Rewriting function using arrow function to fix IE compatibility
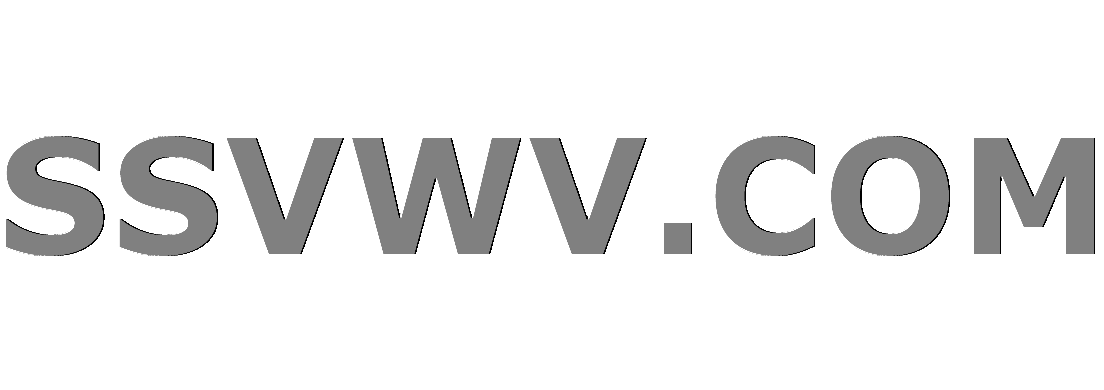
Multi tool use
I don't usually write in javascript and have wrote myself into a bit of a problem. I wrote the following bit of code to interact with some online donation software so that when someone clicks on a donation amount button (.amountNum input[type='radio']) on a donation page, it prints how much the total donation will be if they cover the processing fee. Which worked... in every browser except internet explorer. Turned out arrow functions don't work in IE, and I don't know how to rewrite the function without it. Any pointers?
var inputs = document.querySelectorAll(".amountNum input[type='radio']");
inputs.forEach(el =>
el.addEventListener('click', function() {
var value = parseInt(this.value, 10);
var URL = `https://example.com/display-fee?contribution=${value}&max=2500.00`;
jQuery.get(URL, function(data) {
var fee = data.fee;
var total = fee + value;
total = total.toFixed(2);
$("#full-gift-label")[0].innerText = ("I'd like to help cover the transaction fees on my donation. My grand total will be £" + total);
})
}));
javascript jquery arrow-functions
add a comment |
I don't usually write in javascript and have wrote myself into a bit of a problem. I wrote the following bit of code to interact with some online donation software so that when someone clicks on a donation amount button (.amountNum input[type='radio']) on a donation page, it prints how much the total donation will be if they cover the processing fee. Which worked... in every browser except internet explorer. Turned out arrow functions don't work in IE, and I don't know how to rewrite the function without it. Any pointers?
var inputs = document.querySelectorAll(".amountNum input[type='radio']");
inputs.forEach(el =>
el.addEventListener('click', function() {
var value = parseInt(this.value, 10);
var URL = `https://example.com/display-fee?contribution=${value}&max=2500.00`;
jQuery.get(URL, function(data) {
var fee = data.fee;
var total = fee + value;
total = total.toFixed(2);
$("#full-gift-label")[0].innerText = ("I'd like to help cover the transaction fees on my donation. My grand total will be £" + total);
})
}));
javascript jquery arrow-functions
kangax.github.io/compat-table/es6
– KornholioBeavis
Nov 26 '18 at 9:37
How many.amountNum
s are there in your HTML? Is there exactly one, or many?
– CertainPerformance
Nov 26 '18 at 9:41
add a comment |
I don't usually write in javascript and have wrote myself into a bit of a problem. I wrote the following bit of code to interact with some online donation software so that when someone clicks on a donation amount button (.amountNum input[type='radio']) on a donation page, it prints how much the total donation will be if they cover the processing fee. Which worked... in every browser except internet explorer. Turned out arrow functions don't work in IE, and I don't know how to rewrite the function without it. Any pointers?
