Creating an Authentication Header in ExpressJS . Translation of Java to Javascript
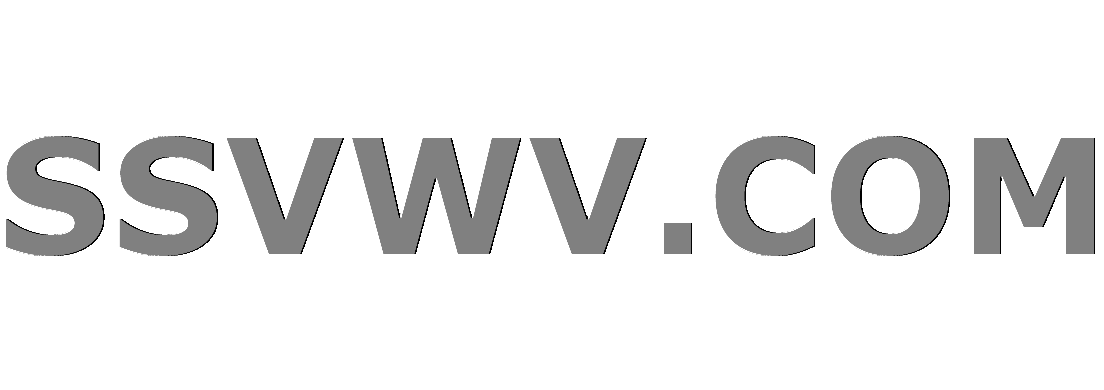
Multi tool use
for the authentication of my Node Server (Express JS) with another API a separate header must be sent within the POST request.
Quote from the documentation:
You need to send a X-Authorization-Ahoi header with this request. The
header value is an encoded JSON string that consists of the
<INSTALLATION_ID>
, a random 16-character string as nonce and the
current date in ISO 8601 format.
Example from the documentation:
{
"installationId":"<INSTALLATION_ID>",
"nonce":"0wYWLarLDBrWU7B2I1Go4A==",
"timestamp":"2018-11-01T11:32:44.413Z"
}
In the documentation of the API the following Java example is given which I try to reproduce in Javascript.
String installationId = <INSTALLATION_ID>;
// create nonce
byte nonce = new byte[16];
SecureRandom.getInstanceStrong().nextBytes(nonce);
String nonceBase64Enc = Base64.getEncoder().encodeToString(nonce);
// create timestamp
String timeStr = Instant.now().toString();
// create json string
String xAuthAhoiJson = String.format("{"installationId":"%s","nonce":"%s","timestamp":"%s"}",
installationId, nonceBase64Enc, timeStr);
// encode encrypted header value
String XAUTH_AHOI_BASE64_URL_ENC = Base64.getUrlEncoder().withoutPadding()
.encodeToString(xAuthAhoiJson.getBytes( StandardCharsets.UTF_8 ));
Here's what I've been trying to do:
const installationId = '<EXAMPLE_INSTALLATIONID>';
const nonce_tmp = crypto.randomBytes(16);
const nonce = nonce_tmp.toString('base64');
const date = new Date();
const timestamp = date.toISOString();
const xAuthAhoiJson = JSON.stringify({installationId, nonce, timestamp});
const XAUTH_AHOI_BASE64_URL_ENC = base64url.encode(xAuthAhoiJson);
const url = 'https://banking-sandbox.starfinanz.de/auth/v1/oauth/token?grant_type=client_credentials';
const options = { headers:
{ 'Authorization': 'Basic ' + Buffer.from(credentials).toString('base64'),
'X-Authorization-Ahoi': XAUTH_AHOI_BASE64_URL_ENC }
};
request.post(url, options, function (e, r, b) { console.log(b); });
For the POST request I use the request-package.
For the encoding in Base64 URL Safe I use the base64url-package.
When executing the POST request I get the following message:
{
"timestamp": 1543229480764,
"status": 400,
"error": "Bad Request",
"message": "Nonce is invalid.",
"path": "/auth/v1/oauth/token"
}
Now my question is: What is wrong with creating my Nonce and how do I fix it?
Or is it the API I'm sending the POST request to?
javascript java express post header
add a comment |
for the authentication of my Node Server (Express JS) with another API a separate header must be sent within the POST request.
Quote from the documentation:
You need to send a X-Authorization-Ahoi header with this request. The
header value is an encoded JSON string that consists of the
<INSTALLATION_ID>
, a random 16-character string as nonce and the
current date in ISO 8601 format.
Example from the documentation:
{
"installationId":"<INSTALLATION_ID>",
"nonce":"0wYWLarLDBrWU7B2I1Go4A==",
"timestamp":"2018-11-01T11:32:44.413Z"
}
In the documentation of the API the following Java example is given which I try to reproduce in Javascript.
