Azure WebJob to: create CSV file from SQL and send as attachment via email
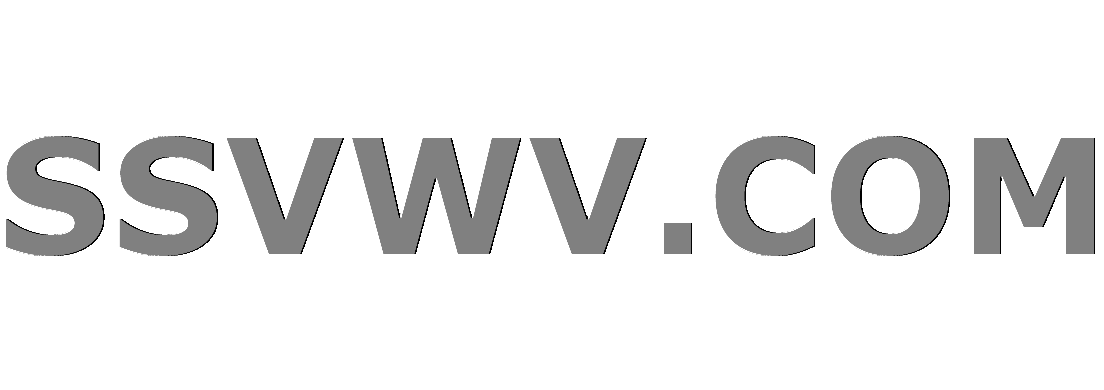
Multi tool use
So far, managed to:
- Create a C# Azure WebJob project via Visual Studio and publish it to a web app, that:
- Connects to an Azure SQL Database and executes SQL query (via SqlDataReader)
- Adds SqlDataReader results into the email body
- Sends email
In addition to the above, somewhere between above points 3 and 4, I need to:
- Create a .CSV file
- Populate the .CSV file from the SqlDataReader
- Send the .CSV file via email as attachment
Result set from SqlDataReader to populate CSV looks like:
asdasd@gmail.com ,11/19/2018
asdasdasd@gmail.com ,11/19/2018
asdasdasasdas@live.co.uk ,11/19/2018
asdasddsa@hotmail.com ,11/19/2018
asdasd@hotmail.com ,11/19/2018
asdasddsa@hotmail.com ,11/19/2018
asdasasd@gmail.com ,11/18/2018
Below is what I have so far:
public static void Main(string args)
{
SmtpClient smtp = new SmtpClient();
int SMTP_PORT = 587;
Int32.TryParse(ConfigurationManager.AppSettings["SMTP_PORT"], out SMTP_PORT);
smtp.Port = SMTP_PORT;
smtp.Credentials = new NetworkCredential(ConfigurationManager.AppSettings["SMTP_USERNAME"], ConfigurationManager.AppSettings["SMTP_PASSWORD"]);
smtp.Host = ConfigurationManager.AppSettings["SMTP_HOST"];
string mailFrom = ConfigurationManager.AppSettings["SMTP_MAIL_FROM"];
string mailSubject = ConfigurationManager.AppSettings["SMTP_MAIL_SUBJECT"];
using (SqlConnection connection = new SqlConnection(ConfigurationManager.AppSettings["AzureDBConnString"]))
{
connection.Open();
var queryString = @"SELECT * FROM MyTable WHERE Status = 1";
using (SqlCommand command = new SqlCommand(queryString, connection))
{
command.CommandTimeout = 120;
SqlDataReader reader = command.ExecuteReader();
if (reader.HasRows)
{
while (reader.Read()) // loop each user and send email
{
bool emailSentSuccess = true;
using (MailMessage mail = new MailMessage())
{
try
{
mail.From = new MailAddress(mailFrom);
mail.To.Add(new MailAddress(reader["EmailAddress"].ToString()));
mail.IsBodyHtml = true;
mail.Subject = mailSubject;
mail.Body = reader["EmailBody"].ToString();
smtp.Send(mail);
}
catch (Exception ex)
{
emailSentSuccess = false;
}
}
}
}
}
}
}
Question: How can I achieve points 5, 6, 7?
