C libcurl smaller than expected response size
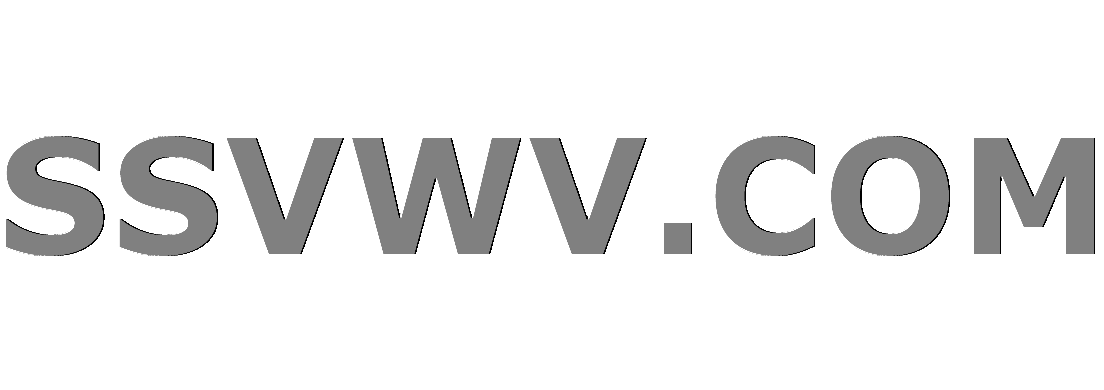
Multi tool use
I'm trying to use libcurl to download the content of a webpage however the responses I get back are much smaller than expected - the documentation says that the maximum size is 16K but the responses I'm getting are much much smaller than that.
For example, requesting the en Wikipedia page "Hello" returns a size of 1239 bytes when the page is 71915 bytes in gedit. Requesting a page from the haveibeenpwned API returns a size of 554, not the actual size which is 19942 bytes.
int callback(char* resultPtr, size_t ignore, size_t resultSize) { // resultPtr points to the start of the response, ignore is always 1, resultSize varies
printf("Response size is %i", resultSize);
for(int i = 0; i < resultSize; i++) { printf("%c", *(resultPtr+i));} // credit @BartFriederichs
return 0;
}
int doCurl(char* paramsPtr) {
char url[43]; // Add space for /0
CURL *curl = curl_easy_init(); // Initialise cURL
strcpy(url, "https://api.pwnedpasswords.com/range/"); // Testing URL
strcat(url, &*paramsPtr); // Always 5 characters
curl_easy_setopt(curl, CURLOPT_URL, url); // cURL URL set to URL specified above
curl_easy_setopt(curl, CURLOPT_BUFFERSIZE, 120000L); // Tried to set this to make the output longer
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, callback);
curl_easy_perform(curl);
return 0;
}
The point of this code is to get the response from HIBP's Pwned Passwords API and compare a string given by the user to the results from the webpage. The search succeed for any strings within the first 554 bytes (first chunk of data output by cURL) but fail after that.
c curl libcurl
|
show 2 more comments
I'm trying to use libcurl to download the content of a webpage however the responses I get back are much smaller than expected - the documentation says that the maximum size is 16K but the responses I'm getting are much much smaller than that.
For example, requesting the en Wikipedia page "Hello" returns a size of 1239 bytes when the page is 71915 bytes in gedit. Requesting a page from the haveibeenpwned API returns a size of 554, not the actual size which is 19942 bytes.
int callback(char* resultPtr, size_t ignore, size_t resultSize) { // resultPtr points to the start of the response, ignore is always 1, resultSize varies
printf("Response size is %i", resultSize);
for(int i = 0; i < resultSize; i++) { printf("%c", *(resultPtr+i));} // credit @BartFriederichs
return 0;
}
int doCurl(char* paramsPtr) {
char url[43]; // Add space for /0
CURL *curl = curl_easy_init(); // Initialise cURL
strcpy(url, "https://api.pwnedpasswords.com/range/"); // Testing URL
strcat(url, &*paramsPtr); // Always 5 characters
curl_easy_setopt(curl, CURLOPT_URL, url); // cURL URL set to URL specified above
curl_easy_setopt(curl, CURLOPT_BUFFERSIZE, 120000L); // Tried to set this to make the output longer
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, callback);
curl_easy_perform(curl);
return 0;
}
The point of this code is to get the response from HIBP's Pwned Passwords API and compare a string given by the user to the results from the webpage. The search succeed for any strings within the first 554 bytes (first chunk of data output by cURL) but fail after that.
c curl libcurl
What is in the result? Is the actual data chopped off, or are you actually getting something different (e.g. headers).
