creating DELETE request using nodejs on sql database
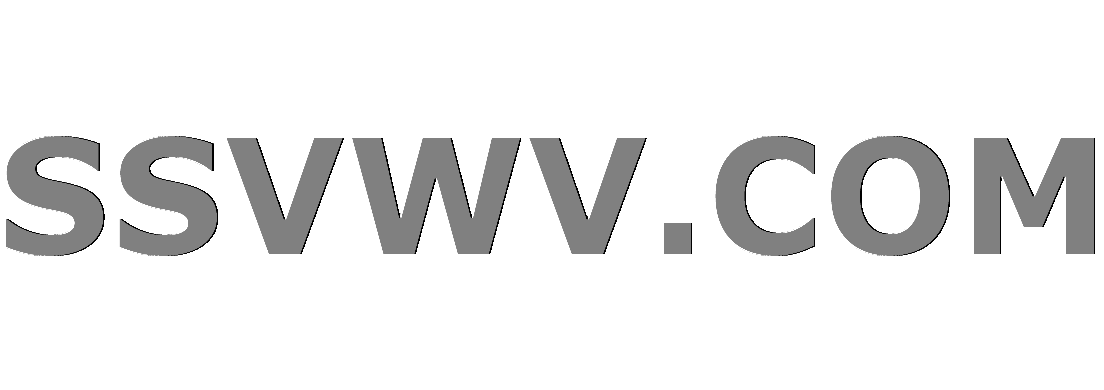
Multi tool use
up vote
2
down vote
favorite
New to node.js
and learning how to use mysql
with node
. I have a form.html
that has two buttons to input a department_no
and a department_name
. I am succecfully able to insert into mysql
database but I have no clue on how to delete a specific dept_no
. What I want to be able to do is enter a dept_no
and the dept_name
and then DELETE
from my database based on the dept_no
. Also I want to be able to check what the user enters to make sure that it is a valid dept_no
and dept_name
. Any ideas on how to get started or what to look into to be able to do this would be extremely helpful. I will paste my node.js
and form.html
down below.
node.js
// DELETE BELOW
app.delete("/deleteEmp/", (req, res) => {
console.log("Department number is " + req.body.department_no);
console.log("Department name is " + req.body.department_name);
const deptNumber = req.body.department_no;
const deptName = req.body.department_name;
const connection = mysql.createConnection({
host: "localhost",
user: "root",
database: "employees"
});
const queryString = "DELETE departments WHERE dept_no = ?";
connection.query(queryString, [department_no], (err, results, fields) => {
if (err) {
console.log("Could not delete" + err);
res.sendStatus(500);
return;
}
console.log("Deleted department_no with dept_name");
res.end();
});
});
form.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>SQLFORM</title>
<style>
#space {
margin-top: 20px;
}
</style>
</head>
<body>
<div>Fill Form Below</div>
<hr />
<div id="space">
<form action="/deleteEmp" method="DELETE">
<input placeholder="Enter Department Number" name="department_no" />
<input placeholder="Enter Department Name" name="department_name" />
<button>Submit</button>
</form>
</div>
</body>
</html>
mysql node.js express
add a comment |
up vote
2
down vote
favorite
New to node.js
and learning how to use mysql
with node
. I have a form.html
that has two buttons to input a department_no
and a department_name
. I am succecfully able to insert into mysql
database but I have no clue on how to delete a specific dept_no
. What I want to be able to do is enter a dept_no
and the dept_name
and then DELETE
from my database based on the dept_no
. Also I want to be able to check what the user enters to make sure that it is a valid dept_no
and dept_name
. Any ideas on how to get started or what to look into to be able to do this would be extremely helpful. I will paste my node.js
and form.html
down below.
node.js
// DELETE BELOW
app.delete("/deleteEmp/", (req, res) => {
console.log("Department number is " + req.body.department_no);
console.log("Department name is " + req.body.department_name);
const deptNumber = req.body.department_no;
const deptName = req.body.department_name;
const connection = mysql.createConnection({
host: "localhost",
user: "root",
database: "employees"
});
const queryString = "DELETE departments WHERE dept_no = ?";
connection.query(queryString, [department_no], (err, results, fields) => {
if (err) {
console.log("Could not delete" + err);
res.sendStatus(500);
return;
}
console.log("Deleted department_no with dept_name");
res.end();
});
});
form.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>SQLFORM</title>
<style>
#space {
margin-top: 20px;
}
</style>
</head>
<body>
<div>Fill Form Below</div>
<hr />
<div id="space">
<form action="/deleteEmp" method="DELETE">
<input placeholder="Enter Department Number" name="department_no" />
<input placeholder="Enter Department Name" name="department_name" />
<button>Submit</button>
</form>
</div>
</body>
</html>
mysql node.js express
Tryconst queryString = "DELETE FROM departments WHERE dept_no = ?";
instead.
– Barns
Nov 18 at 21:38
but how can I use aform
and get the info passed into theform
and delete based on that in mydatabase
– moegizzle
Nov 18 at 21:44
this post doesn't explain how to delete from a mysql database using express though @Barns
– moegizzle
Nov 18 at 22:20
1
You can use sequelizejs (ORM) docs.sequelizejs.com for performing node database operations. It will make easy for you to perform CRUD operations.
