If data in a recycler view item is null don't display the view holder
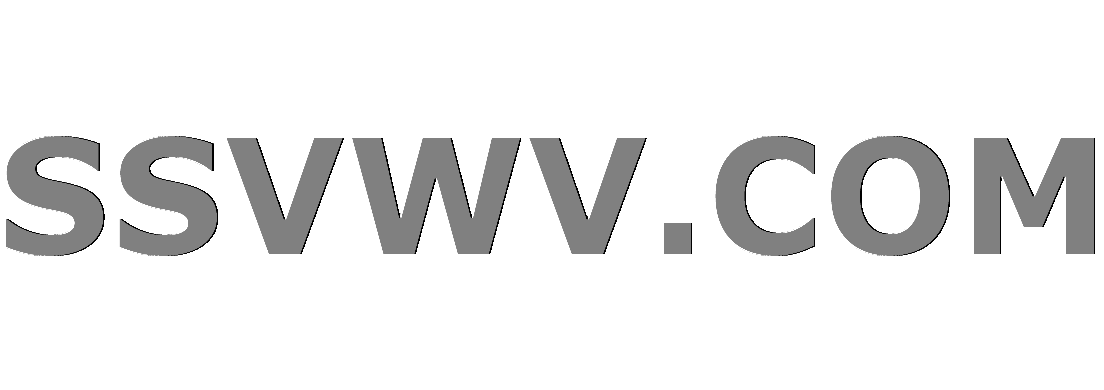
Multi tool use
I want to check if data that comes from the server is null or not. If is not null then populate the recycler view item in my case being a CardView and if it is null don't display the CardView at all.
In my recycler view adapter i have this:
override fun onBindViewHolder(holder: ViewHolder, position: Int) {
var firstName = itemsList[position].firstName
var lastName = itemsList[position].lastName
if (firstName.isNullOrEmpty() && lastName.isNullOrEmpty()) {
//...what shoud i write here ?
} else {
holder.name?.text = firstName + " " + lastName
}
}
class ViewHolder(itemView: View) : RecyclerView.ViewHolder(itemView) {
val name = itemView.name
}

add a comment |
I want to check if data that comes from the server is null or not. If is not null then populate the recycler view item in my case being a CardView and if it is null don't display the CardView at all.
In my recycler view adapter i have this:
override fun onBindViewHolder(holder: ViewHolder, position: Int) {
var firstName = itemsList[position].firstName
var lastName = itemsList[position].lastName
if (firstName.isNullOrEmpty() && lastName.isNullOrEmpty()) {
//...what shoud i write here ?
} else {
holder.name?.text = firstName + " " + lastName
}
}
class ViewHolder(itemView: View) : RecyclerView.ViewHolder(itemView) {
val name = itemView.name
}

I think this should be handled fromgetItemViewType
method ofRecyclerView.Adapter
– Ionut J. Bejan
Nov 20 at 13:27
Can you tell me how exactly can I do that? I am relatively new to this.
– anMC
Nov 20 at 13:33
add a comment |
I want to check if data that comes from the server is null or not. If is not null then populate the recycler view item in my case being a CardView and if it is null don't display the CardView at all.
In my recycler view adapter i have this:
override fun onBindViewHolder(holder: ViewHolder, position: Int) {
var firstName = itemsList[position].firstName
var lastName = itemsList[position].lastName
if (firstName.isNullOrEmpty() && lastName.isNullOrEmpty()) {
//...what shoud i write here ?
} else {
holder.name?.text = firstName + " " + lastName
}
}
class ViewHolder(itemView: View) : RecyclerView.ViewHolder(itemView) {
val name = itemView.name
}

I want to check if data that comes from the server is null or not. If is not null then populate the recycler view item in my case being a CardView and if it is null don't display the CardView at all.
In my recycler view adapter i have this:
override fun onBindViewHolder(holder: ViewHolder, position: Int) {
var firstName = itemsList[position].firstName
var lastName = itemsList[position].lastName
if (firstName.isNullOrEmpty() && lastName.isNullOrEmpty()) {
//...what shoud i write here ?