– Bart Friederichs
Nov 23 '18 at 14:50
You declaredurl
as an array of 35 chars. After copyinghttps://en.wikipedia.org/wiki/
and your 5-character string into this array, you've used up all of these 35 characters. There is no room left for the''
string terminator. What you're probably seeing are 404 error pages.
– squeamish ossifrage
Nov 23 '18 at 14:53
@BartFriederichs: I've just looked, runningfor(int i = 0; i < resultSize; i++) { printf("%c", &*resultPtr); }
outputs this: imgur.com/a/GJwHnqD. I think I may have misunderstood the writefunction, it looks like it's calling it multiple times?
– maxic
Nov 23 '18 at 14:59
@squeamishossifrage a Wikipedia 404 is longer than 1239 bytes.
– Bart Friederichs
Nov 23 '18 at 14:59
@squeamishossifrage Good point - I have changed this however the problem still exists.
– maxic
Nov 23 '18 at 15:00
|
show 2 more comments
I'm trying to use libcurl to download the content of a webpage however the responses I get back are much smaller than expected - the documentation says that the maximum size is 16K but the responses I'm getting are much much smaller than that.
For example, requesting the en Wikipedia page "Hello" returns a size of 1239 bytes when the page is 71915 bytes in gedit. Requesting a page from the haveibeenpwned API returns a size of 554, not the actual size which is 19942 bytes.
int callback(char* resultPtr, size_t ignore, size_t resultSize) { // resultPtr points to the start of the response, ignore is always 1, resultSize varies
printf("Response size is %i", resultSize);
for(int i = 0; i < resultSize; i++) { printf("%c", *(resultPtr+i));} // credit @BartFriederichs
return 0;
}
int doCurl(char* paramsPtr) {
char url[43]; // Add space for /0
CURL *curl = curl_easy_init(); // Initialise cURL
strcpy(url, "https://api.pwnedpasswords.com/range/"); // Testing URL
strcat(url, &*paramsPtr); // Always 5 characters
curl_easy_setopt(curl, CURLOPT_URL, url); // cURL URL set to URL specified above
curl_easy_setopt(curl, CURLOPT_BUFFERSIZE, 120000L); // Tried to set this to make the output longer
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, callback);
curl_easy_perform(curl);
return 0;
}
The point of this code is to get the response from HIBP's Pwned Passwords API and compare a string given by the user to the results from the webpage. The search succeed for any strings within the first 554 bytes (first chunk of data output by cURL) but fail after that.
c curl libcurl
I'm trying to use libcurl to download the content of a webpage however the responses I get back are much smaller than expected - the documentation says that the maximum size is 16K but the responses I'm getting are much much smaller than that.
For example, requesting the en Wikipedia page "Hello" returns a size of 1239 bytes when the page is 71915 bytes in gedit. Requesting a page from the haveibeenpwned API returns a size of 554, not the actual size which is 19942 bytes.
int callback(char* resultPtr, size_t ignore, size_t resultSize) { // resultPtr points to the start of the response, ignore is always 1, resultSize varies
printf("Response size is %i", resultSize);
for(int i = 0; i < resultSize; i++) { printf("%c", *(resultPtr+i));} // credit @BartFriederichs
return 0;
}
int doCurl(char* paramsPtr) {
char url[43]; // Add space for /0
CURL *curl = curl_easy_init(); // Initialise cURL
strcpy(url, "https://api.pwnedpasswords.com/range/"); // Testing URL
strcat(url, &*paramsPtr); // Always 5 characters
curl_easy_setopt(curl, CURLOPT_URL, url); // cURL URL set to URL specified above
curl_easy_setopt(curl, CURLOPT_BUFFERSIZE, 120000L); // Tried to set this to make the output longer
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, callback);
curl_easy_perform(curl);
return 0;
}
The point of this code is to get the response from HIBP's Pwned Passwords API and compare a string given by the user to the results from the webpage. The search succeed for any strings within the first 554 bytes (first chunk of data output by cURL) but fail after that.
