convert stop watch time to seconds
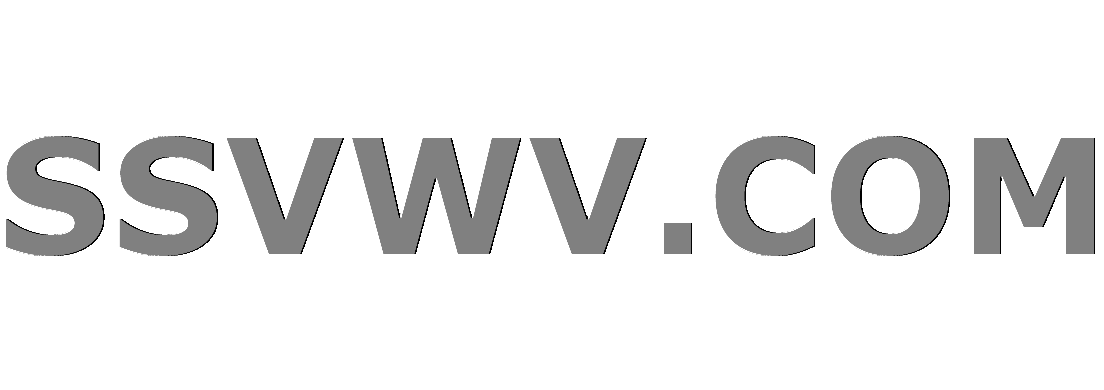
Multi tool use
I have a stopwatch on my website I would like to take the result after the stop button is clicked and convert it into seconds.
is it possible to calculate the given time and turn it into seconds only with javascript or jquery?
after the convert, I would like to send the value into my database
I can see the result in my console
var ss = document.getElementsByClassName('stopwatch');
.forEach.call(ss, function (s) {
var currentTimer = 0,
interval = 0,
lastUpdateTime = new Date().getTime(),
start = s.querySelector('button.start'),
stop = s.querySelector('button.stop'),
reset = s.querySelector('button.reset'),
mins = s.querySelector('span.minutes'),
secs = s.querySelector('span.seconds'),
cents = s.querySelector('span.centiseconds');
start.addEventListener('click', startTimer);
stop.addEventListener('click', stopTimer);
reset.addEventListener('click', resetTimer);
function pad (n) {
return ('00' + n).substr(-2);
}
function update () {
var now = new Date().getTime(),
dt = now - lastUpdateTime;
currentTimer += dt;
var time = new Date(currentTimer);
mins.innerHTML = pad(time.getMinutes());
secs.innerHTML = pad(time.getSeconds());
cents.innerHTML = pad(Math.floor(time.getMilliseconds() / 10));
lastUpdateTime = now;
}
function startTimer () {
if (!interval) {
lastUpdateTime = new Date().getTime();
interval = setInterval(update, 1);
}
}
function stopTimer () {
clearInterval(interval);
interval = 0;
}
function resetTimer () {
stopTimer();
currentTimer = 0;
mins.innerHTML = secs.innerHTML = cents.innerHTML = pad(0);
}
});
$(document).ready(function(){
$(".stop").click(function(){
var m = $('#min').html();
var s = $('#sec').html();
var ms = $('#msec').html();
console.log(m,s,ms);
});
});
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Stopwatch</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
</head>
<body>
<div class="stopwatch">
<div class="controls">
<button class="start">Start</button>
<button id="stp" class="stop">Stop</button>
<button class="reset">Reset</button>
</div>
<div class="display">
<span id="min" class="minutes" value="">00</span><span id="sec" class="seconds" value="">00</span><span id="msec" class="centiseconds" value="">00</span>
</div>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<script src="watch/stopwatch.js"></script>
<script>
</script>
</body>
</html>
javascript jquery html
|
show 3 more comments
I have a stopwatch on my website I would like to take the result after the stop button is clicked and convert it into seconds.
is it possible to calculate the given time and turn it into seconds only with javascript or jquery?
