Cannot set values on a ManyToManyField which specifies an intermediary model
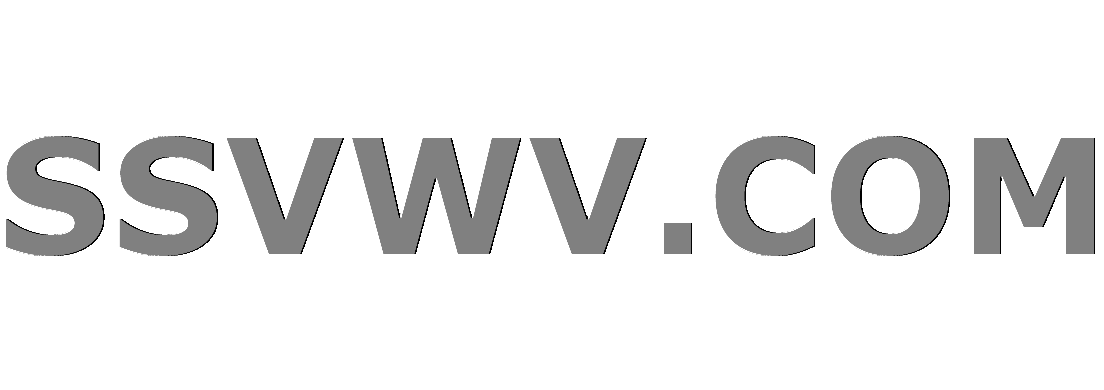
Multi tool use
up vote
0
down vote
favorite
How can I set values on to a ManyToManyField which specifies an intermediary model?
In the models.py
class BookUser(models.Model):
email = models.EmailField()
class Book(models.Model):
author_id= models.CharField(max_length=255)
send_to = models.ManyToManyField(BookUser, through='BookUserRelationship')
book_id = models.AutoField(primary_key=True)
file_size = models.CharField(null=True)
class BookUserRelationship(models.Model):
book = models.ForeignKey(Book, on_delete=models.CASCADE)
user = models.ForeignKey(BookUser, on_delete=models.CASCADE)
shared_date = models.DateTimeField(auto_now_add=True,null=True,blank=True)
Tried to update in serializers.py
class BookSerializer(serializers.ModelSerializer):
send_to = BookUserSerializer(many=True, read_only=True)
class Meta():
model = Book
fields = ('book_id', 'author_id','file_size','send_to')
class BookUserSerializer(serializers.ModelSerializer):
model = BookUser
fields = ('email')
In the views.py for listing the books by passing the book_id as query params
class BookListView(generics.ListCreateAPIView):
serializer_class = serializers.BookSerializer
def get(self, request, *args, **kwargs):
user = self.request.user
book_id = self.request.query_params.get('book_id', None)
if book_id:
book = models.Book.objects.filter(book_id=book_id)
return Response(serializers.BookSerializer(book[0]).data)
django django-rest-framework many-to-many
add a comment |
up vote
0
down vote
favorite
How can I set values on to a ManyToManyField which specifies an intermediary model?
In the models.py
class BookUser(models.Model):
email = models.EmailField()
class Book(models.Model):
author_id= models.CharField(max_length=255)
send_to = models.ManyToManyField(BookUser, through='BookUserRelationship')
book_id = models.AutoField(primary_key=True)
file_size = models.CharField(null=True)
class BookUserRelationship(models.Model):
book = models.ForeignKey(Book, on_delete=models.CASCADE)
user = models.ForeignKey(BookUser, on_delete=models.CASCADE)
shared_date = models.DateTimeField(auto_now_add=True,null=True,blank=True)
Tried to update in serializers.py
class BookSerializer(serializers.ModelSerializer):
send_to = BookUserSerializer(many=True, read_only=True)
class Meta():
model = Book
fields = ('book_id', 'author_id','file_size','send_to')
class BookUserSerializer(serializers.ModelSerializer):
model = BookUser
fields = ('email')
In the views.py for listing the books by passing the book_id as query params
class BookListView(generics.ListCreateAPIView):
serializer_class = serializers.BookSerializer
def get(self, request, *args, **kwargs):
user = self.request.user
book_id = self.request.query_params.get('book_id', None)
if book_id:
book = models.Book.objects.filter(book_id=book_id)
return Response(serializers.BookSerializer(book[0]).data)
django django-rest-framework many-to-many
This is too broad. You need to show your models, and what you tried.
