee { get; set; }
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
//Code
}
}
csproj file :
<ItemGroup>
<PackageReference Include="Microsoft.AspNetCore.App" />
<PackageReference Include="Microsoft.AspNetCore.Razor.Design" Version="2.1.2" PrivateAssets="All" />
<PackageReference Include="Microsoft.EntityFrameworkCore" Version="2.1.4" />
<PackageReference Include="Microsoft.EntityFrameworkCore.SqlServer" Version="2.1.4" />
<PackageReference Include="Microsoft.EntityFrameworkCore.SqlServer.Design" Version="1.1.6" />
<PackageReference Include="Microsoft.EntityFrameworkCore.Tools" Version="2.1.4">
<PrivateAssets>all</PrivateAssets>
<IncludeAssets>runtime; build; native; contentfiles; analyzers</IncludeAssets>
</PackageReference>
<PackageReference Include="Microsoft.VisualStudio.Web.CodeGeneration.Design" Version="2.1.6" />
Controller :
private EmployeeContext _context;
public HomeController(EmployeeContext context)
{
_context = context;
}
public List<TblEmployee> GetEmployees
{
List<TblEmployee> getEmployees = _context.TblEmployee.ToList();
return getEmployees ;
}
appsettings.json :
{
"Logging": {
"LogLevel": {
"Default": "Warning"
}
},
"ConnectionStrings": {
"MyConnection": "connection string"
},
"AllowedHosts": "*"
}
I don't understand where I did wrong. Kindly help
c# entity-framework asp.net-core dependency-injection
c# entity-framework asp.net-core dependency-injection
asked Nov 22 '18 at 8:06
Portgas DragonPortgas Dragon
62
62
SUGGESTION: Make sure you have a valid connection string in yourappsettings.json
. The usual name is "DefaultConnection"; your .json needs a connection string with the name ""MyConnection".
– paulsm4
Nov 22 '18 at 8:11
Thanks for your reply @paulsm4. I am able to read the connection string from appsettings.json file.
– Portgas Dragon
Nov 22 '18 at 8:17
TWO SUGGESTIONS: 1) Change your debug level to "Trace", 2) Try to recreate the problem in a small, self contained example
– paulsm4
Nov 22 '18 at 18:49
Fail to reproduce your issue with your code, is there any demo project to reproduce your issue? Have you run command to create and update the database?
– Tao Zhou
Nov 23 '18 at 8:17
Hi @TaoZhou, Thanks for your reply. I have an already existing db. So I ran a scaffold command to get the entities in my project.
– Portgas Dragon
Nov 26 '18 at 6:11
|
show 2 more comments
SUGGESTION: Make sure you have a valid connection string in yourappsettings.json
. The usual name is "DefaultConnection"; your .json needs a connection string with the name ""MyConnection".
– paulsm4
Nov 22 '18 at 8:11
Thanks for your reply @paulsm4. I am able to read the connection string from appsettings.json file.
– Portgas Dragon
Nov 22 '18 at 8:17
TWO SUGGESTIONS: 1) Change your debug level to "Trace", 2) Try to recreate the problem in a small, self contained example
– paulsm4
Nov 22 '18 at 18:49
Fail to reproduce your issue with your code, is there any demo project to reproduce your issue? Have you run command to create and update the database?
– Tao Zhou
Nov 23 '18 at 8:17
Hi @TaoZhou, Thanks for your reply. I have an already existing db. So I ran a scaffold command to get the entities in my project.
– Portgas Dragon
Nov 26 '18 at 6:11
SUGGESTION: Make sure you have a valid connection string in your
appsettings.json
. The usual name is "DefaultConnection"; your .json needs a connection string with the name ""MyConnection".– paulsm4
Nov 22 '18 at 8:11
SUGGESTION: Make sure you have a valid connection string in your
appsettings.json
. The usual name is "DefaultConnection"; your .json needs a connection string with the name ""MyConnection".– paulsm4
Nov 22 '18 at 8:11
Thanks for your reply @paulsm4. I am able to read the connection string from appsettings.json file.
– Portgas Dragon
Nov 22 '18 at 8:17
Thanks for your reply @paulsm4. I am able to read the connection string from appsettings.json file.
– Portgas Dragon
Nov 22 '18 at 8:17
TWO SUGGESTIONS: 1) Change your debug level to "Trace", 2) Try to recreate the problem in a small, self contained example
– paulsm4
Nov 22 '18 at 18:49
TWO SUGGESTIONS: 1) Change your debug level to "Trace", 2) Try to recreate the problem in a small, self contained example
– paulsm4
Nov 22 '18 at 18:49
Fail to reproduce your issue with your code, is there any demo project to reproduce your issue? Have you run command to create and update the database?
– Tao Zhou
Nov 23 '18 at 8:17
Fail to reproduce your issue with your code, is there any demo project to reproduce your issue? Have you run command to create and update the database?
– Tao Zhou
Nov 23 '18 at 8:17
Hi @TaoZhou, Thanks for your reply. I have an already existing db. So I ran a scaffold command to get the entities in my project.
– Portgas Dragon
Nov 26 '18 at 6:11
Hi @TaoZhou, Thanks for your reply. I have an already existing db. So I ran a scaffold command to get the entities in my project.
– Portgas Dragon
Nov 26 '18 at 6:11
|
show 2 more comments
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53426357%2fno-database-provider-has-been-configured-for-this-dbcontext-asp-net-core-2-1%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53426357%2fno-database-provider-has-been-configured-for-this-dbcontext-asp-net-core-2-1%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
H KGfeeVAH56g2tRo5LfyzyxagYRKFH9v4,j46i,izO,PZNtb25I3 wFfZb 5j8o,WOAHNekXN 8u2zyGJJlsSMbm5WhzAc Jx Bbp
SUGGESTION: Make sure you have a valid connection string in your
appsettings.json
. The usual name is "DefaultConnection"; your .json needs a connection string with the name ""MyConnection".– paulsm4
Nov 22 '18 at 8:11
Thanks for your reply @paulsm4. I am able to read the connection string from appsettings.json file.
– Portgas Dragon
Nov 22 '18 at 8:17
TWO SUGGESTIONS: 1) Change your debug level to "Trace", 2) Try to recreate the problem in a small, self contained example
– paulsm4
Nov 22 '18 at 18:49
Fail to reproduce your issue with your code, is there any demo project to reproduce your issue? Have you run command to create and update the database?
– Tao Zhou
Nov 23 '18 at 8:17
Hi @TaoZhou, Thanks for your reply. I have an already existing db. So I ran a scaffold command to get the entities in my project.
– Portgas Dragon
Nov 26 '18 at 6:11