How to read a file line-by-line into a list?
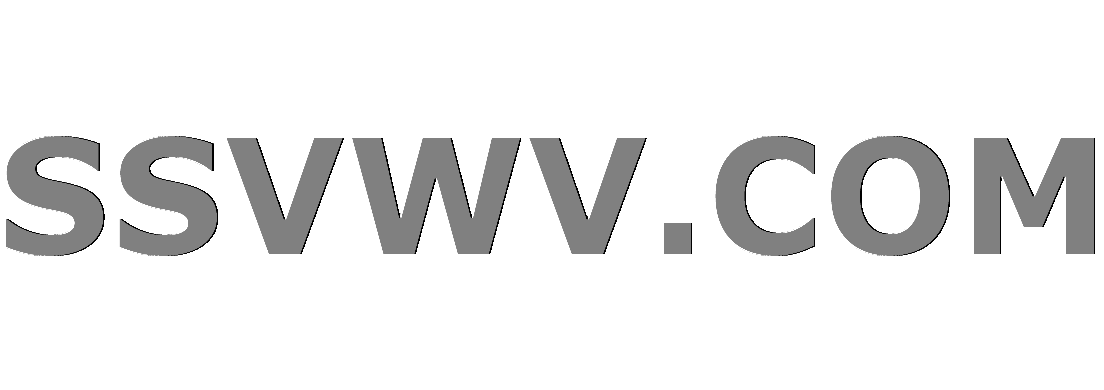
Multi tool use
How do I read every line of a file in Python and store each line as an element in a list?
I want to read the file line by line and append each line to the end of the list.
python string file readlines
add a comment |
How do I read every line of a file in Python and store each line as an element in a list?
I want to read the file line by line and append each line to the end of the list.
python string file readlines
add a comment |
How do I read every line of a file in Python and store each line as an element in a list?
I want to read the file line by line and append each line to the end of the list.
python string file readlines
How do I read every line of a file in Python and store each line as an element in a list?
I want to read the file line by line and append each line to the end of the list.
python string file readlines
python string file readlines
edited Nov 16 '18 at 0:14