after the convert, I would like to send the value into my database
I can see the result in my console
var ss = document.getElementsByClassName('stopwatch');
.forEach.call(ss, function (s) {
var currentTimer = 0,
interval = 0,
lastUpdateTime = new Date().getTime(),
start = s.querySelector('button.start'),
stop = s.querySelector('button.stop'),
reset = s.querySelector('button.reset'),
mins = s.querySelector('span.minutes'),
secs = s.querySelector('span.seconds'),
cents = s.querySelector('span.centiseconds');
start.addEventListener('click', startTimer);
stop.addEventListener('click', stopTimer);
reset.addEventListener('click', resetTimer);
function pad (n) {
return ('00' + n).substr(-2);
}
function update () {
var now = new Date().getTime(),
dt = now - lastUpdateTime;
currentTimer += dt;
var time = new Date(currentTimer);
mins.innerHTML = pad(time.getMinutes());
secs.innerHTML = pad(time.getSeconds());
cents.innerHTML = pad(Math.floor(time.getMilliseconds() / 10));
lastUpdateTime = now;
}
function startTimer () {
if (!interval) {
lastUpdateTime = new Date().getTime();
interval = setInterval(update, 1);
}
}
function stopTimer () {
clearInterval(interval);
interval = 0;
}
function resetTimer () {
stopTimer();
currentTimer = 0;
mins.innerHTML = secs.innerHTML = cents.innerHTML = pad(0);
}
});
$(document).ready(function(){
$(".stop").click(function(){
var m = $('#min').html();
var s = $('#sec').html();
var ms = $('#msec').html();
console.log(m,s,ms);
});
});
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Stopwatch</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
</head>
<body>
<div class="stopwatch">
<div class="controls">
<button class="start">Start</button>
<button id="stp" class="stop">Stop</button>
<button class="reset">Reset</button>
</div>
<div class="display">
<span id="min" class="minutes" value="">00</span><span id="sec" class="seconds" value="">00</span><span id="msec" class="centiseconds" value="">00</span>
</div>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<script src="watch/stopwatch.js"></script>
<script>
</script>
</body>
</html>
javascript jquery html
You should add HTML as well so we can debug it.
– Cata John
Nov 24 '18 at 19:32
i have updated the post
– dror shalit
Nov 24 '18 at 19:43
I'm not sure I understand where do you have difficulties here? You already have the value of span elements for minutes, seconds and milliseconds. Can't you just take those values and store them in a new variable? Was that the question? If you need a way to do this, let me know and I'll write the code. Btw, how do you intend to send/store milliseconds on the server? In the form of floating number? For example: 19.34s?
– Nemanja Glumac
Nov 24 '18 at 19:51
1
1 millisecond = 0.001 seconds
so go ahead and apply this to your code and it should all work.
– Cata John
Nov 24 '18 at 19:52
Btw, ID is called "msec" (millisecond = 1/1000s), but the class on the same element is centisecond which is 1/100s. You should probably fix that.
– Nemanja Glumac
Nov 24 '18 at 19:56
|
show 3 more comments
I have a stopwatch on my website I would like to take the result after the stop button is clicked and convert it into seconds.
is it possible to calculate the given time and turn it into seconds only with javascript or jquery?
after the convert, I would like to send the value into my database
I can see the result in my console
var ss = document.getElementsByClassName('stopwatch');
.forEach.call(ss, function (s) {
var currentTimer = 0,
interval = 0,
lastUpdateTime = new Date().getTime(),
start = s.querySelector('button.start'),
stop = s.querySelector('button.stop'),
reset = s.querySelector('button.reset'),
mins = s.querySelector('span.minutes'),
secs = s.querySelector('span.seconds'),
cents = s.querySelector('span.centiseconds');
start.addEventListener('click', startTimer);
stop.addEventListener('click', stopTimer);
reset.addEventListener('click', resetTimer);
function pad (n) {
return ('00' + n).substr(-2);
}
function update () {
var now = new Date().getTime(),
dt = now - lastUpdateTime;
currentTimer += dt;
var time = new Date(currentTimer);
mins.innerHTML = pad(time.getMinutes());
secs.innerHTML = pad(time.getSeconds());
cents.innerHTML = pad(Math.floor(time.getMilliseconds() / 10));
lastUpdateTime = now;
}
function startTimer () {
if (!interval) {
lastUpdateTime = new Date().getTime();
interval = setInterval(update, 1);
}
}
function stopTimer () {
clearInterval(interval);
interval = 0;
}
function resetTimer () {
stopTimer();
currentTimer = 0;
mins.innerHTML = secs.innerHTML = cents.innerHTML = pad(0);
}
});
$(document).ready(function(){
$(".stop").click(function(){
var m = $('#min').html();
var s = $('#sec').html();
var ms = $('#msec').html();
console.log(m,s,ms);
});
});
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Stopwatch</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
</head>
<body>
<div class="stopwatch">
<div class="controls">
<button class="start">Start</button>
<button id="stp" class="stop">Stop</button>
<button class="reset">Reset</button>
</div>
<div class="display">
<span id="min" class="minutes" value="">00</span><span id="sec" class="seconds" value="">00</span><span id="msec" class="centiseconds" value="">00</span>
</div>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<script src="watch/stopwatch.js"></script>
<script>
</script>
</body>
</html>
javascript jquery html
I have a stopwatch on my website I would like to take the result after the stop button is clicked and convert it into seconds.
