How to remove null values from nested arrays
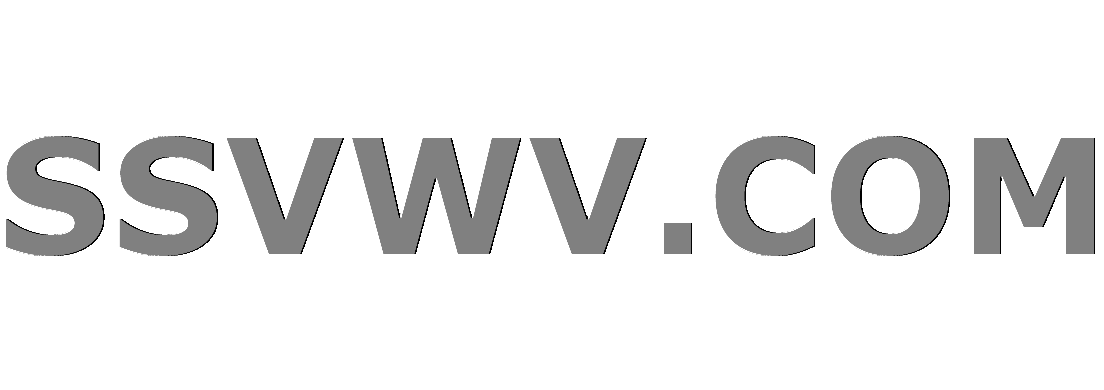
Multi tool use
This code removes all null values from array:
var array = [ 0, 1, null, 2, "", 3, undefined, 3,,,,,, 4,, 4,, 5,, 6,,,, ];
var filtered = array.filter(function (el) {
return el != null;
});
console.log(filtered);
But when I try this on an array with nested arrays that have null values, the nulls are not removed:
var array = [ [ 1, null, 2 ], [ 3, null, 4 ], [ 5, null, 6 ] ];
var filtered = array.filter(function (el) {
return el != null;
});
console.log(filtered);
The expected output is:
[ [ 1, 2 ], [ 3, 4 ], [ 5, 6 ] ]
Instead of the actual output:
[ [ 1, null, 2 ], [ 3, null, 4 ], [ 5, null, 6 ] ]
How can I change my example to filter null values from the nested arrays?
javascript arrays
add a comment |
This code removes all null values from array:
var array = [ 0, 1, null, 2, "", 3, undefined, 3,,,,,, 4,, 4,, 5,, 6,,,, ];
var filtered = array.filter(function (el) {
return el != null;
});
console.log(filtered);
But when I try this on an array with nested arrays that have null values, the nulls are not removed:
var array = [ [ 1, null, 2 ], [ 3, null, 4 ], [ 5, null, 6 ] ];
var filtered = array.filter(function (el) {
return el != null;
});
console.log(filtered);
The expected output is:
[ [ 1, 2 ], [ 3, 4 ], [ 5, 6 ] ]
Instead of the actual output:
[ [ 1, null, 2 ], [ 3, null, 4 ], [ 5, null, 6 ] ]
How can I change my example to filter null values from the nested arrays?
javascript arrays
why index 2? what happens to index 1 of the inner arrays for the result? what is the reason for the nested arrays?
– Nina Scholz
Nov 22 '18 at 18:59
Maybe look to stackoverflow.com/a/38132582/6523409. Filter should be called recursively.
– Filip Š
Nov 22 '18 at 19:01
@Nina Scholz, I have a long array with thouthands of rows with null elements inside. I'd like to make it work with every null element, not only for index 1.
– Jooms
Nov 22 '18 at 19:05
i mean, how do you come from the given array with falsy values to the result?
