AnnotationConfigApplicationContext has not been refreshed yet using ApplicationContextAware
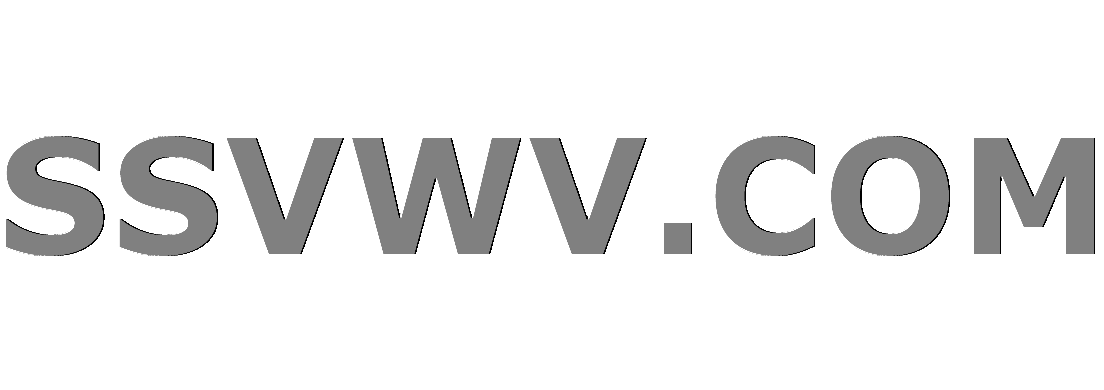
Multi tool use
I am using Spring beans in a non-spring framework and for that I have implemented the ApplicationContextAware to access the spring beans.
@Service
public class ApplicationContextProviderService implements ApplicationContextAware {
private static ApplicationContext applicationContext;
@Override
public void setApplicationContext(ApplicationContext applicationContext) throws BeansException {
ApplicationContextProviderService.applicationContext = applicationContext;
}
public static <T> T getBean(Class<T> beanType) {
System.out.println("bean out: " + applicationContext);
return applicationContext.getBean(beanType);
}
}
I try to access a Spring service: ConnectionStateService from the non-spring class:
this.connectionStateService = ApplicationContextProviderService.getBean(ConnectionStateService.class);
I get the following error:
java.lang.IllegalStateException:
**org.springframework.context.annotation.AnnotationConfigApplicationContext@7f485fda has not been refreshed yet at
** org.springframework.context.support.AbstractApplicationContext.assertBeanFactoryActive(AbstractApplicationContext.java:1072) ~[spring-context-5.0.4.RELEASE.jar:5.0.4.RELEASE] at org.springframework.context.support.AbstractApplicationContext.getBean(AbstractApplicationContext.java:1102) ~[spring-context-5.0.4.RELEASE.jar:5.0.4.RELEASE] at com.example.app.service.ApplicationContextProviderService.getBean(ApplicationContextProviderService.java:19) ~[classes/:na] at com.example.app.CustomFilter.<init>(CustomFilter.java:26) ~[classes/:na]
How to resolve this issue?
java spring spring-boot
add a comment |
I am using Spring beans in a non-spring framework and for that I have implemented the ApplicationContextAware to access the spring beans.
@Service
public class ApplicationContextProviderService implements ApplicationContextAware {
private static ApplicationContext applicationContext;
@Override
public void setApplicationContext(ApplicationContext applicationContext) throws BeansException {
ApplicationContextProviderService.applicationContext = applicationContext;
}
public static <T> T getBean(Class<T> beanType) {
System.out.println("bean out: " + applicationContext);
return applicationContext.getBean(beanType);
}
}
I try to access a Spring service: ConnectionStateService from the non-spring class:
this.connectionStateService = ApplicationContextProviderService.getBean(ConnectionStateService.class);
I get the following error:
java.lang.IllegalStateException:
**org.springframework.context.annotation.AnnotationConfigApplicationContext@7f485fda has not been refreshed yet at
** org.springframework.context.support.AbstractApplicationContext.assertBeanFactoryActive(AbstractApplicationContext.java:1072) ~[spring-context-5.0.4.RELEASE.jar:5.0.4.RELEASE] at org.springframework.context.support.AbstractApplicationContext.getBean(AbstractApplicationContext.java:1102) ~[spring-context-5.0.4.RELEASE.jar:5.0.4.RELEASE] at com.example.app.service.ApplicationContextProviderService.getBean(ApplicationContextProviderService.java:19) ~[classes/:na] at com.example.app.CustomFilter.<init>(CustomFilter.java:26) ~[classes/:na]
How to resolve this issue?
java spring spring-boot
add a comment |
I am using Spring beans in a non-spring framework and for that I have implemented the ApplicationContextAware to access the spring beans.