is it possible to calculate the given time and turn it into seconds only with javascript or jquery?
after the convert, I would like to send the value into my database
I can see the result in my console
var ss = document.getElementsByClassName('stopwatch');
.forEach.call(ss, function (s) {
var currentTimer = 0,
interval = 0,
lastUpdateTime = new Date().getTime(),
start = s.querySelector('button.start'),
stop = s.querySelector('button.stop'),
reset = s.querySelector('button.reset'),
mins = s.querySelector('span.minutes'),
secs = s.querySelector('span.seconds'),
cents = s.querySelector('span.centiseconds');
start.addEventListener('click', startTimer);
stop.addEventListener('click', stopTimer);
reset.addEventListener('click', resetTimer);
function pad (n) {
return ('00' + n).substr(-2);
}
function update () {
var now = new Date().getTime(),
dt = now - lastUpdateTime;
currentTimer += dt;
var time = new Date(currentTimer);
mins.innerHTML = pad(time.getMinutes());
secs.innerHTML = pad(time.getSeconds());
cents.innerHTML = pad(Math.floor(time.getMilliseconds() / 10));
lastUpdateTime = now;
}
function startTimer () {
if (!interval) {
lastUpdateTime = new Date().getTime();
interval = setInterval(update, 1);
}
}
function stopTimer () {
clearInterval(interval);
interval = 0;
}
function resetTimer () {
stopTimer();
currentTimer = 0;
mins.innerHTML = secs.innerHTML = cents.innerHTML = pad(0);
}
});
$(document).ready(function(){
$(".stop").click(function(){
var m = $('#min').html();
var s = $('#sec').html();
var ms = $('#msec').html();
console.log(m,s,ms);
});
});
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Stopwatch</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
</head>
<body>
<div class="stopwatch">
<div class="controls">
<button class="start">Start</button>
<button id="stp" class="stop">Stop</button>
<button class="reset">Reset</button>
</div>
<div class="display">
<span id="min" class="minutes" value="">00</span><span id="sec" class="seconds" value="">00</span><span id="msec" class="centiseconds" value="">00</span>
</div>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<script src="watch/stopwatch.js"></script>
<script>
</script>
</body>
</html>
var ss = document.getElementsByClassName('stopwatch');
.forEach.call(ss, function (s) {
var currentTimer = 0,
interval = 0,
lastUpdateTime = new Date().getTime(),
start = s.querySelector('button.start'),
stop = s.querySelector('button.stop'),
reset = s.querySelector('button.reset'),
mins = s.querySelector('span.minutes'),
secs = s.querySelector('span.seconds'),
cents = s.querySelector('span.centiseconds');
start.addEventListener('click', startTimer);
stop.addEventListener('click', stopTimer);
reset.addEventListener('click', resetTimer);
function pad (n) {
return ('00' + n).substr(-2);
}
function update () {
var now = new Date().getTime(),
dt = now - lastUpdateTime;
currentTimer += dt;
var time = new Date(currentTimer);
mins.innerHTML = pad(time.getMinutes());
secs.innerHTML = pad(time.getSeconds());
cents.innerHTML = pad(Math.floor(time.getMilliseconds() / 10));
lastUpdateTime = now;
}
function startTimer () {
if (!interval) {
lastUpdateTime = new Date().getTime();
interval = setInterval(update, 1);
}
}
function stopTimer () {
clearInterval(interval);
interval = 0;
}
function resetTimer () {
stopTimer();
currentTimer = 0;
mins.innerHTML = secs.innerHTML = cents.innerHTML = pad(0);
}
});
$(document).ready(function(){
$(".stop").click(function(){
var m = $('#min').html();
var s = $('#sec').html();
var ms = $('#msec').html();
console.log(m,s,ms);
});
});
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Stopwatch</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