martineau
67.8k1089182
67.8k1089182
asked Jul 18 '10 at 22:25
Julie RaswickJulie Raswick
9,6653113
9,6653113
add a comment |
add a comment |
33 Answers
33
active
oldest
votes
1 2
next
with open(fname) as f:
content = f.readlines()
# you may also want to remove whitespace characters like `n` at the end of each line
content = [x.strip() for x in content]
139
Don't usefile.readlines()
in afor
-loop, a file object itself is enough:lines = [line.rstrip('n') for line in file]
– jfs
Jan 14 '15 at 10:52
50
In the case you are working with Big Data usingreadlines()
is not very efficient as it can result in MemoryError. In this case it is better to iterate over the file usingfor line in f:
and working with eachline
variable.
– DarkCygnus
Aug 27 '16 at 3:07
3
I checked the memory profile of different ways given in the answers using the procedure mentioned here. The memory usage is far better when each line is read from the file and processed, as suggested by @DevShark here. Holding all lines in a collection object is not a good idea if memory is a constraint or the file is large. The execution time is similar in both the approaches.
– Tirtha R
Mar 2 '18 at 23:24
2
Also,.rstrip()
will work slightly faster if you are stripping whitespace from the ends of lines.
– Gringo Suave
Jun 15 '18 at 19:14
add a comment |
See Input and Ouput:
with open('filename') as f:
lines = f.readlines()
or with stripping the newline character:
lines = [line.rstrip('n') for line in open('filename')]
Editor's note: This answer's original whitespace-stripping command, line.strip()
, as implied by Janus Troelsen's comment, would remove all leading and trailing whitespace, not just the trailing n
.
67
if you only want to discard the newline:lines = (line.rstrip('n') for line in open(filename))
– Janus Troelsen
Oct 11 '12 at 10:14
14
For a list it should belines = [line.rstrip('n') for line in open(filename)]
– Lazik
Oct 12 '13 at 14:32
25
Won't the 2nd option leave the file open (since it's not guarded by a context on its own)?
– yo'
Feb 8 '15 at 19:14
9
@yo' It does, but most people do not care about that in small programs. There is no harm in small programs since the leaked file object are garbage collected, but it is not a good habit to do this.
– Martin Ueding
May 4 '15 at 8:01
17
Safer:with open('filename') as f: lines = [line.rstrip('n') for line in f]
– becko
Feb 10 '16 at 16:36
|
show 7 more comments
This is more explicit than necessary, but does what you want.
with open("file.txt", "r") as ins:
array =
for line in ins:
array.append(line)
8
This is a direct answer to the question
– Joop
Jul 14 '18 at 9:15
7
I prefer this answer since it doesn't require to load the whole file into memory (in this case it is still appended toarray
though, but there might be other circumstances). Certainly for big files this approach might mitigate problems.
– JohannesB
Sep 19 '18 at 12:44
Appending to an array is slow. I cannot think of a use case where this is the best solution.
– Elias Strehle
Oct 4 '18 at 12:48
1
This answer received less votes than it deserve (and do not deserve downvotes). It is much better than usingreadlines
.
– haccks
Oct 20 '18 at 20:12
@haccks is it better because it doesn't load the whole file to memory or is there more?
– OrigamiEye
Dec 1 '18 at 15:39
|
show 2 more comments
This will yield an "array" of lines from the file.
lines = tuple(open(filename, 'r'))
37
open
returns a file which can be iterated over. When you iterate over a file, you get the lines from that file.tuple
can take an iterator and instantiate a tuple instance for you from the iterator that you give it.lines
is a tuple created from the lines of the file.
– Noctis Skytower
Jan 5 '14 at 21:58
24
@MarshallFarrier Trylines = open(filename).read().split('n')
instead.
– Noctis Skytower
Dec 11 '14 at 13:56
10
does it close the file?
– Vanuan
Jan 3 '15 at 2:21
24
@NoctisSkytower I findlines = open(filename).read().splitlines()
a little cleaner, and I believe it also handles DOS line endings better.
– jaynp
May 13 '15 at 5:59
6
@mklement0 Assuming a file of 1000 lines, alist
takes up about 13.22% more space than atuple
. Results come fromfrom sys import getsizeof as g; i = [None] * 1000; round((g(list(i)) / g(tuple(i)) - 1) * 100, 2)
. Creating atuple
takes about 4.17% more time than creating alist
(with a 0.16% standard deviation). Results come from runningfrom timeit import timeit as t; round((t('tuple(i)', 'i = [None] * 1000') / t('list(i)', 'i = [None] * 1000') - 1) * 100, 2)
30 times. My solution favors space over speed when the need for mutability is unknown.
– Noctis Skytower
Jan 4 '16 at 16:17
|
show 4 more comments
If you want the n
included:
with open(fname) as f:
content = f.readlines()
If you do not want n
included:
with open(fname) as f:
content = f.read().splitlines()
add a comment |
You could simply do the following, as has been suggested:
with open('/your/path/file') as f:
my_lines = f.readlines()
Note that this approach has 2 downsides:
1) You store all the lines in memory. In the general case, this is a very bad idea. The file could be very large, and you could run out of memory. Even if it's not large, it is simply a waste of memory.
2) This does not allow processing of each line as you read them. So if you process your lines after this, it is not efficient (requires two passes rather than one).
A better approach for the general case would be the following:
with open('/your/path/file') as f:
for line in f:
process(line)
Where you define your process function any way you want. For example:
def process(line):
if 'save the world' in line.lower():
superman.save_the_world()
(The implementation of the Superman
class is left as an exercise for you).
This will work nicely for any file size and you go through your file in just 1 pass. This is typically how generic parsers will work.
3
This was exactly what I needed - and thanks for explaining the downsides. As a beginner in Python, it's awesome to understand why a solution is the solution. Cheers!
– Ephexx
May 17 '16 at 21:37
3
Think a bit more Corey. Do you really ever want your computer to read each line, without ever doing anything with these lines? Surely you can realize you always need to process them one way or another.
– DevShark
Dec 13 '16 at 7:31
4
You always need to do something with the lines. It can be as simple as printing the lines, or counting them. There is no value in having your process read the lines in memory, but not doing anything with it.
– DevShark
Dec 14 '16 at 10:22
2
You always need to do something with them. I think the point you are trying to make is that you might want to apply a function to all of them at once, rather than one by one. That is indeed the case sometimes. But it is very inefficient from a memory standpoint to do so, and prevents you from reading files if its footprint is larger than your Ram. That's why typically generic parsers operate in the way I described.
– DevShark
Jun 23 '17 at 19:40
2
@PierreOcinom that is correct. Given that the file is opened in read only mode, you couldn't modify the original file with the code above. To open a file for both reading and writing, useopen('file_path', 'r+')
– DevShark
Sep 14 '17 at 9:17
|
show 8 more comments
If you don't care about closing the file, this one-liner works:
lines = open('file.txt').readlines()
The traditional way:
fp = open('file.txt') # Open file on read mode
lines = fp.read().split("n") # Create a list containing all lines
fp.close() # Close file
Using with
and readlines()
(recommended):
with open('file.txt') as fp:
lines = fp.readlines()
3
It might be fine in some cases, but this doesn't close the file, even after the loop has completed - stackoverflow.com/a/1832589/232593
– Merlyn Morgan-Graham
Dec 11 '15 at 0:02
3
Thewith
block closes the file automatically. No need for the finalfp.close()
line in that last example. See: repl.it/IMeA/0
– Merlyn Morgan-Graham
May 23 '17 at 8:57
5
Always care about closing the file! Be a good resource citizen!
– Nick
Jun 29 '18 at 14:38
All the work you're doing here is done by the Pythonreadlines()
method.
– Eric O Lebigot
Oct 23 '18 at 10:58
1
At least for me with Python 3.7.0 it wasfp.readlines()
instead offp.read().readlines()
.
– Erik Nomitch
Nov 28 '18 at 1:57
|
show 2 more comments
Data into list
Assume that we have a text file with our data like in the following lines:
Text file content:
line 1
line 2
line 3
- Open the cmd in the same directory (right click the mouse and choose cmd or PowerShell)
- Run
python
and in the interpreter write:
The Python script
>>> with open("myfile.txt", encoding="utf-8") as file:
... x = [l.strip() for l in file]
>>> x
['line 1','line 2','line 3']
Using append
x =
with open("myfile.txt") as file:
for l in file:
x.append(l.strip())
Or...
>>> x = open("myfile.txt").read().splitlines()
>>> x
['line 1', 'line 2', 'line 3']
Or...
>>> x = open("myfile.txt").readlines()
>>> x
['linea 1n', 'line 2n', 'line 3n']
Or...
>>> y = [x.rstrip() for x in open("my_file.txt")]
>>> y
['line 1','line 2','line 3']
with open('testodiprova.txt', 'r', encoding='utf-8') as file:
file = file.read().splitlines()
print(file)
with open('testodiprova.txt', 'r', encoding='utf-8') as file:
file = file.readlines()
print(file)
is theencoding="utf-8"
required?
– Mausy5043
Jun 3 '18 at 8:53
@Mausy5043 no, but when you read a text file, you can have some strange character (expecially in italian)
– Giovanni Gianni
Jun 3 '18 at 9:55
read().splitlines()
is provided to you by Python: it's simplyreadlines()
(which is probably faster, as it is less wasteful).
– Eric O Lebigot
Oct 23 '18 at 10:57
add a comment |
This should encapsulate the open command.
array =
with open("file.txt", "r") as f:
for line in f:
array.append(line)
2
f.readlines() does the same. no need to append to an empty list.
– Corey Goldberg
Mar 6 '15 at 14:51
6
You are right. This provides insight into a solution if you want to do something while you are reading in the lines. Like some strip/regex transformation.
– cevaris
Mar 6 '15 at 15:44
An answer like this has already existed for 3 years before you posted this.
– Aran-Fey
Oct 27 '18 at 15:23
You Sr. are correct. Unsure why this is front page. stackoverflow.com/questions/3277503/…
– cevaris
Oct 28 '18 at 16:53
add a comment |
Clean and Pythonic Way of Reading the Lines of a File Into a List
First and foremost, you should focus on opening your file and reading its contents in an efficient and pythonic way. Here is an example of the way I personally DO NOT prefer:
infile = open('my_file.txt', 'r') # Open the file for reading.
data = infile.read() # Read the contents of the file.
infile.close() # Close the file since we're done using it.
Instead, I prefer the below method of opening files for both reading and writing as it
is very clean, and does not require an extra step of closing the file
once you are done using it. In the statement below, we're opening the file
for reading, and assigning it to the variable 'infile.' Once the code within
this statement has finished running, the file will be automatically closed.
# Open the file for reading.
with open('my_file.txt', 'r') as infile:
data = infile.read() # Read the contents of the file into memory.
Now we need to focus on bringing this data into a Python List because they are iterable, efficient, and flexible. In your case, the desired goal is to bring each line of the text file into a separate element. To accomplish this, we will use the splitlines() method as follows:
# Return a list of the lines, breaking at line boundaries.
my_list = data.splitlines()
The Final Product:
# Open the file for reading.
with open('my_file.txt', 'r') as infile:
data = infile.read() # Read the contents of the file into memory.
# Return a list of the lines, breaking at line boundaries.
my_list = data.splitlines()
Testing Our Code:
- Contents of the text file:
A fost odatã ca-n povesti,
A fost ca niciodatã,
Din rude mãri împãrãtesti,
O prea frumoasã fatã.
- Print statements for testing purposes:
print my_list # Print the list.
# Print each line in the list.
for line in my_list:
print line
# Print the fourth element in this list.
print my_list[3]
- Output (different-looking because of unicode characters):
['A fost odatxc3xa3 ca-n povesti,', 'A fost ca niciodatxc3xa3,',
'Din rude mxc3xa3ri xc3xaempxc3xa3rxc3xa3testi,', 'O prea
frumoasxc3xa3 fatxc3xa3.']
A fost odatã ca-n povesti, A fost ca niciodatã, Din rude mãri
împãrãtesti, O prea frumoasã fatã.
O prea frumoasã fatã.
add a comment |
To read a file into a list you need to do three things:
- Open the file
- Read the file
- Store the contents as list
Fortunately Python makes it very easy to do these things so the shortest way to read a file into a list is:
lst = list(open(filename))
However I'll add some more explanation.
Opening the file
I assume that you want to open a specific file and you don't deal directly with a file-handle (or a file-like-handle). The most commonly used function to open a file in Python is open
, it takes one mandatory argument and two optional ones in Python 2.7:
- Filename
- Mode
- Buffering (I'll ignore this argument in this answer)
The filename should be a string that represents the path to the file. For example:
open('afile') # opens the file named afile in the current working directory
open('adir/afile') # relative path (relative to the current working directory)
open('C:/users/aname/afile') # absolute path (windows)
open('/usr/local/afile') # absolute path (linux)
Note that the file extension needs to be specified. This is especially important for Windows users because file extensions like .txt
or .doc
, etc. are hidden by default when viewed in the explorer.
The second argument is the mode
, it's r
by default which means "read-only". That's exactly what you need in your case.
But in case you actually want to create a file and/or write to a file you'll need a different argument here. There is an excellent answer if you want an overview.
For reading a file you can omit the mode
or pass it in explicitly:
open(filename)
open(filename, 'r')
Both will open the file in read-only mode. In case you want to read in a binary file on Windows you need to use the mode rb
:
open(filename, 'rb')
On other platforms the 'b'
(binary mode) is simply ignored.
Now that I've shown how to open
the file, let's talk about the fact that you always need to close
it again. Otherwise it will keep an open file-handle to the file until the process exits (or Python garbages the file-handle).
While you could use:
f = open(filename)
# ... do stuff with f
f.close()
That will fail to close the file when something between open
and close
throws an exception. You could avoid that by using a try
and finally
:
f = open(filename)
# nothing in between!
try:
# do stuff with f
finally:
f.close()
However Python provides context managers that have a prettier syntax (but for open
it's almost identical to the try
and finally
above):
with open(filename) as f:
# do stuff with f
# The file is always closed after the with-scope ends.
The last approach is the recommended approach to open a file in Python!
Reading the file
Okay, you've opened the file, now how to read it?
The open
function returns a file
object and it supports Pythons iteration protocol. Each iteration will give you a line:
with open(filename) as f:
for line in f:
print(line)
This will print each line of the file. Note however that each line will contain a newline character n
at the end (you might want to check if your Python is built with universal newlines support - otherwise you could also have rn
on Windows or r
on Mac as newlines). If you don't want that you can could simply remove the last character (or the last two characters on Windows):
with open(filename) as f:
for line in f:
print(line[:-1])
But the last line doesn't necessarily has a trailing newline, so one shouldn't use that. One could check if it ends with a trailing newline and if so remove it:
with open(filename) as f:
for line in f:
if line.endswith('n'):
line = line[:-1]
print(line)
But you could simply remove all whitespaces (including the n
character) from the end of the string, this will also remove all other trailing whitespaces so you have to be careful if these are important:
with open(filename) as f:
for line in f:
print(f.rstrip())
However if the lines end with rn
(Windows "newlines") that .rstrip()
will also take care of the r
!
Store the contents as list
Now that you know how to open the file and read it, it's time to store the contents in a list. The simplest option would be to use the list
function:
with open(filename) as f:
lst = list(f)
In case you want to strip the trailing newlines you could use a list comprehension instead:
with open(filename) as f:
lst = [line.rstrip() for line in f]
Or even simpler: The .readlines()
method of the file
object by default returns a list
of the lines:
with open(filename) as f:
lst = f.readlines()
This will also include the trailing newline characters, if you don't want them I would recommend the [line.rstrip() for line in f]
approach because it avoids keeping two lists containing all the lines in memory.
There's an additional option to get the desired output, however it's rather "suboptimal": read
the complete file in a string and then split on newlines:
with open(filename) as f:
lst = f.read().split('n')
or:
with open(filename) as f:
lst = f.read().splitlines()
These take care of the trailing newlines automatically because the split
character isn't included. However they are not ideal because you keep the file as string and as a list of lines in memory!
Summary
- Use
with open(...) as f
when opening files because you don't need to take care of closing the file yourself and it closes the file even if some exception happens.
file
objects support the iteration protocol so reading a file line-by-line is as simple asfor line in the_file_object:
.- Always browse the documentation for the available functions/classes. Most of the time there's a perfect match for the task or at least one or two good ones. The obvious choice in this case would be
readlines()
but if you want to process the lines before storing them in the list I would recommend a simple list-comprehension.
Excellent answer!
– Peter Zhao
Jan 7 at 8:52
add a comment |
I'd do it like this.
lines =
with open("myfile.txt") as f:
for line in f:
lines.append(line)
We already have 2 answers like this, posted 3 and 6 years before yours...
– Aran-Fey
Oct 27 '18 at 15:26
add a comment |
Here's one more option by using list comprehensions on files;
lines = [line.rstrip() for line in open('file.txt')]
This should be more efficient way as the most of the work is done inside the Python interpreter.
7
rstrip()
potentially strips all trailing whitespace, not just then
; use.rstrip('n')
.
– mklement0
May 22 '15 at 16:39
add a comment |
Another option is numpy.genfromtxt
, for example:
import numpy as np
data = np.genfromtxt("yourfile.dat",delimiter="n")
This will make data
a NumPy array with as many rows as are in your file.
add a comment |
If you'd like to read a file from the command line or from stdin, you can also use the fileinput
module:
# reader.py
import fileinput
content =
for line in fileinput.input():
content.append(line.strip())
fileinput.close()
Pass files to it like so:
$ python reader.py textfile.txt
Read more here: http://docs.python.org/2/library/fileinput.html
add a comment |
The simplest way to do it
A simple way is to:
- Read the whole file as a string
- Split the string line by line
In one line, that would give:
lines = open('C:/path/file.txt').read().splitlines()
add a comment |
Read and write text files with Python 2 and Python 3; it works with Unicode
#!/usr/bin/env python3
# -*- coding: utf-8 -*-
# Define data
lines = [' A first string ',
'A Unicode sample: €',
'German: äöüß']
# Write text file
with open('file.txt', 'w') as fp:
fp.write('n'.join(lines))
# Read text file
with open('file.txt', 'r') as fp:
read_lines = fp.readlines()
read_lines = [line.rstrip('n') for line in read_lines]
print(lines == read_lines)
Things to notice:
with
is a so-called context manager. It makes sure that the opened file is closed again.- All solutions here which simply make
.strip()
or.rstrip()
will fail to reproduce thelines
as they also strip the white space.
Common file endings
.txt
More advanced file writing / reading
- CSV: Super simple format (read & write)
- JSON: Nice for writing human-readable data; VERY commonly used (read & write)
- YAML: YAML is a superset of JSON, but easier to read (read & write, comparison of JSON and YAML)
- pickle: A Python serialization format (read & write)
MessagePack (Python package): More compact representation (read & write)
HDF5 (Python package): Nice for matrices (read & write)- XML: exists too *sigh* (read & write)
For your application, the following might be important:
- Support by other programming languages
- Reading / writing performance
- Compactness (file size)
See also: Comparison of data serialization formats
In case you are rather looking for a way to make configuration files, you might want to read my short article Configuration files in Python.
add a comment |
Introduced in Python 3.4, pathlib
has a really convenient method for reading in text from files, as follows:
from pathlib import Path
p = Path('my_text_file')
lines = p.read_text().splitlines()
(The splitlines
call is what turns it from a string containing the whole contents of the file to a list of lines in the file).
pathlib
has a lot of handy conveniences in it. read_text
is nice and concise, and you don't have to worry about opening and closing the file. If all you need to do with the file is read it all in in one go, it's a good choice.
add a comment |
f = open("your_file.txt",'r')
out = f.readlines() # will append in the list out
Now variable out is a list (array) of what you want. You could either do:
for line in out:
print line
or
for line in f:
print line
you'll get the same results.
add a comment |
Just use the splitlines() functions. Here is an example.
inp = "file.txt"
data = open(inp)
dat = data.read()
lst = dat.splitlines()
print lst
# print(lst) # for python 3
In the output you will have the list of lines.
add a comment |
A real easy way:
with open(file) as g:
stuff = g.readlines()
If you want to make it a fully-fledged program, type this in:
file = raw_input ("Enter EXACT file name: ")
with open(file) as g:
stuff = g.readlines()
print (stuff)
exit = raw_input("Press enter when you are done.")
For some reason, it doesn't read .py files properly.
add a comment |
You can just open your file for reading using:
file1 = open("filename","r")
# And for reading use
lines = file1.readlines()
file1.close()
The list lines
will contain all your lines as individual elements, and you can call a specific element using lines["linenumber-1"]
as Python starts its counting from 0.
1
The Pythonic way would be:with open("filename") as f: lines = f.readlines()
.
– Eric O Lebigot
Oct 23 '18 at 10:55
add a comment |
If you want to are faced with a very large / huge file and want to read faster (imagine you are in a Topcoder/Hackerrank coding competition), you might read a considerably bigger chunk of lines into a memory buffer at one time, rather than just iterate line by line at file level.
buffersize = 2**16
with open(path) as f:
while True:
lines_buffer = f.readlines(buffersize)
if not lines_buffer:
break
for line in lines_buffer:
process(line)
what does process(line) do? I get an error that there is not such variable defined. I guess something needs importing and I tried to import multiprocessing.Process, but that's not it I guess. Could you please elaborate? Thanks
– Newskooler
Apr 6 '17 at 8:40
1
process(line)
is a function that you need to implement to process the data. for example, instead of that line, if you useprint(line)
, it will print each line from the lines_buffer.
– Khanal
Apr 26 '17 at 13:27
f.readlines(buffersize) returns an immutable buffer. if you want to directly read into your buffer you need to use readinto() function. I will be much faster.
– David Dehghan
Jun 30 '18 at 10:28
add a comment |
To my knowledge Python doesn't have a native array data structure. But it does support the list data structure which is much simpler to use than an array.
array = #declaring a list with name '**array**'
with open(PATH,'r') as reader :
for line in reader :
array.append(line)
python does have an array (see the standard library'sarray
module), but the question asked for a list.
– Corey Goldberg
Dec 12 '16 at 23:22
add a comment |
Use this:
import pandas as pd
data = pd.read_csv(filename) # You can also add parameters such as header, sep, etc.
array = data.values
data
is a dataframe type, and uses values to get ndarray. You can also get a list by using array.tolist()
.
add a comment |
You can easily do it by the following piece of code:
lines = open(filePath).readlines()
2
You should use awith
statement though.
– Aran-Fey
Oct 29 '18 at 17:51
add a comment |
You could also use the loadtxt command in NumPy. This checks for fewer conditions than genfromtxt, so it may be faster.
import numpy
data = numpy.loadtxt(filename, delimiter="n")
add a comment |
Check out this short snippet
fileOb=open("filename.txt","r")
data=fileOb.readlines() #returns a array of lines.
or
fileOb=open("filename.txt","r")
data=list(fileOb) #returns a array of lines.
refer docs for reference
add a comment |
Outline and Summary
With a filename
, handling the file from a Path(filename)
object, or directly with open(filename) as f
, do one of the following:
list(fileinput.input(filename))
- using
with path.open() as f
, callf.readlines()
list(f)
path.read_text().splitlines()
path.read_text().splitlines(keepends=True)
- iterate over
fileinput.input
orf
andlist.append
each line one at a time - pass
f
to a boundlist.extend
method - use
f
in a list comprehension
I explain the use-case for each below.
In Python, how do I read a file line-by-line?
This is an excellent question. First, let's create some sample data:
from pathlib import Path
Path('filename').write_text('foonbarnbaz')
File objects are lazy iterators, so just iterate over it.
filename = 'filename'
with open(filename) as f:
for line in f:
line # do something with the line
Alternatively, if you have multiple files, use fileinput.input
, another lazy iterator. With just one file:
import fileinput
for line in fileinput.input(filename):
line # process the line
or for multiple files, pass it a list of filenames:
for line in fileinput.input([filename]*2):
line # process the line
Again, f
and fileinput.input
above both are/return lazy iterators.
You can only use an iterator one time, so to provide functional code while avoiding verbosity I'll use the slightly more terse fileinput.input(filename)
where apropos from here.
In Python, how do I read a file line-by-line into a list?
Ah but you want it in a list for some reason? I'd avoid that if possible. But if you insist... just pass the result of fileinput.input(filename)
to list
:
list(fileinput.input(filename))
Another direct answer is to call f.readlines
, which returns the contents of the file (up to an optional hint
number of characters, so you could break this up into multiple lists that way).
You can get to this file object two ways. One way is to pass the filename to the open
builtin:
filename = 'filename'
with open(filename) as f:
f.readlines()
or using the new Path object from the pathlib
module (which I have become quite fond of, and will use from here on):
from pathlib import Path
path = Path(filename)
with path.open() as f:
f.readlines()
list
will also consume the file iterator and return a list - a quite direct method as well:
with path.open() as f:
list(f)
If you don't mind reading the entire text into memory as a single string before splitting it, you can do this as a one-liner with the Path
object and the splitlines()
string method. By default, splitlines
removes the newlines:
path.read_text().splitlines()
If you want to keep the newlines, pass keepends=True
:
path.read_text().splitlines(keepends=True)
I want to read the file line by line and append each line to the end of the list.
Now this is a bit silly to ask for, given that we've demonstrated the end result easily with several methods. But you might need to filter or operate on the lines as you make your list, so let's humor this request.
Using list.append
would allow you to filter or operate on each line before you append it:
line_list =
for line in fileinput.input(filename):
line_list.append(line)
line_list
Using list.extend
would be a bit more direct, and perhaps useful if you have a preexisting list:
line_list =
line_list.extend(fileinput.input(filename))
line_list
Or more idiomatically, we could instead use a list comprehension, and map and filter inside it if desirable:
[line for line in fileinput.input(filename)]
Or even more directly, to close the circle, just pass it to list to create a new list directly without operating on the lines:
list(fileinput.input(filename))
Conclusion
You've seen many ways to get lines from a file into a list, but I'd recommend you avoid materializing large quantities of data into a list and instead use Python's lazy iteration to process the data if possible.
That is, prefer fileinput.input
or with path.open() as f
.
add a comment |
Command line version
#!/bin/python3
import os
import sys
abspath = os.path.abspath(__file__)
dname = os.path.dirname(abspath)
filename = dname + sys.argv[1]
arr = open(filename).read().split("n")
print(arr)
Run with:
python3 somefile.py input_file_name.txt
add a comment |
1 2
next
33 Answers
33
active
oldest
votes
33 Answers
33
active
oldest
votes
active
oldest
votes
active
oldest
votes
1 2
next
with open(fname) as f:
content = f.readlines()
# you may also want to remove whitespace characters like `n` at the end of each line
content = [x.strip() for x in content]
139
Don't usefile.readlines()
in afor
-loop, a file object itself is enough:lines = [line.rstrip('n') for line in file]
– jfs
Jan 14 '15 at 10:52
50
In the case you are working with Big Data usingreadlines()
is not very efficient as it can result in MemoryError. In this case it is better to iterate over the file usingfor line in f:
and working with eachline
variable.
– DarkCygnus
Aug 27 '16 at 3:07
3
I checked the memory profile of different ways given in the answers using the procedure mentioned here. The memory usage is far better when each line is read from the file and processed, as suggested by @DevShark here. Holding all lines in a collection object is not a good idea if memory is a constraint or the file is large. The execution time is similar in both the approaches.
– Tirtha R
Mar 2 '18 at 23:24
2
Also,.rstrip()
will work slightly faster if you are stripping whitespace from the ends of lines.
– Gringo Suave
Jun 15 '18 at 19:14
add a comment |
with open(fname) as f:
content = f.readlines()
# you may also want to remove whitespace characters like `n` at the end of each line
content = [x.strip() for x in content]
139
Don't usefile.readlines()
in afor
-loop, a file object itself is enough:lines = [line.rstrip('n') for line in file]
– jfs
Jan 14 '15 at 10:52
50
In the case you are working with Big Data usingreadlines()
is not very efficient as it can result in MemoryError. In this case it is better to iterate over the file usingfor line in f:
and working with eachline
variable.
– DarkCygnus
Aug 27 '16 at 3:07
3
I checked the memory profile of different ways given in the answers using the procedure mentioned here. The memory usage is far better when each line is read from the file and processed, as suggested by @DevShark here. Holding all lines in a collection object is not a good idea if memory is a constraint or the file is large. The execution time is similar in both the approaches.
– Tirtha R
Mar 2 '18 at 23:24
2
Also,.rstrip()
will work slightly faster if you are stripping whitespace from the ends of lines.
– Gringo Suave
Jun 15 '18 at 19:14
add a comment |
with open(fname) as f:
content = f.readlines()
# you may also want to remove whitespace characters like `n` at the end of each line
content = [x.strip() for x in content]
with open(fname) as f:
content = f.readlines()
# you may also want to remove whitespace characters like `n` at the end of each line
content = [x.strip() for x in content]
edited Jul 18 '18 at 6:49
bluish
14k1694148
14k1694148
answered Jul 18 '10 at 22:28
SilentGhostSilentGhost
195k47266263
195k47266263
139
Don't usefile.readlines()
in afor
-loop, a file object itself is enough:lines = [line.rstrip('n') for line in file]
– jfs
Jan 14 '15 at 10:52
50
In the case you are working with Big Data usingreadlines()
is not very efficient as it can result in MemoryError. In this case it is better to iterate over the file usingfor line in f:
and working with eachline
variable.
– DarkCygnus
Aug 27 '16 at 3:07
3
I checked the memory profile of different ways given in the answers using the procedure mentioned here. The memory usage is far better when each line is read from the file and processed, as suggested by @DevShark here. Holding all lines in a collection object is not a good idea if memory is a constraint or the file is large. The execution time is similar in both the approaches.
– Tirtha R
Mar 2 '18 at 23:24
2
Also,.rstrip()
will work slightly faster if you are stripping whitespace from the ends of lines.
– Gringo Suave
Jun 15 '18 at 19:14
add a comment |
139
Don't usefile.readlines()
in afor
-loop, a file object itself is enough:lines = [line.rstrip('n') for line in file]
– jfs
Jan 14 '15 at 10:52
50
In the case you are working with Big Data usingreadlines()
is not very efficient as it can result in MemoryError. In this case it is better to iterate over the file usingfor line in f:
and working with eachline
variable.
– DarkCygnus
Aug 27 '16 at 3:07
3
I checked the memory profile of different ways given in the answers using the procedure mentioned here. The memory usage is far better when each line is read from the file and processed, as suggested by @DevShark here. Holding all lines in a collection object is not a good idea if memory is a constraint or the file is large. The execution time is similar in both the approaches.
– Tirtha R
Mar 2 '18 at 23:24
2
Also,.rstrip()
will work slightly faster if you are stripping whitespace from the ends of lines.
– Gringo Suave
Jun 15 '18 at 19:14
139
139
Don't use
file.readlines()
in a for
-loop, a file object itself is enough: lines = [line.rstrip('n') for line in file]
– jfs
Jan 14 '15 at 10:52
Don't use
file.readlines()
in a for
-loop, a file object itself is enough: lines = [line.rstrip('n') for line in file]
– jfs
Jan 14 '15 at 10:52
50
50
In the case you are working with Big Data using
readlines()
is not very efficient as it can result in MemoryError. In this case it is better to iterate over the file using for line in f:
and working with each line
variable.– DarkCygnus
Aug 27 '16 at 3:07
In the case you are working with Big Data using
readlines()
is not very efficient as it can result in MemoryError. In this case it is better to iterate over the file using for line in f:
and working with each line
variable.– DarkCygnus
Aug 27 '16 at 3:07
3
3
I checked the memory profile of different ways given in the answers using the procedure mentioned here. The memory usage is far better when each line is read from the file and processed, as suggested by @DevShark here. Holding all lines in a collection object is not a good idea if memory is a constraint or the file is large. The execution time is similar in both the approaches.
– Tirtha R
Mar 2 '18 at 23:24
I checked the memory profile of different ways given in the answers using the procedure mentioned here. The memory usage is far better when each line is read from the file and processed, as suggested by @DevShark here. Holding all lines in a collection object is not a good idea if memory is a constraint or the file is large. The execution time is similar in both the approaches.
– Tirtha R
Mar 2 '18 at 23:24
2
2
Also,
.rstrip()
will work slightly faster if you are stripping whitespace from the ends of lines.– Gringo Suave
Jun 15 '18 at 19:14
Also,
.rstrip()
will work slightly faster if you are stripping whitespace from the ends of lines.– Gringo Suave
Jun 15 '18 at 19:14
add a comment |
See Input and Ouput:
with open('filename') as f:
lines = f.readlines()
or with stripping the newline character:
lines = [line.rstrip('n') for line in open('filename')]
Editor's note: This answer's original whitespace-stripping command, line.strip()
, as implied by Janus Troelsen's comment, would remove all leading and trailing whitespace, not just the trailing n
.
67
if you only want to discard the newline:lines = (line.rstrip('n') for line in open(filename))
– Janus Troelsen
Oct 11 '12 at 10:14
14
For a list it should belines = [line.rstrip('n') for line in open(filename)]
– Lazik
Oct 12 '13 at 14:32
25
Won't the 2nd option leave the file open (since it's not guarded by a context on its own)?
– yo'
Feb 8 '15 at 19:14
9
@yo' It does, but most people do not care about that in small programs. There is no harm in small programs since the leaked file object are garbage collected, but it is not a good habit to do this.
– Martin Ueding
May 4 '15 at 8:01
17
Safer:with open('filename') as f: lines = [line.rstrip('n') for line in f]
– becko
Feb 10 '16 at 16:36
|
show 7 more comments
See Input and Ouput:
with open('filename') as f:
lines = f.readlines()
or with stripping the newline character:
lines = [line.rstrip('n') for line in open('filename')]
Editor's note: This answer's original whitespace-stripping command, line.strip()
, as implied by Janus Troelsen's comment, would remove all leading and trailing whitespace, not just the trailing n
.
67
if you only want to discard the newline:lines = (line.rstrip('n') for line in open(filename))
– Janus Troelsen
Oct 11 '12 at 10:14
14
For a list it should belines = [line.rstrip('n') for line in open(filename)]
– Lazik
Oct 12 '13 at 14:32
25
Won't the 2nd option leave the file open (since it's not guarded by a context on its own)?
– yo'
Feb 8 '15 at 19:14
9
@yo' It does, but most people do not care about that in small programs. There is no harm in small programs since the leaked file object are garbage collected, but it is not a good habit to do this.
– Martin Ueding
May 4 '15 at 8:01
17
Safer:with open('filename') as f: lines = [line.rstrip('n') for line in f]
– becko
Feb 10 '16 at 16:36
|
show 7 more comments
See Input and Ouput:
with open('filename') as f:
lines = f.readlines()
or with stripping the newline character:
lines = [line.rstrip('n') for line in open('filename')]
Editor's note: This answer's original whitespace-stripping command, line.strip()
, as implied by Janus Troelsen's comment, would remove all leading and trailing whitespace, not just the trailing n
.
See Input and Ouput:
with open('filename') as f:
lines = f.readlines()
or with stripping the newline character:
lines = [line.rstrip('n') for line in open('filename')]
Editor's note: This answer's original whitespace-stripping command, line.strip()
, as implied by Janus Troelsen's comment, would remove all leading and trailing whitespace, not just the trailing n
.
edited May 22 '15 at 16:44
mklement0
131k20245282
131k20245282
answered Jul 18 '10 at 22:28


