OrderBy an list in a task function async
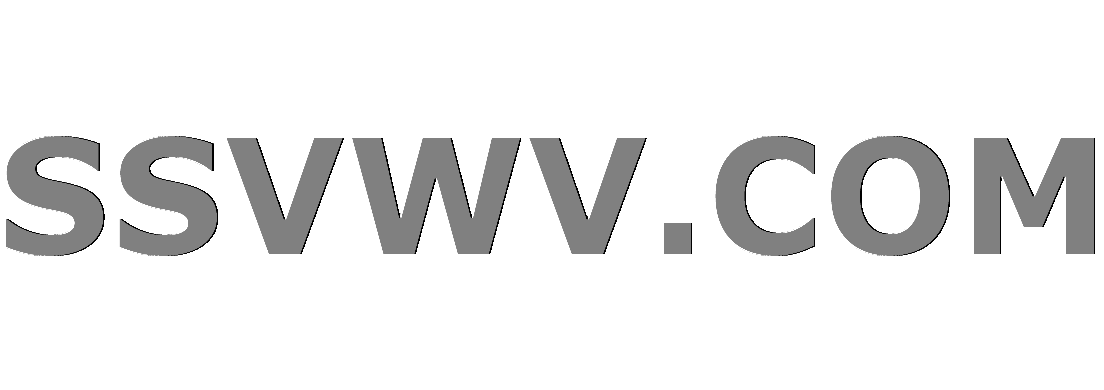
Multi tool use
up vote
1
down vote
favorite
Okay i am working with orleans and all i really want to do is sort an list after a value in state. i try to this by doing this
public async Task SortEntries()
{
State.Entries.OrderBy(GetComparator);
}
private async decimal GetComparator(IEntryGrain x)
{
var a = await x.GetState();
return Task.FromResult(a);
}
but this got two wrongs in them that i am trying to solve. first of the Task SortEntries task lacks an await operator which i guess might still work the problem is that GetComparator says an async method must be void, Task or Task.The most neat way i first thought would be to do all the sorting in SortEntries like this
State.Entries.OrderBy((x) => x.GetState().Result.TotalPoints);
But the GetState() need to be async with an await but i cant do that on the orderBy or sort. Anyone who can push me in the right direction or encountered something similar
c# asynchronous orleans
add a comment |
up vote
1
down vote
favorite
Okay i am working with orleans and all i really want to do is sort an list after a value in state. i try to this by doing this
public async Task SortEntries()
{
State.Entries.OrderBy(GetComparator);
}
private async decimal GetComparator(IEntryGrain x)
{
var a = await x.GetState();
return Task.FromResult(a);
}
but this got two wrongs in them that i am trying to solve. first of the Task SortEntries task lacks an await operator which i guess might still work the problem is that GetComparator says an async method must be void, Task or Task.The most neat way i first thought would be to do all the sorting in SortEntries like this
State.Entries.OrderBy((x) => x.GetState().Result.TotalPoints);
But the GetState() need to be async with an await but i cant do that on the orderBy or sort. Anyone who can push me in the right direction or encountered something similar
c# asynchronous orleans
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
Okay i am working with orleans and all i really want to do is sort an list after a value in state. i try to this by doing this
public async Task SortEntries()
{
State.Entries.OrderBy(GetComparator);
}
private async decimal GetComparator(IEntryGrain x)
{
var a = await x.GetState();
return Task.FromResult(a);
}
but this got two wrongs in them that i am trying to solve. first of the Task SortEntries task lacks an await operator which i guess might still work the problem is that GetComparator says an async method must be void, Task or Task.The most neat way i first thought would be to do all the sorting in SortEntries like this
State.Entries.OrderBy((x) => x.GetState().Result.TotalPoints);
But the GetState() need to be async with an await but i cant do that on the orderBy or sort. Anyone who can push me in the right direction or encountered something similar
c# asynchronous orleans
Okay i am working with orleans and all i really want to do is sort an list after a value in state. i try to this by doing this
public async Task SortEntries()
{
State.Entries.OrderBy(GetComparator);
}
private async decimal GetComparator(IEntryGrain x)
{
var a = await x.GetState();
return Task.FromResult(a);
}
but this got two wrongs in them that i am trying to solve. first of the Task SortEntries task lacks an await operator which i guess might still work the problem is that GetComparator says an async method must be void, Task or Task.The most neat way i first thought would be to do all the sorting in SortEntries like this
