WPF custom a movable usercontrol consists of a vertical line and a horizontal line
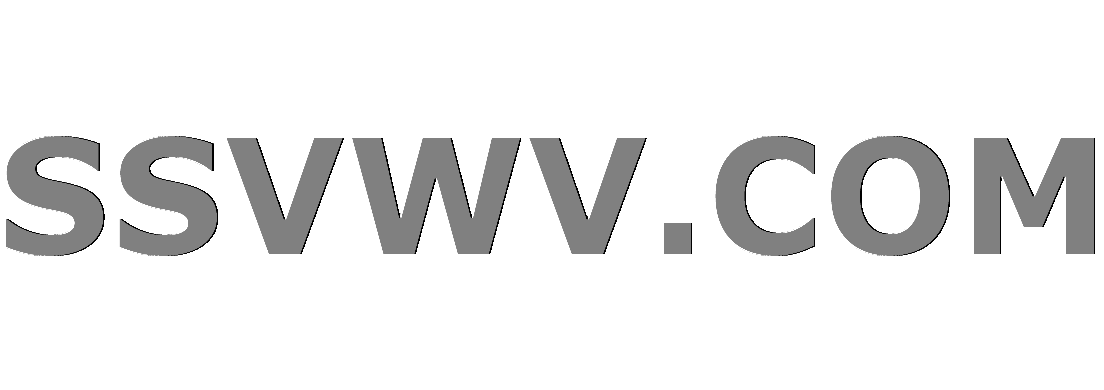
Multi tool use
First,I need to draw a vertical line and a horizontal line. I used the GeometryGroup to realize this.It looks like this
The custom class code:
public class QuadrantGate1 : Shape
{
#region Constructors
/// <summary>
/// Instantiate a new instance of a line.
/// </summary>
public QuadrantGate1()
{
}
#endregion
#region Dynamic Properties
public double VerticalY1
{
get { return (double)GetValue(VerticalY1Property); }
set { SetValue(VerticalY1Property, value); }
}
// Using a DependencyProperty as the backing store for VerticalY1. This enables animation, styling, binding, etc...
public static readonly DependencyProperty VerticalY1Property =
DependencyProperty.Register("VerticalY1", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(0.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double VerticalY2
{
get { return (double)GetValue(VerticalY2Property); }
set { SetValue(VerticalY2Property, value); }
}
// Using a DependencyProperty as the backing store for VerticalY2. This enables animation, styling, binding, etc...
public static readonly DependencyProperty VerticalY2Property =
DependencyProperty.Register("VerticalY2", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(256.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double VerticalX
{
get { return (double)GetValue(VerticalXProperty); }
set { SetValue(VerticalXProperty, value); }
}
// Using a DependencyProperty as the backing store for VerticalX1. This enables animation, styling, binding, etc...
public static readonly DependencyProperty VerticalXProperty =
DependencyProperty.Register("VerticalX", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(128.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double HorizontalX1
{
get { return (double)GetValue(HorizontalX1Property); }
set { SetValue(HorizontalX1Property, value); }
}
// Using a DependencyProperty as the backing store for HorizontalX1. This enables animation, styling, binding, etc...
public static readonly DependencyProperty HorizontalX1Property =
DependencyProperty.Register("HorizontalX1", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(0.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double HorizontalX2
{
get { return (double)GetValue(HorizontalX2Property); }
set { SetValue(HorizontalX2Property, value); }
}
// Using a DependencyProperty as the backing store for HorizontalX2. This enables animation, styling, binding, etc...
public static readonly DependencyProperty HorizontalX2Property =
DependencyProperty.Register("HorizontalX2", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(256.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double HorizontalY
{
get { return (double)GetValue(HorizontalYProperty); }
set { SetValue(HorizontalYProperty, value); }
}
// Using a DependencyProperty as the backing store for HorizontalY. This enables animation, styling, binding, etc...
public static readonly DependencyProperty HorizontalYProperty =
DependencyProperty.Register("HorizontalY", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(128.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
#endregion
#region Protected Methods and Properties
protected override Geometry DefiningGeometry
{
get
{
_geometryGroup = new GeometryGroup();
_geometryGroup.FillRule = FillRule.Nonzero;
DrawTwoLinesGeometry(_geometryGroup);
_path = new Path();
_path.Data = _geometryGroup;
return _geometryGroup;
}
}
private void DrawTwoLinesGeometry(GeometryGroup geometryGroup)
{
try
{
_lineGeometry1 = new LineGeometry()
{
StartPoint = new Point { X = HorizontalX1, Y = HorizontalY },
EndPoint = new Point { X = HorizontalX2, Y = HorizontalY }
};
_lineGeometry2 = new LineGeometry()
{
StartPoint = new Point { X = VerticalX, Y = VerticalY1 },
EndPoint = new Point { X = VerticalX, Y = VerticalY2 }
};
_geometryGroup.Children.Add(_lineGeometry1);
_geometryGroup.Children.Add(_lineGeometry2);
}
catch (Exception e)
{
}
}
#endregion
#region Private Methods and Members
private GeometryGroup _geometryGroup;
private LineGeometry _lineGeometry1;
private LineGeometry _lineGeometry2;
private Path _path;
#endregion
}
The xaml code:
Second,I need to move the two lines. When the mouse is on the horizontal line,the horizontal line can be moved up or down,the vertical line stays still.
When the mouse is on the vertical line,the vertical line can be moved left or right,the horizontal line stays still.
When the mouse is on the cross point of the two lines,both of the two lines can be moved,and the horizontal line moved up and down,the vertical line moved left and right.
I want to move two lines first,so the custom class code:
public class QuadrantGate1 : Shape
{
#region Constructors
/// <summary>
/// Instantiate a new instance of a line.
