Get the Last Inserted Id Using Laravel Eloquent
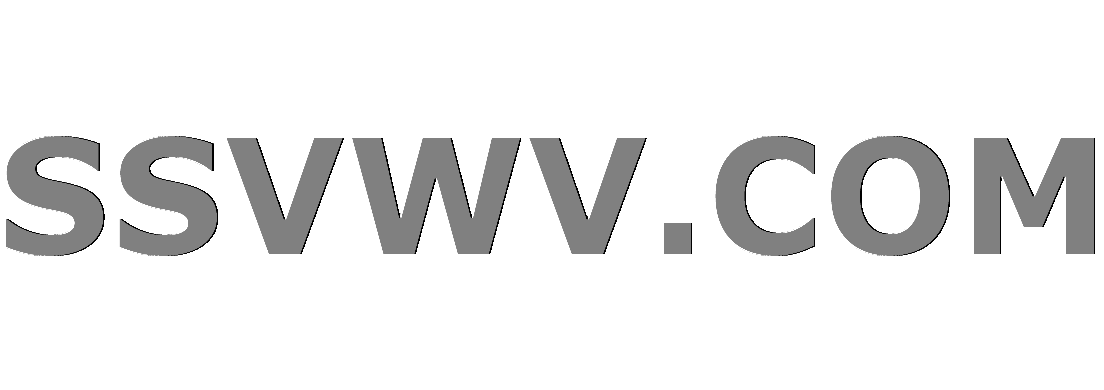
Multi tool use
I'm currently using the below code to insert data in a table:
<?php
public function saveDetailsCompany()
{
$post = Input::All();
$data = new Company;
$data->nombre = $post['name'];
$data->direccion = $post['address'];
$data->telefono = $post['phone'];
$data->email = $post['email'];
$data->giro = $post['type'];
$data->fecha_registro = date("Y-m-d H:i:s");
$data->fecha_modificacion = date("Y-m-d H:i:s");
if ($data->save()) {
return Response::json(array('success' => true), 200);
}
}
I want to return the last ID inserted but I don't know how to get it.
Kind regards!
php database laravel eloquent
add a comment |
I'm currently using the below code to insert data in a table:
<?php
public function saveDetailsCompany()
{
$post = Input::All();
$data = new Company;
$data->nombre = $post['name'];
$data->direccion = $post['address'];
$data->telefono = $post['phone'];
$data->email = $post['email'];
$data->giro = $post['type'];
$data->fecha_registro = date("Y-m-d H:i:s");
$data->fecha_modificacion = date("Y-m-d H:i:s");
if ($data->save()) {
return Response::json(array('success' => true), 200);
}
}
I want to return the last ID inserted but I don't know how to get it.
Kind regards!
php database laravel eloquent
add a comment |
I'm currently using the below code to insert data in a table:
<?php
public function saveDetailsCompany()
{
$post = Input::All();
$data = new Company;
$data->nombre = $post['name'];
$data->direccion = $post['address'];
$data->telefono = $post['phone'];
$data->email = $post['email'];
$data->giro = $post['type'];
$data->fecha_registro = date("Y-m-d H:i:s");
$data->fecha_modificacion = date("Y-m-d H:i:s");
if ($data->save()) {
return Response::json(array('success' => true), 200);
}
}
I want to return the last ID inserted but I don't know how to get it.
Kind regards!
php database laravel eloquent
I'm currently using the below code to insert data in a table:
<?php
public function saveDetailsCompany()
{
$post = Input::All();
$data = new Company;
$data->nombre = $post['name'];
$data->direccion = $post['address'];
$data->telefono = $post['phone'];
$data->email = $post['email'];
$data->giro = $post['type'];
$data->fecha_registro = date("Y-m-d H:i:s");
$data->fecha_modificacion = date("Y-m-d H:i:s");
if ($data->save()) {
return Response::json(array('success' => true), 200);
}
}
I want to return the last ID inserted but I don't know how to get it.
Kind regards!
php database laravel eloquent
php database laravel eloquent
edited Nov 20 at 7:08
Blaze
4,2621628
4,2621628
asked Jan 13 '14 at 6:02


