JavaScript isPrototypeOf vs instanceof usage
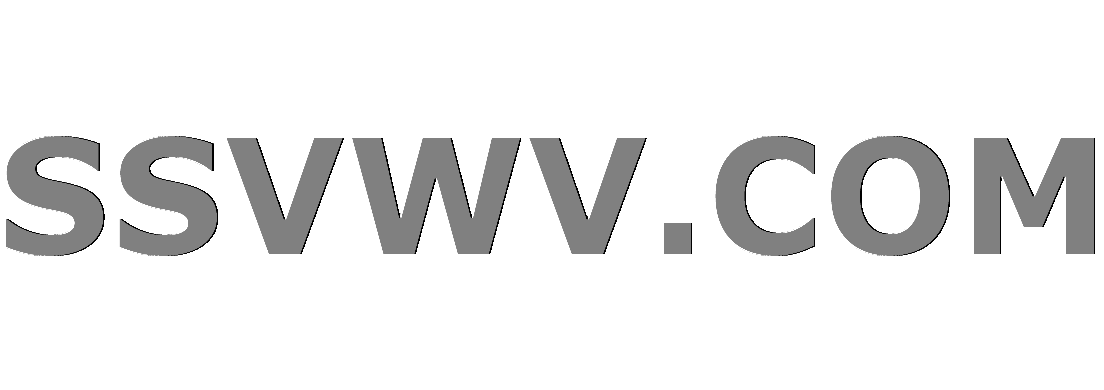
Multi tool use
Suppose we have the following:
function Super() {
// init code
}
function Sub() {
Super.call(this);
// other init code
}
Sub.prototype = new Super();
var sub = new Sub();
Then, in some other part of our ocde, we can use either of the following to check for the relationship:
sub instanceof Super;
or
Super.prototype.isPrototypeOf( sub )
Either way, we need to have both the object (sub), and the parent constructor (Super). So, is there any reason why you'd use one vs the other? Is there some other situation where the distinction is more clear?
I've already carefully read 2464426, but didn't find a specific enough answer.
javascript
add a comment |
Suppose we have the following:
function Super() {
// init code
}
function Sub() {
Super.call(this);
// other init code
}
Sub.prototype = new Super();
var sub = new Sub();
Then, in some other part of our ocde, we can use either of the following to check for the relationship:
sub instanceof Super;
or
Super.prototype.isPrototypeOf( sub )
Either way, we need to have both the object (sub), and the parent constructor (Super). So, is there any reason why you'd use one vs the other? Is there some other situation where the distinction is more clear?
I've already carefully read 2464426, but didn't find a specific enough answer.
javascript
possible duplicate of Why do we need the isPrototypeOf at all?
– plalx
Aug 20 '13 at 19:54
Based on the OP's comment, I'd say this question is closer to "Why do we needinstanceof
at all?" but I agree that it's probably close enough.
– apsillers
Aug 20 '13 at 20:22
add a comment |
Suppose we have the following:
function Super() {
// init code
}
function Sub() {
Super.call(this);
// other init code
}
Sub.prototype = new Super();
var sub = new Sub();
Then, in some other part of our ocde, we can use either of the following to check for the relationship:
sub instanceof Super;
or
Super.prototype.isPrototypeOf( sub )
Either way, we need to have both the object (sub), and the parent constructor (Super). So, is there any reason why you'd use one vs the other? Is there some other situation where the distinction is more clear?
I've already carefully read 2464426, but didn't find a specific enough answer.
javascript
Suppose we have the following:
function Super() {
// init code
}
function Sub() {
Super.call(this);
// other init code
}
Sub.prototype = new Super();
var sub = new Sub();
Then, in some other part of our ocde, we can use either of the following to check for the relationship:
sub instanceof Super;
or
Super.prototype.isPrototypeOf( sub )
Either way, we need to have both the object (sub), and the parent constructor (Super). So, is there any reason why you'd use one vs the other? Is there some other situation where the distinction is more clear?
I've already carefully read 2464426, but didn't find a specific enough answer.
javascript
javascript
edited May 23 '17 at 12:25
Community♦
11
11
asked Aug 20 '13 at 19:33
user1689498user1689498
163125
163125
possible duplicate of Why do we need the isPrototypeOf at all?
– plalx
Aug 20 '13 at 19:54
Based on the OP's comment, I'd say this question is closer to "Why do we needinstanceof
at all?" but I agree that it's probably close enough.
– apsillers
Aug 20 '13 at 20:22
add a comment |
possible duplicate of Why do we need the isPrototypeOf at all?
