No suitable constructor found for ClassUser(no arguments)
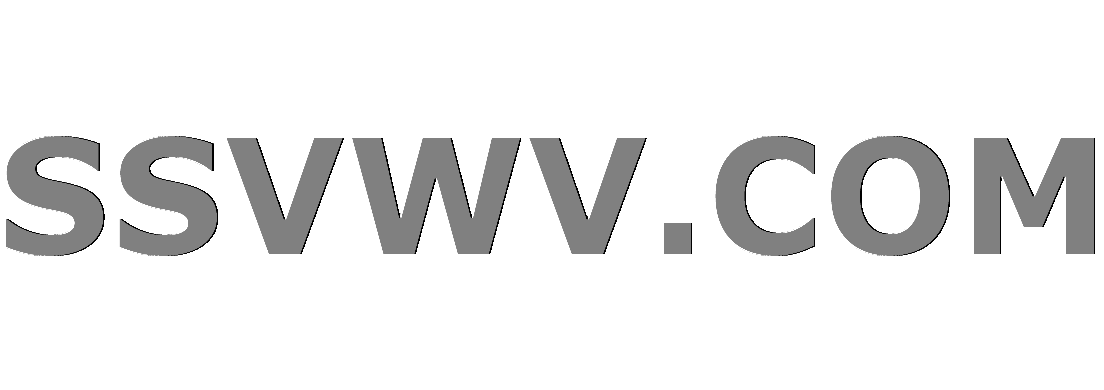
Multi tool use
I'm new to Java and I'm trying to get my head around inheritance.
The error is no suitable constructor found for ClassUser(no arguments)
appears at public ClassAdmin(String data)
and I haven't found any solutions of help to me.
This is my a snippet of my ClassUser
:
public class ClassUser {
public String id;
public String password;
public String name;
public String address;
public String contact;
public String role;
public ClassUser(String id, String password, String name, String address, String contact, String role){
this.id = id;
this.password = password;
this.name = name;
this.address = address;
this.contact = contact;
this.role = role;
}
public ClassUser(String data){
String dataArray = data.split(",");
this.id = dataArray[0];
this.password = dataArray[1];
this.name = dataArray[2];
this.address = dataArray[3];
this.contact = dataArray[4];
this.role = dataArray[5];
}
This is a snippet of my ClassAdmin
:
public class ClassAdmin extends ClassUser{
public String email;
public ClassAdmin(String id, String password, String name, String contact,
String email)
{
super(id+password+name+contact);
this.email = email;
}
public ClassAdmin(String data){ //problem
String dataArray = data.split(",");
this.id = dataArray[0];
this.password = dataArray[1];
this.name = dataArray[2];
this.contact = dataArray[3];
this.email = dataArray[4];
}
java inheritance netbeans
add a comment |
I'm new to Java and I'm trying to get my head around inheritance.
The error is no suitable constructor found for ClassUser(no arguments)
appears at public ClassAdmin(String data)
and I haven't found any solutions of help to me.
This is my a snippet of my ClassUser
:
public class ClassUser {
public String id;
public String password;
public String name;
public String address;
public String contact;
public String role;
public ClassUser(String id, String password, String name, String address, String contact, String role){
this.id = id;
this.password = password;
this.name = name;
this.address = address;
this.contact = contact;
this.role = role;
}
public ClassUser(String data){
String dataArray = data.split(",");
this.id = dataArray[0];
this.password = dataArray[1];
this.name = dataArray[2];
this.address = dataArray[3];
this.contact = dataArray[4];
this.role = dataArray[5];
}
This is a snippet of my ClassAdmin
:
public class ClassAdmin extends ClassUser{
public String email;
public ClassAdmin(String id, String password, String name, String contact,
String email)
{
super(id+password+name+contact);
this.email = email;
}
public ClassAdmin(String data){ //problem
String dataArray = data.split(",");
this.id = dataArray[0];
this.password = dataArray[1];
this.name = dataArray[2];
this.contact = dataArray[3];
this.email = dataArray[4];
}
java inheritance netbeans
Please call super constructor first in "public ClassAdmin(String data){"
– Raheela Aslam
Nov 22 '18 at 11:46
add a comment |
I'm new to Java and I'm trying to get my head around inheritance.
The error is no suitable constructor found for ClassUser(no arguments)
appears at public ClassAdmin(String data)
and I haven't found any solutions of help to me.
