Would ES7 behave like the function was fully synchronous here?
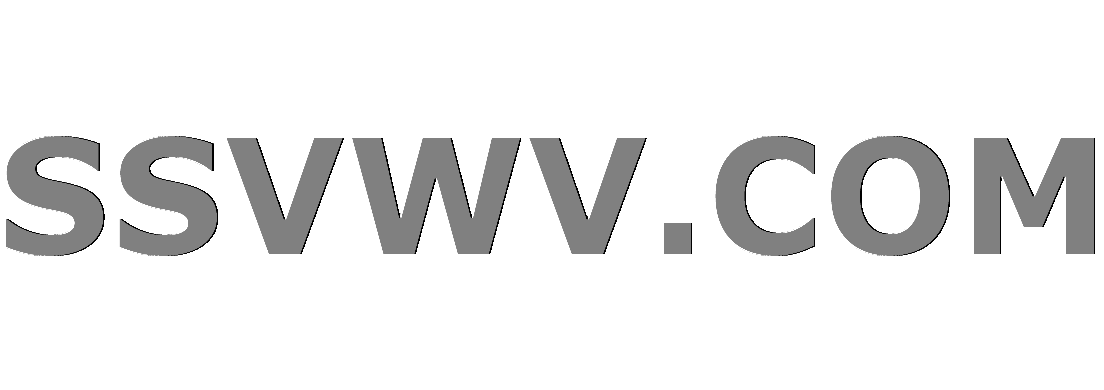
Multi tool use
up vote
3
down vote
favorite
The following case:
I have a wrapperfunction "InitializePage()" which is called when loading the page.
This one contains the functions a-d out of which a and b contain AJAX. It would look like this:
function wrapperFunction() {
a() //contains AJAX
b() //contains AJAX
c() //Synchronous
d() //Synchronous
}
Now, I want function b to start only if function a has finished already.
I know that I could easily do this by making the wrapperFunction "async", use "await" in front of function a and then return the promise from function a (using JQuery Ajax) like this:
function a() {
return $.post('somefile.php', {
//someCode
})
}
But I want to know one thing:
function a by itself should be treated by JS as SYNCHRONOUS code until it hits the JqueryAJAX inside the function, right?
Only when it hits the AJAX call, JS hands it off to the C++ API and continues to the next bit of code no matter whether the AJAX call has finished execution yet or not, right?
So lets assume, even though it would be unnecessarily hacky, I would do this:
async function a() {
await $.post('someFile.php', {
//some code
})
}
Since I synchronized the AJAX part of function a(), would this mean that at the level of wrapperFunction(), JS does NOT procede until function a() and all of its contents have finished execution?
javascript jquery ajax ecmascript-7
|
show 1 more comment
up vote
3
down vote
favorite
The following case:
I have a wrapperfunction "InitializePage()" which is called when loading the page.
This one contains the functions a-d out of which a and b contain AJAX. It would look like this:
function wrapperFunction() {
a() //contains AJAX
b() //contains AJAX
c() //Synchronous
d() //Synchronous
}
Now, I want function b to start only if function a has finished already.
I know that I could easily do this by making the wrapperFunction "async", use "await" in front of function a and then return the promise from function a (using JQuery Ajax) like this:
function a() {
return $.post('somefile.php', {
//someCode
})
}
But I want to know one thing:
function a by itself should be treated by JS as SYNCHRONOUS code until it hits the JqueryAJAX inside the function, right?
Only when it hits the AJAX call, JS hands it off to the C++ API and continues to the next bit of code no matter whether the AJAX call has finished execution yet or not, right?
So lets assume, even though it would be unnecessarily hacky, I would do this:
async function a() {
await $.post('someFile.php', {
//some code
})
}
Since I synchronized the AJAX part of function a(), would this mean that at the level of wrapperFunction(), JS does NOT procede until function a() and all of its contents have finished execution?
javascript jquery ajax ecmascript-7
1
It might help to look at theawait
code and think of what it would look like when it was written with.then()
– Bergi
Nov 19 at 9:26
You need to writeawait a()
inwrapperFunction()
to make it seem like it's synchronous. And then you need to declareasync function wrapperFunction()
– Barmar
Nov 19 at 9:27
@Barmar I know this way, but out of curiosity, I wanted to know whether the way I described would work as well or not.