sql




add a comment |
So far, managed to:
- Create a C# Azure WebJob project via Visual Studio and publish it to a web app, that:
- Connects to an Azure SQL Database and executes SQL query (via SqlDataReader)
- Adds SqlDataReader results into the email body
- Sends email
In addition to the above, somewhere between above points 3 and 4, I need to:
- Create a .CSV file
- Populate the .CSV file from the SqlDataReader
- Send the .CSV file via email as attachment
Result set from SqlDataReader to populate CSV looks like:
asdasd@gmail.com ,11/19/2018
asdasdasd@gmail.com ,11/19/2018
asdasdasasdas@live.co.uk ,11/19/2018
asdasddsa@hotmail.com ,11/19/2018
asdasd@hotmail.com ,11/19/2018
asdasddsa@hotmail.com ,11/19/2018
asdasasd@gmail.com ,11/18/2018
Below is what I have so far:
public static void Main(string args)
{
SmtpClient smtp = new SmtpClient();
int SMTP_PORT = 587;
Int32.TryParse(ConfigurationManager.AppSettings["SMTP_PORT"], out SMTP_PORT);
smtp.Port = SMTP_PORT;
smtp.Credentials = new NetworkCredential(ConfigurationManager.AppSettings["SMTP_USERNAME"], ConfigurationManager.AppSettings["SMTP_PASSWORD"]);
smtp.Host = ConfigurationManager.AppSettings["SMTP_HOST"];
string mailFrom = ConfigurationManager.AppSettings["SMTP_MAIL_FROM"];
string mailSubject = ConfigurationManager.AppSettings["SMTP_MAIL_SUBJECT"];
using (SqlConnection connection = new SqlConnection(ConfigurationManager.AppSettings["AzureDBConnString"]))
{
connection.Open();
var queryString = @"SELECT * FROM MyTable WHERE Status = 1";
using (SqlCommand command = new SqlCommand(queryString, connection))
{
command.CommandTimeout = 120;
SqlDataReader reader = command.ExecuteReader();
if (reader.HasRows)
{
while (reader.Read()) // loop each user and send email
{
bool emailSentSuccess = true;
using (MailMessage mail = new MailMessage())
{
try
{
mail.From = new MailAddress(mailFrom);
mail.To.Add(new MailAddress(reader["EmailAddress"].ToString()));
mail.IsBodyHtml = true;
mail.Subject = mailSubject;
mail.Body = reader["EmailBody"].ToString();
smtp.Send(mail);
}
catch (Exception ex)
{
emailSentSuccess = false;
}
}
}
}
}
}
}
Question: How can I achieve points 5, 6, 7?
sql




1
Not sure about web jobs, But you can easily achieve this using logic app, without coding. Refer my article here
– Jayendran
Nov 26 '18 at 3:55
add a comment |
So far, managed to:
- Create a C# Azure WebJob project via Visual Studio and publish it to a web app, that:
- Connects to an Azure SQL Database and executes SQL query (via SqlDataReader)
- Adds SqlDataReader results into the email body
- Sends email
In addition to the above, somewhere between above points 3 and 4, I need to:
- Create a .CSV file
- Populate the .CSV file from the SqlDataReader
- Send the .CSV file via email as attachment
Result set from SqlDataReader to populate CSV looks like:
asdasd@gmail.com ,11/19/2018
asdasdasd@gmail.com ,11/19/2018
asdasdasasdas@live.co.uk ,11/19/2018
asdasddsa@hotmail.com ,11/19/2018
asdasd@hotmail.com ,11/19/2018
asdasddsa@hotmail.com ,11/19/2018
asdasasd@gmail.com ,11/18/2018
Below is what I have so far:
public static void Main(string args)
{
SmtpClient smtp = new SmtpClient();
int SMTP_PORT = 587;
Int32.TryParse(ConfigurationManager.AppSettings["SMTP_PORT"], out SMTP_PORT);
smtp.Port = SMTP_PORT;
smtp.Credentials = new NetworkCredential(ConfigurationManager.AppSettings["SMTP_USERNAME"], ConfigurationManager.AppSettings["SMTP_PASSWORD"]);
smtp.Host = ConfigurationManager.AppSettings["SMTP_HOST"];
string mailFrom = ConfigurationManager.AppSettings["SMTP_MAIL_FROM"];
string mailSubject = ConfigurationManager.AppSettings["SMTP_MAIL_SUBJECT"];
using (SqlConnection connection = new SqlConnection(ConfigurationManager.AppSettings["AzureDBConnString"]))
{
connection.Open();
var queryString = @"SELECT * FROM MyTable WHERE Status = 1";
using (SqlCommand command = new SqlCommand(queryString, connection))
{
command.CommandTimeout = 120;
SqlDataReader reader = command.ExecuteReader();
if (reader.HasRows)
{
while (reader.Read()) // loop each user and send email
{
bool emailSentSuccess = true;
using (MailMessage mail = new MailMessage())
{
try
{
mail.From = new MailAddress(mailFrom);
mail.To.Add(new MailAddress(reader["EmailAddress"].ToString()));
mail.IsBodyHtml = true;
mail.Subject = mailSubject;
mail.Body = reader["EmailBody"].ToString();
smtp.Send(mail);
}
catch (Exception ex)
{
emailSentSuccess = false;
}
}
}
}
}
}
}
Question: How can I achieve points 5, 6, 7?
