Is there a way to disable member function in a template class in c++ for visual studio 2010 ( no default...
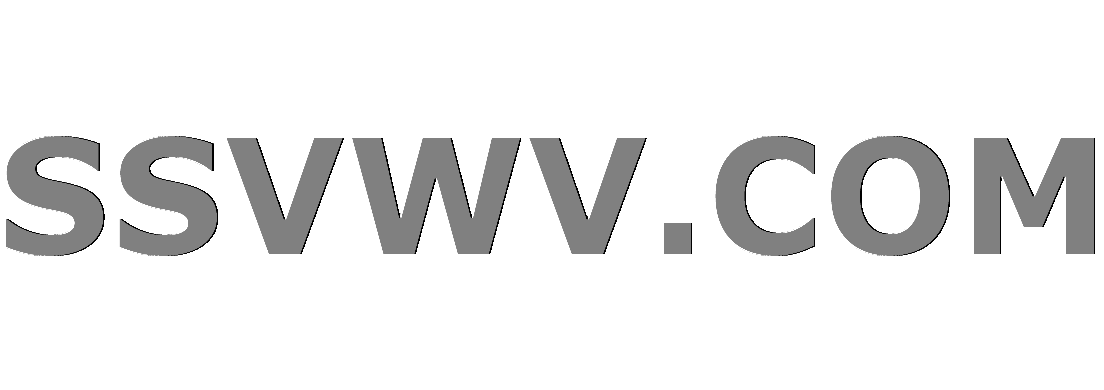
Multi tool use
For example
#include "boost/type_traits.hpp"
template <bool enable>
struct Foo {
template <bool e = enable>
typename boost::enable_if_c<e,void>::type DoStuff(){}
};
int main(){
// Compiles
Foo<true>().DoStuff();
// Fails
Foo<false>().DoStuff();
}
will work in modern compilers but not with visual studio 2010 which does not allow default template params for functions / methods. Is there another way to formulate the same task that will work with VS2010?
c++ visual-studio-2010 enable-if
|
show 1 more comment
For example
#include "boost/type_traits.hpp"
template <bool enable>
struct Foo {
template <bool e = enable>
typename boost::enable_if_c<e,void>::type DoStuff(){}
};
int main(){
// Compiles
Foo<true>().DoStuff();
// Fails
Foo<false>().DoStuff();
}
will work in modern compilers but not with visual studio 2010 which does not allow default template params for functions / methods. Is there another way to formulate the same task that will work with VS2010?
c++ visual-studio-2010 enable-if
What about uncompilable template specialization for false?
– Yuriy
Nov 21 '18 at 17:10
I'm not quite sure what you mean sorry.
– bradgonesurfing
Nov 21 '18 at 17:12
tag dispatching/full specialization/or CRTP
– Piotr Skotnicki
Nov 21 '18 at 17:15
You can create specialization of Foo<false> with some kind of static_assert inside void DoStuff(){}. Or just do as @NathanOliver says)
– Yuriy
Nov 21 '18 at 17:25
2
@jesper legacy code with legacy support contracts to paying customers. a.k.a the real world.
– bradgonesurfing
Nov 21 '18 at 17:50
|
show 1 more comment
For example
#include "boost/type_traits.hpp"
template <bool enable>
struct Foo {
template <bool e = enable>
typename boost::enable_if_c<e,void>::type DoStuff(){}
};
int main(){
// Compiles
Foo<true>().DoStuff();
// Fails
Foo<false>().DoStuff();
}
will work in modern compilers but not with visual studio 2010 which does not allow default template params for functions / methods. Is there another way to formulate the same task that will work with VS2010?
c++ visual-studio-2010 enable-if
For example
#include "boost/type_traits.hpp"
template <bool enable>
struct Foo {
template <bool e = enable>
typename boost::enable_if_c<e,void>::type DoStuff(){}
};
int main(){
// Compiles
Foo<true>().DoStuff();
// Fails
Foo<false>().DoStuff();
}
will work in modern compilers but not with visual studio 2010 which does not allow default template params for functions / methods. Is there another way to formulate the same task that will work with VS2010?
c++ visual-studio-2010 enable-if
c++ visual-studio-2010 enable-if
asked Nov 21 '18 at 17:03
bradgonesurfingbradgonesurfing
16.2k1083151
16.2k1083151
What about uncompilable template specialization for false?