– Daniel Roseman
Nov 19 at 9:46
I am very new in the Django drf programming, can you please help me with the problem? Edited the question and added the models.
– kkr
Nov 19 at 10:09
Thanks but you also need to show how you are trying to set the values.
– Daniel Roseman
Nov 19 at 10:11
Can you please help me with solution
– kkr
Nov 19 at 11:08
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
How can I set values on to a ManyToManyField which specifies an intermediary model?
In the models.py
class BookUser(models.Model):
email = models.EmailField()
class Book(models.Model):
author_id= models.CharField(max_length=255)
send_to = models.ManyToManyField(BookUser, through='BookUserRelationship')
book_id = models.AutoField(primary_key=True)
file_size = models.CharField(null=True)
class BookUserRelationship(models.Model):
book = models.ForeignKey(Book, on_delete=models.CASCADE)
user = models.ForeignKey(BookUser, on_delete=models.CASCADE)
shared_date = models.DateTimeField(auto_now_add=True,null=True,blank=True)
Tried to update in serializers.py
class BookSerializer(serializers.ModelSerializer):
send_to = BookUserSerializer(many=True, read_only=True)
class Meta():
model = Book
fields = ('book_id', 'author_id','file_size','send_to')
class BookUserSerializer(serializers.ModelSerializer):
model = BookUser
fields = ('email')
In the views.py for listing the books by passing the book_id as query params
class BookListView(generics.ListCreateAPIView):
serializer_class = serializers.BookSerializer
def get(self, request, *args, **kwargs):
user = self.request.user
book_id = self.request.query_params.get('book_id', None)
if book_id:
book = models.Book.objects.filter(book_id=book_id)
return Response(serializers.BookSerializer(book[0]).data)
django django-rest-framework many-to-many
How can I set values on to a ManyToManyField which specifies an intermediary model?
In the models.py
class BookUser(models.Model):
email = models.EmailField()
class Book(models.Model):
author_id= models.CharField(max_length=255)
send_to = models.ManyToManyField(BookUser, through='BookUserRelationship')
book_id = models.AutoField(primary_key=True)
file_size = models.CharField(null=True)
class BookUserRelationship(models.Model):
book = models.ForeignKey(Book, on_delete=models.CASCADE)
user = models.ForeignKey(BookUser, on_delete=models.CASCADE)
shared_date = models.DateTimeField(auto_now_add=True,null=True,blank=True)
Tried to update in serializers.py
class BookSerializer(serializers.ModelSerializer):
send_to = BookUserSerializer(many=True, read_only=True)
class Meta():
model = Book
fields = ('book_id', 'author_id','file_size','send_to')
class BookUserSerializer(serializers.ModelSerializer):
model = BookUser
fields = ('email')
In the views.py for listing the books by passing the book_id as query params
class BookListView(generics.ListCreateAPIView):
serializer_class = serializers.BookSerializer
def get(self, request, *args, **kwargs):
user = self.request.user
book_id = self.request.query_params.get('book_id', None)
if book_id:
book = models.Book.objects.filter(book_id=book_id)
return Response(serializers.BookSerializer(book[0]).data)
django django-rest-framework many-to-many
django django-rest-framework many-to-many
edited Nov 20 at 5:13
asked Nov 19 at 9:20


kkr
85
85
This is too broad. You need to show your models, and what you tried.
– Daniel Roseman
Nov 19 at 9:46
I am very new in the Django drf programming, can you please help me with the problem? Edited the question and added the models.