– plalx
Aug 20 '13 at 19:54
Based on the OP's comment, I'd say this question is closer to "Why do we needinstanceof
at all?" but I agree that it's probably close enough.
– apsillers
Aug 20 '13 at 20:22
possible duplicate of Why do we need the isPrototypeOf at all?
– plalx
Aug 20 '13 at 19:54
possible duplicate of Why do we need the isPrototypeOf at all?
– plalx
Aug 20 '13 at 19:54
Based on the OP's comment, I'd say this question is closer to "Why do we need
instanceof
at all?" but I agree that it's probably close enough.– apsillers
Aug 20 '13 at 20:22
Based on the OP's comment, I'd say this question is closer to "Why do we need
instanceof
at all?" but I agree that it's probably close enough.– apsillers
Aug 20 '13 at 20:22
add a comment |
5 Answers
5
active
oldest
votes
Imagine you don't use constructors in your code, but instead use Object.create
to generate objects with a particular prototype. Your program might be architected to use no constructors at all:
var superProto = {
// some super properties
}
var subProto = Object.create(superProto);
subProto.someProp = 5;
var sub = Object.create(subProto);
console.log(superProto.isPrototypeOf(sub)); // true
console.log(sub instanceof superProto); // TypeError
Here, you don't have a constructor function to use with instanceof
. You can only use subProto.isPrototypeOf(sub)
.
2
Thanks for the answer, and I have one quick follow up: Why do we need instanceof, since isPrototypeOf would seem to work in all cases? Is it historical or are there performance advantages, or something else?
– user1689498
Aug 20 '13 at 19:48
@user1689498 Simply becauseisPrototypeOf
has been implemented later.
– plalx
Aug 20 '13 at 19:51
2
@user1689498:instanceof
is older, I believe, and looks similar to Java, which was a big deal when JavaScript was first introduced. It also is a little cleaner. But yes,isPrototypeOf
is the more generally useful one.
– Scott Sauyet
Aug 20 '13 at 19:52
add a comment |
It makes little difference when you use constructor functions. instanceof
is a little cleaner, perhaps. But when you don't...:
var human = {mortal: true}
var socrates = Object.create(human);
human.isPrototypeOf(socrates); //=> true
socrates instanceof human; //=> ERROR!
So isPrototypeOf
is more general.
1
Great! :) But one thing. «human» in this example should be a «Human». I mean it should be a class (All people), not a single instance (a human).
– Naeel Maqsudov
Jun 4 '14 at 4:37
@NaeelMaqsudov: Beware analogies between languages. Javascript has no classes. Even in when the travesty ofclass
syntactic sugar is added in ES6, the language will still not have something really like, say, Java classes. Prototypal inheritance has some parallels with classical inheritance, but the analogies are rough ones. In any way of doing inheritance, there will be an object such as this --- perhaps the prototype of a constructor function. But note:var naeel = Object.create(human); naeel instanceof human; //=> true
. This strips away some of the sugar of constructor functions.
– Scott Sauyet
Jun 4 '14 at 13:00
Sorry, bad cut-and-paste error.naeel instanceof human; //=> ERROR.
Buthuman.prototypOf(naeel); //=> true
.
– Scott Sauyet
Jun 4 '14 at 14:20
Wow. I think I need some caffeine.isPrototypeOf
.
– Scott Sauyet
Jun 4 '14 at 17:20
1
@NaeelMaqsudov: Most importantly, note that although historically constructor functions predatedObject.create
, it's the latter which is the more fundamental construct. The constructor function could be easily built on top ofObject.create
, which is the fundamental behavior, andinstanceOf
could be built on top ofisPrototypeOf
, but the reverse of these are not true, or at least have no obvious solutions. Using this mechanism, you can just as easily claim that all such constructed object are 'human' objects as you can with the constructor function.
– Scott Sauyet
Jun 4 '14 at 20:47
add a comment |
var neuesArray = Object.create(Array);
Array.isPrototypeOf(neuesArray); // true
neuesArray instanceof Array // false
neuesArray instanceof Object // true
Array.isArray(neuesArray); // false
Array.prototype.isPrototypeOf(neuesArray); // false
Object.prototype.isPrototypeOf(neuesArray); // true
Do you understand my friend :) - is simple
I'm not sure what you're trying to convey and how it's relevant to the problem. Can you add explanation to this?
– Unihedron
Sep 21 '14 at 11:19
the difference by "instanceof" - Operator and the Object.isPrototypeOf() - methode - or?
