Sending output to a dictionary
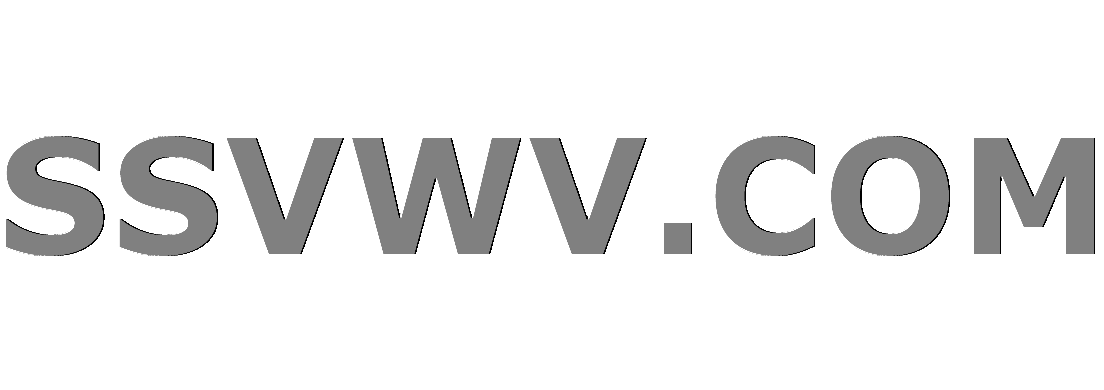
Multi tool use
I'm just a beginner at this but was looking for some help. I'm trying to make a spot it game and I have a card generator (taken from: https://math.stackexchange.com/questions/1303497/what-is-the-algorithm-to-generate-the-cards-in-the-game-dobble-known-as-spo)
Im trying to send the print output to a dictionary where the card number is the key and the generated card ids are listed in a set as a value.
This is what I have so far:
nIm = 4
n = nIm - 1
r = range(n)
rp1 = range(n+1)
c = 0
a=dict={}
# First card
c+=1
for i in rp1:
a['Card %2d:' %c]=(i+1)
print(a)
print()
# n following cards
for j in r:
if j not in r:
c = c+1
a['Card %2d:' %c]=(1)
print(a)
for k in r:
a['Card %2d:' %c]=(n+2 + n*j +k)
print(a)
print()
Does anyone have any ideas how I can do this?
Thanks in advance!
python
add a comment |
I'm just a beginner at this but was looking for some help. I'm trying to make a spot it game and I have a card generator (taken from: https://math.stackexchange.com/questions/1303497/what-is-the-algorithm-to-generate-the-cards-in-the-game-dobble-known-as-spo)
Im trying to send the print output to a dictionary where the card number is the key and the generated card ids are listed in a set as a value.
This is what I have so far:
nIm = 4
n = nIm - 1
r = range(n)
rp1 = range(n+1)
c = 0
a=dict={}
# First card
c+=1
for i in rp1:
a['Card %2d:' %c]=(i+1)
print(a)
print()
# n following cards
for j in r:
if j not in r:
c = c+1
a['Card %2d:' %c]=(1)
print(a)
for k in r:
a['Card %2d:' %c]=(n+2 + n*j +k)
print(a)
print()
Does anyone have any ideas how I can do this?
Thanks in advance!
python
add a comment |
I'm just a beginner at this but was looking for some help. I'm trying to make a spot it game and I have a card generator (taken from: https://math.stackexchange.com/questions/1303497/what-is-the-algorithm-to-generate-the-cards-in-the-game-dobble-known-as-spo)
Im trying to send the print output to a dictionary where the card number is the key and the generated card ids are listed in a set as a value.
This is what I have so far:
nIm = 4
n = nIm - 1
r = range(n)
rp1 = range(n+1)
c = 0
a=dict={}
# First card
c+=1
for i in rp1:
a['Card %2d:' %c]=(i+1)
print(a)
print()
# n following cards
for j in r:
if j not in r:
c = c+1
a['Card %2d:' %c]=(1)
print(a)
for k in r:
a['Card %2d:' %c]=(n+2 + n*j +k)
print(a)
print()
Does anyone have any ideas how I can do this?
