NoSuchMethodError:...
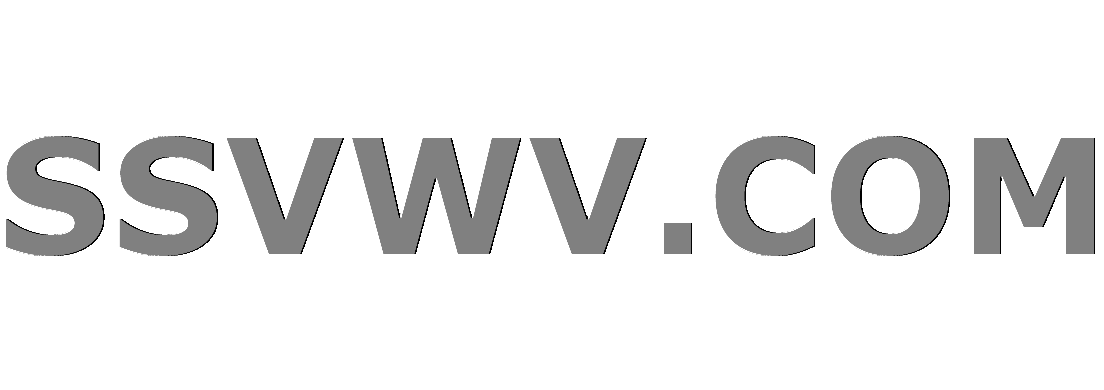
Multi tool use
up vote
2
down vote
favorite
I'm trying to create an API which, when triggered, sends a request to Dialogflow.
To do that, I'm using the Java SDK, as found here.
The problematic code snippet is copy-pasted from here, to the bottom of the page.
try (SessionsClient sessionsClient = SessionsClient.create()) {
SessionName session = SessionName.of(genericProjectId, genericSessionId);
QueryInput queryInput = QueryInput.newBuilder().build();
DetectIntentResponse response = sessionsClient.detectIntent(session,
queryInput);
}
When I run "SessionsClient.create()", the error
javax.ejb.TransactionRolledbackLocalException: Exception thrown from bean: java.lang.NoSuchMethodError: com.google.common.base.Preconditions.checkArgument(ZLjava/lang/String;CLjava/lang/Object;)V
is thrown at runtime. After research, it looks like this has something to do with different versions of the libraries being included, mainly protobuf and guava; however, I trimmed my POM as much as possible, and it doesn't go away.
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.mycompany</groupId>
<artifactId>MYPROJECTNAME</artifactId>
<version>1.0-SNAPSHOT</version>
<packaging>war</packaging>
<dependencies>
<dependency>
<groupId>com.google.cloud</groupId>
<artifactId>google-cloud-dialogflow</artifactId>
<version>0.71.0-alpha</version>
</dependency>
<dependency>
<groupId>javax</groupId>
<artifactId>javaee-api</artifactId>
<version>7.0</version>
<scope>provided</scope>
</dependency>
</dependencies>
<build>
<finalName>MYPROJECTNAME</finalName>
<plugins>
</plugins>
</build>
<properties>
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
<failOnMissingWebXml>false</failOnMissingWebXml>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<projectId>MYPROJECTID</projectId>
</properties>
</project>
Running mvn depenceny:tree doesn't show any problems, and by opening the WAR archive created I can't see any duplicate library. Tried compiling both with NetBeans and by command line, the problem still persists. The application is running on Windows, using Java 8 and Payara as server. What am I doing wrong?
Thanks for any help
java dialogflow nosuchmethoderror
add a comment |
up vote
2
down vote
favorite
I'm trying to create an API which, when triggered, sends a request to Dialogflow.
To do that, I'm using the Java SDK, as found here.
The problematic code snippet is copy-pasted from here, to the bottom of the page.
try (SessionsClient sessionsClient = SessionsClient.create()) {
SessionName session = SessionName.of(genericProjectId, genericSessionId);
QueryInput queryInput = QueryInput.newBuilder().build();
DetectIntentResponse response = sessionsClient.detectIntent(session,
queryInput);
}
When I run "SessionsClient.create()", the error
javax.ejb.TransactionRolledbackLocalException: Exception thrown from bean: java.lang.NoSuchMethodError: com.google.common.base.Preconditions.checkArgument(ZLjava/lang/String;CLjava/lang/Object;)V
is thrown at runtime. After research, it looks like this has something to do with different versions of the libraries being included, mainly protobuf and guava; however, I trimmed my POM as much as possible, and it doesn't go away.
