Mutate using Dply depeding upon the condition
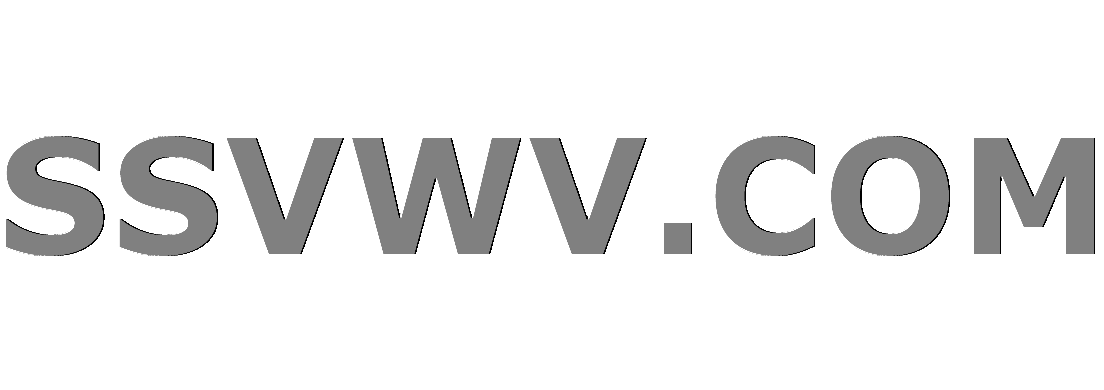
Multi tool use
I have create marks of 15 students for 5 subjects. I have also create a vector of passmarks in each subject. I want to mutate each column of marks with Pass or Fail depending upon the values given in the vector passmarks... I have been able to manually, but I want to use some looping function so that it maps the passmarks to columns and then mutates creating a new column or new dataframe with Pass or Fail.
create a sample vector with 15 positions
x <- runif(15)
#convert to DF
marks <- as.data.frame(x)
marks[1:5] <- sapply(1:5, "+", rnorm(5,60,15))
names(marks) <- paste0("sub", 1:5)
marks
colMeans(marks)
lapply(marks, range)
lapply(marks, is.na)
colSums(is.na(marks))
marks1 = round(marks,2)
passmarks = c(60, 65, 62,70, 45)
names(marks1)
marks1 %>% mutate(sub1a = ifelse(sub1 <= passmarks[1], 'F','P'), sub2a = ifelse(sub2 <= passmarks[2], 'F','P'), sub3a = ifelse(sub3 <= passmarks[3], 'F','P'), sub4a = ifelse(sub4 <= passmarks[4], 'F','P'), sub5a = ifelse(sub5 <= passmarks[5], 'F','P'))
marks1 %>% summarise_at(vars(sub1:sub5), mean, na.rm=T)
marks1 %>% mutate_all(funs(./75))
Part-II of the problem::::
This stage of problem was resolved. I have to calculate SGPA for each student. If I have marks I have assign grades to each subject of students. This depends on mean of each subject and std dev of certain sequence. This grades depends on Mean of each column of marks and std value which are same for all columns.
Next if we have grades we have to calculate Total Grade Points secured. For each subject column there are credit points which is known. Also grade point wrt to each grade is also known. I want to calculate numerical sum of credit points for each student.. I have tried to code some part of it... I would seek your help to make it simpler and elegant...