– svenskanda
Sep 21 '14 at 11:29
add a comment |
According to this MDN Ref:
isPrototypeOf()
differs from theinstanceof
operator. In the expressionobject instanceof AFunction
, the object prototype chain is checked againstAFunction.prototype
, not againstAFunction
itself.
add a comment |
Just complement @apsillers's answer
object instanceof constructor
var superProto = {}
// subProto.__proto__.__proto__ === superProto
var subProto = Object.create(superProto);
subProto.someProp = 5;
// sub.__proto__.__proto__ === subProto
var sub = Object.create(subProto);
console.log(superProto.isPrototypeOf(sub)); // true
console.log(sub instanceof superProto); // TypeError: Right-hand side of 'instanceof' is not callable
// helper utility to see if `o1` is
// related to (delegates to) `o2`
function isRelatedTo(o1, o2) {
function F(){}
F.prototype = o2;
// ensure the right-hand side of 'instanceof' is callable
return o1 instanceof F;
}
isRelatedTo( b, a );
TypeError: Right-hand side of 'instanceof' is not callable
instanceof
need the right-hand value to be callable, which means it must be a function(MDN call it as the constructor)
and instanceof
tests the presence of constructor.prototype
in object's prototype chain.
but isPrototypeOf()
don't have such limit. While instanceof
checks superProto.prototype
, isPrototypeOf()
checks superProto
directly.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f18343545%2fjavascript-isprototypeof-vs-instanceof-usage%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
Imagine you don't use constructors in your code, but instead use Object.create
to generate objects with a particular prototype. Your program might be architected to use no constructors at all:
var superProto = {
// some super properties
}
var subProto = Object.create(superProto);
subProto.someProp = 5;
var sub = Object.create(subProto);
console.log(superProto.isPrototypeOf(sub)); // true
console.log(sub instanceof superProto); // TypeError
Here, you don't have a constructor function to use with instanceof
. You can only use subProto.isPrototypeOf(sub)
.
2
Thanks for the answer, and I have one quick follow up: Why do we need instanceof, since isPrototypeOf would seem to work in all cases? Is it historical or are there performance advantages, or something else?
– user1689498
Aug 20 '13 at 19:48
@user1689498 Simply becauseisPrototypeOf
has been implemented later.
– plalx
Aug 20 '13 at 19:51
2
@user1689498:instanceof
is older, I believe, and looks similar to Java, which was a big deal when JavaScript was first introduced. It also is a little cleaner. But yes,isPrototypeOf
is the more generally useful one.
– Scott Sauyet
Aug 20 '13 at 19:52
add a comment |
Imagine you don't use constructors in your code, but instead use Object.create
to generate objects with a particular prototype. Your program might be architected to use no constructors at all:
var superProto = {
// some super properties
}
var subProto = Object.create(superProto);
subProto.someProp = 5;
var sub = Object.create(subProto);
console.log(superProto.isPrototypeOf(sub)); // true
console.log(sub instanceof superProto); // TypeError
Here, you don't have a constructor function to use with instanceof
. You can only use subProto.isPrototypeOf(sub)
.
2
Thanks for the answer, and I have one quick follow up: Why do we need instanceof, since isPrototypeOf would seem to work in all cases? Is it historical or are there performance advantages, or something else?
– user1689498
Aug 20 '13 at 19:48
@user1689498 Simply becauseisPrototypeOf
has been implemented later.
– plalx
Aug 20 '13 at 19:51
2
@user1689498:instanceof
is older, I believe, and looks similar to Java, which was a big deal when JavaScript was first introduced. It also is a little cleaner. But yes,isPrototypeOf
is the more generally useful one.
– Scott Sauyet
Aug 20 '13 at 19:52
add a comment |
Imagine you don't use constructors in your code, but instead use Object.create
to generate objects with a particular prototype. Your program might be architected to use no constructors at all:
var superProto = {
// some super properties
}
var subProto = Object.create(superProto);
subProto.someProp = 5;
var sub = Object.create(subProto);
console.log(superProto.isPrototypeOf(sub)); // true
console.log(sub instanceof superProto); // TypeError
Here, you don't have a constructor function to use with instanceof
. You can only use subProto.isPrototypeOf(sub)
.
Imagine you don't use constructors in your code, but instead use Object.create
to generate objects with a particular prototype. Your program might be architected to use no constructors at all:
var superProto = {
// some super properties
}
var subProto = Object.create(superProto);
subProto.someProp = 5;
var sub = Object.create(subProto);
console.log(superProto.isPrototypeOf(sub)); // true
console.log(sub instanceof superProto); // TypeError
Here, you don't have a constructor function to use with instanceof
. You can only use subProto.isPrototypeOf(sub)
.
edited Jun 2 '16 at 7:50
raaj
135
135
answered Aug 20 '13 at 19:41
apsillersapsillers
82k9161189
82k9161189
2
Thanks for the answer, and I have one quick follow up: Why do we need instanceof, since isPrototypeOf would seem to work in all cases? Is it historical or are there performance advantages, or something else?