} else {
holder.name?.text = firstName + " " + lastName
}
}
class ViewHolder(itemView: View) : RecyclerView.ViewHolder(itemView) {
val name = itemView.name
}


asked Nov 20 at 13:24
anMC
256
256
I think this should be handled fromgetItemViewType
method ofRecyclerView.Adapter
– Ionut J. Bejan
Nov 20 at 13:27
Can you tell me how exactly can I do that? I am relatively new to this.
– anMC
Nov 20 at 13:33
add a comment |
I think this should be handled fromgetItemViewType
method ofRecyclerView.Adapter
– Ionut J. Bejan
Nov 20 at 13:27
Can you tell me how exactly can I do that? I am relatively new to this.
– anMC
Nov 20 at 13:33
I think this should be handled from
getItemViewType
method of RecyclerView.Adapter
– Ionut J. Bejan
Nov 20 at 13:27
I think this should be handled from
getItemViewType
method of RecyclerView.Adapter
– Ionut J. Bejan
Nov 20 at 13:27
Can you tell me how exactly can I do that? I am relatively new to this.
– anMC
Nov 20 at 13:33
Can you tell me how exactly can I do that? I am relatively new to this.
– anMC
Nov 20 at 13:33
add a comment |
2 Answers
2
active
oldest
votes
you should do something like this:
override fun onBindViewHolder(holder: ViewHolder, position: Int) {
var firstName = itemsList[position].firstName
var lastName = itemsList[position].lastName
if (firstName.isNullOrEmpty() && lastName.isNullOrEmpty()) {
yourCardView.visibility = View.INVISIBLE //or GONE if you do not want to keep its space
holder.name?.text = ""
} else {
yourCardView.visibility = View.VISIBLE
holder.name?.text = firstName + " " + lastName
}
}
class ViewHolder(itemView: View) : RecyclerView.ViewHolder(itemView) {
val name = itemView.name
}
but if cardview is your root item and you do not want to show the list item at all,you should change your main list with a code like this in your adapter constructor:
filteredList = itemsList?.filter {
(it.firstname.isNullOrEmpty() || it.lastName.isNullOrEmpty()).not()
}
Can I set my cardView visibility in another way? I can't access it in adapter.
– anMC
Nov 26 at 8:20
if cardview is in your list-item you should findViewById it in ViewHolder and access it same as holder.name (for example holder.cardView.visibility) but if cardview is in your activity you should hide it by passing an interface that implemented in your activity or pass it in adapter constructor
– Reza.Abedini
Nov 26 at 8:50
Thank you so much it works now. One more thing though...even if I set the visibility to View.GONE the space of the cardView is still showing in the list.
– anMC
Nov 26 at 8:59
set your item's height wrap_content and add this code in your activity : recyclerView.hasFixedSize = false it should works
– Reza.Abedini
Nov 26 at 9:20
add a comment |
Even if you change the visibility of the CardView
the gap in the RecyclerView
will be there.
It's better to remove the empty items from the list, prior to setting the adapter:
list.removeIf { it.firstName.isNullOrEmpty() && it.lastName.isNullOrEmpty() }
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53394003%2fif-data-in-a-recycler-view-item-is-null-dont-display-the-view-holder%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
you should do something like this:
override fun onBindViewHolder(holder: ViewHolder, position: Int) {
var firstName = itemsList[position].firstName
var lastName = itemsList[position].lastName
if (firstName.isNullOrEmpty() && lastName.isNullOrEmpty()) {
yourCardView.visibility = View.INVISIBLE //or GONE if you do not want to keep its space
holder.name?.text = ""
} else {
yourCardView.visibility = View.VISIBLE
holder.name?.text = firstName + " " + lastName
}
}
class ViewHolder(itemView: View) : RecyclerView.ViewHolder(itemView) {
val name = itemView.name
}
but if cardview is your root item and you do not want to show the list item at all,you should change your main list with a code like this in your adapter constructor:
filteredList = itemsList?.filter {
(it.firstname.isNullOrEmpty() || it.lastName.isNullOrEmpty()).not()
}
Can I set my cardView visibility in another way? I can't access it in adapter.
