How to leave existing data unchanged is a condition is false
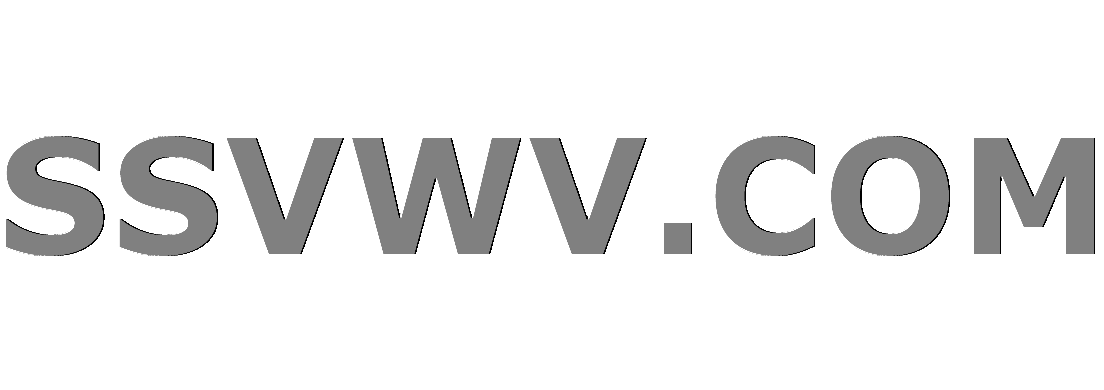
Multi tool use
up vote
0
down vote
favorite
I am currently working on a project where I need to be able to rent a truck to a customer but also need to add the customer details if not existing. My problem is even though through the WPF form I input the exact same details of a customer, there would be a new set of data added thus creating a new Customer ID for one person. How would I be able to get the database disregard the existing customer details?
My data service code:
public class DataService
{
public static void rentTruck(TruckRental toRent, bool isNewCustomer)
{
using (var ctx = new DAD_TruckRental_RGMContext())
{
if (!isNewCustomer)
{
ctx.Entry(toRent.Customer).State = EntityState.Unchanged;//doesnt leave existing customer unchanged
}
ctx.Entry(toRent.Truck).State = EntityState.Modified;
ctx.TruckRental.Add(toRent);
ctx.SaveChanges();
}
}
My cs code:
private void Button_Click(object sender, RoutedEventArgs e)
{
TruckCustomer cust = new TruckCustomer();
cust.Age = int.Parse(ageTextBox.Text);
cust.LicenseNumber = licenseNumberTextBox.Text;
cust.LicenseExpiryDate = licenseExpiryDateDatePicker.SelectedDate.Value.Date;
TruckPerson per = new TruckPerson();
per.Address = addressTextBox.Text;
per.Telephone = telephoneTextBox.Text;
per.Name = nameTextBox.Text;
cust.Customer = per;
int truckId = int.Parse(truckIdTextBox.Text);
IndividualTruck truck = DataService.searchTruckByID(truckId);
decimal priceTotal = decimal.Parse(totalPriceTextBox.Text);
TruckRental toRent = new TruckRental();
toRent.TotalPrice = priceTotal;
toRent.RentDate = rentDateDatePicker.SelectedDate.Value.Date;
toRent.ReturnDueDate = returnDueDateDatePicker.SelectedDate.Value.Date;
toRent.Customer = cust;
toRent.Truck = truck;
truck.Status = "Rented";
DataService.rentTruck(toRent, true);
MessageBox.Show("Truck rented succesfully");
}
c#

|
show 6 more comments
up vote
0
down vote
favorite
I am currently working on a project where I need to be able to rent a truck to a customer but also need to add the customer details if not existing. My problem is even though through the WPF form I input the exact same details of a customer, there would be a new set of data added thus creating a new Customer ID for one person. How would I be able to get the database disregard the existing customer details?
