Python - Changing the format of the bottom values to show the month
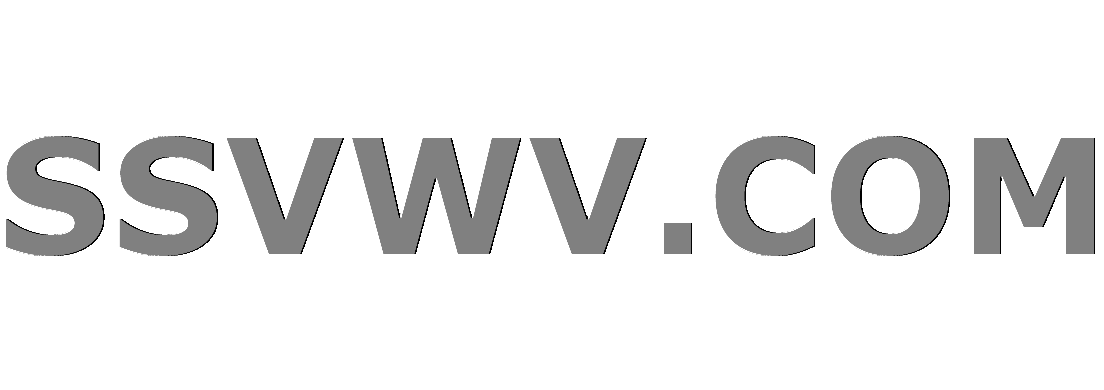
Multi tool use
New to python and pandas, I got my function working correctly by looking for existing code however I want to change the bottom values from numbers to actual texts, which in this case would be months.
from sklearn.cluster import AgglomerativeClustering as AC
def scale_df(df,axis=0):
return (df - df.mean(axis=axis))/df.std(axis=axis)
colormap = sns.diverging_palette(220, 10, as_cmap=True)
def plot_hmap(df, ix=None, cmap=colormap):
if ix is None:
ix = np.arange(df.shape[0])
plt.imshow(df.iloc[ix,:], cmap=cmap)
plt.colorbar(fraction=0.03)
plt.yticks(np.arange(df.shape[0]), df.index[ix])
plt.xticks(np.arange(df.shape[1]))
plt.grid(False)
plt.show()
def scale_and_plot(df, ix=None):
df_marginal_scaled = scale_df(df.T).T
if ix is None:
ix = AC(4).fit(df_marginal_scaled).labels_.argsort() # a trick to make better heatmaps
cap = np.min([np.max(df_marginal_scaled.values), np.abs(np.min(df_marginal_scaled.values))])
df_marginal_scaled = np.clip(df_marginal_scaled, -1*cap, cap)
plot_hmap(df_marginal_scaled, ix=ix)
month_by_type = crimeDataMonth.pivot_table(values='Month', index='Crime type', columns=crimeDataMonth.index.month, aggfunc=np.size).fillna(0)
plt.figure(figsize=(15,12))
scale_and_plot(month_by_type)
My table is the following which has the month:
python pandas matplotlib seaborn
add a comment |
New to python and pandas, I got my function working correctly by looking for existing code however I want to change the bottom values from numbers to actual texts, which in this case would be months.
from sklearn.cluster import AgglomerativeClustering as AC
def scale_df(df,axis=0):
return (df - df.mean(axis=axis))/df.std(axis=axis)
colormap = sns.diverging_palette(220, 10, as_cmap=True)
def plot_hmap(df, ix=None, cmap=colormap):
if ix is None:
ix = np.arange(df.shape[0])
plt.imshow(df.iloc[ix,:], cmap=cmap)
plt.colorbar(fraction=0.03)
plt.yticks(np.arange(df.shape[0]), df.index[ix])
plt.xticks(np.arange(df.shape[1]))
plt.grid(False)
plt.show()
def scale_and_plot(df, ix=None):
df_marginal_scaled = scale_df(df.T).T
if ix is None:
ix = AC(4).fit(df_marginal_scaled).labels_.argsort() # a trick to make better heatmaps
cap = np.min([np.max(df_marginal_scaled.values), np.abs(np.min(df_marginal_scaled.values))])
df_marginal_scaled = np.clip(df_marginal_scaled, -1*cap, cap)
plot_hmap(df_marginal_scaled, ix=ix)
month_by_type = crimeDataMonth.pivot_table(values='Month', index='Crime type', columns=crimeDataMonth.index.month, aggfunc=np.size).fillna(0)
plt.figure(figsize=(15,12))
scale_and_plot(month_by_type)
My table is the following which has the month:
python pandas matplotlib seaborn
Can you share a sample ofmonth_by_type
instead?