– Yuriy
Nov 21 '18 at 17:10
I'm not quite sure what you mean sorry.
– bradgonesurfing
Nov 21 '18 at 17:12
tag dispatching/full specialization/or CRTP
– Piotr Skotnicki
Nov 21 '18 at 17:15
You can create specialization of Foo<false> with some kind of static_assert inside void DoStuff(){}. Or just do as @NathanOliver says)
– Yuriy
Nov 21 '18 at 17:25
2
@jesper legacy code with legacy support contracts to paying customers. a.k.a the real world.
– bradgonesurfing
Nov 21 '18 at 17:50
|
show 1 more comment
What about uncompilable template specialization for false?
– Yuriy
Nov 21 '18 at 17:10
I'm not quite sure what you mean sorry.
– bradgonesurfing
Nov 21 '18 at 17:12
tag dispatching/full specialization/or CRTP
– Piotr Skotnicki
Nov 21 '18 at 17:15
You can create specialization of Foo<false> with some kind of static_assert inside void DoStuff(){}. Or just do as @NathanOliver says)
– Yuriy
Nov 21 '18 at 17:25
2
@jesper legacy code with legacy support contracts to paying customers. a.k.a the real world.
– bradgonesurfing
Nov 21 '18 at 17:50
What about uncompilable template specialization for false?
– Yuriy
Nov 21 '18 at 17:10
What about uncompilable template specialization for false?
– Yuriy
Nov 21 '18 at 17:10
I'm not quite sure what you mean sorry.
– bradgonesurfing
Nov 21 '18 at 17:12
I'm not quite sure what you mean sorry.
– bradgonesurfing
Nov 21 '18 at 17:12
tag dispatching/full specialization/or CRTP
– Piotr Skotnicki
Nov 21 '18 at 17:15
tag dispatching/full specialization/or CRTP
– Piotr Skotnicki
Nov 21 '18 at 17:15
You can create specialization of Foo<false> with some kind of static_assert inside void DoStuff(){}. Or just do as @NathanOliver says)
– Yuriy
Nov 21 '18 at 17:25
You can create specialization of Foo<false> with some kind of static_assert inside void DoStuff(){}. Or just do as @NathanOliver says)
– Yuriy
Nov 21 '18 at 17:25
2
2
@jesper legacy code with legacy support contracts to paying customers. a.k.a the real world.
– bradgonesurfing
Nov 21 '18 at 17:50
@jesper legacy code with legacy support contracts to paying customers. a.k.a the real world.
– bradgonesurfing
Nov 21 '18 at 17:50
|
show 1 more comment
2 Answers
2
active
oldest
votes
You could specialize the entire class like
template <bool enable>
struct Foo {};
template <>
struct Foo<true> {
void DoStuff(){}
};
template <>
struct Foo<false> {
};
And then
int main(){
// Compiles
Foo<true>().DoStuff();
// Fails
Foo<false>().DoStuff();
}
Specialising the entire class is difficult if I only have one method I want to disable and 50 standard ones. But a useful answer anyway.
– bradgonesurfing
Nov 21 '18 at 17:52
1
@bradgonesurfing In that case you could leverage inheritance. Put all of the common functions in a base and specialize for the differences. This will save a lot of code replication (it's the DRY way).