State.Entries.OrderBy((x) => x.GetState().Result.TotalPoints);
But the GetState() need to be async with an await but i cant do that on the orderBy or sort. Anyone who can push me in the right direction or encountered something similar
c# asynchronous orleans
c# asynchronous orleans
asked Nov 16 at 8:42


Johan Jönsson
548
548
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
up vote
2
down vote
accepted
1.) OrderBy
doesn't work with async/await. A qiuck fix would be to use the Result property of GetState in GetComparator
private decimal GetComparator(IEntryGrain x)
{
var task = x.GetState();
task.Wait();
if(task.IsFaulted)
throw new Exception($"could not get the state: {task.Exception}", task.Exception);
return task.Result;
}
2.) OrderBy
return only an IEnumerable
, so it wont be ordered there. Need to enumerate it once to execute the order by. And you need to return the result because OrderBy
only returns a ordered list, but does not order the list itself.
public IList<IEntryGrain> SortEntries()
{
return State.Entries.OrderBy(GetComparator)
.ToList();
}
or write it back to the property if it is writable and thread safe
public void SortEntries()
{
State.Entries = State.Entries.OrderBy(GetComparator)
.ToList();
}
So you get:
public IList<IEntryGrain> SortEntries()
{
return State.Entries.OrderBy(GetComparator)
.ToList();
}
private decimal GetComparator(IEntryGrain x)
{
var task = x.GetState();
task.Wait();
if(task.IsFaulted)
throw new Exception($"could not get the state: {task.Exception}", task.Exception);
return task.Result;
}
State.Entries.OrderBy(async (x) => await GetComparator(x));
andState.Entries.OrderBy(GetComparator);
behave identially other than the fact that your version allocates more objects and takes more time. Other than the reduced performance, there's no observable difference between the two. Additionally your second and final methods don't compile at all, because you're returning a type that the compiler is not expecting to be returned for those methods.
– Servy
Nov 16 at 14:20
3
Now you're just synchronously blocking on asynchronous operations and that's going to just deadlock, and not do the work asynchronously, which is the whole point of having asynchronous operations here in the first place.
– Servy
Nov 16 at 17:45
1
It will kill Orleans scheduler, never use blocking calls in Orleans! I would prefer to rethink the whole problem. Do you really want to call another grain for each comparison during each sort? It will kill the performance even without the blocking call: entry grains will be loaded into memory from DB, usually on other nodes, etc. If the Entries are some references to grains, cache the values used for comparison. But do you really need grains for the entries at all? Or just POCOs? Are the entries DDD/aggregate roots?
– lmagyar
Nov 18 at 15:22
1
Converting the async method to sync is a bad idea, as @Servy has suggested, you shall just use plain Async
– Mrinal Kamboj
Nov 19 at 4:40
add a comment |
up vote
0
down vote
I don't see any issue in what you want to achieve, but there's a discrepancy in your question, let me point that out:
From GetComparator
method, x.GetState()
leads decimal
return value, but then finally your OrderBy
statement is: .OrderBy((x) => x.GetState().Result.TotalPoints)
, which is not feasible, therefore GetState()
shall lead to a type, which has a decimal
property TotalPoints
, Now to the Main question.