/// </summary>
public QuadrantGate1()
{
this.MouseDown += QuadrantGate_MouseDown;
this.MouseMove += QuadrantGate_MouseMove;
this.MouseLeftButtonUp += QuadrantGate_MouseLeftButtonUp;
}
#endregion
#region Dynamic Properties
public double VerticalY1
{
get { return (double)GetValue(VerticalY1Property); }
set { SetValue(VerticalY1Property, value); }
}
// Using a DependencyProperty as the backing store for VerticalY1. This enables animation, styling, binding, etc...
public static readonly DependencyProperty VerticalY1Property =
DependencyProperty.Register("VerticalY1", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(0.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double VerticalY2
{
get { return (double)GetValue(VerticalY2Property); }
set { SetValue(VerticalY2Property, value); }
}
// Using a DependencyProperty as the backing store for VerticalY2. This enables animation, styling, binding, etc...
public static readonly DependencyProperty VerticalY2Property =
DependencyProperty.Register("VerticalY2", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(256.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double VerticalX
{
get { return (double)GetValue(VerticalXProperty); }
set { SetValue(VerticalXProperty, value); }
}
// Using a DependencyProperty as the backing store for VerticalX1. This enables animation, styling, binding, etc...
public static readonly DependencyProperty VerticalXProperty =
DependencyProperty.Register("VerticalX", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(128.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double HorizontalX1
{
get { return (double)GetValue(HorizontalX1Property); }
set { SetValue(HorizontalX1Property, value); }
}
// Using a DependencyProperty as the backing store for HorizontalX1. This enables animation, styling, binding, etc...
public static readonly DependencyProperty HorizontalX1Property =
DependencyProperty.Register("HorizontalX1", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(0.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double HorizontalX2
{
get { return (double)GetValue(HorizontalX2Property); }
set { SetValue(HorizontalX2Property, value); }
}
// Using a DependencyProperty as the backing store for HorizontalX2. This enables animation, styling, binding, etc...
public static readonly DependencyProperty HorizontalX2Property =
DependencyProperty.Register("HorizontalX2", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(256.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double HorizontalY
{
get { return (double)GetValue(HorizontalYProperty); }
set { SetValue(HorizontalYProperty, value); }
}
// Using a DependencyProperty as the backing store for HorizontalY. This enables animation, styling, binding, etc...
public static readonly DependencyProperty HorizontalYProperty =
DependencyProperty.Register("HorizontalY", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(128.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
#endregion
#region Protected Methods and Properties
protected override Geometry DefiningGeometry
{
get
{
_geometryGroup = new GeometryGroup();
_geometryGroup.FillRule = FillRule.Nonzero;
DrawTwoLinesGeometry(_geometryGroup);
_path = new Path();
_path.Data = _geometryGroup;
return _geometryGroup;
}
}
private void DrawTwoLinesGeometry(GeometryGroup geometryGroup)
{
try
{
_lineGeometry1 = new LineGeometry()
{
StartPoint = new Point { X = HorizontalX1, Y = HorizontalY },
EndPoint = new Point { X = HorizontalX2, Y = HorizontalY }
};
_lineGeometry2 = new LineGeometry()
{
StartPoint = new Point { X = VerticalX, Y = VerticalY1 },
EndPoint = new Point { X = VerticalX, Y = VerticalY2 }
};
_geometryGroup.Children.Add(_lineGeometry1);
_geometryGroup.Children.Add(_lineGeometry2);
}
catch (Exception e)
{
}
}
#endregion
#region Events Methods
private void QuadrantGate_MouseDown(object sender, MouseButtonEventArgs e)
{
if (e.OriginalSource.GetType() == typeof(QuadrantGate1))
{
this.mouseBefore = e.GetPosition(this);
QuadrantGate1 quadrantGate = (QuadrantGate1)e.OriginalSource;
startBefore.X = VerticalX;
startBefore.Y = HorizontalY;
quadrantGate.CaptureMouse();
}
}
GeometryCollection GeometryGroupChildren(GeometryGroup geometryGroup)
{
GeometryCollection geometries = new GeometryCollection();
if (geometryGroup == null)
return null;
else
{
geometries = geometryGroup.Children;
return geometries;
}
}
private void QuadrantGate_MouseMove(object sender, MouseEventArgs e)
{
if (e.LeftButton == MouseButtonState.Pressed)
{
if (e.OriginalSource != null && e.OriginalSource.GetType() == typeof(QuadrantGate1))
{
QuadrantGate1 quadrantGate = (QuadrantGate1)e.OriginalSource;
Point p = e.GetPosition(this);
VerticalX = startBefore.X + (p.X - mouseBefore.X);
HorizontalY = startBefore.Y + (p.Y - mouseBefore.Y);
}
}
}
private void QuadrantGate_MouseLeftButtonUp(object sender, MouseButtonEventArgs e)
{
if (e.OriginalSource.GetType() == typeof(QuadrantGate1))
{
QuadrantGate1 quadrantGate = (QuadrantGate1)e.OriginalSource;
quadrantGate.ReleaseMouseCapture();
}
}
#endregion
#region Private Methods and Members
private GeometryGroup _geometryGroup;
private LineGeometry _lineGeometry1;
private LineGeometry _lineGeometry2;
private Path _path;
Point mouseBefore;
Point startBefore;
#endregion
}
But the two lines don't move as I want.The movement of lines is inconsistent with mouse.
The strange part is if one of these lines _geometryGroup.Children.Add(_lineGeometry1);
or this _geometryGroup.Children.Add(_lineGeometry2);
is commented out, the rest line can be moved sucessfully. This is the horizontal line:
,
and the vertical line:
c# wpf
add a comment |
First,I need to draw a vertical line and a horizontal line. I used the GeometryGroup to realize this.It looks like this
The custom class code:
public class QuadrantGate1 : Shape
{
#region Constructors
/// <summary>
/// Instantiate a new instance of a line.