@Service
public class ApplicationContextProviderService implements ApplicationContextAware {
private static ApplicationContext applicationContext;
@Override
public void setApplicationContext(ApplicationContext applicationContext) throws BeansException {
ApplicationContextProviderService.applicationContext = applicationContext;
}
public static <T> T getBean(Class<T> beanType) {
System.out.println("bean out: " + applicationContext);
return applicationContext.getBean(beanType);
}
}
I try to access a Spring service: ConnectionStateService from the non-spring class:
this.connectionStateService = ApplicationContextProviderService.getBean(ConnectionStateService.class);
I get the following error:
java.lang.IllegalStateException:
**org.springframework.context.annotation.AnnotationConfigApplicationContext@7f485fda has not been refreshed yet at
** org.springframework.context.support.AbstractApplicationContext.assertBeanFactoryActive(AbstractApplicationContext.java:1072) ~[spring-context-5.0.4.RELEASE.jar:5.0.4.RELEASE] at org.springframework.context.support.AbstractApplicationContext.getBean(AbstractApplicationContext.java:1102) ~[spring-context-5.0.4.RELEASE.jar:5.0.4.RELEASE] at com.example.app.service.ApplicationContextProviderService.getBean(ApplicationContextProviderService.java:19) ~[classes/:na] at com.example.app.CustomFilter.<init>(CustomFilter.java:26) ~[classes/:na]
How to resolve this issue?
java spring spring-boot
I am using Spring beans in a non-spring framework and for that I have implemented the ApplicationContextAware to access the spring beans.
@Service
public class ApplicationContextProviderService implements ApplicationContextAware {
private static ApplicationContext applicationContext;
@Override
public void setApplicationContext(ApplicationContext applicationContext) throws BeansException {
ApplicationContextProviderService.applicationContext = applicationContext;
}
public static <T> T getBean(Class<T> beanType) {
System.out.println("bean out: " + applicationContext);
return applicationContext.getBean(beanType);
}
}
I try to access a Spring service: ConnectionStateService from the non-spring class:
this.connectionStateService = ApplicationContextProviderService.getBean(ConnectionStateService.class);
I get the following error:
java.lang.IllegalStateException:
**org.springframework.context.annotation.AnnotationConfigApplicationContext@7f485fda has not been refreshed yet at
** org.springframework.context.support.AbstractApplicationContext.assertBeanFactoryActive(AbstractApplicationContext.java:1072) ~[spring-context-5.0.4.RELEASE.jar:5.0.4.RELEASE] at org.springframework.context.support.AbstractApplicationContext.getBean(AbstractApplicationContext.java:1102) ~[spring-context-5.0.4.RELEASE.jar:5.0.4.RELEASE] at com.example.app.service.ApplicationContextProviderService.getBean(ApplicationContextProviderService.java:19) ~[classes/:na] at com.example.app.CustomFilter.<init>(CustomFilter.java:26) ~[classes/:na]
How to resolve this issue?
java spring spring-boot
java spring spring-boot
edited Nov 25 '18 at 23:55
conquester
asked Nov 25 '18 at 23:47
conquesterconquester
15012
15012
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
The issue is related when the application tries to call to ApplicationContext before the application runs, so you won't be able to have application context because it wasn't created. The solution is create a singleton for ConnectionStateService class so you won't need to create a service for calling the ApplicationContext.
public class ConnectionStateService {
// static variable instance of type Singleton
private static ConnectionStateService single_instance = null;
// private constructor restricted to this class itself
private ConnectionStateService() {
// some stuffs for initialize here
}
// static method to create instance of Singleton class
public static ConnectionStateService getInstance() {
if (instance == null)
instance = new ConnectionStateService();
return instance;
}
}
Service annotation is inhereited from @Component
– Mykhailo Moskura
Nov 26 '18 at 3:55
You said it’s better to use to inject it before so what it should change ?
– Mykhailo Moskura
Nov 26 '18 at 3:56
Yes I guess, I did a test before and I noticed that it is first Component injected that is referenced like Bean I think that your issue is in method you are calling the context. Could you please add that method?
– Jonathan Johx
Nov 26 '18 at 4:16
Mykhailo has started a chat and I have posted several details there: chat.stackoverflow.com/rooms/184244/… My first post gives the main details.