– Nina Scholz
Nov 22 '18 at 19:06
I've edited your question to hopefully clarify for everyone. If I have deviated too far from your original intent, please feel free to roll back my edit via the revisions page
– Tiny Giant
Nov 22 '18 at 19:47
add a comment |
This code removes all null values from array:
var array = [ 0, 1, null, 2, "", 3, undefined, 3,,,,,, 4,, 4,, 5,, 6,,,, ];
var filtered = array.filter(function (el) {
return el != null;
});
console.log(filtered);
But when I try this on an array with nested arrays that have null values, the nulls are not removed:
var array = [ [ 1, null, 2 ], [ 3, null, 4 ], [ 5, null, 6 ] ];
var filtered = array.filter(function (el) {
return el != null;
});
console.log(filtered);
The expected output is:
[ [ 1, 2 ], [ 3, 4 ], [ 5, 6 ] ]
Instead of the actual output:
[ [ 1, null, 2 ], [ 3, null, 4 ], [ 5, null, 6 ] ]
How can I change my example to filter null values from the nested arrays?
javascript arrays
This code removes all null values from array:
var array = [ 0, 1, null, 2, "", 3, undefined, 3,,,,,, 4,, 4,, 5,, 6,,,, ];
var filtered = array.filter(function (el) {
return el != null;
});
console.log(filtered);
But when I try this on an array with nested arrays that have null values, the nulls are not removed:
var array = [ [ 1, null, 2 ], [ 3, null, 4 ], [ 5, null, 6 ] ];
var filtered = array.filter(function (el) {
return el != null;
});
console.log(filtered);
The expected output is:
[ [ 1, 2 ], [ 3, 4 ], [ 5, 6 ] ]
Instead of the actual output:
[ [ 1, null, 2 ], [ 3, null, 4 ], [ 5, null, 6 ] ]
How can I change my example to filter null values from the nested arrays?
var array = [ 0, 1, null, 2, "", 3, undefined, 3,,,,,, 4,, 4,, 5,, 6,,,, ];
var filtered = array.filter(function (el) {
return el != null;
});
console.log(filtered);
var array = [ 0, 1, null, 2, "", 3, undefined, 3,,,,,, 4,, 4,, 5,, 6,,,, ];
var filtered = array.filter(function (el) {
return el != null;
});
console.log(filtered);
var array = [ [ 1, null, 2 ], [ 3, null, 4 ], [ 5, null, 6 ] ];
var filtered = array.filter(function (el) {
return el != null;
});
console.log(filtered);
var array = [ [ 1, null, 2 ], [ 3, null, 4 ], [ 5, null, 6 ] ];
var filtered = array.filter(function (el) {
return el != null;
});
console.log(filtered);
javascript arrays
javascript arrays
edited Nov 22 '18 at 19:48


Tiny Giant
13.3k64056
13.3k64056
asked Nov 22 '18 at 18:57
JoomsJooms
417
417
why index 2? what happens to index 1 of the inner arrays for the result? what is the reason for the nested arrays?
– Nina Scholz
Nov 22 '18 at 18:59
Maybe look to stackoverflow.com/a/38132582/6523409. Filter should be called recursively.
– Filip Š
Nov 22 '18 at 19:01
@Nina Scholz, I have a long array with thouthands of rows with null elements inside. I'd like to make it work with every null element, not only for index 1.
– Jooms
Nov 22 '18 at 19:05
i mean, how do you come from the given array with falsy values to the result?
– Nina Scholz
Nov 22 '18 at 19:06
I've edited your question to hopefully clarify for everyone. If I have deviated too far from your original intent, please feel free to roll back my edit via the revisions page
– Tiny Giant
Nov 22 '18 at 19:47
add a comment |
why index 2? what happens to index 1 of the inner arrays for the result? what is the reason for the nested arrays?
– Nina Scholz
Nov 22 '18 at 18:59
Maybe look to stackoverflow.com/a/38132582/6523409. Filter should be called recursively.
– Filip Š
Nov 22 '18 at 19:01
@Nina Scholz, I have a long array with thouthands of rows with null elements inside. I'd like to make it work with every null element, not only for index 1.
– Jooms
Nov 22 '18 at 19:05
i mean, how do you come from the given array with falsy values to the result?
