Understanding .map and Stringify with objects
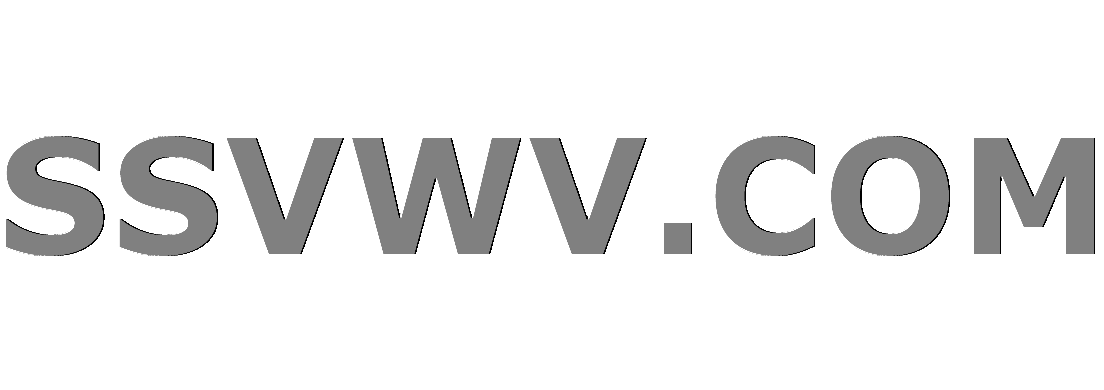
Multi tool use
I was trying different thing on JS to understand how they perform.
For my test I wanted to understand what happens if I do
JSON.stringify(something)
directly vs doing it with Map.
With JSON.stringify(something)
directly
var rockets = [
{ country:'Russia', launches:32 },
{ country:'US', launches:23 },
{ country:'China', launches:16 },
{ country:'Europe(ESA)', launches:7 },
{ country:'India', launches:4 },
{ country:'Japan', launches:3 }
];
console.log(JSON.stringify(rockets))
Where I am getting this in my console
[{"country":"Russia","launches":32},{"country":"US","launches":23},{"country":"China","launches":16},{"country":"Europe(ESA)","launches":7},{"country":"India","launches":4},{"country":"Japan","launches":3}]
Vs with Map
I got this
var rockets = [
{ country:'Russia', launches:32 },
{ country:'US', launches:23 },
{ country:'China', launches:16 },
{ country:'Europe(ESA)', launches:7 },
{ country:'India', launches:4 },
{ country:'Japan', launches:3 }
];
console.log(rockets.map(d => JSON.stringify(d)))
Where I am getting something like this
["{"country":"Russia","launches":32}", "{"country":"US","launches":23}", "{"country":"China","launches":16}", "{"country":"Europe(ESA)","launches":7}", "{"country":"India","launches":4}", "{"country":"Japan","launches":3}"]
Now, I was trying to comprehend both the code and JSON.stringify(something)
does make sense but when I look at Map
, I see a "
being added after [
which I am unable to understand from where does it come?
Also, The result from chrome console and stackoverflow comes out to be different, can someone also explain me why am I seeing before
"
in stackoverflow result
example [ "{"country":"Russia","launches":32}",
To summarise, my Prime Question is how does .map work with json stringify?
javascript
add a comment |
I was trying different thing on JS to understand how they perform.
For my test I wanted to understand what happens if I do
JSON.stringify(something)
directly vs doing it with Map.
With JSON.stringify(something)
directly
var rockets = [
{ country:'Russia', launches:32 },
{ country:'US', launches:23 },
{ country:'China', launches:16 },
{ country:'Europe(ESA)', launches:7 },
{ country:'India', launches:4 },
{ country:'Japan', launches:3 }
];
console.log(JSON.stringify(rockets))
Where I am getting this in my console
[{"country":"Russia","launches":32},{"country":"US","launches":23},{"country":"China","launches":16},{"country":"Europe(ESA)","launches":7},{"country":"India","launches":4},{"country":"Japan","launches":3}]
Vs with Map
I got this
var rockets = [
{ country:'Russia', launches:32 },
{ country:'US', launches:23 },
{ country:'China', launches:16 },
{ country:'Europe(ESA)', launches:7 },
{ country:'India', launches:4 },
{ country:'Japan', launches:3 }
];
console.log(rockets.map(d => JSON.stringify(d)))
Where I am getting something like this
["{"country":"Russia","launches":32}", "{"country":"US","launches":23}", "{"country":"China","launches":16}", "{"country":"Europe(ESA)","launches":7}", "{"country":"India","launches":4}", "{"country":"Japan","launches":3}"]
Now, I was trying to comprehend both the code and JSON.stringify(something)
does make sense but when I look at Map
, I see a "
being added after [
which I am unable to understand from where does it come?