sql




So far, managed to:
- Create a C# Azure WebJob project via Visual Studio and publish it to a web app, that:
- Connects to an Azure SQL Database and executes SQL query (via SqlDataReader)
- Adds SqlDataReader results into the email body
- Sends email
In addition to the above, somewhere between above points 3 and 4, I need to:
- Create a .CSV file
- Populate the .CSV file from the SqlDataReader
- Send the .CSV file via email as attachment
Result set from SqlDataReader to populate CSV looks like:
asdasd@gmail.com ,11/19/2018
asdasdasd@gmail.com ,11/19/2018
asdasdasasdas@live.co.uk ,11/19/2018
asdasddsa@hotmail.com ,11/19/2018
asdasd@hotmail.com ,11/19/2018
asdasddsa@hotmail.com ,11/19/2018
asdasasd@gmail.com ,11/18/2018
Below is what I have so far:
public static void Main(string args)
{
SmtpClient smtp = new SmtpClient();
int SMTP_PORT = 587;
Int32.TryParse(ConfigurationManager.AppSettings["SMTP_PORT"], out SMTP_PORT);
smtp.Port = SMTP_PORT;
smtp.Credentials = new NetworkCredential(ConfigurationManager.AppSettings["SMTP_USERNAME"], ConfigurationManager.AppSettings["SMTP_PASSWORD"]);
smtp.Host = ConfigurationManager.AppSettings["SMTP_HOST"];
string mailFrom = ConfigurationManager.AppSettings["SMTP_MAIL_FROM"];
string mailSubject = ConfigurationManager.AppSettings["SMTP_MAIL_SUBJECT"];
using (SqlConnection connection = new SqlConnection(ConfigurationManager.AppSettings["AzureDBConnString"]))
{
connection.Open();
var queryString = @"SELECT * FROM MyTable WHERE Status = 1";
using (SqlCommand command = new SqlCommand(queryString, connection))
{
command.CommandTimeout = 120;
SqlDataReader reader = command.ExecuteReader();
if (reader.HasRows)
{
while (reader.Read()) // loop each user and send email
{
bool emailSentSuccess = true;
using (MailMessage mail = new MailMessage())
{
try
{
mail.From = new MailAddress(mailFrom);
mail.To.Add(new MailAddress(reader["EmailAddress"].ToString()));
mail.IsBodyHtml = true;
mail.Subject = mailSubject;
mail.Body = reader["EmailBody"].ToString();
smtp.Send(mail);
}
catch (Exception ex)
{
emailSentSuccess = false;
}
}
}
}
}
}
}
Question: How can I achieve points 5, 6, 7?
sql




sql




asked Nov 23 '18 at 17:08
alexalex
49111748
49111748
1
Not sure about web jobs, But you can easily achieve this using logic app, without coding. Refer my article here
– Jayendran
Nov 26 '18 at 3:55
add a comment |
1
Not sure about web jobs, But you can easily achieve this using logic app, without coding. Refer my article here
– Jayendran
Nov 26 '18 at 3:55
1
1
Not sure about web jobs, But you can easily achieve this using logic app, without coding. Refer my article here
– Jayendran
Nov 26 '18 at 3:55
Not sure about web jobs, But you can easily achieve this using logic app, without coding. Refer my article here
– Jayendran
Nov 26 '18 at 3:55
add a comment |
1 Answer
1
active
oldest
votes
You could refer to the following code in your SqlCommand
.
