Why is JPA unable to merge the multiple (but same) results returned by hibernate?
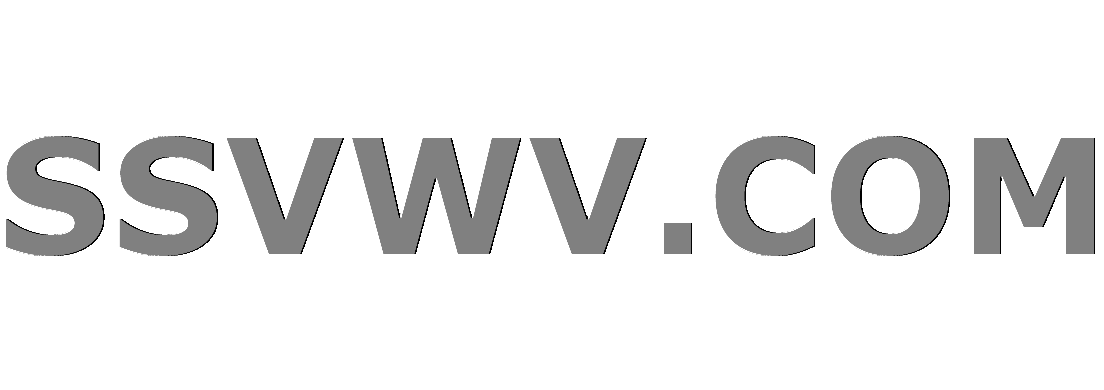
Multi tool use
I am relatively new to hibernate. I am using hibernate with Spring boot 1.5 and I am encountering a strange problem which I have no idea how to fix.
In the image below, you will notice the same email field is repeated.
User Model Object
@Data
@Entity
@Table(name = "user")
@EntityListeners(AuditingEntityListener.class)
@JsonIgnoreProperties(value = {"createdAt", "updatedAt"}, allowGetters = false)
@DynamicUpdate
public class User {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Integer id;
...
private Boolean isActive;
@OneToMany(mappedBy = "user", orphanRemoval = true, cascade = CascadeType.ALL, fetch = FetchType.EAGER)
private List<UserEmail> emails = new ArrayList<>();
@ManyToMany(cascade = CascadeType.ALL, fetch = FetchType.EAGER)
@JoinTable(name = "users_roles",
joinColumns = @JoinColumn(name = "user_id"), inverseJoinColumns = @JoinColumn(name = "role_id"))
private Set<Role> roles;
public void addUserEmail(UserEmail email) {
email.setUser(this);
this.emails.add(email);
}
public void removeUserEmail(UserEmail email) {
emails.remove(email);
email.setUser(null);
}
}
UserEmail Model Object
@Data
@Entity
@EntityListeners(AuditingEntityListener.class)
public class UserEmail {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Integer id;
@ManyToOne
@JoinColumn(name = "user_id")
private User user;
@Email
@NaturalId(mutable = true)
@Column(name = "email", unique = true)
private String email;
}
But in the database, the email is not actually repeated.
The following is the sql query executed when I want to fetch a user:
Hibernate: select user0_.id as id1_2_0_, user0_.created_at as created_2_2_0_,
user0_.first_name as first_na3_2_0_, user0_.is_active as is_activ4_2_0_,
user0_.last_name as last_nam5_2_0_, user0_.password as password6_2_0_,
user0_.phone as phone7_2_0_, user0_.updated_at as updated_8_2_0_,
user0_.username as username9_2_0_, emails1_.user_id as user_id3_4_1_,
emails1_.id as id1_4_1_, emails1_.id as id1_4_2_, emails1_.email as
email2_4_2_, emails1_.user_id as user_id3_4_2_, roles2_.user_id as
user_id1_5_3_, role3_.id as role_id2_5_3_, role3_.id as id1_1_4_, role3_.name
as name2_1_4_ from user user0_ left outer join user_email emails1_ on
user0_.id=emails1_.user_id left outer join users_roles roles2_ on
user0_.id=roles2_.user_id left outer join role role3_ on
roles2_.role_id=role3_.id where user0_.id=1
which gives me the following result
In the picture, we can see that two rows are returned. The sql query constructed by hibernate is somewhat fetching the same email result for each role. I understand (and have cross-checked) that this is happening only in cases of users with more than one role only. Now since the emails are the same, shouldn't hibernate/jpa identify properly and merge the two emails into one?
How do I fix this problem? Any help is appreciated. I understand that my question may not be well-explained so if you have confusions, please ask.
java hibernate spring-boot jpa thymeleaf
add a comment |
I am relatively new to hibernate. I am using hibernate with Spring boot 1.5 and I am encountering a strange problem which I have no idea how to fix.
In the image below, you will notice the same email field is repeated.