Also, The result from chrome console and stackoverflow comes out to be different, can someone also explain me why am I seeing before
"
in stackoverflow result
example [ "{"country":"Russia","launches":32}",
To summarise, my Prime Question is how does .map work with json stringify?
javascript
Unrelated, but you don’t need the arg in the map, you can just pass the reference toJSON.stringify
.
– Dave Newton
Nov 24 '18 at 23:19
add a comment |
I was trying different thing on JS to understand how they perform.
For my test I wanted to understand what happens if I do
JSON.stringify(something)
directly vs doing it with Map.
With JSON.stringify(something)
directly
var rockets = [
{ country:'Russia', launches:32 },
{ country:'US', launches:23 },
{ country:'China', launches:16 },
{ country:'Europe(ESA)', launches:7 },
{ country:'India', launches:4 },
{ country:'Japan', launches:3 }
];
console.log(JSON.stringify(rockets))
Where I am getting this in my console
[{"country":"Russia","launches":32},{"country":"US","launches":23},{"country":"China","launches":16},{"country":"Europe(ESA)","launches":7},{"country":"India","launches":4},{"country":"Japan","launches":3}]
Vs with Map
I got this
var rockets = [
{ country:'Russia', launches:32 },
{ country:'US', launches:23 },
{ country:'China', launches:16 },
{ country:'Europe(ESA)', launches:7 },
{ country:'India', launches:4 },
{ country:'Japan', launches:3 }
];
console.log(rockets.map(d => JSON.stringify(d)))
Where I am getting something like this
["{"country":"Russia","launches":32}", "{"country":"US","launches":23}", "{"country":"China","launches":16}", "{"country":"Europe(ESA)","launches":7}", "{"country":"India","launches":4}", "{"country":"Japan","launches":3}"]
Now, I was trying to comprehend both the code and JSON.stringify(something)
does make sense but when I look at Map
, I see a "
being added after [
which I am unable to understand from where does it come?
Also, The result from chrome console and stackoverflow comes out to be different, can someone also explain me why am I seeing before
"
in stackoverflow result
example [ "{"country":"Russia","launches":32}",
To summarise, my Prime Question is how does .map work with json stringify?
javascript
I was trying different thing on JS to understand how they perform.
For my test I wanted to understand what happens if I do
JSON.stringify(something)
directly vs doing it with Map.
With JSON.stringify(something)
directly
var rockets = [
{ country:'Russia', launches:32 },
{ country:'US', launches:23 },
{ country:'China', launches:16 },
{ country:'Europe(ESA)', launches:7 },
{ country:'India', launches:4 },
{ country:'Japan', launches:3 }
];
console.log(JSON.stringify(rockets))
Where I am getting this in my console
[{"country":"Russia","launches":32},{"country":"US","launches":23},{"country":"China","launches":16},{"country":"Europe(ESA)","launches":7},{"country":"India","launches":4},{"country":"Japan","launches":3}]
Vs with Map
I got this
var rockets = [
{ country:'Russia', launches:32 },
{ country:'US', launches:23 },
{ country:'China', launches:16 },
{ country:'Europe(ESA)', launches:7 },
{ country:'India', launches:4 },
{ country:'Japan', launches:3 }
];
console.log(rockets.map(d => JSON.stringify(d)))
Where I am getting something like this
["{"country":"Russia","launches":32}", "{"country":"US","launches":23}", "{"country":"China","launches":16}", "{"country":"Europe(ESA)","launches":7}", "{"country":"India","launches":4}", "{"country":"Japan","launches":3}"]
Now, I was trying to comprehend both the code and JSON.stringify(something)
does make sense but when I look at Map
, I see a "
being added after [
which I am unable to understand from where does it come?