Check out the following sample, where I have made few assumptions to compile the code
public async Task<IOrderedEnumerable<State>> SortEntries(List<State> stateEntries)
{
var orderedEnumerable = stateEntries.OrderBy(async y => await GetComparator(y));
return await Task.FromResult(orderedEnumerable);
}
private async Task<decimal> GetComparator(State x)
{
var a = await x.GetState();
return a.TotalPoints;
}
public class State
{
public int Id {get; set;}
public decimal TotalPoints {get;set;}
public async Task<State> GetState()
{
return await Task.FromResult(this);
}
}
Assumptions:
State
class defines the Schema, which containsAsync
methodGetState
, which is used for further logical processing
State
class containsTotalPoints
, which is used for the Ordered Processing
How it Works:
Async
method shall always return aTask
and have at-least 1await
for being truly Asynchronous call, rest of the call can be Synchronous, we normally expect a Network call to be Async / Non blocking
Lambda
can beAsync
, as shown in the code, which Async processing for theOrderBy
call- Wrapping up in
Task.FromResult
is a convenience, which is used often for testing frameworks
SortEntries
entries method needn'tAsync
, until and unlessOrleans
or similar framework needs it. Though ideallyAsync
shall be all the way to the entry point likeMain
method in the Console, which is entry / starting point
Important point related to Async calls, Await keyword:
- They can return
void / Task / Task<T>
, with method signature containingasync
, it can become truly async on usage of keywordawait
at least once (to avoid blocking Network calls).await
is mostly called on methods returning these three types. There's another optionValueTask
.
await
onvoid
andTask
return functions has not return value, but when used overTask<T>
, it unwraps theTask<T>
to fetch the resultT
, now this value T can be utilized in the function locally and when we return likereturn T
, for a function with return valueTask<T>
, framework would automatically wrap in aTask
, so that it can be further awaited till the entry point, which Framework can awaits Asynchronously
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
1.) OrderBy
doesn't work with async/await. A qiuck fix would be to use the Result property of GetState in GetComparator
private decimal GetComparator(IEntryGrain x)
{
var task = x.GetState();
task.Wait();
if(task.IsFaulted)
throw new Exception($"could not get the state: {task.Exception}", task.Exception);
return task.Result;
}
2.) OrderBy
return only an IEnumerable
, so it wont be ordered there. Need to enumerate it once to execute the order by. And you need to return the result because OrderBy
only returns a ordered list, but does not order the list itself.
public IList<IEntryGrain> SortEntries()
{
return State.Entries.OrderBy(GetComparator)
.ToList();
}
or write it back to the property if it is writable and thread safe
public void SortEntries()
{
State.Entries = State.Entries.OrderBy(GetComparator)
.ToList();
}
So you get:
public IList<IEntryGrain> SortEntries()
{
return State.Entries.OrderBy(GetComparator)
.ToList();
}
private decimal GetComparator(IEntryGrain x)
{
var task = x.GetState();
task.Wait();
if(task.IsFaulted)
throw new Exception($"could not get the state: {task.Exception}", task.Exception);
return task.Result;
}
State.Entries.OrderBy(async (x) => await GetComparator(x));
andState.Entries.OrderBy(GetComparator);
behave identially other than the fact that your version allocates more objects and takes more time. Other than the reduced performance, there's no observable difference between the two. Additionally your second and final methods don't compile at all, because you're returning a type that the compiler is not expecting to be returned for those methods.
– Servy
Nov 16 at 14:20
3
Now you're just synchronously blocking on asynchronous operations and that's going to just deadlock, and not do the work asynchronously, which is the whole point of having asynchronous operations here in the first place.
– Servy
Nov 16 at 17:45
1
It will kill Orleans scheduler, never use blocking calls in Orleans! I would prefer to rethink the whole problem. Do you really want to call another grain for each comparison during each sort? It will kill the performance even without the blocking call: entry grains will be loaded into memory from DB, usually on other nodes, etc. If the Entries are some references to grains, cache the values used for comparison. But do you really need grains for the entries at all? Or just POCOs? Are the entries DDD/aggregate roots?