– user1689498
Aug 20 '13 at 19:48
@user1689498 Simply becauseisPrototypeOf
has been implemented later.
– plalx
Aug 20 '13 at 19:51
2
@user1689498:instanceof
is older, I believe, and looks similar to Java, which was a big deal when JavaScript was first introduced. It also is a little cleaner. But yes,isPrototypeOf
is the more generally useful one.
– Scott Sauyet
Aug 20 '13 at 19:52
add a comment |
2
Thanks for the answer, and I have one quick follow up: Why do we need instanceof, since isPrototypeOf would seem to work in all cases? Is it historical or are there performance advantages, or something else?
– user1689498
Aug 20 '13 at 19:48
@user1689498 Simply becauseisPrototypeOf
has been implemented later.
– plalx
Aug 20 '13 at 19:51
2
@user1689498:instanceof
is older, I believe, and looks similar to Java, which was a big deal when JavaScript was first introduced. It also is a little cleaner. But yes,isPrototypeOf
is the more generally useful one.
– Scott Sauyet
Aug 20 '13 at 19:52
2
2
Thanks for the answer, and I have one quick follow up: Why do we need instanceof, since isPrototypeOf would seem to work in all cases? Is it historical or are there performance advantages, or something else?
– user1689498
Aug 20 '13 at 19:48
Thanks for the answer, and I have one quick follow up: Why do we need instanceof, since isPrototypeOf would seem to work in all cases? Is it historical or are there performance advantages, or something else?
– user1689498
Aug 20 '13 at 19:48
@user1689498 Simply because
isPrototypeOf
has been implemented later.– plalx
Aug 20 '13 at 19:51
@user1689498 Simply because
isPrototypeOf
has been implemented later.– plalx
Aug 20 '13 at 19:51
2
2
@user1689498:
instanceof
is older, I believe, and looks similar to Java, which was a big deal when JavaScript was first introduced. It also is a little cleaner. But yes, isPrototypeOf
is the more generally useful one.– Scott Sauyet
Aug 20 '13 at 19:52
@user1689498:
instanceof
is older, I believe, and looks similar to Java, which was a big deal when JavaScript was first introduced. It also is a little cleaner. But yes, isPrototypeOf
is the more generally useful one.– Scott Sauyet
Aug 20 '13 at 19:52
add a comment |
It makes little difference when you use constructor functions. instanceof
is a little cleaner, perhaps. But when you don't...:
var human = {mortal: true}
var socrates = Object.create(human);
human.isPrototypeOf(socrates); //=> true
socrates instanceof human; //=> ERROR!
So isPrototypeOf
is more general.
1
Great! :) But one thing. «human» in this example should be a «Human». I mean it should be a class (All people), not a single instance (a human).
– Naeel Maqsudov
Jun 4 '14 at 4:37
@NaeelMaqsudov: Beware analogies between languages. Javascript has no classes. Even in when the travesty ofclass
syntactic sugar is added in ES6, the language will still not have something really like, say, Java classes. Prototypal inheritance has some parallels with classical inheritance, but the analogies are rough ones. In any way of doing inheritance, there will be an object such as this --- perhaps the prototype of a constructor function. But note:var naeel = Object.create(human); naeel instanceof human; //=> true
. This strips away some of the sugar of constructor functions.
– Scott Sauyet
Jun 4 '14 at 13:00
Sorry, bad cut-and-paste error.naeel instanceof human; //=> ERROR.
Buthuman.prototypOf(naeel); //=> true
.
– Scott Sauyet
Jun 4 '14 at 14:20
Wow. I think I need some caffeine.isPrototypeOf
.
– Scott Sauyet
Jun 4 '14 at 17:20
1
@NaeelMaqsudov: Most importantly, note that although historically constructor functions predatedObject.create
, it's the latter which is the more fundamental construct. The constructor function could be easily built on top ofObject.create
, which is the fundamental behavior, andinstanceOf
could be built on top ofisPrototypeOf
, but the reverse of these are not true, or at least have no obvious solutions. Using this mechanism, you can just as easily claim that all such constructed object are 'human' objects as you can with the constructor function.