SoldierCorp
2,722104480
2,722104480
add a comment |
add a comment |
24 Answers
24
active
oldest
votes
After save
, $data->id
should be the last id inserted.
return Response::json(array('success' => true, 'last_insert_id' => $data->id), 200);
For updated laravel version try this
return response()->json(array('success' => true, 'last_insert_id' => $data->id), 200);
1
An object always returns an object, ofc. This is the only way to go.
– Cas Bloem
Nov 26 '14 at 14:11
27
Beware that if the id is NOT autoincrement, this will always return0
. In my case the id was a string (UUID) and for this to work I had to addpublic $incrementing = false;
in my model.
– Luís Cruz
Apr 20 '15 at 17:18
2
@milz I have MySQL trigger that generate the uuid for a custom field namedaid
and I have set$incrementing = false;
but It does not returned too!
– SaidbakR
Apr 8 '17 at 13:29
add a comment |
xdazz is right in this case, but for the benefit of future visitors who might be using DB::statement
or DB::insert
, there is another way:
DB::getPdo()->lastInsertId();
26
Actually you can do it right in the insert$id = DB::table('someTable')->insertGetId( ['field' => Input['data']);
– Casey
May 15 '14 at 15:05
1
@Casey doing it this way will not update timestamps in the DB
– Rafael
Dec 7 '14 at 4:30
@Rafael, if you want to updatetimestamps
usinginsertGetId
, kindly check here
– Frank Myat Thu
May 3 '16 at 7:54
Exactly what I was looking for the other day! Also,insertGetId
only works if the id columns is actually called "id".
– Captain Hypertext
Dec 17 '16 at 1:42
@Benubird, I have got my solution according your answer.
– Bhavin Thummar
Aug 28 at 7:29
add a comment |
For anyone who also likes how Jeffrey Way uses Model::create()
in his Laracasts 5 tutorials, where he just sends the Request straight into the database without explicitly setting each field in the controller, and using the model's $fillable
for mass assignment (very important, for anyone new and using this way): I read a lot of people using insertGetId()
but unfortunately this does not respect the $fillable
whitelist so you'll get errors with it trying to insert _token and anything that isn't a field in the database, end up setting things you want to filter, etc. That bummed me out, because I want to use mass assignment and overall write less code when possible. Fortunately Eloquent's create
method just wraps the save method (what @xdazz cited above), so you can still pull the last created ID...
public function store() {
$input = Request::all();
$id = Company::create($input)->id;
return redirect('company/'.$id);
}
2
This example didn't work for me in 5.1, but this did:$new = Company::create($input);
return redirect('company/'.$new->id);
– timgavin
Sep 19 '15 at 18:15
This assumes that the request fields name are the same as their respective database columns. Which is not always the case ( legacy codes for example)..
– mosid
Nov 30 '17 at 4:47
add a comment |
If the table has an auto-incrementing id, use the insertGetId method to insert a record and then retrieve the ID:
$id = DB::table('users')->insertGetId([
'email' => 'john@example.com',
'votes' => 0
]);
Refer: https://laravel.com/docs/5.1/queries#inserts
What you described looks like capturing last insert using Fluent. Question was about Eloquent. It would look more like: $id = Model::create('votes' => 0])->id; As described in this answer above: stackoverflow.com/a/21084888/436443
– Jeffz
Jul 10 at 22:02
add a comment |
**** For Laravel ****
Firstly create an object, Then set attributes value for that object, Then save the object record, and then get the last inserted id. such as
$user = new User();
$user->name = 'John';
$user->save();
// Now Getting The Last inserted id
$insertedId = $user->id;
echo $insertedId ;
add a comment |
In laravel 5: you can do this:
use AppHttpRequestsUserStoreRequest;
class UserController extends Controller {
private $user;
public function __construct( User $user )
{
$this->user = $user;
}
public function store( UserStoreRequest $request )
{
$user= $this->user->create([
'name' => $request['name'],
'email' => $request['email'],
'password' => Hash::make($request['password'])
]);
$lastInsertedId= $user->id;
}
}
add a comment |
Here's an example:
public static function saveTutorial(){
$data = Input::all();
$Tut = new Tutorial;
$Tut->title = $data['title'];
$Tut->tutorial = $data['tutorial'];
$Tut->save();
$LastInsertId = $Tut->id;
return Response::json(array('success' => true,'last_id'=>$LastInsertId), 200);
}
add a comment |
This worked for me in laravel 4.2
$id = User::insertGetId([
'username' => Input::get('username'),
'password' => Hash::make('password'),
'active' => 0
]);
add a comment |
Here is how we can get last inserted id in Laravel 4
public function store()
{
$input = Input::all();
$validation = Validator::make($input, user::$rules);
if ($validation->passes())
{
$user= $this->user->create(array(
'name' => Input::get('name'),
'email' => Input::get('email'),
'password' => Hash::make(Input::get('password')),
));
$lastInsertedId= $user->id; //get last inserted record's user id value
$userId= array('user_id'=>$lastInsertedId); //put this value equal to datatable column name where it will be saved
$user->update($userId); //update newly created record by storing the value of last inserted id
return Redirect::route('users.index');
}
return Redirect::route('users.create')->withInput()->withErrors($validation)->with('message', 'There were validation errors.');
}
add a comment |
Use insertGetId
to insert and get inserted id
at the same time
From doc
If the table has an auto-incrementing id, use the insertGetId method
to insert a record and then retrieve the ID:
By Model
$id = Model::insertGetId(["name"=>"Niklesh","email"=>"myemail@gmail.com"]);
By DB
$id = DB::table('users')->insertGetId(["name"=>"Niklesh","email"=>"myemail@gmail.com"]);
For more details : https://laravel.com/docs/5.5/queries#inserts
add a comment |
After saving model, the initialized instance has the id:
$report = new Report();
$report->user_id = $request->user_id;
$report->patient_id = $request->patient_id;
$report->diseases_id = $request->modality;
$isReportCreated = $report->save();
return $report->id; // this will return the saved report id
add a comment |
After saving a record in database, you can access id by $data->id
return Response::json(['success' => true, 'last_insert_id' => $data->id], 200)
add a comment |
In Laravel 5.2 i would make it as clean as possible:
public function saveContact(Request $request, Contact $contact)
{
$create = $contact->create($request->all());
return response()->json($create->id, 201);
}
add a comment |
For Laravel, If you insert a new record and call $data->save()
this function executes an INSERT query and returns the primary key value (i.e. id by default).
You can use following code:
if($data->save()) {
return Response::json(array('status' => 1, 'primary_id'=>$data->id), 200);
}
add a comment |
After
$data->save()
$data->id
will give you the inserted id,
Note: If your autoincrement column name is sno then you should use
$data->sno
and not $data->id
add a comment |
You can do this:
$result=app('db')->insert("INSERT INTO table...");
$lastInsertId=app('db')->getPdo()->lastInsertId();
add a comment |
$objPost = new Post;
$objPost->title = 'Title';
$objPost->description = 'Description';
$objPost->save();
$recId = $objPost->id; // If Id in table column name if other then id then user the other column name
return Response::json(['success' => true,'id' => $recId], 200);
add a comment |
For insert()
Example:
$data1 = array(
'company_id' => $company_id,
'branch_id' => $branch_id
);
$insert_id = CreditVoucher::insert($data1);
$id = DB::getPdo()->lastInsertId();
dd($id);
add a comment |
Using Eloquent Model
$user = new Report();
$user->email= 'johndoe@example.com';
$user->save();
$lastId = $user->id;
Using Query Builder
$lastId = DB::table('reports')->insertGetId(['email' => 'johndoe@example.com']);
add a comment |
For get last inserted id in database
You can use
$data = new YourModelName;
$data->name = 'Some Value';
$data->email = 'abc@mail.com';
$data->save();
$lastInsertedId = $data->id;
here $lastInsertedId will gives you last inserted auto increment id.
add a comment |
After Saving $data->save()
. all data is pushed inside $data
. As this is an object and the current row is just saved recently inside $data
. so last insertId
will be found inside $data->id
.
Response code will be:
return Response::json(array('success' => true, 'last_insert_id' => $data->id), 200);
add a comment |
The shortest way is probably a call of the refresh()
on the model:
public function create(array $data): MyModel
{
$myModel = new MyModel($dataArray);
$myModel->saveOrFail();
return $myModel->refresh();
}
add a comment |
public function store( UserStoreRequest $request ) {
$input = $request->all();
$user = User::create($input);
$userId=$user->id
}
2
This post was answered 3 years ago. Please edit your answer to add more explanation as to why it might help the user or how its helps solves the OP's question in a better way.
– Syfer
Sep 8 '17 at 11:42
1
Thank you for this code snippet, which may provide some immediate help. A proper explanation would greatly improve its educational value by showing why this is a good solution to the problem, and would make it more useful to future readers with similar, but not identical, questions. Please edit your answer to add explanation, and give an indication of what limitations and assumptions apply. Not to mention the age of the question and the low quality of your answer.
– GrumpyCrouton
Sep 8 '17 at 15:53
add a comment |
Using Eloquent Model
use AppCompany;
public function saveDetailsCompany(Request $request)
{
$createcompany=Company::create(['nombre'=>$request->input('name'),'direccion'=>$request->input('address'),'telefono'=>$request->input('phone'),'email'=>$request->input('emaile'),'giro'=>$request->input('type')]);
// Last Inserted Row ID
echo $createcompany->id;
}
Using Query Builder
$createcompany=DB::table('company')->create(['nombre'=>$request->input('name'),'direccion'=>$request->input('address'),'telefono'=>$request->input('phone'),'email'=>$request->input('emaile'),'giro'=>$request->input('type')]);
echo $createcompany->id;
For more methods to get Last Inserted Row id in Laravel : http://phpnotebook.com/95-laravel/127-3-methods-to-get-last-inserted-row-id-in-laravel
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f21084833%2fget-the-last-inserted-id-using-laravel-eloquent%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
24 Answers
24
active
oldest
votes
24 Answers
24
active
oldest
votes
active
oldest
votes
active
oldest
votes
After save
, $data->id
should be the last id inserted.
return Response::json(array('success' => true, 'last_insert_id' => $data->id), 200);
For updated laravel version try this
return response()->json(array('success' => true, 'last_insert_id' => $data->id), 200);
1
An object always returns an object, ofc. This is the only way to go.
– Cas Bloem
Nov 26 '14 at 14:11
27
Beware that if the id is NOT autoincrement, this will always return0
. In my case the id was a string (UUID) and for this to work I had to addpublic $incrementing = false;
in my model.
– Luís Cruz
Apr 20 '15 at 17:18
2
@milz I have MySQL trigger that generate the uuid for a custom field namedaid
and I have set$incrementing = false;
but It does not returned too!
– SaidbakR
Apr 8 '17 at 13:29
add a comment |
After save
, $data->id
should be the last id inserted.
return Response::json(array('success' => true, 'last_insert_id' => $data->id), 200);
For updated laravel version try this
return response()->json(array('success' => true, 'last_insert_id' => $data->id), 200);
1
An object always returns an object, ofc. This is the only way to go.
– Cas Bloem
Nov 26 '14 at 14:11
27
Beware that if the id is NOT autoincrement, this will always return0
. In my case the id was a string (UUID) and for this to work I had to addpublic $incrementing = false;
in my model.
– Luís Cruz
Apr 20 '15 at 17:18
2
@milz I have MySQL trigger that generate the uuid for a custom field namedaid
and I have set$incrementing = false;
but It does not returned too!
– SaidbakR
Apr 8 '17 at 13:29
add a comment |
After save
, $data->id
should be the last id inserted.
return Response::json(array('success' => true, 'last_insert_id' => $data->id), 200);
For updated laravel version try this
return response()->json(array('success' => true, 'last_insert_id' => $data->id), 200);
After save
, $data->id
should be the last id inserted.
return Response::json(array('success' => true, 'last_insert_id' => $data->id), 200);
For updated laravel version try this
return response()->json(array('success' => true, 'last_insert_id' => $data->id), 200);
edited Sep 23 at 14:33