Also, The result from chrome console and stackoverflow comes out to be different, can someone also explain me why am I seeing before
"
in stackoverflow result
example [ "{"country":"Russia","launches":32}",
To summarise, my Prime Question is how does .map work with json stringify?
var rockets = [
{ country:'Russia', launches:32 },
{ country:'US', launches:23 },
{ country:'China', launches:16 },
{ country:'Europe(ESA)', launches:7 },
{ country:'India', launches:4 },
{ country:'Japan', launches:3 }
];
console.log(JSON.stringify(rockets))
var rockets = [
{ country:'Russia', launches:32 },
{ country:'US', launches:23 },
{ country:'China', launches:16 },
{ country:'Europe(ESA)', launches:7 },
{ country:'India', launches:4 },
{ country:'Japan', launches:3 }
];
console.log(JSON.stringify(rockets))
var rockets = [
{ country:'Russia', launches:32 },
{ country:'US', launches:23 },
{ country:'China', launches:16 },
{ country:'Europe(ESA)', launches:7 },
{ country:'India', launches:4 },
{ country:'Japan', launches:3 }
];
console.log(rockets.map(d => JSON.stringify(d)))
var rockets = [
{ country:'Russia', launches:32 },
{ country:'US', launches:23 },
{ country:'China', launches:16 },
{ country:'Europe(ESA)', launches:7 },
{ country:'India', launches:4 },
{ country:'Japan', launches:3 }
];
console.log(rockets.map(d => JSON.stringify(d)))
javascript
javascript
asked Nov 24 '18 at 23:08
anny123anny123
642116
642116
Unrelated, but you don’t need the arg in the map, you can just pass the reference toJSON.stringify
.
– Dave Newton
Nov 24 '18 at 23:19
add a comment |
Unrelated, but you don’t need the arg in the map, you can just pass the reference toJSON.stringify
.
– Dave Newton
Nov 24 '18 at 23:19
Unrelated, but you don’t need the arg in the map, you can just pass the reference to
JSON.stringify
.– Dave Newton
Nov 24 '18 at 23:19
Unrelated, but you don’t need the arg in the map, you can just pass the reference to
JSON.stringify
.– Dave Newton
Nov 24 '18 at 23:19
add a comment |
2 Answers
2
active
oldest
votes
The first JSON.stringify(rockets)
results in a single long string, which contains serialized data that can be turned back into an array of objects with JSON.parse
.
The second rockets.map(d => JSON.stringify(d)
results in an array, each of whose items is a string that can be deserialized back into a small object.
The backslashes before the "
s in the lower code are a bit confusing, they're because the stack console delimits strings with "
. It would be clearer if it used '
- with '
s, the bottom result would look like
[
'{"country": "Russia","launches": 32}',
'{"country": "US","launches": 23}',
'{"country": "China","launches": 16}',
// ...
]
This is the exact same array that results from your second snippet
var rockets = [
{ country:'Russia', launches:32 },
{ country:'US', launches:23 },
{ country:'China', launches:16 },
{ country:'Europe(ESA)', launches:7 },
{ country:'India', launches:4 },
{ country:'Japan', launches:3 }
];
console.log(rockets.map(d => JSON.stringify(d)))
except that it's displayed in a format that's easier to read, because the array items (strings) are delimited with '
s, so the literal "
s in the strings don't need to be escaped with a backslash first.
(just like a line
const str = 'foo'
is equivalent to using
const str = "foo"
, and how
const str2 = 'fo"o'
is equivalent to
const str2 = "fo"o"
: that is, to denote the same character that you're using as the string's delimiter, you must put a backslash in front of it first)
Note that JSON strings require keys and string values to be surrounded with double quotes "
, but object literals (such as displayed above) do not.
The first JSON.stringify(rockets) results in a single long string then shouldn't the result be like"[ { country:'Russia', launches:32 }, { country:'US', launches:23 }, { country:'China', launches:16 }, { country:'Europe(ESA)', launches:7 }, { country:'India', launches:4 }, { country:'Japan', launches:3 } ]"
and theconsole.log(JSON.stringify(typeof rockets))
should say string (currently it says object)?
– anny123
Nov 24 '18 at 23:29
1
Whenconsole.log
ging a string alone, the strings delimiters are not seen in the output - tryconsole.log('foo')
and you'll just seefoo
, not'foo'
or"foo"
. Stringifying an object does not change the original object - it only creates a new string that represents the object. Also,typeof rockets
resolves toobject
, soconsole.log(JSON.stringify(typeof rockets))
is equivalent toconsole.log(JSON.stringify('object'))
. Instead, tryconsole.log(typeof JSON.stringify(rockets))
and you'll getstring
.