– Nina Scholz
Nov 22 '18 at 19:06
I've edited your question to hopefully clarify for everyone. If I have deviated too far from your original intent, please feel free to roll back my edit via the revisions page
– Tiny Giant
Nov 22 '18 at 19:47
why index 2? what happens to index 1 of the inner arrays for the result? what is the reason for the nested arrays?
– Nina Scholz
Nov 22 '18 at 18:59
why index 2? what happens to index 1 of the inner arrays for the result? what is the reason for the nested arrays?
– Nina Scholz
Nov 22 '18 at 18:59
Maybe look to stackoverflow.com/a/38132582/6523409. Filter should be called recursively.
– Filip Š
Nov 22 '18 at 19:01
Maybe look to stackoverflow.com/a/38132582/6523409. Filter should be called recursively.
– Filip Š
Nov 22 '18 at 19:01
@Nina Scholz, I have a long array with thouthands of rows with null elements inside. I'd like to make it work with every null element, not only for index 1.
– Jooms
Nov 22 '18 at 19:05
@Nina Scholz, I have a long array with thouthands of rows with null elements inside. I'd like to make it work with every null element, not only for index 1.
– Jooms
Nov 22 '18 at 19:05
i mean, how do you come from the given array with falsy values to the result?
– Nina Scholz
Nov 22 '18 at 19:06
i mean, how do you come from the given array with falsy values to the result?
– Nina Scholz
Nov 22 '18 at 19:06
I've edited your question to hopefully clarify for everyone. If I have deviated too far from your original intent, please feel free to roll back my edit via the revisions page
– Tiny Giant
Nov 22 '18 at 19:47
I've edited your question to hopefully clarify for everyone. If I have deviated too far from your original intent, please feel free to roll back my edit via the revisions page
– Tiny Giant
Nov 22 '18 at 19:47
add a comment |
5 Answers
5
active
oldest
votes
If your array-of-arrays only has one level, then you can just map
it like this:
var filtered = array.map(subarray => subarray.filter(el => el != null));
console.log(filtered);
Good work. This is the only answer that need be considered. This problem is too simple to overengineer with reduce, etc.
– Ben Steward
Nov 22 '18 at 19:42
Pedro LM, thank you.
– Jooms
Nov 22 '18 at 20:04
add a comment |
You're examples remove undefined
values as well as null
values, and your expected output reflects that, so I'm going to assume that you mean you want to recursively remove both undefined
and null
values. Your example uses a loose equality comparison which means that it will match both null
and undefined
. While this works, it is much better to be explicit about what you're checking for with strict equality comparison using ===
.
You're going to need to use recursion:
Recursion
An act of a function calling itself. Recursion is used to solve problems that contain smaller sub-problems.
- https://developer.mozilla.org/en-US/docs/Glossary/Recursion
This also means that you're going to want to use Array#reduce
instead of Array#filter
. Use a new array as the accumulator.
Then for each element in the input array where the element is not null or undefined:
- if the element is an instance of Array, push the result of calling this function on the element onto the accumulator array,
- otherwise push the element onto the accumulator array
Return the accumulator array at the end of the reduce callback as the accumulator
const input = [ [ 1, null, 2 ], null,,,,, [ 3, null, 4 ],,,,, [ 5, null, 6 ],,,,, [ 7, [ 8, undefined, 9 ], 10 ] ]
function recursiveValues(input) {
if(!(input instanceof Array)) return null
return input.reduce((output, element) => {
if(element !== null && element !== undefined) {
if(element instanceof Array) {
output.push(recursiveValues(element))
} else {
output.push(element)
}
}
return output
}, )
}
const output = recursiveValues(input)
console.log(JSON.stringify(output))
add a comment |
var arraylist = [0, 1, null, 5];
var i = arraylist.length;
var j =0;
var newlist = ;
while(j < i){
if(arraylist[j] != null){
newlist.push(arraylist[j]);
}
j++;
}
console.log(newlist);
https://jsfiddle.net/L4nmtg75/
add a comment |
You need to recursively filter for null, like so:
function removeNull(array) {
return array
.filter(item => item !== null)
.map(item => Array.isArray(item) ? removeNull(item) : item);
}
This function takes an array, and recursively removes all instances of null.
