How to randomly order two synchronized lists using javascript?
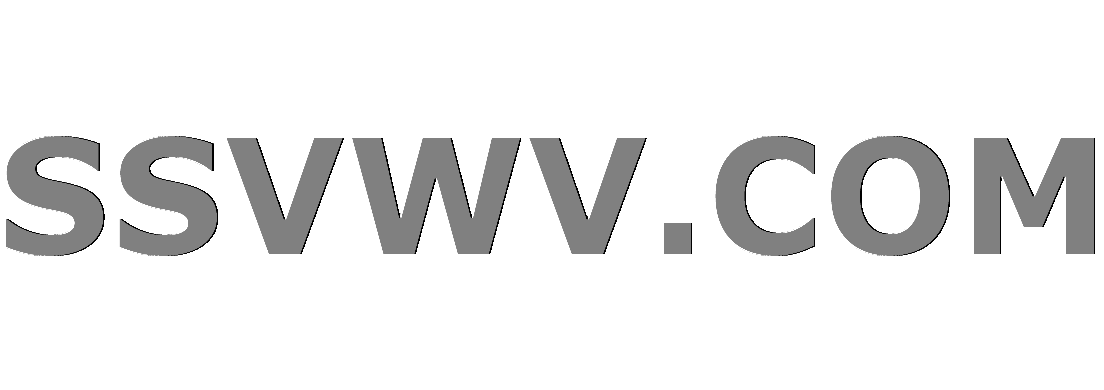
Multi tool use
Aparently shuffling an array is not so complicated:
How to randomize (shuffle) a JavaScript array?
But what if I have to (Html DOM) lists that are synchronized and I need to shuffle the order of the elements, but they should have the same final order?
For example, initial state:
<!-- List A) -->
<ul>
<li>First title</li>
<li>Second Title</li>
<li>Thrid title</li>
</ul>
<!-- List B) -->
<ul>
<li>First text</li>
<li>Second text</li>
<li>Thhird text</li>
</ul>
After shuffle:
<!-- List A) -->
<ul>
<li>Second title</li>
<li>First Title</li>
<li>Thrid text</li>
</ul>
<!-- List B) -->
<ul>
<li>Second text</li>
<li>First text</li>
<li>Third text</li>
</ul>
How can this be achieved?
javascript html random shuffle
add a comment |
Aparently shuffling an array is not so complicated:
How to randomize (shuffle) a JavaScript array?
But what if I have to (Html DOM) lists that are synchronized and I need to shuffle the order of the elements, but they should have the same final order?
For example, initial state:
<!-- List A) -->
<ul>
<li>First title</li>
<li>Second Title</li>
<li>Thrid title</li>
</ul>
<!-- List B) -->
<ul>
<li>First text</li>
<li>Second text</li>
<li>Thhird text</li>
</ul>
After shuffle:
<!-- List A) -->
<ul>
<li>Second title</li>
<li>First Title</li>
<li>Thrid text</li>
</ul>
<!-- List B) -->
<ul>
<li>Second text</li>
<li>First text</li>
<li>Third text</li>
</ul>
How can this be achieved?
javascript html random shuffle
So you want to shuffle the first array and have the second array have the same order as the first, correct?
– George
Nov 26 '18 at 10:47
@George thats correct
– Toni Michel Caubet
Nov 26 '18 at 10:52
add a comment |
Aparently shuffling an array is not so complicated:
How to randomize (shuffle) a JavaScript array?
But what if I have to (Html DOM) lists that are synchronized and I need to shuffle the order of the elements, but they should have the same final order?
For example, initial state:
<!-- List A) -->
<ul>
<li>First title</li>
<li>Second Title</li>
<li>Thrid title</li>
</ul>
<!-- List B) -->
<ul>
<li>First text</li>
<li>Second text</li>
<li>Thhird text</li>
</ul>
After shuffle:
<!-- List A) -->
<ul>
<li>Second title</li>
<li>First Title</li>
<li>Thrid text</li>
</ul>
<!-- List B) -->
<ul>
<li>Second text</li>
<li>First text</li>
<li>Third text</li>
</ul>
How can this be achieved?
javascript html random shuffle
Aparently shuffling an array is not so complicated:
How to randomize (shuffle) a JavaScript array?