My data service code:
public class DataService
{
public static void rentTruck(TruckRental toRent, bool isNewCustomer)
{
using (var ctx = new DAD_TruckRental_RGMContext())
{
if (!isNewCustomer)
{
ctx.Entry(toRent.Customer).State = EntityState.Unchanged;//doesnt leave existing customer unchanged
}
ctx.Entry(toRent.Truck).State = EntityState.Modified;
ctx.TruckRental.Add(toRent);
ctx.SaveChanges();
}
}
My cs code:
private void Button_Click(object sender, RoutedEventArgs e)
{
TruckCustomer cust = new TruckCustomer();
cust.Age = int.Parse(ageTextBox.Text);
cust.LicenseNumber = licenseNumberTextBox.Text;
cust.LicenseExpiryDate = licenseExpiryDateDatePicker.SelectedDate.Value.Date;
TruckPerson per = new TruckPerson();
per.Address = addressTextBox.Text;
per.Telephone = telephoneTextBox.Text;
per.Name = nameTextBox.Text;
cust.Customer = per;
int truckId = int.Parse(truckIdTextBox.Text);
IndividualTruck truck = DataService.searchTruckByID(truckId);
decimal priceTotal = decimal.Parse(totalPriceTextBox.Text);
TruckRental toRent = new TruckRental();
toRent.TotalPrice = priceTotal;
toRent.RentDate = rentDateDatePicker.SelectedDate.Value.Date;
toRent.ReturnDueDate = returnDueDateDatePicker.SelectedDate.Value.Date;
toRent.Customer = cust;
toRent.Truck = truck;
truck.Status = "Rented";
DataService.rentTruck(toRent, true);
MessageBox.Show("Truck rented succesfully");
}
c#

That looks like a problem in the DataService.rentTruck method, not the code you have posted. Do you have access to that?
– Robin Bennett
Nov 19 at 9:42
@RobinBennett yes its the code above
– Grace Delos Reyes
Nov 19 at 9:44
@GraceDelosReyes what is the unique key in customer table ?
– Saif
Nov 19 at 9:52
@Saif CustomerID
– Grace Delos Reyes
Nov 19 at 9:53
@GraceDelosReyes do you key in CustomerId or auto generate ?
– Saif
Nov 19 at 9:54
|
show 6 more comments
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am currently working on a project where I need to be able to rent a truck to a customer but also need to add the customer details if not existing. My problem is even though through the WPF form I input the exact same details of a customer, there would be a new set of data added thus creating a new Customer ID for one person. How would I be able to get the database disregard the existing customer details?
My data service code:
public class DataService
{
public static void rentTruck(TruckRental toRent, bool isNewCustomer)
{
using (var ctx = new DAD_TruckRental_RGMContext())
{
if (!isNewCustomer)
{
ctx.Entry(toRent.Customer).State = EntityState.Unchanged;//doesnt leave existing customer unchanged
}
ctx.Entry(toRent.Truck).State = EntityState.Modified;
ctx.TruckRental.Add(toRent);
ctx.SaveChanges();
}
}
My cs code:
private void Button_Click(object sender, RoutedEventArgs e)
{
TruckCustomer cust = new TruckCustomer();
cust.Age = int.Parse(ageTextBox.Text);
cust.LicenseNumber = licenseNumberTextBox.Text;
cust.LicenseExpiryDate = licenseExpiryDateDatePicker.SelectedDate.Value.Date;
TruckPerson per = new TruckPerson();
per.Address = addressTextBox.Text;
per.Telephone = telephoneTextBox.Text;
per.Name = nameTextBox.Text;
cust.Customer = per;
int truckId = int.Parse(truckIdTextBox.Text);
IndividualTruck truck = DataService.searchTruckByID(truckId);
decimal priceTotal = decimal.Parse(totalPriceTextBox.Text);
TruckRental toRent = new TruckRental();
toRent.TotalPrice = priceTotal;
toRent.RentDate = rentDateDatePicker.SelectedDate.Value.Date;
toRent.ReturnDueDate = returnDueDateDatePicker.SelectedDate.Value.Date;
toRent.Customer = cust;
toRent.Truck = truck;
truck.Status = "Rented";
DataService.rentTruck(toRent, true);
MessageBox.Show("Truck rented succesfully");
}
c#

I am currently working on a project where I need to be able to rent a truck to a customer but also need to add the customer details if not existing. My problem is even though through the WPF form I input the exact same details of a customer, there would be a new set of data added thus creating a new Customer ID for one person. How would I be able to get the database disregard the existing customer details?
