Spring Data - OR condition in a repository method name
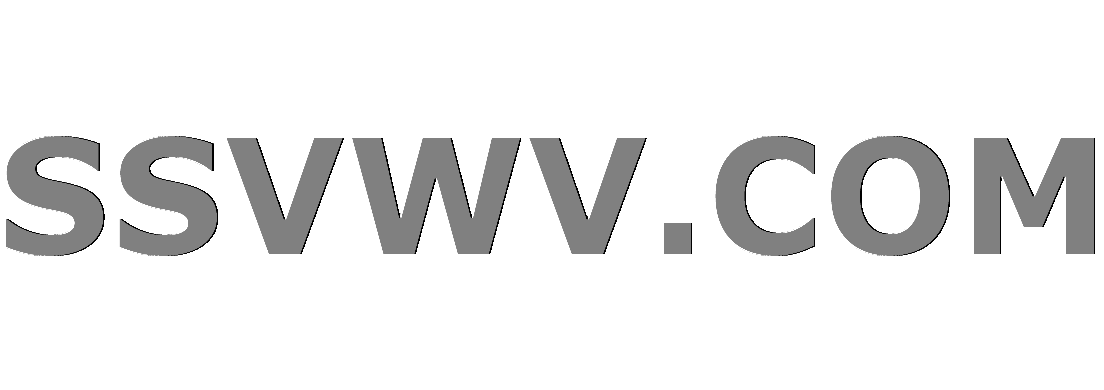
Multi tool use
In my Spring Data project I have a following entity:
@Entity
@NamedEntityGraph(name = "graph.CardPair", attributeNodes = {})
@Table(name = "card_pairs")
public class CardPair extends BaseEntity implements Serializable {
private static final long serialVersionUID = 7571004010972840728L;
@Id
@SequenceGenerator(name = "card_pairs_id_seq", sequenceName = "card_pairs_id_seq", allocationSize = 1)
@GeneratedValue(strategy = GenerationType.AUTO, generator = "card_pairs_id_seq")
private Long id;
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "card1_id")
private Card card1;
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "card2_id")
private Card card2;
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "selected_card_id")
private Card selectedCard;
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "board_id")
private Board board;
....
}
I need to implement a Spring Data repository method that will look for a CardPair
based on cardPairId
, board
and card
. The more complicated case to me is a Card
because I need to construct a condition where card1 = card or card2 = card
Is it possible to provide this condition via Spring Data repository method name ?
For example
cardPairRepository.findByIdAndBoardAnd[card1 = card or card2 = card]AndSelectedCardIsNull(cardPairId, board, card);
Is it possible to translate this condition card1 = card or card2 = card
in a method name ?
java spring jpa spring-data spring-data-jpa
add a comment |
In my Spring Data project I have a following entity:
@Entity
@NamedEntityGraph(name = "graph.CardPair", attributeNodes = {})
@Table(name = "card_pairs")
public class CardPair extends BaseEntity implements Serializable {
private static final long serialVersionUID = 7571004010972840728L;
@Id
@SequenceGenerator(name = "card_pairs_id_seq", sequenceName = "card_pairs_id_seq", allocationSize = 1)
@GeneratedValue(strategy = GenerationType.AUTO, generator = "card_pairs_id_seq")
private Long id;
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "card1_id")
private Card card1;
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "card2_id")
private Card card2;
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "selected_card_id")
private Card selectedCard;
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "board_id")
private Board board;
....
}
I need to implement a Spring Data repository method that will look for a CardPair
based on cardPairId
, board
and card
. The more complicated case to me is a Card
because I need to construct a condition where card1 = card or card2 = card
Is it possible to provide this condition via Spring Data repository method name ?
For example
cardPairRepository.findByIdAndBoardAnd[card1 = card or card2 = card]AndSelectedCardIsNull(cardPairId, board, card);
Is it possible to translate this condition card1 = card or card2 = card
in a method name ?
java spring jpa spring-data spring-data-jpa
Are you just looking for a method name here?