– CertainPerformance
Nov 24 '18 at 23:34
Git it. Thanks for answering
– anny123
Nov 24 '18 at 23:35
add a comment |
So what you are trying to differentiate between JSON.stringify(something)
and .map(JSON.stringify)
is that the first one makes a string, and the second one makes a list of strings.
The "
afer [
in the result of using .map
is because your console is trying to say that what you have in your console.log
is a list of strings. Whereas in the result of JSON.stringify(something)
, the entire output is a string so the entire output is prepended and appended by a "
.
The difference between StackOverflow and Chrome's output, the , is just is just an escape character.
if the entire output is string forJSON.stringify(something)
then shouldn't the result be like"[ { country:'Russia', launches:32 }, { country:'US', launches:23 }, { country:'China', launches:16 }, { country:'Europe(ESA)', launches:7 }, { country:'India', launches:4 }, { country:'Japan', launches:3 } ]"
and theconsole.log(JSON.stringify(typeof rockets))
should say string (currently it says object)?
– anny123
Nov 24 '18 at 23:30
Also, Arno thanks for answering and can you also please extend/explain you answer in stretch? still unable to understand it.
– anny123
Nov 24 '18 at 23:33
console.log
omits the starting and ending"
for string outputs! Try runningconsole.log('foo')
thenconsole.log(['foo'])
– Arno Gau
Nov 24 '18 at 23:33
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53463159%2funderstanding-map-and-stringify-with-objects%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
The first JSON.stringify(rockets)
results in a single long string, which contains serialized data that can be turned back into an array of objects with JSON.parse
.
The second rockets.map(d => JSON.stringify(d)
results in an array, each of whose items is a string that can be deserialized back into a small object.
The backslashes before the "
s in the lower code are a bit confusing, they're because the stack console delimits strings with "
. It would be clearer if it used '
- with '
s, the bottom result would look like
[
'{"country": "Russia","launches": 32}',
'{"country": "US","launches": 23}',
'{"country": "China","launches": 16}',
// ...
]
This is the exact same array that results from your second snippet
var rockets = [
{ country:'Russia', launches:32 },
{ country:'US', launches:23 },
{ country:'China', launches:16 },
{ country:'Europe(ESA)', launches:7 },
{ country:'India', launches:4 },
{ country:'Japan', launches:3 }
];
console.log(rockets.map(d => JSON.stringify(d)))
except that it's displayed in a format that's easier to read, because the array items (strings) are delimited with '
s, so the literal "
s in the strings don't need to be escaped with a backslash first.
(just like a line
const str = 'foo'
is equivalent to using
const str = "foo"
, and how
const str2 = 'fo"o'
is equivalent to
const str2 = "fo"o"
: that is, to denote the same character that you're using as the string's delimiter, you must put a backslash in front of it first)
Note that JSON strings require keys and string values to be surrounded with double quotes "
, but object literals (such as displayed above) do not.
The first JSON.stringify(rockets) results in a single long string then shouldn't the result be like"[ { country:'Russia', launches:32 }, { country:'US', launches:23 }, { country:'China', launches:16 }, { country:'Europe(ESA)', launches:7 }, { country:'India', launches:4 }, { country:'Japan', launches:3 } ]"
and theconsole.log(JSON.stringify(typeof rockets))
should say string (currently it says object)?
– anny123
Nov 24 '18 at 23:29
1
Whenconsole.log
ging a string alone, the strings delimiters are not seen in the output - tryconsole.log('foo')
and you'll just seefoo
, not'foo'
or"foo"
. Stringifying an object does not change the original object - it only creates a new string that represents the object. Also,typeof rockets
resolves toobject
, soconsole.log(JSON.stringify(typeof rockets))
is equivalent toconsole.log(JSON.stringify('object'))
. Instead, tryconsole.log(typeof JSON.stringify(rockets))
and you'll getstring
.
– CertainPerformance
Nov 24 '18 at 23:34
Git it. Thanks for answering
– anny123
Nov 24 '18 at 23:35
add a comment |
The first JSON.stringify(rockets)
results in a single long string, which contains serialized data that can be turned back into an array of objects with JSON.parse
.
The second rockets.map(d => JSON.stringify(d)
results in an array, each of whose items is a string that can be deserialized back into a small object.