/// </summary>
public QuadrantGate1()
{
}
#endregion
#region Dynamic Properties
public double VerticalY1
{
get { return (double)GetValue(VerticalY1Property); }
set { SetValue(VerticalY1Property, value); }
}
// Using a DependencyProperty as the backing store for VerticalY1. This enables animation, styling, binding, etc...
public static readonly DependencyProperty VerticalY1Property =
DependencyProperty.Register("VerticalY1", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(0.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double VerticalY2
{
get { return (double)GetValue(VerticalY2Property); }
set { SetValue(VerticalY2Property, value); }
}
// Using a DependencyProperty as the backing store for VerticalY2. This enables animation, styling, binding, etc...
public static readonly DependencyProperty VerticalY2Property =
DependencyProperty.Register("VerticalY2", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(256.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double VerticalX
{
get { return (double)GetValue(VerticalXProperty); }
set { SetValue(VerticalXProperty, value); }
}
// Using a DependencyProperty as the backing store for VerticalX1. This enables animation, styling, binding, etc...
public static readonly DependencyProperty VerticalXProperty =
DependencyProperty.Register("VerticalX", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(128.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double HorizontalX1
{
get { return (double)GetValue(HorizontalX1Property); }
set { SetValue(HorizontalX1Property, value); }
}
// Using a DependencyProperty as the backing store for HorizontalX1. This enables animation, styling, binding, etc...
public static readonly DependencyProperty HorizontalX1Property =
DependencyProperty.Register("HorizontalX1", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(0.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double HorizontalX2
{
get { return (double)GetValue(HorizontalX2Property); }
set { SetValue(HorizontalX2Property, value); }
}
// Using a DependencyProperty as the backing store for HorizontalX2. This enables animation, styling, binding, etc...
public static readonly DependencyProperty HorizontalX2Property =
DependencyProperty.Register("HorizontalX2", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(256.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double HorizontalY
{
get { return (double)GetValue(HorizontalYProperty); }
set { SetValue(HorizontalYProperty, value); }
}
// Using a DependencyProperty as the backing store for HorizontalY. This enables animation, styling, binding, etc...
public static readonly DependencyProperty HorizontalYProperty =
DependencyProperty.Register("HorizontalY", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(128.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
#endregion
#region Protected Methods and Properties
protected override Geometry DefiningGeometry
{
get
{
_geometryGroup = new GeometryGroup();
_geometryGroup.FillRule = FillRule.Nonzero;
DrawTwoLinesGeometry(_geometryGroup);
_path = new Path();
_path.Data = _geometryGroup;
return _geometryGroup;
}
}
private void DrawTwoLinesGeometry(GeometryGroup geometryGroup)
{
try
{
_lineGeometry1 = new LineGeometry()
{
StartPoint = new Point { X = HorizontalX1, Y = HorizontalY },
EndPoint = new Point { X = HorizontalX2, Y = HorizontalY }
};
_lineGeometry2 = new LineGeometry()
{
StartPoint = new Point { X = VerticalX, Y = VerticalY1 },
EndPoint = new Point { X = VerticalX, Y = VerticalY2 }
};
_geometryGroup.Children.Add(_lineGeometry1);
_geometryGroup.Children.Add(_lineGeometry2);
}
catch (Exception e)
{
}
}
#endregion
#region Private Methods and Members
private GeometryGroup _geometryGroup;
private LineGeometry _lineGeometry1;
private LineGeometry _lineGeometry2;
private Path _path;
#endregion
}
The xaml code:
Second,I need to move the two lines. When the mouse is on the horizontal line,the horizontal line can be moved up or down,the vertical line stays still.
When the mouse is on the vertical line,the vertical line can be moved left or right,the horizontal line stays still.
When the mouse is on the cross point of the two lines,both of the two lines can be moved,and the horizontal line moved up and down,the vertical line moved left and right.
I want to move two lines first,so the custom class code:
public class QuadrantGate1 : Shape
{
#region Constructors
/// <summary>
/// Instantiate a new instance of a line.
/// </summary>
public QuadrantGate1()
{
this.MouseDown += QuadrantGate_MouseDown;
this.MouseMove += QuadrantGate_MouseMove;
this.MouseLeftButtonUp += QuadrantGate_MouseLeftButtonUp;
}
#endregion
#region Dynamic Properties
public double VerticalY1
{
get { return (double)GetValue(VerticalY1Property); }
set { SetValue(VerticalY1Property, value); }
}
// Using a DependencyProperty as the backing store for VerticalY1. This enables animation, styling, binding, etc...
public static readonly DependencyProperty VerticalY1Property =
DependencyProperty.Register("VerticalY1", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(0.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double VerticalY2
{
get { return (double)GetValue(VerticalY2Property); }
set { SetValue(VerticalY2Property, value); }
}
// Using a DependencyProperty as the backing store for VerticalY2. This enables animation, styling, binding, etc...
public static readonly DependencyProperty VerticalY2Property =
DependencyProperty.Register("VerticalY2", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(256.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double VerticalX
{
get { return (double)GetValue(VerticalXProperty); }
set { SetValue(VerticalXProperty, value); }
}
// Using a DependencyProperty as the backing store for VerticalX1. This enables animation, styling, binding, etc...
public static readonly DependencyProperty VerticalXProperty =
DependencyProperty.Register("VerticalX", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(128.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double HorizontalX1
{
get { return (double)GetValue(HorizontalX1Property); }
set { SetValue(HorizontalX1Property, value); }
}
// Using a DependencyProperty as the backing store for HorizontalX1. This enables animation, styling, binding, etc...
public static readonly DependencyProperty HorizontalX1Property =
DependencyProperty.Register("HorizontalX1", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(0.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double HorizontalX2
{
get { return (double)GetValue(HorizontalX2Property); }
set { SetValue(HorizontalX2Property, value); }
}
// Using a DependencyProperty as the backing store for HorizontalX2. This enables animation, styling, binding, etc...
public static readonly DependencyProperty HorizontalX2Property =
DependencyProperty.Register("HorizontalX2", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(256.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double HorizontalY
{
get { return (double)GetValue(HorizontalYProperty); }
set { SetValue(HorizontalYProperty, value); }
}
// Using a DependencyProperty as the backing store for HorizontalY. This enables animation, styling, binding, etc...