– lmagyar
Nov 18 at 15:22
1
Converting the async method to sync is a bad idea, as @Servy has suggested, you shall just use plain Async
– Mrinal Kamboj
Nov 19 at 4:40
add a comment |
up vote
2
down vote
accepted
1.) OrderBy
doesn't work with async/await. A qiuck fix would be to use the Result property of GetState in GetComparator
private decimal GetComparator(IEntryGrain x)
{
var task = x.GetState();
task.Wait();
if(task.IsFaulted)
throw new Exception($"could not get the state: {task.Exception}", task.Exception);
return task.Result;
}
2.) OrderBy
return only an IEnumerable
, so it wont be ordered there. Need to enumerate it once to execute the order by. And you need to return the result because OrderBy
only returns a ordered list, but does not order the list itself.
public IList<IEntryGrain> SortEntries()
{
return State.Entries.OrderBy(GetComparator)
.ToList();
}
or write it back to the property if it is writable and thread safe
public void SortEntries()
{
State.Entries = State.Entries.OrderBy(GetComparator)
.ToList();
}
So you get:
public IList<IEntryGrain> SortEntries()
{
return State.Entries.OrderBy(GetComparator)
.ToList();
}
private decimal GetComparator(IEntryGrain x)
{
var task = x.GetState();
task.Wait();
if(task.IsFaulted)
throw new Exception($"could not get the state: {task.Exception}", task.Exception);
return task.Result;
}
State.Entries.OrderBy(async (x) => await GetComparator(x));
andState.Entries.OrderBy(GetComparator);
behave identially other than the fact that your version allocates more objects and takes more time. Other than the reduced performance, there's no observable difference between the two. Additionally your second and final methods don't compile at all, because you're returning a type that the compiler is not expecting to be returned for those methods.
– Servy
Nov 16 at 14:20
3
Now you're just synchronously blocking on asynchronous operations and that's going to just deadlock, and not do the work asynchronously, which is the whole point of having asynchronous operations here in the first place.
– Servy
Nov 16 at 17:45
1
It will kill Orleans scheduler, never use blocking calls in Orleans! I would prefer to rethink the whole problem. Do you really want to call another grain for each comparison during each sort? It will kill the performance even without the blocking call: entry grains will be loaded into memory from DB, usually on other nodes, etc. If the Entries are some references to grains, cache the values used for comparison. But do you really need grains for the entries at all? Or just POCOs? Are the entries DDD/aggregate roots?
– lmagyar
Nov 18 at 15:22
1
Converting the async method to sync is a bad idea, as @Servy has suggested, you shall just use plain Async
– Mrinal Kamboj
Nov 19 at 4:40
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
1.) OrderBy
doesn't work with async/await. A qiuck fix would be to use the Result property of GetState in GetComparator
private decimal GetComparator(IEntryGrain x)
{
var task = x.GetState();
task.Wait();
if(task.IsFaulted)
throw new Exception($"could not get the state: {task.Exception}", task.Exception);
return task.Result;
}
2.) OrderBy
return only an IEnumerable
, so it wont be ordered there. Need to enumerate it once to execute the order by. And you need to return the result because OrderBy
only returns a ordered list, but does not order the list itself.
public IList<IEntryGrain> SortEntries()
{
return State.Entries.OrderBy(GetComparator)
.ToList();
}
or write it back to the property if it is writable and thread safe
public void SortEntries()
{
State.Entries = State.Entries.OrderBy(GetComparator)
.ToList();
}
So you get:
public IList<IEntryGrain> SortEntries()
{
return State.Entries.OrderBy(GetComparator)
.ToList();
}
private decimal GetComparator(IEntryGrain x)
{
var task = x.GetState();
task.Wait();
if(task.IsFaulted)
throw new Exception($"could not get the state: {task.Exception}", task.Exception);
return task.Result;
}
1.) OrderBy
doesn't work with async/await. A qiuck fix would be to use the Result property of GetState in GetComparator
private decimal GetComparator(IEntryGrain x)
{
var task = x.GetState();
task.Wait();
if(task.IsFaulted)
throw new Exception($"could not get the state: {task.Exception}", task.Exception);
return task.Result;
}
2.) OrderBy
return only an IEnumerable
, so it wont be ordered there. Need to enumerate it once to execute the order by. And you need to return the result because OrderBy
only returns a ordered list, but does not order the list itself.