c curl libcurl
c curl libcurl
edited Nov 23 '18 at 15:06
maxic
asked Nov 23 '18 at 14:39


maxicmaxic
34
34
What is in the result? Is the actual data chopped off, or are you actually getting something different (e.g. headers).
– Bart Friederichs
Nov 23 '18 at 14:50
You declaredurl
as an array of 35 chars. After copyinghttps://en.wikipedia.org/wiki/
and your 5-character string into this array, you've used up all of these 35 characters. There is no room left for the''
string terminator. What you're probably seeing are 404 error pages.
– squeamish ossifrage
Nov 23 '18 at 14:53
@BartFriederichs: I've just looked, runningfor(int i = 0; i < resultSize; i++) { printf("%c", &*resultPtr); }
outputs this: imgur.com/a/GJwHnqD. I think I may have misunderstood the writefunction, it looks like it's calling it multiple times?
– maxic
Nov 23 '18 at 14:59
@squeamishossifrage a Wikipedia 404 is longer than 1239 bytes.
– Bart Friederichs
Nov 23 '18 at 14:59
@squeamishossifrage Good point - I have changed this however the problem still exists.
– maxic
Nov 23 '18 at 15:00
|
show 2 more comments
What is in the result? Is the actual data chopped off, or are you actually getting something different (e.g. headers).
– Bart Friederichs
Nov 23 '18 at 14:50
You declaredurl
as an array of 35 chars. After copyinghttps://en.wikipedia.org/wiki/
and your 5-character string into this array, you've used up all of these 35 characters. There is no room left for the''
string terminator. What you're probably seeing are 404 error pages.
– squeamish ossifrage
Nov 23 '18 at 14:53
@BartFriederichs: I've just looked, runningfor(int i = 0; i < resultSize; i++) { printf("%c", &*resultPtr); }
outputs this: imgur.com/a/GJwHnqD. I think I may have misunderstood the writefunction, it looks like it's calling it multiple times?
– maxic
Nov 23 '18 at 14:59
@squeamishossifrage a Wikipedia 404 is longer than 1239 bytes.
– Bart Friederichs
Nov 23 '18 at 14:59
@squeamishossifrage Good point - I have changed this however the problem still exists.
– maxic
Nov 23 '18 at 15:00
What is in the result? Is the actual data chopped off, or are you actually getting something different (e.g. headers).
– Bart Friederichs
Nov 23 '18 at 14:50
What is in the result? Is the actual data chopped off, or are you actually getting something different (e.g. headers).
– Bart Friederichs
Nov 23 '18 at 14:50
You declared
url
as an array of 35 chars. After copying https://en.wikipedia.org/wiki/
and your 5-character string into this array, you've used up all of these 35 characters. There is no room left for the ''
string terminator. What you're probably seeing are 404 error pages.– squeamish ossifrage
Nov 23 '18 at 14:53
You declared
url
as an array of 35 chars. After copying https://en.wikipedia.org/wiki/
and your 5-character string into this array, you've used up all of these 35 characters. There is no room left for the ''
string terminator. What you're probably seeing are 404 error pages.– squeamish ossifrage
Nov 23 '18 at 14:53
@BartFriederichs: I've just looked, running
for(int i = 0; i < resultSize; i++) { printf("%c", &*resultPtr); }
outputs this: imgur.com/a/GJwHnqD. I think I may have misunderstood the writefunction, it looks like it's calling it multiple times?– maxic
Nov 23 '18 at 14:59
@BartFriederichs: I've just looked, running
for(int i = 0; i < resultSize; i++) { printf("%c", &*resultPtr); }
outputs this: imgur.com/a/GJwHnqD. I think I may have misunderstood the writefunction, it looks like it's calling it multiple times?– maxic
Nov 23 '18 at 14:59
@squeamishossifrage a Wikipedia 404 is longer than 1239 bytes.