This is my a snippet of my ClassUser
:
public class ClassUser {
public String id;
public String password;
public String name;
public String address;
public String contact;
public String role;
public ClassUser(String id, String password, String name, String address, String contact, String role){
this.id = id;
this.password = password;
this.name = name;
this.address = address;
this.contact = contact;
this.role = role;
}
public ClassUser(String data){
String dataArray = data.split(",");
this.id = dataArray[0];
this.password = dataArray[1];
this.name = dataArray[2];
this.address = dataArray[3];
this.contact = dataArray[4];
this.role = dataArray[5];
}
This is a snippet of my ClassAdmin
:
public class ClassAdmin extends ClassUser{
public String email;
public ClassAdmin(String id, String password, String name, String contact,
String email)
{
super(id+password+name+contact);
this.email = email;
}
public ClassAdmin(String data){ //problem
String dataArray = data.split(",");
this.id = dataArray[0];
this.password = dataArray[1];
this.name = dataArray[2];
this.contact = dataArray[3];
this.email = dataArray[4];
}
java inheritance netbeans
I'm new to Java and I'm trying to get my head around inheritance.
The error is no suitable constructor found for ClassUser(no arguments)
appears at public ClassAdmin(String data)
and I haven't found any solutions of help to me.
This is my a snippet of my ClassUser
:
public class ClassUser {
public String id;
public String password;
public String name;
public String address;
public String contact;
public String role;
public ClassUser(String id, String password, String name, String address, String contact, String role){
this.id = id;
this.password = password;
this.name = name;
this.address = address;
this.contact = contact;
this.role = role;
}
public ClassUser(String data){
String dataArray = data.split(",");
this.id = dataArray[0];
this.password = dataArray[1];
this.name = dataArray[2];
this.address = dataArray[3];
this.contact = dataArray[4];
this.role = dataArray[5];
}
This is a snippet of my ClassAdmin
:
public class ClassAdmin extends ClassUser{
public String email;
public ClassAdmin(String id, String password, String name, String contact,
String email)
{
super(id+password+name+contact);
this.email = email;
}
public ClassAdmin(String data){ //problem
String dataArray = data.split(",");
this.id = dataArray[0];
this.password = dataArray[1];
this.name = dataArray[2];
this.contact = dataArray[3];
this.email = dataArray[4];
}
java inheritance netbeans
java inheritance netbeans
edited Nov 22 '18 at 12:22
Aleksandr Podkutin
2,10711325
2,10711325
asked Nov 22 '18 at 11:45
bk23bk23
103
103
Please call super constructor first in "public ClassAdmin(String data){"
– Raheela Aslam
Nov 22 '18 at 11:46
add a comment |
Please call super constructor first in "public ClassAdmin(String data){"
– Raheela Aslam
Nov 22 '18 at 11:46
Please call super constructor first in "public ClassAdmin(String data){"
– Raheela Aslam
Nov 22 '18 at 11:46
Please call super constructor first in "public ClassAdmin(String data){"
– Raheela Aslam
Nov 22 '18 at 11:46
add a comment |
5 Answers
5
active
oldest
votes
You need to call supper constructor into :
public class ClassAdmin extends ClassUser {
public String email;
public ClassAdmin(String id, String password, String name, String contact,
String email) {
super(id + password + name + contact);
this.email = email;
}
public ClassAdmin(String data) { //problem
super(data);
String dataArray = data.split(",");
this.id = dataArray[0];
this.password = dataArray[1];
this.name = dataArray[2];
this.contact = dataArray[3];
this.email = dataArray[4];
}
}
add a comment |
Since there is no default constructor
present in ClassUser
but parameterised constructor present, so while extending the ClassUser
you should call
ClassUser(data)
or
ClassUser(String id, String password, String name, String address, String contact, String role)
using super
super- java docs
super(data)
or
super(id, password, name, address, contact, role)
add a comment |
You didn't call super()
explicitly in the second ClassAdmin
constructor so the compiler add super()
call without any parameters. You don't have parameterless ClassUser
constructor so you are getting the error.
Add explicit super
call
public ClassAdmin(String data) {
super(data.split(",")[0] + data.split(",")[1] + data.split(",")[2] + data.split(",")[3]);
//...
}
Or use private ClassAdmin
constructor to avoid repeating use of data.split(",")
(still two uses though)
public ClassAdmin(String data) {
this(data.split(","));
//...
}
private ClassAdmin(String dataArray) {
super(dataArray[0] + dataArray[1] + dataArray[2] + dataArray[3]);
}
Or create parameterless ClassUser
constructor
public ClassUser() { }
@Eran sure, fixed.
