Remove all articles and other strings from a string using Go?
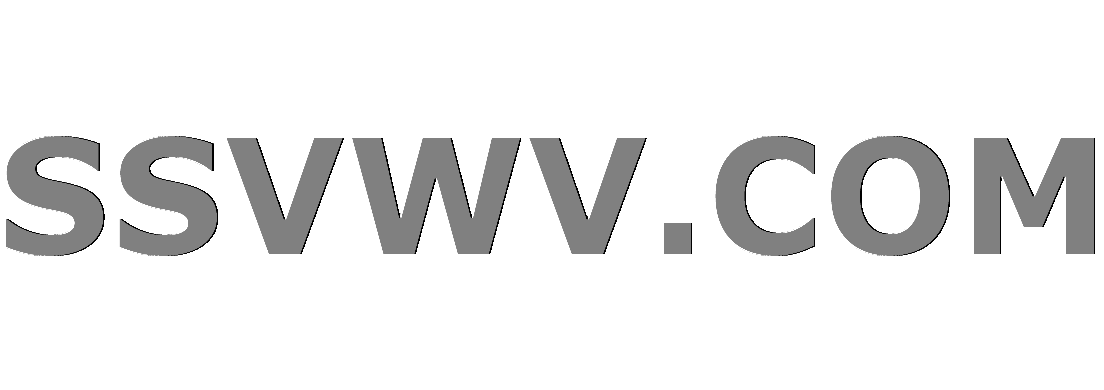
Multi tool use
Is there any method in Go or having regular expression that it will remove only the articles used in the string?
I have tried below code that will do it but it will also remove other words from the string I'm showing the code below:
removalString := "This is a string"
stringToRemove := string{"a", "an", "the", "is"}
for _, wordToRemove := range stringToRemove {
removalString = strings.Replace(removalString, wordToRemove, "", -1)
}
space := regexp.MustCompile(`s+`)
trimedExtraSpaces := space.ReplaceAllString(removalString, " ")
spacesCovertedtoDashes := strings.Replace(trimedExtraSpaces, " ", "-", -1)
slug := strings.ToLower(spacesCovertedtoDashes)
fmt.Println(slug)
Edited
Play link
In this It will remove the is
which is used in the this
.
The Expected output is this-string
regex string go
add a comment |
Is there any method in Go or having regular expression that it will remove only the articles used in the string?
I have tried below code that will do it but it will also remove other words from the string I'm showing the code below:
removalString := "This is a string"
stringToRemove := string{"a", "an", "the", "is"}
for _, wordToRemove := range stringToRemove {
removalString = strings.Replace(removalString, wordToRemove, "", -1)
}
space := regexp.MustCompile(`s+`)
trimedExtraSpaces := space.ReplaceAllString(removalString, " ")
spacesCovertedtoDashes := strings.Replace(trimedExtraSpaces, " ", "-", -1)
slug := strings.ToLower(spacesCovertedtoDashes)
fmt.Println(slug)
Edited
Play link
In this It will remove the is
which is used in the this
.
The Expected output is this-string
regex string go
If your request is for an existing implementation, that is off-topic, as resource requests are not allowed here. If your question is how to improve or fix your current code, you need to be more specific: What problems are you facing? What help do you need?
– Flimzy
Nov 26 '18 at 11:23
1
Show examples of input and expected output and what fails.
– Poul Bak
Nov 26 '18 at 11:24
1
Also note that "is" is not an article, it's a verb.
– Flimzy
Nov 26 '18 at 11:25
add a comment |
Is there any method in Go or having regular expression that it will remove only the articles used in the string?
I have tried below code that will do it but it will also remove other words from the string I'm showing the code below:
removalString := "This is a string"
stringToRemove := string{"a", "an", "the", "is"}
for _, wordToRemove := range stringToRemove {
removalString = strings.Replace(removalString, wordToRemove, "", -1)
}
space := regexp.MustCompile(`s+`)
trimedExtraSpaces := space.ReplaceAllString(removalString, " ")
spacesCovertedtoDashes := strings.Replace(trimedExtraSpaces, " ", "-", -1)
slug := strings.ToLower(spacesCovertedtoDashes)
fmt.Println(slug)
Edited
Play link
In this It will remove the is
which is used in the this
.
