C++ - Why is std::function invalid?
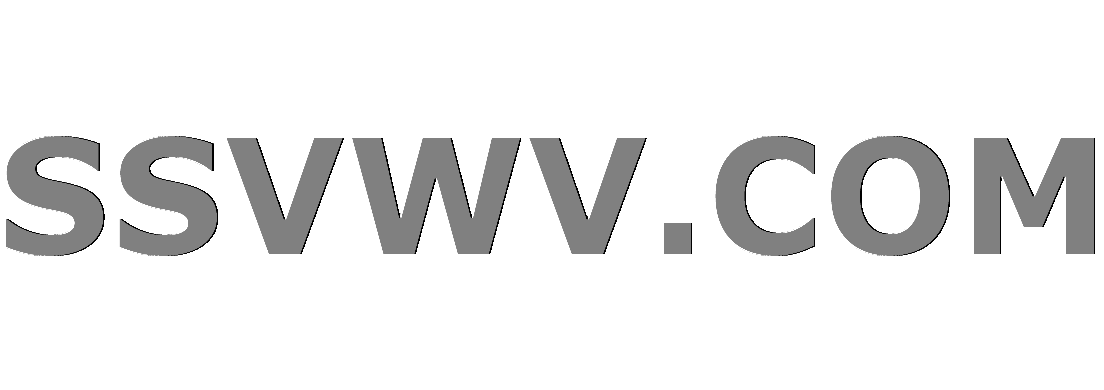
Multi tool use
In C++, if I try to do this:
std::function<void(bool,void)>
then the compiler will throw errors. Why is this? It's useful in many cases. One example:
//g++ -std=c++17 prblm.cpp
#include <cstdio>
#include <functional>
template<class type_t>
class some_callback
{
public:
using callback_t = std::function<void(bool,type_t)>;
some_callback(callback_t _myfunc)
{
this->myfunc = _myfunc;
}
callback_t myfunc;
};
using callback_with_just_bool = some_callback<void>;
using callback_with_an_int_too = some_callback<int>;
int main()
{
auto my_callback_with_int = callback_with_an_int_too((bool x, int y)
{
}); //OK
auto my_callback_just_bool = callback_with_just_bool((bool x)
{
}); //Error
auto my_callback_just_bool = callback_with_just_bool((bool x,void z)
{
}); //Error
return 0;
}
This allows for a very clean syntax if the user would like to optionally have additional data in their callback, but not have to. However, the compiler will reject code that tries to initialize an object of callback_with_just_bool
Why is it like this, and is there a clean way around it? Thanks.
Edit: The specific reason I'm trying to do this, in real world code, is in an event system. There is data provided to the event system about an individual object that wishes to conditionally receive events (e.x. "if you're close enough to the source, you'll receive a sound event") as well as data provided to a callback about the event (e.x. "a 10khz noise at X200 Y200"). Most of the time, the data needed to check the requirements will exist inside the data provided to the callback about the event, but I wanted to provided an optional additional data structure if that was not the case. Hence, the user would specify "void" if they didn't need this additional data structure.
c++ templates c++17 void
add a comment |
In C++, if I try to do this:
std::function<void(bool,void)>
then the compiler will throw errors. Why is this? It's useful in many cases. One example:
//g++ -std=c++17 prblm.cpp
#include <cstdio>
#include <functional>
template<class type_t>
class some_callback
{
public:
using callback_t = std::function<void(bool,type_t)>;
some_callback(callback_t _myfunc)
{
this->myfunc = _myfunc;
}
callback_t myfunc;
};
using callback_with_just_bool = some_callback<void>;
using callback_with_an_int_too = some_callback<int>;
int main()
{
auto my_callback_with_int = callback_with_an_int_too((bool x, int y)
{
}); //OK
auto my_callback_just_bool = callback_with_just_bool((bool x)
{
}); //Error
auto my_callback_just_bool = callback_with_just_bool((bool x,void z)
{
}); //Error
return 0;
}
This allows for a very clean syntax if the user would like to optionally have additional data in their callback, but not have to. However, the compiler will reject code that tries to initialize an object of callback_with_just_bool
Why is it like this, and is there a clean way around it? Thanks.