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.mycompany</groupId>
<artifactId>MYPROJECTNAME</artifactId>
<version>1.0-SNAPSHOT</version>
<packaging>war</packaging>
<dependencies>
<dependency>
<groupId>com.google.cloud</groupId>
<artifactId>google-cloud-dialogflow</artifactId>
<version>0.71.0-alpha</version>
</dependency>
<dependency>
<groupId>javax</groupId>
<artifactId>javaee-api</artifactId>
<version>7.0</version>
<scope>provided</scope>
</dependency>
</dependencies>
<build>
<finalName>MYPROJECTNAME</finalName>
<plugins>
</plugins>
</build>
<properties>
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
<failOnMissingWebXml>false</failOnMissingWebXml>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<projectId>MYPROJECTID</projectId>
</properties>
</project>
Running mvn depenceny:tree doesn't show any problems, and by opening the WAR archive created I can't see any duplicate library. Tried compiling both with NetBeans and by command line, the problem still persists. The application is running on Windows, using Java 8 and Payara as server. What am I doing wrong?
Thanks for any help
java dialogflow nosuchmethoderror
You'd have to check which librarycom.google.common.base.Preconditions
belongs to (I'd assume Guava) and which versions of that library contain that method. It could be that your build somehow uses a version that is too old or too new and thus the method doesn't exist yet/anymore.
– Thomas
Nov 19 at 13:46
Maven decided to use the version 26.0-android. Tried to manually set it to version 27, 26-jre, 23, 22 and 20, with no success.
– Dod
Nov 19 at 14:24
add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
I'm trying to create an API which, when triggered, sends a request to Dialogflow.
To do that, I'm using the Java SDK, as found here.
The problematic code snippet is copy-pasted from here, to the bottom of the page.
try (SessionsClient sessionsClient = SessionsClient.create()) {
SessionName session = SessionName.of(genericProjectId, genericSessionId);
QueryInput queryInput = QueryInput.newBuilder().build();
DetectIntentResponse response = sessionsClient.detectIntent(session,
queryInput);
}
When I run "SessionsClient.create()", the error
javax.ejb.TransactionRolledbackLocalException: Exception thrown from bean: java.lang.NoSuchMethodError: com.google.common.base.Preconditions.checkArgument(ZLjava/lang/String;CLjava/lang/Object;)V
is thrown at runtime. After research, it looks like this has something to do with different versions of the libraries being included, mainly protobuf and guava; however, I trimmed my POM as much as possible, and it doesn't go away.
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.mycompany</groupId>
<artifactId>MYPROJECTNAME</artifactId>
<version>1.0-SNAPSHOT</version>
<packaging>war</packaging>
<dependencies>
<dependency>
<groupId>com.google.cloud</groupId>
<artifactId>google-cloud-dialogflow</artifactId>
<version>0.71.0-alpha</version>
</dependency>
<dependency>
<groupId>javax</groupId>
<artifactId>javaee-api</artifactId>
<version>7.0</version>
<scope>provided</scope>
</dependency>
</dependencies>
<build>
<finalName>MYPROJECTNAME</finalName>
<plugins>
</plugins>
</build>
<properties>
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
<failOnMissingWebXml>false</failOnMissingWebXml>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<projectId>MYPROJECTID</projectId>
</properties>
</project>
Running mvn depenceny:tree doesn't show any problems, and by opening the WAR archive created I can't see any duplicate library. Tried compiling both with NetBeans and by command line, the problem still persists. The application is running on Windows, using Java 8 and Payara as server. What am I doing wrong?
Thanks for any help
java dialogflow nosuchmethoderror
I'm trying to create an API which, when triggered, sends a request to Dialogflow.
To do that, I'm using the Java SDK, as found here.
The problematic code snippet is copy-pasted from here, to the bottom of the page.
try (SessionsClient sessionsClient = SessionsClient.create()) {
SessionName session = SessionName.of(genericProjectId, genericSessionId);
QueryInput queryInput = QueryInput.newBuilder().build();
DetectIntentResponse response = sessionsClient.detectIntent(session,
queryInput);
}
When I run "SessionsClient.create()", the error
javax.ejb.TransactionRolledbackLocalException: Exception thrown from bean: java.lang.NoSuchMethodError: com.google.common.base.Preconditions.checkArgument(ZLjava/lang/String;CLjava/lang/Object;)V
is thrown at runtime. After research, it looks like this has something to do with different versions of the libraries being included, mainly protobuf and guava; however, I trimmed my POM as much as possible, and it doesn't go away.