The Expected output is this-string
regex string go
Is there any method in Go or having regular expression that it will remove only the articles used in the string?
I have tried below code that will do it but it will also remove other words from the string I'm showing the code below:
removalString := "This is a string"
stringToRemove := string{"a", "an", "the", "is"}
for _, wordToRemove := range stringToRemove {
removalString = strings.Replace(removalString, wordToRemove, "", -1)
}
space := regexp.MustCompile(`s+`)
trimedExtraSpaces := space.ReplaceAllString(removalString, " ")
spacesCovertedtoDashes := strings.Replace(trimedExtraSpaces, " ", "-", -1)
slug := strings.ToLower(spacesCovertedtoDashes)
fmt.Println(slug)
Edited
Play link
In this It will remove the is
which is used in the this
.
The Expected output is this-string
regex string go
regex string go
edited Nov 26 '18 at 11:31
puneet55667788
asked Nov 26 '18 at 11:12
puneet55667788puneet55667788
417
417
If your request is for an existing implementation, that is off-topic, as resource requests are not allowed here. If your question is how to improve or fix your current code, you need to be more specific: What problems are you facing? What help do you need?
– Flimzy
Nov 26 '18 at 11:23
1
Show examples of input and expected output and what fails.
– Poul Bak
Nov 26 '18 at 11:24
1
Also note that "is" is not an article, it's a verb.
– Flimzy
Nov 26 '18 at 11:25
add a comment |
If your request is for an existing implementation, that is off-topic, as resource requests are not allowed here. If your question is how to improve or fix your current code, you need to be more specific: What problems are you facing? What help do you need?
– Flimzy
Nov 26 '18 at 11:23
1
Show examples of input and expected output and what fails.
– Poul Bak
Nov 26 '18 at 11:24
1
Also note that "is" is not an article, it's a verb.
– Flimzy
Nov 26 '18 at 11:25
If your request is for an existing implementation, that is off-topic, as resource requests are not allowed here. If your question is how to improve or fix your current code, you need to be more specific: What problems are you facing? What help do you need?
– Flimzy
Nov 26 '18 at 11:23
If your request is for an existing implementation, that is off-topic, as resource requests are not allowed here. If your question is how to improve or fix your current code, you need to be more specific: What problems are you facing? What help do you need?
– Flimzy
Nov 26 '18 at 11:23
1
1
Show examples of input and expected output and what fails.
– Poul Bak
Nov 26 '18 at 11:24
Show examples of input and expected output and what fails.
– Poul Bak
Nov 26 '18 at 11:24
1
1
Also note that "is" is not an article, it's a verb.
– Flimzy
Nov 26 '18 at 11:25
Also note that "is" is not an article, it's a verb.
– Flimzy
Nov 26 '18 at 11:25
add a comment |
2 Answers
2
active
oldest
votes
You can use strings.Split
and strings.Join
plus a loop for filtering and then building it together again:
removalString := "This is a string"
stringToRemove := string{"a", "an", "the", "is"}
filteredStrings := make(string, 0)
for _, w := range strings.Split(removalString, " ") {
shouldAppend := true
lowered := strings.ToLower(w)
for _, w2 := range stringToRemove {
if lowered == w2 {
shouldAppend = false
break
}
}
if shouldAppend {
filteredStrings = append(filteredStrings, lowered)
}
}
resultString := strings.Join(filteredStrings, "-")
fmt.Printf(resultString)
Outpus:
this-string
Program exited.