– JSONBUG123
Nov 19 at 9:29
The simple answer is no. Declaring a functionasync
is just syntactic sugar for automatically returning a promise. It doesn't make it act synchronous. Theawait
keyword is what makes a call to an async function seem like it's synchronous.
– Barmar
Nov 19 at 9:31
but the keyword "async" doesn't make a function return promises? Instead, an async function enables the await keyword for use INSIDE the async function, which can await promises being returned from any function inside the function which was prefixed with "async" .
– JSONBUG123
Nov 19 at 9:32
|
show 1 more comment
up vote
3
down vote
favorite
up vote
3
down vote
favorite
The following case:
I have a wrapperfunction "InitializePage()" which is called when loading the page.
This one contains the functions a-d out of which a and b contain AJAX. It would look like this:
function wrapperFunction() {
a() //contains AJAX
b() //contains AJAX
c() //Synchronous
d() //Synchronous
}
Now, I want function b to start only if function a has finished already.
I know that I could easily do this by making the wrapperFunction "async", use "await" in front of function a and then return the promise from function a (using JQuery Ajax) like this:
function a() {
return $.post('somefile.php', {
//someCode
})
}
But I want to know one thing:
function a by itself should be treated by JS as SYNCHRONOUS code until it hits the JqueryAJAX inside the function, right?
Only when it hits the AJAX call, JS hands it off to the C++ API and continues to the next bit of code no matter whether the AJAX call has finished execution yet or not, right?
So lets assume, even though it would be unnecessarily hacky, I would do this:
async function a() {
await $.post('someFile.php', {
//some code
})
}
Since I synchronized the AJAX part of function a(), would this mean that at the level of wrapperFunction(), JS does NOT procede until function a() and all of its contents have finished execution?
javascript jquery ajax ecmascript-7
The following case:
I have a wrapperfunction "InitializePage()" which is called when loading the page.
This one contains the functions a-d out of which a and b contain AJAX. It would look like this:
function wrapperFunction() {
a() //contains AJAX
b() //contains AJAX
c() //Synchronous
d() //Synchronous
}
Now, I want function b to start only if function a has finished already.
I know that I could easily do this by making the wrapperFunction "async", use "await" in front of function a and then return the promise from function a (using JQuery Ajax) like this:
function a() {
return $.post('somefile.php', {
//someCode
})
}
But I want to know one thing:
function a by itself should be treated by JS as SYNCHRONOUS code until it hits the JqueryAJAX inside the function, right?
Only when it hits the AJAX call, JS hands it off to the C++ API and continues to the next bit of code no matter whether the AJAX call has finished execution yet or not, right?
So lets assume, even though it would be unnecessarily hacky, I would do this:
async function a() {
await $.post('someFile.php', {
//some code
})
}
Since I synchronized the AJAX part of function a(), would this mean that at the level of wrapperFunction(), JS does NOT procede until function a() and all of its contents have finished execution?
javascript jquery ajax ecmascript-7
javascript jquery ajax ecmascript-7
edited Nov 19 at 9:27
asked Nov 19 at 9:20
JSONBUG123
487
487
1
It might help to look at theawait
code and think of what it would look like when it was written with.then()
– Bergi
Nov 19 at 9:26
You need to writeawait a()
inwrapperFunction()
to make it seem like it's synchronous. And then you need to declareasync function wrapperFunction()
– Barmar
Nov 19 at 9:27
@Barmar I know this way, but out of curiosity, I wanted to know whether the way I described would work as well or not.
– JSONBUG123
Nov 19 at 9:29
The simple answer is no. Declaring a functionasync
is just syntactic sugar for automatically returning a promise. It doesn't make it act synchronous. Theawait
keyword is what makes a call to an async function seem like it's synchronous.