– Scott Sauyet
Jun 4 '14 at 20:47
add a comment |
It makes little difference when you use constructor functions. instanceof
is a little cleaner, perhaps. But when you don't...:
var human = {mortal: true}
var socrates = Object.create(human);
human.isPrototypeOf(socrates); //=> true
socrates instanceof human; //=> ERROR!
So isPrototypeOf
is more general.
1
Great! :) But one thing. «human» in this example should be a «Human». I mean it should be a class (All people), not a single instance (a human).
– Naeel Maqsudov
Jun 4 '14 at 4:37
@NaeelMaqsudov: Beware analogies between languages. Javascript has no classes. Even in when the travesty ofclass
syntactic sugar is added in ES6, the language will still not have something really like, say, Java classes. Prototypal inheritance has some parallels with classical inheritance, but the analogies are rough ones. In any way of doing inheritance, there will be an object such as this --- perhaps the prototype of a constructor function. But note:var naeel = Object.create(human); naeel instanceof human; //=> true
. This strips away some of the sugar of constructor functions.
– Scott Sauyet
Jun 4 '14 at 13:00
Sorry, bad cut-and-paste error.naeel instanceof human; //=> ERROR.
Buthuman.prototypOf(naeel); //=> true
.
– Scott Sauyet
Jun 4 '14 at 14:20
Wow. I think I need some caffeine.isPrototypeOf
.
– Scott Sauyet
Jun 4 '14 at 17:20
1
@NaeelMaqsudov: Most importantly, note that although historically constructor functions predatedObject.create
, it's the latter which is the more fundamental construct. The constructor function could be easily built on top ofObject.create
, which is the fundamental behavior, andinstanceOf
could be built on top ofisPrototypeOf
, but the reverse of these are not true, or at least have no obvious solutions. Using this mechanism, you can just as easily claim that all such constructed object are 'human' objects as you can with the constructor function.
– Scott Sauyet
Jun 4 '14 at 20:47
add a comment |
It makes little difference when you use constructor functions. instanceof
is a little cleaner, perhaps. But when you don't...:
var human = {mortal: true}
var socrates = Object.create(human);
human.isPrototypeOf(socrates); //=> true
socrates instanceof human; //=> ERROR!
So isPrototypeOf
is more general.
It makes little difference when you use constructor functions. instanceof
is a little cleaner, perhaps. But when you don't...:
var human = {mortal: true}
var socrates = Object.create(human);
human.isPrototypeOf(socrates); //=> true
socrates instanceof human; //=> ERROR!
So isPrototypeOf
is more general.
edited May 11 '15 at 12:16
answered Aug 20 '13 at 19:50
Scott SauyetScott Sauyet
20.3k22656
20.3k22656
1
Great! :) But one thing. «human» in this example should be a «Human». I mean it should be a class (All people), not a single instance (a human).
– Naeel Maqsudov
Jun 4 '14 at 4:37
@NaeelMaqsudov: Beware analogies between languages. Javascript has no classes. Even in when the travesty ofclass
syntactic sugar is added in ES6, the language will still not have something really like, say, Java classes. Prototypal inheritance has some parallels with classical inheritance, but the analogies are rough ones. In any way of doing inheritance, there will be an object such as this --- perhaps the prototype of a constructor function. But note:var naeel = Object.create(human); naeel instanceof human; //=> true
. This strips away some of the sugar of constructor functions.
– Scott Sauyet
Jun 4 '14 at 13:00
Sorry, bad cut-and-paste error.naeel instanceof human; //=> ERROR.
Buthuman.prototypOf(naeel); //=> true
.
– Scott Sauyet
Jun 4 '14 at 14:20
Wow. I think I need some caffeine.isPrototypeOf
.
– Scott Sauyet
Jun 4 '14 at 17:20
1
@NaeelMaqsudov: Most importantly, note that although historically constructor functions predatedObject.create
, it's the latter which is the more fundamental construct. The constructor function could be easily built on top ofObject.create
, which is the fundamental behavior, andinstanceOf
could be built on top ofisPrototypeOf
, but the reverse of these are not true, or at least have no obvious solutions. Using this mechanism, you can just as easily claim that all such constructed object are 'human' objects as you can with the constructor function.
– Scott Sauyet
Jun 4 '14 at 20:47
add a comment |
1
Great! :) But one thing. «human» in this example should be a «Human». I mean it should be a class (All people), not a single instance (a human).
– Naeel Maqsudov
Jun 4 '14 at 4:37
@NaeelMaqsudov: Beware analogies between languages. Javascript has no classes. Even in when the travesty ofclass
syntactic sugar is added in ES6, the language will still not have something really like, say, Java classes. Prototypal inheritance has some parallels with classical inheritance, but the analogies are rough ones. In any way of doing inheritance, there will be an object such as this --- perhaps the prototype of a constructor function. But note:var naeel = Object.create(human); naeel instanceof human; //=> true
. This strips away some of the sugar of constructor functions.
