delay with settimeout and for loop
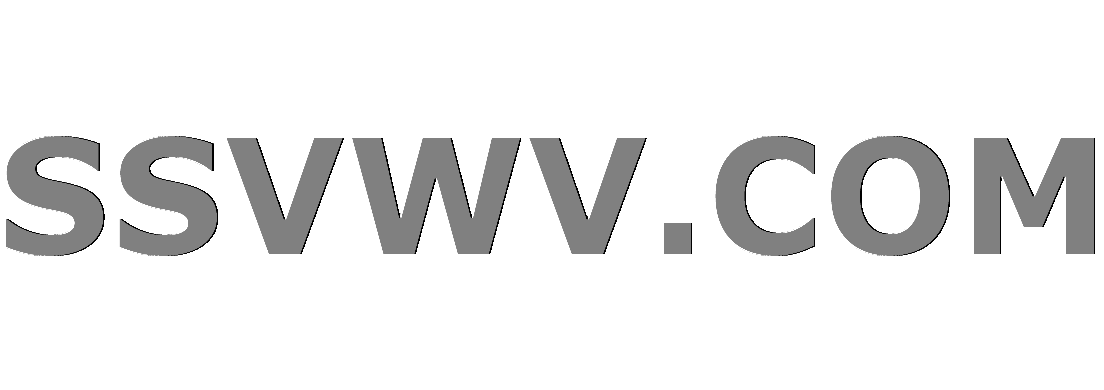
Multi tool use
I have this code from here:
How do I add a delay in a JavaScript loop?
I use it in console in IE, and after this code I call the function with myFunction() in console to run; This first code runs perfectly, it clicks on the second "something" tagnamed element 10 times and between the clicks are 3000 ms delay.
function myFunction() {
(function myLoop (i) {
setTimeout(function () {
document.getElementsByTagName("something")[1].click();
if (--i) myLoop(i); // decrement i and call myLoop again if i > 0
}, 3000)
})(10);
}
I would like to change the number "1" in this code with foor loop, so I want create a code which clicks on elements named "something".
I created this code, but is not working:
for (x=1;x<10;x++){
function myFunction() {
(function myLoop (i) {
setTimeout(function () {
document.getElementsByTagName("something")[1].click();
if (--i) myLoop(i); // decrement i and call myLoop again if i > 0
}, 3000)
})(10);
}
}
javascript loops click delay element
add a comment |
I have this code from here:
How do I add a delay in a JavaScript loop?
I use it in console in IE, and after this code I call the function with myFunction() in console to run; This first code runs perfectly, it clicks on the second "something" tagnamed element 10 times and between the clicks are 3000 ms delay.
function myFunction() {
(function myLoop (i) {
setTimeout(function () {
document.getElementsByTagName("something")[1].click();
if (--i) myLoop(i); // decrement i and call myLoop again if i > 0
}, 3000)
})(10);
}
I would like to change the number "1" in this code with foor loop, so I want create a code which clicks on elements named "something".
I created this code, but is not working:
for (x=1;x<10;x++){
function myFunction() {
(function myLoop (i) {
setTimeout(function () {
document.getElementsByTagName("something")[1].click();
if (--i) myLoop(i); // decrement i and call myLoop again if i > 0
}, 3000)
})(10);
}
}
javascript loops click delay element
add a comment |
I have this code from here:
How do I add a delay in a JavaScript loop?
I use it in console in IE, and after this code I call the function with myFunction() in console to run; This first code runs perfectly, it clicks on the second "something" tagnamed element 10 times and between the clicks are 3000 ms delay.
function myFunction() {
(function myLoop (i) {
setTimeout(function () {
document.getElementsByTagName("something")[1].click();
if (--i) myLoop(i); // decrement i and call myLoop again if i > 0
}, 3000)
})(10);
}
I would like to change the number "1" in this code with foor loop, so I want create a code which clicks on elements named "something".
I created this code, but is not working:
for (x=1;x<10;x++){
function myFunction() {
(function myLoop (i) {
setTimeout(function () {
document.getElementsByTagName("something")[1].click();
if (--i) myLoop(i); // decrement i and call myLoop again if i > 0
}, 3000)
})(10);
}
}
javascript loops click delay element
I have this code from here:
How do I add a delay in a JavaScript loop?
I use it in console in IE, and after this code I call the function with myFunction() in console to run; This first code runs perfectly, it clicks on the second "something" tagnamed element 10 times and between the clicks are 3000 ms delay.
function myFunction() {
(function myLoop (i) {
setTimeout(function () {
document.getElementsByTagName("something")[1].click();
if (--i) myLoop(i); // decrement i and call myLoop again if i > 0
}, 3000)
})(10);
}
I would like to change the number "1" in this code with foor loop, so I want create a code which clicks on elements named "something".