– Bart Friederichs
Nov 23 '18 at 14:59
@squeamishossifrage a Wikipedia 404 is longer than 1239 bytes.
– Bart Friederichs
Nov 23 '18 at 14:59
@squeamishossifrage Good point - I have changed this however the problem still exists.
– maxic
Nov 23 '18 at 15:00
@squeamishossifrage Good point - I have changed this however the problem still exists.
– maxic
Nov 23 '18 at 15:00
|
show 2 more comments
1 Answer
1
active
oldest
votes
Check out the documentation. It says:
The callback function will be passed as much data as possible in all invokes, but you must not make any assumptions. It may be one byte, it may be thousands.
It doesn't say so explicitly, but this function might be called more than once. You can use the userdata
pointer (which you haven't used) to keep track of your previous data. I advise you also take a look at the example code:
struct MemoryStruct {
char *memory;
size_t size;
};
static size_t
WriteMemoryCallback(void *contents, size_t size, size_t nmemb, void *userp)
{
size_t realsize = size * nmemb;
struct MemoryStruct *mem = (struct MemoryStruct *)userp;
char *ptr = realloc(mem->memory, mem->size + realsize + 1);
if(ptr == NULL) {
/* out of memory! */
printf("not enough memory (realloc returned NULL)n");
return 0;
}
mem->memory = ptr;
memcpy(&(mem->memory[mem->size]), contents, realsize);
mem->size += realsize;
mem->memory[mem->size] = 0;
return realsize;
}
1
"It doesn't say so explicitly" ... thanks, I took this feedback and have updated the man page to make this fact more explicit!
– Daniel Stenberg
Nov 24 '18 at 22:07
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53448685%2fc-libcurl-smaller-than-expected-response-size%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Check out the documentation. It says:
The callback function will be passed as much data as possible in all invokes, but you must not make any assumptions. It may be one byte, it may be thousands.
It doesn't say so explicitly, but this function might be called more than once. You can use the userdata
pointer (which you haven't used) to keep track of your previous data. I advise you also take a look at the example code:
struct MemoryStruct {
char *memory;
size_t size;
};
static size_t
WriteMemoryCallback(void *contents, size_t size, size_t nmemb, void *userp)
{
size_t realsize = size * nmemb;
struct MemoryStruct *mem = (struct MemoryStruct *)userp;
char *ptr = realloc(mem->memory, mem->size + realsize + 1);
if(ptr == NULL) {
/* out of memory! */
printf("not enough memory (realloc returned NULL)n");
return 0;
}
mem->memory = ptr;
memcpy(&(mem->memory[mem->size]), contents, realsize);
mem->size += realsize;
mem->memory[mem->size] = 0;
return realsize;
}
1
"It doesn't say so explicitly" ... thanks, I took this feedback and have updated the man page to make this fact more explicit!
– Daniel Stenberg
Nov 24 '18 at 22:07
add a comment |
Check out the documentation. It says:
The callback function will be passed as much data as possible in all invokes, but you must not make any assumptions. It may be one byte, it may be thousands.
It doesn't say so explicitly, but this function might be called more than once. You can use the userdata
pointer (which you haven't used) to keep track of your previous data. I advise you also take a look at the example code:
struct MemoryStruct {
char *memory;
size_t size;
};
static size_t
WriteMemoryCallback(void *contents, size_t size, size_t nmemb, void *userp)
{
size_t realsize = size * nmemb;
struct MemoryStruct *mem = (struct MemoryStruct *)userp;
char *ptr = realloc(mem->memory, mem->size + realsize + 1);
if(ptr == NULL) {
/* out of memory! */
printf("not enough memory (realloc returned NULL)n");
return 0;
}
mem->memory = ptr;
memcpy(&(mem->memory[mem->size]), contents, realsize);
mem->size += realsize;
mem->memory[mem->size] = 0;
return realsize;
}
1
"It doesn't say so explicitly" ... thanks, I took this feedback and have updated the man page to make this fact more explicit!