String installationId = <INSTALLATION_ID>;
// create nonce
byte nonce = new byte[16];
SecureRandom.getInstanceStrong().nextBytes(nonce);
String nonceBase64Enc = Base64.getEncoder().encodeToString(nonce);
// create timestamp
String timeStr = Instant.now().toString();
// create json string
String xAuthAhoiJson = String.format("{"installationId":"%s","nonce":"%s","timestamp":"%s"}",
installationId, nonceBase64Enc, timeStr);
// encode encrypted header value
String XAUTH_AHOI_BASE64_URL_ENC = Base64.getUrlEncoder().withoutPadding()
.encodeToString(xAuthAhoiJson.getBytes( StandardCharsets.UTF_8 ));
Here's what I've been trying to do:
const installationId = '<EXAMPLE_INSTALLATIONID>';
const nonce_tmp = crypto.randomBytes(16);
const nonce = nonce_tmp.toString('base64');
const date = new Date();
const timestamp = date.toISOString();
const xAuthAhoiJson = JSON.stringify({installationId, nonce, timestamp});
const XAUTH_AHOI_BASE64_URL_ENC = base64url.encode(xAuthAhoiJson);
const url = 'https://banking-sandbox.starfinanz.de/auth/v1/oauth/token?grant_type=client_credentials';
const options = { headers:
{ 'Authorization': 'Basic ' + Buffer.from(credentials).toString('base64'),
'X-Authorization-Ahoi': XAUTH_AHOI_BASE64_URL_ENC }
};
request.post(url, options, function (e, r, b) { console.log(b); });
For the POST request I use the request-package.
For the encoding in Base64 URL Safe I use the base64url-package.
When executing the POST request I get the following message:
{
"timestamp": 1543229480764,
"status": 400,
"error": "Bad Request",
"message": "Nonce is invalid.",
"path": "/auth/v1/oauth/token"
}
Now my question is: What is wrong with creating my Nonce and how do I fix it?
Or is it the API I'm sending the POST request to?
javascript java express post header
add a comment |
for the authentication of my Node Server (Express JS) with another API a separate header must be sent within the POST request.
Quote from the documentation:
You need to send a X-Authorization-Ahoi header with this request. The
header value is an encoded JSON string that consists of the
<INSTALLATION_ID>
, a random 16-character string as nonce and the
current date in ISO 8601 format.
Example from the documentation:
{
"installationId":"<INSTALLATION_ID>",
"nonce":"0wYWLarLDBrWU7B2I1Go4A==",
"timestamp":"2018-11-01T11:32:44.413Z"
}
In the documentation of the API the following Java example is given which I try to reproduce in Javascript.
String installationId = <INSTALLATION_ID>;
// create nonce
byte nonce = new byte[16];
SecureRandom.getInstanceStrong().nextBytes(nonce);
String nonceBase64Enc = Base64.getEncoder().encodeToString(nonce);
// create timestamp
String timeStr = Instant.now().toString();
// create json string
String xAuthAhoiJson = String.format("{"installationId":"%s","nonce":"%s","timestamp":"%s"}",
installationId, nonceBase64Enc, timeStr);
// encode encrypted header value
String XAUTH_AHOI_BASE64_URL_ENC = Base64.getUrlEncoder().withoutPadding()
.encodeToString(xAuthAhoiJson.getBytes( StandardCharsets.UTF_8 ));
Here's what I've been trying to do:
const installationId = '<EXAMPLE_INSTALLATIONID>';
const nonce_tmp = crypto.randomBytes(16);
const nonce = nonce_tmp.toString('base64');
const date = new Date();
const timestamp = date.toISOString();
const xAuthAhoiJson = JSON.stringify({installationId, nonce, timestamp});
const XAUTH_AHOI_BASE64_URL_ENC = base64url.encode(xAuthAhoiJson);
const url = 'https://banking-sandbox.starfinanz.de/auth/v1/oauth/token?grant_type=client_credentials';
const options = { headers:
{ 'Authorization': 'Basic ' + Buffer.from(credentials).toString('base64'),
'X-Authorization-Ahoi': XAUTH_AHOI_BASE64_URL_ENC }
};
request.post(url, options, function (e, r, b) { console.log(b); });
For the POST request I use the request-package.
For the encoding in Base64 URL Safe I use the base64url-package.
When executing the POST request I get the following message:
{
"timestamp": 1543229480764,
"status": 400,
"error": "Bad Request",
"message": "Nonce is invalid.",
"path": "/auth/v1/oauth/token"
}
Now my question is: What is wrong with creating my Nonce and how do I fix it?
Or is it the API I'm sending the POST request to?
javascript java express post header
for the authentication of my Node Server (Express JS) with another API a separate header must be sent within the POST request.
Quote from the documentation:
You need to send a X-Authorization-Ahoi header with this request. The
header value is an encoded JSON string that consists of the
<INSTALLATION_ID>
, a random 16-character string as nonce and the
current date in ISO 8601 format.
Example from the documentation:
{
"installationId":"<INSTALLATION_ID>",
"nonce":"0wYWLarLDBrWU7B2I1Go4A==",
"timestamp":"2018-11-01T11:32:44.413Z"
}
In the documentation of the API the following Java example is given which I try to reproduce in Javascript.