– Guy
Nov 22 '18 at 11:55
add a comment |
You need to add invokes of the parent constructor super(data);
to the public ClassAdmin(String data)
constructor as well.
Because if you don't call parent constructor, you can't create child object. First of all, parent object need to be created. And only after that instantiation of the child object can be executed.
The main reason of the error is that, if you're not providing, explicitly, invocation of the super class constructor, Java compiler tries to find default super class constructor with no argument and in your case there is no default no argument constructor in the ClassUser
class.
You can fix in a two ways:
by adding default constructor to the
ClassUser
:
public ClassUser() {
//some additional logic
}
by adding call of the super class constructor:
public ClassAdmin(String data) {
super(data);
String dataArray = data.split(",");
this.id = dataArray[0];
this.password = dataArray[1];
this.name = dataArray[2];
this.contact = dataArray[3];
this.email = dataArray[4];
}
It depends on your implementation, but be aware that after you will have called super class constructor, you will have overridden some of the properties in your ClassAdmin
constructor.
add a comment |
This is how it works. When you create an instance of Child Class, you need to instantiate member variables of parent/super class as well. If you have a default constructor in Super class then those member variables gets initialized to their default values. If you don't have a default constructor you will have to make a call to parameterized constructor to initialize them. That is why you require a call to super(date) in your ClassAdmin constructor.
Also you don't have to initialize super class member variables like id,password,name etc. in ClassAdmnin constructor. Once you add a super(data) call in this constructor, the member variables of parent class will get initialized in parent class constructor itself.
Moreover your current code will throw NullPointerException as you are getting value from an array based on index without checking the size of array. If you see first constructor of ClassAdmin there is no comma (,) in the string which you are sending to Super class constructor. You can append it like super(id+ "," +password+ "," +name + ","+contact)
public ClassAdmin(String data){
super(data); // Add this line and it should fix the problem.
String dataArray = data.split(",");
this.id = dataArray[0];
this.password = dataArray[1];
this.name = dataArray[2];
this.contact = dataArray[3];
this.email = dataArray[4];
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53430296%2fno-suitable-constructor-found-for-classuserno-arguments%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
You need to call supper constructor into :
public class ClassAdmin extends ClassUser {
public String email;
public ClassAdmin(String id, String password, String name, String contact,
String email) {
super(id + password + name + contact);
this.email = email;
}
public ClassAdmin(String data) { //problem
super(data);
String dataArray = data.split(",");
this.id = dataArray[0];
this.password = dataArray[1];
this.name = dataArray[2];
this.contact = dataArray[3];
this.email = dataArray[4];
}
}
add a comment |
You need to call supper constructor into :
public class ClassAdmin extends ClassUser {
public String email;
public ClassAdmin(String id, String password, String name, String contact,
String email) {
super(id + password + name + contact);
this.email = email;
}
public ClassAdmin(String data) { //problem
super(data);
String dataArray = data.split(",");
this.id = dataArray[0];
this.password = dataArray[1];
this.name = dataArray[2];
this.contact = dataArray[3];
this.email = dataArray[4];
}
}
add a comment |
You need to call supper constructor into :
public class ClassAdmin extends ClassUser {
public String email;
public ClassAdmin(String id, String password, String name, String contact,
String email) {
super(id + password + name + contact);
this.email = email;
}
public ClassAdmin(String data) { //problem
super(data);
String dataArray = data.split(",");
this.id = dataArray[0];
this.password = dataArray[1];
this.name = dataArray[2];
this.contact = dataArray[3];
this.email = dataArray[4];
}
}
You need to call supper constructor into :
public class ClassAdmin extends ClassUser {
public String email;
public ClassAdmin(String id, String password, String name, String contact,
String email) {
super(id + password + name + contact);
this.email = email;
}
public ClassAdmin(String data) { //problem
super(data);
String dataArray = data.split(",");
this.id = dataArray[0];
this.password = dataArray[1];
this.name = dataArray[2];
this.contact = dataArray[3];
this.email = dataArray[4];
}
}
answered Nov 22 '18 at 11:58


Raheela AslamRaheela Aslam
36211
36211
add a comment |
add a comment |
Since there is no default constructor
present in ClassUser
but parameterised constructor present, so while extending the ClassUser
you should call
ClassUser(data)
or
ClassUser(String id, String password, String name, String address, String contact, String role)
using super
super- java docs
super(data)
or
super(id, password, name, address, contact, role)
add a comment |
Since there is no default constructor
present in ClassUser
but parameterised constructor present, so while extending the ClassUser
you should call
ClassUser(data)
or
ClassUser(String id, String password, String name, String address, String contact, String role)
using super
super- java docs
super(data)
or
super(id, password, name, address, contact, role)
add a comment |
Since there is no default constructor
present in ClassUser
but parameterised constructor present, so while extending the ClassUser
you should call
ClassUser(data)
or
ClassUser(String id, String password, String name, String address, String contact, String role)
using super
super- java docs
super(data)
or
super(id, password, name, address, contact, role)
Since there is no default constructor
present in ClassUser
but parameterised constructor present, so while extending the ClassUser
you should call
ClassUser(data)
or
ClassUser(String id, String password, String name, String address, String contact, String role)
using super
super- java docs
super(data)
or
super(id, password, name, address, contact, role)
answered Nov 22 '18 at 11:55


secret super starsecret super star
975114
975114
add a comment |
add a comment |
You didn't call super()
explicitly in the second ClassAdmin
constructor so the compiler add super()
call without any parameters. You don't have parameterless ClassUser
constructor so you are getting the error.