The backslashes before the "
s in the lower code are a bit confusing, they're because the stack console delimits strings with "
. It would be clearer if it used '
- with '
s, the bottom result would look like
[
'{"country": "Russia","launches": 32}',
'{"country": "US","launches": 23}',
'{"country": "China","launches": 16}',
// ...
]
This is the exact same array that results from your second snippet
var rockets = [
{ country:'Russia', launches:32 },
{ country:'US', launches:23 },
{ country:'China', launches:16 },
{ country:'Europe(ESA)', launches:7 },
{ country:'India', launches:4 },
{ country:'Japan', launches:3 }
];
console.log(rockets.map(d => JSON.stringify(d)))
except that it's displayed in a format that's easier to read, because the array items (strings) are delimited with '
s, so the literal "
s in the strings don't need to be escaped with a backslash first.
(just like a line
const str = 'foo'
is equivalent to using
const str = "foo"
, and how
const str2 = 'fo"o'
is equivalent to
const str2 = "fo"o"
: that is, to denote the same character that you're using as the string's delimiter, you must put a backslash in front of it first)
Note that JSON strings require keys and string values to be surrounded with double quotes "
, but object literals (such as displayed above) do not.
The first JSON.stringify(rockets) results in a single long string then shouldn't the result be like"[ { country:'Russia', launches:32 }, { country:'US', launches:23 }, { country:'China', launches:16 }, { country:'Europe(ESA)', launches:7 }, { country:'India', launches:4 }, { country:'Japan', launches:3 } ]"
and theconsole.log(JSON.stringify(typeof rockets))
should say string (currently it says object)?
– anny123
Nov 24 '18 at 23:29
1
Whenconsole.log
ging a string alone, the strings delimiters are not seen in the output - tryconsole.log('foo')
and you'll just seefoo
, not'foo'
or"foo"
. Stringifying an object does not change the original object - it only creates a new string that represents the object. Also,typeof rockets
resolves toobject
, soconsole.log(JSON.stringify(typeof rockets))
is equivalent toconsole.log(JSON.stringify('object'))
. Instead, tryconsole.log(typeof JSON.stringify(rockets))
and you'll getstring
.
– CertainPerformance
Nov 24 '18 at 23:34
Git it. Thanks for answering
– anny123
Nov 24 '18 at 23:35
add a comment |
The first JSON.stringify(rockets)
results in a single long string, which contains serialized data that can be turned back into an array of objects with JSON.parse
.
The second rockets.map(d => JSON.stringify(d)
results in an array, each of whose items is a string that can be deserialized back into a small object.
The backslashes before the "
s in the lower code are a bit confusing, they're because the stack console delimits strings with "
. It would be clearer if it used '
- with '
s, the bottom result would look like
[
'{"country": "Russia","launches": 32}',
'{"country": "US","launches": 23}',
'{"country": "China","launches": 16}',
// ...
]
This is the exact same array that results from your second snippet
var rockets = [
{ country:'Russia', launches:32 },
{ country:'US', launches:23 },
{ country:'China', launches:16 },
{ country:'Europe(ESA)', launches:7 },
{ country:'India', launches:4 },
{ country:'Japan', launches:3 }
];
console.log(rockets.map(d => JSON.stringify(d)))
except that it's displayed in a format that's easier to read, because the array items (strings) are delimited with '
s, so the literal "
s in the strings don't need to be escaped with a backslash first.
(just like a line
const str = 'foo'
is equivalent to using
const str = "foo"
, and how
const str2 = 'fo"o'
is equivalent to
const str2 = "fo"o"
: that is, to denote the same character that you're using as the string's delimiter, you must put a backslash in front of it first)
Note that JSON strings require keys and string values to be surrounded with double quotes "
, but object literals (such as displayed above) do not.
The first JSON.stringify(rockets)
results in a single long string, which contains serialized data that can be turned back into an array of objects with JSON.parse
.
The second rockets.map(d => JSON.stringify(d)
results in an array, each of whose items is a string that can be deserialized back into a small object.
The backslashes before the "
s in the lower code are a bit confusing, they're because the stack console delimits strings with "
. It would be clearer if it used '
- with '
s, the bottom result would look like
[
'{"country": "Russia","launches": 32}',
'{"country": "US","launches": 23}',
'{"country": "China","launches": 16}',
// ...