#continued
#find mean of each subject
(meanx = colMeans(marks1))
#(sdx=apply(marks1,2,sd))
(sd1 = seq(1.5, -2,-.5)) #this pattern is same across all subjects
#these are the grades associated with each Std Dev
(names(sd1) = c('AP','A','AM','BP','B','BM','CP','C'))
sd1
sd1['AP'] #test for A+
#this is sample vectorise function created: here meanx should be replace with respective mean of the subject
RgradeAssigned = function(x) { ifelse(x >= meanx * sd1['AP'],'AP', ifelse(x >= meanx + sd1['A'], 'A', ifelse(x >= meanx + sd1['AM'], 'AM', ifelse(x >= meanx + sd1['BP'], 'BP', ifelse(x >= meanx * sd1['B'], 'B',ifelse(x >= meanx + sd1['BM'], 'BM',ifelse(x >= meanx + sd1['CP'], 'CP',ifelse(x >= meanx + sd1['C'], 'C', 'F'))))))))}
RgradeAssigned(marks1)
#meanx should mean from the vector meanx : for sub1 it should be meanx[1]
meanx
#Calculate SGPA
#credit for each subject
(subcredits = c(3,2,4,3,3))
#gradepoints with wrt to each grade
gradeName = c('AP','A','AM','BP','B','BM','CP','C','F')
gradePoint = c(10,9,8,7,6,5,4,3,0)
names(gradePoint) = gradeName
gradePoint
# if a particular grade, find grade point -> multiply by subject credit -> for each student -> find sum of grades ie sum each row
# for eg row grade were
#'AP','A','AM','BP','B' -> 10,9,8,7,6 -> 10*3 + 9*2+ 8*4 + 7*3 + 6*3
#subject credits : 3,2,4,3,3
#Now Mapping of Grade to Point is available
r dplyr mutate
add a comment |
I have create marks of 15 students for 5 subjects. I have also create a vector of passmarks in each subject. I want to mutate each column of marks with Pass or Fail depending upon the values given in the vector passmarks... I have been able to manually, but I want to use some looping function so that it maps the passmarks to columns and then mutates creating a new column or new dataframe with Pass or Fail.
create a sample vector with 15 positions
x <- runif(15)
#convert to DF
marks <- as.data.frame(x)
marks[1:5] <- sapply(1:5, "+", rnorm(5,60,15))
names(marks) <- paste0("sub", 1:5)
marks
colMeans(marks)
lapply(marks, range)
lapply(marks, is.na)
colSums(is.na(marks))
marks1 = round(marks,2)
passmarks = c(60, 65, 62,70, 45)
names(marks1)
marks1 %>% mutate(sub1a = ifelse(sub1 <= passmarks[1], 'F','P'), sub2a = ifelse(sub2 <= passmarks[2], 'F','P'), sub3a = ifelse(sub3 <= passmarks[3], 'F','P'), sub4a = ifelse(sub4 <= passmarks[4], 'F','P'), sub5a = ifelse(sub5 <= passmarks[5], 'F','P'))
marks1 %>% summarise_at(vars(sub1:sub5), mean, na.rm=T)
marks1 %>% mutate_all(funs(./75))
Part-II of the problem::::
This stage of problem was resolved. I have to calculate SGPA for each student. If I have marks I have assign grades to each subject of students. This depends on mean of each subject and std dev of certain sequence. This grades depends on Mean of each column of marks and std value which are same for all columns.
Next if we have grades we have to calculate Total Grade Points secured. For each subject column there are credit points which is known. Also grade point wrt to each grade is also known. I want to calculate numerical sum of credit points for each student.. I have tried to code some part of it... I would seek your help to make it simpler and elegant...
#continued
#find mean of each subject
(meanx = colMeans(marks1))
#(sdx=apply(marks1,2,sd))
(sd1 = seq(1.5, -2,-.5)) #this pattern is same across all subjects
#these are the grades associated with each Std Dev
(names(sd1) = c('AP','A','AM','BP','B','BM','CP','C'))
sd1
sd1['AP'] #test for A+
#this is sample vectorise function created: here meanx should be replace with respective mean of the subject
RgradeAssigned = function(x) { ifelse(x >= meanx * sd1['AP'],'AP', ifelse(x >= meanx + sd1['A'], 'A', ifelse(x >= meanx + sd1['AM'], 'AM', ifelse(x >= meanx + sd1['BP'], 'BP', ifelse(x >= meanx * sd1['B'], 'B',ifelse(x >= meanx + sd1['BM'], 'BM',ifelse(x >= meanx + sd1['CP'], 'CP',ifelse(x >= meanx + sd1['C'], 'C', 'F'))))))))}
RgradeAssigned(marks1)
#meanx should mean from the vector meanx : for sub1 it should be meanx[1]
meanx
#Calculate SGPA
#credit for each subject
(subcredits = c(3,2,4,3,3))
#gradepoints with wrt to each grade
gradeName = c('AP','A','AM','BP','B','BM','CP','C','F')
gradePoint = c(10,9,8,7,6,5,4,3,0)
names(gradePoint) = gradeName
gradePoint
# if a particular grade, find grade point -> multiply by subject credit -> for each student -> find sum of grades ie sum each row
# for eg row grade were
#'AP','A','AM','BP','B' -> 10,9,8,7,6 -> 10*3 + 9*2+ 8*4 + 7*3 + 6*3
#subject credits : 3,2,4,3,3
#Now Mapping of Grade to Point is available
r dplyr mutate
add a comment |
I have create marks of 15 students for 5 subjects. I have also create a vector of passmarks in each subject. I want to mutate each column of marks with Pass or Fail depending upon the values given in the vector passmarks... I have been able to manually, but I want to use some looping function so that it maps the passmarks to columns and then mutates creating a new column or new dataframe with Pass or Fail.