But what if I have to (Html DOM) lists that are synchronized and I need to shuffle the order of the elements, but they should have the same final order?
For example, initial state:
<!-- List A) -->
<ul>
<li>First title</li>
<li>Second Title</li>
<li>Thrid title</li>
</ul>
<!-- List B) -->
<ul>
<li>First text</li>
<li>Second text</li>
<li>Thhird text</li>
</ul>
After shuffle:
<!-- List A) -->
<ul>
<li>Second title</li>
<li>First Title</li>
<li>Thrid text</li>
</ul>
<!-- List B) -->
<ul>
<li>Second text</li>
<li>First text</li>
<li>Third text</li>
</ul>
How can this be achieved?
javascript html random shuffle
javascript html random shuffle
edited Jan 29 at 3:04


Pikachu the Purple Wizard
2,03361329
2,03361329
asked Nov 26 '18 at 10:31
Toni Michel CaubetToni Michel Caubet
7,10346160310
7,10346160310
So you want to shuffle the first array and have the second array have the same order as the first, correct?
– George
Nov 26 '18 at 10:47
@George thats correct
– Toni Michel Caubet
Nov 26 '18 at 10:52
add a comment |
So you want to shuffle the first array and have the second array have the same order as the first, correct?
– George
Nov 26 '18 at 10:47
@George thats correct
– Toni Michel Caubet
Nov 26 '18 at 10:52
So you want to shuffle the first array and have the second array have the same order as the first, correct?
– George
Nov 26 '18 at 10:47
So you want to shuffle the first array and have the second array have the same order as the first, correct?
– George
Nov 26 '18 at 10:47
@George thats correct
– Toni Michel Caubet
Nov 26 '18 at 10:52
@George thats correct
– Toni Michel Caubet
Nov 26 '18 at 10:52
add a comment |
2 Answers
2
active
oldest
votes
Get length of items and loop through it and in loop generate random number and using generated number select an li
and append it end of parent.
var ul = document.querySelectorAll("ul");
var length = ul[0].querySelectorAll("li").length;
for (var i=0; i<length; i++){
var rand = Math.floor(Math.random()*(length));
ul.forEach(function(ele){
ele.appendChild(ele.querySelectorAll("li")[rand]);
});
}
<ul>
<li>First title</li>
<li>Second Title</li>
<li>Thrid title</li>
</ul>
<ul>
<li>First text</li>
<li>Second text</li>
<li>Thhird text</li>
</ul>
Also you can use jQuery to write less code
var $ul = $("ul:first li");
$ul.each(function(){
var rand = Math.floor(Math.random()*$ul.length);
$("ul").each(function(i, ele){
$("li", ele).eq(rand).appendTo(ele);
});
});
$("button").click(function(){
var $ul = $("ul:first li");
$ul.each(function(){
var rand = Math.floor(Math.random()*$ul.length);
$("ul").each(function(i, ele){
$("li", ele).eq(rand).appendTo(ele);
});
});
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<button>Click</button>
<ul>
<li>First title</li>
<li>Second Title</li>
<li>Thrid title</li>
</ul>
<ul>
<li>First text</li>
<li>Second text</li>
<li>Thhird text</li>
</ul>
add a comment |
You can easily shuffle an DOM html in various number of ways. One way that is easy to understand is to convert the DOM elements into an array, then randomly splice each element out of its parent array and append them back to the parent DOM element.
In my snippet below, I used jQuery to make the code easier to read, but it can be done with native javascript just as well.
