Cannot set a property “year” of undefined TypeScript-Array
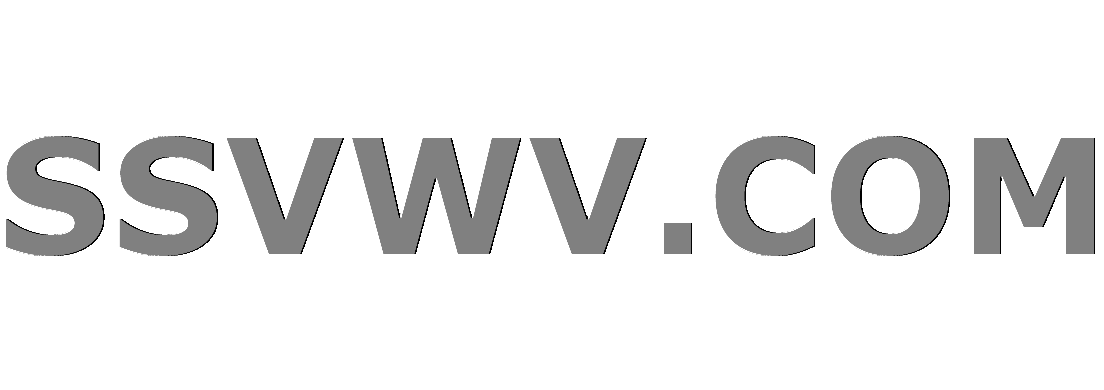
Multi tool use
I have the following declaration in my component:
private years: Array<{
year: string,
colspan: number
}> = ;
Which is supposed to be a years
-Array where each entry has the two properties year
and colspan
. Now I want to modify this variable with the following method called in my ngOnInit
:
fillYearsArray = () => {
var currentIndex : number = 1;
//fill the first entry and do not change it afterwards, error occurs here
this.years[0].year = "";
this.years[0].colspan= 1;
this.items.forEach( (item) => {
//if the first entry.year is empty fill it with the year of the current object
if (this.years[currentIndex].year) {
//if it is already set and the years equal enlarge colspan
//otherwise go to the next entry with the "new" year
if (this.years[currentIndex].year === String(item.year)) {
this.years[currentIndex].colspan += this.columnKeys.length;
} else {
currentIndex++;
this.years[currentIndex].year = String(item.year);
this.years[currentIndex].colspan = this.columnKeys.length;
}
} else {
this.years[currentIndex].year = String(item.year);
}
});
}
But when running ng serve
I get the following output:
AppComponent.html:1 ERROR TypeError: Cannot set property 'year' of undefined
at TabularComponent.fillYearsArray (tabular.component.ts:27)
at TabularComponent.push../src/app/tabular/tabular.component.ts.TabularComponent.ngOnInit (tabular.component.ts:22)
at checkAndUpdateDirectiveInline (core.js:18537)
at checkAndUpdateNodeInline (core.js:19801)
at checkAndUpdateNode (core.js:19763)
at debugCheckAndUpdateNode (core.js:20397)
at debugCheckDirectivesFn (core.js:20357)
at Object.eval [as updateDirectives] (AppComponent.html:1)
at Object.debugUpdateDirectives [as updateDirectives] (core.js:20349)
at checkAndUpdateView (core.js:19745)
So it looks like the first object in the array would be undefined, but shouldn't TS know, that it has an property year
that is changed for the object at the first entry of my years
-array?
arrays

add a comment |
I have the following declaration in my component:
private years: Array<{
year: string,
colspan: number
}> = ;
Which is supposed to be a years
-Array where each entry has the two properties year
and colspan
. Now I want to modify this variable with the following method called in my ngOnInit
:
fillYearsArray = () => {
var currentIndex : number = 1;
//fill the first entry and do not change it afterwards, error occurs here
this.years[0].year = "";
this.years[0].colspan= 1;
this.items.forEach( (item) => {
//if the first entry.year is empty fill it with the year of the current object
if (this.years[currentIndex].year) {
//if it is already set and the years equal enlarge colspan
//otherwise go to the next entry with the "new" year
if (this.years[currentIndex].year === String(item.year)) {
this.years[currentIndex].colspan += this.columnKeys.length;
} else {
currentIndex++;
this.years[currentIndex].year = String(item.year);
this.years[currentIndex].colspan = this.columnKeys.length;
}
} else {
this.years[currentIndex].year = String(item.year);
}
});
}
But when running ng serve
I get the following output:
AppComponent.html:1 ERROR TypeError: Cannot set property 'year' of undefined
at TabularComponent.fillYearsArray (tabular.component.ts:27)
at TabularComponent.push../src/app/tabular/tabular.component.ts.TabularComponent.ngOnInit (tabular.component.ts:22)
at checkAndUpdateDirectiveInline (core.js:18537)
at checkAndUpdateNodeInline (core.js:19801)
at checkAndUpdateNode (core.js:19763)
at debugCheckAndUpdateNode (core.js:20397)
at debugCheckDirectivesFn (core.js:20357)
at Object.eval [as updateDirectives] (AppComponent.html:1)
at Object.debugUpdateDirectives [as updateDirectives] (core.js:20349)
at checkAndUpdateView (core.js:19745)
So it looks like the first object in the array would be undefined, but shouldn't TS know, that it has an property year
that is changed for the object at the first entry of my years
-array?
arrays