– Lalit Dashora
Nov 19 at 9:32
add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
New to node.js
and learning how to use mysql
with node
. I have a form.html
that has two buttons to input a department_no
and a department_name
. I am succecfully able to insert into mysql
database but I have no clue on how to delete a specific dept_no
. What I want to be able to do is enter a dept_no
and the dept_name
and then DELETE
from my database based on the dept_no
. Also I want to be able to check what the user enters to make sure that it is a valid dept_no
and dept_name
. Any ideas on how to get started or what to look into to be able to do this would be extremely helpful. I will paste my node.js
and form.html
down below.
node.js
// DELETE BELOW
app.delete("/deleteEmp/", (req, res) => {
console.log("Department number is " + req.body.department_no);
console.log("Department name is " + req.body.department_name);
const deptNumber = req.body.department_no;
const deptName = req.body.department_name;
const connection = mysql.createConnection({
host: "localhost",
user: "root",
database: "employees"
});
const queryString = "DELETE departments WHERE dept_no = ?";
connection.query(queryString, [department_no], (err, results, fields) => {
if (err) {
console.log("Could not delete" + err);
res.sendStatus(500);
return;
}
console.log("Deleted department_no with dept_name");
res.end();
});
});
form.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>SQLFORM</title>
<style>
#space {
margin-top: 20px;
}
</style>
</head>
<body>
<div>Fill Form Below</div>
<hr />
<div id="space">
<form action="/deleteEmp" method="DELETE">
<input placeholder="Enter Department Number" name="department_no" />
<input placeholder="Enter Department Name" name="department_name" />
<button>Submit</button>
</form>
</div>
</body>
</html>
mysql node.js express
New to node.js
and learning how to use mysql
with node
. I have a form.html
that has two buttons to input a department_no
and a department_name
. I am succecfully able to insert into mysql
database but I have no clue on how to delete a specific dept_no
. What I want to be able to do is enter a dept_no
and the dept_name
and then DELETE
from my database based on the dept_no
. Also I want to be able to check what the user enters to make sure that it is a valid dept_no
and dept_name
. Any ideas on how to get started or what to look into to be able to do this would be extremely helpful. I will paste my node.js
and form.html
down below.
node.js
// DELETE BELOW
app.delete("/deleteEmp/", (req, res) => {
console.log("Department number is " + req.body.department_no);
console.log("Department name is " + req.body.department_name);
const deptNumber = req.body.department_no;
const deptName = req.body.department_name;
const connection = mysql.createConnection({
host: "localhost",
user: "root",
database: "employees"
});
const queryString = "DELETE departments WHERE dept_no = ?";
connection.query(queryString, [department_no], (err, results, fields) => {
if (err) {
console.log("Could not delete" + err);
res.sendStatus(500);
return;
}
console.log("Deleted department_no with dept_name");
res.end();
});
});
form.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>SQLFORM</title>
<style>
#space {
margin-top: 20px;
}
</style>
</head>
<body>
<div>Fill Form Below</div>
<hr />
<div id="space">
<form action="/deleteEmp" method="DELETE">
<input placeholder="Enter Department Number" name="department_no" />
<input placeholder="Enter Department Name" name="department_name" />
<button>Submit</button>
</form>
</div>
</body>
</html>
mysql node.js express
mysql node.js express
asked Nov 18 at 21:19
moegizzle
297
297
Tryconst queryString = "DELETE FROM departments WHERE dept_no = ?";
instead.
– Barns
Nov 18 at 21:38
but how can I use aform
and get the info passed into theform
and delete based on that in mydatabase
– moegizzle
Nov 18 at 21:44
this post doesn't explain how to delete from a mysql database using express though @Barns
– moegizzle
Nov 18 at 22:20
1
You can use sequelizejs (ORM) docs.sequelizejs.com for performing node database operations. It will make easy for you to perform CRUD operations.
– Lalit Dashora
Nov 19 at 9:32
add a comment |
Tryconst queryString = "DELETE FROM departments WHERE dept_no = ?";
instead.
– Barns
Nov 18 at 21:38
but how can I use aform
and get the info passed into theform
and delete based on that in mydatabase
– moegizzle
Nov 18 at 21:44
this post doesn't explain how to delete from a mysql database using express though @Barns
– moegizzle
Nov 18 at 22:20
1
You can use sequelizejs (ORM) docs.sequelizejs.com for performing node database operations. It will make easy for you to perform CRUD operations.
– Lalit Dashora
Nov 19 at 9:32
Try
const queryString = "DELETE FROM departments WHERE dept_no = ?";
instead.– Barns
Nov 18 at 21:38
Try
const queryString = "DELETE FROM departments WHERE dept_no = ?";
instead.– Barns
Nov 18 at 21:38
but how can I use a
form
and get the info passed into the form
and delete based on that in my database
– moegizzle
Nov 18 at 21:44
but how can I use a
form
and get the info passed into the form
and delete based on that in my database
– moegizzle
Nov 18 at 21:44
this post doesn't explain how to delete from a mysql database using express though @Barns
– moegizzle
Nov 18 at 22:20
this post doesn't explain how to delete from a mysql database using express though @Barns
– moegizzle
Nov 18 at 22:20
1
1
You can use sequelizejs (ORM) docs.sequelizejs.com for performing node database operations. It will make easy for you to perform CRUD operations.