]
This is the exact same array that results from your second snippet
var rockets = [
{ country:'Russia', launches:32 },
{ country:'US', launches:23 },
{ country:'China', launches:16 },
{ country:'Europe(ESA)', launches:7 },
{ country:'India', launches:4 },
{ country:'Japan', launches:3 }
];
console.log(rockets.map(d => JSON.stringify(d)))
except that it's displayed in a format that's easier to read, because the array items (strings) are delimited with '
s, so the literal "
s in the strings don't need to be escaped with a backslash first.
(just like a line
const str = 'foo'
is equivalent to using
const str = "foo"
, and how
const str2 = 'fo"o'
is equivalent to
const str2 = "fo"o"
: that is, to denote the same character that you're using as the string's delimiter, you must put a backslash in front of it first)
Note that JSON strings require keys and string values to be surrounded with double quotes "
, but object literals (such as displayed above) do not.
var rockets = [
{ country:'Russia', launches:32 },
{ country:'US', launches:23 },
{ country:'China', launches:16 },
{ country:'Europe(ESA)', launches:7 },
{ country:'India', launches:4 },
{ country:'Japan', launches:3 }
];
console.log(rockets.map(d => JSON.stringify(d)))
var rockets = [
{ country:'Russia', launches:32 },
{ country:'US', launches:23 },
{ country:'China', launches:16 },
{ country:'Europe(ESA)', launches:7 },
{ country:'India', launches:4 },
{ country:'Japan', launches:3 }
];
console.log(rockets.map(d => JSON.stringify(d)))
edited Nov 24 '18 at 23:20
answered Nov 24 '18 at 23:14
CertainPerformanceCertainPerformance
91.2k165179
91.2k165179
The first JSON.stringify(rockets) results in a single long string then shouldn't the result be like"[ { country:'Russia', launches:32 }, { country:'US', launches:23 }, { country:'China', launches:16 }, { country:'Europe(ESA)', launches:7 }, { country:'India', launches:4 }, { country:'Japan', launches:3 } ]"
and theconsole.log(JSON.stringify(typeof rockets))
should say string (currently it says object)?
– anny123
Nov 24 '18 at 23:29
1
Whenconsole.log
ging a string alone, the strings delimiters are not seen in the output - tryconsole.log('foo')
and you'll just seefoo
, not'foo'
or"foo"
. Stringifying an object does not change the original object - it only creates a new string that represents the object. Also,typeof rockets
resolves toobject
, soconsole.log(JSON.stringify(typeof rockets))
is equivalent toconsole.log(JSON.stringify('object'))
. Instead, tryconsole.log(typeof JSON.stringify(rockets))
and you'll getstring
.
– CertainPerformance
Nov 24 '18 at 23:34
Git it. Thanks for answering
– anny123
Nov 24 '18 at 23:35
add a comment |
The first JSON.stringify(rockets) results in a single long string then shouldn't the result be like"[ { country:'Russia', launches:32 }, { country:'US', launches:23 }, { country:'China', launches:16 }, { country:'Europe(ESA)', launches:7 }, { country:'India', launches:4 }, { country:'Japan', launches:3 } ]"
and theconsole.log(JSON.stringify(typeof rockets))
should say string (currently it says object)?
– anny123
Nov 24 '18 at 23:29
1
Whenconsole.log
ging a string alone, the strings delimiters are not seen in the output - tryconsole.log('foo')
and you'll just seefoo
, not'foo'
or"foo"
. Stringifying an object does not change the original object - it only creates a new string that represents the object. Also,typeof rockets
resolves toobject
, soconsole.log(JSON.stringify(typeof rockets))
is equivalent toconsole.log(JSON.stringify('object'))
. Instead, tryconsole.log(typeof JSON.stringify(rockets))
and you'll getstring
.