$('#shuffle').click(function(){
var items = ; // start with an empty array
$('#list_a li').each(function(i,d){
items.push(d); // add all li items into the array in their current order
});
$('#list_a').html(''); // clear the ul list
// execute this loop as many times as items.length
for(var i=items.length-1; i>=0; i--) {
var r = Math.floor(Math.random()*items.length); // pick a random array position
var item = items.splice(r,1); // take that item out of the array
$('#list_a').append(item); // append it back to the ul
}
});
ul
{
background-color: #def;
display: inline-block;
border: 1px solid grey;
padding: 30px;
border-radius: 20px;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.10.1/jquery.min.js"></script>
<b>List A</b>
<ul id="list_a">
<li>First Item</li>
<li>Second Item</li>
<li>Third Item</li>
<li>Fourth Item</li>
<li>Fifth Item</li>
</ul>
<button id="shuffle">Shuffle</button>
Note that this is not ordering two sincronized lists.. but thanks anyway
– Toni Michel Caubet
Nov 26 '18 at 13:25
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53479198%2fhow-to-randomly-order-two-synchronized-lists-using-javascript%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Get length of items and loop through it and in loop generate random number and using generated number select an li
and append it end of parent.
var ul = document.querySelectorAll("ul");
var length = ul[0].querySelectorAll("li").length;
for (var i=0; i<length; i++){
var rand = Math.floor(Math.random()*(length));
ul.forEach(function(ele){
ele.appendChild(ele.querySelectorAll("li")[rand]);
});
}
<ul>
<li>First title</li>
<li>Second Title</li>
<li>Thrid title</li>
</ul>
<ul>
<li>First text</li>
<li>Second text</li>
<li>Thhird text</li>
</ul>
Also you can use jQuery to write less code
var $ul = $("ul:first li");
$ul.each(function(){
var rand = Math.floor(Math.random()*$ul.length);
$("ul").each(function(i, ele){
$("li", ele).eq(rand).appendTo(ele);
});
});
$("button").click(function(){
var $ul = $("ul:first li");
$ul.each(function(){
var rand = Math.floor(Math.random()*$ul.length);
$("ul").each(function(i, ele){
$("li", ele).eq(rand).appendTo(ele);
});
});
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<button>Click</button>
<ul>
<li>First title</li>
<li>Second Title</li>
<li>Thrid title</li>
</ul>
<ul>
<li>First text</li>
<li>Second text</li>
<li>Thhird text</li>
</ul>
add a comment |
Get length of items and loop through it and in loop generate random number and using generated number select an li
and append it end of parent.
var ul = document.querySelectorAll("ul");
var length = ul[0].querySelectorAll("li").length;
for (var i=0; i<length; i++){
var rand = Math.floor(Math.random()*(length));
ul.forEach(function(ele){
ele.appendChild(ele.querySelectorAll("li")[rand]);
});
}
<ul>
<li>First title</li>
<li>Second Title</li>
<li>Thrid title</li>
</ul>
<ul>
<li>First text</li>
<li>Second text</li>
<li>Thhird text</li>
</ul>
Also you can use jQuery to write less code
var $ul = $("ul:first li");
$ul.each(function(){
var rand = Math.floor(Math.random()*$ul.length);
$("ul").each(function(i, ele){
$("li", ele).eq(rand).appendTo(ele);
});
});
$("button").click(function(){
var $ul = $("ul:first li");
$ul.each(function(){
var rand = Math.floor(Math.random()*$ul.length);
$("ul").each(function(i, ele){
$("li", ele).eq(rand).appendTo(ele);
});
});
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<button>Click</button>
<ul>
<li>First title</li>
<li>Second Title</li>
<li>Thrid title</li>
</ul>
<ul>
<li>First text</li>
<li>Second text</li>
<li>Thhird text</li>
</ul>
add a comment |
Get length of items and loop through it and in loop generate random number and using generated number select an li
and append it end of parent.