create a sample vector with 15 positions
x <- runif(15)
#convert to DF
marks <- as.data.frame(x)
marks[1:5] <- sapply(1:5, "+", rnorm(5,60,15))
names(marks) <- paste0("sub", 1:5)
marks
colMeans(marks)
lapply(marks, range)
lapply(marks, is.na)
colSums(is.na(marks))
marks1 = round(marks,2)
passmarks = c(60, 65, 62,70, 45)
names(marks1)
marks1 %>% mutate(sub1a = ifelse(sub1 <= passmarks[1], 'F','P'), sub2a = ifelse(sub2 <= passmarks[2], 'F','P'), sub3a = ifelse(sub3 <= passmarks[3], 'F','P'), sub4a = ifelse(sub4 <= passmarks[4], 'F','P'), sub5a = ifelse(sub5 <= passmarks[5], 'F','P'))
marks1 %>% summarise_at(vars(sub1:sub5), mean, na.rm=T)
marks1 %>% mutate_all(funs(./75))
Part-II of the problem::::
This stage of problem was resolved. I have to calculate SGPA for each student. If I have marks I have assign grades to each subject of students. This depends on mean of each subject and std dev of certain sequence. This grades depends on Mean of each column of marks and std value which are same for all columns.
Next if we have grades we have to calculate Total Grade Points secured. For each subject column there are credit points which is known. Also grade point wrt to each grade is also known. I want to calculate numerical sum of credit points for each student.. I have tried to code some part of it... I would seek your help to make it simpler and elegant...
#continued
#find mean of each subject
(meanx = colMeans(marks1))
#(sdx=apply(marks1,2,sd))
(sd1 = seq(1.5, -2,-.5)) #this pattern is same across all subjects
#these are the grades associated with each Std Dev
(names(sd1) = c('AP','A','AM','BP','B','BM','CP','C'))
sd1
sd1['AP'] #test for A+
#this is sample vectorise function created: here meanx should be replace with respective mean of the subject
RgradeAssigned = function(x) { ifelse(x >= meanx * sd1['AP'],'AP', ifelse(x >= meanx + sd1['A'], 'A', ifelse(x >= meanx + sd1['AM'], 'AM', ifelse(x >= meanx + sd1['BP'], 'BP', ifelse(x >= meanx * sd1['B'], 'B',ifelse(x >= meanx + sd1['BM'], 'BM',ifelse(x >= meanx + sd1['CP'], 'CP',ifelse(x >= meanx + sd1['C'], 'C', 'F'))))))))}
RgradeAssigned(marks1)
#meanx should mean from the vector meanx : for sub1 it should be meanx[1]
meanx
#Calculate SGPA
#credit for each subject
(subcredits = c(3,2,4,3,3))
#gradepoints with wrt to each grade
gradeName = c('AP','A','AM','BP','B','BM','CP','C','F')
gradePoint = c(10,9,8,7,6,5,4,3,0)
names(gradePoint) = gradeName
gradePoint
# if a particular grade, find grade point -> multiply by subject credit -> for each student -> find sum of grades ie sum each row
# for eg row grade were
#'AP','A','AM','BP','B' -> 10,9,8,7,6 -> 10*3 + 9*2+ 8*4 + 7*3 + 6*3
#subject credits : 3,2,4,3,3
#Now Mapping of Grade to Point is available
r dplyr mutate
I have create marks of 15 students for 5 subjects. I have also create a vector of passmarks in each subject. I want to mutate each column of marks with Pass or Fail depending upon the values given in the vector passmarks... I have been able to manually, but I want to use some looping function so that it maps the passmarks to columns and then mutates creating a new column or new dataframe with Pass or Fail.