– Scott Sauyet
Jun 4 '14 at 13:00
Sorry, bad cut-and-paste error.naeel instanceof human; //=> ERROR.
Buthuman.prototypOf(naeel); //=> true
.
– Scott Sauyet
Jun 4 '14 at 14:20
Wow. I think I need some caffeine.isPrototypeOf
.
– Scott Sauyet
Jun 4 '14 at 17:20
1
@NaeelMaqsudov: Most importantly, note that although historically constructor functions predatedObject.create
, it's the latter which is the more fundamental construct. The constructor function could be easily built on top ofObject.create
, which is the fundamental behavior, andinstanceOf
could be built on top ofisPrototypeOf
, but the reverse of these are not true, or at least have no obvious solutions. Using this mechanism, you can just as easily claim that all such constructed object are 'human' objects as you can with the constructor function.
– Scott Sauyet
Jun 4 '14 at 20:47
1
1
Great! :) But one thing. «human» in this example should be a «Human». I mean it should be a class (All people), not a single instance (a human).
– Naeel Maqsudov
Jun 4 '14 at 4:37
Great! :) But one thing. «human» in this example should be a «Human». I mean it should be a class (All people), not a single instance (a human).
– Naeel Maqsudov
Jun 4 '14 at 4:37
@NaeelMaqsudov: Beware analogies between languages. Javascript has no classes. Even in when the travesty of
class
syntactic sugar is added in ES6, the language will still not have something really like, say, Java classes. Prototypal inheritance has some parallels with classical inheritance, but the analogies are rough ones. In any way of doing inheritance, there will be an object such as this --- perhaps the prototype of a constructor function. But note: var naeel = Object.create(human); naeel instanceof human; //=> true
. This strips away some of the sugar of constructor functions.– Scott Sauyet
Jun 4 '14 at 13:00
@NaeelMaqsudov: Beware analogies between languages. Javascript has no classes. Even in when the travesty of
class
syntactic sugar is added in ES6, the language will still not have something really like, say, Java classes. Prototypal inheritance has some parallels with classical inheritance, but the analogies are rough ones. In any way of doing inheritance, there will be an object such as this --- perhaps the prototype of a constructor function. But note: var naeel = Object.create(human); naeel instanceof human; //=> true
. This strips away some of the sugar of constructor functions.– Scott Sauyet
Jun 4 '14 at 13:00
Sorry, bad cut-and-paste error.
naeel instanceof human; //=> ERROR.
But human.prototypOf(naeel); //=> true
.– Scott Sauyet
Jun 4 '14 at 14:20
Sorry, bad cut-and-paste error.
naeel instanceof human; //=> ERROR.
But human.prototypOf(naeel); //=> true
.– Scott Sauyet
Jun 4 '14 at 14:20
Wow. I think I need some caffeine.
isPrototypeOf
.– Scott Sauyet
Jun 4 '14 at 17:20
Wow. I think I need some caffeine.
isPrototypeOf
.– Scott Sauyet
Jun 4 '14 at 17:20
1
1
@NaeelMaqsudov: Most importantly, note that although historically constructor functions predated
Object.create
, it's the latter which is the more fundamental construct. The constructor function could be easily built on top of Object.create
, which is the fundamental behavior, and instanceOf
could be built on top of isPrototypeOf
, but the reverse of these are not true, or at least have no obvious solutions. Using this mechanism, you can just as easily claim that all such constructed object are 'human' objects as you can with the constructor function.– Scott Sauyet
Jun 4 '14 at 20:47
@NaeelMaqsudov: Most importantly, note that although historically constructor functions predated
Object.create
, it's the latter which is the more fundamental construct. The constructor function could be easily built on top of Object.create
, which is the fundamental behavior, and instanceOf
could be built on top of isPrototypeOf
, but the reverse of these are not true, or at least have no obvious solutions. Using this mechanism, you can just as easily claim that all such constructed object are 'human' objects as you can with the constructor function.– Scott Sauyet
Jun 4 '14 at 20:47
add a comment |
var neuesArray = Object.create(Array);
Array.isPrototypeOf(neuesArray); // true
neuesArray instanceof Array // false
neuesArray instanceof Object // true
Array.isArray(neuesArray); // false
Array.prototype.isPrototypeOf(neuesArray); // false
Object.prototype.isPrototypeOf(neuesArray); // true
Do you understand my friend :) - is simple
I'm not sure what you're trying to convey and how it's relevant to the problem. Can you add explanation to this?