Here you have the live example
add a comment |
My version just using regexp
Construct a regexp of the form 'bab|banb|btheb|bisb|' which will find
the words in the list that have "word boundaries" on both sides - so "This" is not matched
Second regexp reduces any spaces to dashes and makes multiple spaces a single dash
package main
import (
"bytes"
"fmt"
"regexp"
)
func main() {
removalString := "This is a strange string"
stringToRemove := string{"a", "an", "the", "is"}
var reg bytes.Buffer
for _, x := range stringToRemove {
reg.WriteString(`b`) // word boundary
reg.WriteString(x)
reg.WriteString(`b`)
reg.WriteString(`|`) // alternation operator
}
regx := regexp.MustCompile(reg.String())
slug := regx.ReplaceAllString(removalString, "")
regx2 := regexp.MustCompile(` +`)
slug = regx2.ReplaceAllString(slug, "-")
fmt.Println(slug)
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53479885%2fremove-all-articles-and-other-strings-from-a-string-using-go%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can use strings.Split
and strings.Join
plus a loop for filtering and then building it together again:
removalString := "This is a string"
stringToRemove := string{"a", "an", "the", "is"}
filteredStrings := make(string, 0)
for _, w := range strings.Split(removalString, " ") {
shouldAppend := true
lowered := strings.ToLower(w)
for _, w2 := range stringToRemove {
if lowered == w2 {
shouldAppend = false
break
}
}
if shouldAppend {
filteredStrings = append(filteredStrings, lowered)
}
}
resultString := strings.Join(filteredStrings, "-")
fmt.Printf(resultString)
Outpus:
this-string
Program exited.
Here you have the live example
add a comment |
You can use strings.Split
and strings.Join
plus a loop for filtering and then building it together again:
removalString := "This is a string"
stringToRemove := string{"a", "an", "the", "is"}
filteredStrings := make(string, 0)
for _, w := range strings.Split(removalString, " ") {
shouldAppend := true
lowered := strings.ToLower(w)
for _, w2 := range stringToRemove {
if lowered == w2 {
shouldAppend = false
break
}
}
if shouldAppend {
filteredStrings = append(filteredStrings, lowered)
}
}
resultString := strings.Join(filteredStrings, "-")
fmt.Printf(resultString)
Outpus:
this-string
Program exited.
Here you have the live example
add a comment |
You can use strings.Split
and strings.Join
plus a loop for filtering and then building it together again:
removalString := "This is a string"
stringToRemove := string{"a", "an", "the", "is"}
filteredStrings := make(string, 0)
for _, w := range strings.Split(removalString, " ") {
shouldAppend := true
lowered := strings.ToLower(w)
for _, w2 := range stringToRemove {
if lowered == w2 {
shouldAppend = false
break
}
}
if shouldAppend {
filteredStrings = append(filteredStrings, lowered)
}
}
resultString := strings.Join(filteredStrings, "-")
fmt.Printf(resultString)
Outpus:
this-string
Program exited.
Here you have the live example
You can use strings.Split
and strings.Join
plus a loop for filtering and then building it together again:
removalString := "This is a string"
stringToRemove := string{"a", "an", "the", "is"}
filteredStrings := make(string, 0)
for _, w := range strings.Split(removalString, " ") {
shouldAppend := true
lowered := strings.ToLower(w)
for _, w2 := range stringToRemove {
if lowered == w2 {
shouldAppend = false
break
}
}
if shouldAppend {
filteredStrings = append(filteredStrings, lowered)
}
}
resultString := strings.Join(filteredStrings, "-")
fmt.Printf(resultString)
Outpus:
this-string
Program exited.