create a sample vector with 15 positions
x <- runif(15)
#convert to DF
marks <- as.data.frame(x)
marks[1:5] <- sapply(1:5, "+", rnorm(5,60,15))
names(marks) <- paste0("sub", 1:5)
marks
colMeans(marks)
lapply(marks, range)
lapply(marks, is.na)
colSums(is.na(marks))
marks1 = round(marks,2)
passmarks = c(60, 65, 62,70, 45)
names(marks1)
marks1 %>% mutate(sub1a = ifelse(sub1 <= passmarks[1], 'F','P'), sub2a = ifelse(sub2 <= passmarks[2], 'F','P'), sub3a = ifelse(sub3 <= passmarks[3], 'F','P'), sub4a = ifelse(sub4 <= passmarks[4], 'F','P'), sub5a = ifelse(sub5 <= passmarks[5], 'F','P'))
marks1 %>% summarise_at(vars(sub1:sub5), mean, na.rm=T)
marks1 %>% mutate_all(funs(./75))
Part-II of the problem::::
This stage of problem was resolved. I have to calculate SGPA for each student. If I have marks I have assign grades to each subject of students. This depends on mean of each subject and std dev of certain sequence. This grades depends on Mean of each column of marks and std value which are same for all columns.
Next if we have grades we have to calculate Total Grade Points secured. For each subject column there are credit points which is known. Also grade point wrt to each grade is also known. I want to calculate numerical sum of credit points for each student.. I have tried to code some part of it... I would seek your help to make it simpler and elegant...
#continued
#find mean of each subject
(meanx = colMeans(marks1))
#(sdx=apply(marks1,2,sd))
(sd1 = seq(1.5, -2,-.5)) #this pattern is same across all subjects
#these are the grades associated with each Std Dev
(names(sd1) = c('AP','A','AM','BP','B','BM','CP','C'))
sd1
sd1['AP'] #test for A+
#this is sample vectorise function created: here meanx should be replace with respective mean of the subject
RgradeAssigned = function(x) { ifelse(x >= meanx * sd1['AP'],'AP', ifelse(x >= meanx + sd1['A'], 'A', ifelse(x >= meanx + sd1['AM'], 'AM', ifelse(x >= meanx + sd1['BP'], 'BP', ifelse(x >= meanx * sd1['B'], 'B',ifelse(x >= meanx + sd1['BM'], 'BM',ifelse(x >= meanx + sd1['CP'], 'CP',ifelse(x >= meanx + sd1['C'], 'C', 'F'))))))))}
RgradeAssigned(marks1)
#meanx should mean from the vector meanx : for sub1 it should be meanx[1]
meanx
#Calculate SGPA
#credit for each subject
(subcredits = c(3,2,4,3,3))
#gradepoints with wrt to each grade
gradeName = c('AP','A','AM','BP','B','BM','CP','C','F')
gradePoint = c(10,9,8,7,6,5,4,3,0)
names(gradePoint) = gradeName
gradePoint
# if a particular grade, find grade point -> multiply by subject credit -> for each student -> find sum of grades ie sum each row
# for eg row grade were
#'AP','A','AM','BP','B' -> 10,9,8,7,6 -> 10*3 + 9*2+ 8*4 + 7*3 + 6*3
#subject credits : 3,2,4,3,3
#Now Mapping of Grade to Point is available
r dplyr mutate
r dplyr mutate
edited Nov 23 '18 at 3:58
Dhiraj Upadhyaya
asked Nov 22 '18 at 12:21


Dhiraj UpadhyayaDhiraj Upadhyaya
214
214
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
You can use mapply
, i.e.