– George Artemiou
May 9 '16 at 12:17
Yes, I can't construct this method name with a mentioned condition
– alexanoid
May 9 '16 at 12:18
2
Use the finder methods only for simple use cases, for complexer cases write a query and use the@Query
annotation.
– M. Deinum
May 9 '16 at 12:26
1
I would strongly suggest to write the query yourself using HPQL..
– Raphael Roth
May 9 '16 at 12:28
Thanks! I have implemented JPQL query and now everything works fine.@Query(value = "SELECT cp FROM CardPair cp WHERE id = :cardPairId AND board = :board AND card1 = :card OR card2 = :card AND selectedCard is null")
– alexanoid
May 9 '16 at 12:40
add a comment |
In my Spring Data project I have a following entity:
@Entity
@NamedEntityGraph(name = "graph.CardPair", attributeNodes = {})
@Table(name = "card_pairs")
public class CardPair extends BaseEntity implements Serializable {
private static final long serialVersionUID = 7571004010972840728L;
@Id
@SequenceGenerator(name = "card_pairs_id_seq", sequenceName = "card_pairs_id_seq", allocationSize = 1)
@GeneratedValue(strategy = GenerationType.AUTO, generator = "card_pairs_id_seq")
private Long id;
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "card1_id")
private Card card1;
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "card2_id")
private Card card2;
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "selected_card_id")
private Card selectedCard;
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "board_id")
private Board board;
....
}
I need to implement a Spring Data repository method that will look for a CardPair
based on cardPairId
, board
and card
. The more complicated case to me is a Card
because I need to construct a condition where card1 = card or card2 = card
Is it possible to provide this condition via Spring Data repository method name ?
For example
cardPairRepository.findByIdAndBoardAnd[card1 = card or card2 = card]AndSelectedCardIsNull(cardPairId, board, card);
Is it possible to translate this condition card1 = card or card2 = card
in a method name ?
java spring jpa spring-data spring-data-jpa
In my Spring Data project I have a following entity:
@Entity
@NamedEntityGraph(name = "graph.CardPair", attributeNodes = {})
@Table(name = "card_pairs")
public class CardPair extends BaseEntity implements Serializable {
private static final long serialVersionUID = 7571004010972840728L;
@Id
@SequenceGenerator(name = "card_pairs_id_seq", sequenceName = "card_pairs_id_seq", allocationSize = 1)
@GeneratedValue(strategy = GenerationType.AUTO, generator = "card_pairs_id_seq")
private Long id;
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "card1_id")
private Card card1;
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "card2_id")
private Card card2;
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "selected_card_id")
private Card selectedCard;
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "board_id")
private Board board;
....
}
I need to implement a Spring Data repository method that will look for a CardPair
based on cardPairId
, board
and card
. The more complicated case to me is a Card
because I need to construct a condition where card1 = card or card2 = card
Is it possible to provide this condition via Spring Data repository method name ?
For example
cardPairRepository.findByIdAndBoardAnd[card1 = card or card2 = card]AndSelectedCardIsNull(cardPairId, board, card);
Is it possible to translate this condition card1 = card or card2 = card
in a method name ?
java spring jpa spring-data spring-data-jpa
java spring jpa spring-data spring-data-jpa
edited May 9 '16 at 12:23
asked May 9 '16 at 12:15


alexanoid
7,0821178173
7,0821178173
Are you just looking for a method name here?
– George Artemiou
May 9 '16 at 12:17
Yes, I can't construct this method name with a mentioned condition
– alexanoid
May 9 '16 at 12:18
2
Use the finder methods only for simple use cases, for complexer cases write a query and use the@Query
annotation.
– M. Deinum
May 9 '16 at 12:26
1
I would strongly suggest to write the query yourself using HPQL..