Edit: The specific reason I'm trying to do this, in real world code, is in an event system. There is data provided to the event system about an individual object that wishes to conditionally receive events (e.x. "if you're close enough to the source, you'll receive a sound event") as well as data provided to a callback about the event (e.x. "a 10khz noise at X200 Y200"). Most of the time, the data needed to check the requirements will exist inside the data provided to the callback about the event, but I wanted to provided an optional additional data structure if that was not the case. Hence, the user would specify "void" if they didn't need this additional data structure.
c++ templates c++17 void
2
It's like that becausevoid
is not a valid parameter type.
– Peter Ruderman
Nov 23 '18 at 19:50
Sounds like you needstd::optional
or a pointer for your additional data structure.
– Jon Harper
Nov 23 '18 at 21:37
Yea, that would work, but I'm being pedantic about performance because there is a good likelihood that thousands of events are going to be triggered per tick, which will probably 90 ticks per second+ as I am working on a VR game. It'd be cool if there was a type-system equivalent of what std::optional does for values.
– ai221
Nov 24 '18 at 0:04
add a comment |
In C++, if I try to do this:
std::function<void(bool,void)>
then the compiler will throw errors. Why is this? It's useful in many cases. One example:
//g++ -std=c++17 prblm.cpp
#include <cstdio>
#include <functional>
template<class type_t>
class some_callback
{
public:
using callback_t = std::function<void(bool,type_t)>;
some_callback(callback_t _myfunc)
{
this->myfunc = _myfunc;
}
callback_t myfunc;
};
using callback_with_just_bool = some_callback<void>;
using callback_with_an_int_too = some_callback<int>;
int main()
{
auto my_callback_with_int = callback_with_an_int_too((bool x, int y)
{
}); //OK
auto my_callback_just_bool = callback_with_just_bool((bool x)
{
}); //Error
auto my_callback_just_bool = callback_with_just_bool((bool x,void z)
{
}); //Error
return 0;
}
This allows for a very clean syntax if the user would like to optionally have additional data in their callback, but not have to. However, the compiler will reject code that tries to initialize an object of callback_with_just_bool
Why is it like this, and is there a clean way around it? Thanks.
Edit: The specific reason I'm trying to do this, in real world code, is in an event system. There is data provided to the event system about an individual object that wishes to conditionally receive events (e.x. "if you're close enough to the source, you'll receive a sound event") as well as data provided to a callback about the event (e.x. "a 10khz noise at X200 Y200"). Most of the time, the data needed to check the requirements will exist inside the data provided to the callback about the event, but I wanted to provided an optional additional data structure if that was not the case. Hence, the user would specify "void" if they didn't need this additional data structure.
c++ templates c++17 void
In C++, if I try to do this:
std::function<void(bool,void)>
then the compiler will throw errors. Why is this? It's useful in many cases. One example:
//g++ -std=c++17 prblm.cpp
#include <cstdio>
#include <functional>
template<class type_t>
class some_callback
{
public:
using callback_t = std::function<void(bool,type_t)>;
some_callback(callback_t _myfunc)
{
this->myfunc = _myfunc;
}
callback_t myfunc;
};
using callback_with_just_bool = some_callback<void>;
using callback_with_an_int_too = some_callback<int>;
int main()
{
auto my_callback_with_int = callback_with_an_int_too((bool x, int y)
{
}); //OK
auto my_callback_just_bool = callback_with_just_bool((bool x)
{
}); //Error
auto my_callback_just_bool = callback_with_just_bool((bool x,void z)
{
}); //Error
return 0;
}
This allows for a very clean syntax if the user would like to optionally have additional data in their callback, but not have to. However, the compiler will reject code that tries to initialize an object of callback_with_just_bool
Why is it like this, and is there a clean way around it? Thanks.
Edit: The specific reason I'm trying to do this, in real world code, is in an event system. There is data provided to the event system about an individual object that wishes to conditionally receive events (e.x. "if you're close enough to the source, you'll receive a sound event") as well as data provided to a callback about the event (e.x. "a 10khz noise at X200 Y200"). Most of the time, the data needed to check the requirements will exist inside the data provided to the callback about the event, but I wanted to provided an optional additional data structure if that was not the case. Hence, the user would specify "void" if they didn't need this additional data structure.
c++ templates c++17 void
c++ templates c++17 void
edited Nov 23 '18 at 20:01
ai221
asked Nov 23 '18 at 19:46


ai221ai221
254
254
2
It's like that becausevoid
is not a valid parameter type.
– Peter Ruderman
Nov 23 '18 at 19:50
Sounds like you needstd::optional
or a pointer for your additional data structure.