</head>
<body>
<div class="stopwatch">
<div class="controls">
<button class="start">Start</button>
<button id="stp" class="stop">Stop</button>
<button class="reset">Reset</button>
</div>
<div class="display">
<span id="min" class="minutes" value="">00</span><span id="sec" class="seconds" value="">00</span><span id="msec" class="centiseconds" value="">00</span>
</div>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<script src="watch/stopwatch.js"></script>
<script>
</script>
</body>
</html>
var ss = document.getElementsByClassName('stopwatch');
.forEach.call(ss, function (s) {
var currentTimer = 0,
interval = 0,
lastUpdateTime = new Date().getTime(),
start = s.querySelector('button.start'),
stop = s.querySelector('button.stop'),
reset = s.querySelector('button.reset'),
mins = s.querySelector('span.minutes'),
secs = s.querySelector('span.seconds'),
cents = s.querySelector('span.centiseconds');
start.addEventListener('click', startTimer);
stop.addEventListener('click', stopTimer);
reset.addEventListener('click', resetTimer);
function pad (n) {
return ('00' + n).substr(-2);
}
function update () {
var now = new Date().getTime(),
dt = now - lastUpdateTime;
currentTimer += dt;
var time = new Date(currentTimer);
mins.innerHTML = pad(time.getMinutes());
secs.innerHTML = pad(time.getSeconds());
cents.innerHTML = pad(Math.floor(time.getMilliseconds() / 10));
lastUpdateTime = now;
}
function startTimer () {
if (!interval) {
lastUpdateTime = new Date().getTime();
interval = setInterval(update, 1);
}
}
function stopTimer () {
clearInterval(interval);
interval = 0;
}
function resetTimer () {
stopTimer();
currentTimer = 0;
mins.innerHTML = secs.innerHTML = cents.innerHTML = pad(0);
}
});
$(document).ready(function(){
$(".stop").click(function(){
var m = $('#min').html();
var s = $('#sec').html();
var ms = $('#msec').html();
console.log(m,s,ms);
});
});
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Stopwatch</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
</head>
<body>
<div class="stopwatch">
<div class="controls">
<button class="start">Start</button>
<button id="stp" class="stop">Stop</button>
<button class="reset">Reset</button>
</div>
<div class="display">
<span id="min" class="minutes" value="">00</span><span id="sec" class="seconds" value="">00</span><span id="msec" class="centiseconds" value="">00</span>
</div>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<script src="watch/stopwatch.js"></script>
<script>
</script>
</body>
</html>
javascript jquery html
javascript jquery html
edited Nov 24 '18 at 21:07
Jack Bashford
10.7k31643
10.7k31643
asked Nov 24 '18 at 19:26


dror shalitdror shalit
748
748
You should add HTML as well so we can debug it.
– Cata John
Nov 24 '18 at 19:32
i have updated the post
– dror shalit
Nov 24 '18 at 19:43
I'm not sure I understand where do you have difficulties here? You already have the value of span elements for minutes, seconds and milliseconds. Can't you just take those values and store them in a new variable? Was that the question? If you need a way to do this, let me know and I'll write the code. Btw, how do you intend to send/store milliseconds on the server? In the form of floating number? For example: 19.34s?
– Nemanja Glumac
Nov 24 '18 at 19:51
1
1 millisecond = 0.001 seconds
so go ahead and apply this to your code and it should all work.
– Cata John
Nov 24 '18 at 19:52
Btw, ID is called "msec" (millisecond = 1/1000s), but the class on the same element is centisecond which is 1/100s. You should probably fix that.
– Nemanja Glumac
Nov 24 '18 at 19:56
|
show 3 more comments
You should add HTML as well so we can debug it.