– NathanOliver
Nov 21 '18 at 17:55
add a comment |
You could discard DoStuff via SFINAE:
template<bool enable>
struct Foo {
private:
static void SFINAE_Helper(std::true_type);
typedef std::integral_constant<bool, enable> tag;
public:
decltype(SFINAE_Helper(tag())) DoStuff() { }
};
Besides the fact that this code is quite unreadable it has the advantage, that you do not need to make extra specializations but can have all your code in one class template.
EDIT
An alternative could look like that:
template<bool enable>
struct Foo {
private:
typedef std::enable_if<enable> enable_tag;
public:
typename enable_tag::type DoStuff() {}
};
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53417181%2fis-there-a-way-to-disable-member-function-in-a-template-class-in-c-for-visual%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You could specialize the entire class like
template <bool enable>
struct Foo {};
template <>
struct Foo<true> {
void DoStuff(){}
};
template <>
struct Foo<false> {
};
And then
int main(){
// Compiles
Foo<true>().DoStuff();
// Fails
Foo<false>().DoStuff();
}
Specialising the entire class is difficult if I only have one method I want to disable and 50 standard ones. But a useful answer anyway.
– bradgonesurfing
Nov 21 '18 at 17:52
1
@bradgonesurfing In that case you could leverage inheritance. Put all of the common functions in a base and specialize for the differences. This will save a lot of code replication (it's the DRY way).
– NathanOliver
Nov 21 '18 at 17:55
add a comment |
You could specialize the entire class like
template <bool enable>
struct Foo {};
template <>
struct Foo<true> {
void DoStuff(){}
};
template <>
struct Foo<false> {
};
And then
int main(){
// Compiles
Foo<true>().DoStuff();
// Fails
Foo<false>().DoStuff();
}
Specialising the entire class is difficult if I only have one method I want to disable and 50 standard ones. But a useful answer anyway.
– bradgonesurfing
Nov 21 '18 at 17:52
1
@bradgonesurfing In that case you could leverage inheritance. Put all of the common functions in a base and specialize for the differences. This will save a lot of code replication (it's the DRY way).
– NathanOliver
Nov 21 '18 at 17:55
add a comment |
You could specialize the entire class like
template <bool enable>
struct Foo {};
template <>
struct Foo<true> {
void DoStuff(){}
};
template <>
struct Foo<false> {
};
And then
int main(){
// Compiles
Foo<true>().DoStuff();
// Fails
Foo<false>().DoStuff();
}
You could specialize the entire class like
template <bool enable>
struct Foo {};
template <>
struct Foo<true> {
void DoStuff(){}
};
template <>
struct Foo<false> {
};
And then
int main(){
// Compiles
Foo<true>().DoStuff();
// Fails
Foo<false>().DoStuff();
}
answered Nov 21 '18 at 17:16


NathanOliverNathanOliver
89.1k15120187
89.1k15120187
Specialising the entire class is difficult if I only have one method I want to disable and 50 standard ones. But a useful answer anyway.
– bradgonesurfing
Nov 21 '18 at 17:52
1
@bradgonesurfing In that case you could leverage inheritance. Put all of the common functions in a base and specialize for the differences. This will save a lot of code replication (it's the DRY way).
– NathanOliver
Nov 21 '18 at 17:55
add a comment |
Specialising the entire class is difficult if I only have one method I want to disable and 50 standard ones. But a useful answer anyway.
– bradgonesurfing
Nov 21 '18 at 17:52
1
@bradgonesurfing In that case you could leverage inheritance. Put all of the common functions in a base and specialize for the differences. This will save a lot of code replication (it's the DRY way).
– NathanOliver
Nov 21 '18 at 17:55
Specialising the entire class is difficult if I only have one method I want to disable and 50 standard ones. But a useful answer anyway.
– bradgonesurfing
Nov 21 '18 at 17:52
Specialising the entire class is difficult if I only have one method I want to disable and 50 standard ones. But a useful answer anyway.