public IList<IEntryGrain> SortEntries()
{
return State.Entries.OrderBy(GetComparator)
.ToList();
}
or write it back to the property if it is writable and thread safe
public void SortEntries()
{
State.Entries = State.Entries.OrderBy(GetComparator)
.ToList();
}
So you get:
public IList<IEntryGrain> SortEntries()
{
return State.Entries.OrderBy(GetComparator)
.ToList();
}
private decimal GetComparator(IEntryGrain x)
{
var task = x.GetState();
task.Wait();
if(task.IsFaulted)
throw new Exception($"could not get the state: {task.Exception}", task.Exception);
return task.Result;
}
edited 2 days ago
answered Nov 16 at 8:52


Maximilian Ast
1,88152232
1,88152232
State.Entries.OrderBy(async (x) => await GetComparator(x));
andState.Entries.OrderBy(GetComparator);
behave identially other than the fact that your version allocates more objects and takes more time. Other than the reduced performance, there's no observable difference between the two. Additionally your second and final methods don't compile at all, because you're returning a type that the compiler is not expecting to be returned for those methods.
– Servy
Nov 16 at 14:20
3
Now you're just synchronously blocking on asynchronous operations and that's going to just deadlock, and not do the work asynchronously, which is the whole point of having asynchronous operations here in the first place.
– Servy
Nov 16 at 17:45
1
It will kill Orleans scheduler, never use blocking calls in Orleans! I would prefer to rethink the whole problem. Do you really want to call another grain for each comparison during each sort? It will kill the performance even without the blocking call: entry grains will be loaded into memory from DB, usually on other nodes, etc. If the Entries are some references to grains, cache the values used for comparison. But do you really need grains for the entries at all? Or just POCOs? Are the entries DDD/aggregate roots?
– lmagyar
Nov 18 at 15:22
1
Converting the async method to sync is a bad idea, as @Servy has suggested, you shall just use plain Async
– Mrinal Kamboj
Nov 19 at 4:40
add a comment |
State.Entries.OrderBy(async (x) => await GetComparator(x));
andState.Entries.OrderBy(GetComparator);
behave identially other than the fact that your version allocates more objects and takes more time. Other than the reduced performance, there's no observable difference between the two. Additionally your second and final methods don't compile at all, because you're returning a type that the compiler is not expecting to be returned for those methods.
– Servy
Nov 16 at 14:20
3
Now you're just synchronously blocking on asynchronous operations and that's going to just deadlock, and not do the work asynchronously, which is the whole point of having asynchronous operations here in the first place.
– Servy
Nov 16 at 17:45
1
It will kill Orleans scheduler, never use blocking calls in Orleans! I would prefer to rethink the whole problem. Do you really want to call another grain for each comparison during each sort? It will kill the performance even without the blocking call: entry grains will be loaded into memory from DB, usually on other nodes, etc. If the Entries are some references to grains, cache the values used for comparison. But do you really need grains for the entries at all? Or just POCOs? Are the entries DDD/aggregate roots?