– Jon Harper
Nov 23 '18 at 21:37
Yea, that would work, but I'm being pedantic about performance because there is a good likelihood that thousands of events are going to be triggered per tick, which will probably 90 ticks per second+ as I am working on a VR game. It'd be cool if there was a type-system equivalent of what std::optional does for values.
– ai221
Nov 24 '18 at 0:04
add a comment |
2
It's like that becausevoid
is not a valid parameter type.
– Peter Ruderman
Nov 23 '18 at 19:50
Sounds like you needstd::optional
or a pointer for your additional data structure.
– Jon Harper
Nov 23 '18 at 21:37
Yea, that would work, but I'm being pedantic about performance because there is a good likelihood that thousands of events are going to be triggered per tick, which will probably 90 ticks per second+ as I am working on a VR game. It'd be cool if there was a type-system equivalent of what std::optional does for values.
– ai221
Nov 24 '18 at 0:04
2
2
It's like that because
void
is not a valid parameter type.– Peter Ruderman
Nov 23 '18 at 19:50
It's like that because
void
is not a valid parameter type.– Peter Ruderman
Nov 23 '18 at 19:50
Sounds like you need
std::optional
or a pointer for your additional data structure.– Jon Harper
Nov 23 '18 at 21:37
Sounds like you need
std::optional
or a pointer for your additional data structure.– Jon Harper
Nov 23 '18 at 21:37
Yea, that would work, but I'm being pedantic about performance because there is a good likelihood that thousands of events are going to be triggered per tick, which will probably 90 ticks per second+ as I am working on a VR game. It'd be cool if there was a type-system equivalent of what std::optional does for values.
– ai221
Nov 24 '18 at 0:04
Yea, that would work, but I'm being pedantic about performance because there is a good likelihood that thousands of events are going to be triggered per tick, which will probably 90 ticks per second+ as I am working on a VR game. It'd be cool if there was a type-system equivalent of what std::optional does for values.
– ai221
Nov 24 '18 at 0:04
add a comment |
6 Answers
6
active
oldest
votes
There is a proposal to add "regular void" to the language.
In it, void becomes a monostate type that all other types implicitly convert to.
You can emulate this with
struct monostate_t {
template<class T>
monostate_t(T&&) {}
};
then
using callback_with_just_bool = some_callback<monostate_t>;
auto my_callback_just_bool = callback_with_just_bool((bool x, auto&&...)
{
});
works roughly as you like.
Thanks! This will work much better than template specialization since the class I want to use this in is quite complicated.
– ai221
Nov 25 '18 at 5:43
add a comment |
"Why is this?"
Because the only permitted usage of void
in a parameter list is to show that the function doesn't accept any parameters.
From [function]:
void
Indicates that the function takes no parameters, it is the exact synonym for an empty parameter list:
int f(void);
andint f();
declare the same function. Note that the type void (possibly cv-qualified) cannot be used in a parameter list otherwise:int f(void, int);
andint f(const void);
are errors (although derived types, such asvoid*
can be used)
"Is there a clean way around it?"
I would suggest to specialize for void
:
template<class type_t>
class some_callback
{
std::function<void(bool,type_t)> myfunc;
};
template<>
class some_callback<void>
{
std::function<void(bool)> myfunc;
};
Fair enough, but the rules seem to decrease the generality of the C++ language IMHO. I don't know why they would make void(void) be valid but void(int, void) be invalid.
– ai221
Nov 23 '18 at 20:10
add a comment |
Variadic templates are one way to do this:
template <class... T>
struct some_callback
{
std::function<void(bool, T...)> myfunc;
};
using callback_with_just_bool = some_callback<>;
using callback_with_an_int_too = some_callback<int>;
This is even cleaner with an alias template:
template <class... T> using callback_type = std::function<void(bool, T...)>;
int main()
{
callback_type<int> my_callback_with_int = (bool x, int y)
{
}; //OK
callback_type<> my_callback_just_bool = (bool x)
{
}; //OK now too...
}
add a comment |
You might create specialization:
template<class type_t>
class some_callback
{
std::function<void(bool,type_t)> myfunc;
};
template<>
class some_callback<void>
{
std::function<void(bool)> myfunc;
};
add a comment |
The problem is not the code you showed, it is because in C/C++, you cannot define a function, f, as
void f(bool b, void v) {}
The reason is that, as @Peter Ruderman said, void
is not a valid parameter type.
add a comment |
Other answers have explained the why. This answers "is there a clean way around it?"