First, I took your solution and wrapped it in a function so that it is able to be called.
Then, after the items are filtered, it's as simple as mapping over the remaining items, checking if each one is an array, and then for each one that is, calling removeNull on it.
EDIT: I had a typo in my code originally, but it should work now.
add a comment |
var filterFn = function(item) {
if (item instanceof Array) {
// do this if you want to remove empty arrays:
var items = item.splice(0).filter(filterFn);
var length = items.length;
Array.prototype.push.apply(item, items);
return length;
// if you want to keep empty arrays do this:
var items = item.splice(0);
Array.prototype.push.apply(item, items.filter(filterFn))
return true;
}
return item != null;
};
array = array.filter(filterFn);
This will also work on more than 2 level, as it's recursive.
Frane Poljak, thank you. But it returns an empty array playcode.io/156503?tabs=console&script.js&output
– Jooms
Nov 22 '18 at 19:10
You have to choose between two options in the if (...) block, and remove another one.
– Frane Poljak
Nov 22 '18 at 19:12
Ah, I see, the .length here doesn't work.
– Frane Poljak
Nov 22 '18 at 19:17
I've updated an answer.
– Frane Poljak
Nov 22 '18 at 19:18
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53436773%2fhow-to-remove-null-values-from-nested-arrays%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
If your array-of-arrays only has one level, then you can just map
it like this:
var filtered = array.map(subarray => subarray.filter(el => el != null));
console.log(filtered);
Good work. This is the only answer that need be considered. This problem is too simple to overengineer with reduce, etc.
– Ben Steward
Nov 22 '18 at 19:42
Pedro LM, thank you.
– Jooms
Nov 22 '18 at 20:04
add a comment |
If your array-of-arrays only has one level, then you can just map
it like this:
var filtered = array.map(subarray => subarray.filter(el => el != null));
console.log(filtered);
Good work. This is the only answer that need be considered. This problem is too simple to overengineer with reduce, etc.
– Ben Steward
Nov 22 '18 at 19:42
Pedro LM, thank you.
– Jooms
Nov 22 '18 at 20:04
add a comment |
If your array-of-arrays only has one level, then you can just map
it like this:
var filtered = array.map(subarray => subarray.filter(el => el != null));
console.log(filtered);
If your array-of-arrays only has one level, then you can just map
it like this:
var filtered = array.map(subarray => subarray.filter(el => el != null));
console.log(filtered);
answered Nov 22 '18 at 19:04
Pedro LMPedro LM
45727
45727
Good work. This is the only answer that need be considered. This problem is too simple to overengineer with reduce, etc.
– Ben Steward
Nov 22 '18 at 19:42
Pedro LM, thank you.
– Jooms
Nov 22 '18 at 20:04
add a comment |
Good work. This is the only answer that need be considered. This problem is too simple to overengineer with reduce, etc.
– Ben Steward
Nov 22 '18 at 19:42
Pedro LM, thank you.
– Jooms
Nov 22 '18 at 20:04
Good work. This is the only answer that need be considered. This problem is too simple to overengineer with reduce, etc.
– Ben Steward
Nov 22 '18 at 19:42
Good work. This is the only answer that need be considered. This problem is too simple to overengineer with reduce, etc.
– Ben Steward
Nov 22 '18 at 19:42
Pedro LM, thank you.
– Jooms
Nov 22 '18 at 20:04
Pedro LM, thank you.
– Jooms
Nov 22 '18 at 20:04
add a comment |
You're examples remove undefined
values as well as null
values, and your expected output reflects that, so I'm going to assume that you mean you want to recursively remove both undefined
and null
values. Your example uses a loose equality comparison which means that it will match both null
and undefined
. While this works, it is much better to be explicit about what you're checking for with strict equality comparison using ===
.
