convert String to Option[String]
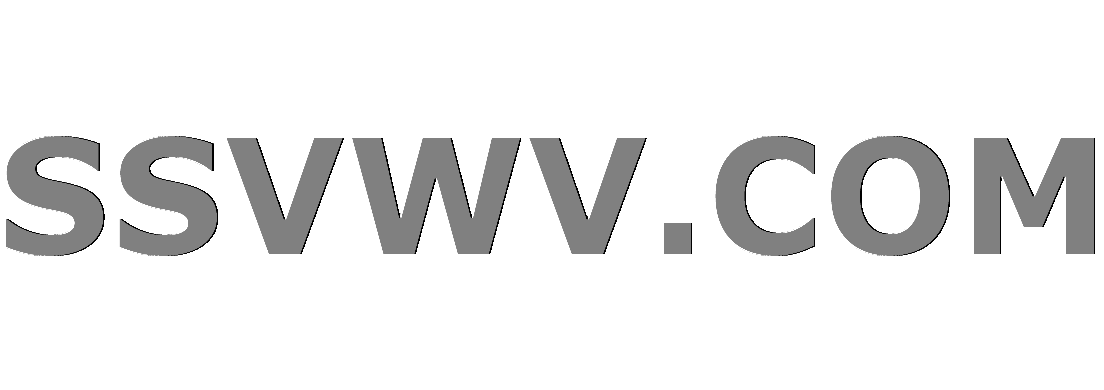
Multi tool use
I am new to scala programming and am facing difficulty to convert from String to Option[String]. I am not sure if the approach I am following is correct or not. Here is the code:
EmpInfo.scala:
package model
case class EmpInfo(empType: Option[String]) extends Named {
override def name: String = clientName
}
EmpReconciliator.scala
import scalaz._
import Scalaz._
import scala.concurrent.Future
import play.api.mvc.Action
import play.api.mvc.AnyContent
import model.EmpInfo
trait EmpReconciliator[A] extends BaseController[A] {
val oldName = "abc"
val newName = "xyz"
def reconcileGeneric(genericResource: A) = {
(genericResource match {
case empInfo : EmpInfo => reconcileEmpInfo(empInfo)
case other@_ => other
}).asInstanceOf[A]
}
def reconcileEmpInfo(empInfo: EmpInfo) = {
empInfo.empTypeType match {
case Some(oldName) => empInfo.copy(empType = Some(newName))
case _ => empInfo
}
}
}
Here, I need to replace all instances of oldName
with newName
.
But instead, I am getting "1"
in the response. I cannot change type of empType: Option[String]
to String
(need it that way).
I know it's a very minor change needed but cannot place what.
Please help.
scala playframework
add a comment |
I am new to scala programming and am facing difficulty to convert from String to Option[String]. I am not sure if the approach I am following is correct or not. Here is the code:
EmpInfo.scala:
package model
case class EmpInfo(empType: Option[String]) extends Named {
override def name: String = clientName
}
EmpReconciliator.scala
import scalaz._
import Scalaz._
import scala.concurrent.Future
import play.api.mvc.Action
import play.api.mvc.AnyContent
import model.EmpInfo
trait EmpReconciliator[A] extends BaseController[A] {
val oldName = "abc"
val newName = "xyz"
def reconcileGeneric(genericResource: A) = {
(genericResource match {
case empInfo : EmpInfo => reconcileEmpInfo(empInfo)
case other@_ => other
}).asInstanceOf[A]
}
def reconcileEmpInfo(empInfo: EmpInfo) = {
empInfo.empTypeType match {
case Some(oldName) => empInfo.copy(empType = Some(newName))
case _ => empInfo
}
}
}
Here, I need to replace all instances of oldName
with newName
.
But instead, I am getting "1"
in the response. I cannot change type of empType: Option[String]
to String
(need it that way).
I know it's a very minor change needed but cannot place what.
Please help.
scala playframework
1
For what input do you get"1"
as a response?