– Barmar
Nov 19 at 9:31
but the keyword "async" doesn't make a function return promises? Instead, an async function enables the await keyword for use INSIDE the async function, which can await promises being returned from any function inside the function which was prefixed with "async" .
– JSONBUG123
Nov 19 at 9:32
|
show 1 more comment
1
It might help to look at theawait
code and think of what it would look like when it was written with.then()
– Bergi
Nov 19 at 9:26
You need to writeawait a()
inwrapperFunction()
to make it seem like it's synchronous. And then you need to declareasync function wrapperFunction()
– Barmar
Nov 19 at 9:27
@Barmar I know this way, but out of curiosity, I wanted to know whether the way I described would work as well or not.
– JSONBUG123
Nov 19 at 9:29
The simple answer is no. Declaring a functionasync
is just syntactic sugar for automatically returning a promise. It doesn't make it act synchronous. Theawait
keyword is what makes a call to an async function seem like it's synchronous.
– Barmar
Nov 19 at 9:31
but the keyword "async" doesn't make a function return promises? Instead, an async function enables the await keyword for use INSIDE the async function, which can await promises being returned from any function inside the function which was prefixed with "async" .
– JSONBUG123
Nov 19 at 9:32
1
1
It might help to look at the
await
code and think of what it would look like when it was written with .then()
– Bergi
Nov 19 at 9:26
It might help to look at the
await
code and think of what it would look like when it was written with .then()
– Bergi
Nov 19 at 9:26
You need to write
await a()
in wrapperFunction()
to make it seem like it's synchronous. And then you need to declare async function wrapperFunction()
– Barmar
Nov 19 at 9:27
You need to write
await a()
in wrapperFunction()
to make it seem like it's synchronous. And then you need to declare async function wrapperFunction()
– Barmar
Nov 19 at 9:27
@Barmar I know this way, but out of curiosity, I wanted to know whether the way I described would work as well or not.
– JSONBUG123
Nov 19 at 9:29
@Barmar I know this way, but out of curiosity, I wanted to know whether the way I described would work as well or not.
– JSONBUG123
Nov 19 at 9:29
The simple answer is no. Declaring a function
async
is just syntactic sugar for automatically returning a promise. It doesn't make it act synchronous. The await
keyword is what makes a call to an async function seem like it's synchronous.– Barmar
Nov 19 at 9:31
The simple answer is no. Declaring a function
async
is just syntactic sugar for automatically returning a promise. It doesn't make it act synchronous. The await
keyword is what makes a call to an async function seem like it's synchronous.– Barmar
Nov 19 at 9:31
but the keyword "async" doesn't make a function return promises? Instead, an async function enables the await keyword for use INSIDE the async function, which can await promises being returned from any function inside the function which was prefixed with "async" .
– JSONBUG123
Nov 19 at 9:32
but the keyword "async" doesn't make a function return promises? Instead, an async function enables the await keyword for use INSIDE the async function, which can await promises being returned from any function inside the function which was prefixed with "async" .
– JSONBUG123
Nov 19 at 9:32
|
show 1 more comment
2 Answers
2
active
oldest
votes
up vote
1
down vote
accepted
The async function declaration defines an asynchronous function, which returns an AsyncFunction object. An asynchronous function is a function which operates asynchronously via the event loop, using an implicit Promise to return its result. But the syntax and structure of your code using async functions is much more like using standard synchronous functions.
So as per your question.
async function a() {
await $.post('someFile.php', {
//some code
})
}
yes it makes it asynchronous. but it is always better to use await where we are calling this function something like below.
for more refrenece please take a look here.
function resolveAfter2Seconds() {
return new Promise(resolve => {
setTimeout(() => {
resolve('resolved');
}, 2000);
});
}
async function asyncCall() {
console.log('calling');
var result = await resolveAfter2Seconds();
console.log(result);
// expected output: 'resolved'
}
asyncCall();
Thanks, yes it seems to work! :=) And yeah I know the way you outlined is better, I usually do it that way as well. But this time I have to operate from an AJAX thenable where a series of further AJAX calls is made. Since I want to avoid a huge pyramide of thenables and at the same time cant use the "await" keyword inside a then(), I want to do it this way. Or can you show me how to use the await keyword inside a then? If so, I can open up another question for this, thanks a lot!