public static readonly DependencyProperty HorizontalYProperty =
DependencyProperty.Register("HorizontalY", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(128.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
#endregion
#region Protected Methods and Properties
protected override Geometry DefiningGeometry
{
get
{
_geometryGroup = new GeometryGroup();
_geometryGroup.FillRule = FillRule.Nonzero;
DrawTwoLinesGeometry(_geometryGroup);
_path = new Path();
_path.Data = _geometryGroup;
return _geometryGroup;
}
}
private void DrawTwoLinesGeometry(GeometryGroup geometryGroup)
{
try
{
_lineGeometry1 = new LineGeometry()
{
StartPoint = new Point { X = HorizontalX1, Y = HorizontalY },
EndPoint = new Point { X = HorizontalX2, Y = HorizontalY }
};
_lineGeometry2 = new LineGeometry()
{
StartPoint = new Point { X = VerticalX, Y = VerticalY1 },
EndPoint = new Point { X = VerticalX, Y = VerticalY2 }
};
_geometryGroup.Children.Add(_lineGeometry1);
_geometryGroup.Children.Add(_lineGeometry2);
}
catch (Exception e)
{
}
}
#endregion
#region Events Methods
private void QuadrantGate_MouseDown(object sender, MouseButtonEventArgs e)
{
if (e.OriginalSource.GetType() == typeof(QuadrantGate1))
{
this.mouseBefore = e.GetPosition(this);
QuadrantGate1 quadrantGate = (QuadrantGate1)e.OriginalSource;
startBefore.X = VerticalX;
startBefore.Y = HorizontalY;
quadrantGate.CaptureMouse();
}
}
GeometryCollection GeometryGroupChildren(GeometryGroup geometryGroup)
{
GeometryCollection geometries = new GeometryCollection();
if (geometryGroup == null)
return null;
else
{
geometries = geometryGroup.Children;
return geometries;
}
}
private void QuadrantGate_MouseMove(object sender, MouseEventArgs e)
{
if (e.LeftButton == MouseButtonState.Pressed)
{
if (e.OriginalSource != null && e.OriginalSource.GetType() == typeof(QuadrantGate1))
{
QuadrantGate1 quadrantGate = (QuadrantGate1)e.OriginalSource;
Point p = e.GetPosition(this);
VerticalX = startBefore.X + (p.X - mouseBefore.X);
HorizontalY = startBefore.Y + (p.Y - mouseBefore.Y);
}
}
}
private void QuadrantGate_MouseLeftButtonUp(object sender, MouseButtonEventArgs e)
{
if (e.OriginalSource.GetType() == typeof(QuadrantGate1))
{
QuadrantGate1 quadrantGate = (QuadrantGate1)e.OriginalSource;
quadrantGate.ReleaseMouseCapture();
}
}
#endregion
#region Private Methods and Members
private GeometryGroup _geometryGroup;
private LineGeometry _lineGeometry1;
private LineGeometry _lineGeometry2;
private Path _path;
Point mouseBefore;
Point startBefore;
#endregion
}
But the two lines don't move as I want.The movement of lines is inconsistent with mouse.
The strange part is if one of these lines _geometryGroup.Children.Add(_lineGeometry1);
or this _geometryGroup.Children.Add(_lineGeometry2);
is commented out, the rest line can be moved sucessfully. This is the horizontal line:
,
and the vertical line:
c# wpf
add a comment |
First,I need to draw a vertical line and a horizontal line. I used the GeometryGroup to realize this.It looks like this
The custom class code:
public class QuadrantGate1 : Shape
{
#region Constructors
/// <summary>
/// Instantiate a new instance of a line.
/// </summary>
public QuadrantGate1()
{
}
#endregion
#region Dynamic Properties
public double VerticalY1
{
get { return (double)GetValue(VerticalY1Property); }
set { SetValue(VerticalY1Property, value); }
}
// Using a DependencyProperty as the backing store for VerticalY1. This enables animation, styling, binding, etc...
public static readonly DependencyProperty VerticalY1Property =
DependencyProperty.Register("VerticalY1", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(0.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double VerticalY2
{
get { return (double)GetValue(VerticalY2Property); }
set { SetValue(VerticalY2Property, value); }
}
// Using a DependencyProperty as the backing store for VerticalY2. This enables animation, styling, binding, etc...
public static readonly DependencyProperty VerticalY2Property =
DependencyProperty.Register("VerticalY2", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(256.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double VerticalX
{
get { return (double)GetValue(VerticalXProperty); }
set { SetValue(VerticalXProperty, value); }
}
// Using a DependencyProperty as the backing store for VerticalX1. This enables animation, styling, binding, etc...
public static readonly DependencyProperty VerticalXProperty =
DependencyProperty.Register("VerticalX", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(128.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double HorizontalX1
{
get { return (double)GetValue(HorizontalX1Property); }
set { SetValue(HorizontalX1Property, value); }
}
// Using a DependencyProperty as the backing store for HorizontalX1. This enables animation, styling, binding, etc...
public static readonly DependencyProperty HorizontalX1Property =
DependencyProperty.Register("HorizontalX1", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(0.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double HorizontalX2
{
get { return (double)GetValue(HorizontalX2Property); }
set { SetValue(HorizontalX2Property, value); }
}
// Using a DependencyProperty as the backing store for HorizontalX2. This enables animation, styling, binding, etc...
public static readonly DependencyProperty HorizontalX2Property =
DependencyProperty.Register("HorizontalX2", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(256.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double HorizontalY
{
get { return (double)GetValue(HorizontalYProperty); }
set { SetValue(HorizontalYProperty, value); }
}
// Using a DependencyProperty as the backing store for HorizontalY. This enables animation, styling, binding, etc...