– yatu
Nov 23 '18 at 20:37
add a comment |
New to python and pandas, I got my function working correctly by looking for existing code however I want to change the bottom values from numbers to actual texts, which in this case would be months.
from sklearn.cluster import AgglomerativeClustering as AC
def scale_df(df,axis=0):
return (df - df.mean(axis=axis))/df.std(axis=axis)
colormap = sns.diverging_palette(220, 10, as_cmap=True)
def plot_hmap(df, ix=None, cmap=colormap):
if ix is None:
ix = np.arange(df.shape[0])
plt.imshow(df.iloc[ix,:], cmap=cmap)
plt.colorbar(fraction=0.03)
plt.yticks(np.arange(df.shape[0]), df.index[ix])
plt.xticks(np.arange(df.shape[1]))
plt.grid(False)
plt.show()
def scale_and_plot(df, ix=None):
df_marginal_scaled = scale_df(df.T).T
if ix is None:
ix = AC(4).fit(df_marginal_scaled).labels_.argsort() # a trick to make better heatmaps
cap = np.min([np.max(df_marginal_scaled.values), np.abs(np.min(df_marginal_scaled.values))])
df_marginal_scaled = np.clip(df_marginal_scaled, -1*cap, cap)
plot_hmap(df_marginal_scaled, ix=ix)
month_by_type = crimeDataMonth.pivot_table(values='Month', index='Crime type', columns=crimeDataMonth.index.month, aggfunc=np.size).fillna(0)
plt.figure(figsize=(15,12))
scale_and_plot(month_by_type)
My table is the following which has the month:
python pandas matplotlib seaborn
New to python and pandas, I got my function working correctly by looking for existing code however I want to change the bottom values from numbers to actual texts, which in this case would be months.
from sklearn.cluster import AgglomerativeClustering as AC
def scale_df(df,axis=0):
return (df - df.mean(axis=axis))/df.std(axis=axis)
colormap = sns.diverging_palette(220, 10, as_cmap=True)
def plot_hmap(df, ix=None, cmap=colormap):
if ix is None:
ix = np.arange(df.shape[0])
plt.imshow(df.iloc[ix,:], cmap=cmap)
plt.colorbar(fraction=0.03)
plt.yticks(np.arange(df.shape[0]), df.index[ix])
plt.xticks(np.arange(df.shape[1]))
plt.grid(False)
plt.show()
def scale_and_plot(df, ix=None):
df_marginal_scaled = scale_df(df.T).T
if ix is None:
ix = AC(4).fit(df_marginal_scaled).labels_.argsort() # a trick to make better heatmaps
cap = np.min([np.max(df_marginal_scaled.values), np.abs(np.min(df_marginal_scaled.values))])
df_marginal_scaled = np.clip(df_marginal_scaled, -1*cap, cap)
plot_hmap(df_marginal_scaled, ix=ix)
month_by_type = crimeDataMonth.pivot_table(values='Month', index='Crime type', columns=crimeDataMonth.index.month, aggfunc=np.size).fillna(0)
plt.figure(figsize=(15,12))
scale_and_plot(month_by_type)
My table is the following which has the month:
python pandas matplotlib seaborn
python pandas matplotlib seaborn
edited Nov 23 '18 at 20:07


Abhi
2,490320
2,490320
asked Nov 23 '18 at 19:50


Stuart AllenStuart Allen
132
132
Can you share a sample ofmonth_by_type
instead?
– yatu
Nov 23 '18 at 20:37
add a comment |
Can you share a sample ofmonth_by_type
instead?