var ul = document.querySelectorAll("ul");
var length = ul[0].querySelectorAll("li").length;
for (var i=0; i<length; i++){
var rand = Math.floor(Math.random()*(length));
ul.forEach(function(ele){
ele.appendChild(ele.querySelectorAll("li")[rand]);
});
}
<ul>
<li>First title</li>
<li>Second Title</li>
<li>Thrid title</li>
</ul>
<ul>
<li>First text</li>
<li>Second text</li>
<li>Thhird text</li>
</ul>
Also you can use jQuery to write less code
var $ul = $("ul:first li");
$ul.each(function(){
var rand = Math.floor(Math.random()*$ul.length);
$("ul").each(function(i, ele){
$("li", ele).eq(rand).appendTo(ele);
});
});
$("button").click(function(){
var $ul = $("ul:first li");
$ul.each(function(){
var rand = Math.floor(Math.random()*$ul.length);
$("ul").each(function(i, ele){
$("li", ele).eq(rand).appendTo(ele);
});
});
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<button>Click</button>
<ul>
<li>First title</li>
<li>Second Title</li>
<li>Thrid title</li>
</ul>
<ul>
<li>First text</li>
<li>Second text</li>
<li>Thhird text</li>
</ul>
Get length of items and loop through it and in loop generate random number and using generated number select an li
and append it end of parent.
var ul = document.querySelectorAll("ul");
var length = ul[0].querySelectorAll("li").length;
for (var i=0; i<length; i++){
var rand = Math.floor(Math.random()*(length));
ul.forEach(function(ele){
ele.appendChild(ele.querySelectorAll("li")[rand]);
});
}
<ul>
<li>First title</li>
<li>Second Title</li>
<li>Thrid title</li>
</ul>
<ul>
<li>First text</li>
<li>Second text</li>
<li>Thhird text</li>
</ul>
Also you can use jQuery to write less code
var $ul = $("ul:first li");
$ul.each(function(){
var rand = Math.floor(Math.random()*$ul.length);
$("ul").each(function(i, ele){
$("li", ele).eq(rand).appendTo(ele);
});
});
$("button").click(function(){
var $ul = $("ul:first li");
$ul.each(function(){
var rand = Math.floor(Math.random()*$ul.length);
$("ul").each(function(i, ele){
$("li", ele).eq(rand).appendTo(ele);
});
});
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<button>Click</button>
<ul>
<li>First title</li>
<li>Second Title</li>
<li>Thrid title</li>
</ul>
<ul>
<li>First text</li>
<li>Second text</li>
<li>Thhird text</li>
</ul>
var ul = document.querySelectorAll("ul");
var length = ul[0].querySelectorAll("li").length;
for (var i=0; i<length; i++){
var rand = Math.floor(Math.random()*(length));
ul.forEach(function(ele){
ele.appendChild(ele.querySelectorAll("li")[rand]);
});
}
<ul>
<li>First title</li>
<li>Second Title</li>
<li>Thrid title</li>
</ul>
<ul>
<li>First text</li>
<li>Second text</li>
<li>Thhird text</li>
</ul>
var ul = document.querySelectorAll("ul");
var length = ul[0].querySelectorAll("li").length;
for (var i=0; i<length; i++){
var rand = Math.floor(Math.random()*(length));
ul.forEach(function(ele){
ele.appendChild(ele.querySelectorAll("li")[rand]);
});
}
<ul>
<li>First title</li>
<li>Second Title</li>
<li>Thrid title</li>
</ul>
<ul>
<li>First text</li>
<li>Second text</li>
<li>Thhird text</li>
</ul>
$("button").click(function(){
var $ul = $("ul:first li");
$ul.each(function(){
var rand = Math.floor(Math.random()*$ul.length);
$("ul").each(function(i, ele){
$("li", ele).eq(rand).appendTo(ele);
});
});
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<button>Click</button>
<ul>
<li>First title</li>
<li>Second Title</li>
<li>Thrid title</li>
</ul>
<ul>
<li>First text</li>
<li>Second text</li>
<li>Thhird text</li>
</ul>
$("button").click(function(){
var $ul = $("ul:first li");
$ul.each(function(){
var rand = Math.floor(Math.random()*$ul.length);
$("ul").each(function(i, ele){
$("li", ele).eq(rand).appendTo(ele);
});
});
});
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<button>Click</button>
<ul>
<li>First title</li>
<li>Second Title</li>
<li>Thrid title</li>
</ul>
<ul>
<li>First text</li>
<li>Second text</li>
<li>Thhird text</li>
</ul>
edited Nov 26 '18 at 11:12
answered Nov 26 '18 at 10:49
MohammadMohammad
15.9k123766
15.9k123766
add a comment |
add a comment |
You can easily shuffle an DOM html in various number of ways. One way that is easy to understand is to convert the DOM elements into an array, then randomly splice each element out of its parent array and append them back to the parent DOM element.