public static readonly DependencyProperty HorizontalYProperty =
DependencyProperty.Register("HorizontalY", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(128.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
#endregion
#region Protected Methods and Properties
protected override Geometry DefiningGeometry
{
get
{
_geometryGroup = new GeometryGroup();
_geometryGroup.FillRule = FillRule.Nonzero;
DrawTwoLinesGeometry(_geometryGroup);
_path = new Path();
_path.Data = _geometryGroup;
return _geometryGroup;
}
}
private void DrawTwoLinesGeometry(GeometryGroup geometryGroup)
{
try
{
_lineGeometry1 = new LineGeometry()
{
StartPoint = new Point { X = HorizontalX1, Y = HorizontalY },
EndPoint = new Point { X = HorizontalX2, Y = HorizontalY }
};
_lineGeometry2 = new LineGeometry()
{
StartPoint = new Point { X = VerticalX, Y = VerticalY1 },
EndPoint = new Point { X = VerticalX, Y = VerticalY2 }
};
_geometryGroup.Children.Add(_lineGeometry1);
_geometryGroup.Children.Add(_lineGeometry2);
}
catch (Exception e)
{
}
}
#endregion
#region Private Methods and Members
private GeometryGroup _geometryGroup;
private LineGeometry _lineGeometry1;
private LineGeometry _lineGeometry2;
private Path _path;
#endregion
}
The xaml code:
Second,I need to move the two lines. When the mouse is on the horizontal line,the horizontal line can be moved up or down,the vertical line stays still.
When the mouse is on the vertical line,the vertical line can be moved left or right,the horizontal line stays still.
When the mouse is on the cross point of the two lines,both of the two lines can be moved,and the horizontal line moved up and down,the vertical line moved left and right.
I want to move two lines first,so the custom class code:
public class QuadrantGate1 : Shape
{
#region Constructors
/// <summary>
/// Instantiate a new instance of a line.
/// </summary>
public QuadrantGate1()
{
this.MouseDown += QuadrantGate_MouseDown;
this.MouseMove += QuadrantGate_MouseMove;
this.MouseLeftButtonUp += QuadrantGate_MouseLeftButtonUp;
}
#endregion
#region Dynamic Properties
public double VerticalY1
{
get { return (double)GetValue(VerticalY1Property); }
set { SetValue(VerticalY1Property, value); }
}
// Using a DependencyProperty as the backing store for VerticalY1. This enables animation, styling, binding, etc...
public static readonly DependencyProperty VerticalY1Property =
DependencyProperty.Register("VerticalY1", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(0.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double VerticalY2
{
get { return (double)GetValue(VerticalY2Property); }
set { SetValue(VerticalY2Property, value); }
}
// Using a DependencyProperty as the backing store for VerticalY2. This enables animation, styling, binding, etc...
public static readonly DependencyProperty VerticalY2Property =
DependencyProperty.Register("VerticalY2", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(256.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double VerticalX
{
get { return (double)GetValue(VerticalXProperty); }
set { SetValue(VerticalXProperty, value); }
}
// Using a DependencyProperty as the backing store for VerticalX1. This enables animation, styling, binding, etc...
public static readonly DependencyProperty VerticalXProperty =
DependencyProperty.Register("VerticalX", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(128.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double HorizontalX1
{
get { return (double)GetValue(HorizontalX1Property); }
set { SetValue(HorizontalX1Property, value); }
}
// Using a DependencyProperty as the backing store for HorizontalX1. This enables animation, styling, binding, etc...
public static readonly DependencyProperty HorizontalX1Property =
DependencyProperty.Register("HorizontalX1", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(0.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double HorizontalX2
{
get { return (double)GetValue(HorizontalX2Property); }
set { SetValue(HorizontalX2Property, value); }
}
// Using a DependencyProperty as the backing store for HorizontalX2. This enables animation, styling, binding, etc...
public static readonly DependencyProperty HorizontalX2Property =
DependencyProperty.Register("HorizontalX2", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(256.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double HorizontalY
{
get { return (double)GetValue(HorizontalYProperty); }
set { SetValue(HorizontalYProperty, value); }
}
// Using a DependencyProperty as the backing store for HorizontalY. This enables animation, styling, binding, etc...
public static readonly DependencyProperty HorizontalYProperty =
DependencyProperty.Register("HorizontalY", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(128.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
#endregion
#region Protected Methods and Properties
protected override Geometry DefiningGeometry
{
get
{
_geometryGroup = new GeometryGroup();
_geometryGroup.FillRule = FillRule.Nonzero;
DrawTwoLinesGeometry(_geometryGroup);
_path = new Path();
_path.Data = _geometryGroup;
return _geometryGroup;
}
}
private void DrawTwoLinesGeometry(GeometryGroup geometryGroup)
{
try
{
_lineGeometry1 = new LineGeometry()
{
StartPoint = new Point { X = HorizontalX1, Y = HorizontalY },
EndPoint = new Point { X = HorizontalX2, Y = HorizontalY }
};
_lineGeometry2 = new LineGeometry()
{
StartPoint = new Point { X = VerticalX, Y = VerticalY1 },
EndPoint = new Point { X = VerticalX, Y = VerticalY2 }
};
_geometryGroup.Children.Add(_lineGeometry1);
_geometryGroup.Children.Add(_lineGeometry2);
}
catch (Exception e)
{
}
}
#endregion
#region Events Methods
private void QuadrantGate_MouseDown(object sender, MouseButtonEventArgs e)
{
if (e.OriginalSource.GetType() == typeof(QuadrantGate1))
{
this.mouseBefore = e.GetPosition(this);
QuadrantGate1 quadrantGate = (QuadrantGate1)e.OriginalSource;
startBefore.X = VerticalX;
startBefore.Y = HorizontalY;
quadrantGate.CaptureMouse();
}
}
GeometryCollection GeometryGroupChildren(GeometryGroup geometryGroup)
{
GeometryCollection geometries = new GeometryCollection();
if (geometryGroup == null)
return null;
else
{
geometries = geometryGroup.Children;
return geometries;
}
}
private void QuadrantGate_MouseMove(object sender, MouseEventArgs e)
{
if (e.LeftButton == MouseButtonState.Pressed)
{
if (e.OriginalSource != null && e.OriginalSource.GetType() == typeof(QuadrantGate1))
{
QuadrantGate1 quadrantGate = (QuadrantGate1)e.OriginalSource;
Point p = e.GetPosition(this);
VerticalX = startBefore.X + (p.X - mouseBefore.X);
HorizontalY = startBefore.Y + (p.Y - mouseBefore.Y);
}
}
}
private void QuadrantGate_MouseLeftButtonUp(object sender, MouseButtonEventArgs e)
{
if (e.OriginalSource.GetType() == typeof(QuadrantGate1))
{
QuadrantGate1 quadrantGate = (QuadrantGate1)e.OriginalSource;
quadrantGate.ReleaseMouseCapture();
}
}
#endregion
#region Private Methods and Members
private GeometryGroup _geometryGroup;
private LineGeometry _lineGeometry1;
private LineGeometry _lineGeometry2;
private Path _path;
Point mouseBefore;
Point startBefore;
#endregion
}
But the two lines don't move as I want.The movement of lines is inconsistent with mouse.