mapply(`<`, passmarks, marks1)
which gives,
[,1] [,2] [,3] [,4] [,5]
[1,] FALSE FALSE FALSE FALSE TRUE
[2,] FALSE FALSE FALSE FALSE TRUE
[3,] FALSE FALSE FALSE FALSE TRUE
[4,] TRUE TRUE TRUE FALSE TRUE
[5,] FALSE FALSE FALSE FALSE TRUE
[6,] FALSE FALSE FALSE FALSE TRUE
[7,] FALSE FALSE FALSE FALSE TRUE
[8,] FALSE FALSE FALSE FALSE TRUE
[9,] TRUE TRUE TRUE FALSE TRUE
[10,] FALSE FALSE FALSE FALSE TRUE
[11,] FALSE FALSE FALSE FALSE TRUE
[12,] FALSE FALSE FALSE FALSE TRUE
[13,] FALSE FALSE FALSE FALSE TRUE
[14,] TRUE FALSE TRUE FALSE TRUE
[15,] FALSE FALSE FALSE FALSE TRUE
To get a matrix with PASS
and FAIL
, then one way to do it could be
matrix(c('FAIL', 'PASS')[mapply(`<`, passmarks, marks1) + 1], ncol = ncol(marks1))
1
@RonakShah I thought so too, but did not map it one-on-one when I tried it...
– Sotos
Nov 22 '18 at 12:36
To replicate the exact format from OP's manual solution:mapply(function(x, y) ifelse(x > y, 'P', 'F'), marks, passmarks) %>% cbind(marks, .)
– jdobres
Nov 22 '18 at 12:41
@RonakShah Any idea how they were mapped? I m curious but do not have the time to investigate it now
– Sotos
Nov 22 '18 at 12:50
@Sotos I think it starts comparing column-wise and then recycles. Sopassmarks[1]
withmarks[1, 1]
,passmarks[2]
withmarks[2, 1]
and so on.
– Ronak Shah
Nov 22 '18 at 13:05
I have part-2 of the problem in my original question. Can I seek your valuable help
– Dhiraj Upadhyaya
Nov 23 '18 at 3:58
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53430919%2fmutate-using-dply-depeding-upon-the-condition%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can use mapply
, i.e.
mapply(`<`, passmarks, marks1)
which gives,
[,1] [,2] [,3] [,4] [,5]
[1,] FALSE FALSE FALSE FALSE TRUE
[2,] FALSE FALSE FALSE FALSE TRUE
[3,] FALSE FALSE FALSE FALSE TRUE
[4,] TRUE TRUE TRUE FALSE TRUE
[5,] FALSE FALSE FALSE FALSE TRUE
[6,] FALSE FALSE FALSE FALSE TRUE
[7,] FALSE FALSE FALSE FALSE TRUE
[8,] FALSE FALSE FALSE FALSE TRUE
[9,] TRUE TRUE TRUE FALSE TRUE
[10,] FALSE FALSE FALSE FALSE TRUE
[11,] FALSE FALSE FALSE FALSE TRUE
[12,] FALSE FALSE FALSE FALSE TRUE
[13,] FALSE FALSE FALSE FALSE TRUE
[14,] TRUE FALSE TRUE FALSE TRUE
[15,] FALSE FALSE FALSE FALSE TRUE
To get a matrix with PASS
and FAIL
, then one way to do it could be
matrix(c('FAIL', 'PASS')[mapply(`<`, passmarks, marks1) + 1], ncol = ncol(marks1))
1
@RonakShah I thought so too, but did not map it one-on-one when I tried it...
– Sotos
Nov 22 '18 at 12:36
To replicate the exact format from OP's manual solution:mapply(function(x, y) ifelse(x > y, 'P', 'F'), marks, passmarks) %>% cbind(marks, .)
– jdobres
Nov 22 '18 at 12:41
@RonakShah Any idea how they were mapped? I m curious but do not have the time to investigate it now
– Sotos
Nov 22 '18 at 12:50
@Sotos I think it starts comparing column-wise and then recycles. Sopassmarks[1]
withmarks[1, 1]
,passmarks[2]
withmarks[2, 1]
and so on.