Felix KlingFelix Kling
554k128862919
554k128862919
67
if you only want to discard the newline:lines = (line.rstrip('n') for line in open(filename))
– Janus Troelsen
Oct 11 '12 at 10:14
14
For a list it should belines = [line.rstrip('n') for line in open(filename)]
– Lazik
Oct 12 '13 at 14:32
25
Won't the 2nd option leave the file open (since it's not guarded by a context on its own)?
– yo'
Feb 8 '15 at 19:14
9
@yo' It does, but most people do not care about that in small programs. There is no harm in small programs since the leaked file object are garbage collected, but it is not a good habit to do this.
– Martin Ueding
May 4 '15 at 8:01
17
Safer:with open('filename') as f: lines = [line.rstrip('n') for line in f]
– becko
Feb 10 '16 at 16:36
|
show 7 more comments
67
if you only want to discard the newline:lines = (line.rstrip('n') for line in open(filename))
– Janus Troelsen
Oct 11 '12 at 10:14
14
For a list it should belines = [line.rstrip('n') for line in open(filename)]
– Lazik
Oct 12 '13 at 14:32
25
Won't the 2nd option leave the file open (since it's not guarded by a context on its own)?
– yo'
Feb 8 '15 at 19:14
9
@yo' It does, but most people do not care about that in small programs. There is no harm in small programs since the leaked file object are garbage collected, but it is not a good habit to do this.
– Martin Ueding
May 4 '15 at 8:01
17
Safer:with open('filename') as f: lines = [line.rstrip('n') for line in f]
– becko
Feb 10 '16 at 16:36
67
67
if you only want to discard the newline:
lines = (line.rstrip('n') for line in open(filename))
– Janus Troelsen
Oct 11 '12 at 10:14
if you only want to discard the newline:
lines = (line.rstrip('n') for line in open(filename))
– Janus Troelsen
Oct 11 '12 at 10:14
14
14
For a list it should be
lines = [line.rstrip('n') for line in open(filename)]
– Lazik
Oct 12 '13 at 14:32
For a list it should be
lines = [line.rstrip('n') for line in open(filename)]
– Lazik
Oct 12 '13 at 14:32
25
25
Won't the 2nd option leave the file open (since it's not guarded by a context on its own)?
– yo'
Feb 8 '15 at 19:14
Won't the 2nd option leave the file open (since it's not guarded by a context on its own)?
– yo'
Feb 8 '15 at 19:14
9
9
@yo' It does, but most people do not care about that in small programs. There is no harm in small programs since the leaked file object are garbage collected, but it is not a good habit to do this.
– Martin Ueding
May 4 '15 at 8:01
@yo' It does, but most people do not care about that in small programs. There is no harm in small programs since the leaked file object are garbage collected, but it is not a good habit to do this.
– Martin Ueding
May 4 '15 at 8:01
17
17
Safer:
with open('filename') as f: lines = [line.rstrip('n') for line in f]
– becko
Feb 10 '16 at 16:36
Safer:
with open('filename') as f: lines = [line.rstrip('n') for line in f]
– becko
Feb 10 '16 at 16:36
|
show 7 more comments
This is more explicit than necessary, but does what you want.
with open("file.txt", "r") as ins:
array =
for line in ins:
array.append(line)
8
This is a direct answer to the question
– Joop
Jul 14 '18 at 9:15
7
I prefer this answer since it doesn't require to load the whole file into memory (in this case it is still appended toarray
though, but there might be other circumstances). Certainly for big files this approach might mitigate problems.
– JohannesB
Sep 19 '18 at 12:44
Appending to an array is slow. I cannot think of a use case where this is the best solution.
– Elias Strehle
Oct 4 '18 at 12:48
1
This answer received less votes than it deserve (and do not deserve downvotes). It is much better than usingreadlines
.
– haccks
Oct 20 '18 at 20:12
@haccks is it better because it doesn't load the whole file to memory or is there more?
– OrigamiEye
Dec 1 '18 at 15:39
|
show 2 more comments
This is more explicit than necessary, but does what you want.
with open("file.txt", "r") as ins:
array =
for line in ins:
array.append(line)
8
This is a direct answer to the question
– Joop
Jul 14 '18 at 9:15
7
I prefer this answer since it doesn't require to load the whole file into memory (in this case it is still appended toarray
though, but there might be other circumstances). Certainly for big files this approach might mitigate problems.
– JohannesB
Sep 19 '18 at 12:44
Appending to an array is slow. I cannot think of a use case where this is the best solution.
– Elias Strehle
Oct 4 '18 at 12:48
1
This answer received less votes than it deserve (and do not deserve downvotes). It is much better than usingreadlines
.
– haccks
Oct 20 '18 at 20:12
@haccks is it better because it doesn't load the whole file to memory or is there more?
– OrigamiEye
Dec 1 '18 at 15:39
|
show 2 more comments
This is more explicit than necessary, but does what you want.
with open("file.txt", "r") as ins:
array =
for line in ins:
array.append(line)
This is more explicit than necessary, but does what you want.
with open("file.txt", "r") as ins:
array =
for line in ins:
array.append(line)
edited Jan 8 '15 at 8:50
Martin Thoma
42.8k59303525
42.8k59303525
answered Jul 18 '10 at 22:27
robertrobert
22.7k84668
22.7k84668
8
This is a direct answer to the question
– Joop
Jul 14 '18 at 9:15
7
I prefer this answer since it doesn't require to load the whole file into memory (in this case it is still appended toarray
though, but there might be other circumstances). Certainly for big files this approach might mitigate problems.
– JohannesB
Sep 19 '18 at 12:44
Appending to an array is slow. I cannot think of a use case where this is the best solution.
– Elias Strehle
Oct 4 '18 at 12:48
1
This answer received less votes than it deserve (and do not deserve downvotes). It is much better than usingreadlines
.
– haccks
Oct 20 '18 at 20:12
@haccks is it better because it doesn't load the whole file to memory or is there more?
– OrigamiEye
Dec 1 '18 at 15:39
|
show 2 more comments
8
This is a direct answer to the question
– Joop
Jul 14 '18 at 9:15
7
I prefer this answer since it doesn't require to load the whole file into memory (in this case it is still appended toarray
though, but there might be other circumstances). Certainly for big files this approach might mitigate problems.
– JohannesB
Sep 19 '18 at 12:44
Appending to an array is slow. I cannot think of a use case where this is the best solution.
– Elias Strehle
Oct 4 '18 at 12:48
1
This answer received less votes than it deserve (and do not deserve downvotes). It is much better than usingreadlines
.
– haccks
Oct 20 '18 at 20:12
@haccks is it better because it doesn't load the whole file to memory or is there more?
– OrigamiEye
Dec 1 '18 at 15:39
8
8
This is a direct answer to the question
– Joop
Jul 14 '18 at 9:15
This is a direct answer to the question
– Joop
Jul 14 '18 at 9:15
7
7
I prefer this answer since it doesn't require to load the whole file into memory (in this case it is still appended to
array
though, but there might be other circumstances). Certainly for big files this approach might mitigate problems.– JohannesB
Sep 19 '18 at 12:44
I prefer this answer since it doesn't require to load the whole file into memory (in this case it is still appended to
array
though, but there might be other circumstances). Certainly for big files this approach might mitigate problems.– JohannesB
Sep 19 '18 at 12:44
Appending to an array is slow. I cannot think of a use case where this is the best solution.
– Elias Strehle
Oct 4 '18 at 12:48
Appending to an array is slow. I cannot think of a use case where this is the best solution.
– Elias Strehle
Oct 4 '18 at 12:48
1
1
This answer received less votes than it deserve (and do not deserve downvotes). It is much better than using
readlines
.– haccks
Oct 20 '18 at 20:12
This answer received less votes than it deserve (and do not deserve downvotes). It is much better than using
readlines
.– haccks
Oct 20 '18 at 20:12
@haccks is it better because it doesn't load the whole file to memory or is there more?
– OrigamiEye
Dec 1 '18 at 15:39
@haccks is it better because it doesn't load the whole file to memory or is there more?
– OrigamiEye
Dec 1 '18 at 15:39
|
show 2 more comments
This will yield an "array" of lines from the file.
lines = tuple(open(filename, 'r'))
37
open
returns a file which can be iterated over. When you iterate over a file, you get the lines from that file.tuple
can take an iterator and instantiate a tuple instance for you from the iterator that you give it.lines
is a tuple created from the lines of the file.
– Noctis Skytower
Jan 5 '14 at 21:58
24
@MarshallFarrier Trylines = open(filename).read().split('n')
instead.
– Noctis Skytower
Dec 11 '14 at 13:56
10
does it close the file?
– Vanuan
Jan 3 '15 at 2:21
24
@NoctisSkytower I findlines = open(filename).read().splitlines()
a little cleaner, and I believe it also handles DOS line endings better.
– jaynp
May 13 '15 at 5:59
6
@mklement0 Assuming a file of 1000 lines, alist
takes up about 13.22% more space than atuple
. Results come fromfrom sys import getsizeof as g; i = [None] * 1000; round((g(list(i)) / g(tuple(i)) - 1) * 100, 2)
. Creating atuple
takes about 4.17% more time than creating alist
(with a 0.16% standard deviation). Results come from runningfrom timeit import timeit as t; round((t('tuple(i)', 'i = [None] * 1000') / t('list(i)', 'i = [None] * 1000') - 1) * 100, 2)
30 times. My solution favors space over speed when the need for mutability is unknown.
– Noctis Skytower
Jan 4 '16 at 16:17
|
show 4 more comments
This will yield an "array" of lines from the file.
lines = tuple(open(filename, 'r'))
37
open
returns a file which can be iterated over. When you iterate over a file, you get the lines from that file.tuple
can take an iterator and instantiate a tuple instance for you from the iterator that you give it.lines
is a tuple created from the lines of the file.
– Noctis Skytower
Jan 5 '14 at 21:58
24
@MarshallFarrier Trylines = open(filename).read().split('n')
instead.
– Noctis Skytower
Dec 11 '14 at 13:56
10
does it close the file?
– Vanuan
Jan 3 '15 at 2:21
24
@NoctisSkytower I findlines = open(filename).read().splitlines()
a little cleaner, and I believe it also handles DOS line endings better.
– jaynp
May 13 '15 at 5:59
6
@mklement0 Assuming a file of 1000 lines, alist
takes up about 13.22% more space than atuple
. Results come fromfrom sys import getsizeof as g; i = [None] * 1000; round((g(list(i)) / g(tuple(i)) - 1) * 100, 2)
. Creating atuple
takes about 4.17% more time than creating alist
(with a 0.16% standard deviation). Results come from runningfrom timeit import timeit as t; round((t('tuple(i)', 'i = [None] * 1000') / t('list(i)', 'i = [None] * 1000') - 1) * 100, 2)
30 times. My solution favors space over speed when the need for mutability is unknown.
– Noctis Skytower
Jan 4 '16 at 16:17
|
show 4 more comments
This will yield an "array" of lines from the file.
lines = tuple(open(filename, 'r'))
This will yield an "array" of lines from the file.
lines = tuple(open(filename, 'r'))
answered Jul 18 '10 at 22:27
Noctis SkytowerNoctis Skytower
13.7k116088
13.7k116088
37
open
returns a file which can be iterated over. When you iterate over a file, you get the lines from that file.tuple
can take an iterator and instantiate a tuple instance for you from the iterator that you give it.lines
is a tuple created from the lines of the file.
– Noctis Skytower
Jan 5 '14 at 21:58
24
@MarshallFarrier Trylines = open(filename).read().split('n')
instead.
– Noctis Skytower
Dec 11 '14 at 13:56
10
does it close the file?
– Vanuan
Jan 3 '15 at 2:21
24
@NoctisSkytower I findlines = open(filename).read().splitlines()
a little cleaner, and I believe it also handles DOS line endings better.
– jaynp
May 13 '15 at 5:59
6
@mklement0 Assuming a file of 1000 lines, alist
takes up about 13.22% more space than atuple
. Results come fromfrom sys import getsizeof as g; i = [None] * 1000; round((g(list(i)) / g(tuple(i)) - 1) * 100, 2)
. Creating atuple
takes about 4.17% more time than creating alist
(with a 0.16% standard deviation). Results come from runningfrom timeit import timeit as t; round((t('tuple(i)', 'i = [None] * 1000') / t('list(i)', 'i = [None] * 1000') - 1) * 100, 2)
30 times. My solution favors space over speed when the need for mutability is unknown.
– Noctis Skytower
Jan 4 '16 at 16:17
|
show 4 more comments
37
open
returns a file which can be iterated over. When you iterate over a file, you get the lines from that file.tuple
can take an iterator and instantiate a tuple instance for you from the iterator that you give it.lines
is a tuple created from the lines of the file.
– Noctis Skytower
Jan 5 '14 at 21:58
24
@MarshallFarrier Trylines = open(filename).read().split('n')
instead.
– Noctis Skytower
Dec 11 '14 at 13:56
10
does it close the file?
– Vanuan
Jan 3 '15 at 2:21
24
@NoctisSkytower I findlines = open(filename).read().splitlines()
a little cleaner, and I believe it also handles DOS line endings better.
– jaynp
May 13 '15 at 5:59
6
@mklement0 Assuming a file of 1000 lines, alist
takes up about 13.22% more space than atuple
. Results come fromfrom sys import getsizeof as g; i = [None] * 1000; round((g(list(i)) / g(tuple(i)) - 1) * 100, 2)
. Creating atuple
takes about 4.17% more time than creating alist
(with a 0.16% standard deviation). Results come from runningfrom timeit import timeit as t; round((t('tuple(i)', 'i = [None] * 1000') / t('list(i)', 'i = [None] * 1000') - 1) * 100, 2)
30 times. My solution favors space over speed when the need for mutability is unknown.
– Noctis Skytower
Jan 4 '16 at 16:17
37
37
open
returns a file which can be iterated over. When you iterate over a file, you get the lines from that file. tuple
can take an iterator and instantiate a tuple instance for you from the iterator that you give it. lines
is a tuple created from the lines of the file.– Noctis Skytower
Jan 5 '14 at 21:58
open
returns a file which can be iterated over. When you iterate over a file, you get the lines from that file. tuple
can take an iterator and instantiate a tuple instance for you from the iterator that you give it. lines
is a tuple created from the lines of the file.– Noctis Skytower
Jan 5 '14 at 21:58
24
24
@MarshallFarrier Try
lines = open(filename).read().split('n')
instead.– Noctis Skytower
Dec 11 '14 at 13:56
@MarshallFarrier Try
lines = open(filename).read().split('n')
instead.– Noctis Skytower
Dec 11 '14 at 13:56
10
10
does it close the file?
– Vanuan
Jan 3 '15 at 2:21
does it close the file?
– Vanuan
Jan 3 '15 at 2:21
24
24
@NoctisSkytower I find
lines = open(filename).read().splitlines()
a little cleaner, and I believe it also handles DOS line endings better.– jaynp
May 13 '15 at 5:59
@NoctisSkytower I find
lines = open(filename).read().splitlines()
a little cleaner, and I believe it also handles DOS line endings better.– jaynp
May 13 '15 at 5:59
6
6
@mklement0 Assuming a file of 1000 lines, a
list
takes up about 13.22% more space than a tuple
. Results come from from sys import getsizeof as g; i = [None] * 1000; round((g(list(i)) / g(tuple(i)) - 1) * 100, 2)
. Creating a tuple
takes about 4.17% more time than creating a list
(with a 0.16% standard deviation). Results come from running from timeit import timeit as t; round((t('tuple(i)', 'i = [None] * 1000') / t('list(i)', 'i = [None] * 1000') - 1) * 100, 2)
30 times. My solution favors space over speed when the need for mutability is unknown.– Noctis Skytower
Jan 4 '16 at 16:17
@mklement0 Assuming a file of 1000 lines, a
list
takes up about 13.22% more space than a tuple
. Results come from from sys import getsizeof as g; i = [None] * 1000; round((g(list(i)) / g(tuple(i)) - 1) * 100, 2)
. Creating a tuple
takes about 4.17% more time than creating a list
(with a 0.16% standard deviation). Results come from running from timeit import timeit as t; round((t('tuple(i)', 'i = [None] * 1000') / t('list(i)', 'i = [None] * 1000') - 1) * 100, 2)
30 times. My solution favors space over speed when the need for mutability is unknown.– Noctis Skytower
Jan 4 '16 at 16:17
|
show 4 more comments
If you want the n
included:
with open(fname) as f:
content = f.readlines()
If you do not want n
included:
with open(fname) as f:
content = f.read().splitlines()
add a comment |
If you want the n
included:
with open(fname) as f:
content = f.readlines()
If you do not want n
included:
with open(fname) as f:
content = f.read().splitlines()
add a comment |
If you want the n
included:
with open(fname) as f:
content = f.readlines()
If you do not want n
included:
with open(fname) as f:
content = f.read().splitlines()
If you want the n
included:
with open(fname) as f:
content = f.readlines()
If you do not want n
included:
with open(fname) as f:
content = f.read().splitlines()
answered Mar 2 '14 at 4:22


Eneko AlonsoEneko Alonso
11.9k53755
11.9k53755
add a comment |
add a comment |
You could simply do the following, as has been suggested:
with open('/your/path/file') as f:
my_lines = f.readlines()
Note that this approach has 2 downsides:
1) You store all the lines in memory. In the general case, this is a very bad idea. The file could be very large, and you could run out of memory. Even if it's not large, it is simply a waste of memory.
2) This does not allow processing of each line as you read them. So if you process your lines after this, it is not efficient (requires two passes rather than one).
A better approach for the general case would be the following:
with open('/your/path/file') as f:
for line in f:
process(line)
Where you define your process function any way you want. For example:
def process(line):
if 'save the world' in line.lower():
superman.save_the_world()
(The implementation of the Superman
class is left as an exercise for you).
This will work nicely for any file size and you go through your file in just 1 pass. This is typically how generic parsers will work.
3
This was exactly what I needed - and thanks for explaining the downsides. As a beginner in Python, it's awesome to understand why a solution is the solution. Cheers!
– Ephexx
May 17 '16 at 21:37
3
Think a bit more Corey. Do you really ever want your computer to read each line, without ever doing anything with these lines? Surely you can realize you always need to process them one way or another.
– DevShark
Dec 13 '16 at 7:31
4
You always need to do something with the lines. It can be as simple as printing the lines, or counting them. There is no value in having your process read the lines in memory, but not doing anything with it.
– DevShark
Dec 14 '16 at 10:22
2
You always need to do something with them. I think the point you are trying to make is that you might want to apply a function to all of them at once, rather than one by one. That is indeed the case sometimes. But it is very inefficient from a memory standpoint to do so, and prevents you from reading files if its footprint is larger than your Ram. That's why typically generic parsers operate in the way I described.
– DevShark
Jun 23 '17 at 19:40
2
@PierreOcinom that is correct. Given that the file is opened in read only mode, you couldn't modify the original file with the code above. To open a file for both reading and writing, useopen('file_path', 'r+')
– DevShark
Sep 14 '17 at 9:17
|
show 8 more comments
You could simply do the following, as has been suggested:
with open('/your/path/file') as f:
my_lines = f.readlines()
Note that this approach has 2 downsides:
1) You store all the lines in memory. In the general case, this is a very bad idea. The file could be very large, and you could run out of memory. Even if it's not large, it is simply a waste of memory.
2) This does not allow processing of each line as you read them. So if you process your lines after this, it is not efficient (requires two passes rather than one).
A better approach for the general case would be the following:
with open('/your/path/file') as f:
for line in f:
process(line)
Where you define your process function any way you want. For example:
def process(line):
if 'save the world' in line.lower():
superman.save_the_world()
(The implementation of the Superman
class is left as an exercise for you).
This will work nicely for any file size and you go through your file in just 1 pass. This is typically how generic parsers will work.
3
This was exactly what I needed - and thanks for explaining the downsides. As a beginner in Python, it's awesome to understand why a solution is the solution. Cheers!
– Ephexx
May 17 '16 at 21:37
3
Think a bit more Corey. Do you really ever want your computer to read each line, without ever doing anything with these lines? Surely you can realize you always need to process them one way or another.
– DevShark
Dec 13 '16 at 7:31
4
You always need to do something with the lines. It can be as simple as printing the lines, or counting them. There is no value in having your process read the lines in memory, but not doing anything with it.
– DevShark
Dec 14 '16 at 10:22
2
You always need to do something with them. I think the point you are trying to make is that you might want to apply a function to all of them at once, rather than one by one. That is indeed the case sometimes. But it is very inefficient from a memory standpoint to do so, and prevents you from reading files if its footprint is larger than your Ram. That's why typically generic parsers operate in the way I described.
– DevShark
Jun 23 '17 at 19:40
2
@PierreOcinom that is correct. Given that the file is opened in read only mode, you couldn't modify the original file with the code above. To open a file for both reading and writing, useopen('file_path', 'r+')
– DevShark
Sep 14 '17 at 9:17
|
show 8 more comments
You could simply do the following, as has been suggested:
with open('/your/path/file') as f:
my_lines = f.readlines()
Note that this approach has 2 downsides:
1) You store all the lines in memory. In the general case, this is a very bad idea. The file could be very large, and you could run out of memory. Even if it's not large, it is simply a waste of memory.
2) This does not allow processing of each line as you read them. So if you process your lines after this, it is not efficient (requires two passes rather than one).
A better approach for the general case would be the following:
with open('/your/path/file') as f:
for line in f:
process(line)
Where you define your process function any way you want. For example:
def process(line):
if 'save the world' in line.lower():
superman.save_the_world()
(The implementation of the Superman
class is left as an exercise for you).
This will work nicely for any file size and you go through your file in just 1 pass. This is typically how generic parsers will work.
You could simply do the following, as has been suggested:
with open('/your/path/file') as f:
my_lines = f.readlines()
Note that this approach has 2 downsides:
1) You store all the lines in memory. In the general case, this is a very bad idea. The file could be very large, and you could run out of memory. Even if it's not large, it is simply a waste of memory.
2) This does not allow processing of each line as you read them. So if you process your lines after this, it is not efficient (requires two passes rather than one).
A better approach for the general case would be the following:
with open('/your/path/file') as f:
for line in f:
process(line)
Where you define your process function any way you want. For example:
def process(line):
if 'save the world' in line.lower():
superman.save_the_world()
(The implementation of the Superman
class is left as an exercise for you).
This will work nicely for any file size and you go through your file in just 1 pass. This is typically how generic parsers will work.
edited Aug 24 '16 at 18:04
answered Feb 25 '16 at 9:13