String installationId = <INSTALLATION_ID>;
// create nonce
byte nonce = new byte[16];
SecureRandom.getInstanceStrong().nextBytes(nonce);
String nonceBase64Enc = Base64.getEncoder().encodeToString(nonce);
// create timestamp
String timeStr = Instant.now().toString();
// create json string
String xAuthAhoiJson = String.format("{"installationId":"%s","nonce":"%s","timestamp":"%s"}",
installationId, nonceBase64Enc, timeStr);
// encode encrypted header value
String XAUTH_AHOI_BASE64_URL_ENC = Base64.getUrlEncoder().withoutPadding()
.encodeToString(xAuthAhoiJson.getBytes( StandardCharsets.UTF_8 ));
Here's what I've been trying to do:
const installationId = '<EXAMPLE_INSTALLATIONID>';
const nonce_tmp = crypto.randomBytes(16);
const nonce = nonce_tmp.toString('base64');
const date = new Date();
const timestamp = date.toISOString();
const xAuthAhoiJson = JSON.stringify({installationId, nonce, timestamp});
const XAUTH_AHOI_BASE64_URL_ENC = base64url.encode(xAuthAhoiJson);
const url = 'https://banking-sandbox.starfinanz.de/auth/v1/oauth/token?grant_type=client_credentials';
const options = { headers:
{ 'Authorization': 'Basic ' + Buffer.from(credentials).toString('base64'),
'X-Authorization-Ahoi': XAUTH_AHOI_BASE64_URL_ENC }
};
request.post(url, options, function (e, r, b) { console.log(b); });
For the POST request I use the request-package.
For the encoding in Base64 URL Safe I use the base64url-package.
When executing the POST request I get the following message:
{
"timestamp": 1543229480764,
"status": 400,
"error": "Bad Request",
"message": "Nonce is invalid.",
"path": "/auth/v1/oauth/token"
}
Now my question is: What is wrong with creating my Nonce and how do I fix it?
Or is it the API I'm sending the POST request to?
javascript java express post header
javascript java express post header
edited Nov 26 '18 at 14:20
ChrisP
asked Nov 26 '18 at 12:12
ChrisPChrisP
185
185
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
You just forgot to convert the nonceBase64Enc
to string. You have done that in java but not in javascript.
Just add the .toString('hex');
at the end of nonceBase64Enc
Example : const nonceBase64Enc = Buffer.from(nonce, 'base64').toString('hex');
You are done now! 🎉🍾
That's exactly what solved my problem. Thank you very much!
– ChrisP
Nov 26 '18 at 15:32
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53480894%2fcreating-an-authentication-header-in-expressjs-translation-of-java-to-javascri%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You just forgot to convert the nonceBase64Enc
to string. You have done that in java but not in javascript.
Just add the .toString('hex');
at the end of nonceBase64Enc
Example : const nonceBase64Enc = Buffer.from(nonce, 'base64').toString('hex');
You are done now! 🎉🍾
That's exactly what solved my problem. Thank you very much!
– ChrisP
Nov 26 '18 at 15:32
add a comment |
You just forgot to convert the nonceBase64Enc
to string. You have done that in java but not in javascript.
Just add the .toString('hex');
at the end of nonceBase64Enc
Example : const nonceBase64Enc = Buffer.from(nonce, 'base64').toString('hex');
You are done now! 🎉🍾
That's exactly what solved my problem. Thank you very much!
– ChrisP
Nov 26 '18 at 15:32
add a comment |
You just forgot to convert the nonceBase64Enc
to string. You have done that in java but not in javascript.
Just add the .toString('hex');
at the end of nonceBase64Enc
Example : const nonceBase64Enc = Buffer.from(nonce, 'base64').toString('hex');
You are done now! 🎉🍾
You just forgot to convert the nonceBase64Enc
to string. You have done that in java but not in javascript.
Just add the .toString('hex');
at the end of nonceBase64Enc
Example : const nonceBase64Enc = Buffer.from(nonce, 'base64').toString('hex');
You are done now! 🎉🍾
answered Nov 26 '18 at 14:31


Hemil PatelHemil Patel
1707
1707
That's exactly what solved my problem. Thank you very much!
– ChrisP
Nov 26 '18 at 15:32
add a comment |
That's exactly what solved my problem. Thank you very much!
– ChrisP
Nov 26 '18 at 15:32
That's exactly what solved my problem. Thank you very much!
– ChrisP
Nov 26 '18 at 15:32
That's exactly what solved my problem. Thank you very much!
– ChrisP
Nov 26 '18 at 15:32
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53480894%2fcreating-an-authentication-header-in-expressjs-translation-of-java-to-javascri%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
FMDOTnRP,wHzNYu,V NdDO9wf HkJX5btmcl9uXD7JQFHMen,X,93kbozNtHlAB6oO2B,z5coY