– CertainPerformance
Nov 24 '18 at 23:34
Git it. Thanks for answering
– anny123
Nov 24 '18 at 23:35
The first JSON.stringify(rockets) results in a single long string then shouldn't the result be like
"[ { country:'Russia', launches:32 }, { country:'US', launches:23 }, { country:'China', launches:16 }, { country:'Europe(ESA)', launches:7 }, { country:'India', launches:4 }, { country:'Japan', launches:3 } ]"
and the console.log(JSON.stringify(typeof rockets))
should say string (currently it says object)?– anny123
Nov 24 '18 at 23:29
The first JSON.stringify(rockets) results in a single long string then shouldn't the result be like
"[ { country:'Russia', launches:32 }, { country:'US', launches:23 }, { country:'China', launches:16 }, { country:'Europe(ESA)', launches:7 }, { country:'India', launches:4 }, { country:'Japan', launches:3 } ]"
and the console.log(JSON.stringify(typeof rockets))
should say string (currently it says object)?– anny123
Nov 24 '18 at 23:29
1
1
When
console.log
ging a string alone, the strings delimiters are not seen in the output - try console.log('foo')
and you'll just see foo
, not 'foo'
or "foo"
. Stringifying an object does not change the original object - it only creates a new string that represents the object. Also, typeof rockets
resolves to object
, so console.log(JSON.stringify(typeof rockets))
is equivalent to console.log(JSON.stringify('object'))
. Instead, try console.log(typeof JSON.stringify(rockets))
and you'll get string
.– CertainPerformance
Nov 24 '18 at 23:34
When
console.log
ging a string alone, the strings delimiters are not seen in the output - try console.log('foo')
and you'll just see foo
, not 'foo'
or "foo"
. Stringifying an object does not change the original object - it only creates a new string that represents the object. Also, typeof rockets
resolves to object
, so console.log(JSON.stringify(typeof rockets))
is equivalent to console.log(JSON.stringify('object'))
. Instead, try console.log(typeof JSON.stringify(rockets))
and you'll get string
.– CertainPerformance
Nov 24 '18 at 23:34
Git it. Thanks for answering
– anny123
Nov 24 '18 at 23:35
Git it. Thanks for answering
– anny123
Nov 24 '18 at 23:35
add a comment |
So what you are trying to differentiate between JSON.stringify(something)
and .map(JSON.stringify)
is that the first one makes a string, and the second one makes a list of strings.
The "
afer [
in the result of using .map
is because your console is trying to say that what you have in your console.log
is a list of strings. Whereas in the result of JSON.stringify(something)
, the entire output is a string so the entire output is prepended and appended by a "
.
The difference between StackOverflow and Chrome's output, the , is just is just an escape character.
if the entire output is string forJSON.stringify(something)
then shouldn't the result be like"[ { country:'Russia', launches:32 }, { country:'US', launches:23 }, { country:'China', launches:16 }, { country:'Europe(ESA)', launches:7 }, { country:'India', launches:4 }, { country:'Japan', launches:3 } ]"
and theconsole.log(JSON.stringify(typeof rockets))
should say string (currently it says object)?
– anny123
Nov 24 '18 at 23:30
Also, Arno thanks for answering and can you also please extend/explain you answer in stretch? still unable to understand it.
– anny123
Nov 24 '18 at 23:33
console.log
omits the starting and ending"
for string outputs! Try runningconsole.log('foo')
thenconsole.log(['foo'])
– Arno Gau
Nov 24 '18 at 23:33
add a comment |
So what you are trying to differentiate between JSON.stringify(something)
and .map(JSON.stringify)
is that the first one makes a string, and the second one makes a list of strings.
The "
afer [
in the result of using .map
is because your console is trying to say that what you have in your console.log
is a list of strings. Whereas in the result of JSON.stringify(something)
, the entire output is a string so the entire output is prepended and appended by a "
.
The difference between StackOverflow and Chrome's output, the , is just is just an escape character.
if the entire output is string forJSON.stringify(something)
then shouldn't the result be like"[ { country:'Russia', launches:32 }, { country:'US', launches:23 }, { country:'China', launches:16 }, { country:'Europe(ESA)', launches:7 }, { country:'India', launches:4 }, { country:'Japan', launches:3 } ]"
and theconsole.log(JSON.stringify(typeof rockets))
should say string (currently it says object)?
– anny123
Nov 24 '18 at 23:30
Also, Arno thanks for answering and can you also please extend/explain you answer in stretch? still unable to understand it.
– anny123
Nov 24 '18 at 23:33
console.log
omits the starting and ending"
for string outputs! Try runningconsole.log('foo')
thenconsole.log(['foo'])
– Arno Gau
Nov 24 '18 at 23:33
add a comment |
So what you are trying to differentiate between JSON.stringify(something)
and .map(JSON.stringify)
is that the first one makes a string, and the second one makes a list of strings.