– Cyrille Corpet
May 22 '17 at 9:25
add a comment |
I am new to scala programming and am facing difficulty to convert from String to Option[String]. I am not sure if the approach I am following is correct or not. Here is the code:
EmpInfo.scala:
package model
case class EmpInfo(empType: Option[String]) extends Named {
override def name: String = clientName
}
EmpReconciliator.scala
import scalaz._
import Scalaz._
import scala.concurrent.Future
import play.api.mvc.Action
import play.api.mvc.AnyContent
import model.EmpInfo
trait EmpReconciliator[A] extends BaseController[A] {
val oldName = "abc"
val newName = "xyz"
def reconcileGeneric(genericResource: A) = {
(genericResource match {
case empInfo : EmpInfo => reconcileEmpInfo(empInfo)
case other@_ => other
}).asInstanceOf[A]
}
def reconcileEmpInfo(empInfo: EmpInfo) = {
empInfo.empTypeType match {
case Some(oldName) => empInfo.copy(empType = Some(newName))
case _ => empInfo
}
}
}
Here, I need to replace all instances of oldName
with newName
.
But instead, I am getting "1"
in the response. I cannot change type of empType: Option[String]
to String
(need it that way).
I know it's a very minor change needed but cannot place what.
Please help.
scala playframework
I am new to scala programming and am facing difficulty to convert from String to Option[String]. I am not sure if the approach I am following is correct or not. Here is the code:
EmpInfo.scala:
package model
case class EmpInfo(empType: Option[String]) extends Named {
override def name: String = clientName
}
EmpReconciliator.scala
import scalaz._
import Scalaz._
import scala.concurrent.Future
import play.api.mvc.Action
import play.api.mvc.AnyContent
import model.EmpInfo
trait EmpReconciliator[A] extends BaseController[A] {
val oldName = "abc"
val newName = "xyz"
def reconcileGeneric(genericResource: A) = {
(genericResource match {
case empInfo : EmpInfo => reconcileEmpInfo(empInfo)
case other@_ => other
}).asInstanceOf[A]
}
def reconcileEmpInfo(empInfo: EmpInfo) = {
empInfo.empTypeType match {
case Some(oldName) => empInfo.copy(empType = Some(newName))
case _ => empInfo
}
}
}
Here, I need to replace all instances of oldName
with newName
.
But instead, I am getting "1"
in the response. I cannot change type of empType: Option[String]
to String
(need it that way).
I know it's a very minor change needed but cannot place what.
Please help.
scala playframework
scala playframework
edited May 22 '17 at 9:24
Jasper-M
9,09211329
9,09211329
asked May 22 '17 at 8:57
b1399877b1399877
125
125
1
For what input do you get"1"
as a response?
– Cyrille Corpet
May 22 '17 at 9:25
add a comment |
1
For what input do you get"1"
as a response?
– Cyrille Corpet
May 22 '17 at 9:25
1
1
For what input do you get
"1"
as a response?– Cyrille Corpet
May 22 '17 at 9:25
For what input do you get
"1"
as a response?– Cyrille Corpet
May 22 '17 at 9:25
add a comment |
3 Answers
3
active
oldest
votes
I don't understand your question. But if you want to use oldName
as a pattern, you have to capitalize it.
val OldName = "abc"
val newName = "xyz"
empInfo.empType match {
case Some(OldName) => empInfo.copy(empType = Some(newName))
case _ => empInfo
}
If that doesn't solve the problem you're having, please state your problem more clearly and precisely.
1
see, also, stackoverflow.com/questions/7078022/…
– Yaneeve
May 22 '17 at 10:24
Type ofSome("a string")
:Option[String]
. GetString
Value of aOption[String]
type:str.get
– Seraf
Aug 24 '17 at 17:29
add a comment |
I think you have to add toString()
here
case Some(oldName) => empInfo.copy(empType =
Some(newName)).toString()
case _ => empInfo
add a comment |
As I understand, the problem that you are facing is to convert a String
type to Option[String]
type.
Then try wrapping it like this: Option(<variablename>)
, as in Option(newvalue)
in your example.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f44108671%2fconvert-string-to-optionstring%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
I don't understand your question. But if you want to use oldName
as a pattern, you have to capitalize it.
val OldName = "abc"
val newName = "xyz"
empInfo.empType match {
case Some(OldName) => empInfo.copy(empType = Some(newName))
case _ => empInfo
}
If that doesn't solve the problem you're having, please state your problem more clearly and precisely.