Thanks in advance!
python
I'm just a beginner at this but was looking for some help. I'm trying to make a spot it game and I have a card generator (taken from: https://math.stackexchange.com/questions/1303497/what-is-the-algorithm-to-generate-the-cards-in-the-game-dobble-known-as-spo)
Im trying to send the print output to a dictionary where the card number is the key and the generated card ids are listed in a set as a value.
This is what I have so far:
nIm = 4
n = nIm - 1
r = range(n)
rp1 = range(n+1)
c = 0
a=dict={}
# First card
c+=1
for i in rp1:
a['Card %2d:' %c]=(i+1)
print(a)
print()
# n following cards
for j in r:
if j not in r:
c = c+1
a['Card %2d:' %c]=(1)
print(a)
for k in r:
a['Card %2d:' %c]=(n+2 + n*j +k)
print(a)
print()
Does anyone have any ideas how I can do this?
Thanks in advance!
python
python
asked Nov 24 '18 at 23:12
BeginnerBeginner
1
1
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
I looks like you are setting the key/value pairs to your dict ok with statements like:
a['Card %2d:' %c]=(i+1)
and
a['Card %2d:' %c]=(n+2 + n*j +k)
I do not see the ids you mention. Assuming your ids are in a list called ids
you could add them to your dict like so:
a['ids'] = ids
Here's what a
would look like afterwards.
print(a) # {'Card 2:': 4, 'ids': [1, 2, 3, 4, 5]}
This assumes ids = [1,2,3,4,5]
.
add a comment |
You're pretty close to it. For each card, you first need to create an empty set as a value within the dict, and then call the set's "add" method to add each id to the set.
# First card
c+=1
a['Card %2d:' %c] = set()
for i in rp1:
a['Card %2d:' %c].add(i+1)
print(a)
print()
# n following cards
for j in r:
if j not in r:
c = c+1
a['Card %2d:' %c] = {1}
print(a)
for k in r:
a['Card %2d:' %c].add(n+2 + n*j +k)
print(a)
print()
Also, at the beginning where you have "a=dict={}", you can just say either
a = {}
or
a = dict()
Looks good! Later on you might take a look at "defaultdict" in the collections module which you could use to automatically create an empty set (or other kind of value) as a default value when the key doesn't exist yet in the dictionary. But what you have works fine.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53463190%2fsending-output-to-a-dictionary%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
I looks like you are setting the key/value pairs to your dict ok with statements like:
a['Card %2d:' %c]=(i+1)
and
a['Card %2d:' %c]=(n+2 + n*j +k)
I do not see the ids you mention. Assuming your ids are in a list called ids
you could add them to your dict like so:
a['ids'] = ids
Here's what a
would look like afterwards.
print(a) # {'Card 2:': 4, 'ids': [1, 2, 3, 4, 5]}
This assumes ids = [1,2,3,4,5]
.
add a comment |
I looks like you are setting the key/value pairs to your dict ok with statements like:
a['Card %2d:' %c]=(i+1)
and
a['Card %2d:' %c]=(n+2 + n*j +k)
I do not see the ids you mention. Assuming your ids are in a list called ids
you could add them to your dict like so:
a['ids'] = ids
Here's what a
would look like afterwards.
print(a) # {'Card 2:': 4, 'ids': [1, 2, 3, 4, 5]}
This assumes ids = [1,2,3,4,5]
.
add a comment |
I looks like you are setting the key/value pairs to your dict ok with statements like:
a['Card %2d:' %c]=(i+1)
and
a['Card %2d:' %c]=(n+2 + n*j +k)
I do not see the ids you mention. Assuming your ids are in a list called ids
you could add them to your dict like so:
a['ids'] = ids
Here's what a
would look like afterwards.
print(a) # {'Card 2:': 4, 'ids': [1, 2, 3, 4, 5]}
This assumes ids = [1,2,3,4,5]
.