The strange part is if one of these lines _geometryGroup.Children.Add(_lineGeometry1);
or this _geometryGroup.Children.Add(_lineGeometry2);
is commented out, the rest line can be moved sucessfully. This is the horizontal line:
,
and the vertical line:
c# wpf
First,I need to draw a vertical line and a horizontal line. I used the GeometryGroup to realize this.It looks like this
The custom class code:
public class QuadrantGate1 : Shape
{
#region Constructors
/// <summary>
/// Instantiate a new instance of a line.
/// </summary>
public QuadrantGate1()
{
}
#endregion
#region Dynamic Properties
public double VerticalY1
{
get { return (double)GetValue(VerticalY1Property); }
set { SetValue(VerticalY1Property, value); }
}
// Using a DependencyProperty as the backing store for VerticalY1. This enables animation, styling, binding, etc...
public static readonly DependencyProperty VerticalY1Property =
DependencyProperty.Register("VerticalY1", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(0.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double VerticalY2
{
get { return (double)GetValue(VerticalY2Property); }
set { SetValue(VerticalY2Property, value); }
}
// Using a DependencyProperty as the backing store for VerticalY2. This enables animation, styling, binding, etc...
public static readonly DependencyProperty VerticalY2Property =
DependencyProperty.Register("VerticalY2", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(256.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double VerticalX
{
get { return (double)GetValue(VerticalXProperty); }
set { SetValue(VerticalXProperty, value); }
}
// Using a DependencyProperty as the backing store for VerticalX1. This enables animation, styling, binding, etc...
public static readonly DependencyProperty VerticalXProperty =
DependencyProperty.Register("VerticalX", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(128.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double HorizontalX1
{
get { return (double)GetValue(HorizontalX1Property); }
set { SetValue(HorizontalX1Property, value); }
}
// Using a DependencyProperty as the backing store for HorizontalX1. This enables animation, styling, binding, etc...
public static readonly DependencyProperty HorizontalX1Property =
DependencyProperty.Register("HorizontalX1", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(0.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double HorizontalX2
{
get { return (double)GetValue(HorizontalX2Property); }
set { SetValue(HorizontalX2Property, value); }
}
// Using a DependencyProperty as the backing store for HorizontalX2. This enables animation, styling, binding, etc...
public static readonly DependencyProperty HorizontalX2Property =
DependencyProperty.Register("HorizontalX2", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(256.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double HorizontalY
{
get { return (double)GetValue(HorizontalYProperty); }
set { SetValue(HorizontalYProperty, value); }
}
// Using a DependencyProperty as the backing store for HorizontalY. This enables animation, styling, binding, etc...
public static readonly DependencyProperty HorizontalYProperty =
DependencyProperty.Register("HorizontalY", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(128.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
#endregion
#region Protected Methods and Properties
protected override Geometry DefiningGeometry
{
get
{
_geometryGroup = new GeometryGroup();
_geometryGroup.FillRule = FillRule.Nonzero;
DrawTwoLinesGeometry(_geometryGroup);
_path = new Path();
_path.Data = _geometryGroup;
return _geometryGroup;
}
}
private void DrawTwoLinesGeometry(GeometryGroup geometryGroup)
{
try
{
_lineGeometry1 = new LineGeometry()
{
StartPoint = new Point { X = HorizontalX1, Y = HorizontalY },
EndPoint = new Point { X = HorizontalX2, Y = HorizontalY }
};
_lineGeometry2 = new LineGeometry()
{
StartPoint = new Point { X = VerticalX, Y = VerticalY1 },
EndPoint = new Point { X = VerticalX, Y = VerticalY2 }
};
_geometryGroup.Children.Add(_lineGeometry1);
_geometryGroup.Children.Add(_lineGeometry2);
}
catch (Exception e)
{
}
}
#endregion
#region Private Methods and Members
private GeometryGroup _geometryGroup;
private LineGeometry _lineGeometry1;
private LineGeometry _lineGeometry2;
private Path _path;
#endregion
}
The xaml code:
Second,I need to move the two lines. When the mouse is on the horizontal line,the horizontal line can be moved up or down,the vertical line stays still.
When the mouse is on the vertical line,the vertical line can be moved left or right,the horizontal line stays still.
When the mouse is on the cross point of the two lines,both of the two lines can be moved,and the horizontal line moved up and down,the vertical line moved left and right.
I want to move two lines first,so the custom class code:
public class QuadrantGate1 : Shape
{
#region Constructors
/// <summary>
/// Instantiate a new instance of a line.
