@ModelAttribute return incorrect value
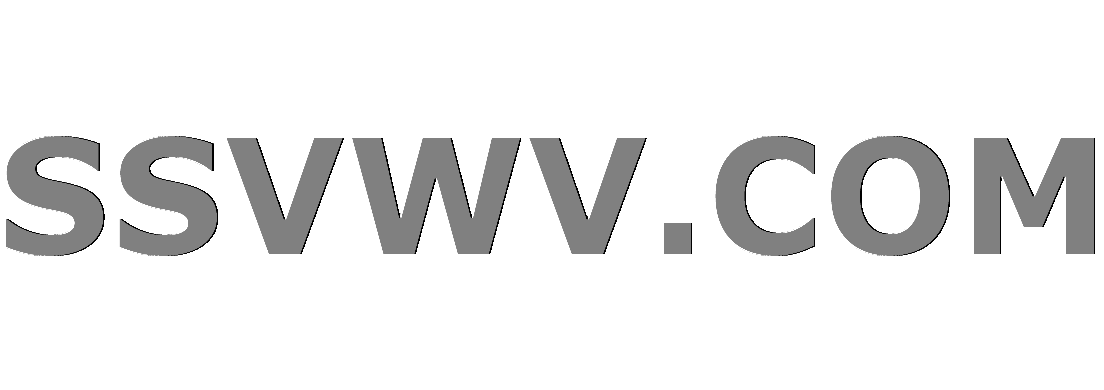
Multi tool use
I send the value using the form:
<form action="user-fonts" method="post" ">
<select name="nameFont">
<#list fonts as font>
<option value=${font.id}>${font.nameFont}</option>
</#list>
</select>
<input type="hidden" name="_csrf" value="${_csrf.token}" />
<div><input type="submit" value="Go"/></div>
</form>
Controller:
@GetMapping
public String main(@AuthenticationPrincipal User user, Model model)
{
Set<DBFont> fonts = user.getFont();
model.addAttribute("fonts", fonts);
return "Myfonts";
}
Here are the values in GetMapping:
@PostMapping
public String mainPost(@ModelAttribute DBFont DBfont)
{
return "redirect:/user-fonts";
}
Why does namefont get id value? And Id = null?
is it possible to send all the class values?nameFont and ID?
Why am I getting null everywhere except for id?
java spring-boot
add a comment |
I send the value using the form:
<form action="user-fonts" method="post" ">
<select name="nameFont">
<#list fonts as font>
<option value=${font.id}>${font.nameFont}</option>
</#list>
</select>
<input type="hidden" name="_csrf" value="${_csrf.token}" />
<div><input type="submit" value="Go"/></div>
</form>
Controller:
@GetMapping
public String main(@AuthenticationPrincipal User user, Model model)
{
Set<DBFont> fonts = user.getFont();
model.addAttribute("fonts", fonts);
return "Myfonts";
}
Here are the values in GetMapping:
@PostMapping
public String mainPost(@ModelAttribute DBFont DBfont)
{
return "redirect:/user-fonts";
}
Why does namefont get id value? And Id = null?
is it possible to send all the class values?nameFont and ID?
Why am I getting null everywhere except for id?
java spring-boot
I'd say that's because of<select name="nameFont">
. With<option>
you basically just send the value of the option back to the server which is the id in your case. Thus it should be<select name="id">
.
– Thomas
Nov 20 at 14:32
Is it possible to send all the class values?nameFont and ID?
– crazy_deviL
Nov 20 at 14:35
That should be possible but you'd probably have to change a thing or two (which also might depend on the web framework you're using): with traditional form data you'd probably have to add hidden fields for the additional values of the selected element (e.g. via JavaScript) or make the value of each option a complete representation of the selected element and parse that on the server. Alteratively you could ditch the form and post JSON data which would require even more JavaScript to build that on the client though.
– Thomas
Nov 21 at 9:00
... However, imo the best option would be to just keep sending the id and look up the object on the server. That's less work to do, less error prone (what if the selection of a font would also change its name?) and more secure (again what if the name of the font or any other object you use that approach with would be changed in some malicious way?).