User Model Object
@Data
@Entity
@Table(name = "user")
@EntityListeners(AuditingEntityListener.class)
@JsonIgnoreProperties(value = {"createdAt", "updatedAt"}, allowGetters = false)
@DynamicUpdate
public class User {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Integer id;
...
private Boolean isActive;
@OneToMany(mappedBy = "user", orphanRemoval = true, cascade = CascadeType.ALL, fetch = FetchType.EAGER)
private List<UserEmail> emails = new ArrayList<>();
@ManyToMany(cascade = CascadeType.ALL, fetch = FetchType.EAGER)
@JoinTable(name = "users_roles",
joinColumns = @JoinColumn(name = "user_id"), inverseJoinColumns = @JoinColumn(name = "role_id"))
private Set<Role> roles;
public void addUserEmail(UserEmail email) {
email.setUser(this);
this.emails.add(email);
}
public void removeUserEmail(UserEmail email) {
emails.remove(email);
email.setUser(null);
}
}
UserEmail Model Object
@Data
@Entity
@EntityListeners(AuditingEntityListener.class)
public class UserEmail {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Integer id;
@ManyToOne
@JoinColumn(name = "user_id")
private User user;
@Email
@NaturalId(mutable = true)
@Column(name = "email", unique = true)
private String email;
}
But in the database, the email is not actually repeated.
The following is the sql query executed when I want to fetch a user:
Hibernate: select user0_.id as id1_2_0_, user0_.created_at as created_2_2_0_,
user0_.first_name as first_na3_2_0_, user0_.is_active as is_activ4_2_0_,
user0_.last_name as last_nam5_2_0_, user0_.password as password6_2_0_,
user0_.phone as phone7_2_0_, user0_.updated_at as updated_8_2_0_,
user0_.username as username9_2_0_, emails1_.user_id as user_id3_4_1_,
emails1_.id as id1_4_1_, emails1_.id as id1_4_2_, emails1_.email as
email2_4_2_, emails1_.user_id as user_id3_4_2_, roles2_.user_id as
user_id1_5_3_, role3_.id as role_id2_5_3_, role3_.id as id1_1_4_, role3_.name
as name2_1_4_ from user user0_ left outer join user_email emails1_ on
user0_.id=emails1_.user_id left outer join users_roles roles2_ on
user0_.id=roles2_.user_id left outer join role role3_ on
roles2_.role_id=role3_.id where user0_.id=1
which gives me the following result
In the picture, we can see that two rows are returned. The sql query constructed by hibernate is somewhat fetching the same email result for each role. I understand (and have cross-checked) that this is happening only in cases of users with more than one role only. Now since the emails are the same, shouldn't hibernate/jpa identify properly and merge the two emails into one?
How do I fix this problem? Any help is appreciated. I understand that my question may not be well-explained so if you have confusions, please ask.
java hibernate spring-boot jpa thymeleaf
1
Possible duplicate of Getting Duplicate Entries using Hibernate
– Alan Hay
Nov 24 '18 at 10:38
add a comment |
I am relatively new to hibernate. I am using hibernate with Spring boot 1.5 and I am encountering a strange problem which I have no idea how to fix.
In the image below, you will notice the same email field is repeated.
User Model Object
@Data
@Entity
@Table(name = "user")
@EntityListeners(AuditingEntityListener.class)
@JsonIgnoreProperties(value = {"createdAt", "updatedAt"}, allowGetters = false)
@DynamicUpdate
public class User {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Integer id;
...
private Boolean isActive;
@OneToMany(mappedBy = "user", orphanRemoval = true, cascade = CascadeType.ALL, fetch = FetchType.EAGER)
private List<UserEmail> emails = new ArrayList<>();
@ManyToMany(cascade = CascadeType.ALL, fetch = FetchType.EAGER)
@JoinTable(name = "users_roles",
joinColumns = @JoinColumn(name = "user_id"), inverseJoinColumns = @JoinColumn(name = "role_id"))
private Set<Role> roles;
public void addUserEmail(UserEmail email) {
email.setUser(this);
this.emails.add(email);
}
public void removeUserEmail(UserEmail email) {
emails.remove(email);
email.setUser(null);
}
}
UserEmail Model Object
@Data
@Entity
@EntityListeners(AuditingEntityListener.class)
public class UserEmail {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Integer id;
@ManyToOne
@JoinColumn(name = "user_id")
private User user;
@Email
@NaturalId(mutable = true)
@Column(name = "email", unique = true)
private String email;
}
But in the database, the email is not actually repeated.
The following is the sql query executed when I want to fetch a user:
Hibernate: select user0_.id as id1_2_0_, user0_.created_at as created_2_2_0_,
user0_.first_name as first_na3_2_0_, user0_.is_active as is_activ4_2_0_,
user0_.last_name as last_nam5_2_0_, user0_.password as password6_2_0_,
user0_.phone as phone7_2_0_, user0_.updated_at as updated_8_2_0_,
user0_.username as username9_2_0_, emails1_.user_id as user_id3_4_1_,
emails1_.id as id1_4_1_, emails1_.id as id1_4_2_, emails1_.email as
email2_4_2_, emails1_.user_id as user_id3_4_2_, roles2_.user_id as
user_id1_5_3_, role3_.id as role_id2_5_3_, role3_.id as id1_1_4_, role3_.name
as name2_1_4_ from user user0_ left outer join user_email emails1_ on
user0_.id=emails1_.user_id left outer join users_roles roles2_ on
user0_.id=roles2_.user_id left outer join role role3_ on
roles2_.role_id=role3_.id where user0_.id=1
which gives me the following result
In the picture, we can see that two rows are returned. The sql query constructed by hibernate is somewhat fetching the same email result for each role. I understand (and have cross-checked) that this is happening only in cases of users with more than one role only. Now since the emails are the same, shouldn't hibernate/jpa identify properly and merge the two emails into one?