/// </summary>
public QuadrantGate1()
{
this.MouseDown += QuadrantGate_MouseDown;
this.MouseMove += QuadrantGate_MouseMove;
this.MouseLeftButtonUp += QuadrantGate_MouseLeftButtonUp;
}
#endregion
#region Dynamic Properties
public double VerticalY1
{
get { return (double)GetValue(VerticalY1Property); }
set { SetValue(VerticalY1Property, value); }
}
// Using a DependencyProperty as the backing store for VerticalY1. This enables animation, styling, binding, etc...
public static readonly DependencyProperty VerticalY1Property =
DependencyProperty.Register("VerticalY1", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(0.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double VerticalY2
{
get { return (double)GetValue(VerticalY2Property); }
set { SetValue(VerticalY2Property, value); }
}
// Using a DependencyProperty as the backing store for VerticalY2. This enables animation, styling, binding, etc...
public static readonly DependencyProperty VerticalY2Property =
DependencyProperty.Register("VerticalY2", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(256.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double VerticalX
{
get { return (double)GetValue(VerticalXProperty); }
set { SetValue(VerticalXProperty, value); }
}
// Using a DependencyProperty as the backing store for VerticalX1. This enables animation, styling, binding, etc...
public static readonly DependencyProperty VerticalXProperty =
DependencyProperty.Register("VerticalX", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(128.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double HorizontalX1
{
get { return (double)GetValue(HorizontalX1Property); }
set { SetValue(HorizontalX1Property, value); }
}
// Using a DependencyProperty as the backing store for HorizontalX1. This enables animation, styling, binding, etc...
public static readonly DependencyProperty HorizontalX1Property =
DependencyProperty.Register("HorizontalX1", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(0.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double HorizontalX2
{
get { return (double)GetValue(HorizontalX2Property); }
set { SetValue(HorizontalX2Property, value); }
}
// Using a DependencyProperty as the backing store for HorizontalX2. This enables animation, styling, binding, etc...
public static readonly DependencyProperty HorizontalX2Property =
DependencyProperty.Register("HorizontalX2", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(256.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
public double HorizontalY
{
get { return (double)GetValue(HorizontalYProperty); }
set { SetValue(HorizontalYProperty, value); }
}
// Using a DependencyProperty as the backing store for HorizontalY. This enables animation, styling, binding, etc...
public static readonly DependencyProperty HorizontalYProperty =
DependencyProperty.Register("HorizontalY", typeof(double), typeof(QuadrantGate1), new FrameworkPropertyMetadata(128.0, FrameworkPropertyMetadataOptions.AffectsMeasure));
#endregion
#region Protected Methods and Properties
protected override Geometry DefiningGeometry
{
get
{
_geometryGroup = new GeometryGroup();
_geometryGroup.FillRule = FillRule.Nonzero;
DrawTwoLinesGeometry(_geometryGroup);
_path = new Path();
_path.Data = _geometryGroup;
return _geometryGroup;
}
}
private void DrawTwoLinesGeometry(GeometryGroup geometryGroup)
{
try
{
_lineGeometry1 = new LineGeometry()
{
StartPoint = new Point { X = HorizontalX1, Y = HorizontalY },
EndPoint = new Point { X = HorizontalX2, Y = HorizontalY }
};
_lineGeometry2 = new LineGeometry()
{
StartPoint = new Point { X = VerticalX, Y = VerticalY1 },
EndPoint = new Point { X = VerticalX, Y = VerticalY2 }
};
_geometryGroup.Children.Add(_lineGeometry1);
_geometryGroup.Children.Add(_lineGeometry2);
}
catch (Exception e)
{
}
}
#endregion
#region Events Methods
private void QuadrantGate_MouseDown(object sender, MouseButtonEventArgs e)
{
if (e.OriginalSource.GetType() == typeof(QuadrantGate1))
{
this.mouseBefore = e.GetPosition(this);
QuadrantGate1 quadrantGate = (QuadrantGate1)e.OriginalSource;
startBefore.X = VerticalX;
startBefore.Y = HorizontalY;
quadrantGate.CaptureMouse();
}
}
GeometryCollection GeometryGroupChildren(GeometryGroup geometryGroup)
{
GeometryCollection geometries = new GeometryCollection();
if (geometryGroup == null)
return null;
else
{
geometries = geometryGroup.Children;
return geometries;
}
}
private void QuadrantGate_MouseMove(object sender, MouseEventArgs e)
{
if (e.LeftButton == MouseButtonState.Pressed)
{
if (e.OriginalSource != null && e.OriginalSource.GetType() == typeof(QuadrantGate1))
{
QuadrantGate1 quadrantGate = (QuadrantGate1)e.OriginalSource;
Point p = e.GetPosition(this);
VerticalX = startBefore.X + (p.X - mouseBefore.X);
HorizontalY = startBefore.Y + (p.Y - mouseBefore.Y);
}
}
}
private void QuadrantGate_MouseLeftButtonUp(object sender, MouseButtonEventArgs e)
{
if (e.OriginalSource.GetType() == typeof(QuadrantGate1))
{
QuadrantGate1 quadrantGate = (QuadrantGate1)e.OriginalSource;
quadrantGate.ReleaseMouseCapture();
}
}
#endregion
#region Private Methods and Members
private GeometryGroup _geometryGroup;
private LineGeometry _lineGeometry1;
private LineGeometry _lineGeometry2;
private Path _path;
Point mouseBefore;
Point startBefore;
#endregion
}
But the two lines don't move as I want.The movement of lines is inconsistent with mouse.
The strange part is if one of these lines _geometryGroup.Children.Add(_lineGeometry1);
or this _geometryGroup.Children.Add(_lineGeometry2);
is commented out, the rest line can be moved sucessfully. This is the horizontal line:
,
and the vertical line:
c# wpf
c# wpf
edited Nov 24 '18 at 9:11


Foo
1
1
asked Nov 24 '18 at 3:41
user10697764user10697764
1
1
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
By implementing your code, I see render issue and you could also see if you just do the mouse down & minimize the screen, open it again you can see the line moved to a different location.
Instead of GeometryGroup
, could you try StreamGeometryContext, below is the code DefiningGeometry get Property.
protected override Geometry DefiningGeometry
{
get
{
StreamGeometry geometry = new StreamGeometry();
geometry.FillRule = FillRule.EvenOdd;
using (StreamGeometryContext ctx = geometry.Open())
{
ctx.BeginFigure(new Point(HorizontalX1, HorizontalY), true /* is filled */, true /* is closed */);
ctx.LineTo(new Point(HorizontalX2, HorizontalY), true /* is stroked */, false /* is smooth join */);
ctx.BeginFigure(new Point(VerticalX, VerticalY1), true /* is filled */, true /* is closed */);
ctx.LineTo(new Point(VerticalX, VerticalY2), true /* is stroked */, false /* is smooth join */);
}
//geometry.Freeze();
_path = new Path();
_path.Data = geometry;
return geometry;
}
}
Thanks, but I couldn't understand the first paragraph. Why not the control can move smoothly?