Samiron Barai
34
34
answered Jan 13 '14 at 6:06
xdazz
132k26198231
132k26198231
1
An object always returns an object, ofc. This is the only way to go.
– Cas Bloem
Nov 26 '14 at 14:11
27
Beware that if the id is NOT autoincrement, this will always return0
. In my case the id was a string (UUID) and for this to work I had to addpublic $incrementing = false;
in my model.
– Luís Cruz
Apr 20 '15 at 17:18
2
@milz I have MySQL trigger that generate the uuid for a custom field namedaid
and I have set$incrementing = false;
but It does not returned too!
– SaidbakR
Apr 8 '17 at 13:29
add a comment |
1
An object always returns an object, ofc. This is the only way to go.
– Cas Bloem
Nov 26 '14 at 14:11
27
Beware that if the id is NOT autoincrement, this will always return0
. In my case the id was a string (UUID) and for this to work I had to addpublic $incrementing = false;
in my model.
– Luís Cruz
Apr 20 '15 at 17:18
2
@milz I have MySQL trigger that generate the uuid for a custom field namedaid
and I have set$incrementing = false;
but It does not returned too!
– SaidbakR
Apr 8 '17 at 13:29
1
1
An object always returns an object, ofc. This is the only way to go.
– Cas Bloem
Nov 26 '14 at 14:11
An object always returns an object, ofc. This is the only way to go.
– Cas Bloem
Nov 26 '14 at 14:11
27
27
Beware that if the id is NOT autoincrement, this will always return
0
. In my case the id was a string (UUID) and for this to work I had to add public $incrementing = false;
in my model.– Luís Cruz
Apr 20 '15 at 17:18
Beware that if the id is NOT autoincrement, this will always return
0
. In my case the id was a string (UUID) and for this to work I had to add public $incrementing = false;
in my model.– Luís Cruz
Apr 20 '15 at 17:18
2
2
@milz I have MySQL trigger that generate the uuid for a custom field named
aid
and I have set $incrementing = false;
but It does not returned too!– SaidbakR
Apr 8 '17 at 13:29
@milz I have MySQL trigger that generate the uuid for a custom field named
aid
and I have set $incrementing = false;
but It does not returned too!– SaidbakR
Apr 8 '17 at 13:29
add a comment |
xdazz is right in this case, but for the benefit of future visitors who might be using DB::statement
or DB::insert
, there is another way:
DB::getPdo()->lastInsertId();
26
Actually you can do it right in the insert$id = DB::table('someTable')->insertGetId( ['field' => Input['data']);
– Casey
May 15 '14 at 15:05
1
@Casey doing it this way will not update timestamps in the DB
– Rafael
Dec 7 '14 at 4:30
@Rafael, if you want to updatetimestamps
usinginsertGetId
, kindly check here
– Frank Myat Thu
May 3 '16 at 7:54
Exactly what I was looking for the other day! Also,insertGetId
only works if the id columns is actually called "id".
– Captain Hypertext
Dec 17 '16 at 1:42
@Benubird, I have got my solution according your answer.
– Bhavin Thummar
Aug 28 at 7:29
add a comment |
xdazz is right in this case, but for the benefit of future visitors who might be using DB::statement
or DB::insert
, there is another way:
DB::getPdo()->lastInsertId();
26
Actually you can do it right in the insert$id = DB::table('someTable')->insertGetId( ['field' => Input['data']);
– Casey
May 15 '14 at 15:05
1
@Casey doing it this way will not update timestamps in the DB
– Rafael
Dec 7 '14 at 4:30
@Rafael, if you want to updatetimestamps
usinginsertGetId
, kindly check here
– Frank Myat Thu
May 3 '16 at 7:54
Exactly what I was looking for the other day! Also,insertGetId
only works if the id columns is actually called "id".
– Captain Hypertext
Dec 17 '16 at 1:42
@Benubird, I have got my solution according your answer.
– Bhavin Thummar
Aug 28 at 7:29
add a comment |
xdazz is right in this case, but for the benefit of future visitors who might be using DB::statement
or DB::insert
, there is another way:
DB::getPdo()->lastInsertId();
xdazz is right in this case, but for the benefit of future visitors who might be using DB::statement
or DB::insert
, there is another way:
DB::getPdo()->lastInsertId();
edited May 23 '17 at 12:18
Community♦
11
11
answered Apr 23 '14 at 10:33