– conquester
Nov 26 '18 at 4:18
1
This issue was primarily because of application context not being initialized. Although the cause is unknown to me but that was the reason.
– conquester
Nov 28 '18 at 22:17
|
show 1 more comment
I think you are getting the bean when the context is not initialized
So actually be sure you are invoking this piece of code :
this.connectionStateService = ApplicationContextProviderService.getBean(ConnectionStateService.class);
after context is initialized
ApplicationContext object has no refresh method. The bean does get returned when called from a spring context but it doesn't work when called from a non-spring class.
– conquester
Nov 26 '18 at 2:35
AnnotationConfigApplicationContext has it
– Mykhailo Moskura
Nov 26 '18 at 2:43
And you are having an exception. My purposes are that context is not initialized yet
– Mykhailo Moskura
Nov 26 '18 at 2:45
When I do that, I get the error that the context can be refreshed only once.
– conquester
Nov 26 '18 at 2:46
When you are calling your method from non bean class ?
– Mykhailo Moskura
Nov 26 '18 at 2:56
|
show 8 more comments
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53473155%2fannotationconfigapplicationcontext-has-not-been-refreshed-yet-using-applicationc%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
The issue is related when the application tries to call to ApplicationContext before the application runs, so you won't be able to have application context because it wasn't created. The solution is create a singleton for ConnectionStateService class so you won't need to create a service for calling the ApplicationContext.
public class ConnectionStateService {
// static variable instance of type Singleton
private static ConnectionStateService single_instance = null;
// private constructor restricted to this class itself
private ConnectionStateService() {
// some stuffs for initialize here
}
// static method to create instance of Singleton class
public static ConnectionStateService getInstance() {
if (instance == null)
instance = new ConnectionStateService();
return instance;
}
}
Service annotation is inhereited from @Component
– Mykhailo Moskura
Nov 26 '18 at 3:55
You said it’s better to use to inject it before so what it should change ?
– Mykhailo Moskura
Nov 26 '18 at 3:56
Yes I guess, I did a test before and I noticed that it is first Component injected that is referenced like Bean I think that your issue is in method you are calling the context. Could you please add that method?
– Jonathan Johx
Nov 26 '18 at 4:16
Mykhailo has started a chat and I have posted several details there: chat.stackoverflow.com/rooms/184244/… My first post gives the main details.
– conquester
Nov 26 '18 at 4:18
1
This issue was primarily because of application context not being initialized. Although the cause is unknown to me but that was the reason.
– conquester
Nov 28 '18 at 22:17
|
show 1 more comment
The issue is related when the application tries to call to ApplicationContext before the application runs, so you won't be able to have application context because it wasn't created. The solution is create a singleton for ConnectionStateService class so you won't need to create a service for calling the ApplicationContext.
public class ConnectionStateService {
// static variable instance of type Singleton
private static ConnectionStateService single_instance = null;
// private constructor restricted to this class itself
private ConnectionStateService() {
// some stuffs for initialize here
}
// static method to create instance of Singleton class
public static ConnectionStateService getInstance() {
if (instance == null)
instance = new ConnectionStateService();
return instance;
}
}
Service annotation is inhereited from @Component
– Mykhailo Moskura
Nov 26 '18 at 3:55
You said it’s better to use to inject it before so what it should change ?
– Mykhailo Moskura
Nov 26 '18 at 3:56
Yes I guess, I did a test before and I noticed that it is first Component injected that is referenced like Bean I think that your issue is in method you are calling the context. Could you please add that method?
– Jonathan Johx
Nov 26 '18 at 4:16
Mykhailo has started a chat and I have posted several details there: chat.stackoverflow.com/rooms/184244/… My first post gives the main details.
– conquester
Nov 26 '18 at 4:18
1
This issue was primarily because of application context not being initialized. Although the cause is unknown to me but that was the reason.