The "
afer [
in the result of using .map
is because your console is trying to say that what you have in your console.log
is a list of strings. Whereas in the result of JSON.stringify(something)
, the entire output is a string so the entire output is prepended and appended by a "
.
The difference between StackOverflow and Chrome's output, the , is just is just an escape character.
So what you are trying to differentiate between JSON.stringify(something)
and .map(JSON.stringify)
is that the first one makes a string, and the second one makes a list of strings.
The "
afer [
in the result of using .map
is because your console is trying to say that what you have in your console.log
is a list of strings. Whereas in the result of JSON.stringify(something)
, the entire output is a string so the entire output is prepended and appended by a "
.
The difference between StackOverflow and Chrome's output, the , is just is just an escape character.
answered Nov 24 '18 at 23:19
Arno GauArno Gau
243
243
if the entire output is string forJSON.stringify(something)
then shouldn't the result be like"[ { country:'Russia', launches:32 }, { country:'US', launches:23 }, { country:'China', launches:16 }, { country:'Europe(ESA)', launches:7 }, { country:'India', launches:4 }, { country:'Japan', launches:3 } ]"
and theconsole.log(JSON.stringify(typeof rockets))
should say string (currently it says object)?
– anny123
Nov 24 '18 at 23:30
Also, Arno thanks for answering and can you also please extend/explain you answer in stretch? still unable to understand it.
– anny123
Nov 24 '18 at 23:33
console.log
omits the starting and ending"
for string outputs! Try runningconsole.log('foo')
thenconsole.log(['foo'])
– Arno Gau
Nov 24 '18 at 23:33
add a comment |
if the entire output is string forJSON.stringify(something)
then shouldn't the result be like"[ { country:'Russia', launches:32 }, { country:'US', launches:23 }, { country:'China', launches:16 }, { country:'Europe(ESA)', launches:7 }, { country:'India', launches:4 }, { country:'Japan', launches:3 } ]"
and theconsole.log(JSON.stringify(typeof rockets))
should say string (currently it says object)?
– anny123
Nov 24 '18 at 23:30
Also, Arno thanks for answering and can you also please extend/explain you answer in stretch? still unable to understand it.
– anny123
Nov 24 '18 at 23:33
console.log
omits the starting and ending"
for string outputs! Try runningconsole.log('foo')
thenconsole.log(['foo'])
– Arno Gau
Nov 24 '18 at 23:33
if the entire output is string for
JSON.stringify(something)
then shouldn't the result be like "[ { country:'Russia', launches:32 }, { country:'US', launches:23 }, { country:'China', launches:16 }, { country:'Europe(ESA)', launches:7 }, { country:'India', launches:4 }, { country:'Japan', launches:3 } ]"
and the console.log(JSON.stringify(typeof rockets))
should say string (currently it says object)?– anny123
Nov 24 '18 at 23:30
if the entire output is string for
JSON.stringify(something)
then shouldn't the result be like "[ { country:'Russia', launches:32 }, { country:'US', launches:23 }, { country:'China', launches:16 }, { country:'Europe(ESA)', launches:7 }, { country:'India', launches:4 }, { country:'Japan', launches:3 } ]"
and the console.log(JSON.stringify(typeof rockets))
should say string (currently it says object)?– anny123
Nov 24 '18 at 23:30
Also, Arno thanks for answering and can you also please extend/explain you answer in stretch? still unable to understand it.
– anny123
Nov 24 '18 at 23:33
Also, Arno thanks for answering and can you also please extend/explain you answer in stretch? still unable to understand it.
– anny123
Nov 24 '18 at 23:33
console.log
omits the starting and ending "
for string outputs! Try running console.log('foo')
then console.log(['foo'])
– Arno Gau
Nov 24 '18 at 23:33
console.log
omits the starting and ending "
for string outputs! Try running console.log('foo')
then console.log(['foo'])
– Arno Gau
Nov 24 '18 at 23:33
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53463159%2funderstanding-map-and-stringify-with-objects%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
L6 Rp r Z,K0Zy,5h269k,Xy2kvN6LlJDiv0FnERhZZsfNVPpspry73X2A uF,Hc5yOGDZxdJwxEpaNidclEPOw IlkXYoEuE89g aW13 8nz
Unrelated, but you don’t need the arg in the map, you can just pass the reference to
JSON.stringify
.– Dave Newton
Nov 24 '18 at 23:19