I looks like you are setting the key/value pairs to your dict ok with statements like:
a['Card %2d:' %c]=(i+1)
and
a['Card %2d:' %c]=(n+2 + n*j +k)
I do not see the ids you mention. Assuming your ids are in a list called ids
you could add them to your dict like so:
a['ids'] = ids
Here's what a
would look like afterwards.
print(a) # {'Card 2:': 4, 'ids': [1, 2, 3, 4, 5]}
This assumes ids = [1,2,3,4,5]
.
edited Nov 24 '18 at 23:27
answered Nov 24 '18 at 23:17


Red CricketRed Cricket
4,511103386
4,511103386
add a comment |
add a comment |
You're pretty close to it. For each card, you first need to create an empty set as a value within the dict, and then call the set's "add" method to add each id to the set.
# First card
c+=1
a['Card %2d:' %c] = set()
for i in rp1:
a['Card %2d:' %c].add(i+1)
print(a)
print()
# n following cards
for j in r:
if j not in r:
c = c+1
a['Card %2d:' %c] = {1}
print(a)
for k in r:
a['Card %2d:' %c].add(n+2 + n*j +k)
print(a)
print()
Also, at the beginning where you have "a=dict={}", you can just say either
a = {}
or
a = dict()
Looks good! Later on you might take a look at "defaultdict" in the collections module which you could use to automatically create an empty set (or other kind of value) as a default value when the key doesn't exist yet in the dictionary. But what you have works fine.
add a comment |
You're pretty close to it. For each card, you first need to create an empty set as a value within the dict, and then call the set's "add" method to add each id to the set.
# First card
c+=1
a['Card %2d:' %c] = set()
for i in rp1:
a['Card %2d:' %c].add(i+1)
print(a)
print()
# n following cards
for j in r:
if j not in r:
c = c+1
a['Card %2d:' %c] = {1}
print(a)
for k in r:
a['Card %2d:' %c].add(n+2 + n*j +k)
print(a)
print()
Also, at the beginning where you have "a=dict={}", you can just say either
a = {}
or
a = dict()
Looks good! Later on you might take a look at "defaultdict" in the collections module which you could use to automatically create an empty set (or other kind of value) as a default value when the key doesn't exist yet in the dictionary. But what you have works fine.
add a comment |
You're pretty close to it. For each card, you first need to create an empty set as a value within the dict, and then call the set's "add" method to add each id to the set.
# First card
c+=1
a['Card %2d:' %c] = set()
for i in rp1:
a['Card %2d:' %c].add(i+1)
print(a)
print()
# n following cards
for j in r:
if j not in r:
c = c+1
a['Card %2d:' %c] = {1}
print(a)
for k in r:
a['Card %2d:' %c].add(n+2 + n*j +k)
print(a)
print()
Also, at the beginning where you have "a=dict={}", you can just say either
a = {}
or
a = dict()
Looks good! Later on you might take a look at "defaultdict" in the collections module which you could use to automatically create an empty set (or other kind of value) as a default value when the key doesn't exist yet in the dictionary. But what you have works fine.
You're pretty close to it. For each card, you first need to create an empty set as a value within the dict, and then call the set's "add" method to add each id to the set.
# First card
c+=1
a['Card %2d:' %c] = set()
for i in rp1:
a['Card %2d:' %c].add(i+1)
print(a)
print()
# n following cards
for j in r:
if j not in r:
c = c+1
a['Card %2d:' %c] = {1}
print(a)
for k in r:
a['Card %2d:' %c].add(n+2 + n*j +k)
print(a)
print()
Also, at the beginning where you have "a=dict={}", you can just say either
a = {}
or
a = dict()
Looks good! Later on you might take a look at "defaultdict" in the collections module which you could use to automatically create an empty set (or other kind of value) as a default value when the key doesn't exist yet in the dictionary. But what you have works fine.
answered Nov 24 '18 at 23:54
ehaymoreehaymore
1874
1874
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53463190%2fsending-output-to-a-dictionary%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
416EMgB0JOlBX