var inputs = document.querySelectorAll(".amountNum input[type='radio']");
inputs.forEach(el =>
el.addEventListener('click', function() {
var value = parseInt(this.value, 10);
var URL = `https://example.com/display-fee?contribution=${value}&max=2500.00`;
jQuery.get(URL, function(data) {
var fee = data.fee;
var total = fee + value;
total = total.toFixed(2);
$("#full-gift-label")[0].innerText = ("I'd like to help cover the transaction fees on my donation. My grand total will be £" + total);
})
}));
javascript jquery arrow-functions
I don't usually write in javascript and have wrote myself into a bit of a problem. I wrote the following bit of code to interact with some online donation software so that when someone clicks on a donation amount button (.amountNum input[type='radio']) on a donation page, it prints how much the total donation will be if they cover the processing fee. Which worked... in every browser except internet explorer. Turned out arrow functions don't work in IE, and I don't know how to rewrite the function without it. Any pointers?
var inputs = document.querySelectorAll(".amountNum input[type='radio']");
inputs.forEach(el =>
el.addEventListener('click', function() {
var value = parseInt(this.value, 10);
var URL = `https://example.com/display-fee?contribution=${value}&max=2500.00`;
jQuery.get(URL, function(data) {
var fee = data.fee;
var total = fee + value;
total = total.toFixed(2);
$("#full-gift-label")[0].innerText = ("I'd like to help cover the transaction fees on my donation. My grand total will be £" + total);
})
}));
javascript jquery arrow-functions
javascript jquery arrow-functions
asked Nov 26 '18 at 9:33
Georgia O'BrienGeorgia O'Brien
234
234
kangax.github.io/compat-table/es6
– KornholioBeavis
Nov 26 '18 at 9:37
How many.amountNum
s are there in your HTML? Is there exactly one, or many?
– CertainPerformance
Nov 26 '18 at 9:41
add a comment |
kangax.github.io/compat-table/es6
– KornholioBeavis
Nov 26 '18 at 9:37
How many.amountNum
s are there in your HTML? Is there exactly one, or many?
– CertainPerformance
Nov 26 '18 at 9:41
kangax.github.io/compat-table/es6
– KornholioBeavis
Nov 26 '18 at 9:37
kangax.github.io/compat-table/es6
– KornholioBeavis
Nov 26 '18 at 9:37
How many
.amountNum
s are there in your HTML? Is there exactly one, or many?– CertainPerformance
Nov 26 '18 at 9:41
How many
.amountNum
s are there in your HTML? Is there exactly one, or many?– CertainPerformance
Nov 26 '18 at 9:41
add a comment |
1 Answer
1
active
oldest
votes
In this case, the arrow function performs identically to a standard function - just replace the el =>
with function(el) {
.
But, there's another problem: if you want to support IE, querySelectorAll
returns a NodeList
, and only on newer browsers can you forEach
directly over a NodeList
. So, you'll have to use Array.prototype.forEach
instead.
In addition to that, template literals aren't supported by IE either, so use ordinary string concatenation instead:
var inputs = document.querySelectorAll(".amountNum input[type='radio']");
Array.prototype.forEach.call(
inputs,
function(el) {
el.addEventListener('click', function() {
var value = parseInt(this.value, 10);
var URL = 'https://example.com/display-fee?contribution=' + value + '&max=2500.00';
jQuery.get(URL, function(data) {
var fee = data.fee;
var total = fee + value;
total = total.toFixed(2);
$("#full-gift-label")[0].innerText = ("I'd like to help cover the transaction fees on my donation. My grand total will be £" + total);
})
})
}
);
In the general case, it's best to use Babel to automatically transpile your code from the latest and greatest version of the language down to ES5 automatically, allowing you to write code with the most ease, and for older browsers to be able to understand it:
https://babeljs.io/repl/
Ah, see I'd tried just rewriting to a standard function but when it didn't work (probably because the the forEach issue you've flagged) I assumed there was something else at play. I'm now left with the issue that IE doesn't seem to like the backticks I've used around the var URL?
– Georgia O'Brien
Nov 26 '18 at 9:47
Ah, still ES6ing it. Used Babel and solved. Thanks so much!
– Georgia O'Brien
Nov 26 '18 at 9:50
Yes, that's a "template literal" which is another ES6 feature (therefore unsupported in IE). In this case you can just dovar URL = "https://example.com/display-fee?contribution="+value+"&max=2500.00";
– Robin Zigmond
Nov 26 '18 at 9:50
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53478166%2frewriting-function-using-arrow-function-to-fix-ie-compatibility%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
In this case, the arrow function performs identically to a standard function - just replace the el =>
with function(el) {
.
But, there's another problem: if you want to support IE, querySelectorAll
returns a NodeList
, and only on newer browsers can you forEach
directly over a NodeList
. So, you'll have to use Array.prototype.forEach
instead.