My data service code:
public class DataService
{
public static void rentTruck(TruckRental toRent, bool isNewCustomer)
{
using (var ctx = new DAD_TruckRental_RGMContext())
{
if (!isNewCustomer)
{
ctx.Entry(toRent.Customer).State = EntityState.Unchanged;//doesnt leave existing customer unchanged
}
ctx.Entry(toRent.Truck).State = EntityState.Modified;
ctx.TruckRental.Add(toRent);
ctx.SaveChanges();
}
}
My cs code:
private void Button_Click(object sender, RoutedEventArgs e)
{
TruckCustomer cust = new TruckCustomer();
cust.Age = int.Parse(ageTextBox.Text);
cust.LicenseNumber = licenseNumberTextBox.Text;
cust.LicenseExpiryDate = licenseExpiryDateDatePicker.SelectedDate.Value.Date;
TruckPerson per = new TruckPerson();
per.Address = addressTextBox.Text;
per.Telephone = telephoneTextBox.Text;
per.Name = nameTextBox.Text;
cust.Customer = per;
int truckId = int.Parse(truckIdTextBox.Text);
IndividualTruck truck = DataService.searchTruckByID(truckId);
decimal priceTotal = decimal.Parse(totalPriceTextBox.Text);
TruckRental toRent = new TruckRental();
toRent.TotalPrice = priceTotal;
toRent.RentDate = rentDateDatePicker.SelectedDate.Value.Date;
toRent.ReturnDueDate = returnDueDateDatePicker.SelectedDate.Value.Date;
toRent.Customer = cust;
toRent.Truck = truck;
truck.Status = "Rented";
DataService.rentTruck(toRent, true);
MessageBox.Show("Truck rented succesfully");
}
c#

c#

asked Nov 19 at 9:38


Grace Delos Reyes
376
376
That looks like a problem in the DataService.rentTruck method, not the code you have posted. Do you have access to that?
– Robin Bennett
Nov 19 at 9:42
@RobinBennett yes its the code above
– Grace Delos Reyes
Nov 19 at 9:44
@GraceDelosReyes what is the unique key in customer table ?
– Saif
Nov 19 at 9:52
@Saif CustomerID
– Grace Delos Reyes
Nov 19 at 9:53
@GraceDelosReyes do you key in CustomerId or auto generate ?
– Saif
Nov 19 at 9:54
|
show 6 more comments
That looks like a problem in the DataService.rentTruck method, not the code you have posted. Do you have access to that?
– Robin Bennett
Nov 19 at 9:42
@RobinBennett yes its the code above
– Grace Delos Reyes
Nov 19 at 9:44
@GraceDelosReyes what is the unique key in customer table ?
– Saif
Nov 19 at 9:52
@Saif CustomerID
– Grace Delos Reyes
Nov 19 at 9:53
@GraceDelosReyes do you key in CustomerId or auto generate ?
– Saif
Nov 19 at 9:54
That looks like a problem in the DataService.rentTruck method, not the code you have posted. Do you have access to that?
– Robin Bennett
Nov 19 at 9:42
That looks like a problem in the DataService.rentTruck method, not the code you have posted. Do you have access to that?
– Robin Bennett
Nov 19 at 9:42
@RobinBennett yes its the code above
– Grace Delos Reyes
Nov 19 at 9:44
@RobinBennett yes its the code above
– Grace Delos Reyes
Nov 19 at 9:44
@GraceDelosReyes what is the unique key in customer table ?
– Saif
Nov 19 at 9:52
@GraceDelosReyes what is the unique key in customer table ?
– Saif
Nov 19 at 9:52
@Saif CustomerID
– Grace Delos Reyes
Nov 19 at 9:53
@Saif CustomerID
– Grace Delos Reyes
Nov 19 at 9:53
@GraceDelosReyes do you key in CustomerId or auto generate ?
– Saif
Nov 19 at 9:54
@GraceDelosReyes do you key in CustomerId or auto generate ?