– user10697764
Nov 26 '18 at 3:46
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53454969%2fwpf-custom-a-movable-usercontrol-consists-of-a-vertical-line-and-a-horizontal-li%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
By implementing your code, I see render issue and you could also see if you just do the mouse down & minimize the screen, open it again you can see the line moved to a different location.
Instead of GeometryGroup
, could you try StreamGeometryContext, below is the code DefiningGeometry get Property.
protected override Geometry DefiningGeometry
{
get
{
StreamGeometry geometry = new StreamGeometry();
geometry.FillRule = FillRule.EvenOdd;
using (StreamGeometryContext ctx = geometry.Open())
{
ctx.BeginFigure(new Point(HorizontalX1, HorizontalY), true /* is filled */, true /* is closed */);
ctx.LineTo(new Point(HorizontalX2, HorizontalY), true /* is stroked */, false /* is smooth join */);
ctx.BeginFigure(new Point(VerticalX, VerticalY1), true /* is filled */, true /* is closed */);
ctx.LineTo(new Point(VerticalX, VerticalY2), true /* is stroked */, false /* is smooth join */);
}
//geometry.Freeze();
_path = new Path();
_path.Data = geometry;
return geometry;
}
}
Thanks, but I couldn't understand the first paragraph. Why not the control can move smoothly?
– user10697764
Nov 26 '18 at 3:46
add a comment |
By implementing your code, I see render issue and you could also see if you just do the mouse down & minimize the screen, open it again you can see the line moved to a different location.
Instead of GeometryGroup
, could you try StreamGeometryContext, below is the code DefiningGeometry get Property.
protected override Geometry DefiningGeometry
{
get
{
StreamGeometry geometry = new StreamGeometry();
geometry.FillRule = FillRule.EvenOdd;
using (StreamGeometryContext ctx = geometry.Open())
{
ctx.BeginFigure(new Point(HorizontalX1, HorizontalY), true /* is filled */, true /* is closed */);
ctx.LineTo(new Point(HorizontalX2, HorizontalY), true /* is stroked */, false /* is smooth join */);
ctx.BeginFigure(new Point(VerticalX, VerticalY1), true /* is filled */, true /* is closed */);
ctx.LineTo(new Point(VerticalX, VerticalY2), true /* is stroked */, false /* is smooth join */);
}
//geometry.Freeze();
_path = new Path();
_path.Data = geometry;
return geometry;
}
}
Thanks, but I couldn't understand the first paragraph. Why not the control can move smoothly?
– user10697764
Nov 26 '18 at 3:46
add a comment |
By implementing your code, I see render issue and you could also see if you just do the mouse down & minimize the screen, open it again you can see the line moved to a different location.
Instead of GeometryGroup
, could you try StreamGeometryContext, below is the code DefiningGeometry get Property.
protected override Geometry DefiningGeometry
{
get
{
StreamGeometry geometry = new StreamGeometry();
geometry.FillRule = FillRule.EvenOdd;
using (StreamGeometryContext ctx = geometry.Open())
{
ctx.BeginFigure(new Point(HorizontalX1, HorizontalY), true /* is filled */, true /* is closed */);
ctx.LineTo(new Point(HorizontalX2, HorizontalY), true /* is stroked */, false /* is smooth join */);
ctx.BeginFigure(new Point(VerticalX, VerticalY1), true /* is filled */, true /* is closed */);
ctx.LineTo(new Point(VerticalX, VerticalY2), true /* is stroked */, false /* is smooth join */);
}
//geometry.Freeze();
_path = new Path();
_path.Data = geometry;
return geometry;
}
}
By implementing your code, I see render issue and you could also see if you just do the mouse down & minimize the screen, open it again you can see the line moved to a different location.
Instead of GeometryGroup
, could you try StreamGeometryContext, below is the code DefiningGeometry get Property.
protected override Geometry DefiningGeometry
{
get
{
StreamGeometry geometry = new StreamGeometry();
geometry.FillRule = FillRule.EvenOdd;
using (StreamGeometryContext ctx = geometry.Open())
{
ctx.BeginFigure(new Point(HorizontalX1, HorizontalY), true /* is filled */, true /* is closed */);
ctx.LineTo(new Point(HorizontalX2, HorizontalY), true /* is stroked */, false /* is smooth join */);
ctx.BeginFigure(new Point(VerticalX, VerticalY1), true /* is filled */, true /* is closed */);
ctx.LineTo(new Point(VerticalX, VerticalY2), true /* is stroked */, false /* is smooth join */);
}
//geometry.Freeze();
_path = new Path();
_path.Data = geometry;
return geometry;
}
}
answered Nov 24 '18 at 8:35
Satish PaiSatish Pai
7841510
7841510
Thanks, but I couldn't understand the first paragraph. Why not the control can move smoothly?
– user10697764
Nov 26 '18 at 3:46
add a comment |
Thanks, but I couldn't understand the first paragraph. Why not the control can move smoothly?
– user10697764
Nov 26 '18 at 3:46
Thanks, but I couldn't understand the first paragraph. Why not the control can move smoothly?
– user10697764
Nov 26 '18 at 3:46
Thanks, but I couldn't understand the first paragraph. Why not the control can move smoothly?
– user10697764
Nov 26 '18 at 3:46
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53454969%2fwpf-custom-a-movable-usercontrol-consists-of-a-vertical-line-and-a-horizontal-li%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
YEZ3OORUZIujEO EF1uVyGPNm3XciSH3,34dL675A5FlDH3O2 ZVcVri4aq7yQ