I created this code, but is not working:
for (x=1;x<10;x++){
function myFunction() {
(function myLoop (i) {
setTimeout(function () {
document.getElementsByTagName("something")[1].click();
if (--i) myLoop(i); // decrement i and call myLoop again if i > 0
}, 3000)
})(10);
}
}
javascript loops click delay element
javascript loops click delay element
edited Nov 21 '18 at 8:26
johndoel
asked Nov 21 '18 at 8:18
johndoeljohndoel
406
406
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
If you want to print each element at an interval you need to multiply the timing value with an integer, otherwise all of them will be logged at one time.
Also you may not need to create myFunction
inside the loop
for (var x = 1; x < 5; x++) {
(function(i) {
setTimeout(function() {
console.log(i);
}, i * 1000)
}(x))
}
add a comment |
It usually easier to use setInterval
rather the a loop with setTimeout
. Everything is just simpler:
var count = 10
var intv = setInterval(function(){
if (count === 0 ) {
clearInterval(intv)
console.log("done")
return
}
// do something
console.log(count--)
}, 1000)
But you can recursively call setTimeout
:
(function myLoop (i) {
setTimeout(function () {
console.log("loop: ", i)
if (--i) myLoop(i);
}, 1000)
})(10);
Putting the whole thing in a for loop AND calling it recursively is strange though, because the loop will run and make a bunch of individual timeouts that will all run independently, which I don't think is what you want.
add a comment |
function myFunction() {
(function myLoop (i) {
setTimeout(function () {
document.getElementsByTagName("div")[1].click();
if (--i) myLoop(i); // decrement i and call myLoop again if i > 0
}, 3000)
})(10);
}
for (x=1;x<10;x++){
myFunction();
}
<div>1</div>
Try like this. I used div
instead of something
This code also clicks on the second div element, I want loop through the div elements-> (div)[x]
– johndoel
Nov 21 '18 at 8:32
I tried your code with some changes: i pass the argument x in the foor loop to the myFunction, so the function definition will be function myFunction(x){} and inside the for loop is myFunction(x); but this also doesnt work, because now clicks on the last element in the foor loop only, for example if i define the for loop as for(x=1;x<5;x++) then only the 4. elemnt will be clicked.
– johndoel
Nov 21 '18 at 8:35
Did it work then?
– Dananjaya Ariyasena
Nov 21 '18 at 8:37
brk has right, it is needed to multiply the timing value with an integer.
– johndoel
Nov 21 '18 at 8:57
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53407769%2fdelay-with-settimeout-and-for-loop%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
If you want to print each element at an interval you need to multiply the timing value with an integer, otherwise all of them will be logged at one time.
Also you may not need to create myFunction
inside the loop
for (var x = 1; x < 5; x++) {
(function(i) {
setTimeout(function() {
console.log(i);
}, i * 1000)
}(x))
}
add a comment |
If you want to print each element at an interval you need to multiply the timing value with an integer, otherwise all of them will be logged at one time.
Also you may not need to create myFunction
inside the loop
for (var x = 1; x < 5; x++) {
(function(i) {
setTimeout(function() {
console.log(i);
}, i * 1000)
}(x))
}
add a comment |
If you want to print each element at an interval you need to multiply the timing value with an integer, otherwise all of them will be logged at one time.
Also you may not need to create myFunction
inside the loop
for (var x = 1; x < 5; x++) {
(function(i) {
setTimeout(function() {
console.log(i);
}, i * 1000)
}(x))
}
If you want to print each element at an interval you need to multiply the timing value with an integer, otherwise all of them will be logged at one time.
Also you may not need to create myFunction
inside the loop
for (var x = 1; x < 5; x++) {
(function(i) {
setTimeout(function() {
console.log(i);
}, i * 1000)
}(x))
}
for (var x = 1; x < 5; x++) {
(function(i) {
setTimeout(function() {
console.log(i);
}, i * 1000)
}(x))
}
for (var x = 1; x < 5; x++) {
(function(i) {
setTimeout(function() {
console.log(i);
}, i * 1000)
}(x))
}
answered Nov 21 '18 at 8:27
brkbrk
25.6k31940
25.6k31940
add a comment |
add a comment |
It usually easier to use setInterval
rather the a loop with setTimeout
. Everything is just simpler:
var count = 10
var intv = setInterval(function(){
if (count === 0 ) {
clearInterval(intv)
console.log("done")
return
}
// do something
console.log(count--)
}, 1000)
But you can recursively call setTimeout
:
(function myLoop (i) {
setTimeout(function () {
console.log("loop: ", i)
if (--i) myLoop(i);
}, 1000)
})(10);
Putting the whole thing in a for loop AND calling it recursively is strange though, because the loop will run and make a bunch of individual timeouts that will all run independently, which I don't think is what you want.