– Unihedron
Sep 21 '14 at 11:19
the difference by "instanceof" - Operator and the Object.isPrototypeOf() - methode - or?
– svenskanda
Sep 21 '14 at 11:29
add a comment |
var neuesArray = Object.create(Array);
Array.isPrototypeOf(neuesArray); // true
neuesArray instanceof Array // false
neuesArray instanceof Object // true
Array.isArray(neuesArray); // false
Array.prototype.isPrototypeOf(neuesArray); // false
Object.prototype.isPrototypeOf(neuesArray); // true
Do you understand my friend :) - is simple
I'm not sure what you're trying to convey and how it's relevant to the problem. Can you add explanation to this?
– Unihedron
Sep 21 '14 at 11:19
the difference by "instanceof" - Operator and the Object.isPrototypeOf() - methode - or?
– svenskanda
Sep 21 '14 at 11:29
add a comment |
var neuesArray = Object.create(Array);
Array.isPrototypeOf(neuesArray); // true
neuesArray instanceof Array // false
neuesArray instanceof Object // true
Array.isArray(neuesArray); // false
Array.prototype.isPrototypeOf(neuesArray); // false
Object.prototype.isPrototypeOf(neuesArray); // true
Do you understand my friend :) - is simple
var neuesArray = Object.create(Array);
Array.isPrototypeOf(neuesArray); // true
neuesArray instanceof Array // false
neuesArray instanceof Object // true
Array.isArray(neuesArray); // false
Array.prototype.isPrototypeOf(neuesArray); // false
Object.prototype.isPrototypeOf(neuesArray); // true
Do you understand my friend :) - is simple
edited Oct 18 '16 at 6:02


ozanmuyes
4851020
4851020
answered Sep 21 '14 at 11:17
svenskandasvenskanda
11
11
I'm not sure what you're trying to convey and how it's relevant to the problem. Can you add explanation to this?
– Unihedron
Sep 21 '14 at 11:19
the difference by "instanceof" - Operator and the Object.isPrototypeOf() - methode - or?
– svenskanda
Sep 21 '14 at 11:29
add a comment |
I'm not sure what you're trying to convey and how it's relevant to the problem. Can you add explanation to this?
– Unihedron
Sep 21 '14 at 11:19
the difference by "instanceof" - Operator and the Object.isPrototypeOf() - methode - or?
– svenskanda
Sep 21 '14 at 11:29
I'm not sure what you're trying to convey and how it's relevant to the problem. Can you add explanation to this?
– Unihedron
Sep 21 '14 at 11:19
I'm not sure what you're trying to convey and how it's relevant to the problem. Can you add explanation to this?
– Unihedron
Sep 21 '14 at 11:19
the difference by "instanceof" - Operator and the Object.isPrototypeOf() - methode - or?
– svenskanda
Sep 21 '14 at 11:29
the difference by "instanceof" - Operator and the Object.isPrototypeOf() - methode - or?
– svenskanda
Sep 21 '14 at 11:29
add a comment |
According to this MDN Ref:
isPrototypeOf()
differs from theinstanceof
operator. In the expressionobject instanceof AFunction
, the object prototype chain is checked againstAFunction.prototype
, not againstAFunction
itself.
add a comment |
According to this MDN Ref:
isPrototypeOf()
differs from theinstanceof
operator. In the expressionobject instanceof AFunction
, the object prototype chain is checked againstAFunction.prototype
, not againstAFunction
itself.
add a comment |
According to this MDN Ref:
isPrototypeOf()
differs from theinstanceof
operator. In the expressionobject instanceof AFunction
, the object prototype chain is checked againstAFunction.prototype
, not againstAFunction
itself.
According to this MDN Ref:
isPrototypeOf()
differs from theinstanceof
operator. In the expressionobject instanceof AFunction
, the object prototype chain is checked againstAFunction.prototype
, not againstAFunction
itself.
answered Oct 3 '18 at 5:21
S.SerpooshanS.Serpooshan
4,08821637
4,08821637
add a comment |
add a comment |
Just complement @apsillers's answer
object instanceof constructor
var superProto = {}
// subProto.__proto__.__proto__ === superProto
var subProto = Object.create(superProto);
subProto.someProp = 5;
// sub.__proto__.__proto__ === subProto
var sub = Object.create(subProto);
console.log(superProto.isPrototypeOf(sub)); // true
console.log(sub instanceof superProto); // TypeError: Right-hand side of 'instanceof' is not callable
// helper utility to see if `o1` is
// related to (delegates to) `o2`
function isRelatedTo(o1, o2) {
function F(){}
F.prototype = o2;
// ensure the right-hand side of 'instanceof' is callable
return o1 instanceof F;
}
isRelatedTo( b, a );
TypeError: Right-hand side of 'instanceof' is not callable
instanceof
need the right-hand value to be callable, which means it must be a function(MDN call it as the constructor)
and instanceof
tests the presence of constructor.prototype
in object's prototype chain.