You're going to need to use recursion:
Recursion
An act of a function calling itself. Recursion is used to solve problems that contain smaller sub-problems.
- https://developer.mozilla.org/en-US/docs/Glossary/Recursion
This also means that you're going to want to use Array#reduce
instead of Array#filter
. Use a new array as the accumulator.
Then for each element in the input array where the element is not null or undefined:
- if the element is an instance of Array, push the result of calling this function on the element onto the accumulator array,
- otherwise push the element onto the accumulator array
Return the accumulator array at the end of the reduce callback as the accumulator
const input = [ [ 1, null, 2 ], null,,,,, [ 3, null, 4 ],,,,, [ 5, null, 6 ],,,,, [ 7, [ 8, undefined, 9 ], 10 ] ]
function recursiveValues(input) {
if(!(input instanceof Array)) return null
return input.reduce((output, element) => {
if(element !== null && element !== undefined) {
if(element instanceof Array) {
output.push(recursiveValues(element))
} else {
output.push(element)
}
}
return output
}, )
}
const output = recursiveValues(input)
console.log(JSON.stringify(output))
add a comment |
You're examples remove undefined
values as well as null
values, and your expected output reflects that, so I'm going to assume that you mean you want to recursively remove both undefined
and null
values. Your example uses a loose equality comparison which means that it will match both null
and undefined
. While this works, it is much better to be explicit about what you're checking for with strict equality comparison using ===
.
You're going to need to use recursion:
Recursion
An act of a function calling itself. Recursion is used to solve problems that contain smaller sub-problems.
- https://developer.mozilla.org/en-US/docs/Glossary/Recursion
This also means that you're going to want to use Array#reduce
instead of Array#filter
. Use a new array as the accumulator.
Then for each element in the input array where the element is not null or undefined:
- if the element is an instance of Array, push the result of calling this function on the element onto the accumulator array,
- otherwise push the element onto the accumulator array
Return the accumulator array at the end of the reduce callback as the accumulator
const input = [ [ 1, null, 2 ], null,,,,, [ 3, null, 4 ],,,,, [ 5, null, 6 ],,,,, [ 7, [ 8, undefined, 9 ], 10 ] ]
function recursiveValues(input) {
if(!(input instanceof Array)) return null
return input.reduce((output, element) => {
if(element !== null && element !== undefined) {
if(element instanceof Array) {
output.push(recursiveValues(element))
} else {
output.push(element)
}
}
return output
}, )
}
const output = recursiveValues(input)
console.log(JSON.stringify(output))
add a comment |
You're examples remove undefined
values as well as null
values, and your expected output reflects that, so I'm going to assume that you mean you want to recursively remove both undefined
and null
values. Your example uses a loose equality comparison which means that it will match both null
and undefined
. While this works, it is much better to be explicit about what you're checking for with strict equality comparison using ===
.
You're going to need to use recursion:
Recursion
An act of a function calling itself. Recursion is used to solve problems that contain smaller sub-problems.
- https://developer.mozilla.org/en-US/docs/Glossary/Recursion
This also means that you're going to want to use Array#reduce
instead of Array#filter
. Use a new array as the accumulator.
Then for each element in the input array where the element is not null or undefined:
- if the element is an instance of Array, push the result of calling this function on the element onto the accumulator array,
- otherwise push the element onto the accumulator array
Return the accumulator array at the end of the reduce callback as the accumulator
const input = [ [ 1, null, 2 ], null,,,,, [ 3, null, 4 ],,,,, [ 5, null, 6 ],,,,, [ 7, [ 8, undefined, 9 ], 10 ] ]
function recursiveValues(input) {
if(!(input instanceof Array)) return null
return input.reduce((output, element) => {
if(element !== null && element !== undefined) {
if(element instanceof Array) {
output.push(recursiveValues(element))
} else {
output.push(element)
}
}
return output
}, )
}
const output = recursiveValues(input)
console.log(JSON.stringify(output))
You're examples remove undefined
values as well as null
values, and your expected output reflects that, so I'm going to assume that you mean you want to recursively remove both undefined
and null
values. Your example uses a loose equality comparison which means that it will match both null
and undefined
. While this works, it is much better to be explicit about what you're checking for with strict equality comparison using ===
.