– lmagyar
Nov 18 at 15:22
1
Converting the async method to sync is a bad idea, as @Servy has suggested, you shall just use plain Async
– Mrinal Kamboj
Nov 19 at 4:40
State.Entries.OrderBy(async (x) => await GetComparator(x));
and State.Entries.OrderBy(GetComparator);
behave identially other than the fact that your version allocates more objects and takes more time. Other than the reduced performance, there's no observable difference between the two. Additionally your second and final methods don't compile at all, because you're returning a type that the compiler is not expecting to be returned for those methods.– Servy
Nov 16 at 14:20
State.Entries.OrderBy(async (x) => await GetComparator(x));
and State.Entries.OrderBy(GetComparator);
behave identially other than the fact that your version allocates more objects and takes more time. Other than the reduced performance, there's no observable difference between the two. Additionally your second and final methods don't compile at all, because you're returning a type that the compiler is not expecting to be returned for those methods.– Servy
Nov 16 at 14:20
3
3
Now you're just synchronously blocking on asynchronous operations and that's going to just deadlock, and not do the work asynchronously, which is the whole point of having asynchronous operations here in the first place.
– Servy
Nov 16 at 17:45
Now you're just synchronously blocking on asynchronous operations and that's going to just deadlock, and not do the work asynchronously, which is the whole point of having asynchronous operations here in the first place.
– Servy
Nov 16 at 17:45
1
1
It will kill Orleans scheduler, never use blocking calls in Orleans! I would prefer to rethink the whole problem. Do you really want to call another grain for each comparison during each sort? It will kill the performance even without the blocking call: entry grains will be loaded into memory from DB, usually on other nodes, etc. If the Entries are some references to grains, cache the values used for comparison. But do you really need grains for the entries at all? Or just POCOs? Are the entries DDD/aggregate roots?
– lmagyar
Nov 18 at 15:22
It will kill Orleans scheduler, never use blocking calls in Orleans! I would prefer to rethink the whole problem. Do you really want to call another grain for each comparison during each sort? It will kill the performance even without the blocking call: entry grains will be loaded into memory from DB, usually on other nodes, etc. If the Entries are some references to grains, cache the values used for comparison. But do you really need grains for the entries at all? Or just POCOs? Are the entries DDD/aggregate roots?
– lmagyar
Nov 18 at 15:22
1
1
Converting the async method to sync is a bad idea, as @Servy has suggested, you shall just use plain Async
– Mrinal Kamboj
Nov 19 at 4:40
Converting the async method to sync is a bad idea, as @Servy has suggested, you shall just use plain Async
– Mrinal Kamboj
Nov 19 at 4:40
add a comment |
up vote
0
down vote
I don't see any issue in what you want to achieve, but there's a discrepancy in your question, let me point that out:
From GetComparator
method, x.GetState()
leads decimal
return value, but then finally your OrderBy
statement is: .OrderBy((x) => x.GetState().Result.TotalPoints)
, which is not feasible, therefore GetState()
shall lead to a type, which has a decimal
property TotalPoints
, Now to the Main question.
Check out the following sample, where I have made few assumptions to compile the code
public async Task<IOrderedEnumerable<State>> SortEntries(List<State> stateEntries)
{
var orderedEnumerable = stateEntries.OrderBy(async y => await GetComparator(y));
return await Task.FromResult(orderedEnumerable);
}
private async Task<decimal> GetComparator(State x)
{
var a = await x.GetState();
return a.TotalPoints;
}
public class State
{
public int Id {get; set;}
public decimal TotalPoints {get;set;}
public async Task<State> GetState()
{
return await Task.FromResult(this);
}
}
Assumptions:
State
class defines the Schema, which containsAsync
methodGetState
, which is used for further logical processing
State
class containsTotalPoints
, which is used for the Ordered Processing
How it Works:
Async
method shall always return aTask
and have at-least 1await
for being truly Asynchronous call, rest of the call can be Synchronous, we normally expect a Network call to be Async / Non blocking
Lambda
can beAsync
, as shown in the code, which Async processing for theOrderBy
call- Wrapping up in
Task.FromResult
is a convenience, which is used often for testing frameworks
SortEntries
entries method needn'tAsync
, until and unlessOrleans
or similar framework needs it. Though ideallyAsync
shall be all the way to the entry point likeMain
method in the Console, which is entry / starting point
Important point related to Async calls, Await keyword:
- They can return
void / Task / Task<T>
, with method signature containingasync
, it can become truly async on usage of keywordawait
at least once (to avoid blocking Network calls).await
is mostly called on methods returning these three types. There's another optionValueTask
.