– Saif
Nov 19 at 9:54
|
show 6 more comments
1 Answer
1
active
oldest
votes
up vote
0
down vote
Here is my suggestion
1- First check if customer details already exist in db using LicenseNumber
2- The first step will be either null
or have details, so if null then add received customer details otherwise update
here is the code
public class DataService
{
public static void rentTruck(TruckRental toRent, bool isNewCustomer, TruckCustomer tcustomer)
{
using (var ctx = new DAD_TruckRental_RGMContext())
{
var ob = ctx.TruckCustomer.Where(c => c.LicenseNumber == customer.LicenseNumber);
if ( ob != null) //not exist
{
//create new here
ctx.TruckCustomer.Add(tcustomer);
}
//exist then just update State
ctx.ob.State = EntityState.Modified;
ctx.AddOrUpdate(ob);
ctx.TruckRental.Add(toRent);
ctx.SaveChanges();
}
}
I hope this can help you
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
Here is my suggestion
1- First check if customer details already exist in db using LicenseNumber
2- The first step will be either null
or have details, so if null then add received customer details otherwise update
here is the code
public class DataService
{
public static void rentTruck(TruckRental toRent, bool isNewCustomer, TruckCustomer tcustomer)
{
using (var ctx = new DAD_TruckRental_RGMContext())
{
var ob = ctx.TruckCustomer.Where(c => c.LicenseNumber == customer.LicenseNumber);
if ( ob != null) //not exist
{
//create new here
ctx.TruckCustomer.Add(tcustomer);
}
//exist then just update State
ctx.ob.State = EntityState.Modified;
ctx.AddOrUpdate(ob);
ctx.TruckRental.Add(toRent);
ctx.SaveChanges();
}
}
I hope this can help you
add a comment |
up vote
0
down vote
Here is my suggestion
1- First check if customer details already exist in db using LicenseNumber
2- The first step will be either null
or have details, so if null then add received customer details otherwise update
here is the code
public class DataService
{
public static void rentTruck(TruckRental toRent, bool isNewCustomer, TruckCustomer tcustomer)
{
using (var ctx = new DAD_TruckRental_RGMContext())
{
var ob = ctx.TruckCustomer.Where(c => c.LicenseNumber == customer.LicenseNumber);
if ( ob != null) //not exist
{
//create new here
ctx.TruckCustomer.Add(tcustomer);
}
//exist then just update State
ctx.ob.State = EntityState.Modified;
ctx.AddOrUpdate(ob);
ctx.TruckRental.Add(toRent);
ctx.SaveChanges();
}
}
I hope this can help you
add a comment |
up vote
0
down vote
up vote
0
down vote
Here is my suggestion
1- First check if customer details already exist in db using LicenseNumber
2- The first step will be either null
or have details, so if null then add received customer details otherwise update
here is the code
public class DataService
{
public static void rentTruck(TruckRental toRent, bool isNewCustomer, TruckCustomer tcustomer)
{
using (var ctx = new DAD_TruckRental_RGMContext())
{
var ob = ctx.TruckCustomer.Where(c => c.LicenseNumber == customer.LicenseNumber);
if ( ob != null) //not exist
{
//create new here
ctx.TruckCustomer.Add(tcustomer);
}
//exist then just update State
ctx.ob.State = EntityState.Modified;
ctx.AddOrUpdate(ob);
ctx.TruckRental.Add(toRent);
ctx.SaveChanges();
}
}
I hope this can help you
Here is my suggestion
1- First check if customer details already exist in db using LicenseNumber
2- The first step will be either null
or have details, so if null then add received customer details otherwise update
here is the code
public class DataService
{
public static void rentTruck(TruckRental toRent, bool isNewCustomer, TruckCustomer tcustomer)
{
using (var ctx = new DAD_TruckRental_RGMContext())
{
var ob = ctx.TruckCustomer.Where(c => c.LicenseNumber == customer.LicenseNumber);
if ( ob != null) //not exist
{
//create new here
ctx.TruckCustomer.Add(tcustomer);
}
//exist then just update State
ctx.ob.State = EntityState.Modified;
ctx.AddOrUpdate(ob);
ctx.TruckRental.Add(toRent);
ctx.SaveChanges();
}
}
I hope this can help you
answered Nov 19 at 10:24
Saif
1,0381723
1,0381723
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53371813%2fhow-to-leave-existing-data-unchanged-is-a-condition-is-false%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
8FkuxBWsVNzF3oSqc7ft9P HeoF6UN3fa6vlP5CZcEJJBy,b
That looks like a problem in the DataService.rentTruck method, not the code you have posted. Do you have access to that?
– Robin Bennett
Nov 19 at 9:42
@RobinBennett yes its the code above
– Grace Delos Reyes
Nov 19 at 9:44
@GraceDelosReyes what is the unique key in customer table ?
– Saif
Nov 19 at 9:52
@Saif CustomerID
– Grace Delos Reyes
Nov 19 at 9:53
@GraceDelosReyes do you key in CustomerId or auto generate ?
– Saif
Nov 19 at 9:54