What I usually do for callbacks is use lambdas.
std::function<void(void)> myfunc;
bool b = false;
int i = 42;
myfunc = [&]() { if (b) ++i; };
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53452226%2fc-why-is-stdfunctionsome-type-t-void-invalid%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
6 Answers
6
active
oldest
votes
6 Answers
6
active
oldest
votes
active
oldest
votes
active
oldest
votes
There is a proposal to add "regular void" to the language.
In it, void becomes a monostate type that all other types implicitly convert to.
You can emulate this with
struct monostate_t {
template<class T>
monostate_t(T&&) {}
};
then
using callback_with_just_bool = some_callback<monostate_t>;
auto my_callback_just_bool = callback_with_just_bool((bool x, auto&&...)
{
});
works roughly as you like.
Thanks! This will work much better than template specialization since the class I want to use this in is quite complicated.
– ai221
Nov 25 '18 at 5:43
add a comment |
There is a proposal to add "regular void" to the language.
In it, void becomes a monostate type that all other types implicitly convert to.
You can emulate this with
struct monostate_t {
template<class T>
monostate_t(T&&) {}
};
then
using callback_with_just_bool = some_callback<monostate_t>;
auto my_callback_just_bool = callback_with_just_bool((bool x, auto&&...)
{
});
works roughly as you like.
Thanks! This will work much better than template specialization since the class I want to use this in is quite complicated.
– ai221
Nov 25 '18 at 5:43
add a comment |
There is a proposal to add "regular void" to the language.
In it, void becomes a monostate type that all other types implicitly convert to.
You can emulate this with
struct monostate_t {
template<class T>
monostate_t(T&&) {}
};
then
using callback_with_just_bool = some_callback<monostate_t>;
auto my_callback_just_bool = callback_with_just_bool((bool x, auto&&...)
{
});
works roughly as you like.
There is a proposal to add "regular void" to the language.
In it, void becomes a monostate type that all other types implicitly convert to.
You can emulate this with
struct monostate_t {
template<class T>
monostate_t(T&&) {}
};
then
using callback_with_just_bool = some_callback<monostate_t>;
auto my_callback_just_bool = callback_with_just_bool((bool x, auto&&...)
{
});
works roughly as you like.
answered Nov 24 '18 at 16:21
Yakk - Adam NevraumontYakk - Adam Nevraumont
186k19195379
186k19195379
Thanks! This will work much better than template specialization since the class I want to use this in is quite complicated.
– ai221
Nov 25 '18 at 5:43
add a comment |
Thanks! This will work much better than template specialization since the class I want to use this in is quite complicated.
– ai221
Nov 25 '18 at 5:43
Thanks! This will work much better than template specialization since the class I want to use this in is quite complicated.
– ai221
Nov 25 '18 at 5:43
Thanks! This will work much better than template specialization since the class I want to use this in is quite complicated.
– ai221
Nov 25 '18 at 5:43
add a comment |
"Why is this?"
Because the only permitted usage of void
in a parameter list is to show that the function doesn't accept any parameters.
From [function]:
void
Indicates that the function takes no parameters, it is the exact synonym for an empty parameter list:
int f(void);
andint f();
declare the same function. Note that the type void (possibly cv-qualified) cannot be used in a parameter list otherwise:int f(void, int);
andint f(const void);
are errors (although derived types, such asvoid*
can be used)
"Is there a clean way around it?"
I would suggest to specialize for void
:
template<class type_t>
class some_callback
{
std::function<void(bool,type_t)> myfunc;
};
template<>
class some_callback<void>
{
std::function<void(bool)> myfunc;
};
Fair enough, but the rules seem to decrease the generality of the C++ language IMHO. I don't know why they would make void(void) be valid but void(int, void) be invalid.
– ai221
Nov 23 '18 at 20:10
add a comment |
"Why is this?"
Because the only permitted usage of void
in a parameter list is to show that the function doesn't accept any parameters.
From [function]:
void
Indicates that the function takes no parameters, it is the exact synonym for an empty parameter list:
int f(void);
andint f();
declare the same function. Note that the type void (possibly cv-qualified) cannot be used in a parameter list otherwise:int f(void, int);
andint f(const void);
are errors (although derived types, such asvoid*
can be used)
"Is there a clean way around it?"