– JSONBUG123
Nov 19 at 9:48
add a comment |
up vote
0
down vote
If we take "fully asynchronous" to mean that there is nothing put on the Event Loop then no, your function a
would not become fully synchronous.
Using async/await does not make asynchronous code synchronous, it is simply syntax that helps the developer reason with Promises more easily, essentially by abstracting away the Promise API into the language.
Since I synchronized the AJAX part of function a(), would this mean that at the level of wrapperFunction(), JS does NOT procede until function a() and all of its contents have finished execution?
With the caveat that your function a
is not "synchronized" by using async/await, callers of a
can still wait for its execution to complete before continuing to the next statement, but only if the call to a
is made using the await
keyword, like so:
async function a () {
return someAsyncOperation()
}
async function waitsOnA () {
console.log('a started')
await a()
console.log('a completed')
}
// equivalent to
function waitsOnA () {
console.log('a started')
a().then(() => {
console.log('a completed')
})
}
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
The async function declaration defines an asynchronous function, which returns an AsyncFunction object. An asynchronous function is a function which operates asynchronously via the event loop, using an implicit Promise to return its result. But the syntax and structure of your code using async functions is much more like using standard synchronous functions.
So as per your question.
async function a() {
await $.post('someFile.php', {
//some code
})
}
yes it makes it asynchronous. but it is always better to use await where we are calling this function something like below.
for more refrenece please take a look here.
function resolveAfter2Seconds() {
return new Promise(resolve => {
setTimeout(() => {
resolve('resolved');
}, 2000);
});
}
async function asyncCall() {
console.log('calling');
var result = await resolveAfter2Seconds();
console.log(result);
// expected output: 'resolved'
}
asyncCall();
Thanks, yes it seems to work! :=) And yeah I know the way you outlined is better, I usually do it that way as well. But this time I have to operate from an AJAX thenable where a series of further AJAX calls is made. Since I want to avoid a huge pyramide of thenables and at the same time cant use the "await" keyword inside a then(), I want to do it this way. Or can you show me how to use the await keyword inside a then? If so, I can open up another question for this, thanks a lot!
– JSONBUG123
Nov 19 at 9:48
add a comment |
up vote
1
down vote
accepted
The async function declaration defines an asynchronous function, which returns an AsyncFunction object. An asynchronous function is a function which operates asynchronously via the event loop, using an implicit Promise to return its result. But the syntax and structure of your code using async functions is much more like using standard synchronous functions.
So as per your question.
async function a() {
await $.post('someFile.php', {
//some code
})
}
yes it makes it asynchronous. but it is always better to use await where we are calling this function something like below.
for more refrenece please take a look here.
function resolveAfter2Seconds() {
return new Promise(resolve => {
setTimeout(() => {
resolve('resolved');
}, 2000);
});
}
async function asyncCall() {
console.log('calling');
var result = await resolveAfter2Seconds();
console.log(result);
// expected output: 'resolved'
}
asyncCall();
Thanks, yes it seems to work! :=) And yeah I know the way you outlined is better, I usually do it that way as well. But this time I have to operate from an AJAX thenable where a series of further AJAX calls is made. Since I want to avoid a huge pyramide of thenables and at the same time cant use the "await" keyword inside a then(), I want to do it this way. Or can you show me how to use the await keyword inside a then? If so, I can open up another question for this, thanks a lot!
– JSONBUG123
Nov 19 at 9:48
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
The async function declaration defines an asynchronous function, which returns an AsyncFunction object. An asynchronous function is a function which operates asynchronously via the event loop, using an implicit Promise to return its result. But the syntax and structure of your code using async functions is much more like using standard synchronous functions.
