get exit code always returns 1
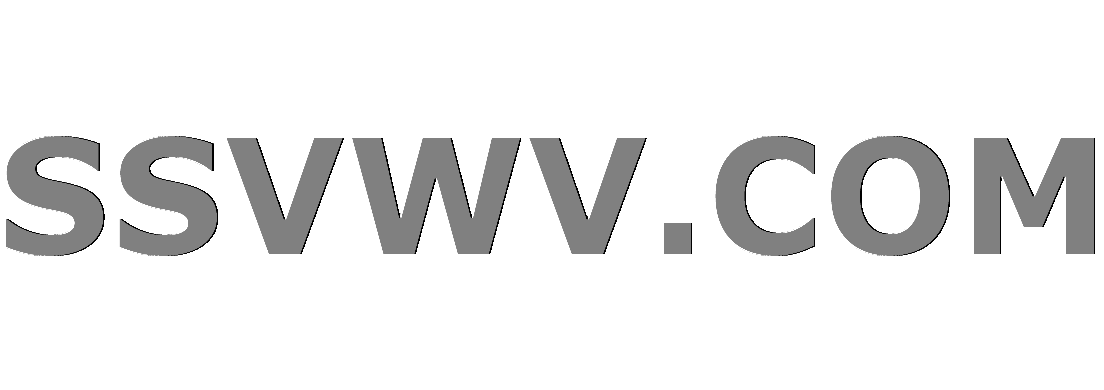
Multi tool use
I am working on a project that includes threads and processes. I open multithreads and for each thread I open a proess that runs an exe file.
In case that a program is finished with a returned value that is not 0 it is crashed, and the returned value is supposed to be printed. in order to get the returned value I use the WIN API function GetExitCodeProcess()
my problem is that this function always return the value 1, even when the program supposed to be crashed, but why?
here is my relevent code:
static DWORD WINAPI RunningTests(test_s *test)
{
PROCESS_INFORMATION procinfo;
DWORD waitcode;
DWORD exitcode;
int status = 0, crashed = 0;
char cmdLineString[MAX_NUMBER_OF_CHARS_IN_CMD_LINE]="";
char *cmdLineStringPtr = cmdLineString;
(test)->isCrashed = 0;
CreateCmdLine((*test).testExePath, &cmdLineStringPtr);
status = CreateProcessSimple(_T(cmdLineString), &procinfo);
if (status == -1)
{
return 1;
}
waitcode = WaitForSingleObject(procinfo.hProcess,
TIME_UNTIL_TIMED_OUT_IN_MILLISEC);
if (waitcode == WAIT_TIMEOUT) /* Process is still alive */
{
strcpy((*test).status, "Timed Out");
CloseHandle(procinfo.hProcess);
CloseHandle(procinfo.hThread);
return 0;
}
crashed = GetExitCodeProcess(procinfo.hProcess, &exitcode);
if (crashed == 0) /* Process is crashed */
{
strcpy((*test).status, "Crashed");
(*test).isCrashed = 1;
(*test).returnedCrashedValue = exitcode;
CloseHandle(procinfo.hProcess);
CloseHandle(procinfo.hThread);
return 0;
}
CloseHandle(procinfo.hProcess);
CloseHandle(procinfo.hThread);
return CompareFiles(&test);
}
c windows exit-code
add a comment |
I am working on a project that includes threads and processes. I open multithreads and for each thread I open a proess that runs an exe file.
In case that a program is finished with a returned value that is not 0 it is crashed, and the returned value is supposed to be printed. in order to get the returned value I use the WIN API function GetExitCodeProcess()
my problem is that this function always return the value 1, even when the program supposed to be crashed, but why?
here is my relevent code:
static DWORD WINAPI RunningTests(test_s *test)
{
PROCESS_INFORMATION procinfo;
DWORD waitcode;
DWORD exitcode;
int status = 0, crashed = 0;
char cmdLineString[MAX_NUMBER_OF_CHARS_IN_CMD_LINE]="";
char *cmdLineStringPtr = cmdLineString;
(test)->isCrashed = 0;
CreateCmdLine((*test).testExePath, &cmdLineStringPtr);
status = CreateProcessSimple(_T(cmdLineString), &procinfo);
if (status == -1)
{
return 1;
}
waitcode = WaitForSingleObject(procinfo.hProcess,
TIME_UNTIL_TIMED_OUT_IN_MILLISEC);
if (waitcode == WAIT_TIMEOUT) /* Process is still alive */
{
strcpy((*test).status, "Timed Out");
CloseHandle(procinfo.hProcess);
CloseHandle(procinfo.hThread);
return 0;
}
crashed = GetExitCodeProcess(procinfo.hProcess, &exitcode);
if (crashed == 0) /* Process is crashed */
{
strcpy((*test).status, "Crashed");
(*test).isCrashed = 1;
(*test).returnedCrashedValue = exitcode;
CloseHandle(procinfo.hProcess);
CloseHandle(procinfo.hThread);
return 0;
}
CloseHandle(procinfo.hProcess);
CloseHandle(procinfo.hThread);
return CompareFiles(&test);
}
c windows exit-code
3
From MSDN: If the function succeeds, the return value is nonzero. If the function fails, the return value is zero. To get extended error information, call GetLastError.