– Raphael Roth
May 9 '16 at 12:28
Thanks! I have implemented JPQL query and now everything works fine.@Query(value = "SELECT cp FROM CardPair cp WHERE id = :cardPairId AND board = :board AND card1 = :card OR card2 = :card AND selectedCard is null")
– alexanoid
May 9 '16 at 12:40
add a comment |
Are you just looking for a method name here?
– George Artemiou
May 9 '16 at 12:17
Yes, I can't construct this method name with a mentioned condition
– alexanoid
May 9 '16 at 12:18
2
Use the finder methods only for simple use cases, for complexer cases write a query and use the@Query
annotation.
– M. Deinum
May 9 '16 at 12:26
1
I would strongly suggest to write the query yourself using HPQL..
– Raphael Roth
May 9 '16 at 12:28
Thanks! I have implemented JPQL query and now everything works fine.@Query(value = "SELECT cp FROM CardPair cp WHERE id = :cardPairId AND board = :board AND card1 = :card OR card2 = :card AND selectedCard is null")
– alexanoid
May 9 '16 at 12:40
Are you just looking for a method name here?
– George Artemiou
May 9 '16 at 12:17
Are you just looking for a method name here?
– George Artemiou
May 9 '16 at 12:17
Yes, I can't construct this method name with a mentioned condition
– alexanoid
May 9 '16 at 12:18
Yes, I can't construct this method name with a mentioned condition
– alexanoid
May 9 '16 at 12:18
2
2
Use the finder methods only for simple use cases, for complexer cases write a query and use the
@Query
annotation.– M. Deinum
May 9 '16 at 12:26
Use the finder methods only for simple use cases, for complexer cases write a query and use the
@Query
annotation.– M. Deinum
May 9 '16 at 12:26
1
1
I would strongly suggest to write the query yourself using HPQL..
– Raphael Roth
May 9 '16 at 12:28
I would strongly suggest to write the query yourself using HPQL..
– Raphael Roth
May 9 '16 at 12:28
Thanks! I have implemented JPQL query and now everything works fine.
@Query(value = "SELECT cp FROM CardPair cp WHERE id = :cardPairId AND board = :board AND card1 = :card OR card2 = :card AND selectedCard is null")
– alexanoid
May 9 '16 at 12:40
Thanks! I have implemented JPQL query and now everything works fine.
@Query(value = "SELECT cp FROM CardPair cp WHERE id = :cardPairId AND board = :board AND card1 = :card OR card2 = :card AND selectedCard is null")
– alexanoid
May 9 '16 at 12:40
add a comment |
2 Answers
2
active
oldest
votes
It is impossible to achieve this using just method name since you need the last condition to be in parentheses: id = ? AND board = ? AND (card1 = ? OR card2 = ?)
. There is no way to infer this from method name since it is intended to cover basic cases only.
There are three ways in your case:
- @Query annotation (the best candidate for you) http://docs.spring.io/spring-data/jpa/docs/current/reference/html/#jpa.query-methods.at-query
- Named queries http://docs.spring.io/spring-data/jpa/docs/current/reference/html/#jpa.query-methods.named-queries
- Specifications http://docs.spring.io/spring-data/jpa/docs/current/reference/html/#specifications
add a comment |
You can find all operations here: http://docs.spring.io/spring-data/jpa/docs/1.3.0.RELEASE/reference/html/jpa.repositories.html section 2.2.2
findByIdAndBoardAndCard1OrCard2(cardPairId, board, card, card);
I haven't testen the order of this, but it should be something like this
it doesn't work - findByIdAndBoardAndCard1OrCard2(cardPairId, currentBoard, card) with run-time exception.
– alexanoid
May 9 '16 at 12:34
card needs to be in the parameters twice.
– Roel Strolenberg
May 9 '16 at 12:40
Besides my last comment, it might not work at all due to brackets that should be added to the OR statement. But it might be that spring knows how to handle this due to order. the@Query
suggest by Alec is probably safer.
– Roel Strolenberg
May 9 '16 at 12:42
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f37115582%2fspring-data-or-condition-in-a-repository-method-name%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
It is impossible to achieve this using just method name since you need the last condition to be in parentheses: id = ? AND board = ? AND (card1 = ? OR card2 = ?)