– Lalit Dashora
Nov 19 at 9:32
You can use sequelizejs (ORM) docs.sequelizejs.com for performing node database operations. It will make easy for you to perform CRUD operations.
– Lalit Dashora
Nov 19 at 9:32
add a comment |
2 Answers
2
active
oldest
votes
up vote
1
down vote
HTML forms do not support DELETE
requests in general. Node apps can use a work-around like this one - https://github.com/expressjs/method-override
add a comment |
up vote
1
down vote
Another alternative is to perform an AJAX request that doesn't have restrictions on allowed methods. A simplified example:
document.querySelector('#space form').addEventListener('submit', (event) => {
event.preventDefault();
let formData = new FormData();
formData.append('department_no', document.querySelector("input[name='department_no']").value);
formData.append('department_name', document.querySelector("input[name='department_name']").value);
fetch('https://yoururl.com/deleteEmp', {
method: 'DELETE',
headers: {
"Content-type": "application/x-form-urlencoded"
},
body: formData
});
});
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
HTML forms do not support DELETE
requests in general. Node apps can use a work-around like this one - https://github.com/expressjs/method-override
add a comment |
up vote
1
down vote
HTML forms do not support DELETE
requests in general. Node apps can use a work-around like this one - https://github.com/expressjs/method-override
add a comment |
up vote
1
down vote
up vote
1
down vote
HTML forms do not support DELETE
requests in general. Node apps can use a work-around like this one - https://github.com/expressjs/method-override
HTML forms do not support DELETE
requests in general. Node apps can use a work-around like this one - https://github.com/expressjs/method-override
answered Nov 19 at 4:07


Pruthvi Kumar
57718
57718
add a comment |
add a comment |
up vote
1
down vote
Another alternative is to perform an AJAX request that doesn't have restrictions on allowed methods. A simplified example:
document.querySelector('#space form').addEventListener('submit', (event) => {
event.preventDefault();
let formData = new FormData();
formData.append('department_no', document.querySelector("input[name='department_no']").value);
formData.append('department_name', document.querySelector("input[name='department_name']").value);
fetch('https://yoururl.com/deleteEmp', {
method: 'DELETE',
headers: {
"Content-type": "application/x-form-urlencoded"
},
body: formData
});
});
add a comment |
up vote
1
down vote
Another alternative is to perform an AJAX request that doesn't have restrictions on allowed methods. A simplified example:
document.querySelector('#space form').addEventListener('submit', (event) => {
event.preventDefault();
let formData = new FormData();
formData.append('department_no', document.querySelector("input[name='department_no']").value);
formData.append('department_name', document.querySelector("input[name='department_name']").value);
fetch('https://yoururl.com/deleteEmp', {
method: 'DELETE',
headers: {
"Content-type": "application/x-form-urlencoded"
},
body: formData
});
});
add a comment |
up vote
1
down vote
up vote
1
down vote
Another alternative is to perform an AJAX request that doesn't have restrictions on allowed methods. A simplified example:
document.querySelector('#space form').addEventListener('submit', (event) => {
event.preventDefault();
let formData = new FormData();
formData.append('department_no', document.querySelector("input[name='department_no']").value);
formData.append('department_name', document.querySelector("input[name='department_name']").value);
fetch('https://yoururl.com/deleteEmp', {
method: 'DELETE',
headers: {
"Content-type": "application/x-form-urlencoded"
},
body: formData
});
});
Another alternative is to perform an AJAX request that doesn't have restrictions on allowed methods. A simplified example:
document.querySelector('#space form').addEventListener('submit', (event) => {
event.preventDefault();
let formData = new FormData();
formData.append('department_no', document.querySelector("input[name='department_no']").value);
formData.append('department_name', document.querySelector("input[name='department_name']").value);
fetch('https://yoururl.com/deleteEmp', {
method: 'DELETE',
headers: {
"Content-type": "application/x-form-urlencoded"
},
body: formData
});
});
edited Nov 21 at 8:58
answered Nov 19 at 9:29


Anton Pastukhov
1406
1406
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53365580%2fcreating-delete-request-using-nodejs-on-sql-database%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
nyc CLelrss k2R,NxwWryoz9cWWp,Mm 9h,pKvkxRY41I K2R5flSY1N599 AjLpfO1 o16n
Try
const queryString = "DELETE FROM departments WHERE dept_no = ?";
instead.– Barns
Nov 18 at 21:38
but how can I use a
form
and get the info passed into theform
and delete based on that in mydatabase
– moegizzle
Nov 18 at 21:44
this post doesn't explain how to delete from a mysql database using express though @Barns
– moegizzle
Nov 18 at 22:20
1
You can use sequelizejs (ORM) docs.sequelizejs.com for performing node database operations. It will make easy for you to perform CRUD operations.
– Lalit Dashora
Nov 19 at 9:32