So as per your question.
async function a() {
await $.post('someFile.php', {
//some code
})
}
yes it makes it asynchronous. but it is always better to use await where we are calling this function something like below.
for more refrenece please take a look here.
function resolveAfter2Seconds() {
return new Promise(resolve => {
setTimeout(() => {
resolve('resolved');
}, 2000);
});
}
async function asyncCall() {
console.log('calling');
var result = await resolveAfter2Seconds();
console.log(result);
// expected output: 'resolved'
}
asyncCall();
The async function declaration defines an asynchronous function, which returns an AsyncFunction object. An asynchronous function is a function which operates asynchronously via the event loop, using an implicit Promise to return its result. But the syntax and structure of your code using async functions is much more like using standard synchronous functions.
So as per your question.
async function a() {
await $.post('someFile.php', {
//some code
})
}
yes it makes it asynchronous. but it is always better to use await where we are calling this function something like below.
for more refrenece please take a look here.
function resolveAfter2Seconds() {
return new Promise(resolve => {
setTimeout(() => {
resolve('resolved');
}, 2000);
});
}
async function asyncCall() {
console.log('calling');
var result = await resolveAfter2Seconds();
console.log(result);
// expected output: 'resolved'
}
asyncCall();
edited Nov 19 at 9:56
answered Nov 19 at 9:42


Negi Rox
1,6321511
1,6321511
Thanks, yes it seems to work! :=) And yeah I know the way you outlined is better, I usually do it that way as well. But this time I have to operate from an AJAX thenable where a series of further AJAX calls is made. Since I want to avoid a huge pyramide of thenables and at the same time cant use the "await" keyword inside a then(), I want to do it this way. Or can you show me how to use the await keyword inside a then? If so, I can open up another question for this, thanks a lot!
– JSONBUG123
Nov 19 at 9:48
add a comment |
Thanks, yes it seems to work! :=) And yeah I know the way you outlined is better, I usually do it that way as well. But this time I have to operate from an AJAX thenable where a series of further AJAX calls is made. Since I want to avoid a huge pyramide of thenables and at the same time cant use the "await" keyword inside a then(), I want to do it this way. Or can you show me how to use the await keyword inside a then? If so, I can open up another question for this, thanks a lot!
– JSONBUG123
Nov 19 at 9:48
Thanks, yes it seems to work! :=) And yeah I know the way you outlined is better, I usually do it that way as well. But this time I have to operate from an AJAX thenable where a series of further AJAX calls is made. Since I want to avoid a huge pyramide of thenables and at the same time cant use the "await" keyword inside a then(), I want to do it this way. Or can you show me how to use the await keyword inside a then? If so, I can open up another question for this, thanks a lot!
– JSONBUG123
Nov 19 at 9:48
Thanks, yes it seems to work! :=) And yeah I know the way you outlined is better, I usually do it that way as well. But this time I have to operate from an AJAX thenable where a series of further AJAX calls is made. Since I want to avoid a huge pyramide of thenables and at the same time cant use the "await" keyword inside a then(), I want to do it this way. Or can you show me how to use the await keyword inside a then? If so, I can open up another question for this, thanks a lot!
– JSONBUG123
Nov 19 at 9:48
add a comment |
up vote
0
down vote
If we take "fully asynchronous" to mean that there is nothing put on the Event Loop then no, your function a
would not become fully synchronous.
Using async/await does not make asynchronous code synchronous, it is simply syntax that helps the developer reason with Promises more easily, essentially by abstracting away the Promise API into the language.
Since I synchronized the AJAX part of function a(), would this mean that at the level of wrapperFunction(), JS does NOT procede until function a() and all of its contents have finished execution?