– anMC
Nov 26 at 8:20
if cardview is in your list-item you should findViewById it in ViewHolder and access it same as holder.name (for example holder.cardView.visibility) but if cardview is in your activity you should hide it by passing an interface that implemented in your activity or pass it in adapter constructor
– Reza.Abedini
Nov 26 at 8:50
Thank you so much it works now. One more thing though...even if I set the visibility to View.GONE the space of the cardView is still showing in the list.
– anMC
Nov 26 at 8:59
set your item's height wrap_content and add this code in your activity : recyclerView.hasFixedSize = false it should works
– Reza.Abedini
Nov 26 at 9:20
add a comment |
you should do something like this:
override fun onBindViewHolder(holder: ViewHolder, position: Int) {
var firstName = itemsList[position].firstName
var lastName = itemsList[position].lastName
if (firstName.isNullOrEmpty() && lastName.isNullOrEmpty()) {
yourCardView.visibility = View.INVISIBLE //or GONE if you do not want to keep its space
holder.name?.text = ""
} else {
yourCardView.visibility = View.VISIBLE
holder.name?.text = firstName + " " + lastName
}
}
class ViewHolder(itemView: View) : RecyclerView.ViewHolder(itemView) {
val name = itemView.name
}
but if cardview is your root item and you do not want to show the list item at all,you should change your main list with a code like this in your adapter constructor:
filteredList = itemsList?.filter {
(it.firstname.isNullOrEmpty() || it.lastName.isNullOrEmpty()).not()
}
Can I set my cardView visibility in another way? I can't access it in adapter.
– anMC
Nov 26 at 8:20
if cardview is in your list-item you should findViewById it in ViewHolder and access it same as holder.name (for example holder.cardView.visibility) but if cardview is in your activity you should hide it by passing an interface that implemented in your activity or pass it in adapter constructor
– Reza.Abedini
Nov 26 at 8:50
Thank you so much it works now. One more thing though...even if I set the visibility to View.GONE the space of the cardView is still showing in the list.
– anMC
Nov 26 at 8:59
set your item's height wrap_content and add this code in your activity : recyclerView.hasFixedSize = false it should works
– Reza.Abedini
Nov 26 at 9:20
add a comment |
you should do something like this:
override fun onBindViewHolder(holder: ViewHolder, position: Int) {
var firstName = itemsList[position].firstName
var lastName = itemsList[position].lastName
if (firstName.isNullOrEmpty() && lastName.isNullOrEmpty()) {
yourCardView.visibility = View.INVISIBLE //or GONE if you do not want to keep its space
holder.name?.text = ""
} else {
yourCardView.visibility = View.VISIBLE
holder.name?.text = firstName + " " + lastName
}
}
class ViewHolder(itemView: View) : RecyclerView.ViewHolder(itemView) {
val name = itemView.name
}
but if cardview is your root item and you do not want to show the list item at all,you should change your main list with a code like this in your adapter constructor:
filteredList = itemsList?.filter {
(it.firstname.isNullOrEmpty() || it.lastName.isNullOrEmpty()).not()
}
you should do something like this:
override fun onBindViewHolder(holder: ViewHolder, position: Int) {
var firstName = itemsList[position].firstName
var lastName = itemsList[position].lastName
if (firstName.isNullOrEmpty() && lastName.isNullOrEmpty()) {
yourCardView.visibility = View.INVISIBLE //or GONE if you do not want to keep its space
holder.name?.text = ""
} else {
yourCardView.visibility = View.VISIBLE
holder.name?.text = firstName + " " + lastName
}
}
class ViewHolder(itemView: View) : RecyclerView.ViewHolder(itemView) {
val name = itemView.name
}
but if cardview is your root item and you do not want to show the list item at all,you should change your main list with a code like this in your adapter constructor:
filteredList = itemsList?.filter {
(it.firstname.isNullOrEmpty() || it.lastName.isNullOrEmpty()).not()
}
edited Nov 20 at 13:40
answered Nov 20 at 13:33


Reza.Abedini
1,009813
1,009813
Can I set my cardView visibility in another way? I can't access it in adapter.
– anMC
Nov 26 at 8:20
if cardview is in your list-item you should findViewById it in ViewHolder and access it same as holder.name (for example holder.cardView.visibility) but if cardview is in your activity you should hide it by passing an interface that implemented in your activity or pass it in adapter constructor
– Reza.Abedini
Nov 26 at 8:50
Thank you so much it works now. One more thing though...even if I set the visibility to View.GONE the space of the cardView is still showing in the list.