– Johnny Mopp
Nov 20 '18 at 20:46
howGetExitCodeProcess
success or failure related to process crash ?! if you passed to api valid process handle withPROCESS_QUERY_INFORMATION
orPROCESS_QUERY_LIMITED_INFORMATION
access - theGetExitCodeProcess
will return true. becauseprocinfo.hProcess
always valid and have required access - theGetExitCodeProcess
and must always return true
– RbMm
Nov 20 '18 at 20:50
and look forexitcode
exit sense only ifGetExitCodeProcess
return true. but you do opposite - look it on failure, what is wrong. and you close handles from 3 places, instead of single
– RbMm
Nov 20 '18 at 20:54
add a comment |
I am working on a project that includes threads and processes. I open multithreads and for each thread I open a proess that runs an exe file.
In case that a program is finished with a returned value that is not 0 it is crashed, and the returned value is supposed to be printed. in order to get the returned value I use the WIN API function GetExitCodeProcess()
my problem is that this function always return the value 1, even when the program supposed to be crashed, but why?
here is my relevent code:
static DWORD WINAPI RunningTests(test_s *test)
{
PROCESS_INFORMATION procinfo;
DWORD waitcode;
DWORD exitcode;
int status = 0, crashed = 0;
char cmdLineString[MAX_NUMBER_OF_CHARS_IN_CMD_LINE]="";
char *cmdLineStringPtr = cmdLineString;
(test)->isCrashed = 0;
CreateCmdLine((*test).testExePath, &cmdLineStringPtr);
status = CreateProcessSimple(_T(cmdLineString), &procinfo);
if (status == -1)
{
return 1;
}
waitcode = WaitForSingleObject(procinfo.hProcess,
TIME_UNTIL_TIMED_OUT_IN_MILLISEC);
if (waitcode == WAIT_TIMEOUT) /* Process is still alive */
{
strcpy((*test).status, "Timed Out");
CloseHandle(procinfo.hProcess);
CloseHandle(procinfo.hThread);
return 0;
}
crashed = GetExitCodeProcess(procinfo.hProcess, &exitcode);
if (crashed == 0) /* Process is crashed */
{
strcpy((*test).status, "Crashed");
(*test).isCrashed = 1;
(*test).returnedCrashedValue = exitcode;
CloseHandle(procinfo.hProcess);
CloseHandle(procinfo.hThread);
return 0;
}
CloseHandle(procinfo.hProcess);
CloseHandle(procinfo.hThread);
return CompareFiles(&test);
}
c windows exit-code
I am working on a project that includes threads and processes. I open multithreads and for each thread I open a proess that runs an exe file.
In case that a program is finished with a returned value that is not 0 it is crashed, and the returned value is supposed to be printed. in order to get the returned value I use the WIN API function GetExitCodeProcess()
my problem is that this function always return the value 1, even when the program supposed to be crashed, but why?
here is my relevent code:
static DWORD WINAPI RunningTests(test_s *test)
{
PROCESS_INFORMATION procinfo;
DWORD waitcode;
DWORD exitcode;
int status = 0, crashed = 0;
char cmdLineString[MAX_NUMBER_OF_CHARS_IN_CMD_LINE]="";
char *cmdLineStringPtr = cmdLineString;
(test)->isCrashed = 0;
CreateCmdLine((*test).testExePath, &cmdLineStringPtr);
status = CreateProcessSimple(_T(cmdLineString), &procinfo);
if (status == -1)
{
return 1;
}
waitcode = WaitForSingleObject(procinfo.hProcess,
TIME_UNTIL_TIMED_OUT_IN_MILLISEC);
if (waitcode == WAIT_TIMEOUT) /* Process is still alive */
{
strcpy((*test).status, "Timed Out");
CloseHandle(procinfo.hProcess);
CloseHandle(procinfo.hThread);
return 0;
}
crashed = GetExitCodeProcess(procinfo.hProcess, &exitcode);
if (crashed == 0) /* Process is crashed */
{
strcpy((*test).status, "Crashed");
(*test).isCrashed = 1;
(*test).returnedCrashedValue = exitcode;
CloseHandle(procinfo.hProcess);
CloseHandle(procinfo.hThread);
return 0;
}
CloseHandle(procinfo.hProcess);
CloseHandle(procinfo.hThread);
return CompareFiles(&test);
}
c windows exit-code
c windows exit-code
asked Nov 20 '18 at 20:40
shaked
273
273
3
From MSDN: If the function succeeds, the return value is nonzero. If the function fails, the return value is zero. To get extended error information, call GetLastError.