With the caveat that your function a
is not "synchronized" by using async/await, callers of a
can still wait for its execution to complete before continuing to the next statement, but only if the call to a
is made using the await
keyword, like so:
async function a () {
return someAsyncOperation()
}
async function waitsOnA () {
console.log('a started')
await a()
console.log('a completed')
}
// equivalent to
function waitsOnA () {
console.log('a started')
a().then(() => {
console.log('a completed')
})
}
add a comment |
up vote
0
down vote
If we take "fully asynchronous" to mean that there is nothing put on the Event Loop then no, your function a
would not become fully synchronous.
Using async/await does not make asynchronous code synchronous, it is simply syntax that helps the developer reason with Promises more easily, essentially by abstracting away the Promise API into the language.
Since I synchronized the AJAX part of function a(), would this mean that at the level of wrapperFunction(), JS does NOT procede until function a() and all of its contents have finished execution?
With the caveat that your function a
is not "synchronized" by using async/await, callers of a
can still wait for its execution to complete before continuing to the next statement, but only if the call to a
is made using the await
keyword, like so:
async function a () {
return someAsyncOperation()
}
async function waitsOnA () {
console.log('a started')
await a()
console.log('a completed')
}
// equivalent to
function waitsOnA () {
console.log('a started')
a().then(() => {
console.log('a completed')
})
}
add a comment |
up vote
0
down vote
up vote
0
down vote
If we take "fully asynchronous" to mean that there is nothing put on the Event Loop then no, your function a
would not become fully synchronous.
Using async/await does not make asynchronous code synchronous, it is simply syntax that helps the developer reason with Promises more easily, essentially by abstracting away the Promise API into the language.
Since I synchronized the AJAX part of function a(), would this mean that at the level of wrapperFunction(), JS does NOT procede until function a() and all of its contents have finished execution?
With the caveat that your function a
is not "synchronized" by using async/await, callers of a
can still wait for its execution to complete before continuing to the next statement, but only if the call to a
is made using the await
keyword, like so:
async function a () {
return someAsyncOperation()
}
async function waitsOnA () {
console.log('a started')
await a()
console.log('a completed')
}
// equivalent to
function waitsOnA () {
console.log('a started')
a().then(() => {
console.log('a completed')
})
}
If we take "fully asynchronous" to mean that there is nothing put on the Event Loop then no, your function a
would not become fully synchronous.
Using async/await does not make asynchronous code synchronous, it is simply syntax that helps the developer reason with Promises more easily, essentially by abstracting away the Promise API into the language.
Since I synchronized the AJAX part of function a(), would this mean that at the level of wrapperFunction(), JS does NOT procede until function a() and all of its contents have finished execution?
With the caveat that your function a
is not "synchronized" by using async/await, callers of a
can still wait for its execution to complete before continuing to the next statement, but only if the call to a
is made using the await
keyword, like so:
async function a () {
return someAsyncOperation()
}
async function waitsOnA () {
console.log('a started')
await a()
console.log('a completed')
}
// equivalent to
function waitsOnA () {
console.log('a started')
a().then(() => {
console.log('a completed')
})
}
answered Nov 19 at 9:42


sdgluck
9,54612548
9,54612548
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53371525%2fwould-es7-behave-like-the-function-was-fully-synchronous-here%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
pF0VNldpBS ER5Vc8w1
1
It might help to look at the
await
code and think of what it would look like when it was written with.then()
– Bergi
Nov 19 at 9:26
You need to write
await a()
inwrapperFunction()
to make it seem like it's synchronous. And then you need to declareasync function wrapperFunction()
– Barmar
Nov 19 at 9:27
@Barmar I know this way, but out of curiosity, I wanted to know whether the way I described would work as well or not.
– JSONBUG123
Nov 19 at 9:29
The simple answer is no. Declaring a function
async
is just syntactic sugar for automatically returning a promise. It doesn't make it act synchronous. Theawait
keyword is what makes a call to an async function seem like it's synchronous.– Barmar
Nov 19 at 9:31
but the keyword "async" doesn't make a function return promises? Instead, an async function enables the await keyword for use INSIDE the async function, which can await promises being returned from any function inside the function which was prefixed with "async" .
– JSONBUG123
Nov 19 at 9:32