You're going to need to use recursion:
Recursion
An act of a function calling itself. Recursion is used to solve problems that contain smaller sub-problems.
- https://developer.mozilla.org/en-US/docs/Glossary/Recursion
This also means that you're going to want to use Array#reduce
instead of Array#filter
. Use a new array as the accumulator.
Then for each element in the input array where the element is not null or undefined:
- if the element is an instance of Array, push the result of calling this function on the element onto the accumulator array,
- otherwise push the element onto the accumulator array
Return the accumulator array at the end of the reduce callback as the accumulator
const input = [ [ 1, null, 2 ], null,,,,, [ 3, null, 4 ],,,,, [ 5, null, 6 ],,,,, [ 7, [ 8, undefined, 9 ], 10 ] ]
function recursiveValues(input) {
if(!(input instanceof Array)) return null
return input.reduce((output, element) => {
if(element !== null && element !== undefined) {
if(element instanceof Array) {
output.push(recursiveValues(element))
} else {
output.push(element)
}
}
return output
}, )
}
const output = recursiveValues(input)
console.log(JSON.stringify(output))
const input = [ [ 1, null, 2 ], null,,,,, [ 3, null, 4 ],,,,, [ 5, null, 6 ],,,,, [ 7, [ 8, undefined, 9 ], 10 ] ]
function recursiveValues(input) {
if(!(input instanceof Array)) return null
return input.reduce((output, element) => {
if(element !== null && element !== undefined) {
if(element instanceof Array) {
output.push(recursiveValues(element))
} else {
output.push(element)
}
}
return output
}, )
}
const output = recursiveValues(input)
console.log(JSON.stringify(output))
const input = [ [ 1, null, 2 ], null,,,,, [ 3, null, 4 ],,,,, [ 5, null, 6 ],,,,, [ 7, [ 8, undefined, 9 ], 10 ] ]
function recursiveValues(input) {
if(!(input instanceof Array)) return null
return input.reduce((output, element) => {
if(element !== null && element !== undefined) {
if(element instanceof Array) {
output.push(recursiveValues(element))
} else {
output.push(element)
}
}
return output
}, )
}
const output = recursiveValues(input)
console.log(JSON.stringify(output))
answered Nov 22 '18 at 19:33


Tiny GiantTiny Giant
13.3k64056
13.3k64056
add a comment |
add a comment |
var arraylist = [0, 1, null, 5];
var i = arraylist.length;
var j =0;
var newlist = ;
while(j < i){
if(arraylist[j] != null){
newlist.push(arraylist[j]);
}
j++;
}
console.log(newlist);
https://jsfiddle.net/L4nmtg75/
add a comment |
var arraylist = [0, 1, null, 5];
var i = arraylist.length;
var j =0;
var newlist = ;
while(j < i){
if(arraylist[j] != null){
newlist.push(arraylist[j]);
}
j++;
}
console.log(newlist);
https://jsfiddle.net/L4nmtg75/
add a comment |
var arraylist = [0, 1, null, 5];
var i = arraylist.length;
var j =0;
var newlist = ;
while(j < i){
if(arraylist[j] != null){
newlist.push(arraylist[j]);
}
j++;
}
console.log(newlist);
https://jsfiddle.net/L4nmtg75/
var arraylist = [0, 1, null, 5];
var i = arraylist.length;
var j =0;
var newlist = ;
while(j < i){
if(arraylist[j] != null){
newlist.push(arraylist[j]);
}
j++;
}
console.log(newlist);
https://jsfiddle.net/L4nmtg75/
answered Nov 22 '18 at 19:04


Banujan BalendrakumarBanujan Balendrakumar
9011212
9011212
add a comment |
add a comment |
You need to recursively filter for null, like so:
function removeNull(array) {
return array
.filter(item => item !== null)
.map(item => Array.isArray(item) ? removeNull(item) : item);
}
This function takes an array, and recursively removes all instances of null.