I would suggest to specialize for void
:
template<class type_t>
class some_callback
{
std::function<void(bool,type_t)> myfunc;
};
template<>
class some_callback<void>
{
std::function<void(bool)> myfunc;
};
Fair enough, but the rules seem to decrease the generality of the C++ language IMHO. I don't know why they would make void(void) be valid but void(int, void) be invalid.
– ai221
Nov 23 '18 at 20:10
add a comment |
"Why is this?"
Because the only permitted usage of void
in a parameter list is to show that the function doesn't accept any parameters.
From [function]:
void
Indicates that the function takes no parameters, it is the exact synonym for an empty parameter list:
int f(void);
andint f();
declare the same function. Note that the type void (possibly cv-qualified) cannot be used in a parameter list otherwise:int f(void, int);
andint f(const void);
are errors (although derived types, such asvoid*
can be used)
"Is there a clean way around it?"
I would suggest to specialize for void
:
template<class type_t>
class some_callback
{
std::function<void(bool,type_t)> myfunc;
};
template<>
class some_callback<void>
{
std::function<void(bool)> myfunc;
};
"Why is this?"
Because the only permitted usage of void
in a parameter list is to show that the function doesn't accept any parameters.
From [function]:
void
Indicates that the function takes no parameters, it is the exact synonym for an empty parameter list:
int f(void);
andint f();
declare the same function. Note that the type void (possibly cv-qualified) cannot be used in a parameter list otherwise:int f(void, int);
andint f(const void);
are errors (although derived types, such asvoid*
can be used)
"Is there a clean way around it?"
I would suggest to specialize for void
:
template<class type_t>
class some_callback
{
std::function<void(bool,type_t)> myfunc;
};
template<>
class some_callback<void>
{
std::function<void(bool)> myfunc;
};
edited Nov 23 '18 at 19:59
answered Nov 23 '18 at 19:53


Edgar RokjānEdgar Rokjān
15.2k42952
15.2k42952
Fair enough, but the rules seem to decrease the generality of the C++ language IMHO. I don't know why they would make void(void) be valid but void(int, void) be invalid.
– ai221
Nov 23 '18 at 20:10
add a comment |
Fair enough, but the rules seem to decrease the generality of the C++ language IMHO. I don't know why they would make void(void) be valid but void(int, void) be invalid.
– ai221
Nov 23 '18 at 20:10
Fair enough, but the rules seem to decrease the generality of the C++ language IMHO. I don't know why they would make void(void) be valid but void(int, void) be invalid.
– ai221
Nov 23 '18 at 20:10
Fair enough, but the rules seem to decrease the generality of the C++ language IMHO. I don't know why they would make void(void) be valid but void(int, void) be invalid.
– ai221
Nov 23 '18 at 20:10
add a comment |
Variadic templates are one way to do this:
template <class... T>
struct some_callback
{
std::function<void(bool, T...)> myfunc;
};
using callback_with_just_bool = some_callback<>;
using callback_with_an_int_too = some_callback<int>;
This is even cleaner with an alias template:
template <class... T> using callback_type = std::function<void(bool, T...)>;
int main()
{
callback_type<int> my_callback_with_int = (bool x, int y)
{
}; //OK
callback_type<> my_callback_just_bool = (bool x)
{
}; //OK now too...
}
add a comment |
Variadic templates are one way to do this:
template <class... T>
struct some_callback
{
std::function<void(bool, T...)> myfunc;
};
using callback_with_just_bool = some_callback<>;
using callback_with_an_int_too = some_callback<int>;
This is even cleaner with an alias template:
template <class... T> using callback_type = std::function<void(bool, T...)>;
int main()
{
callback_type<int> my_callback_with_int = (bool x, int y)
{
}; //OK
callback_type<> my_callback_just_bool = (bool x)
{
}; //OK now too...
}
add a comment |
Variadic templates are one way to do this:
template <class... T>
struct some_callback
{
std::function<void(bool, T...)> myfunc;
};
using callback_with_just_bool = some_callback<>;
using callback_with_an_int_too = some_callback<int>;
This is even cleaner with an alias template:
template <class... T> using callback_type = std::function<void(bool, T...)>;
int main()
{
callback_type<int> my_callback_with_int = (bool x, int y)
{
}; //OK
callback_type<> my_callback_just_bool = (bool x)
{
}; //OK now too...