. There is no way to infer this from method name since it is intended to cover basic cases only.
There are three ways in your case:
- @Query annotation (the best candidate for you) http://docs.spring.io/spring-data/jpa/docs/current/reference/html/#jpa.query-methods.at-query
- Named queries http://docs.spring.io/spring-data/jpa/docs/current/reference/html/#jpa.query-methods.named-queries
- Specifications http://docs.spring.io/spring-data/jpa/docs/current/reference/html/#specifications
add a comment |
It is impossible to achieve this using just method name since you need the last condition to be in parentheses: id = ? AND board = ? AND (card1 = ? OR card2 = ?)
. There is no way to infer this from method name since it is intended to cover basic cases only.
There are three ways in your case:
- @Query annotation (the best candidate for you) http://docs.spring.io/spring-data/jpa/docs/current/reference/html/#jpa.query-methods.at-query
- Named queries http://docs.spring.io/spring-data/jpa/docs/current/reference/html/#jpa.query-methods.named-queries
- Specifications http://docs.spring.io/spring-data/jpa/docs/current/reference/html/#specifications
add a comment |
It is impossible to achieve this using just method name since you need the last condition to be in parentheses: id = ? AND board = ? AND (card1 = ? OR card2 = ?)
. There is no way to infer this from method name since it is intended to cover basic cases only.
There are three ways in your case:
- @Query annotation (the best candidate for you) http://docs.spring.io/spring-data/jpa/docs/current/reference/html/#jpa.query-methods.at-query
- Named queries http://docs.spring.io/spring-data/jpa/docs/current/reference/html/#jpa.query-methods.named-queries
- Specifications http://docs.spring.io/spring-data/jpa/docs/current/reference/html/#specifications
It is impossible to achieve this using just method name since you need the last condition to be in parentheses: id = ? AND board = ? AND (card1 = ? OR card2 = ?)
. There is no way to infer this from method name since it is intended to cover basic cases only.
There are three ways in your case:
- @Query annotation (the best candidate for you) http://docs.spring.io/spring-data/jpa/docs/current/reference/html/#jpa.query-methods.at-query
- Named queries http://docs.spring.io/spring-data/jpa/docs/current/reference/html/#jpa.query-methods.named-queries
- Specifications http://docs.spring.io/spring-data/jpa/docs/current/reference/html/#specifications
edited Nov 20 at 9:44
answered May 9 '16 at 12:40
Alec Kravets
2,595811
2,595811
add a comment |
add a comment |
You can find all operations here: http://docs.spring.io/spring-data/jpa/docs/1.3.0.RELEASE/reference/html/jpa.repositories.html section 2.2.2
findByIdAndBoardAndCard1OrCard2(cardPairId, board, card, card);
I haven't testen the order of this, but it should be something like this
it doesn't work - findByIdAndBoardAndCard1OrCard2(cardPairId, currentBoard, card) with run-time exception.
– alexanoid
May 9 '16 at 12:34
card needs to be in the parameters twice.
– Roel Strolenberg
May 9 '16 at 12:40
Besides my last comment, it might not work at all due to brackets that should be added to the OR statement. But it might be that spring knows how to handle this due to order. the@Query
suggest by Alec is probably safer.
– Roel Strolenberg
May 9 '16 at 12:42
add a comment |
You can find all operations here: http://docs.spring.io/spring-data/jpa/docs/1.3.0.RELEASE/reference/html/jpa.repositories.html section 2.2.2
findByIdAndBoardAndCard1OrCard2(cardPairId, board, card, card);
I haven't testen the order of this, but it should be something like this
it doesn't work - findByIdAndBoardAndCard1OrCard2(cardPairId, currentBoard, card) with run-time exception.
– alexanoid
May 9 '16 at 12:34
card needs to be in the parameters twice.