In my snippet below, I used jQuery to make the code easier to read, but it can be done with native javascript just as well.
$('#shuffle').click(function(){
var items = ; // start with an empty array
$('#list_a li').each(function(i,d){
items.push(d); // add all li items into the array in their current order
});
$('#list_a').html(''); // clear the ul list
// execute this loop as many times as items.length
for(var i=items.length-1; i>=0; i--) {
var r = Math.floor(Math.random()*items.length); // pick a random array position
var item = items.splice(r,1); // take that item out of the array
$('#list_a').append(item); // append it back to the ul
}
});
ul
{
background-color: #def;
display: inline-block;
border: 1px solid grey;
padding: 30px;
border-radius: 20px;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.10.1/jquery.min.js"></script>
<b>List A</b>
<ul id="list_a">
<li>First Item</li>
<li>Second Item</li>
<li>Third Item</li>
<li>Fourth Item</li>
<li>Fifth Item</li>
</ul>
<button id="shuffle">Shuffle</button>
Note that this is not ordering two sincronized lists.. but thanks anyway
– Toni Michel Caubet
Nov 26 '18 at 13:25
add a comment |
You can easily shuffle an DOM html in various number of ways. One way that is easy to understand is to convert the DOM elements into an array, then randomly splice each element out of its parent array and append them back to the parent DOM element.
In my snippet below, I used jQuery to make the code easier to read, but it can be done with native javascript just as well.
$('#shuffle').click(function(){
var items = ; // start with an empty array
$('#list_a li').each(function(i,d){
items.push(d); // add all li items into the array in their current order
});
$('#list_a').html(''); // clear the ul list
// execute this loop as many times as items.length
for(var i=items.length-1; i>=0; i--) {
var r = Math.floor(Math.random()*items.length); // pick a random array position
var item = items.splice(r,1); // take that item out of the array
$('#list_a').append(item); // append it back to the ul
}
});
ul
{
background-color: #def;
display: inline-block;
border: 1px solid grey;
padding: 30px;
border-radius: 20px;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.10.1/jquery.min.js"></script>
<b>List A</b>
<ul id="list_a">
<li>First Item</li>
<li>Second Item</li>
<li>Third Item</li>
<li>Fourth Item</li>
<li>Fifth Item</li>
</ul>
<button id="shuffle">Shuffle</button>
Note that this is not ordering two sincronized lists.. but thanks anyway
– Toni Michel Caubet
Nov 26 '18 at 13:25
add a comment |
You can easily shuffle an DOM html in various number of ways. One way that is easy to understand is to convert the DOM elements into an array, then randomly splice each element out of its parent array and append them back to the parent DOM element.
In my snippet below, I used jQuery to make the code easier to read, but it can be done with native javascript just as well.
$('#shuffle').click(function(){
var items = ; // start with an empty array
$('#list_a li').each(function(i,d){
items.push(d); // add all li items into the array in their current order
});
$('#list_a').html(''); // clear the ul list
// execute this loop as many times as items.length
for(var i=items.length-1; i>=0; i--) {
var r = Math.floor(Math.random()*items.length); // pick a random array position
var item = items.splice(r,1); // take that item out of the array
$('#list_a').append(item); // append it back to the ul
}
});
ul
{
background-color: #def;
display: inline-block;
border: 1px solid grey;
padding: 30px;
border-radius: 20px;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.10.1/jquery.min.js"></script>
<b>List A</b>
<ul id="list_a">
<li>First Item</li>
<li>Second Item</li>
<li>Third Item</li>
<li>Fourth Item</li>
<li>Fifth Item</li>
</ul>
<button id="shuffle">Shuffle</button>
You can easily shuffle an DOM html in various number of ways. One way that is easy to understand is to convert the DOM elements into an array, then randomly splice each element out of its parent array and append them back to the parent DOM element.