using (SqlCommand command = new SqlCommand(queryString, connection))
{
command.CommandTimeout = 120;
SqlDataReader reader = command.ExecuteReader();
if (reader.HasRows)
{
StringBuilder sb = new StringBuilder();
List<string> columnNames = new List<string>();
List<string> rows = new List<string>();
for (int i = 0; i < reader.FieldCount; i++)
{
string tmpColumnName = reader.GetName(i);
columnNames.Add(tmpColumnName);
}
sb.Append(string.Join(",", columnNames.ToArray())).Append("rn");
List<string> currentRow = new List<string>();
while (reader.Read())
{
for (int i = 0; i < reader.FieldCount; i++)
{
object item = reader[i];
sb.Append(item.ToString().Replace(",", ";") + ',');
}
sb.Append("rn");
}
bool emailSentSuccess = true;
using (MailMessage mail = new MailMessage())
{
try
{
using (MemoryStream stream = new MemoryStream(Encoding.ASCII.GetBytes(sb.ToString())))
{
Attachment attachment = new Attachment(stream, new ContentType("text/csv"));
attachment.Name = "hello.csv";
mail.Attachments.Add(attachment);
mail.From = new MailAddress(mailFrom);
mail.To.Add(new MailAddress(reader["EmailAddress"].ToString()));
mail.IsBodyHtml = true;
mail.Subject = mailSubject;
mail.Body = reader["EmailBody"].ToString();
smtp.Send(mail);
}
}
catch (Exception ex)
{
emailSentSuccess = false;
}
}
}
}
The output I test is as below:
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53450626%2fazure-webjob-to-create-csv-file-from-sql-and-send-as-attachment-via-email%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You could refer to the following code in your SqlCommand
.
using (SqlCommand command = new SqlCommand(queryString, connection))
{
command.CommandTimeout = 120;
SqlDataReader reader = command.ExecuteReader();
if (reader.HasRows)
{
StringBuilder sb = new StringBuilder();
List<string> columnNames = new List<string>();
List<string> rows = new List<string>();
for (int i = 0; i < reader.FieldCount; i++)
{
string tmpColumnName = reader.GetName(i);
columnNames.Add(tmpColumnName);
}
sb.Append(string.Join(",", columnNames.ToArray())).Append("rn");
List<string> currentRow = new List<string>();
while (reader.Read())
{
for (int i = 0; i < reader.FieldCount; i++)
{
object item = reader[i];
sb.Append(item.ToString().Replace(",", ";") + ',');
}
sb.Append("rn");
}
bool emailSentSuccess = true;
using (MailMessage mail = new MailMessage())
{
try
{
using (MemoryStream stream = new MemoryStream(Encoding.ASCII.GetBytes(sb.ToString())))
{
Attachment attachment = new Attachment(stream, new ContentType("text/csv"));
attachment.Name = "hello.csv";
mail.Attachments.Add(attachment);
mail.From = new MailAddress(mailFrom);
mail.To.Add(new MailAddress(reader["EmailAddress"].ToString()));
mail.IsBodyHtml = true;
mail.Subject = mailSubject;
mail.Body = reader["EmailBody"].ToString();
smtp.Send(mail);
}
}
catch (Exception ex)
{
emailSentSuccess = false;
}
}
}
}
The output I test is as below:
add a comment |
You could refer to the following code in your SqlCommand
.
using (SqlCommand command = new SqlCommand(queryString, connection))
{
command.CommandTimeout = 120;
SqlDataReader reader = command.ExecuteReader();
if (reader.HasRows)
{
StringBuilder sb = new StringBuilder();
List<string> columnNames = new List<string>();
List<string> rows = new List<string>();
for (int i = 0; i < reader.FieldCount; i++)
{
string tmpColumnName = reader.GetName(i);
columnNames.Add(tmpColumnName);
}
sb.Append(string.Join(",", columnNames.ToArray())).Append("rn");
List<string> currentRow = new List<string>();
while (reader.Read())
{
for (int i = 0; i < reader.FieldCount; i++)
{
object item = reader[i];
sb.Append(item.ToString().Replace(",", ";") + ',');
}
sb.Append("rn");
}
bool emailSentSuccess = true;
using (MailMessage mail = new MailMessage())
{
try
{
using (MemoryStream stream = new MemoryStream(Encoding.ASCII.GetBytes(sb.ToString())))
{
Attachment attachment = new Attachment(stream, new ContentType("text/csv"));
attachment.Name = "hello.csv";
mail.Attachments.Add(attachment);
mail.From = new MailAddress(mailFrom);
mail.To.Add(new MailAddress(reader["EmailAddress"].ToString()));
mail.IsBodyHtml = true;
mail.Subject = mailSubject;
mail.Body = reader["EmailBody"].ToString();
smtp.Send(mail);
}
}
catch (Exception ex)
{
emailSentSuccess = false;
}
}
}
}
The output I test is as below:
add a comment |
You could refer to the following code in your SqlCommand
.