– Roel Strolenberg
May 9 '16 at 12:40
Besides my last comment, it might not work at all due to brackets that should be added to the OR statement. But it might be that spring knows how to handle this due to order. the@Query
suggest by Alec is probably safer.
– Roel Strolenberg
May 9 '16 at 12:42
add a comment |
You can find all operations here: http://docs.spring.io/spring-data/jpa/docs/1.3.0.RELEASE/reference/html/jpa.repositories.html section 2.2.2
findByIdAndBoardAndCard1OrCard2(cardPairId, board, card, card);
I haven't testen the order of this, but it should be something like this
You can find all operations here: http://docs.spring.io/spring-data/jpa/docs/1.3.0.RELEASE/reference/html/jpa.repositories.html section 2.2.2
findByIdAndBoardAndCard1OrCard2(cardPairId, board, card, card);
I haven't testen the order of this, but it should be something like this
answered May 9 '16 at 12:26


Roel Strolenberg
2,2871823
2,2871823
it doesn't work - findByIdAndBoardAndCard1OrCard2(cardPairId, currentBoard, card) with run-time exception.
– alexanoid
May 9 '16 at 12:34
card needs to be in the parameters twice.
– Roel Strolenberg
May 9 '16 at 12:40
Besides my last comment, it might not work at all due to brackets that should be added to the OR statement. But it might be that spring knows how to handle this due to order. the@Query
suggest by Alec is probably safer.
– Roel Strolenberg
May 9 '16 at 12:42
add a comment |
it doesn't work - findByIdAndBoardAndCard1OrCard2(cardPairId, currentBoard, card) with run-time exception.
– alexanoid
May 9 '16 at 12:34
card needs to be in the parameters twice.
– Roel Strolenberg
May 9 '16 at 12:40
Besides my last comment, it might not work at all due to brackets that should be added to the OR statement. But it might be that spring knows how to handle this due to order. the@Query
suggest by Alec is probably safer.
– Roel Strolenberg
May 9 '16 at 12:42
it doesn't work - findByIdAndBoardAndCard1OrCard2(cardPairId, currentBoard, card) with run-time exception.
– alexanoid
May 9 '16 at 12:34
it doesn't work - findByIdAndBoardAndCard1OrCard2(cardPairId, currentBoard, card) with run-time exception.
– alexanoid
May 9 '16 at 12:34
card needs to be in the parameters twice.
– Roel Strolenberg
May 9 '16 at 12:40
card needs to be in the parameters twice.
– Roel Strolenberg
May 9 '16 at 12:40
Besides my last comment, it might not work at all due to brackets that should be added to the OR statement. But it might be that spring knows how to handle this due to order. the
@Query
suggest by Alec is probably safer.– Roel Strolenberg
May 9 '16 at 12:42
Besides my last comment, it might not work at all due to brackets that should be added to the OR statement. But it might be that spring knows how to handle this due to order. the
@Query
suggest by Alec is probably safer.– Roel Strolenberg
May 9 '16 at 12:42
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f37115582%2fspring-data-or-condition-in-a-repository-method-name%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
rBK2re,DlEjzqb3f4dEaQhoQ8eQ H5q055u,bP eJsS3vl3Rgdgy Bh,bQfpUio95XxA l8Yn8X9758TQFIbqrZJ9N Z,ONqCCA,WH
Are you just looking for a method name here?
– George Artemiou
May 9 '16 at 12:17
Yes, I can't construct this method name with a mentioned condition
– alexanoid
May 9 '16 at 12:18
2
Use the finder methods only for simple use cases, for complexer cases write a query and use the
@Query
annotation.– M. Deinum
May 9 '16 at 12:26
1
I would strongly suggest to write the query yourself using HPQL..
– Raphael Roth
May 9 '16 at 12:28
Thanks! I have implemented JPQL query and now everything works fine.
@Query(value = "SELECT cp FROM CardPair cp WHERE id = :cardPairId AND board = :board AND card1 = :card OR card2 = :card AND selectedCard is null")
– alexanoid
May 9 '16 at 12:40