DevSharkDevShark
4,59451538
4,59451538
3
This was exactly what I needed - and thanks for explaining the downsides. As a beginner in Python, it's awesome to understand why a solution is the solution. Cheers!
– Ephexx
May 17 '16 at 21:37
3
Think a bit more Corey. Do you really ever want your computer to read each line, without ever doing anything with these lines? Surely you can realize you always need to process them one way or another.
– DevShark
Dec 13 '16 at 7:31
4
You always need to do something with the lines. It can be as simple as printing the lines, or counting them. There is no value in having your process read the lines in memory, but not doing anything with it.
– DevShark
Dec 14 '16 at 10:22
2
You always need to do something with them. I think the point you are trying to make is that you might want to apply a function to all of them at once, rather than one by one. That is indeed the case sometimes. But it is very inefficient from a memory standpoint to do so, and prevents you from reading files if its footprint is larger than your Ram. That's why typically generic parsers operate in the way I described.
– DevShark
Jun 23 '17 at 19:40
2
@PierreOcinom that is correct. Given that the file is opened in read only mode, you couldn't modify the original file with the code above. To open a file for both reading and writing, useopen('file_path', 'r+')
– DevShark
Sep 14 '17 at 9:17
|
show 8 more comments
3
This was exactly what I needed - and thanks for explaining the downsides. As a beginner in Python, it's awesome to understand why a solution is the solution. Cheers!
– Ephexx
May 17 '16 at 21:37
3
Think a bit more Corey. Do you really ever want your computer to read each line, without ever doing anything with these lines? Surely you can realize you always need to process them one way or another.
– DevShark
Dec 13 '16 at 7:31
4
You always need to do something with the lines. It can be as simple as printing the lines, or counting them. There is no value in having your process read the lines in memory, but not doing anything with it.
– DevShark
Dec 14 '16 at 10:22
2
You always need to do something with them. I think the point you are trying to make is that you might want to apply a function to all of them at once, rather than one by one. That is indeed the case sometimes. But it is very inefficient from a memory standpoint to do so, and prevents you from reading files if its footprint is larger than your Ram. That's why typically generic parsers operate in the way I described.
– DevShark
Jun 23 '17 at 19:40
2
@PierreOcinom that is correct. Given that the file is opened in read only mode, you couldn't modify the original file with the code above. To open a file for both reading and writing, useopen('file_path', 'r+')
– DevShark
Sep 14 '17 at 9:17
3
3
This was exactly what I needed - and thanks for explaining the downsides. As a beginner in Python, it's awesome to understand why a solution is the solution. Cheers!
– Ephexx
May 17 '16 at 21:37
This was exactly what I needed - and thanks for explaining the downsides. As a beginner in Python, it's awesome to understand why a solution is the solution. Cheers!
– Ephexx
May 17 '16 at 21:37
3
3
Think a bit more Corey. Do you really ever want your computer to read each line, without ever doing anything with these lines? Surely you can realize you always need to process them one way or another.
– DevShark
Dec 13 '16 at 7:31
Think a bit more Corey. Do you really ever want your computer to read each line, without ever doing anything with these lines? Surely you can realize you always need to process them one way or another.
– DevShark
Dec 13 '16 at 7:31
4
4
You always need to do something with the lines. It can be as simple as printing the lines, or counting them. There is no value in having your process read the lines in memory, but not doing anything with it.
– DevShark
Dec 14 '16 at 10:22
You always need to do something with the lines. It can be as simple as printing the lines, or counting them. There is no value in having your process read the lines in memory, but not doing anything with it.
– DevShark
Dec 14 '16 at 10:22
2
2
You always need to do something with them. I think the point you are trying to make is that you might want to apply a function to all of them at once, rather than one by one. That is indeed the case sometimes. But it is very inefficient from a memory standpoint to do so, and prevents you from reading files if its footprint is larger than your Ram. That's why typically generic parsers operate in the way I described.
– DevShark
Jun 23 '17 at 19:40
You always need to do something with them. I think the point you are trying to make is that you might want to apply a function to all of them at once, rather than one by one. That is indeed the case sometimes. But it is very inefficient from a memory standpoint to do so, and prevents you from reading files if its footprint is larger than your Ram. That's why typically generic parsers operate in the way I described.
– DevShark
Jun 23 '17 at 19:40
2
2
@PierreOcinom that is correct. Given that the file is opened in read only mode, you couldn't modify the original file with the code above. To open a file for both reading and writing, use
open('file_path', 'r+')
– DevShark
Sep 14 '17 at 9:17
@PierreOcinom that is correct. Given that the file is opened in read only mode, you couldn't modify the original file with the code above. To open a file for both reading and writing, use
open('file_path', 'r+')
– DevShark
Sep 14 '17 at 9:17
|
show 8 more comments
If you don't care about closing the file, this one-liner works:
lines = open('file.txt').readlines()
The traditional way:
fp = open('file.txt') # Open file on read mode
lines = fp.read().split("n") # Create a list containing all lines
fp.close() # Close file
Using with
and readlines()
(recommended):
with open('file.txt') as fp:
lines = fp.readlines()
3
It might be fine in some cases, but this doesn't close the file, even after the loop has completed - stackoverflow.com/a/1832589/232593
– Merlyn Morgan-Graham
Dec 11 '15 at 0:02
3
Thewith
block closes the file automatically. No need for the finalfp.close()
line in that last example. See: repl.it/IMeA/0
– Merlyn Morgan-Graham
May 23 '17 at 8:57
5
Always care about closing the file! Be a good resource citizen!
– Nick
Jun 29 '18 at 14:38
All the work you're doing here is done by the Pythonreadlines()
method.
– Eric O Lebigot
Oct 23 '18 at 10:58
1
At least for me with Python 3.7.0 it wasfp.readlines()
instead offp.read().readlines()
.
– Erik Nomitch
Nov 28 '18 at 1:57
|
show 2 more comments
If you don't care about closing the file, this one-liner works:
lines = open('file.txt').readlines()
The traditional way:
fp = open('file.txt') # Open file on read mode
lines = fp.read().split("n") # Create a list containing all lines
fp.close() # Close file
Using with
and readlines()
(recommended):
with open('file.txt') as fp:
lines = fp.readlines()
3
It might be fine in some cases, but this doesn't close the file, even after the loop has completed - stackoverflow.com/a/1832589/232593
– Merlyn Morgan-Graham
Dec 11 '15 at 0:02
3
Thewith
block closes the file automatically. No need for the finalfp.close()
line in that last example. See: repl.it/IMeA/0
– Merlyn Morgan-Graham
May 23 '17 at 8:57
5
Always care about closing the file! Be a good resource citizen!
– Nick
Jun 29 '18 at 14:38
All the work you're doing here is done by the Pythonreadlines()
method.
– Eric O Lebigot
Oct 23 '18 at 10:58
1
At least for me with Python 3.7.0 it wasfp.readlines()
instead offp.read().readlines()
.
– Erik Nomitch
Nov 28 '18 at 1:57
|
show 2 more comments
If you don't care about closing the file, this one-liner works:
lines = open('file.txt').readlines()
The traditional way:
fp = open('file.txt') # Open file on read mode
lines = fp.read().split("n") # Create a list containing all lines
fp.close() # Close file
Using with
and readlines()
(recommended):
with open('file.txt') as fp:
lines = fp.readlines()
If you don't care about closing the file, this one-liner works:
lines = open('file.txt').readlines()
The traditional way:
fp = open('file.txt') # Open file on read mode
lines = fp.read().split("n") # Create a list containing all lines
fp.close() # Close file
Using with
and readlines()
(recommended):
with open('file.txt') as fp:
lines = fp.readlines()
edited Jan 27 at 3:27
answered Apr 20 '15 at 5:53


Pedro LobitoPedro Lobito
49k14136165
49k14136165
3
It might be fine in some cases, but this doesn't close the file, even after the loop has completed - stackoverflow.com/a/1832589/232593
– Merlyn Morgan-Graham
Dec 11 '15 at 0:02
3
Thewith
block closes the file automatically. No need for the finalfp.close()
line in that last example. See: repl.it/IMeA/0
– Merlyn Morgan-Graham
May 23 '17 at 8:57
5
Always care about closing the file! Be a good resource citizen!
– Nick
Jun 29 '18 at 14:38
All the work you're doing here is done by the Pythonreadlines()
method.
– Eric O Lebigot
Oct 23 '18 at 10:58
1
At least for me with Python 3.7.0 it wasfp.readlines()
instead offp.read().readlines()
.
– Erik Nomitch
Nov 28 '18 at 1:57
|
show 2 more comments
3
It might be fine in some cases, but this doesn't close the file, even after the loop has completed - stackoverflow.com/a/1832589/232593
– Merlyn Morgan-Graham
Dec 11 '15 at 0:02
3
Thewith
block closes the file automatically. No need for the finalfp.close()
line in that last example. See: repl.it/IMeA/0
– Merlyn Morgan-Graham
May 23 '17 at 8:57
5
Always care about closing the file! Be a good resource citizen!
– Nick
Jun 29 '18 at 14:38
All the work you're doing here is done by the Pythonreadlines()
method.
– Eric O Lebigot
Oct 23 '18 at 10:58
1
At least for me with Python 3.7.0 it wasfp.readlines()
instead offp.read().readlines()
.
– Erik Nomitch
Nov 28 '18 at 1:57
3
3
It might be fine in some cases, but this doesn't close the file, even after the loop has completed - stackoverflow.com/a/1832589/232593
– Merlyn Morgan-Graham
Dec 11 '15 at 0:02
It might be fine in some cases, but this doesn't close the file, even after the loop has completed - stackoverflow.com/a/1832589/232593
– Merlyn Morgan-Graham
Dec 11 '15 at 0:02
3
3
The
with
block closes the file automatically. No need for the final fp.close()
line in that last example. See: repl.it/IMeA/0– Merlyn Morgan-Graham
May 23 '17 at 8:57
The
with
block closes the file automatically. No need for the final fp.close()
line in that last example. See: repl.it/IMeA/0– Merlyn Morgan-Graham
May 23 '17 at 8:57
5
5
Always care about closing the file! Be a good resource citizen!
– Nick
Jun 29 '18 at 14:38
Always care about closing the file! Be a good resource citizen!
– Nick
Jun 29 '18 at 14:38
All the work you're doing here is done by the Python
readlines()
method.– Eric O Lebigot
Oct 23 '18 at 10:58
All the work you're doing here is done by the Python
readlines()
method.– Eric O Lebigot
Oct 23 '18 at 10:58
1
1
At least for me with Python 3.7.0 it was
fp.readlines()
instead of fp.read().readlines()
.– Erik Nomitch
Nov 28 '18 at 1:57
At least for me with Python 3.7.0 it was
fp.readlines()
instead of fp.read().readlines()
.– Erik Nomitch
Nov 28 '18 at 1:57
|
show 2 more comments
Data into list
Assume that we have a text file with our data like in the following lines:
Text file content:
line 1
line 2
line 3
- Open the cmd in the same directory (right click the mouse and choose cmd or PowerShell)
- Run
python
and in the interpreter write:
The Python script
>>> with open("myfile.txt", encoding="utf-8") as file:
... x = [l.strip() for l in file]
>>> x
['line 1','line 2','line 3']
Using append
x =
with open("myfile.txt") as file:
for l in file:
x.append(l.strip())
Or...
>>> x = open("myfile.txt").read().splitlines()
>>> x
['line 1', 'line 2', 'line 3']
Or...
>>> x = open("myfile.txt").readlines()
>>> x
['linea 1n', 'line 2n', 'line 3n']
Or...
>>> y = [x.rstrip() for x in open("my_file.txt")]
>>> y
['line 1','line 2','line 3']
with open('testodiprova.txt', 'r', encoding='utf-8') as file:
file = file.read().splitlines()
print(file)
with open('testodiprova.txt', 'r', encoding='utf-8') as file:
file = file.readlines()
print(file)
is theencoding="utf-8"
required?
– Mausy5043
Jun 3 '18 at 8:53
@Mausy5043 no, but when you read a text file, you can have some strange character (expecially in italian)
– Giovanni Gianni
Jun 3 '18 at 9:55
read().splitlines()
is provided to you by Python: it's simplyreadlines()
(which is probably faster, as it is less wasteful).
– Eric O Lebigot
Oct 23 '18 at 10:57
add a comment |
Data into list
Assume that we have a text file with our data like in the following lines:
Text file content:
line 1
line 2
line 3
- Open the cmd in the same directory (right click the mouse and choose cmd or PowerShell)
- Run
python
and in the interpreter write:
The Python script
>>> with open("myfile.txt", encoding="utf-8") as file:
... x = [l.strip() for l in file]
>>> x
['line 1','line 2','line 3']
Using append
x =
with open("myfile.txt") as file:
for l in file:
x.append(l.strip())
Or...
>>> x = open("myfile.txt").read().splitlines()
>>> x
['line 1', 'line 2', 'line 3']
Or...
>>> x = open("myfile.txt").readlines()
>>> x
['linea 1n', 'line 2n', 'line 3n']
Or...
>>> y = [x.rstrip() for x in open("my_file.txt")]
>>> y
['line 1','line 2','line 3']
with open('testodiprova.txt', 'r', encoding='utf-8') as file:
file = file.read().splitlines()
print(file)
with open('testodiprova.txt', 'r', encoding='utf-8') as file:
file = file.readlines()
print(file)
is theencoding="utf-8"
required?
– Mausy5043
Jun 3 '18 at 8:53
@Mausy5043 no, but when you read a text file, you can have some strange character (expecially in italian)
– Giovanni Gianni
Jun 3 '18 at 9:55
read().splitlines()
is provided to you by Python: it's simplyreadlines()
(which is probably faster, as it is less wasteful).
– Eric O Lebigot
Oct 23 '18 at 10:57
add a comment |
Data into list
Assume that we have a text file with our data like in the following lines:
Text file content:
line 1
line 2
line 3
- Open the cmd in the same directory (right click the mouse and choose cmd or PowerShell)
- Run
python
and in the interpreter write:
The Python script
>>> with open("myfile.txt", encoding="utf-8") as file:
... x = [l.strip() for l in file]
>>> x
['line 1','line 2','line 3']
Using append
x =
with open("myfile.txt") as file:
for l in file:
x.append(l.strip())
Or...
>>> x = open("myfile.txt").read().splitlines()
>>> x
['line 1', 'line 2', 'line 3']
Or...
>>> x = open("myfile.txt").readlines()
>>> x
['linea 1n', 'line 2n', 'line 3n']
Or...
>>> y = [x.rstrip() for x in open("my_file.txt")]
>>> y
['line 1','line 2','line 3']
with open('testodiprova.txt', 'r', encoding='utf-8') as file:
file = file.read().splitlines()
print(file)
with open('testodiprova.txt', 'r', encoding='utf-8') as file:
file = file.readlines()
print(file)
Data into list
Assume that we have a text file with our data like in the following lines:
Text file content:
line 1
line 2
line 3
- Open the cmd in the same directory (right click the mouse and choose cmd or PowerShell)
- Run
python
and in the interpreter write:
The Python script
>>> with open("myfile.txt", encoding="utf-8") as file:
... x = [l.strip() for l in file]
>>> x
['line 1','line 2','line 3']
Using append
x =
with open("myfile.txt") as file:
for l in file:
x.append(l.strip())
Or...
>>> x = open("myfile.txt").read().splitlines()
>>> x
['line 1', 'line 2', 'line 3']
Or...
>>> x = open("myfile.txt").readlines()
>>> x
['linea 1n', 'line 2n', 'line 3n']
Or...
>>> y = [x.rstrip() for x in open("my_file.txt")]
>>> y
['line 1','line 2','line 3']
with open('testodiprova.txt', 'r', encoding='utf-8') as file:
file = file.read().splitlines()
print(file)
with open('testodiprova.txt', 'r', encoding='utf-8') as file:
file = file.readlines()
print(file)
edited Nov 5 '18 at 5:54
answered Apr 26 '17 at 4:57