How do I fix this problem? Any help is appreciated. I understand that my question may not be well-explained so if you have confusions, please ask.
java hibernate spring-boot jpa thymeleaf
I am relatively new to hibernate. I am using hibernate with Spring boot 1.5 and I am encountering a strange problem which I have no idea how to fix.
In the image below, you will notice the same email field is repeated.
User Model Object
@Data
@Entity
@Table(name = "user")
@EntityListeners(AuditingEntityListener.class)
@JsonIgnoreProperties(value = {"createdAt", "updatedAt"}, allowGetters = false)
@DynamicUpdate
public class User {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Integer id;
...
private Boolean isActive;
@OneToMany(mappedBy = "user", orphanRemoval = true, cascade = CascadeType.ALL, fetch = FetchType.EAGER)
private List<UserEmail> emails = new ArrayList<>();
@ManyToMany(cascade = CascadeType.ALL, fetch = FetchType.EAGER)
@JoinTable(name = "users_roles",
joinColumns = @JoinColumn(name = "user_id"), inverseJoinColumns = @JoinColumn(name = "role_id"))
private Set<Role> roles;
public void addUserEmail(UserEmail email) {
email.setUser(this);
this.emails.add(email);
}
public void removeUserEmail(UserEmail email) {
emails.remove(email);
email.setUser(null);
}
}
UserEmail Model Object
@Data
@Entity
@EntityListeners(AuditingEntityListener.class)
public class UserEmail {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Integer id;
@ManyToOne
@JoinColumn(name = "user_id")
private User user;
@Email
@NaturalId(mutable = true)
@Column(name = "email", unique = true)
private String email;
}
But in the database, the email is not actually repeated.
The following is the sql query executed when I want to fetch a user:
Hibernate: select user0_.id as id1_2_0_, user0_.created_at as created_2_2_0_,
user0_.first_name as first_na3_2_0_, user0_.is_active as is_activ4_2_0_,
user0_.last_name as last_nam5_2_0_, user0_.password as password6_2_0_,
user0_.phone as phone7_2_0_, user0_.updated_at as updated_8_2_0_,
user0_.username as username9_2_0_, emails1_.user_id as user_id3_4_1_,
emails1_.id as id1_4_1_, emails1_.id as id1_4_2_, emails1_.email as
email2_4_2_, emails1_.user_id as user_id3_4_2_, roles2_.user_id as
user_id1_5_3_, role3_.id as role_id2_5_3_, role3_.id as id1_1_4_, role3_.name
as name2_1_4_ from user user0_ left outer join user_email emails1_ on
user0_.id=emails1_.user_id left outer join users_roles roles2_ on
user0_.id=roles2_.user_id left outer join role role3_ on
roles2_.role_id=role3_.id where user0_.id=1
which gives me the following result
In the picture, we can see that two rows are returned. The sql query constructed by hibernate is somewhat fetching the same email result for each role. I understand (and have cross-checked) that this is happening only in cases of users with more than one role only. Now since the emails are the same, shouldn't hibernate/jpa identify properly and merge the two emails into one?
How do I fix this problem? Any help is appreciated. I understand that my question may not be well-explained so if you have confusions, please ask.
java hibernate spring-boot jpa thymeleaf
java hibernate spring-boot jpa thymeleaf
edited Nov 24 '18 at 10:34


Alan Hay
15.7k22870
15.7k22870
asked Nov 24 '18 at 8:58
sbsattersbsatter
10711
10711
1
Possible duplicate of Getting Duplicate Entries using Hibernate
– Alan Hay
Nov 24 '18 at 10:38
add a comment |
1
Possible duplicate of Getting Duplicate Entries using Hibernate
– Alan Hay
Nov 24 '18 at 10:38
1
1
Possible duplicate of Getting Duplicate Entries using Hibernate
– Alan Hay
Nov 24 '18 at 10:38
Possible duplicate of Getting Duplicate Entries using Hibernate
– Alan Hay
Nov 24 '18 at 10:38
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53456661%2fwhy-is-jpa-unable-to-merge-the-multiple-but-same-results-returned-by-hibernate%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53456661%2fwhy-is-jpa-unable-to-merge-the-multiple-but-same-results-returned-by-hibernate%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ndcMGlyyTBfP s8TT,JgyBTULdT,J9e0,ldT xm8I0YaiqKPJrMnlH4Nz05VzEkFuX2EOAJvqjsw69nqJ2y5LO
1
Possible duplicate of Getting Duplicate Entries using Hibernate
– Alan Hay
Nov 24 '18 at 10:38