1
Is your years array set before you go in your function, if not you need to push data into it before doing this.year[0]
– Alann
Nov 26 '18 at 10:39
1
this.years[0] = { year: "", colspan: 1 }
– Fateme Fazli
Nov 26 '18 at 10:43
1
do it like this this.year.push( { year: "", colspan: 1 }) and like that it should work
– Alann
Nov 26 '18 at 10:44
add a comment |
I have the following declaration in my component:
private years: Array<{
year: string,
colspan: number
}> = ;
Which is supposed to be a years
-Array where each entry has the two properties year
and colspan
. Now I want to modify this variable with the following method called in my ngOnInit
:
fillYearsArray = () => {
var currentIndex : number = 1;
//fill the first entry and do not change it afterwards, error occurs here
this.years[0].year = "";
this.years[0].colspan= 1;
this.items.forEach( (item) => {
//if the first entry.year is empty fill it with the year of the current object
if (this.years[currentIndex].year) {
//if it is already set and the years equal enlarge colspan
//otherwise go to the next entry with the "new" year
if (this.years[currentIndex].year === String(item.year)) {
this.years[currentIndex].colspan += this.columnKeys.length;
} else {
currentIndex++;
this.years[currentIndex].year = String(item.year);
this.years[currentIndex].colspan = this.columnKeys.length;
}
} else {
this.years[currentIndex].year = String(item.year);
}
});
}
But when running ng serve
I get the following output:
AppComponent.html:1 ERROR TypeError: Cannot set property 'year' of undefined
at TabularComponent.fillYearsArray (tabular.component.ts:27)
at TabularComponent.push../src/app/tabular/tabular.component.ts.TabularComponent.ngOnInit (tabular.component.ts:22)
at checkAndUpdateDirectiveInline (core.js:18537)
at checkAndUpdateNodeInline (core.js:19801)
at checkAndUpdateNode (core.js:19763)
at debugCheckAndUpdateNode (core.js:20397)
at debugCheckDirectivesFn (core.js:20357)
at Object.eval [as updateDirectives] (AppComponent.html:1)
at Object.debugUpdateDirectives [as updateDirectives] (core.js:20349)
at checkAndUpdateView (core.js:19745)
So it looks like the first object in the array would be undefined, but shouldn't TS know, that it has an property year
that is changed for the object at the first entry of my years
-array?
arrays

I have the following declaration in my component:
private years: Array<{
year: string,
colspan: number
}> = ;
Which is supposed to be a years
-Array where each entry has the two properties year
and colspan
. Now I want to modify this variable with the following method called in my ngOnInit
:
fillYearsArray = () => {
var currentIndex : number = 1;
//fill the first entry and do not change it afterwards, error occurs here
this.years[0].year = "";
this.years[0].colspan= 1;
this.items.forEach( (item) => {
//if the first entry.year is empty fill it with the year of the current object
if (this.years[currentIndex].year) {
//if it is already set and the years equal enlarge colspan
//otherwise go to the next entry with the "new" year
if (this.years[currentIndex].year === String(item.year)) {
this.years[currentIndex].colspan += this.columnKeys.length;
} else {
currentIndex++;
this.years[currentIndex].year = String(item.year);
this.years[currentIndex].colspan = this.columnKeys.length;
}
} else {
this.years[currentIndex].year = String(item.year);
}
});
}
But when running ng serve
I get the following output:
AppComponent.html:1 ERROR TypeError: Cannot set property 'year' of undefined
at TabularComponent.fillYearsArray (tabular.component.ts:27)
at TabularComponent.push../src/app/tabular/tabular.component.ts.TabularComponent.ngOnInit (tabular.component.ts:22)
at checkAndUpdateDirectiveInline (core.js:18537)
at checkAndUpdateNodeInline (core.js:19801)
at checkAndUpdateNode (core.js:19763)
at debugCheckAndUpdateNode (core.js:20397)
at debugCheckDirectivesFn (core.js:20357)
at Object.eval [as updateDirectives] (AppComponent.html:1)
at Object.debugUpdateDirectives [as updateDirectives] (core.js:20349)
at checkAndUpdateView (core.js:19745)
So it looks like the first object in the array would be undefined, but shouldn't TS know, that it has an property year
that is changed for the object at the first entry of my years
-array?
arrays