First, I took your solution and wrapped it in a function so that it is able to be called.
Then, after the items are filtered, it's as simple as mapping over the remaining items, checking if each one is an array, and then for each one that is, calling removeNull on it.
EDIT: I had a typo in my code originally, but it should work now.
add a comment |
You need to recursively filter for null, like so:
function removeNull(array) {
return array
.filter(item => item !== null)
.map(item => Array.isArray(item) ? removeNull(item) : item);
}
This function takes an array, and recursively removes all instances of null.
First, I took your solution and wrapped it in a function so that it is able to be called.
Then, after the items are filtered, it's as simple as mapping over the remaining items, checking if each one is an array, and then for each one that is, calling removeNull on it.
EDIT: I had a typo in my code originally, but it should work now.
add a comment |
You need to recursively filter for null, like so:
function removeNull(array) {
return array
.filter(item => item !== null)
.map(item => Array.isArray(item) ? removeNull(item) : item);
}
This function takes an array, and recursively removes all instances of null.
First, I took your solution and wrapped it in a function so that it is able to be called.
Then, after the items are filtered, it's as simple as mapping over the remaining items, checking if each one is an array, and then for each one that is, calling removeNull on it.
EDIT: I had a typo in my code originally, but it should work now.
You need to recursively filter for null, like so:
function removeNull(array) {
return array
.filter(item => item !== null)
.map(item => Array.isArray(item) ? removeNull(item) : item);
}
This function takes an array, and recursively removes all instances of null.
First, I took your solution and wrapped it in a function so that it is able to be called.
Then, after the items are filtered, it's as simple as mapping over the remaining items, checking if each one is an array, and then for each one that is, calling removeNull on it.
EDIT: I had a typo in my code originally, but it should work now.
edited Nov 22 '18 at 19:16
answered Nov 22 '18 at 19:08
neonfuzneonfuz
1694
1694
add a comment |
add a comment |
var filterFn = function(item) {
if (item instanceof Array) {
// do this if you want to remove empty arrays:
var items = item.splice(0).filter(filterFn);
var length = items.length;
Array.prototype.push.apply(item, items);
return length;
// if you want to keep empty arrays do this:
var items = item.splice(0);
Array.prototype.push.apply(item, items.filter(filterFn))
return true;
}
return item != null;
};
array = array.filter(filterFn);
This will also work on more than 2 level, as it's recursive.
Frane Poljak, thank you. But it returns an empty array playcode.io/156503?tabs=console&script.js&output
– Jooms
Nov 22 '18 at 19:10
You have to choose between two options in the if (...) block, and remove another one.
– Frane Poljak
Nov 22 '18 at 19:12
Ah, I see, the .length here doesn't work.
– Frane Poljak
Nov 22 '18 at 19:17
I've updated an answer.
– Frane Poljak
Nov 22 '18 at 19:18
add a comment |
var filterFn = function(item) {
if (item instanceof Array) {
// do this if you want to remove empty arrays:
var items = item.splice(0).filter(filterFn);
var length = items.length;
Array.prototype.push.apply(item, items);
return length;
// if you want to keep empty arrays do this:
var items = item.splice(0);
Array.prototype.push.apply(item, items.filter(filterFn))
return true;
}
return item != null;
};
array = array.filter(filterFn);
This will also work on more than 2 level, as it's recursive.
Frane Poljak, thank you. But it returns an empty array playcode.io/156503?tabs=console&script.js&output
– Jooms
Nov 22 '18 at 19:10
You have to choose between two options in the if (...) block, and remove another one.
– Frane Poljak
Nov 22 '18 at 19:12
Ah, I see, the .length here doesn't work.
– Frane Poljak
Nov 22 '18 at 19:17
I've updated an answer.