Add explicit super
call
public ClassAdmin(String data) {
super(data.split(",")[0] + data.split(",")[1] + data.split(",")[2] + data.split(",")[3]);
//...
}
Or use private ClassAdmin
constructor to avoid repeating use of data.split(",")
(still two uses though)
public ClassAdmin(String data) {
this(data.split(","));
//...
}
private ClassAdmin(String dataArray) {
super(dataArray[0] + dataArray[1] + dataArray[2] + dataArray[3]);
}
Or create parameterless ClassUser
constructor
public ClassUser() { }
@Eran sure, fixed.
– Guy
Nov 22 '18 at 11:55
add a comment |
You didn't call super()
explicitly in the second ClassAdmin
constructor so the compiler add super()
call without any parameters. You don't have parameterless ClassUser
constructor so you are getting the error.
Add explicit super
call
public ClassAdmin(String data) {
super(data.split(",")[0] + data.split(",")[1] + data.split(",")[2] + data.split(",")[3]);
//...
}
Or use private ClassAdmin
constructor to avoid repeating use of data.split(",")
(still two uses though)
public ClassAdmin(String data) {
this(data.split(","));
//...
}
private ClassAdmin(String dataArray) {
super(dataArray[0] + dataArray[1] + dataArray[2] + dataArray[3]);
}
Or create parameterless ClassUser
constructor
public ClassUser() { }
@Eran sure, fixed.
– Guy
Nov 22 '18 at 11:55
add a comment |
You didn't call super()
explicitly in the second ClassAdmin
constructor so the compiler add super()
call without any parameters. You don't have parameterless ClassUser
constructor so you are getting the error.
Add explicit super
call
public ClassAdmin(String data) {
super(data.split(",")[0] + data.split(",")[1] + data.split(",")[2] + data.split(",")[3]);
//...
}
Or use private ClassAdmin
constructor to avoid repeating use of data.split(",")
(still two uses though)
public ClassAdmin(String data) {
this(data.split(","));
//...
}
private ClassAdmin(String dataArray) {
super(dataArray[0] + dataArray[1] + dataArray[2] + dataArray[3]);
}
Or create parameterless ClassUser
constructor
public ClassUser() { }
You didn't call super()
explicitly in the second ClassAdmin
constructor so the compiler add super()
call without any parameters. You don't have parameterless ClassUser
constructor so you are getting the error.
Add explicit super
call
public ClassAdmin(String data) {
super(data.split(",")[0] + data.split(",")[1] + data.split(",")[2] + data.split(",")[3]);
//...
}
Or use private ClassAdmin
constructor to avoid repeating use of data.split(",")
(still two uses though)
public ClassAdmin(String data) {
this(data.split(","));
//...
}
private ClassAdmin(String dataArray) {
super(dataArray[0] + dataArray[1] + dataArray[2] + dataArray[3]);
}
Or create parameterless ClassUser
constructor
public ClassUser() { }
edited Nov 22 '18 at 12:08
answered Nov 22 '18 at 11:50
GuyGuy
18.5k72149
18.5k72149
@Eran sure, fixed.
– Guy
Nov 22 '18 at 11:55
add a comment |
@Eran sure, fixed.
– Guy
Nov 22 '18 at 11:55
@Eran sure, fixed.
– Guy
Nov 22 '18 at 11:55
@Eran sure, fixed.
– Guy
Nov 22 '18 at 11:55
add a comment |
You need to add invokes of the parent constructor super(data);
to the public ClassAdmin(String data)
constructor as well.