In my snippet below, I used jQuery to make the code easier to read, but it can be done with native javascript just as well.
$('#shuffle').click(function(){
var items = ; // start with an empty array
$('#list_a li').each(function(i,d){
items.push(d); // add all li items into the array in their current order
});
$('#list_a').html(''); // clear the ul list
// execute this loop as many times as items.length
for(var i=items.length-1; i>=0; i--) {
var r = Math.floor(Math.random()*items.length); // pick a random array position
var item = items.splice(r,1); // take that item out of the array
$('#list_a').append(item); // append it back to the ul
}
});
ul
{
background-color: #def;
display: inline-block;
border: 1px solid grey;
padding: 30px;
border-radius: 20px;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.10.1/jquery.min.js"></script>
<b>List A</b>
<ul id="list_a">
<li>First Item</li>
<li>Second Item</li>
<li>Third Item</li>
<li>Fourth Item</li>
<li>Fifth Item</li>
</ul>
<button id="shuffle">Shuffle</button>
$('#shuffle').click(function(){
var items = ; // start with an empty array
$('#list_a li').each(function(i,d){
items.push(d); // add all li items into the array in their current order
});
$('#list_a').html(''); // clear the ul list
// execute this loop as many times as items.length
for(var i=items.length-1; i>=0; i--) {
var r = Math.floor(Math.random()*items.length); // pick a random array position
var item = items.splice(r,1); // take that item out of the array
$('#list_a').append(item); // append it back to the ul
}
});
ul
{
background-color: #def;
display: inline-block;
border: 1px solid grey;
padding: 30px;
border-radius: 20px;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.10.1/jquery.min.js"></script>
<b>List A</b>
<ul id="list_a">
<li>First Item</li>
<li>Second Item</li>
<li>Third Item</li>
<li>Fourth Item</li>
<li>Fifth Item</li>
</ul>
<button id="shuffle">Shuffle</button>
$('#shuffle').click(function(){
var items = ; // start with an empty array
$('#list_a li').each(function(i,d){
items.push(d); // add all li items into the array in their current order
});
$('#list_a').html(''); // clear the ul list
// execute this loop as many times as items.length
for(var i=items.length-1; i>=0; i--) {
var r = Math.floor(Math.random()*items.length); // pick a random array position
var item = items.splice(r,1); // take that item out of the array
$('#list_a').append(item); // append it back to the ul
}
});
ul
{
background-color: #def;
display: inline-block;
border: 1px solid grey;
padding: 30px;
border-radius: 20px;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.10.1/jquery.min.js"></script>
<b>List A</b>
<ul id="list_a">
<li>First Item</li>
<li>Second Item</li>
<li>Third Item</li>
<li>Fourth Item</li>
<li>Fifth Item</li>
</ul>
<button id="shuffle">Shuffle</button>
answered Nov 26 '18 at 10:59
AhmadAhmad
8,35043764
8,35043764
Note that this is not ordering two sincronized lists.. but thanks anyway
– Toni Michel Caubet
Nov 26 '18 at 13:25
add a comment |
Note that this is not ordering two sincronized lists.. but thanks anyway
– Toni Michel Caubet
Nov 26 '18 at 13:25
Note that this is not ordering two sincronized lists.. but thanks anyway
– Toni Michel Caubet
Nov 26 '18 at 13:25
Note that this is not ordering two sincronized lists.. but thanks anyway
– Toni Michel Caubet
Nov 26 '18 at 13:25
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53479198%2fhow-to-randomly-order-two-synchronized-lists-using-javascript%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
3LUbJ7LfkusPK 8iN8ab,vbiQ6MgENoo6vU uAOrc pII7KCmYpndP zZ5,it3M6zFYsdk pdDLCOO6 Cb70
So you want to shuffle the first array and have the second array have the same order as the first, correct?
– George
Nov 26 '18 at 10:47
@George thats correct
– Toni Michel Caubet
Nov 26 '18 at 10:52