add a comment |
It usually easier to use setInterval
rather the a loop with setTimeout
. Everything is just simpler:
var count = 10
var intv = setInterval(function(){
if (count === 0 ) {
clearInterval(intv)
console.log("done")
return
}
// do something
console.log(count--)
}, 1000)
But you can recursively call setTimeout
:
(function myLoop (i) {
setTimeout(function () {
console.log("loop: ", i)
if (--i) myLoop(i);
}, 1000)
})(10);
Putting the whole thing in a for loop AND calling it recursively is strange though, because the loop will run and make a bunch of individual timeouts that will all run independently, which I don't think is what you want.
add a comment |
It usually easier to use setInterval
rather the a loop with setTimeout
. Everything is just simpler:
var count = 10
var intv = setInterval(function(){
if (count === 0 ) {
clearInterval(intv)
console.log("done")
return
}
// do something
console.log(count--)
}, 1000)
But you can recursively call setTimeout
:
(function myLoop (i) {
setTimeout(function () {
console.log("loop: ", i)
if (--i) myLoop(i);
}, 1000)
})(10);
Putting the whole thing in a for loop AND calling it recursively is strange though, because the loop will run and make a bunch of individual timeouts that will all run independently, which I don't think is what you want.
It usually easier to use setInterval
rather the a loop with setTimeout
. Everything is just simpler:
var count = 10
var intv = setInterval(function(){
if (count === 0 ) {
clearInterval(intv)
console.log("done")
return
}
// do something
console.log(count--)
}, 1000)
But you can recursively call setTimeout
:
(function myLoop (i) {
setTimeout(function () {
console.log("loop: ", i)
if (--i) myLoop(i);
}, 1000)
})(10);
Putting the whole thing in a for loop AND calling it recursively is strange though, because the loop will run and make a bunch of individual timeouts that will all run independently, which I don't think is what you want.
var count = 10
var intv = setInterval(function(){
if (count === 0 ) {
clearInterval(intv)
console.log("done")
return
}
// do something
console.log(count--)
}, 1000)
var count = 10
var intv = setInterval(function(){
if (count === 0 ) {
clearInterval(intv)
console.log("done")
return
}
// do something
console.log(count--)
}, 1000)
(function myLoop (i) {
setTimeout(function () {
console.log("loop: ", i)
if (--i) myLoop(i);
}, 1000)
})(10);
(function myLoop (i) {
setTimeout(function () {
console.log("loop: ", i)
if (--i) myLoop(i);
}, 1000)
})(10);
answered Nov 21 '18 at 8:29


Mark MeyerMark Meyer
37.2k33059
37.2k33059
add a comment |
add a comment |
function myFunction() {
(function myLoop (i) {
setTimeout(function () {
document.getElementsByTagName("div")[1].click();
if (--i) myLoop(i); // decrement i and call myLoop again if i > 0
}, 3000)
})(10);
}
for (x=1;x<10;x++){
myFunction();
}
<div>1</div>
Try like this. I used div
instead of something
This code also clicks on the second div element, I want loop through the div elements-> (div)[x]
– johndoel
Nov 21 '18 at 8:32
I tried your code with some changes: i pass the argument x in the foor loop to the myFunction, so the function definition will be function myFunction(x){} and inside the for loop is myFunction(x); but this also doesnt work, because now clicks on the last element in the foor loop only, for example if i define the for loop as for(x=1;x<5;x++) then only the 4. elemnt will be clicked.
– johndoel
Nov 21 '18 at 8:35
Did it work then?
– Dananjaya Ariyasena
Nov 21 '18 at 8:37
brk has right, it is needed to multiply the timing value with an integer.
– johndoel
Nov 21 '18 at 8:57
add a comment |
function myFunction() {
(function myLoop (i) {
setTimeout(function () {
document.getElementsByTagName("div")[1].click();
if (--i) myLoop(i); // decrement i and call myLoop again if i > 0
}, 3000)
})(10);
}
for (x=1;x<10;x++){
myFunction();
}
<div>1</div>
Try like this. I used div
instead of something
This code also clicks on the second div element, I want loop through the div elements-> (div)[x]
– johndoel
Nov 21 '18 at 8:32
I tried your code with some changes: i pass the argument x in the foor loop to the myFunction, so the function definition will be function myFunction(x){} and inside the for loop is myFunction(x); but this also doesnt work, because now clicks on the last element in the foor loop only, for example if i define the for loop as for(x=1;x<5;x++) then only the 4. elemnt will be clicked.
– johndoel
Nov 21 '18 at 8:35
Did it work then?