Giovanni GianniGiovanni Gianni
6,46611623
6,46611623
is theencoding="utf-8"
required?
– Mausy5043
Jun 3 '18 at 8:53
@Mausy5043 no, but when you read a text file, you can have some strange character (expecially in italian)
– Giovanni Gianni
Jun 3 '18 at 9:55
read().splitlines()
is provided to you by Python: it's simplyreadlines()
(which is probably faster, as it is less wasteful).
– Eric O Lebigot
Oct 23 '18 at 10:57
add a comment |
is theencoding="utf-8"
required?
– Mausy5043
Jun 3 '18 at 8:53
@Mausy5043 no, but when you read a text file, you can have some strange character (expecially in italian)
– Giovanni Gianni
Jun 3 '18 at 9:55
read().splitlines()
is provided to you by Python: it's simplyreadlines()
(which is probably faster, as it is less wasteful).
– Eric O Lebigot
Oct 23 '18 at 10:57
is the
encoding="utf-8"
required?– Mausy5043
Jun 3 '18 at 8:53
is the
encoding="utf-8"
required?– Mausy5043
Jun 3 '18 at 8:53
@Mausy5043 no, but when you read a text file, you can have some strange character (expecially in italian)
– Giovanni Gianni
Jun 3 '18 at 9:55
@Mausy5043 no, but when you read a text file, you can have some strange character (expecially in italian)
– Giovanni Gianni
Jun 3 '18 at 9:55
read().splitlines()
is provided to you by Python: it's simply readlines()
(which is probably faster, as it is less wasteful).– Eric O Lebigot
Oct 23 '18 at 10:57
read().splitlines()
is provided to you by Python: it's simply readlines()
(which is probably faster, as it is less wasteful).– Eric O Lebigot
Oct 23 '18 at 10:57
add a comment |
This should encapsulate the open command.
array =
with open("file.txt", "r") as f:
for line in f:
array.append(line)
2
f.readlines() does the same. no need to append to an empty list.
– Corey Goldberg
Mar 6 '15 at 14:51
6
You are right. This provides insight into a solution if you want to do something while you are reading in the lines. Like some strip/regex transformation.
– cevaris
Mar 6 '15 at 15:44
An answer like this has already existed for 3 years before you posted this.
– Aran-Fey
Oct 27 '18 at 15:23
You Sr. are correct. Unsure why this is front page. stackoverflow.com/questions/3277503/…
– cevaris
Oct 28 '18 at 16:53
add a comment |
This should encapsulate the open command.
array =
with open("file.txt", "r") as f:
for line in f:
array.append(line)
2
f.readlines() does the same. no need to append to an empty list.
– Corey Goldberg
Mar 6 '15 at 14:51
6
You are right. This provides insight into a solution if you want to do something while you are reading in the lines. Like some strip/regex transformation.
– cevaris
Mar 6 '15 at 15:44
An answer like this has already existed for 3 years before you posted this.
– Aran-Fey
Oct 27 '18 at 15:23
You Sr. are correct. Unsure why this is front page. stackoverflow.com/questions/3277503/…
– cevaris
Oct 28 '18 at 16:53
add a comment |
This should encapsulate the open command.
array =
with open("file.txt", "r") as f:
for line in f:
array.append(line)
This should encapsulate the open command.
array =
with open("file.txt", "r") as f:
for line in f:
array.append(line)
answered Oct 28 '13 at 15:40
cevariscevaris
4,33513530
4,33513530
2
f.readlines() does the same. no need to append to an empty list.
– Corey Goldberg
Mar 6 '15 at 14:51
6
You are right. This provides insight into a solution if you want to do something while you are reading in the lines. Like some strip/regex transformation.
– cevaris
Mar 6 '15 at 15:44
An answer like this has already existed for 3 years before you posted this.
– Aran-Fey
Oct 27 '18 at 15:23
You Sr. are correct. Unsure why this is front page. stackoverflow.com/questions/3277503/…
– cevaris
Oct 28 '18 at 16:53
add a comment |
2
f.readlines() does the same. no need to append to an empty list.
– Corey Goldberg
Mar 6 '15 at 14:51
6
You are right. This provides insight into a solution if you want to do something while you are reading in the lines. Like some strip/regex transformation.
– cevaris
Mar 6 '15 at 15:44
An answer like this has already existed for 3 years before you posted this.
– Aran-Fey
Oct 27 '18 at 15:23
You Sr. are correct. Unsure why this is front page. stackoverflow.com/questions/3277503/…
– cevaris
Oct 28 '18 at 16:53
2
2
f.readlines() does the same. no need to append to an empty list.
– Corey Goldberg
Mar 6 '15 at 14:51
f.readlines() does the same. no need to append to an empty list.
– Corey Goldberg
Mar 6 '15 at 14:51
6
6
You are right. This provides insight into a solution if you want to do something while you are reading in the lines. Like some strip/regex transformation.
– cevaris
Mar 6 '15 at 15:44
You are right. This provides insight into a solution if you want to do something while you are reading in the lines. Like some strip/regex transformation.
– cevaris
Mar 6 '15 at 15:44
An answer like this has already existed for 3 years before you posted this.
– Aran-Fey
Oct 27 '18 at 15:23
An answer like this has already existed for 3 years before you posted this.
– Aran-Fey
Oct 27 '18 at 15:23
You Sr. are correct. Unsure why this is front page. stackoverflow.com/questions/3277503/…
– cevaris
Oct 28 '18 at 16:53
You Sr. are correct. Unsure why this is front page. stackoverflow.com/questions/3277503/…
– cevaris
Oct 28 '18 at 16:53
add a comment |
Clean and Pythonic Way of Reading the Lines of a File Into a List
First and foremost, you should focus on opening your file and reading its contents in an efficient and pythonic way. Here is an example of the way I personally DO NOT prefer:
infile = open('my_file.txt', 'r') # Open the file for reading.
data = infile.read() # Read the contents of the file.
infile.close() # Close the file since we're done using it.
Instead, I prefer the below method of opening files for both reading and writing as it
is very clean, and does not require an extra step of closing the file
once you are done using it. In the statement below, we're opening the file
for reading, and assigning it to the variable 'infile.' Once the code within
this statement has finished running, the file will be automatically closed.
# Open the file for reading.
with open('my_file.txt', 'r') as infile:
data = infile.read() # Read the contents of the file into memory.
Now we need to focus on bringing this data into a Python List because they are iterable, efficient, and flexible. In your case, the desired goal is to bring each line of the text file into a separate element. To accomplish this, we will use the splitlines() method as follows:
# Return a list of the lines, breaking at line boundaries.
my_list = data.splitlines()
The Final Product:
# Open the file for reading.
with open('my_file.txt', 'r') as infile:
data = infile.read() # Read the contents of the file into memory.
# Return a list of the lines, breaking at line boundaries.
my_list = data.splitlines()
Testing Our Code:
- Contents of the text file:
A fost odatã ca-n povesti,
A fost ca niciodatã,
Din rude mãri împãrãtesti,
O prea frumoasã fatã.
- Print statements for testing purposes:
print my_list # Print the list.
# Print each line in the list.
for line in my_list:
print line
# Print the fourth element in this list.
print my_list[3]
- Output (different-looking because of unicode characters):
['A fost odatxc3xa3 ca-n povesti,', 'A fost ca niciodatxc3xa3,',
'Din rude mxc3xa3ri xc3xaempxc3xa3rxc3xa3testi,', 'O prea
frumoasxc3xa3 fatxc3xa3.']
A fost odatã ca-n povesti, A fost ca niciodatã, Din rude mãri
împãrãtesti, O prea frumoasã fatã.
O prea frumoasã fatã.
add a comment |
Clean and Pythonic Way of Reading the Lines of a File Into a List
First and foremost, you should focus on opening your file and reading its contents in an efficient and pythonic way. Here is an example of the way I personally DO NOT prefer:
infile = open('my_file.txt', 'r') # Open the file for reading.
data = infile.read() # Read the contents of the file.
infile.close() # Close the file since we're done using it.
Instead, I prefer the below method of opening files for both reading and writing as it
is very clean, and does not require an extra step of closing the file
once you are done using it. In the statement below, we're opening the file
for reading, and assigning it to the variable 'infile.' Once the code within
this statement has finished running, the file will be automatically closed.
# Open the file for reading.
with open('my_file.txt', 'r') as infile:
data = infile.read() # Read the contents of the file into memory.
Now we need to focus on bringing this data into a Python List because they are iterable, efficient, and flexible. In your case, the desired goal is to bring each line of the text file into a separate element. To accomplish this, we will use the splitlines() method as follows:
# Return a list of the lines, breaking at line boundaries.
my_list = data.splitlines()
The Final Product:
# Open the file for reading.
with open('my_file.txt', 'r') as infile:
data = infile.read() # Read the contents of the file into memory.
# Return a list of the lines, breaking at line boundaries.
my_list = data.splitlines()
Testing Our Code:
- Contents of the text file:
A fost odatã ca-n povesti,
A fost ca niciodatã,
Din rude mãri împãrãtesti,
O prea frumoasã fatã.
- Print statements for testing purposes:
print my_list # Print the list.
# Print each line in the list.
for line in my_list:
print line
# Print the fourth element in this list.
print my_list[3]
- Output (different-looking because of unicode characters):
['A fost odatxc3xa3 ca-n povesti,', 'A fost ca niciodatxc3xa3,',
'Din rude mxc3xa3ri xc3xaempxc3xa3rxc3xa3testi,', 'O prea
frumoasxc3xa3 fatxc3xa3.']
A fost odatã ca-n povesti, A fost ca niciodatã, Din rude mãri
împãrãtesti, O prea frumoasã fatã.
O prea frumoasã fatã.
add a comment |
Clean and Pythonic Way of Reading the Lines of a File Into a List
First and foremost, you should focus on opening your file and reading its contents in an efficient and pythonic way. Here is an example of the way I personally DO NOT prefer:
infile = open('my_file.txt', 'r') # Open the file for reading.
data = infile.read() # Read the contents of the file.
infile.close() # Close the file since we're done using it.
Instead, I prefer the below method of opening files for both reading and writing as it
is very clean, and does not require an extra step of closing the file
once you are done using it. In the statement below, we're opening the file
for reading, and assigning it to the variable 'infile.' Once the code within
this statement has finished running, the file will be automatically closed.
# Open the file for reading.
with open('my_file.txt', 'r') as infile:
data = infile.read() # Read the contents of the file into memory.
Now we need to focus on bringing this data into a Python List because they are iterable, efficient, and flexible. In your case, the desired goal is to bring each line of the text file into a separate element. To accomplish this, we will use the splitlines() method as follows:
# Return a list of the lines, breaking at line boundaries.
my_list = data.splitlines()
The Final Product:
# Open the file for reading.
with open('my_file.txt', 'r') as infile:
data = infile.read() # Read the contents of the file into memory.
# Return a list of the lines, breaking at line boundaries.
my_list = data.splitlines()
Testing Our Code:
- Contents of the text file:
A fost odatã ca-n povesti,
A fost ca niciodatã,
Din rude mãri împãrãtesti,
O prea frumoasã fatã.
- Print statements for testing purposes:
print my_list # Print the list.
# Print each line in the list.
for line in my_list:
print line
# Print the fourth element in this list.
print my_list[3]
- Output (different-looking because of unicode characters):
['A fost odatxc3xa3 ca-n povesti,', 'A fost ca niciodatxc3xa3,',
'Din rude mxc3xa3ri xc3xaempxc3xa3rxc3xa3testi,', 'O prea
frumoasxc3xa3 fatxc3xa3.']
A fost odatã ca-n povesti, A fost ca niciodatã, Din rude mãri
împãrãtesti, O prea frumoasã fatã.
O prea frumoasã fatã.
Clean and Pythonic Way of Reading the Lines of a File Into a List
First and foremost, you should focus on opening your file and reading its contents in an efficient and pythonic way. Here is an example of the way I personally DO NOT prefer:
infile = open('my_file.txt', 'r') # Open the file for reading.
data = infile.read() # Read the contents of the file.
infile.close() # Close the file since we're done using it.
Instead, I prefer the below method of opening files for both reading and writing as it
is very clean, and does not require an extra step of closing the file
once you are done using it. In the statement below, we're opening the file
for reading, and assigning it to the variable 'infile.' Once the code within
this statement has finished running, the file will be automatically closed.
# Open the file for reading.
with open('my_file.txt', 'r') as infile:
data = infile.read() # Read the contents of the file into memory.
Now we need to focus on bringing this data into a Python List because they are iterable, efficient, and flexible. In your case, the desired goal is to bring each line of the text file into a separate element. To accomplish this, we will use the splitlines() method as follows:
# Return a list of the lines, breaking at line boundaries.
my_list = data.splitlines()
The Final Product:
# Open the file for reading.
with open('my_file.txt', 'r') as infile:
data = infile.read() # Read the contents of the file into memory.
# Return a list of the lines, breaking at line boundaries.
my_list = data.splitlines()
Testing Our Code:
- Contents of the text file:
A fost odatã ca-n povesti,
A fost ca niciodatã,
Din rude mãri împãrãtesti,
O prea frumoasã fatã.
- Print statements for testing purposes:
print my_list # Print the list.
# Print each line in the list.
for line in my_list:
print line
# Print the fourth element in this list.
print my_list[3]
- Output (different-looking because of unicode characters):
['A fost odatxc3xa3 ca-n povesti,', 'A fost ca niciodatxc3xa3,',
'Din rude mxc3xa3ri xc3xaempxc3xa3rxc3xa3testi,', 'O prea
frumoasxc3xa3 fatxc3xa3.']
A fost odatã ca-n povesti, A fost ca niciodatã, Din rude mãri
împãrãtesti, O prea frumoasã fatã.
O prea frumoasã fatã.
answered Dec 20 '14 at 18:31
JohnnyJohnny
1,5521108
1,5521108
add a comment |
add a comment |
To read a file into a list you need to do three things:
- Open the file
- Read the file
- Store the contents as list
Fortunately Python makes it very easy to do these things so the shortest way to read a file into a list is:
lst = list(open(filename))
However I'll add some more explanation.
Opening the file
I assume that you want to open a specific file and you don't deal directly with a file-handle (or a file-like-handle). The most commonly used function to open a file in Python is open
, it takes one mandatory argument and two optional ones in Python 2.7:
- Filename
- Mode
- Buffering (I'll ignore this argument in this answer)
The filename should be a string that represents the path to the file. For example:
open('afile') # opens the file named afile in the current working directory
open('adir/afile') # relative path (relative to the current working directory)
open('C:/users/aname/afile') # absolute path (windows)
open('/usr/local/afile') # absolute path (linux)
Note that the file extension needs to be specified. This is especially important for Windows users because file extensions like .txt
or .doc
, etc. are hidden by default when viewed in the explorer.
The second argument is the mode
, it's r
by default which means "read-only". That's exactly what you need in your case.
But in case you actually want to create a file and/or write to a file you'll need a different argument here. There is an excellent answer if you want an overview.
For reading a file you can omit the mode
or pass it in explicitly:
open(filename)
open(filename, 'r')
Both will open the file in read-only mode. In case you want to read in a binary file on Windows you need to use the mode rb
:
open(filename, 'rb')
On other platforms the 'b'
(binary mode) is simply ignored.
Now that I've shown how to open
the file, let's talk about the fact that you always need to close
it again. Otherwise it will keep an open file-handle to the file until the process exits (or Python garbages the file-handle).
While you could use:
f = open(filename)
# ... do stuff with f
f.close()
That will fail to close the file when something between open
and close
throws an exception. You could avoid that by using a try
and finally
:
f = open(filename)
# nothing in between!
try:
# do stuff with f
finally:
f.close()
However Python provides context managers that have a prettier syntax (but for open
it's almost identical to the try
and finally
above):
with open(filename) as f:
# do stuff with f
# The file is always closed after the with-scope ends.
The last approach is the recommended approach to open a file in Python!
Reading the file
Okay, you've opened the file, now how to read it?
The open
function returns a file
object and it supports Pythons iteration protocol. Each iteration will give you a line:
with open(filename) as f:
for line in f:
print(line)
This will print each line of the file. Note however that each line will contain a newline character n
at the end (you might want to check if your Python is built with universal newlines support - otherwise you could also have rn
on Windows or r
on Mac as newlines). If you don't want that you can could simply remove the last character (or the last two characters on Windows):
with open(filename) as f:
for line in f:
print(line[:-1])
But the last line doesn't necessarily has a trailing newline, so one shouldn't use that. One could check if it ends with a trailing newline and if so remove it:
with open(filename) as f:
for line in f:
if line.endswith('n'):
line = line[:-1]
print(line)
But you could simply remove all whitespaces (including the n
character) from the end of the string, this will also remove all other trailing whitespaces so you have to be careful if these are important:
with open(filename) as f:
for line in f:
print(f.rstrip())
However if the lines end with rn
(Windows "newlines") that .rstrip()
will also take care of the r
!
Store the contents as list
Now that you know how to open the file and read it, it's time to store the contents in a list. The simplest option would be to use the list
function:
with open(filename) as f:
lst = list(f)
In case you want to strip the trailing newlines you could use a list comprehension instead:
with open(filename) as f:
lst = [line.rstrip() for line in f]
Or even simpler: The .readlines()
method of the file
object by default returns a list
of the lines:
with open(filename) as f:
lst = f.readlines()
This will also include the trailing newline characters, if you don't want them I would recommend the [line.rstrip() for line in f]
approach because it avoids keeping two lists containing all the lines in memory.
There's an additional option to get the desired output, however it's rather "suboptimal": read
the complete file in a string and then split on newlines:
with open(filename) as f:
lst = f.read().split('n')
or:
with open(filename) as f:
lst = f.read().splitlines()
These take care of the trailing newlines automatically because the split
character isn't included. However they are not ideal because you keep the file as string and as a list of lines in memory!
Summary
- Use
with open(...) as f
when opening files because you don't need to take care of closing the file yourself and it closes the file even if some exception happens.
file
objects support the iteration protocol so reading a file line-by-line is as simple asfor line in the_file_object:
.- Always browse the documentation for the available functions/classes. Most of the time there's a perfect match for the task or at least one or two good ones. The obvious choice in this case would be
readlines()
but if you want to process the lines before storing them in the list I would recommend a simple list-comprehension.
Excellent answer!
– Peter Zhao
Jan 7 at 8:52
add a comment |
To read a file into a list you need to do three things:
- Open the file
- Read the file
- Store the contents as list
Fortunately Python makes it very easy to do these things so the shortest way to read a file into a list is:
lst = list(open(filename))
However I'll add some more explanation.
Opening the file
I assume that you want to open a specific file and you don't deal directly with a file-handle (or a file-like-handle). The most commonly used function to open a file in Python is open
, it takes one mandatory argument and two optional ones in Python 2.7:
- Filename
- Mode
- Buffering (I'll ignore this argument in this answer)
The filename should be a string that represents the path to the file. For example:
open('afile') # opens the file named afile in the current working directory
open('adir/afile') # relative path (relative to the current working directory)
open('C:/users/aname/afile') # absolute path (windows)
open('/usr/local/afile') # absolute path (linux)
Note that the file extension needs to be specified. This is especially important for Windows users because file extensions like .txt
or .doc
, etc. are hidden by default when viewed in the explorer.
The second argument is the mode
, it's r
by default which means "read-only". That's exactly what you need in your case.
But in case you actually want to create a file and/or write to a file you'll need a different argument here. There is an excellent answer if you want an overview.
For reading a file you can omit the mode
or pass it in explicitly:
open(filename)
open(filename, 'r')
Both will open the file in read-only mode. In case you want to read in a binary file on Windows you need to use the mode rb
:
open(filename, 'rb')
On other platforms the 'b'
(binary mode) is simply ignored.
Now that I've shown how to open
the file, let's talk about the fact that you always need to close
it again. Otherwise it will keep an open file-handle to the file until the process exits (or Python garbages the file-handle).
While you could use:
f = open(filename)
# ... do stuff with f
f.close()
That will fail to close the file when something between open
and close
throws an exception. You could avoid that by using a try
and finally
:
f = open(filename)
# nothing in between!
try:
# do stuff with f
finally:
f.close()
However Python provides context managers that have a prettier syntax (but for open
it's almost identical to the try
and finally
above):
with open(filename) as f:
# do stuff with f
# The file is always closed after the with-scope ends.
The last approach is the recommended approach to open a file in Python!
Reading the file
Okay, you've opened the file, now how to read it?
The open
function returns a file
object and it supports Pythons iteration protocol. Each iteration will give you a line:
with open(filename) as f:
for line in f:
print(line)
This will print each line of the file. Note however that each line will contain a newline character n
at the end (you might want to check if your Python is built with universal newlines support - otherwise you could also have rn
on Windows or r
on Mac as newlines). If you don't want that you can could simply remove the last character (or the last two characters on Windows):
with open(filename) as f:
for line in f:
print(line[:-1])
But the last line doesn't necessarily has a trailing newline, so one shouldn't use that. One could check if it ends with a trailing newline and if so remove it:
with open(filename) as f:
for line in f:
if line.endswith('n'):
line = line[:-1]
print(line)
But you could simply remove all whitespaces (including the n
character) from the end of the string, this will also remove all other trailing whitespaces so you have to be careful if these are important:
with open(filename) as f:
for line in f:
print(f.rstrip())
However if the lines end with rn
(Windows "newlines") that .rstrip()
will also take care of the r
!
Store the contents as list
Now that you know how to open the file and read it, it's time to store the contents in a list. The simplest option would be to use the list
function:
with open(filename) as f:
lst = list(f)
In case you want to strip the trailing newlines you could use a list comprehension instead:
with open(filename) as f:
lst = [line.rstrip() for line in f]
Or even simpler: The .readlines()
method of the file
object by default returns a list
of the lines:
with open(filename) as f:
lst = f.readlines()
This will also include the trailing newline characters, if you don't want them I would recommend the [line.rstrip() for line in f]
approach because it avoids keeping two lists containing all the lines in memory.
There's an additional option to get the desired output, however it's rather "suboptimal": read
the complete file in a string and then split on newlines:
with open(filename) as f:
lst = f.read().split('n')
or:
with open(filename) as f:
lst = f.read().splitlines()
These take care of the trailing newlines automatically because the split
character isn't included. However they are not ideal because you keep the file as string and as a list of lines in memory!
Summary
- Use
with open(...) as f
when opening files because you don't need to take care of closing the file yourself and it closes the file even if some exception happens.
file
objects support the iteration protocol so reading a file line-by-line is as simple asfor line in the_file_object:
.- Always browse the documentation for the available functions/classes. Most of the time there's a perfect match for the task or at least one or two good ones. The obvious choice in this case would be
readlines()
but if you want to process the lines before storing them in the list I would recommend a simple list-comprehension.
Excellent answer!
– Peter Zhao
Jan 7 at 8:52
add a comment |
To read a file into a list you need to do three things:
- Open the file
- Read the file
- Store the contents as list
Fortunately Python makes it very easy to do these things so the shortest way to read a file into a list is:
lst = list(open(filename))
However I'll add some more explanation.
Opening the file
I assume that you want to open a specific file and you don't deal directly with a file-handle (or a file-like-handle). The most commonly used function to open a file in Python is open
, it takes one mandatory argument and two optional ones in Python 2.7:
- Filename
- Mode
- Buffering (I'll ignore this argument in this answer)
The filename should be a string that represents the path to the file. For example:
open('afile') # opens the file named afile in the current working directory
open('adir/afile') # relative path (relative to the current working directory)
open('C:/users/aname/afile') # absolute path (windows)
open('/usr/local/afile') # absolute path (linux)
Note that the file extension needs to be specified. This is especially important for Windows users because file extensions like .txt
or .doc
, etc. are hidden by default when viewed in the explorer.
The second argument is the mode
, it's r
by default which means "read-only". That's exactly what you need in your case.
But in case you actually want to create a file and/or write to a file you'll need a different argument here. There is an excellent answer if you want an overview.
For reading a file you can omit the mode
or pass it in explicitly:
open(filename)
open(filename, 'r')
Both will open the file in read-only mode. In case you want to read in a binary file on Windows you need to use the mode rb
:
open(filename, 'rb')
On other platforms the 'b'
(binary mode) is simply ignored.
Now that I've shown how to open
the file, let's talk about the fact that you always need to close
it again. Otherwise it will keep an open file-handle to the file until the process exits (or Python garbages the file-handle).
While you could use:
f = open(filename)
# ... do stuff with f
f.close()
That will fail to close the file when something between open
and close
throws an exception. You could avoid that by using a try
and finally
:
f = open(filename)
# nothing in between!
try:
# do stuff with f
finally:
f.close()
However Python provides context managers that have a prettier syntax (but for open
it's almost identical to the try
and finally
above):
with open(filename) as f:
# do stuff with f
# The file is always closed after the with-scope ends.
The last approach is the recommended approach to open a file in Python!
Reading the file
Okay, you've opened the file, now how to read it?
The open
function returns a file
object and it supports Pythons iteration protocol. Each iteration will give you a line:
with open(filename) as f:
for line in f:
print(line)
This will print each line of the file. Note however that each line will contain a newline character n
at the end (you might want to check if your Python is built with universal newlines support - otherwise you could also have rn
on Windows or r
on Mac as newlines). If you don't want that you can could simply remove the last character (or the last two characters on Windows):
with open(filename) as f:
for line in f:
print(line[:-1])
But the last line doesn't necessarily has a trailing newline, so one shouldn't use that. One could check if it ends with a trailing newline and if so remove it:
with open(filename) as f:
for line in f:
if line.endswith('n'):
line = line[:-1]
print(line)
But you could simply remove all whitespaces (including the n
character) from the end of the string, this will also remove all other trailing whitespaces so you have to be careful if these are important:
with open(filename) as f:
for line in f:
print(f.rstrip())
However if the lines end with rn
(Windows "newlines") that .rstrip()
will also take care of the r
!
Store the contents as list
Now that you know how to open the file and read it, it's time to store the contents in a list. The simplest option would be to use the list
function:
with open(filename) as f:
lst = list(f)
In case you want to strip the trailing newlines you could use a list comprehension instead:
with open(filename) as f:
lst = [line.rstrip() for line in f]
Or even simpler: The .readlines()
method of the file
object by default returns a list
of the lines:
with open(filename) as f:
lst = f.readlines()
This will also include the trailing newline characters, if you don't want them I would recommend the [line.rstrip() for line in f]
approach because it avoids keeping two lists containing all the lines in memory.
There's an additional option to get the desired output, however it's rather "suboptimal": read
the complete file in a string and then split on newlines:
with open(filename) as f:
lst = f.read().split('n')
or:
with open(filename) as f:
lst = f.read().splitlines()
These take care of the trailing newlines automatically because the split
character isn't included. However they are not ideal because you keep the file as string and as a list of lines in memory!
Summary
- Use
with open(...) as f
when opening files because you don't need to take care of closing the file yourself and it closes the file even if some exception happens.
file
objects support the iteration protocol so reading a file line-by-line is as simple asfor line in the_file_object:
.- Always browse the documentation for the available functions/classes. Most of the time there's a perfect match for the task or at least one or two good ones. The obvious choice in this case would be
readlines()
but if you want to process the lines before storing them in the list I would recommend a simple list-comprehension.
To read a file into a list you need to do three things:
- Open the file
- Read the file
- Store the contents as list
Fortunately Python makes it very easy to do these things so the shortest way to read a file into a list is:
lst = list(open(filename))
However I'll add some more explanation.
Opening the file
I assume that you want to open a specific file and you don't deal directly with a file-handle (or a file-like-handle). The most commonly used function to open a file in Python is open
, it takes one mandatory argument and two optional ones in Python 2.7:
- Filename
- Mode
- Buffering (I'll ignore this argument in this answer)
The filename should be a string that represents the path to the file. For example:
open('afile') # opens the file named afile in the current working directory
open('adir/afile') # relative path (relative to the current working directory)
open('C:/users/aname/afile') # absolute path (windows)
open('/usr/local/afile') # absolute path (linux)
Note that the file extension needs to be specified. This is especially important for Windows users because file extensions like .txt
or .doc
, etc. are hidden by default when viewed in the explorer.
The second argument is the mode
, it's r
by default which means "read-only". That's exactly what you need in your case.
But in case you actually want to create a file and/or write to a file you'll need a different argument here. There is an excellent answer if you want an overview.
For reading a file you can omit the mode
or pass it in explicitly:
open(filename)
open(filename, 'r')
Both will open the file in read-only mode. In case you want to read in a binary file on Windows you need to use the mode rb
:
open(filename, 'rb')
On other platforms the 'b'
(binary mode) is simply ignored.
Now that I've shown how to open
the file, let's talk about the fact that you always need to close
it again. Otherwise it will keep an open file-handle to the file until the process exits (or Python garbages the file-handle).
While you could use:
f = open(filename)
# ... do stuff with f
f.close()
That will fail to close the file when something between open
and close
throws an exception. You could avoid that by using a try
and finally
:
f = open(filename)
# nothing in between!
try:
# do stuff with f
finally:
f.close()
However Python provides context managers that have a prettier syntax (but for open
it's almost identical to the try
and finally
above):
with open(filename) as f:
# do stuff with f
# The file is always closed after the with-scope ends.
The last approach is the recommended approach to open a file in Python!
Reading the file
Okay, you've opened the file, now how to read it?
The open
function returns a file
object and it supports Pythons iteration protocol. Each iteration will give you a line:
with open(filename) as f:
for line in f:
print(line)
This will print each line of the file. Note however that each line will contain a newline character n
at the end (you might want to check if your Python is built with universal newlines support - otherwise you could also have rn
on Windows or r
on Mac as newlines). If you don't want that you can could simply remove the last character (or the last two characters on Windows):
with open(filename) as f:
for line in f:
print(line[:-1])
But the last line doesn't necessarily has a trailing newline, so one shouldn't use that. One could check if it ends with a trailing newline and if so remove it:
with open(filename) as f:
for line in f:
if line.endswith('n'):
line = line[:-1]
print(line)
But you could simply remove all whitespaces (including the n
character) from the end of the string, this will also remove all other trailing whitespaces so you have to be careful if these are important:
with open(filename) as f:
for line in f:
print(f.rstrip())
However if the lines end with rn
(Windows "newlines") that .rstrip()
will also take care of the r
!
Store the contents as list
Now that you know how to open the file and read it, it's time to store the contents in a list. The simplest option would be to use the list
function:
with open(filename) as f:
lst = list(f)
In case you want to strip the trailing newlines you could use a list comprehension instead:
with open(filename) as f:
lst = [line.rstrip() for line in f]
Or even simpler: The .readlines()
method of the file
object by default returns a list
of the lines:
with open(filename) as f:
lst = f.readlines()
This will also include the trailing newline characters, if you don't want them I would recommend the [line.rstrip() for line in f]
approach because it avoids keeping two lists containing all the lines in memory.
There's an additional option to get the desired output, however it's rather "suboptimal": read
the complete file in a string and then split on newlines:
with open(filename) as f:
lst = f.read().split('n')
or:
with open(filename) as f:
lst = f.read().splitlines()
These take care of the trailing newlines automatically because the split
character isn't included. However they are not ideal because you keep the file as string and as a list of lines in memory!
Summary
- Use
with open(...) as f
when opening files because you don't need to take care of closing the file yourself and it closes the file even if some exception happens.
file
objects support the iteration protocol so reading a file line-by-line is as simple asfor line in the_file_object:
.- Always browse the documentation for the available functions/classes. Most of the time there's a perfect match for the task or at least one or two good ones. The obvious choice in this case would be
readlines()
but if you want to process the lines before storing them in the list I would recommend a simple list-comprehension.
edited Mar 19 '18 at 20:40
answered Jan 16 '18 at 22:33
MSeifertMSeifert
76k18147179
76k18147179
Excellent answer!
– Peter Zhao
Jan 7 at 8:52
add a comment |
Excellent answer!
– Peter Zhao
Jan 7 at 8:52
Excellent answer!
– Peter Zhao
Jan 7 at 8:52
Excellent answer!
– Peter Zhao
Jan 7 at 8:52
add a comment |
I'd do it like this.
lines =
with open("myfile.txt") as f:
for line in f:
lines.append(line)
We already have 2 answers like this, posted 3 and 6 years before yours...
– Aran-Fey
Oct 27 '18 at 15:26
add a comment |
I'd do it like this.
lines =
with open("myfile.txt") as f:
for line in f:
lines.append(line)
We already have 2 answers like this, posted 3 and 6 years before yours...
– Aran-Fey
Oct 27 '18 at 15:26
add a comment |
I'd do it like this.
lines =
with open("myfile.txt") as f:
for line in f:
lines.append(line)
I'd do it like this.
lines =
with open("myfile.txt") as f:
for line in f:
lines.append(line)
answered Dec 9 '16 at 18:43
user3394040user3394040
632817
632817
We already have 2 answers like this, posted 3 and 6 years before yours...
– Aran-Fey
Oct 27 '18 at 15:26
add a comment |
We already have 2 answers like this, posted 3 and 6 years before yours...
– Aran-Fey
Oct 27 '18 at 15:26
We already have 2 answers like this, posted 3 and 6 years before yours...
– Aran-Fey
Oct 27 '18 at 15:26
We already have 2 answers like this, posted 3 and 6 years before yours...
– Aran-Fey
Oct 27 '18 at 15:26
add a comment |
Here's one more option by using list comprehensions on files;
lines = [line.rstrip() for line in open('file.txt')]
This should be more efficient way as the most of the work is done inside the Python interpreter.
7
rstrip()
potentially strips all trailing whitespace, not just then
; use.rstrip('n')
.
– mklement0
May 22 '15 at 16:39
add a comment |
Here's one more option by using list comprehensions on files;
lines = [line.rstrip() for line in open('file.txt')]
This should be more efficient way as the most of the work is done inside the Python interpreter.
7
rstrip()
potentially strips all trailing whitespace, not just then
; use.rstrip('n')
.
– mklement0
May 22 '15 at 16:39
add a comment |
Here's one more option by using list comprehensions on files;
lines = [line.rstrip() for line in open('file.txt')]
This should be more efficient way as the most of the work is done inside the Python interpreter.
Here's one more option by using list comprehensions on files;
lines = [line.rstrip() for line in open('file.txt')]
This should be more efficient way as the most of the work is done inside the Python interpreter.
answered May 27 '14 at 12:21
user1833244user1833244
35123
35123
7
rstrip()
potentially strips all trailing whitespace, not just then
; use.rstrip('n')
.
– mklement0
May 22 '15 at 16:39
add a comment |
7
rstrip()
potentially strips all trailing whitespace, not just then
; use.rstrip('n')
.
– mklement0
May 22 '15 at 16:39
7
7
rstrip()
potentially strips all trailing whitespace, not just the n
; use .rstrip('n')
.– mklement0
May 22 '15 at 16:39
rstrip()
potentially strips all trailing whitespace, not just the n
; use .rstrip('n')
.– mklement0
May 22 '15 at 16:39
add a comment |
Another option is numpy.genfromtxt
, for example:
import numpy as np
data = np.genfromtxt("yourfile.dat",delimiter="n")
This will make data
a NumPy array with as many rows as are in your file.
add a comment |
Another option is numpy.genfromtxt
, for example:
import numpy as np
data = np.genfromtxt("yourfile.dat",delimiter="n")
This will make data
a NumPy array with as many rows as are in your file.
add a comment |
Another option is numpy.genfromtxt
, for example:
import numpy as np
data = np.genfromtxt("yourfile.dat",delimiter="n")
This will make data
a NumPy array with as many rows as are in your file.
Another option is numpy.genfromtxt
, for example:
import numpy as np
data = np.genfromtxt("yourfile.dat",delimiter="n")
This will make data
a NumPy array with as many rows as are in your file.
edited Mar 4 '17 at 21:52