In addition to that, template literals aren't supported by IE either, so use ordinary string concatenation instead:
var inputs = document.querySelectorAll(".amountNum input[type='radio']");
Array.prototype.forEach.call(
inputs,
function(el) {
el.addEventListener('click', function() {
var value = parseInt(this.value, 10);
var URL = 'https://example.com/display-fee?contribution=' + value + '&max=2500.00';
jQuery.get(URL, function(data) {
var fee = data.fee;
var total = fee + value;
total = total.toFixed(2);
$("#full-gift-label")[0].innerText = ("I'd like to help cover the transaction fees on my donation. My grand total will be £" + total);
})
})
}
);
In the general case, it's best to use Babel to automatically transpile your code from the latest and greatest version of the language down to ES5 automatically, allowing you to write code with the most ease, and for older browsers to be able to understand it:
https://babeljs.io/repl/
Ah, see I'd tried just rewriting to a standard function but when it didn't work (probably because the the forEach issue you've flagged) I assumed there was something else at play. I'm now left with the issue that IE doesn't seem to like the backticks I've used around the var URL?
– Georgia O'Brien
Nov 26 '18 at 9:47
Ah, still ES6ing it. Used Babel and solved. Thanks so much!
– Georgia O'Brien
Nov 26 '18 at 9:50
Yes, that's a "template literal" which is another ES6 feature (therefore unsupported in IE). In this case you can just dovar URL = "https://example.com/display-fee?contribution="+value+"&max=2500.00";
– Robin Zigmond
Nov 26 '18 at 9:50
add a comment |
In this case, the arrow function performs identically to a standard function - just replace the el =>
with function(el) {
.
But, there's another problem: if you want to support IE, querySelectorAll
returns a NodeList
, and only on newer browsers can you forEach
directly over a NodeList
. So, you'll have to use Array.prototype.forEach
instead.
In addition to that, template literals aren't supported by IE either, so use ordinary string concatenation instead:
var inputs = document.querySelectorAll(".amountNum input[type='radio']");
Array.prototype.forEach.call(
inputs,
function(el) {
el.addEventListener('click', function() {
var value = parseInt(this.value, 10);
var URL = 'https://example.com/display-fee?contribution=' + value + '&max=2500.00';
jQuery.get(URL, function(data) {
var fee = data.fee;
var total = fee + value;
total = total.toFixed(2);
$("#full-gift-label")[0].innerText = ("I'd like to help cover the transaction fees on my donation. My grand total will be £" + total);
})
})
}
);
In the general case, it's best to use Babel to automatically transpile your code from the latest and greatest version of the language down to ES5 automatically, allowing you to write code with the most ease, and for older browsers to be able to understand it:
https://babeljs.io/repl/
Ah, see I'd tried just rewriting to a standard function but when it didn't work (probably because the the forEach issue you've flagged) I assumed there was something else at play. I'm now left with the issue that IE doesn't seem to like the backticks I've used around the var URL?
– Georgia O'Brien
Nov 26 '18 at 9:47
Ah, still ES6ing it. Used Babel and solved. Thanks so much!
– Georgia O'Brien
Nov 26 '18 at 9:50
Yes, that's a "template literal" which is another ES6 feature (therefore unsupported in IE). In this case you can just dovar URL = "https://example.com/display-fee?contribution="+value+"&max=2500.00";
– Robin Zigmond
Nov 26 '18 at 9:50
add a comment |
In this case, the arrow function performs identically to a standard function - just replace the el =>
with function(el) {
.
But, there's another problem: if you want to support IE, querySelectorAll
returns a NodeList
, and only on newer browsers can you forEach
directly over a NodeList
. So, you'll have to use Array.prototype.forEach
instead.
In addition to that, template literals aren't supported by IE either, so use ordinary string concatenation instead:
var inputs = document.querySelectorAll(".amountNum input[type='radio']");
Array.prototype.forEach.call(
inputs,
function(el) {
el.addEventListener('click', function() {
var value = parseInt(this.value, 10);
var URL = 'https://example.com/display-fee?contribution=' + value + '&max=2500.00';
jQuery.get(URL, function(data) {
var fee = data.fee;
var total = fee + value;
total = total.toFixed(2);
$("#full-gift-label")[0].innerText = ("I'd like to help cover the transaction fees on my donation. My grand total will be £" + total);
})
})
}
);
In the general case, it's best to use Babel to automatically transpile your code from the latest and greatest version of the language down to ES5 automatically, allowing you to write code with the most ease, and for older browsers to be able to understand it:
https://babeljs.io/repl/
In this case, the arrow function performs identically to a standard function - just replace the el =>
with function(el) {
.