Because if you don't call parent constructor, you can't create child object. First of all, parent object need to be created. And only after that instantiation of the child object can be executed.
The main reason of the error is that, if you're not providing, explicitly, invocation of the super class constructor, Java compiler tries to find default super class constructor with no argument and in your case there is no default no argument constructor in the ClassUser
class.
You can fix in a two ways:
by adding default constructor to the
ClassUser
:
public ClassUser() {
//some additional logic
}
by adding call of the super class constructor:
public ClassAdmin(String data) {
super(data);
String dataArray = data.split(",");
this.id = dataArray[0];
this.password = dataArray[1];
this.name = dataArray[2];
this.contact = dataArray[3];
this.email = dataArray[4];
}
It depends on your implementation, but be aware that after you will have called super class constructor, you will have overridden some of the properties in your ClassAdmin
constructor.
add a comment |
You need to add invokes of the parent constructor super(data);
to the public ClassAdmin(String data)
constructor as well.
Because if you don't call parent constructor, you can't create child object. First of all, parent object need to be created. And only after that instantiation of the child object can be executed.
The main reason of the error is that, if you're not providing, explicitly, invocation of the super class constructor, Java compiler tries to find default super class constructor with no argument and in your case there is no default no argument constructor in the ClassUser
class.
You can fix in a two ways:
by adding default constructor to the
ClassUser
:
public ClassUser() {
//some additional logic
}
by adding call of the super class constructor:
public ClassAdmin(String data) {
super(data);
String dataArray = data.split(",");
this.id = dataArray[0];
this.password = dataArray[1];
this.name = dataArray[2];
this.contact = dataArray[3];
this.email = dataArray[4];
}
It depends on your implementation, but be aware that after you will have called super class constructor, you will have overridden some of the properties in your ClassAdmin
constructor.
add a comment |
You need to add invokes of the parent constructor super(data);
to the public ClassAdmin(String data)
constructor as well.
Because if you don't call parent constructor, you can't create child object. First of all, parent object need to be created. And only after that instantiation of the child object can be executed.
The main reason of the error is that, if you're not providing, explicitly, invocation of the super class constructor, Java compiler tries to find default super class constructor with no argument and in your case there is no default no argument constructor in the ClassUser
class.
You can fix in a two ways:
by adding default constructor to the
ClassUser
:
public ClassUser() {
//some additional logic
}
by adding call of the super class constructor:
public ClassAdmin(String data) {
super(data);
String dataArray = data.split(",");
this.id = dataArray[0];
this.password = dataArray[1];
this.name = dataArray[2];
this.contact = dataArray[3];
this.email = dataArray[4];
}
It depends on your implementation, but be aware that after you will have called super class constructor, you will have overridden some of the properties in your ClassAdmin
constructor.
You need to add invokes of the parent constructor super(data);
to the public ClassAdmin(String data)
constructor as well.
Because if you don't call parent constructor, you can't create child object. First of all, parent object need to be created. And only after that instantiation of the child object can be executed.
The main reason of the error is that, if you're not providing, explicitly, invocation of the super class constructor, Java compiler tries to find default super class constructor with no argument and in your case there is no default no argument constructor in the ClassUser
class.
You can fix in a two ways:
by adding default constructor to the
ClassUser
:
public ClassUser() {
//some additional logic
}
by adding call of the super class constructor:
public ClassAdmin(String data) {
super(data);
String dataArray = data.split(",");
this.id = dataArray[0];
this.password = dataArray[1];
this.name = dataArray[2];
this.contact = dataArray[3];
this.email = dataArray[4];
}
It depends on your implementation, but be aware that after you will have called super class constructor, you will have overridden some of the properties in your ClassAdmin
constructor.
edited Nov 22 '18 at 12:20
answered Nov 22 '18 at 11:50
Aleksandr PodkutinAleksandr Podkutin
2,10711325
2,10711325
add a comment |
add a comment |
This is how it works. When you create an instance of Child Class, you need to instantiate member variables of parent/super class as well. If you have a default constructor in Super class then those member variables gets initialized to their default values. If you don't have a default constructor you will have to make a call to parameterized constructor to initialize them. That is why you require a call to super(date) in your ClassAdmin constructor.
Also you don't have to initialize super class member variables like id,password,name etc. in ClassAdmnin constructor. Once you add a super(data) call in this constructor, the member variables of parent class will get initialized in parent class constructor itself.