– Ronak Shah
Nov 22 '18 at 13:05
I have part-2 of the problem in my original question. Can I seek your valuable help
– Dhiraj Upadhyaya
Nov 23 '18 at 3:58
add a comment |
You can use mapply
, i.e.
mapply(`<`, passmarks, marks1)
which gives,
[,1] [,2] [,3] [,4] [,5]
[1,] FALSE FALSE FALSE FALSE TRUE
[2,] FALSE FALSE FALSE FALSE TRUE
[3,] FALSE FALSE FALSE FALSE TRUE
[4,] TRUE TRUE TRUE FALSE TRUE
[5,] FALSE FALSE FALSE FALSE TRUE
[6,] FALSE FALSE FALSE FALSE TRUE
[7,] FALSE FALSE FALSE FALSE TRUE
[8,] FALSE FALSE FALSE FALSE TRUE
[9,] TRUE TRUE TRUE FALSE TRUE
[10,] FALSE FALSE FALSE FALSE TRUE
[11,] FALSE FALSE FALSE FALSE TRUE
[12,] FALSE FALSE FALSE FALSE TRUE
[13,] FALSE FALSE FALSE FALSE TRUE
[14,] TRUE FALSE TRUE FALSE TRUE
[15,] FALSE FALSE FALSE FALSE TRUE
To get a matrix with PASS
and FAIL
, then one way to do it could be
matrix(c('FAIL', 'PASS')[mapply(`<`, passmarks, marks1) + 1], ncol = ncol(marks1))
1
@RonakShah I thought so too, but did not map it one-on-one when I tried it...
– Sotos
Nov 22 '18 at 12:36
To replicate the exact format from OP's manual solution:mapply(function(x, y) ifelse(x > y, 'P', 'F'), marks, passmarks) %>% cbind(marks, .)
– jdobres
Nov 22 '18 at 12:41
@RonakShah Any idea how they were mapped? I m curious but do not have the time to investigate it now
– Sotos
Nov 22 '18 at 12:50
@Sotos I think it starts comparing column-wise and then recycles. Sopassmarks[1]
withmarks[1, 1]
,passmarks[2]
withmarks[2, 1]
and so on.
– Ronak Shah
Nov 22 '18 at 13:05
I have part-2 of the problem in my original question. Can I seek your valuable help
– Dhiraj Upadhyaya
Nov 23 '18 at 3:58
add a comment |
You can use mapply
, i.e.
mapply(`<`, passmarks, marks1)
which gives,
[,1] [,2] [,3] [,4] [,5]
[1,] FALSE FALSE FALSE FALSE TRUE
[2,] FALSE FALSE FALSE FALSE TRUE
[3,] FALSE FALSE FALSE FALSE TRUE
[4,] TRUE TRUE TRUE FALSE TRUE
[5,] FALSE FALSE FALSE FALSE TRUE
[6,] FALSE FALSE FALSE FALSE TRUE
[7,] FALSE FALSE FALSE FALSE TRUE
[8,] FALSE FALSE FALSE FALSE TRUE
[9,] TRUE TRUE TRUE FALSE TRUE
[10,] FALSE FALSE FALSE FALSE TRUE
[11,] FALSE FALSE FALSE FALSE TRUE
[12,] FALSE FALSE FALSE FALSE TRUE
[13,] FALSE FALSE FALSE FALSE TRUE
[14,] TRUE FALSE TRUE FALSE TRUE
[15,] FALSE FALSE FALSE FALSE TRUE
To get a matrix with PASS
and FAIL
, then one way to do it could be
matrix(c('FAIL', 'PASS')[mapply(`<`, passmarks, marks1) + 1], ncol = ncol(marks1))
You can use mapply
, i.e.
mapply(`<`, passmarks, marks1)
which gives,
[,1] [,2] [,3] [,4] [,5]
[1,] FALSE FALSE FALSE FALSE TRUE
[2,] FALSE FALSE FALSE FALSE TRUE
[3,] FALSE FALSE FALSE FALSE TRUE
[4,] TRUE TRUE TRUE FALSE TRUE
[5,] FALSE FALSE FALSE FALSE TRUE
[6,] FALSE FALSE FALSE FALSE TRUE
[7,] FALSE FALSE FALSE FALSE TRUE
[8,] FALSE FALSE FALSE FALSE TRUE
[9,] TRUE TRUE TRUE FALSE TRUE
[10,] FALSE FALSE FALSE FALSE TRUE
[11,] FALSE FALSE FALSE FALSE TRUE
[12,] FALSE FALSE FALSE FALSE TRUE
[13,] FALSE FALSE FALSE FALSE TRUE
[14,] TRUE FALSE TRUE FALSE TRUE
[15,] FALSE FALSE FALSE FALSE TRUE
To get a matrix with PASS
and FAIL
, then one way to do it could be
matrix(c('FAIL', 'PASS')[mapply(`<`, passmarks, marks1) + 1], ncol = ncol(marks1))
edited Nov 22 '18 at 12:46
answered Nov 22 '18 at 12:33


SotosSotos
29.3k51640
29.3k51640
1
@RonakShah I thought so too, but did not map it one-on-one when I tried it...