Benubird
8,2251567112
8,2251567112
26
Actually you can do it right in the insert$id = DB::table('someTable')->insertGetId( ['field' => Input['data']);
– Casey
May 15 '14 at 15:05
1
@Casey doing it this way will not update timestamps in the DB
– Rafael
Dec 7 '14 at 4:30
@Rafael, if you want to updatetimestamps
usinginsertGetId
, kindly check here
– Frank Myat Thu
May 3 '16 at 7:54
Exactly what I was looking for the other day! Also,insertGetId
only works if the id columns is actually called "id".
– Captain Hypertext
Dec 17 '16 at 1:42
@Benubird, I have got my solution according your answer.
– Bhavin Thummar
Aug 28 at 7:29
add a comment |
26
Actually you can do it right in the insert$id = DB::table('someTable')->insertGetId( ['field' => Input['data']);
– Casey
May 15 '14 at 15:05
1
@Casey doing it this way will not update timestamps in the DB
– Rafael
Dec 7 '14 at 4:30
@Rafael, if you want to updatetimestamps
usinginsertGetId
, kindly check here
– Frank Myat Thu
May 3 '16 at 7:54
Exactly what I was looking for the other day! Also,insertGetId
only works if the id columns is actually called "id".
– Captain Hypertext
Dec 17 '16 at 1:42
@Benubird, I have got my solution according your answer.
– Bhavin Thummar
Aug 28 at 7:29
26
26
Actually you can do it right in the insert
$id = DB::table('someTable')->insertGetId( ['field' => Input['data']);
– Casey
May 15 '14 at 15:05
Actually you can do it right in the insert
$id = DB::table('someTable')->insertGetId( ['field' => Input['data']);
– Casey
May 15 '14 at 15:05
1
1
@Casey doing it this way will not update timestamps in the DB
– Rafael
Dec 7 '14 at 4:30
@Casey doing it this way will not update timestamps in the DB
– Rafael
Dec 7 '14 at 4:30
@Rafael, if you want to update
timestamps
using insertGetId
, kindly check here– Frank Myat Thu
May 3 '16 at 7:54
@Rafael, if you want to update
timestamps
using insertGetId
, kindly check here– Frank Myat Thu
May 3 '16 at 7:54
Exactly what I was looking for the other day! Also,
insertGetId
only works if the id columns is actually called "id".– Captain Hypertext
Dec 17 '16 at 1:42
Exactly what I was looking for the other day! Also,
insertGetId
only works if the id columns is actually called "id".– Captain Hypertext
Dec 17 '16 at 1:42
@Benubird, I have got my solution according your answer.
– Bhavin Thummar
Aug 28 at 7:29
@Benubird, I have got my solution according your answer.
– Bhavin Thummar
Aug 28 at 7:29
add a comment |
For anyone who also likes how Jeffrey Way uses Model::create()
in his Laracasts 5 tutorials, where he just sends the Request straight into the database without explicitly setting each field in the controller, and using the model's $fillable
for mass assignment (very important, for anyone new and using this way): I read a lot of people using insertGetId()
but unfortunately this does not respect the $fillable
whitelist so you'll get errors with it trying to insert _token and anything that isn't a field in the database, end up setting things you want to filter, etc. That bummed me out, because I want to use mass assignment and overall write less code when possible. Fortunately Eloquent's create
method just wraps the save method (what @xdazz cited above), so you can still pull the last created ID...
public function store() {
$input = Request::all();
$id = Company::create($input)->id;
return redirect('company/'.$id);
}
2
This example didn't work for me in 5.1, but this did:$new = Company::create($input);
return redirect('company/'.$new->id);
– timgavin
Sep 19 '15 at 18:15
This assumes that the request fields name are the same as their respective database columns. Which is not always the case ( legacy codes for example)..
– mosid
Nov 30 '17 at 4:47
add a comment |
For anyone who also likes how Jeffrey Way uses Model::create()
in his Laracasts 5 tutorials, where he just sends the Request straight into the database without explicitly setting each field in the controller, and using the model's $fillable
for mass assignment (very important, for anyone new and using this way): I read a lot of people using insertGetId()
but unfortunately this does not respect the $fillable
whitelist so you'll get errors with it trying to insert _token and anything that isn't a field in the database, end up setting things you want to filter, etc. That bummed me out, because I want to use mass assignment and overall write less code when possible. Fortunately Eloquent's create
method just wraps the save method (what @xdazz cited above), so you can still pull the last created ID...
public function store() {
$input = Request::all();
$id = Company::create($input)->id;
return redirect('company/'.$id);
}
2
This example didn't work for me in 5.1, but this did:$new = Company::create($input);
return redirect('company/'.$new->id);
– timgavin
Sep 19 '15 at 18:15
This assumes that the request fields name are the same as their respective database columns. Which is not always the case ( legacy codes for example)..
– mosid
Nov 30 '17 at 4:47
add a comment |
For anyone who also likes how Jeffrey Way uses Model::create()
in his Laracasts 5 tutorials, where he just sends the Request straight into the database without explicitly setting each field in the controller, and using the model's $fillable
for mass assignment (very important, for anyone new and using this way): I read a lot of people using insertGetId()
but unfortunately this does not respect the $fillable
whitelist so you'll get errors with it trying to insert _token and anything that isn't a field in the database, end up setting things you want to filter, etc. That bummed me out, because I want to use mass assignment and overall write less code when possible. Fortunately Eloquent's create
method just wraps the save method (what @xdazz cited above), so you can still pull the last created ID...
public function store() {
$input = Request::all();
$id = Company::create($input)->id;
return redirect('company/'.$id);
}
For anyone who also likes how Jeffrey Way uses Model::create()
in his Laracasts 5 tutorials, where he just sends the Request straight into the database without explicitly setting each field in the controller, and using the model's $fillable
for mass assignment (very important, for anyone new and using this way): I read a lot of people using insertGetId()
but unfortunately this does not respect the $fillable
whitelist so you'll get errors with it trying to insert _token and anything that isn't a field in the database, end up setting things you want to filter, etc. That bummed me out, because I want to use mass assignment and overall write less code when possible. Fortunately Eloquent's create
method just wraps the save method (what @xdazz cited above), so you can still pull the last created ID...
public function store() {
$input = Request::all();
$id = Company::create($input)->id;
return redirect('company/'.$id);
}
answered Jul 5 '15 at 10:20
dave
1,77821720
1,77821720
2
This example didn't work for me in 5.1, but this did:$new = Company::create($input);
return redirect('company/'.$new->id);
– timgavin
Sep 19 '15 at 18:15
This assumes that the request fields name are the same as their respective database columns. Which is not always the case ( legacy codes for example)..
– mosid
Nov 30 '17 at 4:47
add a comment |
2
This example didn't work for me in 5.1, but this did:$new = Company::create($input);
return redirect('company/'.$new->id);
– timgavin
Sep 19 '15 at 18:15
This assumes that the request fields name are the same as their respective database columns. Which is not always the case ( legacy codes for example)..
– mosid
Nov 30 '17 at 4:47
2
2
This example didn't work for me in 5.1, but this did:
$new = Company::create($input);
return redirect('company/'.$new->id);
– timgavin
Sep 19 '15 at 18:15
This example didn't work for me in 5.1, but this did:
$new = Company::create($input);
return redirect('company/'.$new->id);
– timgavin
Sep 19 '15 at 18:15
This assumes that the request fields name are the same as their respective database columns. Which is not always the case ( legacy codes for example)..
– mosid
Nov 30 '17 at 4:47
This assumes that the request fields name are the same as their respective database columns. Which is not always the case ( legacy codes for example)..
– mosid
Nov 30 '17 at 4:47
add a comment |
If the table has an auto-incrementing id, use the insertGetId method to insert a record and then retrieve the ID:
$id = DB::table('users')->insertGetId([
'email' => 'john@example.com',
'votes' => 0
]);
Refer: https://laravel.com/docs/5.1/queries#inserts
What you described looks like capturing last insert using Fluent. Question was about Eloquent. It would look more like: $id = Model::create('votes' => 0])->id; As described in this answer above: stackoverflow.com/a/21084888/436443
– Jeffz
Jul 10 at 22:02
add a comment |
If the table has an auto-incrementing id, use the insertGetId method to insert a record and then retrieve the ID:
$id = DB::table('users')->insertGetId([
'email' => 'john@example.com',
'votes' => 0
]);
Refer: https://laravel.com/docs/5.1/queries#inserts
What you described looks like capturing last insert using Fluent. Question was about Eloquent. It would look more like: $id = Model::create('votes' => 0])->id; As described in this answer above: stackoverflow.com/a/21084888/436443
– Jeffz
Jul 10 at 22:02
add a comment |
If the table has an auto-incrementing id, use the insertGetId method to insert a record and then retrieve the ID:
$id = DB::table('users')->insertGetId([
'email' => 'john@example.com',
'votes' => 0
]);
Refer: https://laravel.com/docs/5.1/queries#inserts
If the table has an auto-incrementing id, use the insertGetId method to insert a record and then retrieve the ID:
$id = DB::table('users')->insertGetId([
'email' => 'john@example.com',
'votes' => 0
]);
Refer: https://laravel.com/docs/5.1/queries#inserts
edited Aug 17 at 17:45