– yatu
Nov 23 '18 at 20:37
Can you share a sample of
month_by_type
instead?– yatu
Nov 23 '18 at 20:37
Can you share a sample of
month_by_type
instead?– yatu
Nov 23 '18 at 20:37
add a comment |
1 Answer
1
active
oldest
votes
If i understand correctly, you would like to change the labels in the x-axis of the plot from the numbers [0, 1, ... 11]
to the names of the corresponding months ['January', 'February', ... 'December'].
If so, you could do that using this:
# Get the current locations of the labels
locations, labels = plt.xticks()
# Set the values of the new labels
months = ['January', 'February', 'March', 'April', 'May', 'June', 'July',
'August', 'September', 'October', 'November', 'December']
# Place the new labels
plt.xticks(locations, labels=months)
You could do this with less hard-coded values using the calendar
module:
import calendar
locations, labels = plt.xticks()
months = [calendar.month_name[n] for n in range(1, 13, 1)]
plt.xticks(locations, labels=months)
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53452263%2fpython-changing-the-format-of-the-bottom-values-to-show-the-month%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
If i understand correctly, you would like to change the labels in the x-axis of the plot from the numbers [0, 1, ... 11]
to the names of the corresponding months ['January', 'February', ... 'December'].
If so, you could do that using this:
# Get the current locations of the labels
locations, labels = plt.xticks()
# Set the values of the new labels
months = ['January', 'February', 'March', 'April', 'May', 'June', 'July',
'August', 'September', 'October', 'November', 'December']
# Place the new labels
plt.xticks(locations, labels=months)
You could do this with less hard-coded values using the calendar
module:
import calendar
locations, labels = plt.xticks()
months = [calendar.month_name[n] for n in range(1, 13, 1)]
plt.xticks(locations, labels=months)
add a comment |
If i understand correctly, you would like to change the labels in the x-axis of the plot from the numbers [0, 1, ... 11]
to the names of the corresponding months ['January', 'February', ... 'December'].
If so, you could do that using this:
# Get the current locations of the labels
locations, labels = plt.xticks()
# Set the values of the new labels
months = ['January', 'February', 'March', 'April', 'May', 'June', 'July',
'August', 'September', 'October', 'November', 'December']
# Place the new labels
plt.xticks(locations, labels=months)
You could do this with less hard-coded values using the calendar
module:
import calendar
locations, labels = plt.xticks()
months = [calendar.month_name[n] for n in range(1, 13, 1)]
plt.xticks(locations, labels=months)
add a comment |
If i understand correctly, you would like to change the labels in the x-axis of the plot from the numbers [0, 1, ... 11]
to the names of the corresponding months ['January', 'February', ... 'December'].
If so, you could do that using this:
# Get the current locations of the labels
locations, labels = plt.xticks()
# Set the values of the new labels
months = ['January', 'February', 'March', 'April', 'May', 'June', 'July',
'August', 'September', 'October', 'November', 'December']
# Place the new labels
plt.xticks(locations, labels=months)
You could do this with less hard-coded values using the calendar
module:
import calendar
locations, labels = plt.xticks()
months = [calendar.month_name[n] for n in range(1, 13, 1)]
plt.xticks(locations, labels=months)
If i understand correctly, you would like to change the labels in the x-axis of the plot from the numbers [0, 1, ... 11]
to the names of the corresponding months ['January', 'February', ... 'December'].
If so, you could do that using this:
# Get the current locations of the labels
locations, labels = plt.xticks()
# Set the values of the new labels
months = ['January', 'February', 'March', 'April', 'May', 'June', 'July',
'August', 'September', 'October', 'November', 'December']
# Place the new labels
plt.xticks(locations, labels=months)
You could do this with less hard-coded values using the calendar
module:
import calendar
locations, labels = plt.xticks()
months = [calendar.month_name[n] for n in range(1, 13, 1)]
plt.xticks(locations, labels=months)
edited Nov 23 '18 at 21:55
answered Nov 23 '18 at 21:44


Gal AvineriGal Avineri
16210
16210
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53452263%2fpython-changing-the-format-of-the-bottom-values-to-show-the-month%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
MOWWa5xWzN81nQkqBWybLpYWJhrtP3214O1iUGNb2cPQkHKVxDwCKCfCytg1FM0545,TE
Can you share a sample of
month_by_type
instead?– yatu
Nov 23 '18 at 20:37