– bradgonesurfing
Nov 21 '18 at 17:52
1
1
@bradgonesurfing In that case you could leverage inheritance. Put all of the common functions in a base and specialize for the differences. This will save a lot of code replication (it's the DRY way).
– NathanOliver
Nov 21 '18 at 17:55
@bradgonesurfing In that case you could leverage inheritance. Put all of the common functions in a base and specialize for the differences. This will save a lot of code replication (it's the DRY way).
– NathanOliver
Nov 21 '18 at 17:55
add a comment |
You could discard DoStuff via SFINAE:
template<bool enable>
struct Foo {
private:
static void SFINAE_Helper(std::true_type);
typedef std::integral_constant<bool, enable> tag;
public:
decltype(SFINAE_Helper(tag())) DoStuff() { }
};
Besides the fact that this code is quite unreadable it has the advantage, that you do not need to make extra specializations but can have all your code in one class template.
EDIT
An alternative could look like that:
template<bool enable>
struct Foo {
private:
typedef std::enable_if<enable> enable_tag;
public:
typename enable_tag::type DoStuff() {}
};
add a comment |
You could discard DoStuff via SFINAE:
template<bool enable>
struct Foo {
private:
static void SFINAE_Helper(std::true_type);
typedef std::integral_constant<bool, enable> tag;
public:
decltype(SFINAE_Helper(tag())) DoStuff() { }
};
Besides the fact that this code is quite unreadable it has the advantage, that you do not need to make extra specializations but can have all your code in one class template.
EDIT
An alternative could look like that:
template<bool enable>
struct Foo {
private:
typedef std::enable_if<enable> enable_tag;
public:
typename enable_tag::type DoStuff() {}
};
add a comment |
You could discard DoStuff via SFINAE:
template<bool enable>
struct Foo {
private:
static void SFINAE_Helper(std::true_type);
typedef std::integral_constant<bool, enable> tag;
public:
decltype(SFINAE_Helper(tag())) DoStuff() { }
};
Besides the fact that this code is quite unreadable it has the advantage, that you do not need to make extra specializations but can have all your code in one class template.
EDIT
An alternative could look like that:
template<bool enable>
struct Foo {
private:
typedef std::enable_if<enable> enable_tag;
public:
typename enable_tag::type DoStuff() {}
};
You could discard DoStuff via SFINAE:
template<bool enable>
struct Foo {
private:
static void SFINAE_Helper(std::true_type);
typedef std::integral_constant<bool, enable> tag;
public:
decltype(SFINAE_Helper(tag())) DoStuff() { }
};
Besides the fact that this code is quite unreadable it has the advantage, that you do not need to make extra specializations but can have all your code in one class template.
EDIT
An alternative could look like that:
template<bool enable>
struct Foo {
private:
typedef std::enable_if<enable> enable_tag;
public:
typename enable_tag::type DoStuff() {}
};
edited Nov 21 '18 at 17:46
answered Nov 21 '18 at 17:33
KilianKilian
12516
12516
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53417181%2fis-there-a-way-to-disable-member-function-in-a-template-class-in-c-for-visual%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
LgdxHrRU9JVa4Ig1Miqguw,l Uiq1FBDl,TjZEDm131IHncWNK0a9YQJ1 TNh8Be,HW,LIuolIhtoWQUiAK,ir8Iu
What about uncompilable template specialization for false?
– Yuriy
Nov 21 '18 at 17:10
I'm not quite sure what you mean sorry.
– bradgonesurfing
Nov 21 '18 at 17:12
tag dispatching/full specialization/or CRTP
– Piotr Skotnicki
Nov 21 '18 at 17:15
You can create specialization of Foo<false> with some kind of static_assert inside void DoStuff(){}. Or just do as @NathanOliver says)
– Yuriy
Nov 21 '18 at 17:25
2
@jesper legacy code with legacy support contracts to paying customers. a.k.a the real world.
– bradgonesurfing
Nov 21 '18 at 17:50