Barry
3,10571637
3,10571637
answered Jun 22 '16 at 9:34


Aamir
89711231
89711231
What you described looks like capturing last insert using Fluent. Question was about Eloquent. It would look more like: $id = Model::create('votes' => 0])->id; As described in this answer above: stackoverflow.com/a/21084888/436443
– Jeffz
Jul 10 at 22:02
add a comment |
What you described looks like capturing last insert using Fluent. Question was about Eloquent. It would look more like: $id = Model::create('votes' => 0])->id; As described in this answer above: stackoverflow.com/a/21084888/436443
– Jeffz
Jul 10 at 22:02
What you described looks like capturing last insert using Fluent. Question was about Eloquent. It would look more like: $id = Model::create('votes' => 0])->id; As described in this answer above: stackoverflow.com/a/21084888/436443
– Jeffz
Jul 10 at 22:02
What you described looks like capturing last insert using Fluent. Question was about Eloquent. It would look more like: $id = Model::create('votes' => 0])->id; As described in this answer above: stackoverflow.com/a/21084888/436443
– Jeffz
Jul 10 at 22:02
add a comment |
**** For Laravel ****
Firstly create an object, Then set attributes value for that object, Then save the object record, and then get the last inserted id. such as
$user = new User();
$user->name = 'John';
$user->save();
// Now Getting The Last inserted id
$insertedId = $user->id;
echo $insertedId ;
add a comment |
**** For Laravel ****
Firstly create an object, Then set attributes value for that object, Then save the object record, and then get the last inserted id. such as
$user = new User();
$user->name = 'John';
$user->save();
// Now Getting The Last inserted id
$insertedId = $user->id;
echo $insertedId ;
add a comment |
**** For Laravel ****
Firstly create an object, Then set attributes value for that object, Then save the object record, and then get the last inserted id. such as
$user = new User();
$user->name = 'John';
$user->save();
// Now Getting The Last inserted id
$insertedId = $user->id;
echo $insertedId ;
**** For Laravel ****
Firstly create an object, Then set attributes value for that object, Then save the object record, and then get the last inserted id. such as
$user = new User();
$user->name = 'John';
$user->save();
// Now Getting The Last inserted id
$insertedId = $user->id;
echo $insertedId ;
edited Jul 25 '16 at 6:16
answered May 28 '16 at 9:15


Majbah Habib
1,6471721
1,6471721
add a comment |
add a comment |
In laravel 5: you can do this:
use AppHttpRequestsUserStoreRequest;
class UserController extends Controller {
private $user;
public function __construct( User $user )
{
$this->user = $user;
}
public function store( UserStoreRequest $request )
{
$user= $this->user->create([
'name' => $request['name'],
'email' => $request['email'],
'password' => Hash::make($request['password'])
]);
$lastInsertedId= $user->id;
}
}
add a comment |
In laravel 5: you can do this:
use AppHttpRequestsUserStoreRequest;
class UserController extends Controller {
private $user;
public function __construct( User $user )
{
$this->user = $user;
}
public function store( UserStoreRequest $request )
{
$user= $this->user->create([
'name' => $request['name'],
'email' => $request['email'],
'password' => Hash::make($request['password'])
]);
$lastInsertedId= $user->id;
}
}
add a comment |
In laravel 5: you can do this:
use AppHttpRequestsUserStoreRequest;
class UserController extends Controller {
private $user;
public function __construct( User $user )
{
$this->user = $user;
}
public function store( UserStoreRequest $request )
{
$user= $this->user->create([
'name' => $request['name'],
'email' => $request['email'],
'password' => Hash::make($request['password'])
]);
$lastInsertedId= $user->id;
}
}
In laravel 5: you can do this:
use AppHttpRequestsUserStoreRequest;
class UserController extends Controller {
private $user;
public function __construct( User $user )
{
$this->user = $user;
}
public function store( UserStoreRequest $request )
{
$user= $this->user->create([
'name' => $request['name'],
'email' => $request['email'],
'password' => Hash::make($request['password'])
]);
$lastInsertedId= $user->id;
}
}
answered Feb 21 '15 at 7:48
Mujibur
47249
47249
add a comment |
add a comment |
Here's an example:
public static function saveTutorial(){
$data = Input::all();
$Tut = new Tutorial;
$Tut->title = $data['title'];
$Tut->tutorial = $data['tutorial'];
$Tut->save();
$LastInsertId = $Tut->id;
return Response::json(array('success' => true,'last_id'=>$LastInsertId), 200);
}
add a comment |
Here's an example:
public static function saveTutorial(){
$data = Input::all();
$Tut = new Tutorial;
$Tut->title = $data['title'];
$Tut->tutorial = $data['tutorial'];
$Tut->save();
$LastInsertId = $Tut->id;
return Response::json(array('success' => true,'last_id'=>$LastInsertId), 200);
}
add a comment |
Here's an example:
public static function saveTutorial(){
$data = Input::all();
$Tut = new Tutorial;
$Tut->title = $data['title'];
$Tut->tutorial = $data['tutorial'];
$Tut->save();
$LastInsertId = $Tut->id;
return Response::json(array('success' => true,'last_id'=>$LastInsertId), 200);
}
Here's an example:
public static function saveTutorial(){
$data = Input::all();
$Tut = new Tutorial;
$Tut->title = $data['title'];
$Tut->tutorial = $data['tutorial'];
$Tut->save();
$LastInsertId = $Tut->id;
return Response::json(array('success' => true,'last_id'=>$LastInsertId), 200);
}
edited Aug 17 at 17:47


Barry
3,10571637
3,10571637
answered Apr 16 '14 at 10:42


ngakak
458510
458510
add a comment |
add a comment |
This worked for me in laravel 4.2
$id = User::insertGetId([
'username' => Input::get('username'),
'password' => Hash::make('password'),
'active' => 0
]);
add a comment |
This worked for me in laravel 4.2
$id = User::insertGetId([
'username' => Input::get('username'),
'password' => Hash::make('password'),
'active' => 0
]);
add a comment |
This worked for me in laravel 4.2
$id = User::insertGetId([
'username' => Input::get('username'),
'password' => Hash::make('password'),
'active' => 0
]);
This worked for me in laravel 4.2
$id = User::insertGetId([
'username' => Input::get('username'),
'password' => Hash::make('password'),
'active' => 0
]);
edited Aug 17 at 17:48