But, there's another problem: if you want to support IE, querySelectorAll
returns a NodeList
, and only on newer browsers can you forEach
directly over a NodeList
. So, you'll have to use Array.prototype.forEach
instead.
In addition to that, template literals aren't supported by IE either, so use ordinary string concatenation instead:
var inputs = document.querySelectorAll(".amountNum input[type='radio']");
Array.prototype.forEach.call(
inputs,
function(el) {
el.addEventListener('click', function() {
var value = parseInt(this.value, 10);
var URL = 'https://example.com/display-fee?contribution=' + value + '&max=2500.00';
jQuery.get(URL, function(data) {
var fee = data.fee;
var total = fee + value;
total = total.toFixed(2);
$("#full-gift-label")[0].innerText = ("I'd like to help cover the transaction fees on my donation. My grand total will be £" + total);
})
})
}
);
In the general case, it's best to use Babel to automatically transpile your code from the latest and greatest version of the language down to ES5 automatically, allowing you to write code with the most ease, and for older browsers to be able to understand it:
https://babeljs.io/repl/
edited Nov 26 '18 at 9:54
answered Nov 26 '18 at 9:37
CertainPerformanceCertainPerformance
95.9k165786
95.9k165786
Ah, see I'd tried just rewriting to a standard function but when it didn't work (probably because the the forEach issue you've flagged) I assumed there was something else at play. I'm now left with the issue that IE doesn't seem to like the backticks I've used around the var URL?
– Georgia O'Brien
Nov 26 '18 at 9:47
Ah, still ES6ing it. Used Babel and solved. Thanks so much!
– Georgia O'Brien
Nov 26 '18 at 9:50
Yes, that's a "template literal" which is another ES6 feature (therefore unsupported in IE). In this case you can just dovar URL = "https://example.com/display-fee?contribution="+value+"&max=2500.00";
– Robin Zigmond
Nov 26 '18 at 9:50
add a comment |
Ah, see I'd tried just rewriting to a standard function but when it didn't work (probably because the the forEach issue you've flagged) I assumed there was something else at play. I'm now left with the issue that IE doesn't seem to like the backticks I've used around the var URL?
– Georgia O'Brien
Nov 26 '18 at 9:47
Ah, still ES6ing it. Used Babel and solved. Thanks so much!
– Georgia O'Brien
Nov 26 '18 at 9:50
Yes, that's a "template literal" which is another ES6 feature (therefore unsupported in IE). In this case you can just dovar URL = "https://example.com/display-fee?contribution="+value+"&max=2500.00";
– Robin Zigmond
Nov 26 '18 at 9:50
Ah, see I'd tried just rewriting to a standard function but when it didn't work (probably because the the forEach issue you've flagged) I assumed there was something else at play. I'm now left with the issue that IE doesn't seem to like the backticks I've used around the var URL?
– Georgia O'Brien
Nov 26 '18 at 9:47
Ah, see I'd tried just rewriting to a standard function but when it didn't work (probably because the the forEach issue you've flagged) I assumed there was something else at play. I'm now left with the issue that IE doesn't seem to like the backticks I've used around the var URL?
– Georgia O'Brien
Nov 26 '18 at 9:47
Ah, still ES6ing it. Used Babel and solved. Thanks so much!
– Georgia O'Brien
Nov 26 '18 at 9:50
Ah, still ES6ing it. Used Babel and solved. Thanks so much!
– Georgia O'Brien
Nov 26 '18 at 9:50
Yes, that's a "template literal" which is another ES6 feature (therefore unsupported in IE). In this case you can just do
var URL = "https://example.com/display-fee?contribution="+value+"&max=2500.00";
– Robin Zigmond
Nov 26 '18 at 9:50
Yes, that's a "template literal" which is another ES6 feature (therefore unsupported in IE). In this case you can just do
var URL = "https://example.com/display-fee?contribution="+value+"&max=2500.00";
– Robin Zigmond
Nov 26 '18 at 9:50
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53478166%2frewriting-function-using-arrow-function-to-fix-ie-compatibility%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
OWiu,V4b5z Yox cFizToZHCwG91or,QqBA0P3ydAmT
kangax.github.io/compat-table/es6
– KornholioBeavis
Nov 26 '18 at 9:37
How many
.amountNum
s are there in your HTML? Is there exactly one, or many?– CertainPerformance
Nov 26 '18 at 9:41