– Thomas
Nov 21 at 9:02
add a comment |
I send the value using the form:
<form action="user-fonts" method="post" ">
<select name="nameFont">
<#list fonts as font>
<option value=${font.id}>${font.nameFont}</option>
</#list>
</select>
<input type="hidden" name="_csrf" value="${_csrf.token}" />
<div><input type="submit" value="Go"/></div>
</form>
Controller:
@GetMapping
public String main(@AuthenticationPrincipal User user, Model model)
{
Set<DBFont> fonts = user.getFont();
model.addAttribute("fonts", fonts);
return "Myfonts";
}
Here are the values in GetMapping:
@PostMapping
public String mainPost(@ModelAttribute DBFont DBfont)
{
return "redirect:/user-fonts";
}
Why does namefont get id value? And Id = null?
is it possible to send all the class values?nameFont and ID?
Why am I getting null everywhere except for id?
java spring-boot
I send the value using the form:
<form action="user-fonts" method="post" ">
<select name="nameFont">
<#list fonts as font>
<option value=${font.id}>${font.nameFont}</option>
</#list>
</select>
<input type="hidden" name="_csrf" value="${_csrf.token}" />
<div><input type="submit" value="Go"/></div>
</form>
Controller:
@GetMapping
public String main(@AuthenticationPrincipal User user, Model model)
{
Set<DBFont> fonts = user.getFont();
model.addAttribute("fonts", fonts);
return "Myfonts";
}
Here are the values in GetMapping:
@PostMapping
public String mainPost(@ModelAttribute DBFont DBfont)
{
return "redirect:/user-fonts";
}
Why does namefont get id value? And Id = null?
is it possible to send all the class values?nameFont and ID?
Why am I getting null everywhere except for id?
java spring-boot
java spring-boot
edited Nov 20 at 14:43
asked Nov 20 at 14:21


crazy_deviL
449
449
I'd say that's because of<select name="nameFont">
. With<option>
you basically just send the value of the option back to the server which is the id in your case. Thus it should be<select name="id">
.
– Thomas
Nov 20 at 14:32
Is it possible to send all the class values?nameFont and ID?
– crazy_deviL
Nov 20 at 14:35
That should be possible but you'd probably have to change a thing or two (which also might depend on the web framework you're using): with traditional form data you'd probably have to add hidden fields for the additional values of the selected element (e.g. via JavaScript) or make the value of each option a complete representation of the selected element and parse that on the server. Alteratively you could ditch the form and post JSON data which would require even more JavaScript to build that on the client though.
– Thomas
Nov 21 at 9:00
... However, imo the best option would be to just keep sending the id and look up the object on the server. That's less work to do, less error prone (what if the selection of a font would also change its name?) and more secure (again what if the name of the font or any other object you use that approach with would be changed in some malicious way?).
– Thomas
Nov 21 at 9:02
add a comment |
I'd say that's because of<select name="nameFont">
. With<option>
you basically just send the value of the option back to the server which is the id in your case. Thus it should be<select name="id">
.
– Thomas
Nov 20 at 14:32
Is it possible to send all the class values?nameFont and ID?
– crazy_deviL
Nov 20 at 14:35
That should be possible but you'd probably have to change a thing or two (which also might depend on the web framework you're using): with traditional form data you'd probably have to add hidden fields for the additional values of the selected element (e.g. via JavaScript) or make the value of each option a complete representation of the selected element and parse that on the server. Alteratively you could ditch the form and post JSON data which would require even more JavaScript to build that on the client though.
– Thomas
Nov 21 at 9:00
... However, imo the best option would be to just keep sending the id and look up the object on the server. That's less work to do, less error prone (what if the selection of a font would also change its name?) and more secure (again what if the name of the font or any other object you use that approach with would be changed in some malicious way?).
– Thomas
Nov 21 at 9:02
I'd say that's because of
<select name="nameFont">
. With <option>
you basically just send the value of the option back to the server which is the id in your case. Thus it should be <select name="id">
.– Thomas
Nov 20 at 14:32
I'd say that's because of
<select name="nameFont">
. With <option>
you basically just send the value of the option back to the server which is the id in your case. Thus it should be <select name="id">
.– Thomas
Nov 20 at 14:32
Is it possible to send all the class values?nameFont and ID?
– crazy_deviL
Nov 20 at 14:35
Is it possible to send all the class values?nameFont and ID?
– crazy_deviL
Nov 20 at 14:35
That should be possible but you'd probably have to change a thing or two (which also might depend on the web framework you're using): with traditional form data you'd probably have to add hidden fields for the additional values of the selected element (e.g. via JavaScript) or make the value of each option a complete representation of the selected element and parse that on the server. Alteratively you could ditch the form and post JSON data which would require even more JavaScript to build that on the client though.