– conquester
Nov 28 '18 at 22:17
|
show 1 more comment
The issue is related when the application tries to call to ApplicationContext before the application runs, so you won't be able to have application context because it wasn't created. The solution is create a singleton for ConnectionStateService class so you won't need to create a service for calling the ApplicationContext.
public class ConnectionStateService {
// static variable instance of type Singleton
private static ConnectionStateService single_instance = null;
// private constructor restricted to this class itself
private ConnectionStateService() {
// some stuffs for initialize here
}
// static method to create instance of Singleton class
public static ConnectionStateService getInstance() {
if (instance == null)
instance = new ConnectionStateService();
return instance;
}
}
The issue is related when the application tries to call to ApplicationContext before the application runs, so you won't be able to have application context because it wasn't created. The solution is create a singleton for ConnectionStateService class so you won't need to create a service for calling the ApplicationContext.
public class ConnectionStateService {
// static variable instance of type Singleton
private static ConnectionStateService single_instance = null;
// private constructor restricted to this class itself
private ConnectionStateService() {
// some stuffs for initialize here
}
// static method to create instance of Singleton class
public static ConnectionStateService getInstance() {
if (instance == null)
instance = new ConnectionStateService();
return instance;
}
}
edited Nov 27 '18 at 14:57
answered Nov 26 '18 at 3:48


Jonathan JohxJonathan Johx
1,8871418
1,8871418
Service annotation is inhereited from @Component
– Mykhailo Moskura
Nov 26 '18 at 3:55
You said it’s better to use to inject it before so what it should change ?
– Mykhailo Moskura
Nov 26 '18 at 3:56
Yes I guess, I did a test before and I noticed that it is first Component injected that is referenced like Bean I think that your issue is in method you are calling the context. Could you please add that method?
– Jonathan Johx
Nov 26 '18 at 4:16
Mykhailo has started a chat and I have posted several details there: chat.stackoverflow.com/rooms/184244/… My first post gives the main details.
– conquester
Nov 26 '18 at 4:18
1
This issue was primarily because of application context not being initialized. Although the cause is unknown to me but that was the reason.
– conquester
Nov 28 '18 at 22:17
|
show 1 more comment
Service annotation is inhereited from @Component
– Mykhailo Moskura
Nov 26 '18 at 3:55
You said it’s better to use to inject it before so what it should change ?
– Mykhailo Moskura
Nov 26 '18 at 3:56
Yes I guess, I did a test before and I noticed that it is first Component injected that is referenced like Bean I think that your issue is in method you are calling the context. Could you please add that method?
– Jonathan Johx
Nov 26 '18 at 4:16
Mykhailo has started a chat and I have posted several details there: chat.stackoverflow.com/rooms/184244/… My first post gives the main details.
– conquester
Nov 26 '18 at 4:18
1
This issue was primarily because of application context not being initialized. Although the cause is unknown to me but that was the reason.
– conquester
Nov 28 '18 at 22:17
Service annotation is inhereited from @Component
– Mykhailo Moskura
Nov 26 '18 at 3:55
Service annotation is inhereited from @Component
– Mykhailo Moskura
Nov 26 '18 at 3:55
You said it’s better to use to inject it before so what it should change ?
– Mykhailo Moskura
Nov 26 '18 at 3:56
You said it’s better to use to inject it before so what it should change ?
– Mykhailo Moskura
Nov 26 '18 at 3:56
Yes I guess, I did a test before and I noticed that it is first Component injected that is referenced like Bean I think that your issue is in method you are calling the context. Could you please add that method?
– Jonathan Johx
Nov 26 '18 at 4:16
Yes I guess, I did a test before and I noticed that it is first Component injected that is referenced like Bean I think that your issue is in method you are calling the context. Could you please add that method?
– Jonathan Johx
Nov 26 '18 at 4:16
Mykhailo has started a chat and I have posted several details there: chat.stackoverflow.com/rooms/184244/… My first post gives the main details.
– conquester
Nov 26 '18 at 4:18
Mykhailo has started a chat and I have posted several details there: chat.stackoverflow.com/rooms/184244/… My first post gives the main details.
– conquester
Nov 26 '18 at 4:18
1
1
This issue was primarily because of application context not being initialized. Although the cause is unknown to me but that was the reason.
– conquester
Nov 28 '18 at 22:17
This issue was primarily because of application context not being initialized. Although the cause is unknown to me but that was the reason.
– conquester
Nov 28 '18 at 22:17
|
show 1 more comment
I think you are getting the bean when the context is not initialized
So actually be sure you are invoking this piece of code :
this.connectionStateService = ApplicationContextProviderService.getBean(ConnectionStateService.class);
after context is initialized
ApplicationContext object has no refresh method. The bean does get returned when called from a spring context but it doesn't work when called from a non-spring class.
– conquester
Nov 26 '18 at 2:35
AnnotationConfigApplicationContext has it
– Mykhailo Moskura
Nov 26 '18 at 2:43
And you are having an exception. My purposes are that context is not initialized yet
– Mykhailo Moskura
Nov 26 '18 at 2:45
When I do that, I get the error that the context can be refreshed only once.