using (SqlCommand command = new SqlCommand(queryString, connection))
{
command.CommandTimeout = 120;
SqlDataReader reader = command.ExecuteReader();
if (reader.HasRows)
{
StringBuilder sb = new StringBuilder();
List<string> columnNames = new List<string>();
List<string> rows = new List<string>();
for (int i = 0; i < reader.FieldCount; i++)
{
string tmpColumnName = reader.GetName(i);
columnNames.Add(tmpColumnName);
}
sb.Append(string.Join(",", columnNames.ToArray())).Append("rn");
List<string> currentRow = new List<string>();
while (reader.Read())
{
for (int i = 0; i < reader.FieldCount; i++)
{
object item = reader[i];
sb.Append(item.ToString().Replace(",", ";") + ',');
}
sb.Append("rn");
}
bool emailSentSuccess = true;
using (MailMessage mail = new MailMessage())
{
try
{
using (MemoryStream stream = new MemoryStream(Encoding.ASCII.GetBytes(sb.ToString())))
{
Attachment attachment = new Attachment(stream, new ContentType("text/csv"));
attachment.Name = "hello.csv";
mail.Attachments.Add(attachment);
mail.From = new MailAddress(mailFrom);
mail.To.Add(new MailAddress(reader["EmailAddress"].ToString()));
mail.IsBodyHtml = true;
mail.Subject = mailSubject;
mail.Body = reader["EmailBody"].ToString();
smtp.Send(mail);
}
}
catch (Exception ex)
{
emailSentSuccess = false;
}
}
}
}
The output I test is as below:
You could refer to the following code in your SqlCommand
.
using (SqlCommand command = new SqlCommand(queryString, connection))
{
command.CommandTimeout = 120;
SqlDataReader reader = command.ExecuteReader();
if (reader.HasRows)
{
StringBuilder sb = new StringBuilder();
List<string> columnNames = new List<string>();
List<string> rows = new List<string>();
for (int i = 0; i < reader.FieldCount; i++)
{
string tmpColumnName = reader.GetName(i);
columnNames.Add(tmpColumnName);
}
sb.Append(string.Join(",", columnNames.ToArray())).Append("rn");
List<string> currentRow = new List<string>();
while (reader.Read())
{
for (int i = 0; i < reader.FieldCount; i++)
{
object item = reader[i];
sb.Append(item.ToString().Replace(",", ";") + ',');
}
sb.Append("rn");
}
bool emailSentSuccess = true;
using (MailMessage mail = new MailMessage())
{
try
{
using (MemoryStream stream = new MemoryStream(Encoding.ASCII.GetBytes(sb.ToString())))
{
Attachment attachment = new Attachment(stream, new ContentType("text/csv"));
attachment.Name = "hello.csv";
mail.Attachments.Add(attachment);
mail.From = new MailAddress(mailFrom);
mail.To.Add(new MailAddress(reader["EmailAddress"].ToString()));
mail.IsBodyHtml = true;
mail.Subject = mailSubject;
mail.Body = reader["EmailBody"].ToString();
smtp.Send(mail);
}
}
catch (Exception ex)
{
emailSentSuccess = false;
}
}
}
}
The output I test is as below:
answered Nov 26 '18 at 8:50
Joey CaiJoey Cai
5,137129
5,137129
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53450626%2fazure-webjob-to-create-csv-file-from-sql-and-send-as-attachment-via-email%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Oo uyvjz2O5cPWKZsr3,eFFtqklBBXf1X7L7A
1
Not sure about web jobs, But you can easily achieve this using logic app, without coding. Refer my article here
– Jayendran
Nov 26 '18 at 3:55