Moreover your current code will throw NullPointerException as you are getting value from an array based on index without checking the size of array. If you see first constructor of ClassAdmin there is no comma (,) in the string which you are sending to Super class constructor. You can append it like super(id+ "," +password+ "," +name + ","+contact)
public ClassAdmin(String data){
super(data); // Add this line and it should fix the problem.
String dataArray = data.split(",");
this.id = dataArray[0];
this.password = dataArray[1];
this.name = dataArray[2];
this.contact = dataArray[3];
this.email = dataArray[4];
}
add a comment |
This is how it works. When you create an instance of Child Class, you need to instantiate member variables of parent/super class as well. If you have a default constructor in Super class then those member variables gets initialized to their default values. If you don't have a default constructor you will have to make a call to parameterized constructor to initialize them. That is why you require a call to super(date) in your ClassAdmin constructor.
Also you don't have to initialize super class member variables like id,password,name etc. in ClassAdmnin constructor. Once you add a super(data) call in this constructor, the member variables of parent class will get initialized in parent class constructor itself.
Moreover your current code will throw NullPointerException as you are getting value from an array based on index without checking the size of array. If you see first constructor of ClassAdmin there is no comma (,) in the string which you are sending to Super class constructor. You can append it like super(id+ "," +password+ "," +name + ","+contact)
public ClassAdmin(String data){
super(data); // Add this line and it should fix the problem.
String dataArray = data.split(",");
this.id = dataArray[0];
this.password = dataArray[1];
this.name = dataArray[2];
this.contact = dataArray[3];
this.email = dataArray[4];
}
add a comment |
This is how it works. When you create an instance of Child Class, you need to instantiate member variables of parent/super class as well. If you have a default constructor in Super class then those member variables gets initialized to their default values. If you don't have a default constructor you will have to make a call to parameterized constructor to initialize them. That is why you require a call to super(date) in your ClassAdmin constructor.
Also you don't have to initialize super class member variables like id,password,name etc. in ClassAdmnin constructor. Once you add a super(data) call in this constructor, the member variables of parent class will get initialized in parent class constructor itself.
Moreover your current code will throw NullPointerException as you are getting value from an array based on index without checking the size of array. If you see first constructor of ClassAdmin there is no comma (,) in the string which you are sending to Super class constructor. You can append it like super(id+ "," +password+ "," +name + ","+contact)
public ClassAdmin(String data){
super(data); // Add this line and it should fix the problem.
String dataArray = data.split(",");
this.id = dataArray[0];
this.password = dataArray[1];
this.name = dataArray[2];
this.contact = dataArray[3];
this.email = dataArray[4];
}
This is how it works. When you create an instance of Child Class, you need to instantiate member variables of parent/super class as well. If you have a default constructor in Super class then those member variables gets initialized to their default values. If you don't have a default constructor you will have to make a call to parameterized constructor to initialize them. That is why you require a call to super(date) in your ClassAdmin constructor.
Also you don't have to initialize super class member variables like id,password,name etc. in ClassAdmnin constructor. Once you add a super(data) call in this constructor, the member variables of parent class will get initialized in parent class constructor itself.
Moreover your current code will throw NullPointerException as you are getting value from an array based on index without checking the size of array. If you see first constructor of ClassAdmin there is no comma (,) in the string which you are sending to Super class constructor. You can append it like super(id+ "," +password+ "," +name + ","+contact)
public ClassAdmin(String data){
super(data); // Add this line and it should fix the problem.
String dataArray = data.split(",");
this.id = dataArray[0];
this.password = dataArray[1];
this.name = dataArray[2];
this.contact = dataArray[3];
this.email = dataArray[4];
}
public ClassAdmin(String data){
super(data); // Add this line and it should fix the problem.
String dataArray = data.split(",");
this.id = dataArray[0];
this.password = dataArray[1];
this.name = dataArray[2];
this.contact = dataArray[3];
this.email = dataArray[4];
}
public ClassAdmin(String data){
super(data); // Add this line and it should fix the problem.
String dataArray = data.split(",");
this.id = dataArray[0];
this.password = dataArray[1];
this.name = dataArray[2];
this.contact = dataArray[3];
this.email = dataArray[4];
}
edited Nov 22 '18 at 12:26
answered Nov 22 '18 at 12:15


vinay khuranavinay khurana
763
763
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53430296%2fno-suitable-constructor-found-for-classuserno-arguments%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
XQFVHr 3Wpgm3FVN5Nv
Please call super constructor first in "public ClassAdmin(String data){"
– Raheela Aslam
Nov 22 '18 at 11:46