– Dananjaya Ariyasena
Nov 21 '18 at 8:37
brk has right, it is needed to multiply the timing value with an integer.
– johndoel
Nov 21 '18 at 8:57
add a comment |
function myFunction() {
(function myLoop (i) {
setTimeout(function () {
document.getElementsByTagName("div")[1].click();
if (--i) myLoop(i); // decrement i and call myLoop again if i > 0
}, 3000)
})(10);
}
for (x=1;x<10;x++){
myFunction();
}
<div>1</div>
Try like this. I used div
instead of something
function myFunction() {
(function myLoop (i) {
setTimeout(function () {
document.getElementsByTagName("div")[1].click();
if (--i) myLoop(i); // decrement i and call myLoop again if i > 0
}, 3000)
})(10);
}
for (x=1;x<10;x++){
myFunction();
}
<div>1</div>
Try like this. I used div
instead of something
function myFunction() {
(function myLoop (i) {
setTimeout(function () {
document.getElementsByTagName("div")[1].click();
if (--i) myLoop(i); // decrement i and call myLoop again if i > 0
}, 3000)
})(10);
}
for (x=1;x<10;x++){
myFunction();
}
<div>1</div>
function myFunction() {
(function myLoop (i) {
setTimeout(function () {
document.getElementsByTagName("div")[1].click();
if (--i) myLoop(i); // decrement i and call myLoop again if i > 0
}, 3000)
})(10);
}
for (x=1;x<10;x++){
myFunction();
}
<div>1</div>
answered Nov 21 '18 at 8:25


Dananjaya AriyasenaDananjaya Ariyasena
41010
41010
This code also clicks on the second div element, I want loop through the div elements-> (div)[x]
– johndoel
Nov 21 '18 at 8:32
I tried your code with some changes: i pass the argument x in the foor loop to the myFunction, so the function definition will be function myFunction(x){} and inside the for loop is myFunction(x); but this also doesnt work, because now clicks on the last element in the foor loop only, for example if i define the for loop as for(x=1;x<5;x++) then only the 4. elemnt will be clicked.
– johndoel
Nov 21 '18 at 8:35
Did it work then?
– Dananjaya Ariyasena
Nov 21 '18 at 8:37
brk has right, it is needed to multiply the timing value with an integer.
– johndoel
Nov 21 '18 at 8:57
add a comment |
This code also clicks on the second div element, I want loop through the div elements-> (div)[x]
– johndoel
Nov 21 '18 at 8:32
I tried your code with some changes: i pass the argument x in the foor loop to the myFunction, so the function definition will be function myFunction(x){} and inside the for loop is myFunction(x); but this also doesnt work, because now clicks on the last element in the foor loop only, for example if i define the for loop as for(x=1;x<5;x++) then only the 4. elemnt will be clicked.
– johndoel
Nov 21 '18 at 8:35
Did it work then?
– Dananjaya Ariyasena
Nov 21 '18 at 8:37
brk has right, it is needed to multiply the timing value with an integer.
– johndoel
Nov 21 '18 at 8:57
This code also clicks on the second div element, I want loop through the div elements-> (div)[x]
– johndoel
Nov 21 '18 at 8:32
This code also clicks on the second div element, I want loop through the div elements-> (div)[x]
– johndoel
Nov 21 '18 at 8:32
I tried your code with some changes: i pass the argument x in the foor loop to the myFunction, so the function definition will be function myFunction(x){} and inside the for loop is myFunction(x); but this also doesnt work, because now clicks on the last element in the foor loop only, for example if i define the for loop as for(x=1;x<5;x++) then only the 4. elemnt will be clicked.
– johndoel
Nov 21 '18 at 8:35
I tried your code with some changes: i pass the argument x in the foor loop to the myFunction, so the function definition will be function myFunction(x){} and inside the for loop is myFunction(x); but this also doesnt work, because now clicks on the last element in the foor loop only, for example if i define the for loop as for(x=1;x<5;x++) then only the 4. elemnt will be clicked.
– johndoel
Nov 21 '18 at 8:35
Did it work then?
– Dananjaya Ariyasena
Nov 21 '18 at 8:37
Did it work then?
– Dananjaya Ariyasena
Nov 21 '18 at 8:37
brk has right, it is needed to multiply the timing value with an integer.
– johndoel
Nov 21 '18 at 8:57
brk has right, it is needed to multiply the timing value with an integer.
– johndoel
Nov 21 '18 at 8:57
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53407769%2fdelay-with-settimeout-and-for-loop%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
eFNrDw3nE,qFaN8zt1NPT1GU8hrtA0dm m,LvtFnMatj,tsZqov5e,s AG JqylZHZ5CbRbTdhOP