Here you have the live example
edited Nov 26 '18 at 11:37
answered Nov 26 '18 at 11:28
NetwaveNetwave
13.5k22246
13.5k22246
add a comment |
add a comment |
My version just using regexp
Construct a regexp of the form 'bab|banb|btheb|bisb|' which will find
the words in the list that have "word boundaries" on both sides - so "This" is not matched
Second regexp reduces any spaces to dashes and makes multiple spaces a single dash
package main
import (
"bytes"
"fmt"
"regexp"
)
func main() {
removalString := "This is a strange string"
stringToRemove := string{"a", "an", "the", "is"}
var reg bytes.Buffer
for _, x := range stringToRemove {
reg.WriteString(`b`) // word boundary
reg.WriteString(x)
reg.WriteString(`b`)
reg.WriteString(`|`) // alternation operator
}
regx := regexp.MustCompile(reg.String())
slug := regx.ReplaceAllString(removalString, "")
regx2 := regexp.MustCompile(` +`)
slug = regx2.ReplaceAllString(slug, "-")
fmt.Println(slug)
}
add a comment |
My version just using regexp
Construct a regexp of the form 'bab|banb|btheb|bisb|' which will find
the words in the list that have "word boundaries" on both sides - so "This" is not matched
Second regexp reduces any spaces to dashes and makes multiple spaces a single dash
package main
import (
"bytes"
"fmt"
"regexp"
)
func main() {
removalString := "This is a strange string"
stringToRemove := string{"a", "an", "the", "is"}
var reg bytes.Buffer
for _, x := range stringToRemove {
reg.WriteString(`b`) // word boundary
reg.WriteString(x)
reg.WriteString(`b`)
reg.WriteString(`|`) // alternation operator
}
regx := regexp.MustCompile(reg.String())
slug := regx.ReplaceAllString(removalString, "")
regx2 := regexp.MustCompile(` +`)
slug = regx2.ReplaceAllString(slug, "-")
fmt.Println(slug)
}
add a comment |
My version just using regexp
Construct a regexp of the form 'bab|banb|btheb|bisb|' which will find
the words in the list that have "word boundaries" on both sides - so "This" is not matched
Second regexp reduces any spaces to dashes and makes multiple spaces a single dash
package main
import (
"bytes"
"fmt"
"regexp"
)
func main() {
removalString := "This is a strange string"
stringToRemove := string{"a", "an", "the", "is"}
var reg bytes.Buffer
for _, x := range stringToRemove {
reg.WriteString(`b`) // word boundary
reg.WriteString(x)
reg.WriteString(`b`)
reg.WriteString(`|`) // alternation operator
}
regx := regexp.MustCompile(reg.String())
slug := regx.ReplaceAllString(removalString, "")
regx2 := regexp.MustCompile(` +`)
slug = regx2.ReplaceAllString(slug, "-")
fmt.Println(slug)
}
My version just using regexp
Construct a regexp of the form 'bab|banb|btheb|bisb|' which will find
the words in the list that have "word boundaries" on both sides - so "This" is not matched
Second regexp reduces any spaces to dashes and makes multiple spaces a single dash
package main
import (
"bytes"
"fmt"
"regexp"
)
func main() {
removalString := "This is a strange string"
stringToRemove := string{"a", "an", "the", "is"}
var reg bytes.Buffer
for _, x := range stringToRemove {
reg.WriteString(`b`) // word boundary
reg.WriteString(x)
reg.WriteString(`b`)
reg.WriteString(`|`) // alternation operator
}
regx := regexp.MustCompile(reg.String())
slug := regx.ReplaceAllString(removalString, "")
regx2 := regexp.MustCompile(` +`)
slug = regx2.ReplaceAllString(slug, "-")
fmt.Println(slug)
}
answered Nov 26 '18 at 11:48


VorsprungVorsprung
23.4k32246
23.4k32246
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53479885%2fremove-all-articles-and-other-strings-from-a-string-using-go%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
K BPO8EGl9sLJQT cv,k8GZ,7keEL80nTea0,iUYMf09U Q6LnqdDdn5m2DypD
If your request is for an existing implementation, that is off-topic, as resource requests are not allowed here. If your question is how to improve or fix your current code, you need to be more specific: What problems are you facing? What help do you need?
– Flimzy
Nov 26 '18 at 11:23
1
Show examples of input and expected output and what fails.
– Poul Bak
Nov 26 '18 at 11:24
1
Also note that "is" is not an article, it's a verb.
– Flimzy
Nov 26 '18 at 11:25