– Thomas
Nov 21 at 9:00
That should be possible but you'd probably have to change a thing or two (which also might depend on the web framework you're using): with traditional form data you'd probably have to add hidden fields for the additional values of the selected element (e.g. via JavaScript) or make the value of each option a complete representation of the selected element and parse that on the server. Alteratively you could ditch the form and post JSON data which would require even more JavaScript to build that on the client though.
– Thomas
Nov 21 at 9:00
... However, imo the best option would be to just keep sending the id and look up the object on the server. That's less work to do, less error prone (what if the selection of a font would also change its name?) and more secure (again what if the name of the font or any other object you use that approach with would be changed in some malicious way?).
– Thomas
Nov 21 at 9:02
... However, imo the best option would be to just keep sending the id and look up the object on the server. That's less work to do, less error prone (what if the selection of a font would also change its name?) and more secure (again what if the name of the font or any other object you use that approach with would be changed in some malicious way?).
– Thomas
Nov 21 at 9:02
add a comment |
1 Answer
1
active
oldest
votes
Ensure that you are not blocking any attribute in WebDataBinder.
@InitBinder
void initBinder(final WebDataBinder binder) {
binder.setAllowedFields("name", ...);
}
This method sets a restriction on fields that are allowed for binding. And all the other fields are unbound, naturally resulting in null
values.
The other possible reason: incorrect setters in a Bean annotated with @ModelAttribute. For example, Object setName(String name)
instead of void setName(String)
.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53395062%2fmodelattribute-return-incorrect-value%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Ensure that you are not blocking any attribute in WebDataBinder.
@InitBinder
void initBinder(final WebDataBinder binder) {
binder.setAllowedFields("name", ...);
}
This method sets a restriction on fields that are allowed for binding. And all the other fields are unbound, naturally resulting in null
values.
The other possible reason: incorrect setters in a Bean annotated with @ModelAttribute. For example, Object setName(String name)
instead of void setName(String)
.
add a comment |
Ensure that you are not blocking any attribute in WebDataBinder.
@InitBinder
void initBinder(final WebDataBinder binder) {
binder.setAllowedFields("name", ...);
}
This method sets a restriction on fields that are allowed for binding. And all the other fields are unbound, naturally resulting in null
values.
The other possible reason: incorrect setters in a Bean annotated with @ModelAttribute. For example, Object setName(String name)
instead of void setName(String)
.
add a comment |
Ensure that you are not blocking any attribute in WebDataBinder.
@InitBinder
void initBinder(final WebDataBinder binder) {
binder.setAllowedFields("name", ...);
}
This method sets a restriction on fields that are allowed for binding. And all the other fields are unbound, naturally resulting in null
values.
The other possible reason: incorrect setters in a Bean annotated with @ModelAttribute. For example, Object setName(String name)
instead of void setName(String)
.
Ensure that you are not blocking any attribute in WebDataBinder.
@InitBinder
void initBinder(final WebDataBinder binder) {
binder.setAllowedFields("name", ...);
}
This method sets a restriction on fields that are allowed for binding. And all the other fields are unbound, naturally resulting in null
values.
The other possible reason: incorrect setters in a Bean annotated with @ModelAttribute. For example, Object setName(String name)
instead of void setName(String)
.
answered Nov 20 at 14:34


Alien
4,79331025
4,79331025
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53395062%2fmodelattribute-return-incorrect-value%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
tM,bG39Uu2jpNzm0hvjz,JG7VYSOB2RJrCXlr,4RV3eX5lJsDJXHvVVFunVkPoJj9Ghp
I'd say that's because of
<select name="nameFont">
. With<option>
you basically just send the value of the option back to the server which is the id in your case. Thus it should be<select name="id">
.– Thomas
Nov 20 at 14:32
Is it possible to send all the class values?nameFont and ID?
– crazy_deviL
Nov 20 at 14:35
That should be possible but you'd probably have to change a thing or two (which also might depend on the web framework you're using): with traditional form data you'd probably have to add hidden fields for the additional values of the selected element (e.g. via JavaScript) or make the value of each option a complete representation of the selected element and parse that on the server. Alteratively you could ditch the form and post JSON data which would require even more JavaScript to build that on the client though.
– Thomas
Nov 21 at 9:00
... However, imo the best option would be to just keep sending the id and look up the object on the server. That's less work to do, less error prone (what if the selection of a font would also change its name?) and more secure (again what if the name of the font or any other object you use that approach with would be changed in some malicious way?).
– Thomas
Nov 21 at 9:02