Peter Mortensen
13.7k1986111
13.7k1986111
answered Jun 18 '13 at 10:17
atomh33lsatomh33ls
12.2k1268121
12.2k1268121
add a comment |
add a comment |
If you'd like to read a file from the command line or from stdin, you can also use the fileinput
module:
# reader.py
import fileinput
content =
for line in fileinput.input():
content.append(line.strip())
fileinput.close()
Pass files to it like so:
$ python reader.py textfile.txt
Read more here: http://docs.python.org/2/library/fileinput.html
add a comment |
If you'd like to read a file from the command line or from stdin, you can also use the fileinput
module:
# reader.py
import fileinput
content =
for line in fileinput.input():
content.append(line.strip())
fileinput.close()
Pass files to it like so:
$ python reader.py textfile.txt
Read more here: http://docs.python.org/2/library/fileinput.html
add a comment |
If you'd like to read a file from the command line or from stdin, you can also use the fileinput
module:
# reader.py
import fileinput
content =
for line in fileinput.input():
content.append(line.strip())
fileinput.close()
Pass files to it like so:
$ python reader.py textfile.txt
Read more here: http://docs.python.org/2/library/fileinput.html
If you'd like to read a file from the command line or from stdin, you can also use the fileinput
module:
# reader.py
import fileinput
content =
for line in fileinput.input():
content.append(line.strip())
fileinput.close()
Pass files to it like so:
$ python reader.py textfile.txt
Read more here: http://docs.python.org/2/library/fileinput.html
answered Nov 22 '13 at 14:57
olilandoliland
1,074921
1,074921
add a comment |
add a comment |
The simplest way to do it
A simple way is to:
- Read the whole file as a string
- Split the string line by line
In one line, that would give:
lines = open('C:/path/file.txt').read().splitlines()
add a comment |
The simplest way to do it
A simple way is to:
- Read the whole file as a string
- Split the string line by line
In one line, that would give:
lines = open('C:/path/file.txt').read().splitlines()
add a comment |
The simplest way to do it
A simple way is to:
- Read the whole file as a string
- Split the string line by line
In one line, that would give:
lines = open('C:/path/file.txt').read().splitlines()
The simplest way to do it
A simple way is to:
- Read the whole file as a string
- Split the string line by line
In one line, that would give:
lines = open('C:/path/file.txt').read().splitlines()
edited Feb 10 '15 at 5:47
answered Feb 6 '15 at 3:34
Jean-Francois T.Jean-Francois T.
5,16912756
5,16912756
add a comment |
add a comment |
Read and write text files with Python 2 and Python 3; it works with Unicode
#!/usr/bin/env python3
# -*- coding: utf-8 -*-
# Define data
lines = [' A first string ',
'A Unicode sample: €',
'German: äöüß']
# Write text file
with open('file.txt', 'w') as fp:
fp.write('n'.join(lines))
# Read text file
with open('file.txt', 'r') as fp:
read_lines = fp.readlines()
read_lines = [line.rstrip('n') for line in read_lines]
print(lines == read_lines)
Things to notice:
with
is a so-called context manager. It makes sure that the opened file is closed again.- All solutions here which simply make
.strip()
or.rstrip()
will fail to reproduce thelines
as they also strip the white space.
Common file endings
.txt
More advanced file writing / reading
- CSV: Super simple format (read & write)
- JSON: Nice for writing human-readable data; VERY commonly used (read & write)
- YAML: YAML is a superset of JSON, but easier to read (read & write, comparison of JSON and YAML)
- pickle: A Python serialization format (read & write)
MessagePack (Python package): More compact representation (read & write)
HDF5 (Python package): Nice for matrices (read & write)- XML: exists too *sigh* (read & write)
For your application, the following might be important:
- Support by other programming languages
- Reading / writing performance
- Compactness (file size)
See also: Comparison of data serialization formats
In case you are rather looking for a way to make configuration files, you might want to read my short article Configuration files in Python.
add a comment |
Read and write text files with Python 2 and Python 3; it works with Unicode
#!/usr/bin/env python3
# -*- coding: utf-8 -*-
# Define data
lines = [' A first string ',
'A Unicode sample: €',
'German: äöüß']
# Write text file
with open('file.txt', 'w') as fp:
fp.write('n'.join(lines))
# Read text file
with open('file.txt', 'r') as fp:
read_lines = fp.readlines()
read_lines = [line.rstrip('n') for line in read_lines]
print(lines == read_lines)
Things to notice:
with
is a so-called context manager. It makes sure that the opened file is closed again.- All solutions here which simply make
.strip()
or.rstrip()
will fail to reproduce thelines
as they also strip the white space.
Common file endings
.txt
More advanced file writing / reading
- CSV: Super simple format (read & write)
- JSON: Nice for writing human-readable data; VERY commonly used (read & write)
- YAML: YAML is a superset of JSON, but easier to read (read & write, comparison of JSON and YAML)
- pickle: A Python serialization format (read & write)
MessagePack (Python package): More compact representation (read & write)
HDF5 (Python package): Nice for matrices (read & write)- XML: exists too *sigh* (read & write)
For your application, the following might be important:
- Support by other programming languages
- Reading / writing performance
- Compactness (file size)
See also: Comparison of data serialization formats
In case you are rather looking for a way to make configuration files, you might want to read my short article Configuration files in Python.
add a comment |
Read and write text files with Python 2 and Python 3; it works with Unicode
#!/usr/bin/env python3
# -*- coding: utf-8 -*-
# Define data
lines = [' A first string ',
'A Unicode sample: €',
'German: äöüß']
# Write text file
with open('file.txt', 'w') as fp:
fp.write('n'.join(lines))
# Read text file
with open('file.txt', 'r') as fp:
read_lines = fp.readlines()
read_lines = [line.rstrip('n') for line in read_lines]
print(lines == read_lines)
Things to notice:
with
is a so-called context manager. It makes sure that the opened file is closed again.- All solutions here which simply make
.strip()
or.rstrip()
will fail to reproduce thelines
as they also strip the white space.
Common file endings
.txt
More advanced file writing / reading
- CSV: Super simple format (read & write)
- JSON: Nice for writing human-readable data; VERY commonly used (read & write)
- YAML: YAML is a superset of JSON, but easier to read (read & write, comparison of JSON and YAML)
- pickle: A Python serialization format (read & write)
MessagePack (Python package): More compact representation (read & write)
HDF5 (Python package): Nice for matrices (read & write)- XML: exists too *sigh* (read & write)
For your application, the following might be important:
- Support by other programming languages
- Reading / writing performance
- Compactness (file size)
See also: Comparison of data serialization formats
In case you are rather looking for a way to make configuration files, you might want to read my short article Configuration files in Python.
Read and write text files with Python 2 and Python 3; it works with Unicode
#!/usr/bin/env python3
# -*- coding: utf-8 -*-
# Define data
lines = [' A first string ',
'A Unicode sample: €',
'German: äöüß']
# Write text file
with open('file.txt', 'w') as fp:
fp.write('n'.join(lines))
# Read text file
with open('file.txt', 'r') as fp:
read_lines = fp.readlines()
read_lines = [line.rstrip('n') for line in read_lines]
print(lines == read_lines)
Things to notice:
with
is a so-called context manager. It makes sure that the opened file is closed again.- All solutions here which simply make
.strip()
or.rstrip()
will fail to reproduce thelines
as they also strip the white space.
Common file endings
.txt
More advanced file writing / reading
- CSV: Super simple format (read & write)
- JSON: Nice for writing human-readable data; VERY commonly used (read & write)
- YAML: YAML is a superset of JSON, but easier to read (read & write, comparison of JSON and YAML)
- pickle: A Python serialization format (read & write)
MessagePack (Python package): More compact representation (read & write)
HDF5 (Python package): Nice for matrices (read & write)- XML: exists too *sigh* (read & write)
For your application, the following might be important:
- Support by other programming languages
- Reading / writing performance
- Compactness (file size)
See also: Comparison of data serialization formats
In case you are rather looking for a way to make configuration files, you might want to read my short article Configuration files in Python.
edited May 21 '18 at 19:55