– kkr
Nov 19 at 10:09
Thanks but you also need to show how you are trying to set the values.
– Daniel Roseman
Nov 19 at 10:11
Can you please help me with solution
– kkr
Nov 19 at 11:08
add a comment |
This is too broad. You need to show your models, and what you tried.
– Daniel Roseman
Nov 19 at 9:46
I am very new in the Django drf programming, can you please help me with the problem? Edited the question and added the models.
– kkr
Nov 19 at 10:09
Thanks but you also need to show how you are trying to set the values.
– Daniel Roseman
Nov 19 at 10:11
Can you please help me with solution
– kkr
Nov 19 at 11:08
This is too broad. You need to show your models, and what you tried.
– Daniel Roseman
Nov 19 at 9:46
This is too broad. You need to show your models, and what you tried.
– Daniel Roseman
Nov 19 at 9:46
I am very new in the Django drf programming, can you please help me with the problem? Edited the question and added the models.
– kkr
Nov 19 at 10:09
I am very new in the Django drf programming, can you please help me with the problem? Edited the question and added the models.
– kkr
Nov 19 at 10:09
Thanks but you also need to show how you are trying to set the values.
– Daniel Roseman
Nov 19 at 10:11
Thanks but you also need to show how you are trying to set the values.
– Daniel Roseman
Nov 19 at 10:11
Can you please help me with solution
– kkr
Nov 19 at 11:08
Can you please help me with solution
– kkr
Nov 19 at 11:08
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
You don't need to do anything at all. You have already set the relevant data in your for loop, by creating the BookUserRelationship instances. That is the many-to-many relationship; you should just remove the instance.send_to.set(emails)
line.
Thankyou, But while taking the response I am not getting it. Instead of that error 'str' object has no attribute 'values' is coming.
– kkr
Nov 19 at 11:13
Where is that error happening? It doesn't seem to have anything to do with this code. Please edit the question to show the full traceback.
– Daniel Roseman
Nov 19 at 11:15
Can you please have a look into this problem. The send_to field is having the value None
– kkr
Nov 19 at 11:57
Can you show your BookUserSerializer?
– Daniel Roseman
Nov 19 at 12:24
added BookUserSerializer also, Can you please help? Thanks in advance.
– kkr
Nov 20 at 8:45
|
show 5 more comments
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
You don't need to do anything at all. You have already set the relevant data in your for loop, by creating the BookUserRelationship instances. That is the many-to-many relationship; you should just remove the instance.send_to.set(emails)
line.
Thankyou, But while taking the response I am not getting it. Instead of that error 'str' object has no attribute 'values' is coming.
– kkr
Nov 19 at 11:13
Where is that error happening? It doesn't seem to have anything to do with this code. Please edit the question to show the full traceback.
– Daniel Roseman
Nov 19 at 11:15
Can you please have a look into this problem. The send_to field is having the value None
– kkr
Nov 19 at 11:57
Can you show your BookUserSerializer?
– Daniel Roseman
Nov 19 at 12:24
added BookUserSerializer also, Can you please help? Thanks in advance.
– kkr
Nov 20 at 8:45
|
show 5 more comments
up vote
0
down vote
accepted
You don't need to do anything at all. You have already set the relevant data in your for loop, by creating the BookUserRelationship instances. That is the many-to-many relationship; you should just remove the instance.send_to.set(emails)
line.
Thankyou, But while taking the response I am not getting it. Instead of that error 'str' object has no attribute 'values' is coming.
– kkr
Nov 19 at 11:13
Where is that error happening? It doesn't seem to have anything to do with this code. Please edit the question to show the full traceback.
– Daniel Roseman
Nov 19 at 11:15
Can you please have a look into this problem. The send_to field is having the value None
– kkr
Nov 19 at 11:57
Can you show your BookUserSerializer?
– Daniel Roseman
Nov 19 at 12:24
added BookUserSerializer also, Can you please help? Thanks in advance.