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.mycompany</groupId>
<artifactId>MYPROJECTNAME</artifactId>
<version>1.0-SNAPSHOT</version>
<packaging>war</packaging>
<dependencies>
<dependency>
<groupId>com.google.cloud</groupId>
<artifactId>google-cloud-dialogflow</artifactId>
<version>0.71.0-alpha</version>
</dependency>
<dependency>
<groupId>javax</groupId>
<artifactId>javaee-api</artifactId>
<version>7.0</version>
<scope>provided</scope>
</dependency>
</dependencies>
<build>
<finalName>MYPROJECTNAME</finalName>
<plugins>
</plugins>
</build>
<properties>
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
<failOnMissingWebXml>false</failOnMissingWebXml>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<projectId>MYPROJECTID</projectId>
</properties>
</project>
Running mvn depenceny:tree doesn't show any problems, and by opening the WAR archive created I can't see any duplicate library. Tried compiling both with NetBeans and by command line, the problem still persists. The application is running on Windows, using Java 8 and Payara as server. What am I doing wrong?
Thanks for any help
java dialogflow nosuchmethoderror
java dialogflow nosuchmethoderror
edited Nov 19 at 13:52
asked Nov 19 at 13:34
Dod
3316
3316
You'd have to check which librarycom.google.common.base.Preconditions
belongs to (I'd assume Guava) and which versions of that library contain that method. It could be that your build somehow uses a version that is too old or too new and thus the method doesn't exist yet/anymore.
– Thomas
Nov 19 at 13:46
Maven decided to use the version 26.0-android. Tried to manually set it to version 27, 26-jre, 23, 22 and 20, with no success.
– Dod
Nov 19 at 14:24
add a comment |
You'd have to check which librarycom.google.common.base.Preconditions
belongs to (I'd assume Guava) and which versions of that library contain that method. It could be that your build somehow uses a version that is too old or too new and thus the method doesn't exist yet/anymore.
– Thomas
Nov 19 at 13:46
Maven decided to use the version 26.0-android. Tried to manually set it to version 27, 26-jre, 23, 22 and 20, with no success.
– Dod
Nov 19 at 14:24
You'd have to check which library
com.google.common.base.Preconditions
belongs to (I'd assume Guava) and which versions of that library contain that method. It could be that your build somehow uses a version that is too old or too new and thus the method doesn't exist yet/anymore.– Thomas
Nov 19 at 13:46
You'd have to check which library
com.google.common.base.Preconditions
belongs to (I'd assume Guava) and which versions of that library contain that method. It could be that your build somehow uses a version that is too old or too new and thus the method doesn't exist yet/anymore.– Thomas
Nov 19 at 13:46
Maven decided to use the version 26.0-android. Tried to manually set it to version 27, 26-jre, 23, 22 and 20, with no success.
– Dod
Nov 19 at 14:24
Maven decided to use the version 26.0-android. Tried to manually set it to version 27, 26-jre, 23, 22 and 20, with no success.
– Dod
Nov 19 at 14:24
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
After raising an issue on Google Cloud repository, it looks like this was indeed a problem with Guava, so it's a bug on their side. I'm still unsure about how could this pass unnoticed, since somehow their quickstart guide must have worked at some point, but here it is. Thanks to those who bothered upvoting the question.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
After raising an issue on Google Cloud repository, it looks like this was indeed a problem with Guava, so it's a bug on their side. I'm still unsure about how could this pass unnoticed, since somehow their quickstart guide must have worked at some point, but here it is. Thanks to those who bothered upvoting the question.
add a comment |
up vote
0
down vote
After raising an issue on Google Cloud repository, it looks like this was indeed a problem with Guava, so it's a bug on their side. I'm still unsure about how could this pass unnoticed, since somehow their quickstart guide must have worked at some point, but here it is. Thanks to those who bothered upvoting the question.
add a comment |
up vote
0
down vote
up vote
0
down vote
After raising an issue on Google Cloud repository, it looks like this was indeed a problem with Guava, so it's a bug on their side. I'm still unsure about how could this pass unnoticed, since somehow their quickstart guide must have worked at some point, but here it is. Thanks to those who bothered upvoting the question.
After raising an issue on Google Cloud repository, it looks like this was indeed a problem with Guava, so it's a bug on their side. I'm still unsure about how could this pass unnoticed, since somehow their quickstart guide must have worked at some point, but here it is. Thanks to those who bothered upvoting the question.
answered Nov 22 at 9:55
Dod
3316
3316
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53375783%2fnosuchmethoderror-com-google-common-base-preconditions-checkargumentzljava-lan%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
HodXfpF03,XV8Sc3EhnGgUSv2A6I6f
You'd have to check which library
com.google.common.base.Preconditions
belongs to (I'd assume Guava) and which versions of that library contain that method. It could be that your build somehow uses a version that is too old or too new and thus the method doesn't exist yet/anymore.– Thomas
Nov 19 at 13:46
Maven decided to use the version 26.0-android. Tried to manually set it to version 27, 26-jre, 23, 22 and 20, with no success.
– Dod
Nov 19 at 14:24