Peter Mortensen
13.7k1986111
13.7k1986111
answered Jan 16 '18 at 19:42
Martin ThomaMartin Thoma
42.8k59303525
42.8k59303525
add a comment |
add a comment |
Introduced in Python 3.4, pathlib
has a really convenient method for reading in text from files, as follows:
from pathlib import Path
p = Path('my_text_file')
lines = p.read_text().splitlines()
(The splitlines
call is what turns it from a string containing the whole contents of the file to a list of lines in the file).
pathlib
has a lot of handy conveniences in it. read_text
is nice and concise, and you don't have to worry about opening and closing the file. If all you need to do with the file is read it all in in one go, it's a good choice.
add a comment |
Introduced in Python 3.4, pathlib
has a really convenient method for reading in text from files, as follows:
from pathlib import Path
p = Path('my_text_file')
lines = p.read_text().splitlines()
(The splitlines
call is what turns it from a string containing the whole contents of the file to a list of lines in the file).
pathlib
has a lot of handy conveniences in it. read_text
is nice and concise, and you don't have to worry about opening and closing the file. If all you need to do with the file is read it all in in one go, it's a good choice.
add a comment |
Introduced in Python 3.4, pathlib
has a really convenient method for reading in text from files, as follows:
from pathlib import Path
p = Path('my_text_file')
lines = p.read_text().splitlines()
(The splitlines
call is what turns it from a string containing the whole contents of the file to a list of lines in the file).
pathlib
has a lot of handy conveniences in it. read_text
is nice and concise, and you don't have to worry about opening and closing the file. If all you need to do with the file is read it all in in one go, it's a good choice.
Introduced in Python 3.4, pathlib
has a really convenient method for reading in text from files, as follows:
from pathlib import Path
p = Path('my_text_file')
lines = p.read_text().splitlines()
(The splitlines
call is what turns it from a string containing the whole contents of the file to a list of lines in the file).
pathlib
has a lot of handy conveniences in it. read_text
is nice and concise, and you don't have to worry about opening and closing the file. If all you need to do with the file is read it all in in one go, it's a good choice.
edited Dec 2 '18 at 1:33


martineau
67.8k1089182
67.8k1089182
answered Apr 30 '18 at 17:41


LangeHaareLangeHaare
915720
915720
add a comment |
add a comment |
f = open("your_file.txt",'r')
out = f.readlines() # will append in the list out
Now variable out is a list (array) of what you want. You could either do:
for line in out:
print line
or
for line in f:
print line
you'll get the same results.
add a comment |
f = open("your_file.txt",'r')
out = f.readlines() # will append in the list out
Now variable out is a list (array) of what you want. You could either do:
for line in out:
print line
or
for line in f:
print line
you'll get the same results.
add a comment |
f = open("your_file.txt",'r')
out = f.readlines() # will append in the list out
Now variable out is a list (array) of what you want. You could either do:
for line in out:
print line
or
for line in f:
print line
you'll get the same results.
f = open("your_file.txt",'r')
out = f.readlines() # will append in the list out
Now variable out is a list (array) of what you want. You could either do:
for line in out:
print line
or
for line in f:
print line
you'll get the same results.
answered Jan 12 '14 at 10:58
moldoveanmoldovean
1,8932324
1,8932324
add a comment |
add a comment |
Just use the splitlines() functions. Here is an example.
inp = "file.txt"
data = open(inp)
dat = data.read()
lst = dat.splitlines()
print lst
# print(lst) # for python 3
In the output you will have the list of lines.
add a comment |
Just use the splitlines() functions. Here is an example.
inp = "file.txt"
data = open(inp)
dat = data.read()
lst = dat.splitlines()
print lst
# print(lst) # for python 3
In the output you will have the list of lines.
add a comment |
Just use the splitlines() functions. Here is an example.
inp = "file.txt"
data = open(inp)
dat = data.read()
lst = dat.splitlines()
print lst
# print(lst) # for python 3
In the output you will have the list of lines.
Just use the splitlines() functions. Here is an example.
inp = "file.txt"
data = open(inp)
dat = data.read()
lst = dat.splitlines()
print lst
# print(lst) # for python 3
In the output you will have the list of lines.
edited Nov 26 '17 at 7:56


Giovanni Gianni
6,46611623
6,46611623
answered Sep 9 '16 at 9:13
Abdullah BilalAbdullah Bilal
157129
157129
add a comment |
add a comment |
A real easy way:
with open(file) as g:
stuff = g.readlines()
If you want to make it a fully-fledged program, type this in:
file = raw_input ("Enter EXACT file name: ")
with open(file) as g:
stuff = g.readlines()
print (stuff)
exit = raw_input("Press enter when you are done.")
For some reason, it doesn't read .py files properly.
add a comment |
A real easy way:
with open(file) as g:
stuff = g.readlines()
If you want to make it a fully-fledged program, type this in:
file = raw_input ("Enter EXACT file name: ")
with open(file) as g:
stuff = g.readlines()
print (stuff)
exit = raw_input("Press enter when you are done.")
For some reason, it doesn't read .py files properly.
add a comment |
A real easy way:
with open(file) as g:
stuff = g.readlines()
If you want to make it a fully-fledged program, type this in:
file = raw_input ("Enter EXACT file name: ")
with open(file) as g:
stuff = g.readlines()
print (stuff)
exit = raw_input("Press enter when you are done.")
For some reason, it doesn't read .py files properly.
A real easy way:
with open(file) as g:
stuff = g.readlines()
If you want to make it a fully-fledged program, type this in:
file = raw_input ("Enter EXACT file name: ")
with open(file) as g:
stuff = g.readlines()
print (stuff)
exit = raw_input("Press enter when you are done.")
For some reason, it doesn't read .py files properly.
edited Nov 26 '17 at 7:58


Giovanni Gianni
6,46611623
6,46611623
answered Mar 27 '16 at 21:29
user6111687
add a comment |
add a comment |
You can just open your file for reading using:
file1 = open("filename","r")
# And for reading use
lines = file1.readlines()
file1.close()
The list lines
will contain all your lines as individual elements, and you can call a specific element using lines["linenumber-1"]
as Python starts its counting from 0.
1
The Pythonic way would be:with open("filename") as f: lines = f.readlines()
.
– Eric O Lebigot
Oct 23 '18 at 10:55
add a comment |
You can just open your file for reading using:
file1 = open("filename","r")
# And for reading use
lines = file1.readlines()
file1.close()
The list lines
will contain all your lines as individual elements, and you can call a specific element using lines["linenumber-1"]
as Python starts its counting from 0.
1
The Pythonic way would be:with open("filename") as f: lines = f.readlines()
.
– Eric O Lebigot
Oct 23 '18 at 10:55
add a comment |
You can just open your file for reading using:
file1 = open("filename","r")
# And for reading use
lines = file1.readlines()
file1.close()
The list lines
will contain all your lines as individual elements, and you can call a specific element using lines["linenumber-1"]
as Python starts its counting from 0.
You can just open your file for reading using:
file1 = open("filename","r")
# And for reading use
lines = file1.readlines()
file1.close()
The list lines
will contain all your lines as individual elements, and you can call a specific element using lines["linenumber-1"]
as Python starts its counting from 0.
edited May 21 '18 at 19:48


Peter Mortensen
13.7k1986111
13.7k1986111
answered May 19 '17 at 10:50
Diksha DhawanDiksha Dhawan
16118
16118
1
The Pythonic way would be:with open("filename") as f: lines = f.readlines()
.
– Eric O Lebigot
Oct 23 '18 at 10:55
add a comment |
1
The Pythonic way would be:with open("filename") as f: lines = f.readlines()
.
– Eric O Lebigot
Oct 23 '18 at 10:55
1
1
The Pythonic way would be:
with open("filename") as f: lines = f.readlines()
.– Eric O Lebigot
Oct 23 '18 at 10:55
The Pythonic way would be:
with open("filename") as f: lines = f.readlines()
.– Eric O Lebigot
Oct 23 '18 at 10:55
add a comment |
If you want to are faced with a very large / huge file and want to read faster (imagine you are in a Topcoder/Hackerrank coding competition), you might read a considerably bigger chunk of lines into a memory buffer at one time, rather than just iterate line by line at file level.
buffersize = 2**16
with open(path) as f:
while True:
lines_buffer = f.readlines(buffersize)
if not lines_buffer:
break
for line in lines_buffer:
process(line)
what does process(line) do? I get an error that there is not such variable defined. I guess something needs importing and I tried to import multiprocessing.Process, but that's not it I guess. Could you please elaborate? Thanks
– Newskooler
Apr 6 '17 at 8:40
1
process(line)
is a function that you need to implement to process the data. for example, instead of that line, if you useprint(line)
, it will print each line from the lines_buffer.
– Khanal
Apr 26 '17 at 13:27
f.readlines(buffersize) returns an immutable buffer. if you want to directly read into your buffer you need to use readinto() function. I will be much faster.
– David Dehghan
Jun 30 '18 at 10:28
add a comment |
If you want to are faced with a very large / huge file and want to read faster (imagine you are in a Topcoder/Hackerrank coding competition), you might read a considerably bigger chunk of lines into a memory buffer at one time, rather than just iterate line by line at file level.
buffersize = 2**16
with open(path) as f:
while True:
lines_buffer = f.readlines(buffersize)
if not lines_buffer:
break
for line in lines_buffer:
process(line)
what does process(line) do? I get an error that there is not such variable defined. I guess something needs importing and I tried to import multiprocessing.Process, but that's not it I guess. Could you please elaborate? Thanks
– Newskooler
Apr 6 '17 at 8:40
1
process(line)
is a function that you need to implement to process the data. for example, instead of that line, if you useprint(line)
, it will print each line from the lines_buffer.
– Khanal
Apr 26 '17 at 13:27
f.readlines(buffersize) returns an immutable buffer. if you want to directly read into your buffer you need to use readinto() function. I will be much faster.
– David Dehghan
Jun 30 '18 at 10:28
add a comment |
If you want to are faced with a very large / huge file and want to read faster (imagine you are in a Topcoder/Hackerrank coding competition), you might read a considerably bigger chunk of lines into a memory buffer at one time, rather than just iterate line by line at file level.
buffersize = 2**16
with open(path) as f:
while True:
lines_buffer = f.readlines(buffersize)
if not lines_buffer:
break
for line in lines_buffer:
process(line)
If you want to are faced with a very large / huge file and want to read faster (imagine you are in a Topcoder/Hackerrank coding competition), you might read a considerably bigger chunk of lines into a memory buffer at one time, rather than just iterate line by line at file level.
buffersize = 2**16
with open(path) as f:
while True:
lines_buffer = f.readlines(buffersize)
if not lines_buffer:
break
for line in lines_buffer:
process(line)
edited Mar 16 '17 at 4:09


Jonathan Leffler
567k916781030
567k916781030
answered Mar 11 '17 at 8:49
pambdapambda
1,2101426
1,2101426
what does process(line) do? I get an error that there is not such variable defined. I guess something needs importing and I tried to import multiprocessing.Process, but that's not it I guess. Could you please elaborate? Thanks
– Newskooler
Apr 6 '17 at 8:40
1
process(line)
is a function that you need to implement to process the data. for example, instead of that line, if you useprint(line)
, it will print each line from the lines_buffer.
– Khanal
Apr 26 '17 at 13:27
f.readlines(buffersize) returns an immutable buffer. if you want to directly read into your buffer you need to use readinto() function. I will be much faster.
– David Dehghan
Jun 30 '18 at 10:28
add a comment |
what does process(line) do? I get an error that there is not such variable defined. I guess something needs importing and I tried to import multiprocessing.Process, but that's not it I guess. Could you please elaborate? Thanks
– Newskooler
Apr 6 '17 at 8:40
1
process(line)
is a function that you need to implement to process the data. for example, instead of that line, if you useprint(line)
, it will print each line from the lines_buffer.
– Khanal
Apr 26 '17 at 13:27
f.readlines(buffersize) returns an immutable buffer. if you want to directly read into your buffer you need to use readinto() function. I will be much faster.
– David Dehghan
Jun 30 '18 at 10:28
what does process(line) do? I get an error that there is not such variable defined. I guess something needs importing and I tried to import multiprocessing.Process, but that's not it I guess. Could you please elaborate? Thanks
– Newskooler
Apr 6 '17 at 8:40
what does process(line) do? I get an error that there is not such variable defined. I guess something needs importing and I tried to import multiprocessing.Process, but that's not it I guess. Could you please elaborate? Thanks
– Newskooler
Apr 6 '17 at 8:40
1
1
process(line)
is a function that you need to implement to process the data. for example, instead of that line, if you use print(line)
, it will print each line from the lines_buffer.– Khanal
Apr 26 '17 at 13:27
process(line)
is a function that you need to implement to process the data. for example, instead of that line, if you use print(line)
, it will print each line from the lines_buffer.– Khanal
Apr 26 '17 at 13:27
f.readlines(buffersize) returns an immutable buffer. if you want to directly read into your buffer you need to use readinto() function. I will be much faster.
– David Dehghan
Jun 30 '18 at 10:28
f.readlines(buffersize) returns an immutable buffer. if you want to directly read into your buffer you need to use readinto() function. I will be much faster.
– David Dehghan
Jun 30 '18 at 10:28
add a comment |
To my knowledge Python doesn't have a native array data structure. But it does support the list data structure which is much simpler to use than an array.
array = #declaring a list with name '**array**'
with open(PATH,'r') as reader :
for line in reader :
array.append(line)
python does have an array (see the standard library'sarray
module), but the question asked for a list.
– Corey Goldberg
Dec 12 '16 at 23:22
add a comment |
To my knowledge Python doesn't have a native array data structure. But it does support the list data structure which is much simpler to use than an array.
array = #declaring a list with name '**array**'
with open(PATH,'r') as reader :
for line in reader :
array.append(line)
python does have an array (see the standard library'sarray
module), but the question asked for a list.
– Corey Goldberg
Dec 12 '16 at 23:22
add a comment |
To my knowledge Python doesn't have a native array data structure. But it does support the list data structure which is much simpler to use than an array.
array = #declaring a list with name '**array**'
with open(PATH,'r') as reader :
for line in reader :
array.append(line)
To my knowledge Python doesn't have a native array data structure. But it does support the list data structure which is much simpler to use than an array.
array = #declaring a list with name '**array**'
with open(PATH,'r') as reader :
for line in reader :
array.append(line)
edited Mar 4 '17 at 21:54