– Johnny Mopp
Nov 20 '18 at 20:46
howGetExitCodeProcess
success or failure related to process crash ?! if you passed to api valid process handle withPROCESS_QUERY_INFORMATION
orPROCESS_QUERY_LIMITED_INFORMATION
access - theGetExitCodeProcess
will return true. becauseprocinfo.hProcess
always valid and have required access - theGetExitCodeProcess
and must always return true
– RbMm
Nov 20 '18 at 20:50
and look forexitcode
exit sense only ifGetExitCodeProcess
return true. but you do opposite - look it on failure, what is wrong. and you close handles from 3 places, instead of single
– RbMm
Nov 20 '18 at 20:54
add a comment |
3
From MSDN: If the function succeeds, the return value is nonzero. If the function fails, the return value is zero. To get extended error information, call GetLastError.
– Johnny Mopp
Nov 20 '18 at 20:46
howGetExitCodeProcess
success or failure related to process crash ?! if you passed to api valid process handle withPROCESS_QUERY_INFORMATION
orPROCESS_QUERY_LIMITED_INFORMATION
access - theGetExitCodeProcess
will return true. becauseprocinfo.hProcess
always valid and have required access - theGetExitCodeProcess
and must always return true
– RbMm
Nov 20 '18 at 20:50
and look forexitcode
exit sense only ifGetExitCodeProcess
return true. but you do opposite - look it on failure, what is wrong. and you close handles from 3 places, instead of single
– RbMm
Nov 20 '18 at 20:54
3
3
From MSDN: If the function succeeds, the return value is nonzero. If the function fails, the return value is zero. To get extended error information, call GetLastError.
– Johnny Mopp
Nov 20 '18 at 20:46
From MSDN: If the function succeeds, the return value is nonzero. If the function fails, the return value is zero. To get extended error information, call GetLastError.
– Johnny Mopp
Nov 20 '18 at 20:46
how
GetExitCodeProcess
success or failure related to process crash ?! if you passed to api valid process handle with PROCESS_QUERY_INFORMATION
or PROCESS_QUERY_LIMITED_INFORMATION
access - the GetExitCodeProcess
will return true. because procinfo.hProcess
always valid and have required access - the GetExitCodeProcess
and must always return true– RbMm
Nov 20 '18 at 20:50
how
GetExitCodeProcess
success or failure related to process crash ?! if you passed to api valid process handle with PROCESS_QUERY_INFORMATION
or PROCESS_QUERY_LIMITED_INFORMATION
access - the GetExitCodeProcess
will return true. because procinfo.hProcess
always valid and have required access - the GetExitCodeProcess
and must always return true– RbMm
Nov 20 '18 at 20:50
and look for
exitcode
exit sense only if GetExitCodeProcess
return true. but you do opposite - look it on failure, what is wrong. and you close handles from 3 places, instead of single– RbMm
Nov 20 '18 at 20:54
and look for
exitcode
exit sense only if GetExitCodeProcess
return true. but you do opposite - look it on failure, what is wrong. and you close handles from 3 places, instead of single– RbMm
Nov 20 '18 at 20:54
add a comment |
1 Answer
1
active
oldest
votes
You should be looking at exitcode, not crashed;
Return Value
If the function succeeds, the return value is nonzero.
If the function fails, the return value is zero. To get extended error
information, call GetLastError.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53401165%2fget-exit-code-always-returns-1%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You should be looking at exitcode, not crashed;
Return Value
If the function succeeds, the return value is nonzero.
If the function fails, the return value is zero. To get extended error
information, call GetLastError.
add a comment |
You should be looking at exitcode, not crashed;
Return Value
If the function succeeds, the return value is nonzero.
If the function fails, the return value is zero. To get extended error
information, call GetLastError.
add a comment |
You should be looking at exitcode, not crashed;
Return Value
If the function succeeds, the return value is nonzero.
If the function fails, the return value is zero. To get extended error
information, call GetLastError.
You should be looking at exitcode, not crashed;
Return Value
If the function succeeds, the return value is nonzero.
If the function fails, the return value is zero. To get extended error
information, call GetLastError.
answered Nov 20 '18 at 20:47


cleblanc
3,4161913
3,4161913
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53401165%2fget-exit-code-always-returns-1%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
MsSj8BBsjYbE
3
From MSDN: If the function succeeds, the return value is nonzero. If the function fails, the return value is zero. To get extended error information, call GetLastError.
– Johnny Mopp
Nov 20 '18 at 20:46
how
GetExitCodeProcess
success or failure related to process crash ?! if you passed to api valid process handle withPROCESS_QUERY_INFORMATION
orPROCESS_QUERY_LIMITED_INFORMATION
access - theGetExitCodeProcess
will return true. becauseprocinfo.hProcess
always valid and have required access - theGetExitCodeProcess
and must always return true– RbMm
Nov 20 '18 at 20:50
and look for
exitcode
exit sense only ifGetExitCodeProcess
return true. but you do opposite - look it on failure, what is wrong. and you close handles from 3 places, instead of single– RbMm
Nov 20 '18 at 20:54