await
onvoid
andTask
return functions has not return value, but when used overTask<T>
, it unwraps theTask<T>
to fetch the resultT
, now this value T can be utilized in the function locally and when we return likereturn T
, for a function with return valueTask<T>
, framework would automatically wrap in aTask
, so that it can be further awaited till the entry point, which Framework can awaits Asynchronously
add a comment |
up vote
0
down vote
I don't see any issue in what you want to achieve, but there's a discrepancy in your question, let me point that out:
From GetComparator
method, x.GetState()
leads decimal
return value, but then finally your OrderBy
statement is: .OrderBy((x) => x.GetState().Result.TotalPoints)
, which is not feasible, therefore GetState()
shall lead to a type, which has a decimal
property TotalPoints
, Now to the Main question.
Check out the following sample, where I have made few assumptions to compile the code
public async Task<IOrderedEnumerable<State>> SortEntries(List<State> stateEntries)
{
var orderedEnumerable = stateEntries.OrderBy(async y => await GetComparator(y));
return await Task.FromResult(orderedEnumerable);
}
private async Task<decimal> GetComparator(State x)
{
var a = await x.GetState();
return a.TotalPoints;
}
public class State
{
public int Id {get; set;}
public decimal TotalPoints {get;set;}
public async Task<State> GetState()
{
return await Task.FromResult(this);
}
}
Assumptions:
State
class defines the Schema, which containsAsync
methodGetState
, which is used for further logical processing
State
class containsTotalPoints
, which is used for the Ordered Processing
How it Works:
Async
method shall always return aTask
and have at-least 1await
for being truly Asynchronous call, rest of the call can be Synchronous, we normally expect a Network call to be Async / Non blocking
Lambda
can beAsync
, as shown in the code, which Async processing for theOrderBy
call- Wrapping up in
Task.FromResult
is a convenience, which is used often for testing frameworks
SortEntries
entries method needn'tAsync
, until and unlessOrleans
or similar framework needs it. Though ideallyAsync
shall be all the way to the entry point likeMain
method in the Console, which is entry / starting point
Important point related to Async calls, Await keyword:
- They can return
void / Task / Task<T>
, with method signature containingasync
, it can become truly async on usage of keywordawait
at least once (to avoid blocking Network calls).await
is mostly called on methods returning these three types. There's another optionValueTask
.
await
onvoid
andTask
return functions has not return value, but when used overTask<T>
, it unwraps theTask<T>
to fetch the resultT
, now this value T can be utilized in the function locally and when we return likereturn T
, for a function with return valueTask<T>
, framework would automatically wrap in aTask
, so that it can be further awaited till the entry point, which Framework can awaits Asynchronously
add a comment |
up vote
0
down vote
up vote
0
down vote
I don't see any issue in what you want to achieve, but there's a discrepancy in your question, let me point that out:
From GetComparator
method, x.GetState()
leads decimal
return value, but then finally your OrderBy
statement is: .OrderBy((x) => x.GetState().Result.TotalPoints)
, which is not feasible, therefore GetState()
shall lead to a type, which has a decimal
property TotalPoints
, Now to the Main question.
