CORS issue after adding interceptor in angular
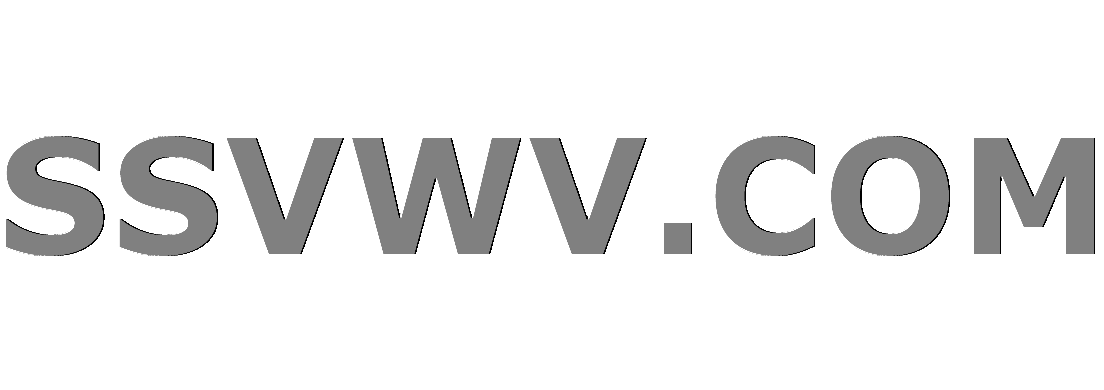
Multi tool use
I was sending API request from angular it had CORS issue so i fixed it in server using below code(laravel).
<?php
namespace AppHttpMiddleware;
use Closure;
class Cors
{
/**
* Handle an incoming request.
*
* @param IlluminateHttpRequest $request
* @param Closure $next
* @return mixed
*/
public function handle($request, Closure $next)
{
return $next($request)
->header('Access-Control-Allow-Origin', '*')
->header('Access-Control-Allow-Methods', 'GET, POST, PUT, DELETE, OPTIONS')
->header('Access-Control-Allow-Headers', '*');
}
}
But, now i changed my angular request it now goes through interceptor and now the CORS issue is back again.
Interceptor code:
import {Injectable, NgModule} from '@angular/core';
import {
HttpRequest,
HttpHandler,
HttpEvent,
HttpInterceptor, HTTP_INTERCEPTORS
} from '@angular/common/http';
import { Observable } from 'rxjs/Observable';
import {LoginService} from './login.service';
@Injectable()
export class TokenInterceptor implements HttpInterceptor {
constructor(public auth: LoginService) {}
intercept(request: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> {
request = request.clone({
setHeaders: {
Authorization: `Bearer ${this.auth.getToken()}`
}
});
return next.handle(request);
}
}
@NgModule({
providers: [
{ provide: HTTP_INTERCEPTORS, useClass: TokenInterceptor, multi: true }
]
})
export class InterceptorModule { }
Error:
Failed to load http://127.0.0.1:8000/api/auth/login: Response to preflight request doesn't pass access control check: No 'Access-Control-Allow-Origin' header is present on the requested resource. Origin 'http://localhost:4200' is therefore not allowed access.
Note: The API calls are working perfect if interceptor is not there.
Sample API Call
loginUser() {
const body = new HttpParams()
.set('password', this.password)
.set('email', this.email);
this.http.post<any>(this.apiUrl.getBaseUrl() + 'auth/login', body).subscribe(data => {
console.log(data);
const status = data['status'];
if (status === 'success') {
this.setSuccess(data['message']);
this.email = '';
this.password = '';
this.loginService.setToken(data['token']);
} else {
this.setError(data['message']);
}
}, err => {
this.setError('Server error occurred');
});
}
angular laravel laravel-5 angular4-httpclient
add a comment |
I was sending API request from angular it had CORS issue so i fixed it in server using below code(laravel).
<?php
namespace AppHttpMiddleware;
use Closure;
class Cors
{
/**
* Handle an incoming request.
*
* @param IlluminateHttpRequest $request
* @param Closure $next
* @return mixed
*/
public function handle($request, Closure $next)
{
return $next($request)
->header('Access-Control-Allow-Origin', '*')
->header('Access-Control-Allow-Methods', 'GET, POST, PUT, DELETE, OPTIONS')
->header('Access-Control-Allow-Headers', '*');
}
}
But, now i changed my angular request it now goes through interceptor and now the CORS issue is back again.
Interceptor code:
import {Injectable, NgModule} from '@angular/core';
import {
HttpRequest,
HttpHandler,
HttpEvent,
HttpInterceptor, HTTP_INTERCEPTORS
} from '@angular/common/http';
import { Observable } from 'rxjs/Observable';
import {LoginService} from './login.service';
@Injectable()
export class TokenInterceptor implements HttpInterceptor {
constructor(public auth: LoginService) {}
intercept(request: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> {
request = request.clone({
setHeaders: {
Authorization: `Bearer ${this.auth.getToken()}`
}
});
return next.handle(request);
}
}
@NgModule({
providers: [
{ provide: HTTP_INTERCEPTORS, useClass: TokenInterceptor, multi: true }
]
})
export class InterceptorModule { }
Error:
Failed to load http://127.0.0.1:8000/api/auth/login: Response to preflight request doesn't pass access control check: No 'Access-Control-Allow-Origin' header is present on the requested resource. Origin 'http://localhost:4200' is therefore not allowed access.