arrays

asked Nov 26 '18 at 10:37
RüdigerRüdiger
221324
221324
1
Is your years array set before you go in your function, if not you need to push data into it before doing this.year[0]
– Alann
Nov 26 '18 at 10:39
1
this.years[0] = { year: "", colspan: 1 }
– Fateme Fazli
Nov 26 '18 at 10:43
1
do it like this this.year.push( { year: "", colspan: 1 }) and like that it should work
– Alann
Nov 26 '18 at 10:44
add a comment |
1
Is your years array set before you go in your function, if not you need to push data into it before doing this.year[0]
– Alann
Nov 26 '18 at 10:39
1
this.years[0] = { year: "", colspan: 1 }
– Fateme Fazli
Nov 26 '18 at 10:43
1
do it like this this.year.push( { year: "", colspan: 1 }) and like that it should work
– Alann
Nov 26 '18 at 10:44
1
1
Is your years array set before you go in your function, if not you need to push data into it before doing this.year[0]
– Alann
Nov 26 '18 at 10:39
Is your years array set before you go in your function, if not you need to push data into it before doing this.year[0]
– Alann
Nov 26 '18 at 10:39
1
1
this.years[0] = { year: "", colspan: 1 }
– Fateme Fazli
Nov 26 '18 at 10:43
this.years[0] = { year: "", colspan: 1 }
– Fateme Fazli
Nov 26 '18 at 10:43
1
1
do it like this this.year.push( { year: "", colspan: 1 }) and like that it should work
– Alann
Nov 26 '18 at 10:44
do it like this this.year.push( { year: "", colspan: 1 }) and like that it should work
– Alann
Nov 26 '18 at 10:44
add a comment |
1 Answer
1
active
oldest
votes
You need to add an element before you change it's value
you try to access
this.years[0]
but this.years length is 0
so you need to do it like this
this.years.push({ year: "", colspan: 1});
if you want to add item in your array you need to use the .push() function.
If you try to go to the index you want to set, it won't work
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53479284%2fcannot-set-a-property-year-of-undefined-typescript-array%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You need to add an element before you change it's value
you try to access
this.years[0]
but this.years length is 0
so you need to do it like this
this.years.push({ year: "", colspan: 1});
if you want to add item in your array you need to use the .push() function.
If you try to go to the index you want to set, it won't work
add a comment |
You need to add an element before you change it's value
you try to access
this.years[0]
but this.years length is 0
so you need to do it like this
this.years.push({ year: "", colspan: 1});
if you want to add item in your array you need to use the .push() function.
If you try to go to the index you want to set, it won't work
add a comment |
You need to add an element before you change it's value
you try to access
this.years[0]
but this.years length is 0
so you need to do it like this
this.years.push({ year: "", colspan: 1});
if you want to add item in your array you need to use the .push() function.
If you try to go to the index you want to set, it won't work
You need to add an element before you change it's value
you try to access
this.years[0]
but this.years length is 0
so you need to do it like this
this.years.push({ year: "", colspan: 1});
if you want to add item in your array you need to use the .push() function.
If you try to go to the index you want to set, it won't work
answered Nov 26 '18 at 10:45
AlannAlann
150112
150112
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53479284%2fcannot-set-a-property-year-of-undefined-typescript-array%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
d6,IGjpHWzH0gtfzTihwd2ZjjY,bKjyBu
1
Is your years array set before you go in your function, if not you need to push data into it before doing this.year[0]
– Alann
Nov 26 '18 at 10:39
1
this.years[0] = { year: "", colspan: 1 }
– Fateme Fazli
Nov 26 '18 at 10:43
1
do it like this this.year.push( { year: "", colspan: 1 }) and like that it should work
– Alann
Nov 26 '18 at 10:44