Check out the following sample, where I have made few assumptions to compile the code
public async Task<IOrderedEnumerable<State>> SortEntries(List<State> stateEntries)
{
var orderedEnumerable = stateEntries.OrderBy(async y => await GetComparator(y));
return await Task.FromResult(orderedEnumerable);
}
private async Task<decimal> GetComparator(State x)
{
var a = await x.GetState();
return a.TotalPoints;
}
public class State
{
public int Id {get; set;}
public decimal TotalPoints {get;set;}
public async Task<State> GetState()
{
return await Task.FromResult(this);
}
}
Assumptions:
State
class defines the Schema, which containsAsync
methodGetState
, which is used for further logical processing
State
class containsTotalPoints
, which is used for the Ordered Processing
How it Works:
Async
method shall always return aTask
and have at-least 1await
for being truly Asynchronous call, rest of the call can be Synchronous, we normally expect a Network call to be Async / Non blocking
Lambda
can beAsync
, as shown in the code, which Async processing for theOrderBy
call- Wrapping up in
Task.FromResult
is a convenience, which is used often for testing frameworks
SortEntries
entries method needn'tAsync
, until and unlessOrleans
or similar framework needs it. Though ideallyAsync
shall be all the way to the entry point likeMain
method in the Console, which is entry / starting point
Important point related to Async calls, Await keyword:
- They can return
void / Task / Task<T>
, with method signature containingasync
, it can become truly async on usage of keywordawait
at least once (to avoid blocking Network calls).await
is mostly called on methods returning these three types. There's another optionValueTask
.
await
onvoid
andTask
return functions has not return value, but when used overTask<T>
, it unwraps theTask<T>
to fetch the resultT
, now this value T can be utilized in the function locally and when we return likereturn T
, for a function with return valueTask<T>
, framework would automatically wrap in aTask
, so that it can be further awaited till the entry point, which Framework can awaits Asynchronously
I don't see any issue in what you want to achieve, but there's a discrepancy in your question, let me point that out:
From GetComparator
method, x.GetState()
leads decimal
return value, but then finally your OrderBy
statement is: .OrderBy((x) => x.GetState().Result.TotalPoints)
, which is not feasible, therefore GetState()
shall lead to a type, which has a decimal
property TotalPoints
, Now to the Main question.
Check out the following sample, where I have made few assumptions to compile the code
public async Task<IOrderedEnumerable<State>> SortEntries(List<State> stateEntries)
{
var orderedEnumerable = stateEntries.OrderBy(async y => await GetComparator(y));
return await Task.FromResult(orderedEnumerable);
}
private async Task<decimal> GetComparator(State x)
{
var a = await x.GetState();
return a.TotalPoints;
}
public class State
{
public int Id {get; set;}
public decimal TotalPoints {get;set;}
public async Task<State> GetState()
{
return await Task.FromResult(this);
}
}
Assumptions:
State
class defines the Schema, which containsAsync
methodGetState
, which is used for further logical processing
State
class containsTotalPoints
, which is used for the Ordered Processing
How it Works:
Async
method shall always return aTask
and have at-least 1await
for being truly Asynchronous call, rest of the call can be Synchronous, we normally expect a Network call to be Async / Non blocking
Lambda
can beAsync
, as shown in the code, which Async processing for theOrderBy
call- Wrapping up in
Task.FromResult
is a convenience, which is used often for testing frameworks
SortEntries
entries method needn'tAsync
, until and unlessOrleans
or similar framework needs it. Though ideallyAsync
shall be all the way to the entry point likeMain
method in the Console, which is entry / starting point
Important point related to Async calls, Await keyword:
- They can return
void / Task / Task<T>
, with method signature containingasync
, it can become truly async on usage of keywordawait
at least once (to avoid blocking Network calls).await
is mostly called on methods returning these three types. There's another optionValueTask
.
await
onvoid
andTask
return functions has not return value, but when used overTask<T>
, it unwraps theTask<T>
to fetch the resultT
, now this value T can be utilized in the function locally and when we return likereturn T
, for a function with return valueTask<T>
, framework would automatically wrap in aTask
, so that it can be further awaited till the entry point, which Framework can awaits Asynchronously
edited Nov 19 at 9:23
answered Nov 19 at 5:13


Mrinal Kamboj
8,21821946
8,21821946
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53334202%2forderby-an-list-in-a-task-function-async%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
shFkht58lcJlgmjXrbfKE97hjpkx2ISiGsYga3AvCLjuC7 VJmtz5Cr PatxXH h,jgy