Peter Mortensen
13.7k1986111
13.7k1986111
answered Mar 7 '16 at 6:54


Strik3rStrik3r
814312
814312
python does have an array (see the standard library'sarray
module), but the question asked for a list.
– Corey Goldberg
Dec 12 '16 at 23:22
add a comment |
python does have an array (see the standard library'sarray
module), but the question asked for a list.
– Corey Goldberg
Dec 12 '16 at 23:22
python does have an array (see the standard library's
array
module), but the question asked for a list.– Corey Goldberg
Dec 12 '16 at 23:22
python does have an array (see the standard library's
array
module), but the question asked for a list.– Corey Goldberg
Dec 12 '16 at 23:22
add a comment |
Use this:
import pandas as pd
data = pd.read_csv(filename) # You can also add parameters such as header, sep, etc.
array = data.values
data
is a dataframe type, and uses values to get ndarray. You can also get a list by using array.tolist()
.
add a comment |
Use this:
import pandas as pd
data = pd.read_csv(filename) # You can also add parameters such as header, sep, etc.
array = data.values
data
is a dataframe type, and uses values to get ndarray. You can also get a list by using array.tolist()
.
add a comment |
Use this:
import pandas as pd
data = pd.read_csv(filename) # You can also add parameters such as header, sep, etc.
array = data.values
data
is a dataframe type, and uses values to get ndarray. You can also get a list by using array.tolist()
.
Use this:
import pandas as pd
data = pd.read_csv(filename) # You can also add parameters such as header, sep, etc.
array = data.values
data
is a dataframe type, and uses values to get ndarray. You can also get a list by using array.tolist()
.
edited Mar 4 '17 at 21:56


Peter Mortensen
13.7k1986111
13.7k1986111
answered Mar 30 '16 at 15:50


ZeroZero
68688
68688
add a comment |
add a comment |
You can easily do it by the following piece of code:
lines = open(filePath).readlines()
2
You should use awith
statement though.
– Aran-Fey
Oct 29 '18 at 17:51
add a comment |
You can easily do it by the following piece of code:
lines = open(filePath).readlines()
2
You should use awith
statement though.
– Aran-Fey
Oct 29 '18 at 17:51
add a comment |
You can easily do it by the following piece of code:
lines = open(filePath).readlines()
You can easily do it by the following piece of code:
lines = open(filePath).readlines()
answered Jun 26 '17 at 1:43


PedramPedram
8281425
8281425
2
You should use awith
statement though.
– Aran-Fey
Oct 29 '18 at 17:51
add a comment |
2
You should use awith
statement though.
– Aran-Fey
Oct 29 '18 at 17:51
2
2
You should use a
with
statement though.– Aran-Fey
Oct 29 '18 at 17:51
You should use a
with
statement though.– Aran-Fey
Oct 29 '18 at 17:51
add a comment |
You could also use the loadtxt command in NumPy. This checks for fewer conditions than genfromtxt, so it may be faster.
import numpy
data = numpy.loadtxt(filename, delimiter="n")
add a comment |
You could also use the loadtxt command in NumPy. This checks for fewer conditions than genfromtxt, so it may be faster.
import numpy
data = numpy.loadtxt(filename, delimiter="n")
add a comment |
You could also use the loadtxt command in NumPy. This checks for fewer conditions than genfromtxt, so it may be faster.
import numpy
data = numpy.loadtxt(filename, delimiter="n")
You could also use the loadtxt command in NumPy. This checks for fewer conditions than genfromtxt, so it may be faster.
import numpy
data = numpy.loadtxt(filename, delimiter="n")
edited May 21 '18 at 19:43


Peter Mortensen
13.7k1986111
13.7k1986111
answered Jul 20 '15 at 17:33
asampat3090asampat3090
8915
8915
add a comment |
add a comment |
Check out this short snippet
fileOb=open("filename.txt","r")
data=fileOb.readlines() #returns a array of lines.
or
fileOb=open("filename.txt","r")
data=list(fileOb) #returns a array of lines.
refer docs for reference
add a comment |
Check out this short snippet
fileOb=open("filename.txt","r")
data=fileOb.readlines() #returns a array of lines.
or
fileOb=open("filename.txt","r")
data=list(fileOb) #returns a array of lines.
refer docs for reference
add a comment |
Check out this short snippet
fileOb=open("filename.txt","r")
data=fileOb.readlines() #returns a array of lines.
or
fileOb=open("filename.txt","r")
data=list(fileOb) #returns a array of lines.
refer docs for reference
Check out this short snippet
fileOb=open("filename.txt","r")
data=fileOb.readlines() #returns a array of lines.
or
fileOb=open("filename.txt","r")
data=list(fileOb) #returns a array of lines.
refer docs for reference
answered Nov 6 '18 at 14:35
SeenivasanSeenivasan
7421615
7421615
add a comment |
add a comment |
Outline and Summary
With a filename
, handling the file from a Path(filename)
object, or directly with open(filename) as f
, do one of the following:
list(fileinput.input(filename))
- using
with path.open() as f
, callf.readlines()
list(f)
path.read_text().splitlines()
path.read_text().splitlines(keepends=True)
- iterate over
fileinput.input
orf
andlist.append
each line one at a time - pass
f
to a boundlist.extend
method - use
f
in a list comprehension
I explain the use-case for each below.
In Python, how do I read a file line-by-line?
This is an excellent question. First, let's create some sample data:
from pathlib import Path
Path('filename').write_text('foonbarnbaz')
File objects are lazy iterators, so just iterate over it.
filename = 'filename'
with open(filename) as f:
for line in f:
line # do something with the line
Alternatively, if you have multiple files, use fileinput.input
, another lazy iterator. With just one file:
import fileinput
for line in fileinput.input(filename):
line # process the line
or for multiple files, pass it a list of filenames:
for line in fileinput.input([filename]*2):
line # process the line
Again, f
and fileinput.input
above both are/return lazy iterators.
You can only use an iterator one time, so to provide functional code while avoiding verbosity I'll use the slightly more terse fileinput.input(filename)
where apropos from here.
In Python, how do I read a file line-by-line into a list?
Ah but you want it in a list for some reason? I'd avoid that if possible. But if you insist... just pass the result of fileinput.input(filename)
to list
:
list(fileinput.input(filename))
Another direct answer is to call f.readlines
, which returns the contents of the file (up to an optional hint
number of characters, so you could break this up into multiple lists that way).
You can get to this file object two ways. One way is to pass the filename to the open
builtin:
filename = 'filename'
with open(filename) as f:
f.readlines()
or using the new Path object from the pathlib
module (which I have become quite fond of, and will use from here on):
from pathlib import Path
path = Path(filename)
with path.open() as f:
f.readlines()
list
will also consume the file iterator and return a list - a quite direct method as well:
with path.open() as f:
list(f)
If you don't mind reading the entire text into memory as a single string before splitting it, you can do this as a one-liner with the Path
object and the splitlines()
string method. By default, splitlines
removes the newlines:
path.read_text().splitlines()
If you want to keep the newlines, pass keepends=True
:
path.read_text().splitlines(keepends=True)
I want to read the file line by line and append each line to the end of the list.
Now this is a bit silly to ask for, given that we've demonstrated the end result easily with several methods. But you might need to filter or operate on the lines as you make your list, so let's humor this request.
Using list.append
would allow you to filter or operate on each line before you append it:
line_list =
for line in fileinput.input(filename):
line_list.append(line)
line_list
Using list.extend
would be a bit more direct, and perhaps useful if you have a preexisting list:
line_list =
line_list.extend(fileinput.input(filename))
line_list
Or more idiomatically, we could instead use a list comprehension, and map and filter inside it if desirable:
[line for line in fileinput.input(filename)]
Or even more directly, to close the circle, just pass it to list to create a new list directly without operating on the lines:
list(fileinput.input(filename))
Conclusion
You've seen many ways to get lines from a file into a list, but I'd recommend you avoid materializing large quantities of data into a list and instead use Python's lazy iteration to process the data if possible.
That is, prefer fileinput.input
or with path.open() as f
.
add a comment |
Outline and Summary
With a filename
, handling the file from a Path(filename)
object, or directly with open(filename) as f
, do one of the following:
list(fileinput.input(filename))
- using
with path.open() as f
, callf.readlines()
list(f)
path.read_text().splitlines()
path.read_text().splitlines(keepends=True)
- iterate over
fileinput.input
orf
andlist.append
each line one at a time - pass
f
to a boundlist.extend
method - use
f
in a list comprehension
I explain the use-case for each below.
In Python, how do I read a file line-by-line?
This is an excellent question. First, let's create some sample data:
from pathlib import Path
Path('filename').write_text('foonbarnbaz')
File objects are lazy iterators, so just iterate over it.
filename = 'filename'
with open(filename) as f:
for line in f:
line # do something with the line
Alternatively, if you have multiple files, use fileinput.input
, another lazy iterator. With just one file:
import fileinput
for line in fileinput.input(filename):
line # process the line
or for multiple files, pass it a list of filenames:
for line in fileinput.input([filename]*2):
line # process the line
Again, f
and fileinput.input
above both are/return lazy iterators.
You can only use an iterator one time, so to provide functional code while avoiding verbosity I'll use the slightly more terse fileinput.input(filename)
where apropos from here.
In Python, how do I read a file line-by-line into a list?
Ah but you want it in a list for some reason? I'd avoid that if possible. But if you insist... just pass the result of fileinput.input(filename)
to list
:
list(fileinput.input(filename))
Another direct answer is to call f.readlines
, which returns the contents of the file (up to an optional hint
number of characters, so you could break this up into multiple lists that way).
You can get to this file object two ways. One way is to pass the filename to the open
builtin:
filename = 'filename'
with open(filename) as f:
f.readlines()
or using the new Path object from the pathlib
module (which I have become quite fond of, and will use from here on):
from pathlib import Path
path = Path(filename)
with path.open() as f:
f.readlines()
list
will also consume the file iterator and return a list - a quite direct method as well:
with path.open() as f:
list(f)
If you don't mind reading the entire text into memory as a single string before splitting it, you can do this as a one-liner with the Path
object and the splitlines()
string method. By default, splitlines
removes the newlines:
path.read_text().splitlines()
If you want to keep the newlines, pass keepends=True
:
path.read_text().splitlines(keepends=True)
I want to read the file line by line and append each line to the end of the list.
Now this is a bit silly to ask for, given that we've demonstrated the end result easily with several methods. But you might need to filter or operate on the lines as you make your list, so let's humor this request.
Using list.append
would allow you to filter or operate on each line before you append it:
line_list =
for line in fileinput.input(filename):
line_list.append(line)
line_list
Using list.extend
would be a bit more direct, and perhaps useful if you have a preexisting list:
line_list =
line_list.extend(fileinput.input(filename))
line_list
Or more idiomatically, we could instead use a list comprehension, and map and filter inside it if desirable:
[line for line in fileinput.input(filename)]
Or even more directly, to close the circle, just pass it to list to create a new list directly without operating on the lines:
list(fileinput.input(filename))
Conclusion
You've seen many ways to get lines from a file into a list, but I'd recommend you avoid materializing large quantities of data into a list and instead use Python's lazy iteration to process the data if possible.
That is, prefer fileinput.input
or with path.open() as f
.
add a comment |
Outline and Summary
With a filename
, handling the file from a Path(filename)
object, or directly with open(filename) as f
, do one of the following:
list(fileinput.input(filename))
- using
with path.open() as f
, callf.readlines()
list(f)
path.read_text().splitlines()
path.read_text().splitlines(keepends=True)
- iterate over
fileinput.input
orf
andlist.append
each line one at a time - pass
f
to a boundlist.extend
method - use
f
in a list comprehension
I explain the use-case for each below.
In Python, how do I read a file line-by-line?
This is an excellent question. First, let's create some sample data:
from pathlib import Path
Path('filename').write_text('foonbarnbaz')
File objects are lazy iterators, so just iterate over it.
filename = 'filename'
with open(filename) as f:
for line in f:
line # do something with the line
Alternatively, if you have multiple files, use fileinput.input
, another lazy iterator. With just one file:
import fileinput
for line in fileinput.input(filename):
line # process the line
or for multiple files, pass it a list of filenames:
for line in fileinput.input([filename]*2):
line # process the line
Again, f
and fileinput.input
above both are/return lazy iterators.
You can only use an iterator one time, so to provide functional code while avoiding verbosity I'll use the slightly more terse fileinput.input(filename)
where apropos from here.
In Python, how do I read a file line-by-line into a list?
Ah but you want it in a list for some reason? I'd avoid that if possible. But if you insist... just pass the result of fileinput.input(filename)
to list
:
list(fileinput.input(filename))
Another direct answer is to call f.readlines
, which returns the contents of the file (up to an optional hint
number of characters, so you could break this up into multiple lists that way).
You can get to this file object two ways. One way is to pass the filename to the open
builtin:
filename = 'filename'
with open(filename) as f:
f.readlines()
or using the new Path object from the pathlib
module (which I have become quite fond of, and will use from here on):
from pathlib import Path
path = Path(filename)
with path.open() as f:
f.readlines()
list
will also consume the file iterator and return a list - a quite direct method as well:
with path.open() as f:
list(f)
If you don't mind reading the entire text into memory as a single string before splitting it, you can do this as a one-liner with the Path
object and the splitlines()
string method. By default, splitlines
removes the newlines:
path.read_text().splitlines()
If you want to keep the newlines, pass keepends=True
:
path.read_text().splitlines(keepends=True)
I want to read the file line by line and append each line to the end of the list.
Now this is a bit silly to ask for, given that we've demonstrated the end result easily with several methods. But you might need to filter or operate on the lines as you make your list, so let's humor this request.
Using list.append
would allow you to filter or operate on each line before you append it:
line_list =
for line in fileinput.input(filename):
line_list.append(line)
line_list
Using list.extend
would be a bit more direct, and perhaps useful if you have a preexisting list:
line_list =
line_list.extend(fileinput.input(filename))
line_list
Or more idiomatically, we could instead use a list comprehension, and map and filter inside it if desirable:
[line for line in fileinput.input(filename)]
Or even more directly, to close the circle, just pass it to list to create a new list directly without operating on the lines:
list(fileinput.input(filename))
Conclusion
You've seen many ways to get lines from a file into a list, but I'd recommend you avoid materializing large quantities of data into a list and instead use Python's lazy iteration to process the data if possible.
That is, prefer fileinput.input
or with path.open() as f
.
Outline and Summary
With a filename
, handling the file from a Path(filename)
object, or directly with open(filename) as f
, do one of the following:
list(fileinput.input(filename))
- using
with path.open() as f
, callf.readlines()
list(f)
path.read_text().splitlines()
path.read_text().splitlines(keepends=True)
- iterate over
fileinput.input
orf
andlist.append
each line one at a time - pass
f
to a boundlist.extend
method - use
f
in a list comprehension
I explain the use-case for each below.
In Python, how do I read a file line-by-line?
This is an excellent question. First, let's create some sample data:
from pathlib import Path
Path('filename').write_text('foonbarnbaz')
File objects are lazy iterators, so just iterate over it.
filename = 'filename'
with open(filename) as f:
for line in f:
line # do something with the line
Alternatively, if you have multiple files, use fileinput.input
, another lazy iterator. With just one file:
import fileinput
for line in fileinput.input(filename):
line # process the line
or for multiple files, pass it a list of filenames:
for line in fileinput.input([filename]*2):
line # process the line
Again, f
and fileinput.input
above both are/return lazy iterators.
You can only use an iterator one time, so to provide functional code while avoiding verbosity I'll use the slightly more terse fileinput.input(filename)
where apropos from here.
In Python, how do I read a file line-by-line into a list?
Ah but you want it in a list for some reason? I'd avoid that if possible. But if you insist... just pass the result of fileinput.input(filename)
to list
:
list(fileinput.input(filename))
Another direct answer is to call f.readlines
, which returns the contents of the file (up to an optional hint
number of characters, so you could break this up into multiple lists that way).
You can get to this file object two ways. One way is to pass the filename to the open
builtin:
filename = 'filename'
with open(filename) as f:
f.readlines()
or using the new Path object from the pathlib
module (which I have become quite fond of, and will use from here on):
from pathlib import Path
path = Path(filename)
with path.open() as f:
f.readlines()
list
will also consume the file iterator and return a list - a quite direct method as well:
with path.open() as f:
list(f)
If you don't mind reading the entire text into memory as a single string before splitting it, you can do this as a one-liner with the Path
object and the splitlines()
string method. By default, splitlines
removes the newlines:
path.read_text().splitlines()
If you want to keep the newlines, pass keepends=True
:
path.read_text().splitlines(keepends=True)
I want to read the file line by line and append each line to the end of the list.
Now this is a bit silly to ask for, given that we've demonstrated the end result easily with several methods. But you might need to filter or operate on the lines as you make your list, so let's humor this request.
Using list.append
would allow you to filter or operate on each line before you append it:
line_list =
for line in fileinput.input(filename):
line_list.append(line)
line_list
Using list.extend
would be a bit more direct, and perhaps useful if you have a preexisting list:
line_list =
line_list.extend(fileinput.input(filename))
line_list
Or more idiomatically, we could instead use a list comprehension, and map and filter inside it if desirable:
[line for line in fileinput.input(filename)]
Or even more directly, to close the circle, just pass it to list to create a new list directly without operating on the lines:
list(fileinput.input(filename))
Conclusion
You've seen many ways to get lines from a file into a list, but I'd recommend you avoid materializing large quantities of data into a list and instead use Python's lazy iteration to process the data if possible.
That is, prefer fileinput.input
or with path.open() as f
.
answered May 16 '18 at 20:17


Aaron Hall♦Aaron Hall
176k51305255
176k51305255
add a comment |
add a comment |
Command line version
#!/bin/python3
import os
import sys
abspath = os.path.abspath(__file__)
dname = os.path.dirname(abspath)
filename = dname + sys.argv[1]
arr = open(filename).read().split("n")
print(arr)
Run with:
python3 somefile.py input_file_name.txt
add a comment |
Command line version
#!/bin/python3
import os
import sys
abspath = os.path.abspath(__file__)
dname = os.path.dirname(abspath)
filename = dname + sys.argv[1]
arr = open(filename).read().split("n")
print(arr)
Run with:
python3 somefile.py input_file_name.txt
add a comment |
Command line version
#!/bin/python3
import os
import sys
abspath = os.path.abspath(__file__)
dname = os.path.dirname(abspath)
filename = dname + sys.argv[1]
arr = open(filename).read().split("n")
print(arr)
Run with:
python3 somefile.py input_file_name.txt
Command line version
#!/bin/python3
import os
import sys
abspath = os.path.abspath(__file__)
dname = os.path.dirname(abspath)
filename = dname + sys.argv[1]
arr = open(filename).read().split("n")
print(arr)
Run with:
python3 somefile.py input_file_name.txt
answered Aug 29 '17 at 23:53


jasonleonhardjasonleonhard
1,9222032
1,9222032
add a comment |
add a comment |
1 2
next
xs8tFojBhC4aQ6zTYB,bO,Z5hOB,tZLmu1JaIxHTT8zE9J,IKCfbXoO7D8VTRKgSZ