– kkr
Nov 20 at 8:45
|
show 5 more comments
up vote
0
down vote
accepted
up vote
0
down vote
accepted
You don't need to do anything at all. You have already set the relevant data in your for loop, by creating the BookUserRelationship instances. That is the many-to-many relationship; you should just remove the instance.send_to.set(emails)
line.
You don't need to do anything at all. You have already set the relevant data in your for loop, by creating the BookUserRelationship instances. That is the many-to-many relationship; you should just remove the instance.send_to.set(emails)
line.
answered Nov 19 at 11:09
Daniel Roseman
440k40572627
440k40572627
Thankyou, But while taking the response I am not getting it. Instead of that error 'str' object has no attribute 'values' is coming.
– kkr
Nov 19 at 11:13
Where is that error happening? It doesn't seem to have anything to do with this code. Please edit the question to show the full traceback.
– Daniel Roseman
Nov 19 at 11:15
Can you please have a look into this problem. The send_to field is having the value None
– kkr
Nov 19 at 11:57
Can you show your BookUserSerializer?
– Daniel Roseman
Nov 19 at 12:24
added BookUserSerializer also, Can you please help? Thanks in advance.
– kkr
Nov 20 at 8:45
|
show 5 more comments
Thankyou, But while taking the response I am not getting it. Instead of that error 'str' object has no attribute 'values' is coming.
– kkr
Nov 19 at 11:13
Where is that error happening? It doesn't seem to have anything to do with this code. Please edit the question to show the full traceback.
– Daniel Roseman
Nov 19 at 11:15
Can you please have a look into this problem. The send_to field is having the value None
– kkr
Nov 19 at 11:57
Can you show your BookUserSerializer?
– Daniel Roseman
Nov 19 at 12:24
added BookUserSerializer also, Can you please help? Thanks in advance.
– kkr
Nov 20 at 8:45
Thankyou, But while taking the response I am not getting it. Instead of that error 'str' object has no attribute 'values' is coming.
– kkr
Nov 19 at 11:13
Thankyou, But while taking the response I am not getting it. Instead of that error 'str' object has no attribute 'values' is coming.
– kkr
Nov 19 at 11:13
Where is that error happening? It doesn't seem to have anything to do with this code. Please edit the question to show the full traceback.
– Daniel Roseman
Nov 19 at 11:15
Where is that error happening? It doesn't seem to have anything to do with this code. Please edit the question to show the full traceback.
– Daniel Roseman
Nov 19 at 11:15
Can you please have a look into this problem. The send_to field is having the value None
– kkr
Nov 19 at 11:57
Can you please have a look into this problem. The send_to field is having the value None
– kkr
Nov 19 at 11:57
Can you show your BookUserSerializer?
– Daniel Roseman
Nov 19 at 12:24
Can you show your BookUserSerializer?
– Daniel Roseman
Nov 19 at 12:24
added BookUserSerializer also, Can you please help? Thanks in advance.
– kkr
Nov 20 at 8:45
added BookUserSerializer also, Can you please help? Thanks in advance.
– kkr
Nov 20 at 8:45
|
show 5 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53371534%2fcannot-set-values-on-a-manytomanyfield-which-specifies-an-intermediary-model%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
d 4B5e5IOmGgx,4yWxmRTQnuKm7TMn4t7yz D,bmsnRlnDob5cs NgVLeKo9IW3QpDbG0bu7JGNTC0wxd0wv,T,5Na4RI,kFoke,yZbL
This is too broad. You need to show your models, and what you tried.
– Daniel Roseman
Nov 19 at 9:46
I am very new in the Django drf programming, can you please help me with the problem? Edited the question and added the models.
– kkr
Nov 19 at 10:09
Thanks but you also need to show how you are trying to set the values.
– Daniel Roseman
Nov 19 at 10:11
Can you please help me with solution
– kkr
Nov 19 at 11:08