}
Variadic templates are one way to do this:
template <class... T>
struct some_callback
{
std::function<void(bool, T...)> myfunc;
};
using callback_with_just_bool = some_callback<>;
using callback_with_an_int_too = some_callback<int>;
This is even cleaner with an alias template:
template <class... T> using callback_type = std::function<void(bool, T...)>;
int main()
{
callback_type<int> my_callback_with_int = (bool x, int y)
{
}; //OK
callback_type<> my_callback_just_bool = (bool x)
{
}; //OK now too...
}
edited Nov 23 '18 at 19:59
answered Nov 23 '18 at 19:53


Peter RudermanPeter Ruderman
10.2k2352
10.2k2352
add a comment |
add a comment |
You might create specialization:
template<class type_t>
class some_callback
{
std::function<void(bool,type_t)> myfunc;
};
template<>
class some_callback<void>
{
std::function<void(bool)> myfunc;
};
add a comment |
You might create specialization:
template<class type_t>
class some_callback
{
std::function<void(bool,type_t)> myfunc;
};
template<>
class some_callback<void>
{
std::function<void(bool)> myfunc;
};
add a comment |
You might create specialization:
template<class type_t>
class some_callback
{
std::function<void(bool,type_t)> myfunc;
};
template<>
class some_callback<void>
{
std::function<void(bool)> myfunc;
};
You might create specialization:
template<class type_t>
class some_callback
{
std::function<void(bool,type_t)> myfunc;
};
template<>
class some_callback<void>
{
std::function<void(bool)> myfunc;
};
answered Nov 23 '18 at 19:52
Jarod42Jarod42
117k12103185
117k12103185
add a comment |
add a comment |
The problem is not the code you showed, it is because in C/C++, you cannot define a function, f, as
void f(bool b, void v) {}
The reason is that, as @Peter Ruderman said, void
is not a valid parameter type.
add a comment |
The problem is not the code you showed, it is because in C/C++, you cannot define a function, f, as
void f(bool b, void v) {}
The reason is that, as @Peter Ruderman said, void
is not a valid parameter type.
add a comment |
The problem is not the code you showed, it is because in C/C++, you cannot define a function, f, as
void f(bool b, void v) {}
The reason is that, as @Peter Ruderman said, void
is not a valid parameter type.
The problem is not the code you showed, it is because in C/C++, you cannot define a function, f, as
void f(bool b, void v) {}
The reason is that, as @Peter Ruderman said, void
is not a valid parameter type.
answered Nov 23 '18 at 19:52
CS PeiCS Pei
8,4082039
8,4082039
add a comment |
add a comment |
Other answers have explained the why. This answers "is there a clean way around it?"
What I usually do for callbacks is use lambdas.
std::function<void(void)> myfunc;
bool b = false;
int i = 42;
myfunc = [&]() { if (b) ++i; };
add a comment |
Other answers have explained the why. This answers "is there a clean way around it?"
What I usually do for callbacks is use lambdas.
std::function<void(void)> myfunc;
bool b = false;
int i = 42;
myfunc = [&]() { if (b) ++i; };
add a comment |
Other answers have explained the why. This answers "is there a clean way around it?"
What I usually do for callbacks is use lambdas.
std::function<void(void)> myfunc;
bool b = false;
int i = 42;
myfunc = [&]() { if (b) ++i; };
Other answers have explained the why. This answers "is there a clean way around it?"
What I usually do for callbacks is use lambdas.
std::function<void(void)> myfunc;
bool b = false;
int i = 42;
myfunc = [&]() { if (b) ++i; };
answered Nov 23 '18 at 20:48


Michael SuretteMichael Surette
484110
484110
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53452226%2fc-why-is-stdfunctionsome-type-t-void-invalid%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
5CCG4lwhEKHzeOw HEKmJC Rt1b
2
It's like that because
void
is not a valid parameter type.– Peter Ruderman
Nov 23 '18 at 19:50
Sounds like you need
std::optional
or a pointer for your additional data structure.– Jon Harper
Nov 23 '18 at 21:37
Yea, that would work, but I'm being pedantic about performance because there is a good likelihood that thousands of events are going to be triggered per tick, which will probably 90 ticks per second+ as I am working on a VR game. It'd be cool if there was a type-system equivalent of what std::optional does for values.
– ai221
Nov 24 '18 at 0:04