– Cata John
Nov 24 '18 at 19:32
i have updated the post
– dror shalit
Nov 24 '18 at 19:43
I'm not sure I understand where do you have difficulties here? You already have the value of span elements for minutes, seconds and milliseconds. Can't you just take those values and store them in a new variable? Was that the question? If you need a way to do this, let me know and I'll write the code. Btw, how do you intend to send/store milliseconds on the server? In the form of floating number? For example: 19.34s?
– Nemanja Glumac
Nov 24 '18 at 19:51
1
1 millisecond = 0.001 seconds
so go ahead and apply this to your code and it should all work.
– Cata John
Nov 24 '18 at 19:52
Btw, ID is called "msec" (millisecond = 1/1000s), but the class on the same element is centisecond which is 1/100s. You should probably fix that.
– Nemanja Glumac
Nov 24 '18 at 19:56
You should add HTML as well so we can debug it.
– Cata John
Nov 24 '18 at 19:32
You should add HTML as well so we can debug it.
– Cata John
Nov 24 '18 at 19:32
i have updated the post
– dror shalit
Nov 24 '18 at 19:43
i have updated the post
– dror shalit
Nov 24 '18 at 19:43
I'm not sure I understand where do you have difficulties here? You already have the value of span elements for minutes, seconds and milliseconds. Can't you just take those values and store them in a new variable? Was that the question? If you need a way to do this, let me know and I'll write the code. Btw, how do you intend to send/store milliseconds on the server? In the form of floating number? For example: 19.34s?
– Nemanja Glumac
Nov 24 '18 at 19:51
I'm not sure I understand where do you have difficulties here? You already have the value of span elements for minutes, seconds and milliseconds. Can't you just take those values and store them in a new variable? Was that the question? If you need a way to do this, let me know and I'll write the code. Btw, how do you intend to send/store milliseconds on the server? In the form of floating number? For example: 19.34s?
– Nemanja Glumac
Nov 24 '18 at 19:51
1
1
1 millisecond = 0.001 seconds
so go ahead and apply this to your code and it should all work.– Cata John
Nov 24 '18 at 19:52
1 millisecond = 0.001 seconds
so go ahead and apply this to your code and it should all work.– Cata John
Nov 24 '18 at 19:52
Btw, ID is called "msec" (millisecond = 1/1000s), but the class on the same element is centisecond which is 1/100s. You should probably fix that.
– Nemanja Glumac
Nov 24 '18 at 19:56
Btw, ID is called "msec" (millisecond = 1/1000s), but the class on the same element is centisecond which is 1/100s. You should probably fix that.
– Nemanja Glumac
Nov 24 '18 at 19:56
|
show 3 more comments
2 Answers
2
active
oldest
votes
Taking your second function (the one logging the values), you can just perform some simple mathematics to get a total number of seconds:
$(document).ready(function(){
$(".stop").click(function(){
var m = $('#min').html();
var s = $('#sec').html();
var ms = $('#msec').html();
console.log(m,s,ms);
var total = (parseInt(m) * 60) + parseInt(s);
console.log(total + "." + ms);
});
});
add a comment |
If I understand you correctly there are 2 questions:
1) How to calculate time and show it? And you already calculated time in seconds, so to display it properly just change HTML to:
<span>Min: </span><span id="min" class="minutes" value="">00</span><span> Sec: </span><span id="sec" class="seconds" value="">00</span><span> Msec: </span><span id="msec" class="centiseconds" value="">00</span>
2) To pass data from js to your "database" (presume that you actually mean server side) you can use ajax (https://api.jquery.com/category/ajax/)
Pass variable string from javascript to php using ajax
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53461643%2fconvert-stop-watch-time-to-seconds%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Taking your second function (the one logging the values), you can just perform some simple mathematics to get a total number of seconds:
$(document).ready(function(){
$(".stop").click(function(){
var m = $('#min').html();
var s = $('#sec').html();
var ms = $('#msec').html();
console.log(m,s,ms);
var total = (parseInt(m) * 60) + parseInt(s);
console.log(total + "." + ms);
});
});
add a comment |
Taking your second function (the one logging the values), you can just perform some simple mathematics to get a total number of seconds:
$(document).ready(function(){
$(".stop").click(function(){
var m = $('#min').html();
var s = $('#sec').html();
var ms = $('#msec').html();
console.log(m,s,ms);