– Sotos
Nov 22 '18 at 12:36
To replicate the exact format from OP's manual solution:mapply(function(x, y) ifelse(x > y, 'P', 'F'), marks, passmarks) %>% cbind(marks, .)
– jdobres
Nov 22 '18 at 12:41
@RonakShah Any idea how they were mapped? I m curious but do not have the time to investigate it now
– Sotos
Nov 22 '18 at 12:50
@Sotos I think it starts comparing column-wise and then recycles. Sopassmarks[1]
withmarks[1, 1]
,passmarks[2]
withmarks[2, 1]
and so on.
– Ronak Shah
Nov 22 '18 at 13:05
I have part-2 of the problem in my original question. Can I seek your valuable help
– Dhiraj Upadhyaya
Nov 23 '18 at 3:58
add a comment |
1
@RonakShah I thought so too, but did not map it one-on-one when I tried it...
– Sotos
Nov 22 '18 at 12:36
To replicate the exact format from OP's manual solution:mapply(function(x, y) ifelse(x > y, 'P', 'F'), marks, passmarks) %>% cbind(marks, .)
– jdobres
Nov 22 '18 at 12:41
@RonakShah Any idea how they were mapped? I m curious but do not have the time to investigate it now
– Sotos
Nov 22 '18 at 12:50
@Sotos I think it starts comparing column-wise and then recycles. Sopassmarks[1]
withmarks[1, 1]
,passmarks[2]
withmarks[2, 1]
and so on.
– Ronak Shah
Nov 22 '18 at 13:05
I have part-2 of the problem in my original question. Can I seek your valuable help
– Dhiraj Upadhyaya
Nov 23 '18 at 3:58
1
1
@RonakShah I thought so too, but did not map it one-on-one when I tried it...
– Sotos
Nov 22 '18 at 12:36
@RonakShah I thought so too, but did not map it one-on-one when I tried it...
– Sotos
Nov 22 '18 at 12:36
To replicate the exact format from OP's manual solution:
mapply(function(x, y) ifelse(x > y, 'P', 'F'), marks, passmarks) %>% cbind(marks, .)
– jdobres
Nov 22 '18 at 12:41
To replicate the exact format from OP's manual solution:
mapply(function(x, y) ifelse(x > y, 'P', 'F'), marks, passmarks) %>% cbind(marks, .)
– jdobres
Nov 22 '18 at 12:41
@RonakShah Any idea how they were mapped? I m curious but do not have the time to investigate it now
– Sotos
Nov 22 '18 at 12:50
@RonakShah Any idea how they were mapped? I m curious but do not have the time to investigate it now
– Sotos
Nov 22 '18 at 12:50
@Sotos I think it starts comparing column-wise and then recycles. So
passmarks[1]
with marks[1, 1]
, passmarks[2]
with marks[2, 1]
and so on.– Ronak Shah
Nov 22 '18 at 13:05
@Sotos I think it starts comparing column-wise and then recycles. So
passmarks[1]
with marks[1, 1]
, passmarks[2]
with marks[2, 1]
and so on.– Ronak Shah
Nov 22 '18 at 13:05
I have part-2 of the problem in my original question. Can I seek your valuable help
– Dhiraj Upadhyaya
Nov 23 '18 at 3:58
I have part-2 of the problem in my original question. Can I seek your valuable help
– Dhiraj Upadhyaya
Nov 23 '18 at 3:58
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53430919%2fmutate-using-dply-depeding-upon-the-condition%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
62,c 9tb 2 myBgL RN