Note: The API calls are working perfect if interceptor is not there.
Sample API Call
loginUser() {
const body = new HttpParams()
.set('password', this.password)
.set('email', this.email);
this.http.post<any>(this.apiUrl.getBaseUrl() + 'auth/login', body).subscribe(data => {
console.log(data);
const status = data['status'];
if (status === 'success') {
this.setSuccess(data['message']);
this.email = '';
this.password = '';
this.loginService.setToken(data['token']);
} else {
this.setError(data['message']);
}
}, err => {
this.setError('Server error occurred');
});
}
angular laravel laravel-5 angular4-httpclient
1
You can try this awesome pacakge github.com/barryvdh/laravel-cors . It works for me everytime
– Sapnesh Naik
Mar 18 '18 at 14:44
add a comment |
I was sending API request from angular it had CORS issue so i fixed it in server using below code(laravel).
<?php
namespace AppHttpMiddleware;
use Closure;
class Cors
{
/**
* Handle an incoming request.
*
* @param IlluminateHttpRequest $request
* @param Closure $next
* @return mixed
*/
public function handle($request, Closure $next)
{
return $next($request)
->header('Access-Control-Allow-Origin', '*')
->header('Access-Control-Allow-Methods', 'GET, POST, PUT, DELETE, OPTIONS')
->header('Access-Control-Allow-Headers', '*');
}
}
But, now i changed my angular request it now goes through interceptor and now the CORS issue is back again.
Interceptor code:
import {Injectable, NgModule} from '@angular/core';
import {
HttpRequest,
HttpHandler,
HttpEvent,
HttpInterceptor, HTTP_INTERCEPTORS
} from '@angular/common/http';
import { Observable } from 'rxjs/Observable';
import {LoginService} from './login.service';
@Injectable()
export class TokenInterceptor implements HttpInterceptor {
constructor(public auth: LoginService) {}
intercept(request: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> {
request = request.clone({
setHeaders: {
Authorization: `Bearer ${this.auth.getToken()}`
}
});
return next.handle(request);
}
}
@NgModule({
providers: [
{ provide: HTTP_INTERCEPTORS, useClass: TokenInterceptor, multi: true }
]
})
export class InterceptorModule { }
Error:
Failed to load http://127.0.0.1:8000/api/auth/login: Response to preflight request doesn't pass access control check: No 'Access-Control-Allow-Origin' header is present on the requested resource. Origin 'http://localhost:4200' is therefore not allowed access.
Note: The API calls are working perfect if interceptor is not there.
Sample API Call
loginUser() {
const body = new HttpParams()
.set('password', this.password)
.set('email', this.email);
this.http.post<any>(this.apiUrl.getBaseUrl() + 'auth/login', body).subscribe(data => {
console.log(data);
const status = data['status'];
if (status === 'success') {
this.setSuccess(data['message']);
this.email = '';
this.password = '';
this.loginService.setToken(data['token']);
} else {
this.setError(data['message']);
}
}, err => {
this.setError('Server error occurred');
});
}
angular laravel laravel-5 angular4-httpclient
I was sending API request from angular it had CORS issue so i fixed it in server using below code(laravel).
<?php
namespace AppHttpMiddleware;
use Closure;
class Cors
{
/**
* Handle an incoming request.
*
* @param IlluminateHttpRequest $request
* @param Closure $next
* @return mixed
*/
public function handle($request, Closure $next)
{
return $next($request)
->header('Access-Control-Allow-Origin', '*')
->header('Access-Control-Allow-Methods', 'GET, POST, PUT, DELETE, OPTIONS')
->header('Access-Control-Allow-Headers', '*');
}
}
But, now i changed my angular request it now goes through interceptor and now the CORS issue is back again.
Interceptor code:
import {Injectable, NgModule} from '@angular/core';
import {
HttpRequest,
HttpHandler,
HttpEvent,
HttpInterceptor, HTTP_INTERCEPTORS
} from '@angular/common/http';
import { Observable } from 'rxjs/Observable';
import {LoginService} from './login.service';
@Injectable()
export class TokenInterceptor implements HttpInterceptor {
constructor(public auth: LoginService) {}
intercept(request: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> {
request = request.clone({
setHeaders: {
Authorization: `Bearer ${this.auth.getToken()}`
}
});
return next.handle(request);
}
}
@NgModule({
providers: [
{ provide: HTTP_INTERCEPTORS, useClass: TokenInterceptor, multi: true }
]
})
export class InterceptorModule { }
Error:
Failed to load http://127.0.0.1:8000/api/auth/login: Response to preflight request doesn't pass access control check: No 'Access-Control-Allow-Origin' header is present on the requested resource. Origin 'http://localhost:4200' is therefore not allowed access.