– conquester
Nov 26 '18 at 2:46
When you are calling your method from non bean class ?
– Mykhailo Moskura
Nov 26 '18 at 2:56
|
show 8 more comments
I think you are getting the bean when the context is not initialized
So actually be sure you are invoking this piece of code :
this.connectionStateService = ApplicationContextProviderService.getBean(ConnectionStateService.class);
after context is initialized
ApplicationContext object has no refresh method. The bean does get returned when called from a spring context but it doesn't work when called from a non-spring class.
– conquester
Nov 26 '18 at 2:35
AnnotationConfigApplicationContext has it
– Mykhailo Moskura
Nov 26 '18 at 2:43
And you are having an exception. My purposes are that context is not initialized yet
– Mykhailo Moskura
Nov 26 '18 at 2:45
When I do that, I get the error that the context can be refreshed only once.
– conquester
Nov 26 '18 at 2:46
When you are calling your method from non bean class ?
– Mykhailo Moskura
Nov 26 '18 at 2:56
|
show 8 more comments
I think you are getting the bean when the context is not initialized
So actually be sure you are invoking this piece of code :
this.connectionStateService = ApplicationContextProviderService.getBean(ConnectionStateService.class);
after context is initialized
I think you are getting the bean when the context is not initialized
So actually be sure you are invoking this piece of code :
this.connectionStateService = ApplicationContextProviderService.getBean(ConnectionStateService.class);
after context is initialized
edited Nov 26 '18 at 1:21
answered Nov 26 '18 at 0:54


Mykhailo MoskuraMykhailo Moskura
867214
867214
ApplicationContext object has no refresh method. The bean does get returned when called from a spring context but it doesn't work when called from a non-spring class.
– conquester
Nov 26 '18 at 2:35
AnnotationConfigApplicationContext has it
– Mykhailo Moskura
Nov 26 '18 at 2:43
And you are having an exception. My purposes are that context is not initialized yet
– Mykhailo Moskura
Nov 26 '18 at 2:45
When I do that, I get the error that the context can be refreshed only once.
– conquester
Nov 26 '18 at 2:46
When you are calling your method from non bean class ?
– Mykhailo Moskura
Nov 26 '18 at 2:56
|
show 8 more comments
ApplicationContext object has no refresh method. The bean does get returned when called from a spring context but it doesn't work when called from a non-spring class.
– conquester
Nov 26 '18 at 2:35
AnnotationConfigApplicationContext has it
– Mykhailo Moskura
Nov 26 '18 at 2:43
And you are having an exception. My purposes are that context is not initialized yet
– Mykhailo Moskura
Nov 26 '18 at 2:45
When I do that, I get the error that the context can be refreshed only once.
– conquester
Nov 26 '18 at 2:46
When you are calling your method from non bean class ?
– Mykhailo Moskura
Nov 26 '18 at 2:56
ApplicationContext object has no refresh method. The bean does get returned when called from a spring context but it doesn't work when called from a non-spring class.
– conquester
Nov 26 '18 at 2:35
ApplicationContext object has no refresh method. The bean does get returned when called from a spring context but it doesn't work when called from a non-spring class.
– conquester
Nov 26 '18 at 2:35
AnnotationConfigApplicationContext has it
– Mykhailo Moskura
Nov 26 '18 at 2:43
AnnotationConfigApplicationContext has it
– Mykhailo Moskura
Nov 26 '18 at 2:43
And you are having an exception. My purposes are that context is not initialized yet
– Mykhailo Moskura
Nov 26 '18 at 2:45
And you are having an exception. My purposes are that context is not initialized yet
– Mykhailo Moskura
Nov 26 '18 at 2:45
When I do that, I get the error that the context can be refreshed only once.
– conquester
Nov 26 '18 at 2:46
When I do that, I get the error that the context can be refreshed only once.
– conquester
Nov 26 '18 at 2:46
When you are calling your method from non bean class ?
– Mykhailo Moskura
Nov 26 '18 at 2:56
When you are calling your method from non bean class ?
– Mykhailo Moskura
Nov 26 '18 at 2:56
|
show 8 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53473155%2fannotationconfigapplicationcontext-has-not-been-refreshed-yet-using-applicationc%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
3Lyb,Bq3Ee3 qoUw343XyXsu8KV5KnGbTyBJrfP2rlHj,exKzP3BBrntzFL8c FKhi0PkF2IO7Kts3,iTC