1
see, also, stackoverflow.com/questions/7078022/…
– Yaneeve
May 22 '17 at 10:24
Type ofSome("a string")
:Option[String]
. GetString
Value of aOption[String]
type:str.get
– Seraf
Aug 24 '17 at 17:29
add a comment |
I don't understand your question. But if you want to use oldName
as a pattern, you have to capitalize it.
val OldName = "abc"
val newName = "xyz"
empInfo.empType match {
case Some(OldName) => empInfo.copy(empType = Some(newName))
case _ => empInfo
}
If that doesn't solve the problem you're having, please state your problem more clearly and precisely.
1
see, also, stackoverflow.com/questions/7078022/…
– Yaneeve
May 22 '17 at 10:24
Type ofSome("a string")
:Option[String]
. GetString
Value of aOption[String]
type:str.get
– Seraf
Aug 24 '17 at 17:29
add a comment |
I don't understand your question. But if you want to use oldName
as a pattern, you have to capitalize it.
val OldName = "abc"
val newName = "xyz"
empInfo.empType match {
case Some(OldName) => empInfo.copy(empType = Some(newName))
case _ => empInfo
}
If that doesn't solve the problem you're having, please state your problem more clearly and precisely.
I don't understand your question. But if you want to use oldName
as a pattern, you have to capitalize it.
val OldName = "abc"
val newName = "xyz"
empInfo.empType match {
case Some(OldName) => empInfo.copy(empType = Some(newName))
case _ => empInfo
}
If that doesn't solve the problem you're having, please state your problem more clearly and precisely.
edited May 22 '17 at 9:35
answered May 22 '17 at 9:30
Jasper-MJasper-M
9,09211329
9,09211329
1
see, also, stackoverflow.com/questions/7078022/…
– Yaneeve
May 22 '17 at 10:24
Type ofSome("a string")
:Option[String]
. GetString
Value of aOption[String]
type:str.get
– Seraf
Aug 24 '17 at 17:29
add a comment |
1
see, also, stackoverflow.com/questions/7078022/…
– Yaneeve
May 22 '17 at 10:24
Type ofSome("a string")
:Option[String]
. GetString
Value of aOption[String]
type:str.get
– Seraf
Aug 24 '17 at 17:29
1
1
see, also, stackoverflow.com/questions/7078022/…
– Yaneeve
May 22 '17 at 10:24
see, also, stackoverflow.com/questions/7078022/…
– Yaneeve
May 22 '17 at 10:24
Type of
Some("a string")
: Option[String]
. Get String
Value of a Option[String]
type: str.get
– Seraf
Aug 24 '17 at 17:29
Type of
Some("a string")
: Option[String]
. Get String
Value of a Option[String]
type: str.get
– Seraf
Aug 24 '17 at 17:29
add a comment |
I think you have to add toString()
here
case Some(oldName) => empInfo.copy(empType =
Some(newName)).toString()
case _ => empInfo
add a comment |
I think you have to add toString()
here
case Some(oldName) => empInfo.copy(empType =
Some(newName)).toString()
case _ => empInfo
add a comment |
I think you have to add toString()
here
case Some(oldName) => empInfo.copy(empType =
Some(newName)).toString()
case _ => empInfo
I think you have to add toString()
here
case Some(oldName) => empInfo.copy(empType =
Some(newName)).toString()
case _ => empInfo
answered May 22 '17 at 9:02
manikandanmanikandan
12
12
add a comment |
add a comment |
As I understand, the problem that you are facing is to convert a String
type to Option[String]
type.
Then try wrapping it like this: Option(<variablename>)
, as in Option(newvalue)
in your example.
add a comment |
As I understand, the problem that you are facing is to convert a String
type to Option[String]
type.
Then try wrapping it like this: Option(<variablename>)
, as in Option(newvalue)
in your example.
add a comment |
As I understand, the problem that you are facing is to convert a String
type to Option[String]
type.
Then try wrapping it like this: Option(<variablename>)
, as in Option(newvalue)
in your example.
As I understand, the problem that you are facing is to convert a String
type to Option[String]
type.
Then try wrapping it like this: Option(<variablename>)
, as in Option(newvalue)
in your example.
edited Nov 23 '18 at 17:00


Alonso Dominguez
6,28311936
6,28311936
answered Nov 23 '18 at 12:38
RaviRavi
1
1
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f44108671%2fconvert-string-to-optionstring%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
3CqTC8k E1pHzRp8hUM2ONJ7uTzl1C95t5CMd0YF np
1
For what input do you get
"1"
as a response?– Cyrille Corpet
May 22 '17 at 9:25