– anMC
Nov 26 at 8:59
set your item's height wrap_content and add this code in your activity : recyclerView.hasFixedSize = false it should works
– Reza.Abedini
Nov 26 at 9:20
add a comment |
Can I set my cardView visibility in another way? I can't access it in adapter.
– anMC
Nov 26 at 8:20
if cardview is in your list-item you should findViewById it in ViewHolder and access it same as holder.name (for example holder.cardView.visibility) but if cardview is in your activity you should hide it by passing an interface that implemented in your activity or pass it in adapter constructor
– Reza.Abedini
Nov 26 at 8:50
Thank you so much it works now. One more thing though...even if I set the visibility to View.GONE the space of the cardView is still showing in the list.
– anMC
Nov 26 at 8:59
set your item's height wrap_content and add this code in your activity : recyclerView.hasFixedSize = false it should works
– Reza.Abedini
Nov 26 at 9:20
Can I set my cardView visibility in another way? I can't access it in adapter.
– anMC
Nov 26 at 8:20
Can I set my cardView visibility in another way? I can't access it in adapter.
– anMC
Nov 26 at 8:20
if cardview is in your list-item you should findViewById it in ViewHolder and access it same as holder.name (for example holder.cardView.visibility) but if cardview is in your activity you should hide it by passing an interface that implemented in your activity or pass it in adapter constructor
– Reza.Abedini
Nov 26 at 8:50
if cardview is in your list-item you should findViewById it in ViewHolder and access it same as holder.name (for example holder.cardView.visibility) but if cardview is in your activity you should hide it by passing an interface that implemented in your activity or pass it in adapter constructor
– Reza.Abedini
Nov 26 at 8:50
Thank you so much it works now. One more thing though...even if I set the visibility to View.GONE the space of the cardView is still showing in the list.
– anMC
Nov 26 at 8:59
Thank you so much it works now. One more thing though...even if I set the visibility to View.GONE the space of the cardView is still showing in the list.
– anMC
Nov 26 at 8:59
set your item's height wrap_content and add this code in your activity : recyclerView.hasFixedSize = false it should works
– Reza.Abedini
Nov 26 at 9:20
set your item's height wrap_content and add this code in your activity : recyclerView.hasFixedSize = false it should works
– Reza.Abedini
Nov 26 at 9:20
add a comment |
Even if you change the visibility of the CardView
the gap in the RecyclerView
will be there.
It's better to remove the empty items from the list, prior to setting the adapter:
list.removeIf { it.firstName.isNullOrEmpty() && it.lastName.isNullOrEmpty() }
add a comment |
Even if you change the visibility of the CardView
the gap in the RecyclerView
will be there.
It's better to remove the empty items from the list, prior to setting the adapter:
list.removeIf { it.firstName.isNullOrEmpty() && it.lastName.isNullOrEmpty() }
add a comment |
Even if you change the visibility of the CardView
the gap in the RecyclerView
will be there.
It's better to remove the empty items from the list, prior to setting the adapter:
list.removeIf { it.firstName.isNullOrEmpty() && it.lastName.isNullOrEmpty() }
Even if you change the visibility of the CardView
the gap in the RecyclerView
will be there.
It's better to remove the empty items from the list, prior to setting the adapter:
list.removeIf { it.firstName.isNullOrEmpty() && it.lastName.isNullOrEmpty() }
answered Nov 20 at 13:41
forpas
7,9221419
7,9221419
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53394003%2fif-data-in-a-recycler-view-item-is-null-dont-display-the-view-holder%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Tve,LeZ7c8rpcsy,K,OPXDVc0qTi0Ez,Zq8 68,w,xo6E eum xl89wRm95ewwC d4Uzh0F8,dCGXkYgt 0w,l48Le 35,I
I think this should be handled from
getItemViewType
method ofRecyclerView.Adapter
– Ionut J. Bejan
Nov 20 at 13:27
Can you tell me how exactly can I do that? I am relatively new to this.
– anMC
Nov 20 at 13:33