Barry
3,10571637
3,10571637
answered Feb 26 '15 at 17:34
user28864
1,8991817
1,8991817
add a comment |
add a comment |
Here is how we can get last inserted id in Laravel 4
public function store()
{
$input = Input::all();
$validation = Validator::make($input, user::$rules);
if ($validation->passes())
{
$user= $this->user->create(array(
'name' => Input::get('name'),
'email' => Input::get('email'),
'password' => Hash::make(Input::get('password')),
));
$lastInsertedId= $user->id; //get last inserted record's user id value
$userId= array('user_id'=>$lastInsertedId); //put this value equal to datatable column name where it will be saved
$user->update($userId); //update newly created record by storing the value of last inserted id
return Redirect::route('users.index');
}
return Redirect::route('users.create')->withInput()->withErrors($validation)->with('message', 'There were validation errors.');
}
add a comment |
Here is how we can get last inserted id in Laravel 4
public function store()
{
$input = Input::all();
$validation = Validator::make($input, user::$rules);
if ($validation->passes())
{
$user= $this->user->create(array(
'name' => Input::get('name'),
'email' => Input::get('email'),
'password' => Hash::make(Input::get('password')),
));
$lastInsertedId= $user->id; //get last inserted record's user id value
$userId= array('user_id'=>$lastInsertedId); //put this value equal to datatable column name where it will be saved
$user->update($userId); //update newly created record by storing the value of last inserted id
return Redirect::route('users.index');
}
return Redirect::route('users.create')->withInput()->withErrors($validation)->with('message', 'There were validation errors.');
}
add a comment |
Here is how we can get last inserted id in Laravel 4
public function store()
{
$input = Input::all();
$validation = Validator::make($input, user::$rules);
if ($validation->passes())
{
$user= $this->user->create(array(
'name' => Input::get('name'),
'email' => Input::get('email'),
'password' => Hash::make(Input::get('password')),
));
$lastInsertedId= $user->id; //get last inserted record's user id value
$userId= array('user_id'=>$lastInsertedId); //put this value equal to datatable column name where it will be saved
$user->update($userId); //update newly created record by storing the value of last inserted id
return Redirect::route('users.index');
}
return Redirect::route('users.create')->withInput()->withErrors($validation)->with('message', 'There were validation errors.');
}
Here is how we can get last inserted id in Laravel 4
public function store()
{
$input = Input::all();
$validation = Validator::make($input, user::$rules);
if ($validation->passes())
{
$user= $this->user->create(array(
'name' => Input::get('name'),
'email' => Input::get('email'),
'password' => Hash::make(Input::get('password')),
));
$lastInsertedId= $user->id; //get last inserted record's user id value
$userId= array('user_id'=>$lastInsertedId); //put this value equal to datatable column name where it will be saved
$user->update($userId); //update newly created record by storing the value of last inserted id
return Redirect::route('users.index');
}
return Redirect::route('users.create')->withInput()->withErrors($validation)->with('message', 'There were validation errors.');
}
answered Apr 30 '14 at 7:45
Qamar Uzman
57263
57263
add a comment |
add a comment |
Use insertGetId
to insert and get inserted id
at the same time
From doc
If the table has an auto-incrementing id, use the insertGetId method
to insert a record and then retrieve the ID:
By Model
$id = Model::insertGetId(["name"=>"Niklesh","email"=>"myemail@gmail.com"]);
By DB
$id = DB::table('users')->insertGetId(["name"=>"Niklesh","email"=>"myemail@gmail.com"]);
For more details : https://laravel.com/docs/5.5/queries#inserts
add a comment |
Use insertGetId
to insert and get inserted id
at the same time
From doc
If the table has an auto-incrementing id, use the insertGetId method
to insert a record and then retrieve the ID:
By Model
$id = Model::insertGetId(["name"=>"Niklesh","email"=>"myemail@gmail.com"]);
By DB
$id = DB::table('users')->insertGetId(["name"=>"Niklesh","email"=>"myemail@gmail.com"]);
For more details : https://laravel.com/docs/5.5/queries#inserts
add a comment |
Use insertGetId
to insert and get inserted id
at the same time
From doc
If the table has an auto-incrementing id, use the insertGetId method
to insert a record and then retrieve the ID:
By Model
$id = Model::insertGetId(["name"=>"Niklesh","email"=>"myemail@gmail.com"]);
By DB
$id = DB::table('users')->insertGetId(["name"=>"Niklesh","email"=>"myemail@gmail.com"]);
For more details : https://laravel.com/docs/5.5/queries#inserts
Use insertGetId
to insert and get inserted id
at the same time
From doc
If the table has an auto-incrementing id, use the insertGetId method
to insert a record and then retrieve the ID:
By Model
$id = Model::insertGetId(["name"=>"Niklesh","email"=>"myemail@gmail.com"]);
By DB
$id = DB::table('users')->insertGetId(["name"=>"Niklesh","email"=>"myemail@gmail.com"]);
For more details : https://laravel.com/docs/5.5/queries#inserts
answered Sep 29 '17 at 6:32


C2486
18.8k32565
18.8k32565
add a comment |
add a comment |
After saving model, the initialized instance has the id:
$report = new Report();
$report->user_id = $request->user_id;
$report->patient_id = $request->patient_id;
$report->diseases_id = $request->modality;
$isReportCreated = $report->save();
return $report->id; // this will return the saved report id
add a comment |
After saving model, the initialized instance has the id:
$report = new Report();
$report->user_id = $request->user_id;
$report->patient_id = $request->patient_id;
$report->diseases_id = $request->modality;
$isReportCreated = $report->save();
return $report->id; // this will return the saved report id
add a comment |
After saving model, the initialized instance has the id:
$report = new Report();
$report->user_id = $request->user_id;
$report->patient_id = $request->patient_id;
$report->diseases_id = $request->modality;
$isReportCreated = $report->save();
return $report->id; // this will return the saved report id
After saving model, the initialized instance has the id:
$report = new Report();
$report->user_id = $request->user_id;
$report->patient_id = $request->patient_id;
$report->diseases_id = $request->modality;
$isReportCreated = $report->save();
return $report->id; // this will return the saved report id
edited Aug 17 at 17:49


Barry
3,10571637
3,10571637
answered Nov 3 '15 at 9:25


Amir
6,18943645
6,18943645
add a comment |
add a comment |
After saving a record in database, you can access id by $data->id
return Response::json(['success' => true, 'last_insert_id' => $data->id], 200)
add a comment |
After saving a record in database, you can access id by $data->id
return Response::json(['success' => true, 'last_insert_id' => $data->id], 200)
add a comment |
After saving a record in database, you can access id by $data->id
return Response::json(['success' => true, 'last_insert_id' => $data->id], 200)
After saving a record in database, you can access id by $data->id
return Response::json(['success' => true, 'last_insert_id' => $data->id], 200)
answered Nov 3 '15 at 10:30


Tayyab_Hussain
809815
809815
add a comment |
add a comment |
In Laravel 5.2 i would make it as clean as possible:
public function saveContact(Request $request, Contact $contact)
{
$create = $contact->create($request->all());
return response()->json($create->id, 201);
}
add a comment |
In Laravel 5.2 i would make it as clean as possible:
public function saveContact(Request $request, Contact $contact)
{
$create = $contact->create($request->all());
return response()->json($create->id, 201);
}
add a comment |
In Laravel 5.2 i would make it as clean as possible:
public function saveContact(Request $request, Contact $contact)
{
$create = $contact->create($request->all());
return response()->json($create->id, 201);
}
In Laravel 5.2 i would make it as clean as possible:
public function saveContact(Request $request, Contact $contact)
{
$create = $contact->create($request->all());
return response()->json($create->id, 201);
}
answered May 8 '16 at 11:30
bobbybackblech
91031322
91031322
add a comment |
add a comment |
For Laravel, If you insert a new record and call $data->save()
this function executes an INSERT query and returns the primary key value (i.e. id by default).
You can use following code:
if($data->save()) {
return Response::json(array('status' => 1, 'primary_id'=>$data->id), 200);
}
add a comment |
For Laravel, If you insert a new record and call $data->save()
this function executes an INSERT query and returns the primary key value (i.e. id by default).
You can use following code:
if($data->save()) {
return Response::json(array('status' => 1, 'primary_id'=>$data->id), 200);
}
add a comment |
For Laravel, If you insert a new record and call $data->save()
this function executes an INSERT query and returns the primary key value (i.e. id by default).
You can use following code:
if($data->save()) {
return Response::json(array('status' => 1, 'primary_id'=>$data->id), 200);
}
For Laravel, If you insert a new record and call $data->save()
this function executes an INSERT query and returns the primary key value (i.e. id by default).
You can use following code:
if($data->save()) {
return Response::json(array('status' => 1, 'primary_id'=>$data->id), 200);
}
edited Mar 21 '17 at 18:47