– Daniel Stenberg
Nov 24 '18 at 22:07
add a comment |
Check out the documentation. It says:
The callback function will be passed as much data as possible in all invokes, but you must not make any assumptions. It may be one byte, it may be thousands.
It doesn't say so explicitly, but this function might be called more than once. You can use the userdata
pointer (which you haven't used) to keep track of your previous data. I advise you also take a look at the example code:
struct MemoryStruct {
char *memory;
size_t size;
};
static size_t
WriteMemoryCallback(void *contents, size_t size, size_t nmemb, void *userp)
{
size_t realsize = size * nmemb;
struct MemoryStruct *mem = (struct MemoryStruct *)userp;
char *ptr = realloc(mem->memory, mem->size + realsize + 1);
if(ptr == NULL) {
/* out of memory! */
printf("not enough memory (realloc returned NULL)n");
return 0;
}
mem->memory = ptr;
memcpy(&(mem->memory[mem->size]), contents, realsize);
mem->size += realsize;
mem->memory[mem->size] = 0;
return realsize;
}
Check out the documentation. It says:
The callback function will be passed as much data as possible in all invokes, but you must not make any assumptions. It may be one byte, it may be thousands.
It doesn't say so explicitly, but this function might be called more than once. You can use the userdata
pointer (which you haven't used) to keep track of your previous data. I advise you also take a look at the example code:
struct MemoryStruct {
char *memory;
size_t size;
};
static size_t
WriteMemoryCallback(void *contents, size_t size, size_t nmemb, void *userp)
{
size_t realsize = size * nmemb;
struct MemoryStruct *mem = (struct MemoryStruct *)userp;
char *ptr = realloc(mem->memory, mem->size + realsize + 1);
if(ptr == NULL) {
/* out of memory! */
printf("not enough memory (realloc returned NULL)n");
return 0;
}
mem->memory = ptr;
memcpy(&(mem->memory[mem->size]), contents, realsize);
mem->size += realsize;
mem->memory[mem->size] = 0;
return realsize;
}
answered Nov 23 '18 at 15:08


Bart FriederichsBart Friederichs
24.7k1164123
24.7k1164123
1
"It doesn't say so explicitly" ... thanks, I took this feedback and have updated the man page to make this fact more explicit!
– Daniel Stenberg
Nov 24 '18 at 22:07
add a comment |
1
"It doesn't say so explicitly" ... thanks, I took this feedback and have updated the man page to make this fact more explicit!
– Daniel Stenberg
Nov 24 '18 at 22:07
1
1
"It doesn't say so explicitly" ... thanks, I took this feedback and have updated the man page to make this fact more explicit!
– Daniel Stenberg
Nov 24 '18 at 22:07
"It doesn't say so explicitly" ... thanks, I took this feedback and have updated the man page to make this fact more explicit!
– Daniel Stenberg
Nov 24 '18 at 22:07
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53448685%2fc-libcurl-smaller-than-expected-response-size%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
NJ1n7pNWi,7NbDKA ktuJQXpcfd9W6iVgo1uTrTqhlC7AwGNFFhRP7,l,vvz H2Kh,4IfZ15vzgHU cLUL9
What is in the result? Is the actual data chopped off, or are you actually getting something different (e.g. headers).
– Bart Friederichs
Nov 23 '18 at 14:50
You declared
url
as an array of 35 chars. After copyinghttps://en.wikipedia.org/wiki/
and your 5-character string into this array, you've used up all of these 35 characters. There is no room left for the''
string terminator. What you're probably seeing are 404 error pages.– squeamish ossifrage
Nov 23 '18 at 14:53
@BartFriederichs: I've just looked, running
for(int i = 0; i < resultSize; i++) { printf("%c", &*resultPtr); }
outputs this: imgur.com/a/GJwHnqD. I think I may have misunderstood the writefunction, it looks like it's calling it multiple times?– maxic
Nov 23 '18 at 14:59
@squeamishossifrage a Wikipedia 404 is longer than 1239 bytes.
– Bart Friederichs
Nov 23 '18 at 14:59
@squeamishossifrage Good point - I have changed this however the problem still exists.
– maxic
Nov 23 '18 at 15:00