but isPrototypeOf()
don't have such limit. While instanceof
checks superProto.prototype
, isPrototypeOf()
checks superProto
directly.
add a comment |
Just complement @apsillers's answer
object instanceof constructor
var superProto = {}
// subProto.__proto__.__proto__ === superProto
var subProto = Object.create(superProto);
subProto.someProp = 5;
// sub.__proto__.__proto__ === subProto
var sub = Object.create(subProto);
console.log(superProto.isPrototypeOf(sub)); // true
console.log(sub instanceof superProto); // TypeError: Right-hand side of 'instanceof' is not callable
// helper utility to see if `o1` is
// related to (delegates to) `o2`
function isRelatedTo(o1, o2) {
function F(){}
F.prototype = o2;
// ensure the right-hand side of 'instanceof' is callable
return o1 instanceof F;
}
isRelatedTo( b, a );
TypeError: Right-hand side of 'instanceof' is not callable
instanceof
need the right-hand value to be callable, which means it must be a function(MDN call it as the constructor)
and instanceof
tests the presence of constructor.prototype
in object's prototype chain.
but isPrototypeOf()
don't have such limit. While instanceof
checks superProto.prototype
, isPrototypeOf()
checks superProto
directly.
add a comment |
Just complement @apsillers's answer
object instanceof constructor
var superProto = {}
// subProto.__proto__.__proto__ === superProto
var subProto = Object.create(superProto);
subProto.someProp = 5;
// sub.__proto__.__proto__ === subProto
var sub = Object.create(subProto);
console.log(superProto.isPrototypeOf(sub)); // true
console.log(sub instanceof superProto); // TypeError: Right-hand side of 'instanceof' is not callable
// helper utility to see if `o1` is
// related to (delegates to) `o2`
function isRelatedTo(o1, o2) {
function F(){}
F.prototype = o2;
// ensure the right-hand side of 'instanceof' is callable
return o1 instanceof F;
}
isRelatedTo( b, a );
TypeError: Right-hand side of 'instanceof' is not callable
instanceof
need the right-hand value to be callable, which means it must be a function(MDN call it as the constructor)
and instanceof
tests the presence of constructor.prototype
in object's prototype chain.
but isPrototypeOf()
don't have such limit. While instanceof
checks superProto.prototype
, isPrototypeOf()
checks superProto
directly.
Just complement @apsillers's answer
object instanceof constructor
var superProto = {}
// subProto.__proto__.__proto__ === superProto
var subProto = Object.create(superProto);
subProto.someProp = 5;
// sub.__proto__.__proto__ === subProto
var sub = Object.create(subProto);
console.log(superProto.isPrototypeOf(sub)); // true
console.log(sub instanceof superProto); // TypeError: Right-hand side of 'instanceof' is not callable
// helper utility to see if `o1` is
// related to (delegates to) `o2`
function isRelatedTo(o1, o2) {
function F(){}
F.prototype = o2;
// ensure the right-hand side of 'instanceof' is callable
return o1 instanceof F;
}
isRelatedTo( b, a );
TypeError: Right-hand side of 'instanceof' is not callable
instanceof
need the right-hand value to be callable, which means it must be a function(MDN call it as the constructor)
and instanceof
tests the presence of constructor.prototype
in object's prototype chain.
but isPrototypeOf()
don't have such limit. While instanceof
checks superProto.prototype
, isPrototypeOf()
checks superProto
directly.
answered Nov 23 '18 at 6:24


Tina ChenTina Chen
8421334
8421334
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f18343545%2fjavascript-isprototypeof-vs-instanceof-usage%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
qrympJyfzrPLeLtklngsaK,kav A38 WgZdVwW cQmCQ5wXx,V ATf K q vb58sh 5j42K3gPsGtcrjZTHoCAFz5Fc 8ViZ,v Cd6b 2rNlVa,8
possible duplicate of Why do we need the isPrototypeOf at all?
– plalx
Aug 20 '13 at 19:54
Based on the OP's comment, I'd say this question is closer to "Why do we need
instanceof
at all?" but I agree that it's probably close enough.– apsillers
Aug 20 '13 at 20:22