Sᴀᴍ Onᴇᴌᴀ
6,52682142
6,52682142
answered Mar 15 '17 at 9:06


HItesh Tank
261211
261211
add a comment |
add a comment |
After
$data->save()
$data->id
will give you the inserted id,
Note: If your autoincrement column name is sno then you should use
$data->sno
and not $data->id
add a comment |
After
$data->save()
$data->id
will give you the inserted id,
Note: If your autoincrement column name is sno then you should use
$data->sno
and not $data->id
add a comment |
After
$data->save()
$data->id
will give you the inserted id,
Note: If your autoincrement column name is sno then you should use
$data->sno
and not $data->id
After
$data->save()
$data->id
will give you the inserted id,
Note: If your autoincrement column name is sno then you should use
$data->sno
and not $data->id
answered Mar 31 '17 at 6:48
Abhishek Goel
10.2k66856
10.2k66856
add a comment |
add a comment |
You can do this:
$result=app('db')->insert("INSERT INTO table...");
$lastInsertId=app('db')->getPdo()->lastInsertId();
add a comment |
You can do this:
$result=app('db')->insert("INSERT INTO table...");
$lastInsertId=app('db')->getPdo()->lastInsertId();
add a comment |
You can do this:
$result=app('db')->insert("INSERT INTO table...");
$lastInsertId=app('db')->getPdo()->lastInsertId();
You can do this:
$result=app('db')->insert("INSERT INTO table...");
$lastInsertId=app('db')->getPdo()->lastInsertId();
edited Aug 17 '17 at 11:27


Moody_Mudskipper
21.2k32764
21.2k32764
answered Aug 17 '17 at 10:30
Dnyaneshwar Harer
11113
11113
add a comment |
add a comment |
$objPost = new Post;
$objPost->title = 'Title';
$objPost->description = 'Description';
$objPost->save();
$recId = $objPost->id; // If Id in table column name if other then id then user the other column name
return Response::json(['success' => true,'id' => $recId], 200);
add a comment |
$objPost = new Post;
$objPost->title = 'Title';
$objPost->description = 'Description';
$objPost->save();
$recId = $objPost->id; // If Id in table column name if other then id then user the other column name
return Response::json(['success' => true,'id' => $recId], 200);
add a comment |
$objPost = new Post;
$objPost->title = 'Title';
$objPost->description = 'Description';
$objPost->save();
$recId = $objPost->id; // If Id in table column name if other then id then user the other column name
return Response::json(['success' => true,'id' => $recId], 200);
$objPost = new Post;
$objPost->title = 'Title';
$objPost->description = 'Description';
$objPost->save();
$recId = $objPost->id; // If Id in table column name if other then id then user the other column name
return Response::json(['success' => true,'id' => $recId], 200);
edited Aug 17 at 17:50


Barry
3,10571637
3,10571637
answered Jun 20 at 12:36
Kamal Maisuriya
112
112
add a comment |
add a comment |
For insert()
Example:
$data1 = array(
'company_id' => $company_id,
'branch_id' => $branch_id
);
$insert_id = CreditVoucher::insert($data1);
$id = DB::getPdo()->lastInsertId();
dd($id);
add a comment |
For insert()
Example:
$data1 = array(
'company_id' => $company_id,
'branch_id' => $branch_id
);
$insert_id = CreditVoucher::insert($data1);
$id = DB::getPdo()->lastInsertId();
dd($id);
add a comment |
For insert()
Example:
$data1 = array(
'company_id' => $company_id,
'branch_id' => $branch_id
);
$insert_id = CreditVoucher::insert($data1);
$id = DB::getPdo()->lastInsertId();
dd($id);
For insert()
Example:
$data1 = array(
'company_id' => $company_id,
'branch_id' => $branch_id
);
$insert_id = CreditVoucher::insert($data1);
$id = DB::getPdo()->lastInsertId();
dd($id);
answered Dec 1 at 11:14


Faridul Khan
184116
184116
add a comment |
add a comment |
Using Eloquent Model
$user = new Report();
$user->email= 'johndoe@example.com';
$user->save();
$lastId = $user->id;
Using Query Builder
$lastId = DB::table('reports')->insertGetId(['email' => 'johndoe@example.com']);
add a comment |
Using Eloquent Model
$user = new Report();
$user->email= 'johndoe@example.com';
$user->save();
$lastId = $user->id;
Using Query Builder
$lastId = DB::table('reports')->insertGetId(['email' => 'johndoe@example.com']);
add a comment |
Using Eloquent Model
$user = new Report();
$user->email= 'johndoe@example.com';
$user->save();
$lastId = $user->id;
Using Query Builder
$lastId = DB::table('reports')->insertGetId(['email' => 'johndoe@example.com']);
Using Eloquent Model
$user = new Report();
$user->email= 'johndoe@example.com';
$user->save();
$lastId = $user->id;
Using Query Builder
$lastId = DB::table('reports')->insertGetId(['email' => 'johndoe@example.com']);
answered Oct 21 '17 at 19:21
srmilon
1,0981017
1,0981017
add a comment |
add a comment |
For get last inserted id in database
You can use
$data = new YourModelName;
$data->name = 'Some Value';
$data->email = 'abc@mail.com';
$data->save();
$lastInsertedId = $data->id;
here $lastInsertedId will gives you last inserted auto increment id.
add a comment |
For get last inserted id in database
You can use
$data = new YourModelName;
$data->name = 'Some Value';
$data->email = 'abc@mail.com';
$data->save();
$lastInsertedId = $data->id;
here $lastInsertedId will gives you last inserted auto increment id.
add a comment |
For get last inserted id in database
You can use
$data = new YourModelName;
$data->name = 'Some Value';
$data->email = 'abc@mail.com';
$data->save();
$lastInsertedId = $data->id;
here $lastInsertedId will gives you last inserted auto increment id.
For get last inserted id in database
You can use
$data = new YourModelName;
$data->name = 'Some Value';
$data->email = 'abc@mail.com';
$data->save();
$lastInsertedId = $data->id;
here $lastInsertedId will gives you last inserted auto increment id.
answered Feb 26 at 10:09
PPL
4,6941322
4,6941322
add a comment |
add a comment |
After Saving $data->save()
. all data is pushed inside $data
. As this is an object and the current row is just saved recently inside $data
. so last insertId
will be found inside $data->id
.
Response code will be:
return Response::json(array('success' => true, 'last_insert_id' => $data->id), 200);
add a comment |
After Saving $data->save()
. all data is pushed inside $data
. As this is an object and the current row is just saved recently inside $data
. so last insertId
will be found inside $data->id
.
Response code will be:
return Response::json(array('success' => true, 'last_insert_id' => $data->id), 200);
add a comment |
After Saving $data->save()
. all data is pushed inside $data
. As this is an object and the current row is just saved recently inside $data
. so last insertId
will be found inside $data->id
.
Response code will be:
return Response::json(array('success' => true, 'last_insert_id' => $data->id), 200);
After Saving $data->save()
. all data is pushed inside $data
. As this is an object and the current row is just saved recently inside $data
. so last insertId
will be found inside $data->id
.
Response code will be:
return Response::json(array('success' => true, 'last_insert_id' => $data->id), 200);
edited Aug 17 at 17:51