– Frane Poljak
Nov 22 '18 at 19:18
add a comment |
var filterFn = function(item) {
if (item instanceof Array) {
// do this if you want to remove empty arrays:
var items = item.splice(0).filter(filterFn);
var length = items.length;
Array.prototype.push.apply(item, items);
return length;
// if you want to keep empty arrays do this:
var items = item.splice(0);
Array.prototype.push.apply(item, items.filter(filterFn))
return true;
}
return item != null;
};
array = array.filter(filterFn);
This will also work on more than 2 level, as it's recursive.
var filterFn = function(item) {
if (item instanceof Array) {
// do this if you want to remove empty arrays:
var items = item.splice(0).filter(filterFn);
var length = items.length;
Array.prototype.push.apply(item, items);
return length;
// if you want to keep empty arrays do this:
var items = item.splice(0);
Array.prototype.push.apply(item, items.filter(filterFn))
return true;
}
return item != null;
};
array = array.filter(filterFn);
This will also work on more than 2 level, as it's recursive.
edited Nov 22 '18 at 19:18
answered Nov 22 '18 at 18:58
Frane PoljakFrane Poljak
1,7181519
1,7181519
Frane Poljak, thank you. But it returns an empty array playcode.io/156503?tabs=console&script.js&output
– Jooms
Nov 22 '18 at 19:10
You have to choose between two options in the if (...) block, and remove another one.
– Frane Poljak
Nov 22 '18 at 19:12
Ah, I see, the .length here doesn't work.
– Frane Poljak
Nov 22 '18 at 19:17
I've updated an answer.
– Frane Poljak
Nov 22 '18 at 19:18
add a comment |
Frane Poljak, thank you. But it returns an empty array playcode.io/156503?tabs=console&script.js&output
– Jooms
Nov 22 '18 at 19:10
You have to choose between two options in the if (...) block, and remove another one.
– Frane Poljak
Nov 22 '18 at 19:12
Ah, I see, the .length here doesn't work.
– Frane Poljak
Nov 22 '18 at 19:17
I've updated an answer.
– Frane Poljak
Nov 22 '18 at 19:18
Frane Poljak, thank you. But it returns an empty array playcode.io/156503?tabs=console&script.js&output
– Jooms
Nov 22 '18 at 19:10
Frane Poljak, thank you. But it returns an empty array playcode.io/156503?tabs=console&script.js&output
– Jooms
Nov 22 '18 at 19:10
You have to choose between two options in the if (...) block, and remove another one.
– Frane Poljak
Nov 22 '18 at 19:12
You have to choose between two options in the if (...) block, and remove another one.
– Frane Poljak
Nov 22 '18 at 19:12
Ah, I see, the .length here doesn't work.
– Frane Poljak
Nov 22 '18 at 19:17
Ah, I see, the .length here doesn't work.
– Frane Poljak
Nov 22 '18 at 19:17
I've updated an answer.
– Frane Poljak
Nov 22 '18 at 19:18
I've updated an answer.
– Frane Poljak
Nov 22 '18 at 19:18
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53436773%2fhow-to-remove-null-values-from-nested-arrays%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
pCd8T,qUn8nPHBTSM8Rw
why index 2? what happens to index 1 of the inner arrays for the result? what is the reason for the nested arrays?
– Nina Scholz
Nov 22 '18 at 18:59
Maybe look to stackoverflow.com/a/38132582/6523409. Filter should be called recursively.
– Filip Š
Nov 22 '18 at 19:01
@Nina Scholz, I have a long array with thouthands of rows with null elements inside. I'd like to make it work with every null element, not only for index 1.
– Jooms
Nov 22 '18 at 19:05
i mean, how do you come from the given array with falsy values to the result?
– Nina Scholz
Nov 22 '18 at 19:06
I've edited your question to hopefully clarify for everyone. If I have deviated too far from your original intent, please feel free to roll back my edit via the revisions page
– Tiny Giant
Nov 22 '18 at 19:47