Note: The API calls are working perfect if interceptor is not there.
Sample API Call
loginUser() {
const body = new HttpParams()
.set('password', this.password)
.set('email', this.email);
this.http.post<any>(this.apiUrl.getBaseUrl() + 'auth/login', body).subscribe(data => {
console.log(data);
const status = data['status'];
if (status === 'success') {
this.setSuccess(data['message']);
this.email = '';
this.password = '';
this.loginService.setToken(data['token']);
} else {
this.setError(data['message']);
}
}, err => {
this.setError('Server error occurred');
});
}
angular laravel laravel-5 angular4-httpclient
angular laravel laravel-5 angular4-httpclient
asked Mar 18 '18 at 14:38
AbhishekAbhishek
447217
447217
1
You can try this awesome pacakge github.com/barryvdh/laravel-cors . It works for me everytime
– Sapnesh Naik
Mar 18 '18 at 14:44
add a comment |
1
You can try this awesome pacakge github.com/barryvdh/laravel-cors . It works for me everytime
– Sapnesh Naik
Mar 18 '18 at 14:44
1
1
You can try this awesome pacakge github.com/barryvdh/laravel-cors . It works for me everytime
– Sapnesh Naik
Mar 18 '18 at 14:44
You can try this awesome pacakge github.com/barryvdh/laravel-cors . It works for me everytime
– Sapnesh Naik
Mar 18 '18 at 14:44
add a comment |
2 Answers
2
active
oldest
votes
Not sure why your API calls work without the interceptor and not with it, probably an issue related to preflight requests and your PHP backend. However, if your API is public you shouldn't enable CORS for any Origin
as it may allow attackers to access your API with impersonated requests (authenticated as the victim). Please take a look at my answer on this question : Angular2 CORS issue on localhost
add a comment |
I had the same problem when I wanted to call a Solr API. When calling the API, a JWT token was added with an interceptor. Then I got the CORS error (without interceptor it worked perfectly).
The solution was to explicitly allow the Authorization
header in Solr web.xml in the "allowed headers" section.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f49349103%2fcors-issue-after-adding-interceptor-in-angular%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Not sure why your API calls work without the interceptor and not with it, probably an issue related to preflight requests and your PHP backend. However, if your API is public you shouldn't enable CORS for any Origin
as it may allow attackers to access your API with impersonated requests (authenticated as the victim). Please take a look at my answer on this question : Angular2 CORS issue on localhost
add a comment |
Not sure why your API calls work without the interceptor and not with it, probably an issue related to preflight requests and your PHP backend. However, if your API is public you shouldn't enable CORS for any Origin
as it may allow attackers to access your API with impersonated requests (authenticated as the victim). Please take a look at my answer on this question : Angular2 CORS issue on localhost
add a comment |
Not sure why your API calls work without the interceptor and not with it, probably an issue related to preflight requests and your PHP backend. However, if your API is public you shouldn't enable CORS for any Origin
as it may allow attackers to access your API with impersonated requests (authenticated as the victim). Please take a look at my answer on this question : Angular2 CORS issue on localhost
Not sure why your API calls work without the interceptor and not with it, probably an issue related to preflight requests and your PHP backend. However, if your API is public you shouldn't enable CORS for any Origin
as it may allow attackers to access your API with impersonated requests (authenticated as the victim). Please take a look at my answer on this question : Angular2 CORS issue on localhost
edited Mar 19 '18 at 10:15
answered Mar 19 '18 at 10:02
YoukouleleYYoukouleleY
2,3981825
2,3981825
add a comment |
add a comment |
I had the same problem when I wanted to call a Solr API. When calling the API, a JWT token was added with an interceptor. Then I got the CORS error (without interceptor it worked perfectly).
The solution was to explicitly allow the Authorization
header in Solr web.xml in the "allowed headers" section.
add a comment |
I had the same problem when I wanted to call a Solr API. When calling the API, a JWT token was added with an interceptor. Then I got the CORS error (without interceptor it worked perfectly).
The solution was to explicitly allow the Authorization
header in Solr web.xml in the "allowed headers" section.
add a comment |
I had the same problem when I wanted to call a Solr API. When calling the API, a JWT token was added with an interceptor. Then I got the CORS error (without interceptor it worked perfectly).
The solution was to explicitly allow the Authorization
header in Solr web.xml in the "allowed headers" section.
I had the same problem when I wanted to call a Solr API. When calling the API, a JWT token was added with an interceptor. Then I got the CORS error (without interceptor it worked perfectly).
The solution was to explicitly allow the Authorization
header in Solr web.xml in the "allowed headers" section.
answered Nov 21 '18 at 17:07
MikeMike
14738
14738
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f49349103%2fcors-issue-after-adding-interceptor-in-angular%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
fYqa4wqKTnk,XzwBj,RxMlPUp
1
You can try this awesome pacakge github.com/barryvdh/laravel-cors . It works for me everytime
– Sapnesh Naik
Mar 18 '18 at 14:44