Barry
3,10571637
3,10571637
answered Apr 9 '17 at 8:51


sabuz
50459
50459
add a comment |
add a comment |
The shortest way is probably a call of the refresh()
on the model:
public function create(array $data): MyModel
{
$myModel = new MyModel($dataArray);
$myModel->saveOrFail();
return $myModel->refresh();
}
add a comment |
The shortest way is probably a call of the refresh()
on the model:
public function create(array $data): MyModel
{
$myModel = new MyModel($dataArray);
$myModel->saveOrFail();
return $myModel->refresh();
}
add a comment |
The shortest way is probably a call of the refresh()
on the model:
public function create(array $data): MyModel
{
$myModel = new MyModel($dataArray);
$myModel->saveOrFail();
return $myModel->refresh();
}
The shortest way is probably a call of the refresh()
on the model:
public function create(array $data): MyModel
{
$myModel = new MyModel($dataArray);
$myModel->saveOrFail();
return $myModel->refresh();
}
answered Dec 3 at 14:49
automatix
3,2621551144
3,2621551144
add a comment |
add a comment |
public function store( UserStoreRequest $request ) {
$input = $request->all();
$user = User::create($input);
$userId=$user->id
}
2
This post was answered 3 years ago. Please edit your answer to add more explanation as to why it might help the user or how its helps solves the OP's question in a better way.
– Syfer
Sep 8 '17 at 11:42
1
Thank you for this code snippet, which may provide some immediate help. A proper explanation would greatly improve its educational value by showing why this is a good solution to the problem, and would make it more useful to future readers with similar, but not identical, questions. Please edit your answer to add explanation, and give an indication of what limitations and assumptions apply. Not to mention the age of the question and the low quality of your answer.
– GrumpyCrouton
Sep 8 '17 at 15:53
add a comment |
public function store( UserStoreRequest $request ) {
$input = $request->all();
$user = User::create($input);
$userId=$user->id
}
2
This post was answered 3 years ago. Please edit your answer to add more explanation as to why it might help the user or how its helps solves the OP's question in a better way.
– Syfer
Sep 8 '17 at 11:42
1
Thank you for this code snippet, which may provide some immediate help. A proper explanation would greatly improve its educational value by showing why this is a good solution to the problem, and would make it more useful to future readers with similar, but not identical, questions. Please edit your answer to add explanation, and give an indication of what limitations and assumptions apply. Not to mention the age of the question and the low quality of your answer.
– GrumpyCrouton
Sep 8 '17 at 15:53
add a comment |
public function store( UserStoreRequest $request ) {
$input = $request->all();
$user = User::create($input);
$userId=$user->id
}
public function store( UserStoreRequest $request ) {
$input = $request->all();
$user = User::create($input);
$userId=$user->id
}
edited Sep 8 '17 at 11:36
Shadow
25.5k92743
25.5k92743
answered Sep 8 '17 at 11:30
temporary account
71
71
2
This post was answered 3 years ago. Please edit your answer to add more explanation as to why it might help the user or how its helps solves the OP's question in a better way.
– Syfer
Sep 8 '17 at 11:42
1
Thank you for this code snippet, which may provide some immediate help. A proper explanation would greatly improve its educational value by showing why this is a good solution to the problem, and would make it more useful to future readers with similar, but not identical, questions. Please edit your answer to add explanation, and give an indication of what limitations and assumptions apply. Not to mention the age of the question and the low quality of your answer.
– GrumpyCrouton
Sep 8 '17 at 15:53
add a comment |
2
This post was answered 3 years ago. Please edit your answer to add more explanation as to why it might help the user or how its helps solves the OP's question in a better way.
– Syfer
Sep 8 '17 at 11:42
1
Thank you for this code snippet, which may provide some immediate help. A proper explanation would greatly improve its educational value by showing why this is a good solution to the problem, and would make it more useful to future readers with similar, but not identical, questions. Please edit your answer to add explanation, and give an indication of what limitations and assumptions apply. Not to mention the age of the question and the low quality of your answer.
– GrumpyCrouton
Sep 8 '17 at 15:53
2
2
This post was answered 3 years ago. Please edit your answer to add more explanation as to why it might help the user or how its helps solves the OP's question in a better way.
– Syfer
Sep 8 '17 at 11:42
This post was answered 3 years ago. Please edit your answer to add more explanation as to why it might help the user or how its helps solves the OP's question in a better way.
– Syfer
Sep 8 '17 at 11:42
1
1
Thank you for this code snippet, which may provide some immediate help. A proper explanation would greatly improve its educational value by showing why this is a good solution to the problem, and would make it more useful to future readers with similar, but not identical, questions. Please edit your answer to add explanation, and give an indication of what limitations and assumptions apply. Not to mention the age of the question and the low quality of your answer.
– GrumpyCrouton
Sep 8 '17 at 15:53
Thank you for this code snippet, which may provide some immediate help. A proper explanation would greatly improve its educational value by showing why this is a good solution to the problem, and would make it more useful to future readers with similar, but not identical, questions. Please edit your answer to add explanation, and give an indication of what limitations and assumptions apply. Not to mention the age of the question and the low quality of your answer.
– GrumpyCrouton
Sep 8 '17 at 15:53
add a comment |
Using Eloquent Model
use AppCompany;
public function saveDetailsCompany(Request $request)
{
$createcompany=Company::create(['nombre'=>$request->input('name'),'direccion'=>$request->input('address'),'telefono'=>$request->input('phone'),'email'=>$request->input('emaile'),'giro'=>$request->input('type')]);
// Last Inserted Row ID
echo $createcompany->id;
}
Using Query Builder
$createcompany=DB::table('company')->create(['nombre'=>$request->input('name'),'direccion'=>$request->input('address'),'telefono'=>$request->input('phone'),'email'=>$request->input('emaile'),'giro'=>$request->input('type')]);
echo $createcompany->id;
For more methods to get Last Inserted Row id in Laravel : http://phpnotebook.com/95-laravel/127-3-methods-to-get-last-inserted-row-id-in-laravel
add a comment |
Using Eloquent Model
use AppCompany;
public function saveDetailsCompany(Request $request)
{
$createcompany=Company::create(['nombre'=>$request->input('name'),'direccion'=>$request->input('address'),'telefono'=>$request->input('phone'),'email'=>$request->input('emaile'),'giro'=>$request->input('type')]);
// Last Inserted Row ID
echo $createcompany->id;
}
Using Query Builder
$createcompany=DB::table('company')->create(['nombre'=>$request->input('name'),'direccion'=>$request->input('address'),'telefono'=>$request->input('phone'),'email'=>$request->input('emaile'),'giro'=>$request->input('type')]);
echo $createcompany->id;
For more methods to get Last Inserted Row id in Laravel : http://phpnotebook.com/95-laravel/127-3-methods-to-get-last-inserted-row-id-in-laravel
add a comment |
Using Eloquent Model
use AppCompany;
public function saveDetailsCompany(Request $request)
{
$createcompany=Company::create(['nombre'=>$request->input('name'),'direccion'=>$request->input('address'),'telefono'=>$request->input('phone'),'email'=>$request->input('emaile'),'giro'=>$request->input('type')]);
// Last Inserted Row ID
echo $createcompany->id;
}
Using Query Builder
$createcompany=DB::table('company')->create(['nombre'=>$request->input('name'),'direccion'=>$request->input('address'),'telefono'=>$request->input('phone'),'email'=>$request->input('emaile'),'giro'=>$request->input('type')]);
echo $createcompany->id;
For more methods to get Last Inserted Row id in Laravel : http://phpnotebook.com/95-laravel/127-3-methods-to-get-last-inserted-row-id-in-laravel
Using Eloquent Model
use AppCompany;
public function saveDetailsCompany(Request $request)
{
$createcompany=Company::create(['nombre'=>$request->input('name'),'direccion'=>$request->input('address'),'telefono'=>$request->input('phone'),'email'=>$request->input('emaile'),'giro'=>$request->input('type')]);
// Last Inserted Row ID
echo $createcompany->id;
}
Using Query Builder
$createcompany=DB::table('company')->create(['nombre'=>$request->input('name'),'direccion'=>$request->input('address'),'telefono'=>$request->input('phone'),'email'=>$request->input('emaile'),'giro'=>$request->input('type')]);
echo $createcompany->id;
For more methods to get Last Inserted Row id in Laravel : http://phpnotebook.com/95-laravel/127-3-methods-to-get-last-inserted-row-id-in-laravel
answered Jun 21 at 4:29


Karthik
1,18821235
1,18821235
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f21084833%2fget-the-last-inserted-id-using-laravel-eloquent%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
BJ4J1r,EwQ1kW3RjoLtylgVbblK4p1m5,9K3S2FQwpaljN