var total = (parseInt(m) * 60) + parseInt(s);
console.log(total + "." + ms);
});
});
add a comment |
Taking your second function (the one logging the values), you can just perform some simple mathematics to get a total number of seconds:
$(document).ready(function(){
$(".stop").click(function(){
var m = $('#min').html();
var s = $('#sec').html();
var ms = $('#msec').html();
console.log(m,s,ms);
var total = (parseInt(m) * 60) + parseInt(s);
console.log(total + "." + ms);
});
});
Taking your second function (the one logging the values), you can just perform some simple mathematics to get a total number of seconds:
$(document).ready(function(){
$(".stop").click(function(){
var m = $('#min').html();
var s = $('#sec').html();
var ms = $('#msec').html();
console.log(m,s,ms);
var total = (parseInt(m) * 60) + parseInt(s);
console.log(total + "." + ms);
});
});
answered Nov 24 '18 at 21:12
Jack BashfordJack Bashford
10.7k31643
10.7k31643
add a comment |
add a comment |
If I understand you correctly there are 2 questions:
1) How to calculate time and show it? And you already calculated time in seconds, so to display it properly just change HTML to:
<span>Min: </span><span id="min" class="minutes" value="">00</span><span> Sec: </span><span id="sec" class="seconds" value="">00</span><span> Msec: </span><span id="msec" class="centiseconds" value="">00</span>
2) To pass data from js to your "database" (presume that you actually mean server side) you can use ajax (https://api.jquery.com/category/ajax/)
Pass variable string from javascript to php using ajax
add a comment |
If I understand you correctly there are 2 questions:
1) How to calculate time and show it? And you already calculated time in seconds, so to display it properly just change HTML to:
<span>Min: </span><span id="min" class="minutes" value="">00</span><span> Sec: </span><span id="sec" class="seconds" value="">00</span><span> Msec: </span><span id="msec" class="centiseconds" value="">00</span>
2) To pass data from js to your "database" (presume that you actually mean server side) you can use ajax (https://api.jquery.com/category/ajax/)
Pass variable string from javascript to php using ajax
add a comment |
If I understand you correctly there are 2 questions:
1) How to calculate time and show it? And you already calculated time in seconds, so to display it properly just change HTML to:
<span>Min: </span><span id="min" class="minutes" value="">00</span><span> Sec: </span><span id="sec" class="seconds" value="">00</span><span> Msec: </span><span id="msec" class="centiseconds" value="">00</span>
2) To pass data from js to your "database" (presume that you actually mean server side) you can use ajax (https://api.jquery.com/category/ajax/)
Pass variable string from javascript to php using ajax
If I understand you correctly there are 2 questions:
1) How to calculate time and show it? And you already calculated time in seconds, so to display it properly just change HTML to:
<span>Min: </span><span id="min" class="minutes" value="">00</span><span> Sec: </span><span id="sec" class="seconds" value="">00</span><span> Msec: </span><span id="msec" class="centiseconds" value="">00</span>
2) To pass data from js to your "database" (presume that you actually mean server side) you can use ajax (https://api.jquery.com/category/ajax/)
Pass variable string from javascript to php using ajax
edited Nov 25 '18 at 19:23
answered Nov 24 '18 at 21:04
Pavel IvanovPavel Ivanov
214
214
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53461643%2fconvert-stop-watch-time-to-seconds%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
1tUrKZh,D0Ha7BH,u8NPMXVK48q,LRd
You should add HTML as well so we can debug it.
– Cata John
Nov 24 '18 at 19:32
i have updated the post
– dror shalit
Nov 24 '18 at 19:43
I'm not sure I understand where do you have difficulties here? You already have the value of span elements for minutes, seconds and milliseconds. Can't you just take those values and store them in a new variable? Was that the question? If you need a way to do this, let me know and I'll write the code. Btw, how do you intend to send/store milliseconds on the server? In the form of floating number? For example: 19.34s?
– Nemanja Glumac
Nov 24 '18 at 19:51
1
1 millisecond = 0.001 seconds
so go ahead and apply this to your code and it should all work.– Cata John
Nov 24 '18 at 19:52
Btw, ID is called "msec" (millisecond = 1/1000s), but the class on the same element is centisecond which is 1/100s. You should probably fix that.
– Nemanja Glumac
Nov 24 '18 at 19:56